Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Markup / StaticExtension.cs / 1 / StaticExtension.cs
/****************************************************************************\ * * File: StaticExtension.cs * * Class for Xaml markup extension for static field and property references. * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Windows; using System.Windows.Input; using System.Reflection; using MS.Utility; namespace System.Windows.Markup { ////// Class for Xaml markup extension for static field and property references. /// [TypeConverter(typeof(StaticExtensionConverter))] [MarkupExtensionReturnType(typeof(object))] public class StaticExtension : MarkupExtension { ////// Constructor that takes no parameters /// public StaticExtension() { } ////// Constructor that takes the member that this is a static reference to. /// This string is of the format /// Prefix:ClassName.FieldOrPropertyName. The Prefix is /// optional, and refers to the XML prefix in a Xaml file. /// public StaticExtension( string member) { if (member == null) { throw new ArgumentNullException("member"); } _member = member; } ////// Return an object that should be set on the targetObject's targetProperty /// for this markup extension. For a StaticExtension this is a static field /// or property value. /// /// Object that can provide services for the markup extension. ////// The object to set on this property. /// public override object ProvideValue(IServiceProvider serviceProvider) { if (_member == null) { throw new InvalidOperationException(SR.Get(SRID.MarkupExtensionStaticMember)); } object value = null; Type type = MemberType; string fieldString = null; string memberFullName = null; if (type != null) { fieldString = _member; memberFullName = type.FullName + "." + _member; } else { memberFullName = _member; // Validate the _member int dotIndex = _member.IndexOf('.'); if (dotIndex < 0) { throw new ArgumentException(SR.Get(SRID.MarkupExtensionBadStatic, _member)); } // Pull out the type substring (this will include any XML prefix, e.g. "av:Button") string typeString = _member.Substring(0, dotIndex); if (typeString == string.Empty) { throw new ArgumentException(SR.Get(SRID.MarkupExtensionBadStatic, _member)); } // Get the IXamlTypeResolver from the service provider IXamlTypeResolver xamlTypeResolver = serviceProvider.GetService(typeof(IXamlTypeResolver)) as IXamlTypeResolver; if (xamlTypeResolver == null) { throw new ArgumentException(SR.Get(SRID.MarkupExtensionNoContext, GetType().Name, "IXamlTypeResolver")); } // Use the type resolver to get a Type instance type = xamlTypeResolver.Resolve(typeString); // Get the member name substring fieldString = _member.Substring(dotIndex + 1, _member.Length - dotIndex - 1); if (fieldString == string.Empty) { throw new ArgumentException(SR.Get(SRID.MarkupExtensionBadStatic, _member)); } } // Use the built-in parser for enum types if (type.IsEnum) { return Enum.Parse(type, fieldString); } // For other types, reflect bool found = false; object fieldOrProp = type.GetField(fieldString, BindingFlags.Public | BindingFlags.FlattenHierarchy | BindingFlags.Static); if (fieldOrProp == null) { fieldOrProp = type.GetProperty(fieldString, BindingFlags.Public | BindingFlags.FlattenHierarchy | BindingFlags.Static); if (fieldOrProp is PropertyInfo) { value = ((PropertyInfo)fieldOrProp).GetValue(null,null); found = true; } } else if (fieldOrProp is FieldInfo) { value = ((FieldInfo)fieldOrProp).GetValue(null); found = true; } if (found) { return value; } else { throw new ArgumentException(SR.Get(SRID.MarkupExtensionBadStatic, memberFullName)); } } ////// The static field or property represented by a string. This string is /// of the format Prefix:ClassName.FieldOrPropertyName. The Prefix is /// optional, and refers to the XML prefix in a Xaml file. /// [ConstructorArgument("member")] public string Member { get { return _member; } set { if (value == null) { throw new ArgumentNullException("value"); } _member = value; } } internal Type MemberType { get { return _memberType; } set { if (value == null) { throw new ArgumentNullException("value"); } _memberType = value; } } private string _member; private Type _memberType; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: StaticExtension.cs * * Class for Xaml markup extension for static field and property references. * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Windows; using System.Windows.Input; using System.Reflection; using MS.Utility; namespace System.Windows.Markup { ////// Class for Xaml markup extension for static field and property references. /// [TypeConverter(typeof(StaticExtensionConverter))] [MarkupExtensionReturnType(typeof(object))] public class StaticExtension : MarkupExtension { ////// Constructor that takes no parameters /// public StaticExtension() { } ////// Constructor that takes the member that this is a static reference to. /// This string is of the format /// Prefix:ClassName.FieldOrPropertyName. The Prefix is /// optional, and refers to the XML prefix in a Xaml file. /// public StaticExtension( string member) { if (member == null) { throw new ArgumentNullException("member"); } _member = member; } ////// Return an object that should be set on the targetObject's targetProperty /// for this markup extension. For a StaticExtension this is a static field /// or property value. /// /// Object that can provide services for the markup extension. ////// The object to set on this property. /// public override object ProvideValue(IServiceProvider serviceProvider) { if (_member == null) { throw new InvalidOperationException(SR.Get(SRID.MarkupExtensionStaticMember)); } object value = null; Type type = MemberType; string fieldString = null; string memberFullName = null; if (type != null) { fieldString = _member; memberFullName = type.FullName + "." + _member; } else { memberFullName = _member; // Validate the _member int dotIndex = _member.IndexOf('.'); if (dotIndex < 0) { throw new ArgumentException(SR.Get(SRID.MarkupExtensionBadStatic, _member)); } // Pull out the type substring (this will include any XML prefix, e.g. "av:Button") string typeString = _member.Substring(0, dotIndex); if (typeString == string.Empty) { throw new ArgumentException(SR.Get(SRID.MarkupExtensionBadStatic, _member)); } // Get the IXamlTypeResolver from the service provider IXamlTypeResolver xamlTypeResolver = serviceProvider.GetService(typeof(IXamlTypeResolver)) as IXamlTypeResolver; if (xamlTypeResolver == null) { throw new ArgumentException(SR.Get(SRID.MarkupExtensionNoContext, GetType().Name, "IXamlTypeResolver")); } // Use the type resolver to get a Type instance type = xamlTypeResolver.Resolve(typeString); // Get the member name substring fieldString = _member.Substring(dotIndex + 1, _member.Length - dotIndex - 1); if (fieldString == string.Empty) { throw new ArgumentException(SR.Get(SRID.MarkupExtensionBadStatic, _member)); } } // Use the built-in parser for enum types if (type.IsEnum) { return Enum.Parse(type, fieldString); } // For other types, reflect bool found = false; object fieldOrProp = type.GetField(fieldString, BindingFlags.Public | BindingFlags.FlattenHierarchy | BindingFlags.Static); if (fieldOrProp == null) { fieldOrProp = type.GetProperty(fieldString, BindingFlags.Public | BindingFlags.FlattenHierarchy | BindingFlags.Static); if (fieldOrProp is PropertyInfo) { value = ((PropertyInfo)fieldOrProp).GetValue(null,null); found = true; } } else if (fieldOrProp is FieldInfo) { value = ((FieldInfo)fieldOrProp).GetValue(null); found = true; } if (found) { return value; } else { throw new ArgumentException(SR.Get(SRID.MarkupExtensionBadStatic, memberFullName)); } } ////// The static field or property represented by a string. This string is /// of the format Prefix:ClassName.FieldOrPropertyName. The Prefix is /// optional, and refers to the XML prefix in a Xaml file. /// [ConstructorArgument("member")] public string Member { get { return _member; } set { if (value == null) { throw new ArgumentNullException("value"); } _member = value; } } internal Type MemberType { get { return _memberType; } set { if (value == null) { throw new ArgumentNullException("value"); } _memberType = value; } } private string _member; private Type _memberType; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
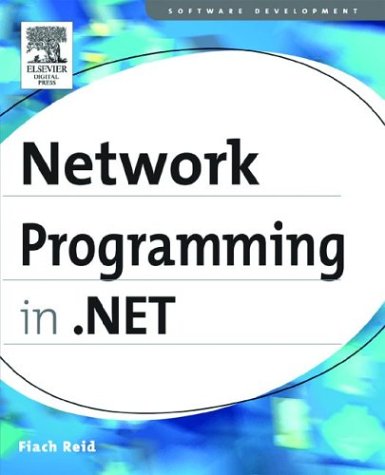
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProvidersHelper.cs
- CultureSpecificStringDictionary.cs
- BitFlagsGenerator.cs
- StatusBarPanelClickEvent.cs
- GridView.cs
- WindowCollection.cs
- CheckPair.cs
- ActivityBuilderXamlWriter.cs
- XamlContextStack.cs
- FocusChangedEventArgs.cs
- ClientSponsor.cs
- DrawingGroup.cs
- ToolStripItemGlyph.cs
- Normalization.cs
- PersonalizationEntry.cs
- StatusBarDrawItemEvent.cs
- DependentList.cs
- PeerToPeerException.cs
- Scheduler.cs
- EditorZoneBase.cs
- BidOverLoads.cs
- KeyToListMap.cs
- ProtectedConfigurationSection.cs
- DataAdapter.cs
- TimelineGroup.cs
- MetadataPropertyCollection.cs
- WebPartAuthorizationEventArgs.cs
- WrappedIUnknown.cs
- DrawingAttributeSerializer.cs
- LocalizationParserHooks.cs
- XAMLParseException.cs
- VScrollProperties.cs
- BooleanToVisibilityConverter.cs
- RelatedPropertyManager.cs
- HelpProvider.cs
- ClientData.cs
- Configuration.cs
- EnumerableRowCollection.cs
- formatter.cs
- Metafile.cs
- RTLAwareMessageBox.cs
- ScalarConstant.cs
- NodeCounter.cs
- CompilerParameters.cs
- CqlLexer.cs
- SchemaElement.cs
- propertyentry.cs
- Stack.cs
- Stack.cs
- EntityDataSourceView.cs
- EncryptedPackage.cs
- CroppedBitmap.cs
- BufferedOutputStream.cs
- PerfCounters.cs
- Column.cs
- DocumentPageTextView.cs
- basenumberconverter.cs
- MetadataUtil.cs
- StrokeNodeOperations.cs
- BitmapFrameDecode.cs
- LocalizabilityAttribute.cs
- FixedSOMPageElement.cs
- XmlMtomReader.cs
- FixedSOMFixedBlock.cs
- ContentPlaceHolder.cs
- SQLConvert.cs
- TextServicesLoader.cs
- NullRuntimeConfig.cs
- NamespaceCollection.cs
- RMEnrollmentPage1.cs
- Int32Rect.cs
- ProviderUtil.cs
- TrimSurroundingWhitespaceAttribute.cs
- Error.cs
- Content.cs
- ObjectStorage.cs
- DataView.cs
- BamlTreeNode.cs
- XmlSerializer.cs
- DataComponentNameHandler.cs
- ServerIdentity.cs
- CodeArrayCreateExpression.cs
- Environment.cs
- StylusShape.cs
- Aes.cs
- ServiceMetadataPublishingElement.cs
- Exception.cs
- AuthenticationModulesSection.cs
- LazyTextWriterCreator.cs
- SelectingProviderEventArgs.cs
- InkPresenter.cs
- PrimitiveXmlSerializers.cs
- GatewayDefinition.cs
- ReflectionTypeLoadException.cs
- HttpListener.cs
- SqlDataSourceCommandEventArgs.cs
- PackageRelationship.cs
- AuthenticationModuleElementCollection.cs
- FormsAuthentication.cs
- WebPart.cs