Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / clr / src / BCL / System / Security / Policy / URLMembershipCondition.cs / 2 / URLMembershipCondition.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // UrlMembershipCondition.cs // // Implementation of membership condition for urls // namespace System.Security.Policy { using System; using System.Collections; using System.Globalization; using System.Security; using System.Security.Util; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class UrlMembershipCondition : IMembershipCondition, IConstantMembershipCondition, IReportMatchMembershipCondition { //------------------------------------------------------ // // PRIVATE STATE DATA // //----------------------------------------------------- private URLString m_url; private SecurityElement m_element; //----------------------------------------------------- // // PUBLIC CONSTRUCTORS // //----------------------------------------------------- internal UrlMembershipCondition() { m_url = null; } public UrlMembershipCondition( String url ) { if (url == null) throw new ArgumentNullException( "url" ); // Parse the Url to m_url = new URLString(url, false /* not parsed */, true /* parse eagerly */); } //------------------------------------------------------ // // PUBLIC ACCESSOR METHODS // //----------------------------------------------------- public String Url { set { if (value == null) throw new ArgumentNullException("value"); m_url = new URLString( value ); } get { if (m_url == null && m_element != null) ParseURL(); return m_url.ToString(); } } //------------------------------------------------------ // // IMEMBERSHIPCONDITION IMPLEMENTATION // //------------------------------------------------------ public bool Check( Evidence evidence ) { object usedEvidence = null; return (this as IReportMatchMembershipCondition).Check(evidence, out usedEvidence); } bool IReportMatchMembershipCondition.Check(Evidence evidence, out object usedEvidence) { usedEvidence = null; if (evidence == null) return false; IEnumerator enumerator = evidence.GetHostEnumerator(); while (enumerator.MoveNext()) { if (enumerator.Current is Url) { if (m_url == null && m_element != null) ParseURL(); if (((Url)enumerator.Current).GetURLString().IsSubsetOf( m_url )) { usedEvidence = enumerator.Current; return true; } } } return false; } public IMembershipCondition Copy() { if (m_url == null && m_element != null) ParseURL(); UrlMembershipCondition mc = new UrlMembershipCondition(); mc.m_url = new URLString( m_url.ToString() ); return mc; } public SecurityElement ToXml() { return ToXml( null ); } public void FromXml( SecurityElement e ) { FromXml( e, null ); } public SecurityElement ToXml( PolicyLevel level ) { if (m_url == null && m_element != null) ParseURL(); SecurityElement root = new SecurityElement( "IMembershipCondition" ); System.Security.Util.XMLUtil.AddClassAttribute( root, this.GetType(), "System.Security.Policy.UrlMembershipCondition" ); // If you hit this assertt then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assertt below. BCLDebug.Assert( this.GetType().FullName.Equals( "System.Security.Policy.UrlMembershipCondition" ), "Class name changed!" ); root.AddAttribute( "version", "1" ); if (m_url != null) root.AddAttribute( "Url", m_url.ToString() ); return root; } public void FromXml( SecurityElement e, PolicyLevel level ) { if (e == null) throw new ArgumentNullException("e"); if (!e.Tag.Equals( "IMembershipCondition" )) { throw new ArgumentException( Environment.GetResourceString( "Argument_MembershipConditionElement" ) ); } lock (this) { m_element = e; m_url = null; } } private void ParseURL() { lock (this) { if (m_element == null) return; String elurl = m_element.Attribute( "Url" ); if (elurl == null) throw new ArgumentException( Environment.GetResourceString( "Argument_UrlCannotBeNull" ) ); else m_url = new URLString( elurl ); m_element = null; } } public override bool Equals( Object o ) { UrlMembershipCondition that = (o as UrlMembershipCondition); if (that != null) { if (this.m_url == null && this.m_element != null) this.ParseURL(); if (that.m_url == null && that.m_element != null) that.ParseURL(); if (Equals( this.m_url, that.m_url )) { return true; } } return false; } public override int GetHashCode() { if (m_url == null && m_element != null) ParseURL(); if (m_url != null) { return m_url.GetHashCode(); } else { return typeof( UrlMembershipCondition ).GetHashCode(); } } public override String ToString() { if (m_url == null && m_element != null) ParseURL(); if (m_url != null) return String.Format( CultureInfo.CurrentCulture, Environment.GetResourceString( "Url_ToStringArg" ), m_url.ToString() ); else return Environment.GetResourceString( "Url_ToString" ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
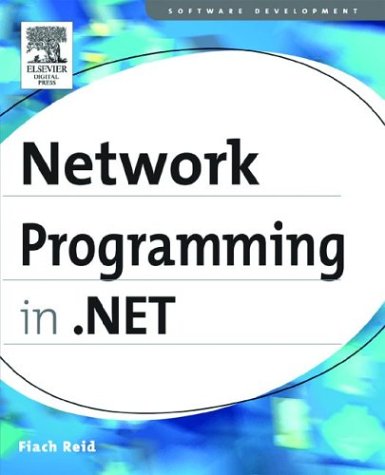
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ArgumentValidation.cs
- Label.cs
- XmlText.cs
- StateBag.cs
- ImageMap.cs
- InstallerTypeAttribute.cs
- MergeLocalizationDirectives.cs
- ApplyHostConfigurationBehavior.cs
- HashCodeCombiner.cs
- SiteMapNodeItem.cs
- OleAutBinder.cs
- DrawToolTipEventArgs.cs
- XmlAttributeCollection.cs
- PropertySegmentSerializationProvider.cs
- Calendar.cs
- InkPresenterAutomationPeer.cs
- CuspData.cs
- PointAnimationUsingKeyFrames.cs
- ApplicationServicesHostFactory.cs
- DateTimeFormat.cs
- ConnectionPoint.cs
- FixedSOMTableRow.cs
- Visitor.cs
- FileReader.cs
- StackSpiller.Bindings.cs
- DetailsView.cs
- CellConstant.cs
- SqlFacetAttribute.cs
- QilInvoke.cs
- WebPartChrome.cs
- DependencyPropertyDescriptor.cs
- AddingNewEventArgs.cs
- XsdDuration.cs
- SystemIPGlobalProperties.cs
- Axis.cs
- SerializableTypeCodeDomSerializer.cs
- SRGSCompiler.cs
- Int16KeyFrameCollection.cs
- MaskedTextProvider.cs
- XNodeValidator.cs
- GridEntry.cs
- CompositionTarget.cs
- HostingEnvironmentSection.cs
- DataListCommandEventArgs.cs
- SubclassTypeValidator.cs
- LateBoundBitmapDecoder.cs
- safemediahandle.cs
- WinEventQueueItem.cs
- RenderDataDrawingContext.cs
- ListParagraph.cs
- BrowsableAttribute.cs
- Image.cs
- LocalValueEnumerator.cs
- ECDiffieHellman.cs
- HostedTcpTransportManager.cs
- MDIControlStrip.cs
- ControlParameter.cs
- Win32SafeHandles.cs
- Separator.cs
- Validator.cs
- GPRECTF.cs
- BinaryCommonClasses.cs
- PermissionSetEnumerator.cs
- Stopwatch.cs
- BindingBase.cs
- OlePropertyStructs.cs
- XPathParser.cs
- Logging.cs
- XmlQueryTypeFactory.cs
- QilTernary.cs
- BuildProviderCollection.cs
- DetailsViewDeleteEventArgs.cs
- DoWorkEventArgs.cs
- PropertyEntry.cs
- AsyncOperationManager.cs
- PersonalizablePropertyEntry.cs
- shaperfactoryquerycacheentry.cs
- WebBrowserContainer.cs
- FormViewCommandEventArgs.cs
- BindingCompleteEventArgs.cs
- State.cs
- CommandID.cs
- SqlGenericUtil.cs
- XmlValidatingReaderImpl.cs
- ExpressionParser.cs
- Debug.cs
- errorpatternmatcher.cs
- UpdateRecord.cs
- VisualStateManager.cs
- _NTAuthentication.cs
- DynamicMethod.cs
- DrawingBrush.cs
- WebPartUtil.cs
- CodeVariableDeclarationStatement.cs
- GraphicsPath.cs
- NavigationService.cs
- CompareValidator.cs
- SafeHandles.cs
- SweepDirectionValidation.cs
- pingexception.cs