Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / IdentityModelDictionary.cs / 1305376 / IdentityModelDictionary.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel { using System.Xml; using System.Collections.Generic; class IdentityModelDictionary : IXmlDictionary { static public readonly IdentityModelDictionary Version1 = new IdentityModelDictionary(new IdentityModelStringsVersion1()); IdentityModelStrings strings; int count; XmlDictionaryString[] dictionaryStrings; Dictionarydictionary; XmlDictionaryString[] versionedDictionaryStrings; public IdentityModelDictionary(IdentityModelStrings strings) { this.strings = strings; this.count = strings.Count; } static public IdentityModelDictionary CurrentVersion { get { return Version1; } } public XmlDictionaryString CreateString(string value, int key) { return new XmlDictionaryString(this, value, key); } public bool TryLookup(string key, out XmlDictionaryString value) { if (key == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("key")); if (this.dictionary == null) { Dictionary dictionary = new Dictionary (count); for (int i = 0; i < count; i++) dictionary.Add(strings[i], i); this.dictionary = dictionary; } int id; if (this.dictionary.TryGetValue(key, out id)) return TryLookup(id, out value); value = null; return false; } public bool TryLookup(int key, out XmlDictionaryString value) { if (key < 0 || key >= count) { value = null; return false; } if (dictionaryStrings == null) dictionaryStrings = new XmlDictionaryString[count]; XmlDictionaryString s = dictionaryStrings[key]; if (s == null) { s = CreateString(strings[key], key); dictionaryStrings[key]= s; } value = s; return true; } public bool TryLookup(XmlDictionaryString key, out XmlDictionaryString value) { if (key == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("key")); if (key.Dictionary == this) { value = key; return true; } if (key.Dictionary == CurrentVersion) { if (versionedDictionaryStrings == null) versionedDictionaryStrings = new XmlDictionaryString[CurrentVersion.count]; XmlDictionaryString s = versionedDictionaryStrings[key.Key]; if (s == null) { if (!TryLookup(key.Value, out s)) { value = null; return false; } versionedDictionaryStrings[key.Key] = s; } value = s; return true; } value = null; return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel { using System.Xml; using System.Collections.Generic; class IdentityModelDictionary : IXmlDictionary { static public readonly IdentityModelDictionary Version1 = new IdentityModelDictionary(new IdentityModelStringsVersion1()); IdentityModelStrings strings; int count; XmlDictionaryString[] dictionaryStrings; Dictionary dictionary; XmlDictionaryString[] versionedDictionaryStrings; public IdentityModelDictionary(IdentityModelStrings strings) { this.strings = strings; this.count = strings.Count; } static public IdentityModelDictionary CurrentVersion { get { return Version1; } } public XmlDictionaryString CreateString(string value, int key) { return new XmlDictionaryString(this, value, key); } public bool TryLookup(string key, out XmlDictionaryString value) { if (key == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("key")); if (this.dictionary == null) { Dictionary dictionary = new Dictionary (count); for (int i = 0; i < count; i++) dictionary.Add(strings[i], i); this.dictionary = dictionary; } int id; if (this.dictionary.TryGetValue(key, out id)) return TryLookup(id, out value); value = null; return false; } public bool TryLookup(int key, out XmlDictionaryString value) { if (key < 0 || key >= count) { value = null; return false; } if (dictionaryStrings == null) dictionaryStrings = new XmlDictionaryString[count]; XmlDictionaryString s = dictionaryStrings[key]; if (s == null) { s = CreateString(strings[key], key); dictionaryStrings[key]= s; } value = s; return true; } public bool TryLookup(XmlDictionaryString key, out XmlDictionaryString value) { if (key == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("key")); if (key.Dictionary == this) { value = key; return true; } if (key.Dictionary == CurrentVersion) { if (versionedDictionaryStrings == null) versionedDictionaryStrings = new XmlDictionaryString[CurrentVersion.count]; XmlDictionaryString s = versionedDictionaryStrings[key.Key]; if (s == null) { if (!TryLookup(key.Value, out s)) { value = null; return false; } versionedDictionaryStrings[key.Key] = s; } value = s; return true; } value = null; return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
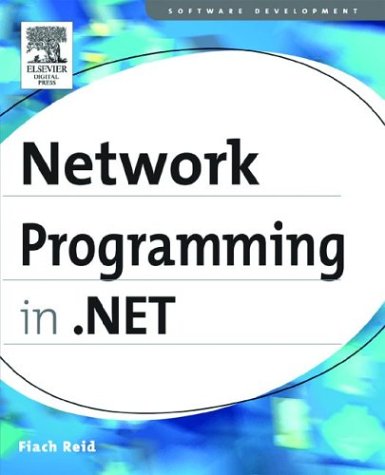
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlConnectionPoolProviderInfo.cs
- BaseEntityWrapper.cs
- WebContext.cs
- CodeVariableDeclarationStatement.cs
- TreeNode.cs
- GlobalAclOperationRequirement.cs
- OracleCommandBuilder.cs
- SettingsSavedEventArgs.cs
- SingleAnimationBase.cs
- SystemIcons.cs
- QueryOutputWriter.cs
- SafeRegistryKey.cs
- ProxyWebPartConnectionCollection.cs
- SiteMapNodeItemEventArgs.cs
- CriticalFinalizerObject.cs
- WindowsStatic.cs
- DbProviderManifest.cs
- NotifyInputEventArgs.cs
- HelpOperationInvoker.cs
- ColumnBinding.cs
- PeerCollaboration.cs
- EditorZoneAutoFormat.cs
- OdbcConnectionHandle.cs
- ToolboxCategory.cs
- WebProxyScriptElement.cs
- WebPartDisplayModeCancelEventArgs.cs
- TableCellCollection.cs
- PositiveTimeSpanValidatorAttribute.cs
- XmlSchemaAppInfo.cs
- WebPartConnectionsDisconnectVerb.cs
- HttpClientCertificate.cs
- OracleRowUpdatedEventArgs.cs
- ConfigurationManagerInternalFactory.cs
- MemberBinding.cs
- SecurityPolicySection.cs
- WinCategoryAttribute.cs
- NavigatorInput.cs
- IChannel.cs
- ColorPalette.cs
- HttpCapabilitiesSectionHandler.cs
- SessionStateModule.cs
- StatusStrip.cs
- SchemaLookupTable.cs
- RegexMatch.cs
- WindowsListViewGroup.cs
- SingleObjectCollection.cs
- XmlAttributeHolder.cs
- COM2FontConverter.cs
- ForEach.cs
- CodeArrayIndexerExpression.cs
- SystemColors.cs
- SoapAttributeOverrides.cs
- GridToolTip.cs
- AlignmentYValidation.cs
- Parser.cs
- DataGridViewRowEventArgs.cs
- WebConfigurationManager.cs
- DataGridViewTopLeftHeaderCell.cs
- SizeChangedInfo.cs
- WebPartChrome.cs
- CompModSwitches.cs
- RelationshipDetailsCollection.cs
- HatchBrush.cs
- PropertyTabChangedEvent.cs
- RelationshipWrapper.cs
- QueryTaskGroupState.cs
- CapiSafeHandles.cs
- CompositeDataBoundControl.cs
- DataContractSet.cs
- MimePart.cs
- TableCell.cs
- EntitySetBase.cs
- OleDbRowUpdatingEvent.cs
- SocketPermission.cs
- SemanticResultKey.cs
- RotationValidation.cs
- PropertyGeneratedEventArgs.cs
- ValueConversionAttribute.cs
- TemplateParser.cs
- SocketElement.cs
- DbConnectionPoolIdentity.cs
- WebPartEditorOkVerb.cs
- CompleteWizardStep.cs
- ReadOnlyDictionary.cs
- FixedFindEngine.cs
- ArgumentNullException.cs
- KeyPullup.cs
- InheritanceRules.cs
- TailCallAnalyzer.cs
- Rfc2898DeriveBytes.cs
- GatewayDefinition.cs
- SimpleColumnProvider.cs
- StackBuilderSink.cs
- TextSegment.cs
- WindowsListViewItemCheckBox.cs
- ChtmlTextWriter.cs
- ResetableIterator.cs
- BitmapEffectInput.cs
- FaultContext.cs
- OutputBuffer.cs