Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Policy / ZoneMembershipCondition.cs / 1305376 / ZoneMembershipCondition.cs
using System.Diagnostics.Contracts; // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // ZoneMembershipCondition.cs // //[....] // // Implementation of membership condition for zones // namespace System.Security.Policy { using System; using SecurityManager = System.Security.SecurityManager; using PermissionSet = System.Security.PermissionSet; using SecurityElement = System.Security.SecurityElement; using System.Collections; using System.Globalization; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class ZoneMembershipCondition : IMembershipCondition, IConstantMembershipCondition, IReportMatchMembershipCondition { //------------------------------------------------------ // // PRIVATE CONSTANTS // //----------------------------------------------------- private static readonly String[] s_names = {"MyComputer", "Intranet", "Trusted", "Internet", "Untrusted"}; //----------------------------------------------------- // // PRIVATE STATE DATA // //----------------------------------------------------- private SecurityZone m_zone; private SecurityElement m_element; //------------------------------------------------------ // // PUBLIC CONSTRUCTORS // //----------------------------------------------------- internal ZoneMembershipCondition() { m_zone = SecurityZone.NoZone; } public ZoneMembershipCondition( SecurityZone zone ) { VerifyZone( zone ); this.SecurityZone = zone; } //------------------------------------------------------ // // PUBLIC ACCESSOR METHODS // //------------------------------------------------------ public SecurityZone SecurityZone { set { VerifyZone( value ); m_zone = value; } get { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); return m_zone; } } //----------------------------------------------------- // // PRIVATE AND PROTECTED HELPERS FOR ACCESSORS AND CONSTRUCTORS // //------------------------------------------------------ private static void VerifyZone( SecurityZone zone ) { if (zone < SecurityZone.MyComputer || zone > SecurityZone.Untrusted) { throw new ArgumentException( Environment.GetResourceString( "Argument_IllegalZone" ) ); } Contract.EndContractBlock(); } //----------------------------------------------------- // // IMEMBERSHIPCONDITION IMPLEMENTATION // //----------------------------------------------------- public bool Check( Evidence evidence ) { object usedEvidence = null; return (this as IReportMatchMembershipCondition).Check(evidence, out usedEvidence); } bool IReportMatchMembershipCondition.Check(Evidence evidence, out object usedEvidence) { usedEvidence = null; if (evidence == null) return false; Zone zone = evidence.GetHostEvidence(); if (zone != null) { if (m_zone == SecurityZone.NoZone && m_element != null) { ParseZone(); } if (zone.SecurityZone == m_zone) { usedEvidence = zone; return true; } } return false; } public IMembershipCondition Copy() { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); return new ZoneMembershipCondition( m_zone ); } public SecurityElement ToXml() { return ToXml( null ); } public void FromXml( SecurityElement e ) { FromXml( e, null ); } public SecurityElement ToXml( PolicyLevel level ) { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); SecurityElement root = new SecurityElement( "IMembershipCondition" ); System.Security.Util.XMLUtil.AddClassAttribute( root, this.GetType(), "System.Security.Policy.ZoneMembershipCondition" ); // If you hit this assert then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assert below. Contract.Assert( this.GetType().FullName.Equals( "System.Security.Policy.ZoneMembershipCondition" ), "Class name changed!" ); root.AddAttribute( "version", "1" ); if (m_zone != SecurityZone.NoZone) root.AddAttribute( "Zone", Enum.GetName( typeof( SecurityZone ), m_zone ) ); return root; } public void FromXml( SecurityElement e, PolicyLevel level ) { if (e == null) throw new ArgumentNullException("e"); if (!e.Tag.Equals( "IMembershipCondition" )) { throw new ArgumentException( Environment.GetResourceString( "Argument_MembershipConditionElement" ) ); } Contract.EndContractBlock(); lock (this) { m_zone = SecurityZone.NoZone; m_element = e; } } private void ParseZone() { lock (this) { if (m_element == null) return; String eZone = m_element.Attribute( "Zone" ); m_zone = SecurityZone.NoZone; if (eZone != null) { m_zone = (SecurityZone)Enum.Parse( typeof( SecurityZone ), eZone ); } else { throw new ArgumentException( Environment.GetResourceString( "Argument_ZoneCannotBeNull" ) ); } VerifyZone(m_zone); m_element = null; } } public override bool Equals( Object o ) { ZoneMembershipCondition that = (o as ZoneMembershipCondition); if (that != null) { if (this.m_zone == SecurityZone.NoZone && this.m_element != null) this.ParseZone(); if (that.m_zone == SecurityZone.NoZone && that.m_element != null) that.ParseZone(); if(this.m_zone == that.m_zone) { return true; } } return false; } public override int GetHashCode() { if (this.m_zone == SecurityZone.NoZone && this.m_element != null) this.ParseZone(); return (int)m_zone; } public override String ToString() { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); return String.Format( CultureInfo.CurrentCulture, Environment.GetResourceString( "Zone_ToString" ), s_names[(int)m_zone] ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Diagnostics.Contracts; // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // ZoneMembershipCondition.cs // // [....] // // Implementation of membership condition for zones // namespace System.Security.Policy { using System; using SecurityManager = System.Security.SecurityManager; using PermissionSet = System.Security.PermissionSet; using SecurityElement = System.Security.SecurityElement; using System.Collections; using System.Globalization; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class ZoneMembershipCondition : IMembershipCondition, IConstantMembershipCondition, IReportMatchMembershipCondition { //------------------------------------------------------ // // PRIVATE CONSTANTS // //----------------------------------------------------- private static readonly String[] s_names = {"MyComputer", "Intranet", "Trusted", "Internet", "Untrusted"}; //----------------------------------------------------- // // PRIVATE STATE DATA // //----------------------------------------------------- private SecurityZone m_zone; private SecurityElement m_element; //------------------------------------------------------ // // PUBLIC CONSTRUCTORS // //----------------------------------------------------- internal ZoneMembershipCondition() { m_zone = SecurityZone.NoZone; } public ZoneMembershipCondition( SecurityZone zone ) { VerifyZone( zone ); this.SecurityZone = zone; } //------------------------------------------------------ // // PUBLIC ACCESSOR METHODS // //------------------------------------------------------ public SecurityZone SecurityZone { set { VerifyZone( value ); m_zone = value; } get { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); return m_zone; } } //----------------------------------------------------- // // PRIVATE AND PROTECTED HELPERS FOR ACCESSORS AND CONSTRUCTORS // //------------------------------------------------------ private static void VerifyZone( SecurityZone zone ) { if (zone < SecurityZone.MyComputer || zone > SecurityZone.Untrusted) { throw new ArgumentException( Environment.GetResourceString( "Argument_IllegalZone" ) ); } Contract.EndContractBlock(); } //----------------------------------------------------- // // IMEMBERSHIPCONDITION IMPLEMENTATION // //----------------------------------------------------- public bool Check( Evidence evidence ) { object usedEvidence = null; return (this as IReportMatchMembershipCondition).Check(evidence, out usedEvidence); } bool IReportMatchMembershipCondition.Check(Evidence evidence, out object usedEvidence) { usedEvidence = null; if (evidence == null) return false; Zone zone = evidence.GetHostEvidence(); if (zone != null) { if (m_zone == SecurityZone.NoZone && m_element != null) { ParseZone(); } if (zone.SecurityZone == m_zone) { usedEvidence = zone; return true; } } return false; } public IMembershipCondition Copy() { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); return new ZoneMembershipCondition( m_zone ); } public SecurityElement ToXml() { return ToXml( null ); } public void FromXml( SecurityElement e ) { FromXml( e, null ); } public SecurityElement ToXml( PolicyLevel level ) { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); SecurityElement root = new SecurityElement( "IMembershipCondition" ); System.Security.Util.XMLUtil.AddClassAttribute( root, this.GetType(), "System.Security.Policy.ZoneMembershipCondition" ); // If you hit this assert then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assert below. Contract.Assert( this.GetType().FullName.Equals( "System.Security.Policy.ZoneMembershipCondition" ), "Class name changed!" ); root.AddAttribute( "version", "1" ); if (m_zone != SecurityZone.NoZone) root.AddAttribute( "Zone", Enum.GetName( typeof( SecurityZone ), m_zone ) ); return root; } public void FromXml( SecurityElement e, PolicyLevel level ) { if (e == null) throw new ArgumentNullException("e"); if (!e.Tag.Equals( "IMembershipCondition" )) { throw new ArgumentException( Environment.GetResourceString( "Argument_MembershipConditionElement" ) ); } Contract.EndContractBlock(); lock (this) { m_zone = SecurityZone.NoZone; m_element = e; } } private void ParseZone() { lock (this) { if (m_element == null) return; String eZone = m_element.Attribute( "Zone" ); m_zone = SecurityZone.NoZone; if (eZone != null) { m_zone = (SecurityZone)Enum.Parse( typeof( SecurityZone ), eZone ); } else { throw new ArgumentException( Environment.GetResourceString( "Argument_ZoneCannotBeNull" ) ); } VerifyZone(m_zone); m_element = null; } } public override bool Equals( Object o ) { ZoneMembershipCondition that = (o as ZoneMembershipCondition); if (that != null) { if (this.m_zone == SecurityZone.NoZone && this.m_element != null) this.ParseZone(); if (that.m_zone == SecurityZone.NoZone && that.m_element != null) that.ParseZone(); if(this.m_zone == that.m_zone) { return true; } } return false; } public override int GetHashCode() { if (this.m_zone == SecurityZone.NoZone && this.m_element != null) this.ParseZone(); return (int)m_zone; } public override String ToString() { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); return String.Format( CultureInfo.CurrentCulture, Environment.GetResourceString( "Zone_ToString" ), s_names[(int)m_zone] ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
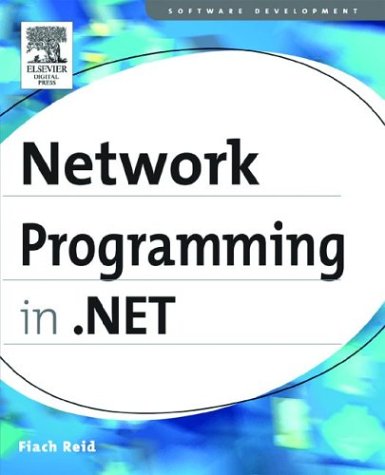
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FormViewCommandEventArgs.cs
- WebControlsSection.cs
- WSSecurityTokenSerializer.cs
- SystemTcpConnection.cs
- MsmqQueue.cs
- remotingproxy.cs
- Quack.cs
- FreezableDefaultValueFactory.cs
- DockPanel.cs
- FileInfo.cs
- GregorianCalendarHelper.cs
- NullReferenceException.cs
- GeometryModel3D.cs
- SqlLiftWhereClauses.cs
- Message.cs
- oledbconnectionstring.cs
- AnnotationResource.cs
- AttributeData.cs
- Point3DAnimationBase.cs
- TemplateField.cs
- XmlWriter.cs
- XmlNode.cs
- CollectionViewGroup.cs
- PathSegmentCollection.cs
- TextSchema.cs
- ObjectListItem.cs
- ProcessModelSection.cs
- AsyncResult.cs
- AdornerHitTestResult.cs
- BindingCollection.cs
- Misc.cs
- MaterialGroup.cs
- WebPartRestoreVerb.cs
- RadioButtonStandardAdapter.cs
- ScriptBehaviorDescriptor.cs
- StringFreezingAttribute.cs
- CollectionBuilder.cs
- FileSecurity.cs
- StrokeIntersection.cs
- OAVariantLib.cs
- ToolboxComponentsCreatingEventArgs.cs
- TextEditorSelection.cs
- SmtpSection.cs
- _BasicClient.cs
- BitmapData.cs
- PasswordPropertyTextAttribute.cs
- Misc.cs
- SerializableAttribute.cs
- IIS7WorkerRequest.cs
- wpf-etw.cs
- BinaryObjectInfo.cs
- NativeDirectoryServicesQueryAPIs.cs
- InputLangChangeRequestEvent.cs
- RedistVersionInfo.cs
- TreeViewImageIndexConverter.cs
- HttpCacheParams.cs
- AmbientProperties.cs
- ContentControl.cs
- ipaddressinformationcollection.cs
- ComponentEditorForm.cs
- ApplicationGesture.cs
- RawStylusInput.cs
- RuntimeConfigLKG.cs
- DataKey.cs
- XamlVector3DCollectionSerializer.cs
- Span.cs
- UnSafeCharBuffer.cs
- CustomAttributeBuilder.cs
- XmlDataSource.cs
- TraceEventCache.cs
- XMLDiffLoader.cs
- CodeExporter.cs
- RSAOAEPKeyExchangeDeformatter.cs
- ConfigurationManagerInternalFactory.cs
- SQLBoolean.cs
- ResourceDescriptionAttribute.cs
- MetadataExchangeClient.cs
- SetIndexBinder.cs
- DataSetMappper.cs
- HtmlTableRowCollection.cs
- PerspectiveCamera.cs
- TypeSystemProvider.cs
- ReferencedCollectionType.cs
- IndexedString.cs
- FunctionQuery.cs
- EmptyEnumerable.cs
- TreeNodeBindingDepthConverter.cs
- TableNameAttribute.cs
- TagNameToTypeMapper.cs
- SecurityVersion.cs
- CompilationSection.cs
- EventLogException.cs
- SqlError.cs
- BrushMappingModeValidation.cs
- Rectangle.cs
- OrderedHashRepartitionStream.cs
- HashCryptoHandle.cs
- ListControlBuilder.cs
- Array.cs
- UserControl.cs