Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Collections / Generic / IDictionary.cs / 1305376 / IDictionary.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Interface: IDictionary ** **[....] ** ** ** Purpose: Base interface for all generic dictionaries. ** ** ===========================================================*/ namespace System.Collections.Generic { using System; using System.Diagnostics.Contracts; // An IDictionary is a possibly unordered set of key-value pairs. // Keys can be any non-null object. Values can be any object. // You can look up a value in an IDictionary via the default indexed // property, Items. [ContractClass(typeof(IDictionaryContract<,>))] public interface IDictionary: ICollection > { // Interfaces are not serializable // The Item property provides methods to read and edit entries // in the Dictionary. TValue this[TKey key] { get; set; } // Returns a collections of the keys in this dictionary. ICollection Keys { get; } // Returns a collections of the values in this dictionary. ICollection Values { get; } // Returns whether this dictionary contains a particular key. // bool ContainsKey(TKey key); // Adds a key-value pair to the dictionary. // void Add(TKey key, TValue value); // Removes a particular key from the dictionary. // bool Remove(TKey key); bool TryGetValue(TKey key, out TValue value); } [ContractClassFor(typeof(IDictionary<,>))] internal class IDictionaryContract : IDictionary { TValue IDictionary .this[TKey key] { get { return default(TValue); } set { } } ICollection IDictionary .Keys { get { Contract.Ensures(Contract.Result >() != null); return default(ICollection ); } } // Returns a collections of the values in this dictionary. ICollection IDictionary .Values { get { Contract.Ensures(Contract.Result >() != null); return default(ICollection ); } } bool IDictionary .ContainsKey(TKey key) { return default(bool); } void IDictionary .Add(TKey key, TValue value) { } bool IDictionary .Remove(TKey key) { //Contract.Ensures(Contract.Result () == false || ((ICollection >)this).Count == Contract.OldValue(((ICollection >)this).Count) - 1); // not threadsafe return default(bool); } bool IDictionary .TryGetValue(TKey key, out TValue value) { value = default(TValue); return default(bool); } #region ICollection > Members void ICollection >.Add(KeyValuePair value) { //Contract.Ensures(((ICollection >)this).Count == Contract.OldValue(((ICollection >)this).Count) + 1); // not threadsafe } bool ICollection >.IsReadOnly { get { return default(bool); } } int ICollection >.Count { get { Contract.Ensures(Contract.Result () >= 0); return default(int); } } void ICollection >.Clear() { } bool ICollection >.Contains(KeyValuePair value) { // Contract.Ensures(((ICollection >)this).Count > 0 || Contract.Result () == false); // not threadsafe return default(bool); } void ICollection >.CopyTo(KeyValuePair [] array, int startIndex) { //Contract.Requires(array != null); //Contract.Requires(startIndex >= 0); //Contract.Requires(startIndex + ((ICollection >)this).Count <= array.Length); } bool ICollection >.Remove(KeyValuePair value) { // No information if removal fails. return default(bool); } IEnumerator > IEnumerable >.GetEnumerator() { Contract.Ensures(Contract.Result >>() != null); return default(IEnumerator >); } IEnumerator IEnumerable.GetEnumerator() { Contract.Ensures(Contract.Result () != null); return default(IEnumerator); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Interface: IDictionary ** ** [....] ** ** ** Purpose: Base interface for all generic dictionaries. ** ** ===========================================================*/ namespace System.Collections.Generic { using System; using System.Diagnostics.Contracts; // An IDictionary is a possibly unordered set of key-value pairs. // Keys can be any non-null object. Values can be any object. // You can look up a value in an IDictionary via the default indexed // property, Items. [ContractClass(typeof(IDictionaryContract<,>))] public interface IDictionary: ICollection > { // Interfaces are not serializable // The Item property provides methods to read and edit entries // in the Dictionary. TValue this[TKey key] { get; set; } // Returns a collections of the keys in this dictionary. ICollection Keys { get; } // Returns a collections of the values in this dictionary. ICollection Values { get; } // Returns whether this dictionary contains a particular key. // bool ContainsKey(TKey key); // Adds a key-value pair to the dictionary. // void Add(TKey key, TValue value); // Removes a particular key from the dictionary. // bool Remove(TKey key); bool TryGetValue(TKey key, out TValue value); } [ContractClassFor(typeof(IDictionary<,>))] internal class IDictionaryContract : IDictionary { TValue IDictionary .this[TKey key] { get { return default(TValue); } set { } } ICollection IDictionary .Keys { get { Contract.Ensures(Contract.Result >() != null); return default(ICollection ); } } // Returns a collections of the values in this dictionary. ICollection IDictionary .Values { get { Contract.Ensures(Contract.Result >() != null); return default(ICollection ); } } bool IDictionary .ContainsKey(TKey key) { return default(bool); } void IDictionary .Add(TKey key, TValue value) { } bool IDictionary .Remove(TKey key) { //Contract.Ensures(Contract.Result () == false || ((ICollection >)this).Count == Contract.OldValue(((ICollection >)this).Count) - 1); // not threadsafe return default(bool); } bool IDictionary .TryGetValue(TKey key, out TValue value) { value = default(TValue); return default(bool); } #region ICollection > Members void ICollection >.Add(KeyValuePair value) { //Contract.Ensures(((ICollection >)this).Count == Contract.OldValue(((ICollection >)this).Count) + 1); // not threadsafe } bool ICollection >.IsReadOnly { get { return default(bool); } } int ICollection >.Count { get { Contract.Ensures(Contract.Result () >= 0); return default(int); } } void ICollection >.Clear() { } bool ICollection >.Contains(KeyValuePair value) { // Contract.Ensures(((ICollection >)this).Count > 0 || Contract.Result () == false); // not threadsafe return default(bool); } void ICollection >.CopyTo(KeyValuePair [] array, int startIndex) { //Contract.Requires(array != null); //Contract.Requires(startIndex >= 0); //Contract.Requires(startIndex + ((ICollection >)this).Count <= array.Length); } bool ICollection >.Remove(KeyValuePair value) { // No information if removal fails. return default(bool); } IEnumerator > IEnumerable >.GetEnumerator() { Contract.Ensures(Contract.Result >>() != null); return default(IEnumerator >); } IEnumerator IEnumerable.GetEnumerator() { Contract.Ensures(Contract.Result () != null); return default(IEnumerator); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
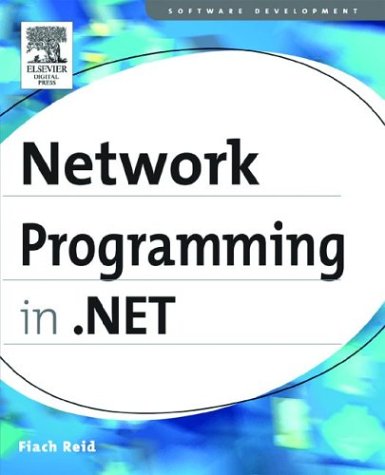
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ViewUtilities.cs
- InnerItemCollectionView.cs
- MetadataArtifactLoaderFile.cs
- ListViewGroup.cs
- AutomationElementIdentifiers.cs
- ParserStreamGeometryContext.cs
- DataGrid.cs
- SerTrace.cs
- SecUtil.cs
- Help.cs
- ReadOnlyCollectionBase.cs
- CheckBoxFlatAdapter.cs
- XomlCompilerResults.cs
- CacheRequest.cs
- PropertyReference.cs
- SymmetricAlgorithm.cs
- DataGridDetailsPresenter.cs
- XmlTextReaderImpl.cs
- DropShadowEffect.cs
- ActivityXamlServices.cs
- IDQuery.cs
- OutputCacheProfile.cs
- EntityClientCacheKey.cs
- SortedDictionary.cs
- Label.cs
- CloseCollectionAsyncResult.cs
- EllipseGeometry.cs
- _FtpDataStream.cs
- CharacterString.cs
- ComboBoxDesigner.cs
- LinkUtilities.cs
- EdmItemCollection.cs
- RepeaterCommandEventArgs.cs
- WebBrowserNavigatingEventHandler.cs
- DataFormats.cs
- AffineTransform3D.cs
- EndpointInfoCollection.cs
- LocatorPart.cs
- Qualifier.cs
- DetailsView.cs
- TableParagraph.cs
- Model3DCollection.cs
- AsynchronousChannelMergeEnumerator.cs
- InitializingNewItemEventArgs.cs
- RowToFieldTransformer.cs
- AutoCompleteStringCollection.cs
- RemotingSurrogateSelector.cs
- __FastResourceComparer.cs
- SessionEndedEventArgs.cs
- TailPinnedEventArgs.cs
- ProxyFragment.cs
- PtsHelper.cs
- DesignerCapabilities.cs
- Subtree.cs
- ExceptionUtil.cs
- XmlIncludeAttribute.cs
- TypeElement.cs
- XmlSchemaType.cs
- XmlImplementation.cs
- ToggleButtonAutomationPeer.cs
- DocumentApplicationJournalEntry.cs
- SecurityKeyIdentifier.cs
- ColumnMapProcessor.cs
- HtmlMeta.cs
- WebPartDeleteVerb.cs
- Deserializer.cs
- RegexInterpreter.cs
- PEFileEvidenceFactory.cs
- NetSectionGroup.cs
- DecoratedNameAttribute.cs
- AppearanceEditorPart.cs
- ListView.cs
- ButtonField.cs
- ThumbButtonInfo.cs
- OSFeature.cs
- Constraint.cs
- RawStylusSystemGestureInputReport.cs
- GZipStream.cs
- ListSortDescriptionCollection.cs
- SecurityProtocolFactory.cs
- MSG.cs
- BaseInfoTable.cs
- DispatcherEventArgs.cs
- MouseEventArgs.cs
- ToolboxCategoryItems.cs
- BaseUriHelper.cs
- StrongNameUtility.cs
- XmlWellformedWriter.cs
- ListViewItem.cs
- CustomCategoryAttribute.cs
- RelatedView.cs
- WebInvokeAttribute.cs
- HttpWriter.cs
- TypeUnloadedException.cs
- RequestCacheManager.cs
- TakeOrSkipWhileQueryOperator.cs
- ValidationUtility.cs
- BitStream.cs
- NGCSerializer.cs
- _RequestCacheProtocol.cs