Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / security / system / security / cryptography / Oid.cs / 1 / Oid.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // Oid.cs // namespace System.Security.Cryptography { using System.Collections; using System.Security.Cryptography.X509Certificates; internal enum OidGroup { AllGroups = 0, HashAlgorithm = 1, EncryptionAlgorithm = 2, PublicKeyAlgorithm = 3, SignatureAlgorithm = 4, Attribute = 5, ExtensionOrAttribute = 6, EnhancedKeyUsage = 7, Policy = 8, Template = 9 } public sealed class Oid { private string m_value = null; private string m_friendlyName = null; private OidGroup m_group = OidGroup.AllGroups; public Oid() {} public Oid(string oid) : this(oid, OidGroup.AllGroups, true) { } internal Oid(string oid, OidGroup group, bool lookupFriendlyName) { if (lookupFriendlyName) { // If we were passed the friendly name, retrieve the value string. string oidValue = X509Utils.FindOidInfo(CAPI.CRYPT_OID_INFO_NAME_KEY, oid, group); if (oidValue == null) oidValue = oid; this.Value = oidValue; } else { this.Value = oid; } m_group = group; } public Oid(string value, string friendlyName) { m_value = value; m_friendlyName = friendlyName; } public Oid(Oid oid) { if (oid == null) throw new ArgumentNullException("oid"); m_value = oid.m_value; m_friendlyName = oid.m_friendlyName; m_group = oid.m_group; } public string Value { get { return m_value; } set { m_value = value; } } public string FriendlyName { get { if(m_friendlyName == null && m_value != null) m_friendlyName = X509Utils.FindOidInfo(CAPI.CRYPT_OID_INFO_OID_KEY, m_value, m_group); return m_friendlyName; } set { m_friendlyName = value; // If we can find the matching OID value, then update it as well if (m_friendlyName != null) { // If FindOidInfo fails, we return a null string string oidValue = X509Utils.FindOidInfo(CAPI.CRYPT_OID_INFO_NAME_KEY, m_friendlyName, m_group); if (oidValue != null) m_value = oidValue; } } } } public sealed class OidCollection : ICollection { private ArrayList m_list; public OidCollection() { m_list = new ArrayList(); } public int Add(Oid oid) { return m_list.Add(oid); } public Oid this[int index] { get { return m_list[index] as Oid; } } // Indexer using an OID friendly name or value. public Oid this[string oid] { get { // If we were passed the friendly name, retrieve the value string. string oidValue = X509Utils.FindOidInfo(CAPI.CRYPT_OID_INFO_NAME_KEY, oid, OidGroup.AllGroups); if (oidValue == null) oidValue = oid; foreach (Oid entry in m_list) { if (entry.Value == oidValue) return entry; } return null; } } public int Count { get { return m_list.Count; } } public OidEnumerator GetEnumerator() { return new OidEnumerator(this); } ///IEnumerator IEnumerable.GetEnumerator() { return new OidEnumerator(this); } /// void ICollection.CopyTo(Array array, int index) { if (array == null) throw new ArgumentNullException("array"); if (array.Rank != 1) throw new ArgumentException(SR.GetString(SR.Arg_RankMultiDimNotSupported)); if (index < 0 || index >= array.Length) throw new ArgumentOutOfRangeException("index", SR.GetString(SR.ArgumentOutOfRange_Index)); if (index + this.Count > array.Length) throw new ArgumentException(SR.GetString(SR.Argument_InvalidOffLen)); for (int i=0; i < this.Count; i++) { array.SetValue(this[i], index); index++; } } public void CopyTo(Oid[] array, int index) { ((ICollection)this).CopyTo(array, index); } public bool IsSynchronized { get { return false; } } public Object SyncRoot { get { return this; } } } public sealed class OidEnumerator : IEnumerator { private OidCollection m_oids; private int m_current; private OidEnumerator() {} internal OidEnumerator(OidCollection oids) { m_oids = oids; m_current = -1; } public Oid Current { get { return m_oids[m_current]; } } /// Object IEnumerator.Current { get { return (Object) m_oids[m_current]; } } public bool MoveNext() { if (m_current == ((int) m_oids.Count - 1)) return false; m_current++; return true; } public void Reset() { m_current = -1; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // Oid.cs // namespace System.Security.Cryptography { using System.Collections; using System.Security.Cryptography.X509Certificates; internal enum OidGroup { AllGroups = 0, HashAlgorithm = 1, EncryptionAlgorithm = 2, PublicKeyAlgorithm = 3, SignatureAlgorithm = 4, Attribute = 5, ExtensionOrAttribute = 6, EnhancedKeyUsage = 7, Policy = 8, Template = 9 } public sealed class Oid { private string m_value = null; private string m_friendlyName = null; private OidGroup m_group = OidGroup.AllGroups; public Oid() {} public Oid(string oid) : this(oid, OidGroup.AllGroups, true) { } internal Oid(string oid, OidGroup group, bool lookupFriendlyName) { if (lookupFriendlyName) { // If we were passed the friendly name, retrieve the value string. string oidValue = X509Utils.FindOidInfo(CAPI.CRYPT_OID_INFO_NAME_KEY, oid, group); if (oidValue == null) oidValue = oid; this.Value = oidValue; } else { this.Value = oid; } m_group = group; } public Oid(string value, string friendlyName) { m_value = value; m_friendlyName = friendlyName; } public Oid(Oid oid) { if (oid == null) throw new ArgumentNullException("oid"); m_value = oid.m_value; m_friendlyName = oid.m_friendlyName; m_group = oid.m_group; } public string Value { get { return m_value; } set { m_value = value; } } public string FriendlyName { get { if(m_friendlyName == null && m_value != null) m_friendlyName = X509Utils.FindOidInfo(CAPI.CRYPT_OID_INFO_OID_KEY, m_value, m_group); return m_friendlyName; } set { m_friendlyName = value; // If we can find the matching OID value, then update it as well if (m_friendlyName != null) { // If FindOidInfo fails, we return a null string string oidValue = X509Utils.FindOidInfo(CAPI.CRYPT_OID_INFO_NAME_KEY, m_friendlyName, m_group); if (oidValue != null) m_value = oidValue; } } } } public sealed class OidCollection : ICollection { private ArrayList m_list; public OidCollection() { m_list = new ArrayList(); } public int Add(Oid oid) { return m_list.Add(oid); } public Oid this[int index] { get { return m_list[index] as Oid; } } // Indexer using an OID friendly name or value. public Oid this[string oid] { get { // If we were passed the friendly name, retrieve the value string. string oidValue = X509Utils.FindOidInfo(CAPI.CRYPT_OID_INFO_NAME_KEY, oid, OidGroup.AllGroups); if (oidValue == null) oidValue = oid; foreach (Oid entry in m_list) { if (entry.Value == oidValue) return entry; } return null; } } public int Count { get { return m_list.Count; } } public OidEnumerator GetEnumerator() { return new OidEnumerator(this); } /// IEnumerator IEnumerable.GetEnumerator() { return new OidEnumerator(this); } /// void ICollection.CopyTo(Array array, int index) { if (array == null) throw new ArgumentNullException("array"); if (array.Rank != 1) throw new ArgumentException(SR.GetString(SR.Arg_RankMultiDimNotSupported)); if (index < 0 || index >= array.Length) throw new ArgumentOutOfRangeException("index", SR.GetString(SR.ArgumentOutOfRange_Index)); if (index + this.Count > array.Length) throw new ArgumentException(SR.GetString(SR.Argument_InvalidOffLen)); for (int i=0; i < this.Count; i++) { array.SetValue(this[i], index); index++; } } public void CopyTo(Oid[] array, int index) { ((ICollection)this).CopyTo(array, index); } public bool IsSynchronized { get { return false; } } public Object SyncRoot { get { return this; } } } public sealed class OidEnumerator : IEnumerator { private OidCollection m_oids; private int m_current; private OidEnumerator() {} internal OidEnumerator(OidCollection oids) { m_oids = oids; m_current = -1; } public Oid Current { get { return m_oids[m_current]; } } /// Object IEnumerator.Current { get { return (Object) m_oids[m_current]; } } public bool MoveNext() { if (m_current == ((int) m_oids.Count - 1)) return false; m_current++; return true; } public void Reset() { m_current = -1; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
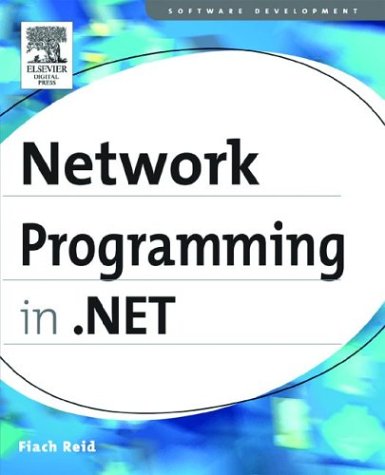
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlConnectionFactory.cs
- WebPartCloseVerb.cs
- ApplicationContext.cs
- UriSection.cs
- ObjectQueryState.cs
- MultiViewDesigner.cs
- EqualityComparer.cs
- PreApplicationStartMethodAttribute.cs
- PointValueSerializer.cs
- SettingsSection.cs
- ByteStorage.cs
- RemoteX509AsymmetricSecurityKey.cs
- RadioButtonRenderer.cs
- DataBoundControlHelper.cs
- State.cs
- ConfigurationSectionGroup.cs
- BamlResourceContent.cs
- FontFamilyIdentifier.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- UserUseLicenseDictionaryLoader.cs
- StackSpiller.Temps.cs
- CommandID.cs
- addressfiltermode.cs
- EditModeSwitchButton.cs
- DataColumnMappingCollection.cs
- DataContractSet.cs
- ElementHost.cs
- ThousandthOfEmRealPoints.cs
- PropertyMapper.cs
- ElementProxy.cs
- ControlParser.cs
- XmlDeclaration.cs
- WebPartConnectionsConfigureVerb.cs
- PropertyCollection.cs
- InfoCardRSACryptoProvider.cs
- Brush.cs
- OperationContextScope.cs
- QilScopedVisitor.cs
- BCLDebug.cs
- DependentList.cs
- TagNameToTypeMapper.cs
- ContentPlaceHolder.cs
- MasterPageParser.cs
- ListSortDescriptionCollection.cs
- SecurityStandardsManager.cs
- ViewManagerAttribute.cs
- GridViewColumnHeader.cs
- PublisherIdentityPermission.cs
- TraceListeners.cs
- StandardOleMarshalObject.cs
- ServiceMoniker.cs
- CodeDOMUtility.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- XmlSchemaObjectTable.cs
- CodeChecksumPragma.cs
- EmptyCollection.cs
- RuleInfoComparer.cs
- Activator.cs
- FormViewDeletedEventArgs.cs
- WebPartActionVerb.cs
- XmlDictionaryReaderQuotas.cs
- Type.cs
- TableRow.cs
- DataGridViewComboBoxEditingControl.cs
- Stack.cs
- Vector3D.cs
- LogRestartAreaEnumerator.cs
- WrappedReader.cs
- ProcessModelInfo.cs
- SafeNativeMemoryHandle.cs
- DragDropManager.cs
- MessageEventSubscriptionService.cs
- SnapLine.cs
- EditBehavior.cs
- ReflectionTypeLoadException.cs
- BrowserInteropHelper.cs
- ObjectFullSpanRewriter.cs
- PrintPreviewControl.cs
- Request.cs
- ListView.cs
- RefExpr.cs
- DataTableReaderListener.cs
- DynamicScriptObject.cs
- XhtmlBasicLiteralTextAdapter.cs
- ObjectAssociationEndMapping.cs
- TextEditorSelection.cs
- ViewValidator.cs
- cryptoapiTransform.cs
- NumberFormatter.cs
- DragDeltaEventArgs.cs
- Geometry3D.cs
- Compress.cs
- AdornerPresentationContext.cs
- CultureTableRecord.cs
- CriticalHandle.cs
- ScriptDescriptor.cs
- PipelineModuleStepContainer.cs
- FormViewInsertEventArgs.cs
- X509ClientCertificateAuthentication.cs
- StatusBarItem.cs