Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / HttpProxyTransportBindingElement.cs / 2 / HttpProxyTransportBindingElement.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace Microsoft.InfoCards { using System.Collections.Generic; using System.ServiceModel.Description; using System.ServiceModel; using System.Net; using System.ServiceModel.Channels; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // This class is used to replace the TransportBindingElement to a binding element which uses the user's proxy // class HttpProxyTransportBindingElement :TransportBindingElement { HttpTransportBindingElement innerHttpTransport; IWebProxy proxy; // // Summary: // Replaces the HttpTransportBindingElement from the collection with HttpProxyTransportBindingElement // // Arguments: // bindingElements: The bindingElements collection to update // proxy: The proxy to be used for HttpTransport // turnOffClientAuthOnTransport: When set to true, the AuthenticationScheme for the transport // binding will be set to ANonymous // // Returns: // Returns the updated bindingElements collection. // public static BindingElementCollection ReplaceHttpTransportWithProxy( BindingElementCollection bindingElements, IWebProxy proxy, bool turnOffClientAuthOnTransport ) { int httpIndex = -1; for( int i = 0; i < bindingElements.Count; i++ ) { if( bindingElements[ i ] is HttpTransportBindingElement ) { httpIndex = i; break; } } if( httpIndex == -1 ) { // no HttpTransport to wrap, just return original binding return bindingElements; } IDT.Assert( httpIndex == bindingElements.Count - 1, "Transport should be last in the Binding Element list" ); HttpTransportBindingElement httpTransport = ( HttpTransportBindingElement )bindingElements[ httpIndex ]; if( turnOffClientAuthOnTransport ) { httpTransport.AuthenticationScheme = AuthenticationSchemes.Anonymous; } HttpProxyTransportBindingElement proxyTransport = new HttpProxyTransportBindingElement( proxy, httpTransport ); bindingElements[ httpIndex ] = proxyTransport; return bindingElements; } HttpProxyTransportBindingElement( IWebProxy proxy, HttpTransportBindingElement innerHttpTransport ) : base() { this.innerHttpTransport = innerHttpTransport; this.proxy = proxy; } HttpProxyTransportBindingElement( HttpProxyTransportBindingElement elementToBeCloned ) : base( elementToBeCloned ) { this.innerHttpTransport = elementToBeCloned.innerHttpTransport; this.proxy = elementToBeCloned.proxy; } public override long MaxBufferPoolSize { get { return this.innerHttpTransport.MaxBufferPoolSize; } set { this.innerHttpTransport.MaxBufferPoolSize = value; } } public override long MaxReceivedMessageSize { get { return this.innerHttpTransport.MaxReceivedMessageSize; } set { this.innerHttpTransport.MaxReceivedMessageSize = value; } } public override string Scheme { get { return this.innerHttpTransport.Scheme; } } public override IChannelFactoryBuildChannelFactory ( BindingContext context ) { this.innerHttpTransport.Proxy = this.proxy; HttpChannelFactory factory = (HttpChannelFactory)this.innerHttpTransport.BuildChannelFactory (context); return (IChannelFactory )(object)factory; } public override IChannelListener BuildChannelListener ( BindingContext context ) { return this.innerHttpTransport.BuildChannelListener ( context ); } public override bool CanBuildChannelFactory ( BindingContext context ) { return this.innerHttpTransport.CanBuildChannelFactory ( context ); } public override bool CanBuildChannelListener ( BindingContext context ) { return this.innerHttpTransport.CanBuildChannelListener ( context ); } public override BindingElement Clone() { return new HttpProxyTransportBindingElement( this ); } public override T GetProperty ( BindingContext context ) { return this.innerHttpTransport.GetProperty ( context ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
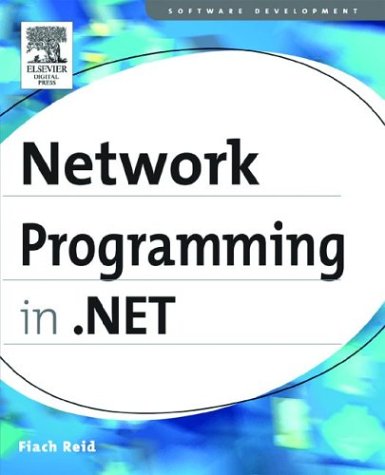
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClientConfigurationSystem.cs
- PageWrapper.cs
- Oid.cs
- SafeFindHandle.cs
- DocComment.cs
- ExecutionProperties.cs
- MetafileHeaderWmf.cs
- ConfigurationStrings.cs
- JavaScriptSerializer.cs
- ImageFormat.cs
- InvalidProgramException.cs
- LocalValueEnumerator.cs
- _NativeSSPI.cs
- ZipIOFileItemStream.cs
- SafePointer.cs
- DbTypeMap.cs
- BoundsDrawingContextWalker.cs
- EnumUnknown.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- MtomMessageEncoder.cs
- BitmapEffectDrawingContextState.cs
- ReachDocumentReferenceCollectionSerializerAsync.cs
- WindowsScroll.cs
- SharedPerformanceCounter.cs
- ManagedFilter.cs
- TableLayoutColumnStyleCollection.cs
- XmlnsDictionary.cs
- DictionaryManager.cs
- DataServiceClientException.cs
- HttpClientCertificate.cs
- FloatUtil.cs
- DataControlCommands.cs
- DefaultValueTypeConverter.cs
- ShaperBuffers.cs
- UIHelper.cs
- ComPlusDiagnosticTraceSchemas.cs
- SourceFileBuildProvider.cs
- GenericTextProperties.cs
- WebBrowser.cs
- WindowsFormsLinkLabel.cs
- WindowsPen.cs
- PolicyValidationException.cs
- PartitionedStreamMerger.cs
- RegexRunner.cs
- AttachedPropertiesService.cs
- XmlDomTextWriter.cs
- Triplet.cs
- Point3DCollection.cs
- PenContext.cs
- WorkflowInstanceQuery.cs
- TemplateColumn.cs
- DataGridViewTextBoxEditingControl.cs
- ZipIOBlockManager.cs
- ProxyElement.cs
- LayoutTable.cs
- SiteMapDataSourceView.cs
- DataView.cs
- XhtmlTextWriter.cs
- HMACMD5.cs
- WebPartZoneBase.cs
- IISMapPath.cs
- HttpServerChannel.cs
- ThreadSafeList.cs
- Publisher.cs
- XmlIterators.cs
- CheckableControlBaseAdapter.cs
- ColumnMapProcessor.cs
- Monitor.cs
- ToolStripKeyboardHandlingService.cs
- HtmlMeta.cs
- HandlerFactoryWrapper.cs
- TextSearch.cs
- ButtonField.cs
- PersistenceMetadataNamespace.cs
- RegexMatchCollection.cs
- Cursors.cs
- LoginNameDesigner.cs
- ConfigurationManagerHelper.cs
- ScrollPatternIdentifiers.cs
- BaseInfoTable.cs
- FixedPageStructure.cs
- Rectangle.cs
- ControlCachePolicy.cs
- RegisteredScript.cs
- StrongNameKeyPair.cs
- NameValueCollection.cs
- ExceptionUtil.cs
- MdiWindowListStrip.cs
- KeyFrames.cs
- MarkupCompilePass1.cs
- OwnerDrawPropertyBag.cs
- EventMappingSettingsCollection.cs
- GiveFeedbackEventArgs.cs
- XmlWriter.cs
- SingleResultAttribute.cs
- BitmapEffect.cs
- SapiGrammar.cs
- SafeViewOfFileHandle.cs
- CaseCqlBlock.cs
- SchemaImporterExtensionElementCollection.cs