Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / TextFormatting / FormatSettings.cs / 1305600 / FormatSettings.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: FormatSettings.cs // // Contents: Settings to format text // // Created: 2-25-2004 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Diagnostics; using System.Security; using System.Windows; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Media.TextFormatting; namespace MS.Internal.TextFormatting { ////// Text formatting state /// internal sealed class FormatSettings { private TextFormatterImp _formatter; // formatter object private TextSource _textSource; // client text source for main text private TextRunCacheImp _runCache; // run cache owned by client private ParaProp _pap; // current paragraph properties private DigitState _digitState; // current number substitution properties private TextLineBreak _previousLineBreak; // break of previous line private int _maxLineWidth; // Max line width of the line being built. Only used in Optimal paragraph for the moment private int _textIndent; // Distance from line start to where text starts private TextFormattingMode _textFormattingMode; private bool _isSideways; internal FormatSettings( TextFormatterImp formatter, TextSource textSource, TextRunCacheImp runCache, ParaProp pap, TextLineBreak previousLineBreak, bool isSingleLineFormatting, TextFormattingMode textFormattingMode, bool isSideways ) { _isSideways = isSideways; _textFormattingMode = textFormattingMode; _formatter = formatter; _textSource = textSource; _runCache = runCache; _pap = pap; _digitState = new DigitState(); _previousLineBreak = previousLineBreak; _maxLineWidth = Constants.IdealInfiniteWidth; if (isSingleLineFormatting) { // Apply text indent on each line in single line mode _textIndent = _pap.Indent; } } internal TextFormattingMode TextFormattingMode { get { return _textFormattingMode; } } internal bool IsSideways { get { return _isSideways; } } ////// Current formatter /// internal TextFormatterImp Formatter { get { return _formatter; } } ////// Current source /// internal TextSource TextSource { get { return _textSource; } } ////// Break of previous line /// internal TextLineBreak PreviousLineBreak { get { return _previousLineBreak; } } ////// paragraph properties /// internal ParaProp Pap { get { return _pap; } } ////// Max width of the line being built. In optimal break mode, client may format /// breakpoints for the line with max width different from the paragraph width. /// internal int MaxLineWidth { get { return _maxLineWidth; } } ////// Update formatting parameters at line start /// internal void UpdateSettingsForCurrentLine( int maxLineWidth, TextLineBreak previousLineBreak, bool isFirstLineInPara ) { _previousLineBreak = previousLineBreak; _digitState = new DigitState(); // reset digit state _maxLineWidth = Math.Max(maxLineWidth, 0); if (isFirstLineInPara) { _textIndent = _pap.Indent; } else { // Do not apply text indentation to all but the first line in para _textIndent = 0; } } ////// Calculate formatting width from specified constraint width /// internal int GetFormatWidth(int finiteFormatWidth) { // LS does not support no wrap, for such case, we would need to // simulate formatting a line within an infinite paragraph width // while still keeping the last legit boundary for trimming purpose. return _pap.Wrap ? finiteFormatWidth : Constants.IdealInfiniteWidth; } ////// Calculate finite formatting width from specified constraint width /// internal int GetFiniteFormatWidth(int paragraphWidth) { // indent is part of our text line but not of LS line // paragraph width == 0 means format width is unlimited int formatWidth = (paragraphWidth <= 0 ? Constants.IdealInfiniteWidth : paragraphWidth); formatWidth = formatWidth - _pap.ParagraphIndent; // sanitize the format width value before passing to LS formatWidth = Math.Max(formatWidth, 0); formatWidth = Math.Min(formatWidth, Constants.IdealInfiniteWidth); return formatWidth; } ////// Fetch text run and character string associated with it /// ////// Critical: This code has unsafe code block that uses pointers. /// TreatAsSafe: This code does not expose the pointers. /// [SecurityCritical, SecurityTreatAsSafe] internal CharacterBufferRange FetchTextRun( int cpFetch, int cpFirst, out TextRun textRun, out int runLength ) { int offsetToFirstCp; textRun = _runCache.FetchTextRun(this, cpFetch, cpFirst, out offsetToFirstCp, out runLength); CharacterBufferRange charString; switch (TextRunInfo.GetRunType(textRun)) { case Plsrun.Text: { CharacterBufferReference charBufferRef = textRun.CharacterBufferReference; charString = new CharacterBufferRange( charBufferRef.CharacterBuffer, charBufferRef.OffsetToFirstChar + offsetToFirstCp, runLength ); break; } case Plsrun.InlineObject: Debug.Assert(offsetToFirstCp == 0); unsafe { charString = new CharacterBufferRange((char*) TextStore.PwchObjectReplacement, 1); } break; case Plsrun.LineBreak: Debug.Assert(offsetToFirstCp == 0); unsafe { // // Line break is to be represented as "Line Separator" such that // it doesn't terminate the optimal paragraph formatting session, but still breaks the line // unambiguously. // charString = new CharacterBufferRange((char*) TextStore.PwchLineSeparator, 1); } break; case Plsrun.ParaBreak: Debug.Assert(offsetToFirstCp == 0); unsafe { // // Paragraph break is special as it also terminates the paragraph. // It should be represented as "Paragraph Separator" such that it will be correctly handled // in Bidi and Optimal paragraph formatting. // charString = new CharacterBufferRange((char*) TextStore.PwchParaSeparator, 1); } break; case Plsrun.Hidden: unsafe { charString = new CharacterBufferRange((char*) TextStore.PwchHidden, 1); } break; default: charString = CharacterBufferRange.Empty; break; } return charString; } ////// Get text immediately preceding cpLimit. /// internal TextSpanGetPrecedingText(int cpLimit) { return _runCache.GetPrecedingText(_textSource, cpLimit); } /// /// Current number substitution propeties /// internal DigitState DigitState { get { return _digitState; } } ////// Distance from line start to where text starts /// internal int TextIndent { get { return _textIndent; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
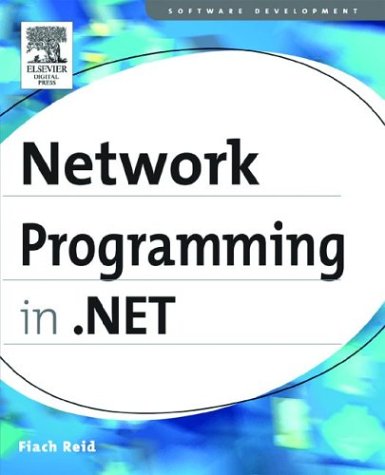
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EvidenceTypeDescriptor.cs
- NamespaceDisplay.xaml.cs
- TextTreeTextElementNode.cs
- WmlValidatorAdapter.cs
- ParameterModifier.cs
- TimeEnumHelper.cs
- VersionValidator.cs
- ObjectRef.cs
- BeginEvent.cs
- ConfigurationFileMap.cs
- DbException.cs
- PropertyDescriptor.cs
- FileChangeNotifier.cs
- PersonalizationProvider.cs
- FixedSOMLineCollection.cs
- RelationshipManager.cs
- DecoderFallbackWithFailureFlag.cs
- CngProvider.cs
- XhtmlBasicControlAdapter.cs
- SelectionPatternIdentifiers.cs
- ExceptionUtil.cs
- HtmlTableRowCollection.cs
- WebPartManagerDesigner.cs
- PropertyGroupDescription.cs
- _ListenerResponseStream.cs
- _TransmitFileOverlappedAsyncResult.cs
- XamlDesignerSerializationManager.cs
- Int64AnimationBase.cs
- ParsedRoute.cs
- SchemaImporter.cs
- ParameterToken.cs
- DataGridLinkButton.cs
- EventSinkActivityDesigner.cs
- SchemaRegistration.cs
- SqlConnectionHelper.cs
- ObjectTypeMapping.cs
- Point3DAnimationUsingKeyFrames.cs
- PostBackTrigger.cs
- ConfigXmlCDataSection.cs
- StylusSystemGestureEventArgs.cs
- HttpConfigurationSystem.cs
- GeneralTransform.cs
- BadImageFormatException.cs
- Hex.cs
- PropertyStore.cs
- Point3DCollection.cs
- BitFlagsGenerator.cs
- ProfileManager.cs
- TaskDesigner.cs
- ExpressionBindingCollection.cs
- SiteMapNodeItem.cs
- ThumbAutomationPeer.cs
- XPathDocumentBuilder.cs
- DateTimeAutomationPeer.cs
- AddInControllerImpl.cs
- Model3D.cs
- TextRenderer.cs
- ToolStripOverflow.cs
- ViewStateException.cs
- SimpleApplicationHost.cs
- ValueType.cs
- EvidenceBase.cs
- SystemWebExtensionsSectionGroup.cs
- AsymmetricKeyExchangeFormatter.cs
- XmlDataDocument.cs
- XmlEncoding.cs
- SecurityStandardsManager.cs
- CompositeCollection.cs
- _NetRes.cs
- CodeAttributeDeclaration.cs
- NamespaceList.cs
- TextReader.cs
- XmlMessageFormatter.cs
- Int16Converter.cs
- XmlNotation.cs
- SHA512Cng.cs
- Encoding.cs
- DataGridViewCellStyleConverter.cs
- TableCellCollection.cs
- CssClassPropertyAttribute.cs
- _DisconnectOverlappedAsyncResult.cs
- HttpRequest.cs
- XmlArrayAttribute.cs
- Variable.cs
- ChannelManager.cs
- BlurEffect.cs
- ReturnValue.cs
- CapabilitiesAssignment.cs
- keycontainerpermission.cs
- ContentControl.cs
- CmsUtils.cs
- UnsafeNativeMethods.cs
- HttpFileCollection.cs
- CompoundFileStorageReference.cs
- TextEditorMouse.cs
- OleDbPermission.cs
- SizeAnimationBase.cs
- SqlWebEventProvider.cs
- PagesChangedEventArgs.cs
- _NestedSingleAsyncResult.cs