Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / SynchronizedMessageSource.cs / 1 / SynchronizedMessageSource.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System.ServiceModel; using System.Threading; class SynchronizedMessageSource { IMessageSource source; ThreadNeutralSemaphore sourceLock; public SynchronizedMessageSource(IMessageSource source) { this.source = source; sourceLock = new ThreadNeutralSemaphore(1); } public IAsyncResult BeginWaitForMessage(TimeSpan timeout, AsyncCallback callback, object state) { return new WaitForMessageAsyncResult(this, timeout, callback, state); } public bool EndWaitForMessage(IAsyncResult result) { return WaitForMessageAsyncResult.End(result); } public bool WaitForMessage(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); if (!sourceLock.TryEnter(timeoutHelper.RemainingTime())) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new TimeoutException(SR.GetString(SR.WaitForMessageTimedOut, timeout), ThreadNeutralSemaphore.CreateEnterTimedOutException(timeout))); } try { return source.WaitForMessage(timeoutHelper.RemainingTime()); } finally { sourceLock.Exit(); } } public IAsyncResult BeginReceive(TimeSpan timeout, AsyncCallback callback, object state) { return new ReceiveAsyncResult(this, timeout, callback, state); } public Message EndReceive(IAsyncResult result) { return ReceiveAsyncResult.End(result); } public Message Receive(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); if (!sourceLock.TryEnter(timeoutHelper.RemainingTime())) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new TimeoutException(SR.GetString(SR.ReceiveTimedOut2, timeout), ThreadNeutralSemaphore.CreateEnterTimedOutException(timeout))); } try { return source.Receive(timeoutHelper.RemainingTime()); } finally { sourceLock.Exit(); } } abstract class SynchronizedAsyncResult: AsyncResult { T returnValue; bool exitLock; SynchronizedMessageSource syncSource; static WaitCallback onEnterComplete = new WaitCallback(OnEnterComplete); TimeoutHelper timeoutHelper; public SynchronizedAsyncResult(SynchronizedMessageSource syncSource, TimeSpan timeout, AsyncCallback callback, object state) : base(callback, state) { this.syncSource = syncSource; this.timeoutHelper = new TimeoutHelper(timeout); if (!syncSource.sourceLock.Enter(onEnterComplete, this)) return; exitLock = true; bool success = false; bool completeSelf; try { completeSelf = PerformOperation(timeoutHelper.RemainingTime()); success = true; } finally { if (!success) { ExitLock(); } } if (completeSelf) { CompleteWithUnlock(true); } } protected IMessageSource Source { get { return syncSource.source; } } protected void SetReturnValue(T returnValue) { this.returnValue = returnValue; } protected abstract bool PerformOperation(TimeSpan timeout); void ExitLock() { if (exitLock) { syncSource.sourceLock.Exit(); exitLock = false; } } protected void CompleteWithUnlock(bool synchronous) { CompleteWithUnlock(synchronous, null); } protected void CompleteWithUnlock(bool synchronous, Exception exception) { ExitLock(); base.Complete(synchronous, exception); } public static T End(IAsyncResult result) { SynchronizedAsyncResult thisPtr = AsyncResult.End >(result); return thisPtr.returnValue; } static void OnEnterComplete(object state) { SynchronizedAsyncResult thisPtr = (SynchronizedAsyncResult )state; Exception completionException = null; bool completeSelf; try { thisPtr.exitLock = true; completeSelf = thisPtr.PerformOperation(thisPtr.timeoutHelper.RemainingTime()); } #pragma warning suppress 56500 // [....], transferring exception to another thread catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) { throw; } completeSelf = true; completionException = e; } if (completeSelf) { thisPtr.CompleteWithUnlock(false, completionException); } } } class ReceiveAsyncResult : SynchronizedAsyncResult { static WaitCallback onReceiveComplete = new WaitCallback(OnReceiveComplete); public ReceiveAsyncResult(SynchronizedMessageSource syncSource, TimeSpan timeout, AsyncCallback callback, object state) : base(syncSource, timeout, callback, state) { } protected override bool PerformOperation(TimeSpan timeout) { if (Source.BeginReceive(timeout, onReceiveComplete, this) == AsyncReceiveResult.Completed) { SetReturnValue(Source.EndReceive()); return true; } return false; } static void OnReceiveComplete(object state) { ReceiveAsyncResult thisPtr = ((ReceiveAsyncResult)state); Exception completionException = null; try { thisPtr.SetReturnValue(thisPtr.Source.EndReceive()); } #pragma warning suppress 56500 // [....], transferring exception to another thread catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) { throw; } completionException = e; } thisPtr.CompleteWithUnlock(false, completionException); } } class WaitForMessageAsyncResult : SynchronizedAsyncResult { static WaitCallback onWaitForMessageComplete = new WaitCallback(OnWaitForMessageComplete); public WaitForMessageAsyncResult(SynchronizedMessageSource syncSource, TimeSpan timeout, AsyncCallback callback, object state) : base(syncSource, timeout, callback, state) { } protected override bool PerformOperation(TimeSpan timeout) { if (Source.BeginWaitForMessage(timeout, onWaitForMessageComplete, this) == AsyncReceiveResult.Completed) { SetReturnValue(Source.EndWaitForMessage()); return true; } return false; } static void OnWaitForMessageComplete(object state) { WaitForMessageAsyncResult thisPtr = (WaitForMessageAsyncResult)state; Exception completionException = null; try { thisPtr.SetReturnValue(thisPtr.Source.EndWaitForMessage()); } #pragma warning suppress 56500 // [....], transferring exception to another thread catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) { throw; } completionException = e; } thisPtr.CompleteWithUnlock(false, completionException); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
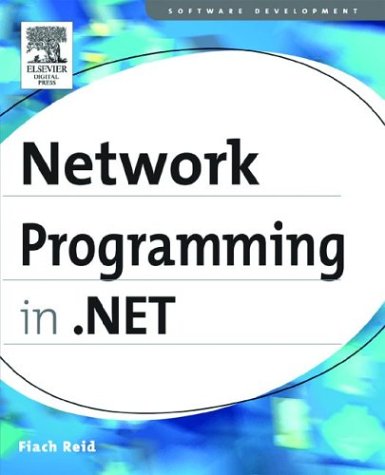
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlDuplicator.cs
- IncrementalCompileAnalyzer.cs
- FileSystemWatcher.cs
- InvalidTimeZoneException.cs
- FieldNameLookup.cs
- SqlCommand.cs
- AssociationSetMetadata.cs
- NetSectionGroup.cs
- FilterUserControlBase.cs
- CodeEntryPointMethod.cs
- UniqueTransportManagerRegistration.cs
- DynamicFilter.cs
- Model3DGroup.cs
- TaskFormBase.cs
- Int32RectValueSerializer.cs
- ControlParameter.cs
- TripleDES.cs
- HybridDictionary.cs
- XmlCharacterData.cs
- DataGridViewImageCell.cs
- QilValidationVisitor.cs
- EntityClientCacheKey.cs
- GridErrorDlg.cs
- CompilerGlobalScopeAttribute.cs
- SwitchCase.cs
- XmlCompatibilityReader.cs
- DataGrid.cs
- HashRepartitionEnumerator.cs
- CollaborationHelperFunctions.cs
- XmlMessageFormatter.cs
- NumericUpDown.cs
- EventToken.cs
- WindowsSecurityToken.cs
- ReachDocumentReferenceSerializer.cs
- DataBindingCollection.cs
- NameValueFileSectionHandler.cs
- HebrewCalendar.cs
- ToolBarButton.cs
- DataGridCell.cs
- HorizontalAlignConverter.cs
- FontClient.cs
- ConfigurationConverterBase.cs
- PackWebResponse.cs
- DbProviderFactories.cs
- StylusCollection.cs
- XmlAnyAttributeAttribute.cs
- HttpProfileGroupBase.cs
- DataFieldConverter.cs
- OperationExecutionFault.cs
- CreateParams.cs
- DataGridViewSelectedRowCollection.cs
- ProbeDuplex11AsyncResult.cs
- SimpleMailWebEventProvider.cs
- DbConnectionStringCommon.cs
- DateTime.cs
- EventEntry.cs
- TextContainerChangedEventArgs.cs
- HtmlTableRowCollection.cs
- InvalidDataException.cs
- DiscoveryDocumentSerializer.cs
- UidPropertyAttribute.cs
- BufferedMessageWriter.cs
- SerialStream.cs
- NetDispatcherFaultException.cs
- NominalTypeEliminator.cs
- SystemInfo.cs
- Sql8ExpressionRewriter.cs
- HttpRuntime.cs
- SQLMoneyStorage.cs
- ColorInterpolationModeValidation.cs
- Rect3D.cs
- SettingsPropertyIsReadOnlyException.cs
- StateMachineSubscription.cs
- QilParameter.cs
- SqlDataSourceCommandEventArgs.cs
- ByteKeyFrameCollection.cs
- TypeSystem.cs
- SQLInt64Storage.cs
- ListBase.cs
- InputMethod.cs
- errorpatternmatcher.cs
- SimpleTextLine.cs
- CompensationHandlingFilter.cs
- DbBuffer.cs
- SynchronizedDispatch.cs
- LocalBuilder.cs
- IdentityHolder.cs
- OleDbMetaDataFactory.cs
- SymbolMethod.cs
- WorkItem.cs
- ListDataHelper.cs
- StreamWriter.cs
- ScrollPattern.cs
- XmlSchemaAttributeGroup.cs
- ColumnMapProcessor.cs
- AppearanceEditorPart.cs
- BitmapFrameDecode.cs
- SRef.cs
- ClientTargetCollection.cs
- RectKeyFrameCollection.cs