Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Common / DbProviderManifest.cs / 3 / DbProviderManifest.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Metadata.Edm; using System.Xml; namespace System.Data.Common { ////// Metadata Interface for all CLR types types /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbProviderManifest { ////// Constructor /// protected DbProviderManifest() { } ///Value to pass to GetInformation to get the StoreSchemaDefinition public static readonly string StoreSchemaDefinition = "StoreSchemaDefinition"; ///Value to pass to GetInformation to get the StoreSchemaMapping public static readonly string StoreSchemaMapping = "StoreSchemaMapping"; ///Value to pass to GetInformation to get the ConceptualSchemaDefinition public static readonly string ConceptualSchemaDefinition = "ConceptualSchemaDefinition"; // System Facet Info ////// Name of the MaxLength Facet /// internal const string MaxLengthFacetName = "MaxLength"; ////// Name of the Unicode Facet /// internal const string UnicodeFacetName = "Unicode"; ////// Name of the FixedLength Facet /// internal const string FixedLengthFacetName = "FixedLength"; ////// Name of the Precision Facet /// internal const string PrecisionFacetName = "Precision"; ////// Name of the Scale Facet /// internal const string ScaleFacetName = "Scale"; ////// Name of the Nullable Facet /// internal const string NullableFacetName = "Nullable"; ////// Name of the DefaultValue Facet /// internal const string DefaultValueFacetName = "DefaultValue"; ////// Name of the Collation Facet /// internal const string CollationFacetName = "Collation"; ////// Returns the namespace used by this provider manifest /// public abstract string NamespaceName {get;} ////// Return the set of types supported by the store /// ///A collection of primitive types public abstract System.Collections.ObjectModel.ReadOnlyCollectionGetStoreTypes(); /// /// Returns all the edm functions supported by the provider manifest. /// ///A collection of edm functions. public abstract System.Collections.ObjectModel.ReadOnlyCollectionGetStoreFunctions(); /// /// Returns all the FacetDescriptions for a particular type /// /// the type to return FacetDescriptions for ///The FacetDescriptions for the type given [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "edm")] public abstract System.Collections.ObjectModel.ReadOnlyCollectionGetFacetDescriptions(EdmType edmType); /// /// This method allows a provider writer to take a type and a set of facets /// and reason about what the best mapped equivalent type in EDM would be. /// /// A TypeUsage encapsulating a store type and a set of facets ///A TypeUsage encapsulating an EDM type and a set of facets [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public abstract TypeUsage GetEdmType(TypeUsage storeType); ////// This method allows a provider writer to take a type and a set of facets /// and reason about what the best mapped equivalent type in the store would be. /// /// A TypeUsage encapsulating an EDM type and a set of facets ///A TypeUsage encapsulating a store type and a set of facets [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "edm")] public abstract TypeUsage GetStoreType(TypeUsage edmType); ////// Providers should override this to return information specific to their provider. /// /// This method should never return null. /// /// The name of the information to be retrieved. ///An XmlReader at the begining of the information requested. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] protected abstract XmlReader GetDbInformation(string informationType); ////// Gets framework and provider specific information /// /// This method should never return null. /// /// The name of the information to be retrieved. ///An XmlReader at the begining of the information requested. public XmlReader GetInformation(string informationType) { XmlReader reader = null; try { reader = GetDbInformation(informationType); } catch (Exception e) { // we should not be wrapping all exceptions if (EntityUtil.IsCatchableExceptionType(e)) { // we don't want folks to have to know all the various types of exceptions that can // occur, so we just rethrow a ProviderIncompatibleException and make whatever we caught // the inner exception of it. throw EntityUtil.ProviderIncompatible( System.Data.Entity.Strings.EntityClient_FailedToGetInformation(informationType), e); } throw; } if (reader == null) { // if the provider returned null for the conceptual schema definition, return the default one if (informationType == ConceptualSchemaDefinition) { return DbProviderServices.GetConceptualSchemaDescription(); } throw EntityUtil.ProviderIncompatible(System.Data.Entity.Strings.ProviderReturnedNullForGetDbInformation(informationType)); } return reader; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Metadata.Edm; using System.Xml; namespace System.Data.Common { ////// Metadata Interface for all CLR types types /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbProviderManifest { ////// Constructor /// protected DbProviderManifest() { } ///Value to pass to GetInformation to get the StoreSchemaDefinition public static readonly string StoreSchemaDefinition = "StoreSchemaDefinition"; ///Value to pass to GetInformation to get the StoreSchemaMapping public static readonly string StoreSchemaMapping = "StoreSchemaMapping"; ///Value to pass to GetInformation to get the ConceptualSchemaDefinition public static readonly string ConceptualSchemaDefinition = "ConceptualSchemaDefinition"; // System Facet Info ////// Name of the MaxLength Facet /// internal const string MaxLengthFacetName = "MaxLength"; ////// Name of the Unicode Facet /// internal const string UnicodeFacetName = "Unicode"; ////// Name of the FixedLength Facet /// internal const string FixedLengthFacetName = "FixedLength"; ////// Name of the Precision Facet /// internal const string PrecisionFacetName = "Precision"; ////// Name of the Scale Facet /// internal const string ScaleFacetName = "Scale"; ////// Name of the Nullable Facet /// internal const string NullableFacetName = "Nullable"; ////// Name of the DefaultValue Facet /// internal const string DefaultValueFacetName = "DefaultValue"; ////// Name of the Collation Facet /// internal const string CollationFacetName = "Collation"; ////// Returns the namespace used by this provider manifest /// public abstract string NamespaceName {get;} ////// Return the set of types supported by the store /// ///A collection of primitive types public abstract System.Collections.ObjectModel.ReadOnlyCollectionGetStoreTypes(); /// /// Returns all the edm functions supported by the provider manifest. /// ///A collection of edm functions. public abstract System.Collections.ObjectModel.ReadOnlyCollectionGetStoreFunctions(); /// /// Returns all the FacetDescriptions for a particular type /// /// the type to return FacetDescriptions for ///The FacetDescriptions for the type given [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "edm")] public abstract System.Collections.ObjectModel.ReadOnlyCollectionGetFacetDescriptions(EdmType edmType); /// /// This method allows a provider writer to take a type and a set of facets /// and reason about what the best mapped equivalent type in EDM would be. /// /// A TypeUsage encapsulating a store type and a set of facets ///A TypeUsage encapsulating an EDM type and a set of facets [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public abstract TypeUsage GetEdmType(TypeUsage storeType); ////// This method allows a provider writer to take a type and a set of facets /// and reason about what the best mapped equivalent type in the store would be. /// /// A TypeUsage encapsulating an EDM type and a set of facets ///A TypeUsage encapsulating a store type and a set of facets [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "edm")] public abstract TypeUsage GetStoreType(TypeUsage edmType); ////// Providers should override this to return information specific to their provider. /// /// This method should never return null. /// /// The name of the information to be retrieved. ///An XmlReader at the begining of the information requested. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] protected abstract XmlReader GetDbInformation(string informationType); ////// Gets framework and provider specific information /// /// This method should never return null. /// /// The name of the information to be retrieved. ///An XmlReader at the begining of the information requested. public XmlReader GetInformation(string informationType) { XmlReader reader = null; try { reader = GetDbInformation(informationType); } catch (Exception e) { // we should not be wrapping all exceptions if (EntityUtil.IsCatchableExceptionType(e)) { // we don't want folks to have to know all the various types of exceptions that can // occur, so we just rethrow a ProviderIncompatibleException and make whatever we caught // the inner exception of it. throw EntityUtil.ProviderIncompatible( System.Data.Entity.Strings.EntityClient_FailedToGetInformation(informationType), e); } throw; } if (reader == null) { // if the provider returned null for the conceptual schema definition, return the default one if (informationType == ConceptualSchemaDefinition) { return DbProviderServices.GetConceptualSchemaDescription(); } throw EntityUtil.ProviderIncompatible(System.Data.Entity.Strings.ProviderReturnedNullForGetDbInformation(informationType)); } return reader; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
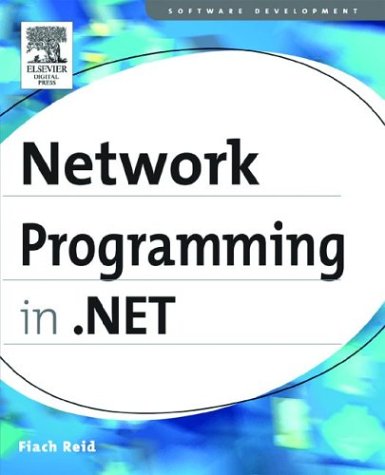
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SequentialUshortCollection.cs
- HttpModuleActionCollection.cs
- FreezableOperations.cs
- CookieParameter.cs
- RecommendedAsConfigurableAttribute.cs
- WebPartTransformerCollection.cs
- ListBox.cs
- ProjectionPruner.cs
- CodeDirectionExpression.cs
- ToolConsole.cs
- FontFamilyValueSerializer.cs
- MatchingStyle.cs
- XmlDictionaryReader.cs
- externdll.cs
- PageThemeParser.cs
- CornerRadiusConverter.cs
- RequestCache.cs
- TimeSpanValidator.cs
- AnnotationAdorner.cs
- TextEditor.cs
- WindowsTab.cs
- StylusTouchDevice.cs
- TimelineGroup.cs
- OutOfProcStateClientManager.cs
- CreatingCookieEventArgs.cs
- DataControlField.cs
- TypeDelegator.cs
- ScriptHandlerFactory.cs
- ToolStripContentPanel.cs
- ControlValuePropertyAttribute.cs
- MDIClient.cs
- DrawingVisual.cs
- CodeConditionStatement.cs
- TdsRecordBufferSetter.cs
- JoinCqlBlock.cs
- EntityDataSourceDesignerHelper.cs
- MetadataImporter.cs
- DataBindingCollectionConverter.cs
- TableRowCollection.cs
- BitmapFrame.cs
- InternalTypeHelper.cs
- SqlExpander.cs
- Assembly.cs
- ObjectConverter.cs
- UpdateException.cs
- ChildTable.cs
- AnonymousIdentificationSection.cs
- MetaData.cs
- WorkflowEventArgs.cs
- AxHost.cs
- MouseActionValueSerializer.cs
- CodePageUtils.cs
- CodeChecksumPragma.cs
- OciLobLocator.cs
- EventLogPermissionEntry.cs
- UnknownWrapper.cs
- ScrollableControl.cs
- SecurityCriticalDataForSet.cs
- InkPresenter.cs
- OleDbEnumerator.cs
- InvalidAsynchronousStateException.cs
- MimeTypeAttribute.cs
- PrinterUnitConvert.cs
- HandlerFactoryCache.cs
- ListView.cs
- SpeechRecognitionEngine.cs
- MarshalByValueComponent.cs
- SqlServices.cs
- ExecutionContext.cs
- UnsafeCollabNativeMethods.cs
- DragEventArgs.cs
- XmlWrappingWriter.cs
- ECDsa.cs
- InvalidPrinterException.cs
- StylusPointPropertyInfo.cs
- basecomparevalidator.cs
- ElapsedEventArgs.cs
- IfAction.cs
- Point3DConverter.cs
- NameValueCollection.cs
- ExtensionSimplifierMarkupObject.cs
- EnumBuilder.cs
- InvokeProviderWrapper.cs
- PropertyKey.cs
- ArrayList.cs
- SourceInterpreter.cs
- Relationship.cs
- ProfilePropertySettings.cs
- HttpRawResponse.cs
- DrawingDrawingContext.cs
- ManagementInstaller.cs
- PersonalizationStateInfo.cs
- TextViewElement.cs
- HtmlCalendarAdapter.cs
- BitSet.cs
- ManagedIStream.cs
- DecoderNLS.cs
- DataGridViewRowHeaderCell.cs
- ServiceModelExtensionElement.cs
- VarRemapper.cs