Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / ComponentModel / COM2Interop / COM2EnumConverter.cs / 1 / COM2EnumConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.ComponentModel.Com2Interop { using System.Diagnostics; using System; using System.ComponentModel; using System.Drawing; using System.Collections; using Microsoft.Win32; using System.Globalization; internal class Com2EnumConverter : TypeConverter { internal readonly Com2Enum com2Enum; private StandardValuesCollection values; public Com2EnumConverter(Com2Enum enumObj) { com2Enum = enumObj; } ////// /// Determines if this converter can convert an object in the given source /// type to the native type of the converter. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } public override bool CanConvertTo(ITypeDescriptorContext context, Type destType) { if (base.CanConvertTo(context, destType)) { return true; } return destType.IsEnum; } ////// /// Converts the given object to the converter's native type. /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is string) { return com2Enum.FromString((string)value); } return base.ConvertFrom(context, culture, value); } ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(string)) { if (value != null) { string str = com2Enum.ToString(value); return (str == null ? "" : str); } } if (destinationType.IsEnum) { return Enum.ToObject(destinationType, value); } return base.ConvertTo(context, culture, value, destinationType); } ////// /// Retrieves a collection containing a set of standard values /// for the data type this validator is designed for. This /// will return null if the data type does not support a /// standard set of values. /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (values == null) { object[] objValues = com2Enum.Values; if (objValues != null) { values = new StandardValuesCollection(objValues); } } return values; } ////// /// Determines if the list of standard values returned from /// GetStandardValues is an exclusive list. If the list /// is exclusive, then no other values are valid, such as /// in an enum data type. If the list is not exclusive, /// then there are other valid values besides the list of /// standard values GetStandardValues provides. /// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return com2Enum.IsStrictEnum; } ////// /// Determines if this object supports a standard set of values /// that can be picked from a list. /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } ////// /// Determines if the given object value is valid for this type. /// public override bool IsValid(ITypeDescriptorContext context, object value) { string strValue = com2Enum.ToString(value); return strValue != null && strValue.Length > 0; } public void RefreshValues() { this.values = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
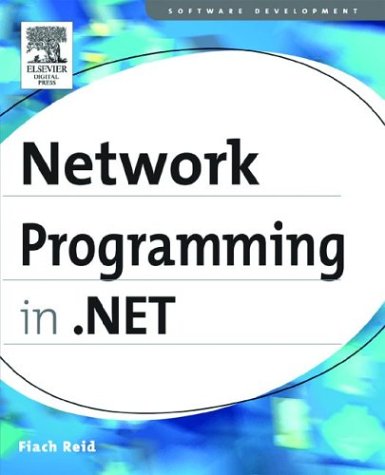
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ParserOptions.cs
- HtmlInputSubmit.cs
- Vector3DAnimationBase.cs
- ReadOnlyMetadataCollection.cs
- ServicePointManagerElement.cs
- ResolvedKeyFrameEntry.cs
- AncestorChangedEventArgs.cs
- XPathSelfQuery.cs
- ComPlusAuthorization.cs
- DashStyle.cs
- OlePropertyStructs.cs
- PingReply.cs
- Misc.cs
- FormViewRow.cs
- ProcessDesigner.cs
- ThicknessKeyFrameCollection.cs
- OdbcUtils.cs
- HandleScope.cs
- SqlProvider.cs
- Section.cs
- EdgeModeValidation.cs
- ServiceBuildProvider.cs
- DataGridView.cs
- SqlNotificationEventArgs.cs
- Table.cs
- BindingGroup.cs
- Compensate.cs
- EditorAttribute.cs
- CommonDialog.cs
- NonParentingControl.cs
- ScriptingWebServicesSectionGroup.cs
- HashMembershipCondition.cs
- Point.cs
- XmlCompatibilityReader.cs
- SystemFonts.cs
- ReceiveParametersContent.cs
- ApplicationDirectoryMembershipCondition.cs
- VariableQuery.cs
- BitmapPalettes.cs
- OleDbSchemaGuid.cs
- ToolStripDropDownClosingEventArgs.cs
- Matrix.cs
- Collection.cs
- SecurityIdentifierConverter.cs
- EntitySqlQueryBuilder.cs
- basecomparevalidator.cs
- DynamicILGenerator.cs
- Profiler.cs
- Point3DAnimation.cs
- XDRSchema.cs
- ViewStateException.cs
- X509Utils.cs
- OLEDB_Enum.cs
- XdrBuilder.cs
- LongValidator.cs
- webeventbuffer.cs
- ServicePerformanceCounters.cs
- TaskFormBase.cs
- EntityCommand.cs
- SymbolEqualComparer.cs
- Thread.cs
- OpCopier.cs
- ColumnBinding.cs
- FillErrorEventArgs.cs
- ScriptResourceInfo.cs
- ObjectViewEntityCollectionData.cs
- MatchingStyle.cs
- UDPClient.cs
- CachedRequestParams.cs
- securestring.cs
- XamlDesignerSerializationManager.cs
- CodeIndexerExpression.cs
- XmlConvert.cs
- RuntimeConfigLKG.cs
- CatalogPartCollection.cs
- ResXBuildProvider.cs
- NativeMethods.cs
- SqlStream.cs
- SeverityFilter.cs
- SQLBytesStorage.cs
- BuildProviderAppliesToAttribute.cs
- SafeThemeHandle.cs
- MouseEventArgs.cs
- StringUtil.cs
- XmlSchemaSearchPattern.cs
- EncoderBestFitFallback.cs
- CharEnumerator.cs
- AnimatedTypeHelpers.cs
- CorrelationScope.cs
- StateItem.cs
- ListBoxItem.cs
- Console.cs
- HttpHeaderCollection.cs
- QueryOutputWriter.cs
- OrderedHashRepartitionStream.cs
- RouteParameter.cs
- IPAddress.cs
- RefreshPropertiesAttribute.cs
- TextContainer.cs
- SmtpMail.cs