Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Animation / RepeatBehaviorConverter.cs / 1 / RepeatBehaviorConverter.cs
//------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2004 // // File: RepeatBehaviorConverter.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; namespace System.Windows.Media.Animation { ////// /// public sealed class RepeatBehaviorConverter : TypeConverter { #region Data private static char[] _iterationCharacter = new char[] { 'x' }; #endregion ////// CanConvertFrom - Returns whether or not this class can convert from a given type /// ///public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } /// /// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible ///public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ( destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } /// /// ConvertFrom /// ///public override object ConvertFrom( ITypeDescriptorContext td, CultureInfo cultureInfo, object value) { string stringValue = value as string; if (stringValue != null) { stringValue = stringValue.Trim(); if (stringValue == "Forever") { return RepeatBehavior.Forever; } else if ( stringValue.Length > 0 && stringValue[stringValue.Length - 1] == _iterationCharacter[0]) { string stringDoubleValue = stringValue.TrimEnd(_iterationCharacter); double doubleValue = (double)TypeDescriptor.GetConverter(typeof(double)).ConvertFrom(td, cultureInfo, stringDoubleValue); return new RepeatBehavior(doubleValue); } } // The value is not Forever or an iteration count so it's either a TimeSpan // or we'll let the TimeSpanConverter raise the appropriate exception. TimeSpan timeSpanValue = (TimeSpan)TypeDescriptor.GetConverter(typeof(TimeSpan)).ConvertFrom(td, cultureInfo, stringValue); return new RepeatBehavior(timeSpanValue); } /// /// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// /// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for RepeatBehavior, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext context, CultureInfo cultureInfo, object value, Type destinationType) { if ( value is RepeatBehavior && destinationType != null) { RepeatBehavior repeatBehavior = (RepeatBehavior)value; if (destinationType == typeof(InstanceDescriptor)) { MemberInfo mi; if (repeatBehavior == RepeatBehavior.Forever) { mi = typeof(RepeatBehavior).GetProperty("Forever"); return new InstanceDescriptor(mi, null); } else if (repeatBehavior.HasCount) { mi = typeof(RepeatBehavior).GetConstructor(new Type[] { typeof(double) }); return new InstanceDescriptor(mi, new object[] { repeatBehavior.Count }); } else if (repeatBehavior.HasDuration) { mi = typeof(RepeatBehavior).GetConstructor(new Type[] { typeof(TimeSpan) }); return new InstanceDescriptor(mi, new object[] { repeatBehavior.Duration }); } else { Debug.Fail("Unknown type of RepeatBehavior passed to RepeatBehaviorConverter."); } } else if (destinationType == typeof(string)) { return repeatBehavior.InternalToString(null, cultureInfo); } } // We can't do the conversion, let the base class raise the // appropriate exception. return base.ConvertTo(context, cultureInfo, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2004 // // File: RepeatBehaviorConverter.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; namespace System.Windows.Media.Animation { ////// /// public sealed class RepeatBehaviorConverter : TypeConverter { #region Data private static char[] _iterationCharacter = new char[] { 'x' }; #endregion ////// CanConvertFrom - Returns whether or not this class can convert from a given type /// ///public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } /// /// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible ///public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ( destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } /// /// ConvertFrom /// ///public override object ConvertFrom( ITypeDescriptorContext td, CultureInfo cultureInfo, object value) { string stringValue = value as string; if (stringValue != null) { stringValue = stringValue.Trim(); if (stringValue == "Forever") { return RepeatBehavior.Forever; } else if ( stringValue.Length > 0 && stringValue[stringValue.Length - 1] == _iterationCharacter[0]) { string stringDoubleValue = stringValue.TrimEnd(_iterationCharacter); double doubleValue = (double)TypeDescriptor.GetConverter(typeof(double)).ConvertFrom(td, cultureInfo, stringDoubleValue); return new RepeatBehavior(doubleValue); } } // The value is not Forever or an iteration count so it's either a TimeSpan // or we'll let the TimeSpanConverter raise the appropriate exception. TimeSpan timeSpanValue = (TimeSpan)TypeDescriptor.GetConverter(typeof(TimeSpan)).ConvertFrom(td, cultureInfo, stringValue); return new RepeatBehavior(timeSpanValue); } /// /// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// /// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for RepeatBehavior, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext context, CultureInfo cultureInfo, object value, Type destinationType) { if ( value is RepeatBehavior && destinationType != null) { RepeatBehavior repeatBehavior = (RepeatBehavior)value; if (destinationType == typeof(InstanceDescriptor)) { MemberInfo mi; if (repeatBehavior == RepeatBehavior.Forever) { mi = typeof(RepeatBehavior).GetProperty("Forever"); return new InstanceDescriptor(mi, null); } else if (repeatBehavior.HasCount) { mi = typeof(RepeatBehavior).GetConstructor(new Type[] { typeof(double) }); return new InstanceDescriptor(mi, new object[] { repeatBehavior.Count }); } else if (repeatBehavior.HasDuration) { mi = typeof(RepeatBehavior).GetConstructor(new Type[] { typeof(TimeSpan) }); return new InstanceDescriptor(mi, new object[] { repeatBehavior.Duration }); } else { Debug.Fail("Unknown type of RepeatBehavior passed to RepeatBehaviorConverter."); } } else if (destinationType == typeof(string)) { return repeatBehavior.InternalToString(null, cultureInfo); } } // We can't do the conversion, let the base class raise the // appropriate exception. return base.ConvertTo(context, cultureInfo, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
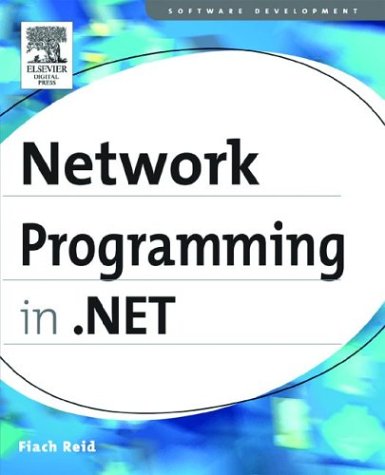
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UndoManager.cs
- DetailsViewRow.cs
- Buffer.cs
- ForeignKeyFactory.cs
- TypefaceMap.cs
- RecordBuilder.cs
- PathFigureCollection.cs
- TimerExtension.cs
- smtppermission.cs
- VectorCollection.cs
- TextEmbeddedObject.cs
- GuidelineSet.cs
- WasEndpointConfigContainer.cs
- SystemException.cs
- RequestChannel.cs
- PreservationFileWriter.cs
- StorageMappingItemCollection.cs
- DocumentGridContextMenu.cs
- QuerySetOp.cs
- OdbcConnectionHandle.cs
- DefaultAssemblyResolver.cs
- DetailsViewInsertedEventArgs.cs
- QuaternionRotation3D.cs
- Style.cs
- UserPreferenceChangingEventArgs.cs
- Int32KeyFrameCollection.cs
- HostProtectionException.cs
- HMACMD5.cs
- Transform.cs
- ButtonPopupAdapter.cs
- BuildProvider.cs
- UIElementParagraph.cs
- WindowsGraphics.cs
- UpdateManifestForBrowserApplication.cs
- CompilerParameters.cs
- View.cs
- SessionPageStatePersister.cs
- WorkflowInstanceExtensionCollection.cs
- TreeView.cs
- PageSettings.cs
- RectangleGeometry.cs
- SoapSchemaMember.cs
- Byte.cs
- InkPresenterAutomationPeer.cs
- LogEntryHeaderSerializer.cs
- ActivityInstance.cs
- OdbcConnectionFactory.cs
- PackWebRequest.cs
- _AutoWebProxyScriptEngine.cs
- IChannel.cs
- TextContainer.cs
- BindingCompleteEventArgs.cs
- DefaultAssemblyResolver.cs
- DetailsViewModeEventArgs.cs
- TreeWalker.cs
- coordinatorfactory.cs
- ObjectDataSourceStatusEventArgs.cs
- RelationshipManager.cs
- DelegatingHeader.cs
- InstanceDataCollectionCollection.cs
- SoapEnumAttribute.cs
- HttpListenerContext.cs
- PropertyEmitterBase.cs
- SerialPinChanges.cs
- TextServicesContext.cs
- WebControlAdapter.cs
- WindowsTreeView.cs
- TimerElapsedEvenArgs.cs
- PictureBox.cs
- SByteStorage.cs
- ThemeableAttribute.cs
- FontCollection.cs
- CompareValidator.cs
- AdCreatedEventArgs.cs
- PropertyValueChangedEvent.cs
- InputElement.cs
- LinqDataSourceContextData.cs
- AutoGeneratedField.cs
- ConfigurationSchemaErrors.cs
- InProcStateClientManager.cs
- ReceiveContext.cs
- AppSettingsReader.cs
- DataGridTableCollection.cs
- DataGridViewCellLinkedList.cs
- RemoteWebConfigurationHostStream.cs
- ProfileProvider.cs
- SolidColorBrush.cs
- RepeatInfo.cs
- PixelFormats.cs
- PackagePartCollection.cs
- ConfigXmlDocument.cs
- AspCompat.cs
- MouseOverProperty.cs
- ThreadStartException.cs
- XmlSchemaObject.cs
- PeerNameRecordCollection.cs
- StretchValidation.cs
- ConsumerConnectionPoint.cs
- UtilityExtension.cs
- XmlHelper.cs