Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / DataServiceRequestOfT.cs / 1305376 / DataServiceRequestOfT.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// typed request object // //--------------------------------------------------------------------- namespace System.Data.Services.Client { #region Namespaces. using System; using System.Diagnostics; #endregion Namespaces. ////// Holds a Uri and type for the request. /// ///The type to construct for the request results public sealed class DataServiceRequest: DataServiceRequest { #region Private fields. /// The UriTranslateResult for the request private readonly QueryComponents queryComponents; ///The ProjectionPlan for the request (if precompiled in a previous page). private readonly ProjectionPlan plan; #endregion Private fields. #region Constructors. ///Create a request for a specific Uri /// The URI for the request. public DataServiceRequest(Uri requestUri) { Util.CheckArgumentNull(requestUri, "requestUri"); Type elementType = typeof(TElement); elementType = ClientConvert.IsKnownType(elementType) ? elementType : TypeSystem.GetElementType(elementType); this.queryComponents = new QueryComponents(requestUri, Util.DataServiceVersionEmpty, elementType, null, null); } ///Create a request for a specific Uri /// The query components for the request /// Projection plan to reuse (possibly null). internal DataServiceRequest(QueryComponents queryComponents, ProjectionPlan plan) { Debug.Assert(queryComponents != null, "queryComponents != null"); this.queryComponents = queryComponents; this.plan = plan; } #endregion Constructors. ///Element Type public override Type ElementType { get { return typeof(TElement); } } ///The URI for the request. public override Uri RequestUri { get { return this.queryComponents.Uri; } } ///The ProjectionPlan for the request, if precompiled in a previous page; null otherwise. internal override ProjectionPlan Plan { get { return this.plan; } } ///The TranslateResult associated with this request internal override QueryComponents QueryComponents { get { return this.queryComponents; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// typed request object // //--------------------------------------------------------------------- namespace System.Data.Services.Client { #region Namespaces. using System; using System.Diagnostics; #endregion Namespaces. ////// Holds a Uri and type for the request. /// ///The type to construct for the request results public sealed class DataServiceRequest: DataServiceRequest { #region Private fields. /// The UriTranslateResult for the request private readonly QueryComponents queryComponents; ///The ProjectionPlan for the request (if precompiled in a previous page). private readonly ProjectionPlan plan; #endregion Private fields. #region Constructors. ///Create a request for a specific Uri /// The URI for the request. public DataServiceRequest(Uri requestUri) { Util.CheckArgumentNull(requestUri, "requestUri"); Type elementType = typeof(TElement); elementType = ClientConvert.IsKnownType(elementType) ? elementType : TypeSystem.GetElementType(elementType); this.queryComponents = new QueryComponents(requestUri, Util.DataServiceVersionEmpty, elementType, null, null); } ///Create a request for a specific Uri /// The query components for the request /// Projection plan to reuse (possibly null). internal DataServiceRequest(QueryComponents queryComponents, ProjectionPlan plan) { Debug.Assert(queryComponents != null, "queryComponents != null"); this.queryComponents = queryComponents; this.plan = plan; } #endregion Constructors. ///Element Type public override Type ElementType { get { return typeof(TElement); } } ///The URI for the request. public override Uri RequestUri { get { return this.queryComponents.Uri; } } ///The ProjectionPlan for the request, if precompiled in a previous page; null otherwise. internal override ProjectionPlan Plan { get { return this.plan; } } ///The TranslateResult associated with this request internal override QueryComponents QueryComponents { get { return this.queryComponents; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
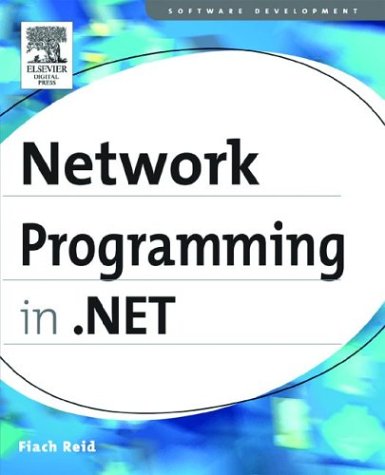
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignerToolboxInfo.cs
- PersonalizationProviderHelper.cs
- LineServicesRun.cs
- RenderTargetBitmap.cs
- TcpAppDomainProtocolHandler.cs
- DataBoundControl.cs
- ExtendedPropertyDescriptor.cs
- SQLByteStorage.cs
- XomlCompiler.cs
- RunWorkerCompletedEventArgs.cs
- IList.cs
- COM2ExtendedTypeConverter.cs
- CoreSwitches.cs
- HandlerBase.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- AutomationPatternInfo.cs
- AssemblyBuilder.cs
- TraceLevelStore.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- SqlProviderServices.cs
- XmlAttributeCache.cs
- XamlTreeBuilderBamlRecordWriter.cs
- ToolStripGrip.cs
- HwndStylusInputProvider.cs
- CacheRequest.cs
- LateBoundBitmapDecoder.cs
- DesignerVerbCollection.cs
- Message.cs
- StylusPointPropertyUnit.cs
- EntityDataSourceValidationException.cs
- HierarchicalDataTemplate.cs
- TabItemWrapperAutomationPeer.cs
- CurrencyManager.cs
- URLMembershipCondition.cs
- XpsDocument.cs
- QilInvokeEarlyBound.cs
- BitmapScalingModeValidation.cs
- LicenseContext.cs
- smtpconnection.cs
- StructuredTypeEmitter.cs
- PagesSection.cs
- XmlArrayItemAttribute.cs
- PassportPrincipal.cs
- DecimalSumAggregationOperator.cs
- CalculatedColumn.cs
- DataSourceCache.cs
- InkCanvasSelection.cs
- CopyAttributesAction.cs
- ProxyHwnd.cs
- GlyphsSerializer.cs
- FamilyMapCollection.cs
- TableCell.cs
- DrawingGroupDrawingContext.cs
- DependencyPropertyKey.cs
- PasswordBox.cs
- QueryResponse.cs
- ColorConvertedBitmap.cs
- SizeConverter.cs
- LineServicesCallbacks.cs
- XslException.cs
- RIPEMD160.cs
- EntityModelSchemaGenerator.cs
- MulticastNotSupportedException.cs
- PackageRelationshipSelector.cs
- ContentPosition.cs
- WindowsHyperlink.cs
- ScaleTransform3D.cs
- IImplicitResourceProvider.cs
- ProxyHwnd.cs
- TextRunProperties.cs
- CodeMethodInvokeExpression.cs
- ProfileManager.cs
- ReservationCollection.cs
- XmlSchemaValidator.cs
- AdPostCacheSubstitution.cs
- FragmentQuery.cs
- MdImport.cs
- DocumentApplication.cs
- ArrayTypeMismatchException.cs
- DependencyProperty.cs
- ListViewItem.cs
- SpeakInfo.cs
- BindStream.cs
- MissingMethodException.cs
- Int16AnimationBase.cs
- ServiceDocument.cs
- TheQuery.cs
- DataExpression.cs
- NullableDecimalSumAggregationOperator.cs
- PolicyStatement.cs
- ToolStripButton.cs
- SimpleTypeResolver.cs
- DocumentPageView.cs
- SqlTypeConverter.cs
- TableItemStyle.cs
- DependencyPropertyHelper.cs
- ValidationErrorEventArgs.cs
- ImageFormatConverter.cs
- TextDecoration.cs
- Token.cs