Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Sys / System / Configuration / SettingsPropertyValueCollection.cs / 1 / SettingsPropertyValueCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Specialized; using System.Runtime.Serialization; using System.Configuration.Provider; using System.Globalization; using System.IO; using System.Runtime.Serialization.Formatters.Binary; using System.Xml.Serialization; using System.ComponentModel; using System.Security.Permissions; using System.Reflection; ////////////////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////////////////// public class SettingsPropertyValueCollection : IEnumerable, ICloneable, ICollection { private Hashtable _Indices = null; private ArrayList _Values = null; private bool _ReadOnly = false; //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public SettingsPropertyValueCollection() { _Indices = new Hashtable(10, CaseInsensitiveHashCodeProvider.Default, CaseInsensitiveComparer.Default); _Values = new ArrayList(); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Add(SettingsPropertyValue property) { if (_ReadOnly) throw new NotSupportedException(); int pos = _Values.Add(property); try { _Indices.Add(property.Name, pos); } catch (Exception) { _Values.RemoveAt(pos); throw; } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Remove(string name) { if (_ReadOnly) throw new NotSupportedException(); object pos = _Indices[name]; if (pos == null || !(pos is int)) return; int ipos = (int)pos; if (ipos >= _Values.Count) return; _Values.RemoveAt(ipos); _Indices.Remove(name); ArrayList al = new ArrayList(); foreach (DictionaryEntry de in _Indices) if ((int)de.Value > ipos) al.Add(de.Key); foreach (string key in al) _Indices[key] = ((int)_Indices[key]) - 1; } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public SettingsPropertyValue this[string name] { get { object pos = _Indices[name]; if (pos == null || !(pos is int)) return null; int ipos = (int)pos; if (ipos >= _Values.Count) return null; return (SettingsPropertyValue)_Values[ipos]; } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public IEnumerator GetEnumerator() { return _Values.GetEnumerator(); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public object Clone() { return new SettingsPropertyValueCollection(_Indices, _Values); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void SetReadOnly() { if (_ReadOnly) return; _ReadOnly = true; _Values = ArrayList.ReadOnly(_Values); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Clear() { _Values.Clear(); _Indices.Clear(); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// // ICollection interface public int Count { get { return _Values.Count; } } public bool IsSynchronized { get { return false; } } public object SyncRoot { get { return this; } } public void CopyTo(Array array, int index) { _Values.CopyTo(array, index); } ///////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////// private SettingsPropertyValueCollection(Hashtable indices, ArrayList values) { _Indices = (Hashtable)indices.Clone(); _Values = (ArrayList)values.Clone(); } } }
Link Menu
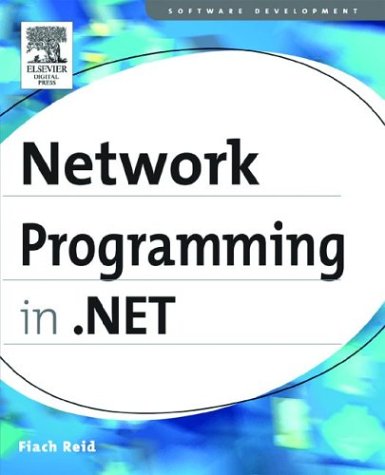
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlTableRow.cs
- ISessionStateStore.cs
- ButtonFlatAdapter.cs
- BitVector32.cs
- DecimalAverageAggregationOperator.cs
- VisualBasicExpressionConverter.cs
- _UriSyntax.cs
- ProcessInfo.cs
- PackUriHelper.cs
- ExceptionUtil.cs
- Membership.cs
- SqlAggregateChecker.cs
- Rect.cs
- AppSettingsReader.cs
- SchemaManager.cs
- GlobalizationAssembly.cs
- DynamicControl.cs
- AppSecurityManager.cs
- XmlSchemaInfo.cs
- TreeBuilderBamlTranslator.cs
- DataGridHeaderBorder.cs
- TableCellsCollectionEditor.cs
- UriTemplateDispatchFormatter.cs
- DataGridViewComboBoxColumn.cs
- DocobjHost.cs
- TextView.cs
- DrawItemEvent.cs
- FontNamesConverter.cs
- XmlCompatibilityReader.cs
- TypeLibConverter.cs
- WebRequestModulesSection.cs
- DesignerOptions.cs
- WebConfigurationManager.cs
- XmlExpressionDumper.cs
- SortKey.cs
- UnsafeNativeMethods.cs
- SortKey.cs
- GenericEnumerator.cs
- RetrieveVirtualItemEventArgs.cs
- BlurBitmapEffect.cs
- NestPullup.cs
- LambdaExpression.cs
- VariantWrapper.cs
- EventLogPermissionAttribute.cs
- XPathCompileException.cs
- SafeTimerHandle.cs
- NetNamedPipeSecurityElement.cs
- RegularExpressionValidator.cs
- RequestSecurityTokenResponse.cs
- PlatformNotSupportedException.cs
- HttpChannelBindingToken.cs
- SerializationEventsCache.cs
- EdmProperty.cs
- _UriTypeConverter.cs
- Polyline.cs
- StateMachineSubscription.cs
- InplaceBitmapMetadataWriter.cs
- WebPartActionVerb.cs
- DESCryptoServiceProvider.cs
- FlowLayoutPanel.cs
- BindingWorker.cs
- XmlEncodedRawTextWriter.cs
- CheckBoxAutomationPeer.cs
- ContentElement.cs
- WorkflowFileItem.cs
- XamlReaderConstants.cs
- Int32AnimationUsingKeyFrames.cs
- ArgumentException.cs
- AutomationPatternInfo.cs
- StackBuilderSink.cs
- _NtlmClient.cs
- VerificationException.cs
- AsymmetricSignatureFormatter.cs
- CheckoutException.cs
- XamlHostingSectionGroup.cs
- ButtonStandardAdapter.cs
- AnnotationAuthorChangedEventArgs.cs
- SortKey.cs
- Viewport3DVisual.cs
- DesignTimeSiteMapProvider.cs
- MetadataHelper.cs
- CachedRequestParams.cs
- TagPrefixCollection.cs
- DataRow.cs
- Propagator.JoinPropagator.SubstitutingCloneVisitor.cs
- KeyValueConfigurationElement.cs
- HttpStaticObjectsCollectionBase.cs
- FileSecurity.cs
- CustomTypeDescriptor.cs
- TransformFinalBlockRequest.cs
- CustomError.cs
- OpCodes.cs
- SafeBitVector32.cs
- MostlySingletonList.cs
- MimeWriter.cs
- DrawingGroup.cs
- APCustomTypeDescriptor.cs
- NativeMethods.cs
- XmlHelper.cs
- COM2ExtendedTypeConverter.cs