Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / DbQueryCommandTree.cs / 1305376 / DbQueryCommandTree.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; using System.Linq; using System.Diagnostics; namespace System.Data.Common.CommandTrees { ////// Represents a query operation expressed as a canonical command tree. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbQueryCommandTree : DbCommandTree { // Query expression private readonly DbExpression _query; // Parameter information (will be retrieved from the components of the command tree before construction) private System.Collections.ObjectModel.ReadOnlyCollection_parameters; private DbQueryCommandTree(MetadataWorkspace metadata, DataSpace dataSpace, DbExpression query, bool validate) : base(metadata, dataSpace) { // Ensure the query expression is non-null EntityUtil.CheckArgumentNull(query, "query"); using (new EntityBid.ScopeAuto(" %d#, query=%d#", this.ObjectId, query.ObjectId)) { if (validate) { // Use the valid workspace and data space to validate the query expression DbExpressionValidator validator = new DbExpressionValidator(metadata, dataSpace); validator.ValidateExpression(query, "query"); this._parameters = validator.Parameters.Select(paramInfo => paramInfo.Value).ToList().AsReadOnly(); } this._query = query; } } /// /// Constructs a new DbQueryCommandTree that uses the specified metadata workspace. /// /// The metadata workspace that the command tree should use. /// The logical 'space' that metadata in the expressions used in this command tree must belong to. /// Athat defines the logic of the query. /// /// or is null /*CQT_PUBLIC_API(*/internal/*)*/ DbQueryCommandTree(MetadataWorkspace metadata, DataSpace dataSpace, DbExpression query) : this(metadata, dataSpace, query, true) { } /// does not represent a valid data space /// Gets a public DbExpression Query { get { return this._query; } } internal override DbCommandTreeKind CommandTreeKind { get { return DbCommandTreeKind.Query; } } internal override IEnumerablethat defines the logic of the query. /// > GetParameters() { if (this._parameters == null) { this._parameters = ParameterRetriever.GetParameters(this); } return this._parameters.Select(p => new KeyValuePair (p.ParameterName, p.ResultType)); } internal override void DumpStructure(ExpressionDumper dumper) { if (this.Query != null) { dumper.Dump(this.Query, "Query"); } } internal override string PrintTree(ExpressionPrinter printer) { return printer.Print(this); } internal static DbQueryCommandTree FromValidExpression(MetadataWorkspace metadata, DataSpace dataSpace, DbExpression query) { #if DEBUG return new DbQueryCommandTree(metadata, dataSpace, query); #else return new DbQueryCommandTree(metadata, dataSpace, query, false); #endif } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; using System.Linq; using System.Diagnostics; namespace System.Data.Common.CommandTrees { ////// Represents a query operation expressed as a canonical command tree. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbQueryCommandTree : DbCommandTree { // Query expression private readonly DbExpression _query; // Parameter information (will be retrieved from the components of the command tree before construction) private System.Collections.ObjectModel.ReadOnlyCollection_parameters; private DbQueryCommandTree(MetadataWorkspace metadata, DataSpace dataSpace, DbExpression query, bool validate) : base(metadata, dataSpace) { // Ensure the query expression is non-null EntityUtil.CheckArgumentNull(query, "query"); using (new EntityBid.ScopeAuto(" %d#, query=%d#", this.ObjectId, query.ObjectId)) { if (validate) { // Use the valid workspace and data space to validate the query expression DbExpressionValidator validator = new DbExpressionValidator(metadata, dataSpace); validator.ValidateExpression(query, "query"); this._parameters = validator.Parameters.Select(paramInfo => paramInfo.Value).ToList().AsReadOnly(); } this._query = query; } } /// /// Constructs a new DbQueryCommandTree that uses the specified metadata workspace. /// /// The metadata workspace that the command tree should use. /// The logical 'space' that metadata in the expressions used in this command tree must belong to. /// Athat defines the logic of the query. /// /// or is null /*CQT_PUBLIC_API(*/internal/*)*/ DbQueryCommandTree(MetadataWorkspace metadata, DataSpace dataSpace, DbExpression query) : this(metadata, dataSpace, query, true) { } /// does not represent a valid data space /// Gets a public DbExpression Query { get { return this._query; } } internal override DbCommandTreeKind CommandTreeKind { get { return DbCommandTreeKind.Query; } } internal override IEnumerablethat defines the logic of the query. /// > GetParameters() { if (this._parameters == null) { this._parameters = ParameterRetriever.GetParameters(this); } return this._parameters.Select(p => new KeyValuePair (p.ParameterName, p.ResultType)); } internal override void DumpStructure(ExpressionDumper dumper) { if (this.Query != null) { dumper.Dump(this.Query, "Query"); } } internal override string PrintTree(ExpressionPrinter printer) { return printer.Print(this); } internal static DbQueryCommandTree FromValidExpression(MetadataWorkspace metadata, DataSpace dataSpace, DbExpression query) { #if DEBUG return new DbQueryCommandTree(metadata, dataSpace, query); #else return new DbQueryCommandTree(metadata, dataSpace, query, false); #endif } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
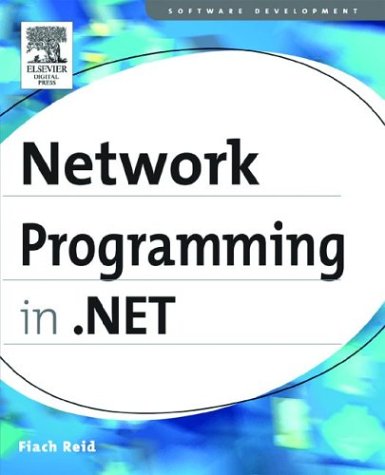
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaResource.cs
- XmlNamespaceManager.cs
- GenerateHelper.cs
- UInt32.cs
- DataGridCheckBoxColumn.cs
- TextPattern.cs
- DesignerAttribute.cs
- BounceEase.cs
- WindowsSlider.cs
- CalendarButton.cs
- RelationshipEnd.cs
- Suspend.cs
- DataGridViewRowsAddedEventArgs.cs
- Parameter.cs
- HostElement.cs
- InstanceCreationEditor.cs
- XPathExpr.cs
- ErrorsHelper.cs
- AnnotationAdorner.cs
- CounterSampleCalculator.cs
- RefType.cs
- EmptyEnumerator.cs
- CharAnimationUsingKeyFrames.cs
- DesigntimeLicenseContext.cs
- WindowsFormsHelpers.cs
- UriExt.cs
- TextRenderingModeValidation.cs
- CodeEventReferenceExpression.cs
- SecurityContextSecurityTokenParameters.cs
- Point.cs
- WebDescriptionAttribute.cs
- TransformerInfo.cs
- MethodAccessException.cs
- BaseUriHelper.cs
- WebPartZone.cs
- NullableFloatSumAggregationOperator.cs
- EntityParameter.cs
- MdImport.cs
- FileEnumerator.cs
- SolidBrush.cs
- HtmlLinkAdapter.cs
- DataBindingCollectionConverter.cs
- DCSafeHandle.cs
- WorkflowStateRollbackService.cs
- SessionEndingEventArgs.cs
- TagPrefixInfo.cs
- StyleXamlTreeBuilder.cs
- IItemContainerGenerator.cs
- InlineUIContainer.cs
- TextProviderWrapper.cs
- HttpCachePolicyElement.cs
- GenericEnumConverter.cs
- ScriptingWebServicesSectionGroup.cs
- SerializationException.cs
- SqlMultiplexer.cs
- TextCollapsingProperties.cs
- TextTrailingWordEllipsis.cs
- SaveWorkflowAsyncResult.cs
- StylusButtonCollection.cs
- LocalizableAttribute.cs
- LambdaCompiler.Address.cs
- HashStream.cs
- ExpressionHelper.cs
- GridEntryCollection.cs
- XPathChildIterator.cs
- RSAPKCS1SignatureFormatter.cs
- DynamicPropertyReader.cs
- XsltLoader.cs
- XmlMemberMapping.cs
- KeyValuePairs.cs
- SessionEndingCancelEventArgs.cs
- WebSysDisplayNameAttribute.cs
- DocumentGridPage.cs
- AssemblyLoader.cs
- ListCollectionView.cs
- XmlNodeList.cs
- PrtTicket_Editor.cs
- RequestCache.cs
- ListViewItemEventArgs.cs
- CodeThrowExceptionStatement.cs
- MessageBox.cs
- RelatedView.cs
- EventListenerClientSide.cs
- XmlText.cs
- ProxyGenerationError.cs
- Documentation.cs
- XmlSchemaComplexContentExtension.cs
- ReadOnlyDataSourceView.cs
- WinEventHandler.cs
- TogglePatternIdentifiers.cs
- DesignTimeSiteMapProvider.cs
- SaveFileDialog.cs
- RequestCache.cs
- DBPropSet.cs
- DataSourceView.cs
- EntityDataSourceEntityTypeFilterItem.cs
- Automation.cs
- BamlCollectionHolder.cs
- Choices.cs
- RadioButton.cs