Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Imaging / LateBoundBitmapDecoder.cs / 1 / LateBoundBitmapDecoder.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: LateBoundBitmapDecoder.cs // //----------------------------------------------------------------------------- #pragma warning disable 1634, 1691 // Allow suppression of certain presharp messages using System; using System.IO; using System.IO.Packaging; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Collections.ObjectModel; using System.Reflection; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Win32.PresentationCore; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Threading; using System.Windows.Threading; using System.Windows.Media.Imaging; using MS.Internal.PresentationCore; // SecurityHelper using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Net; using System.Net.Cache; namespace System.Windows.Media.Imaging { #region LateBoundBitmapDecoder ////// LateBoundBitmapDecoder is a container for bitmap frames. Each bitmap frame is an BitmapFrame. /// Any BitmapFrame it returns are frozen /// be immutable. /// public sealed class LateBoundBitmapDecoder : BitmapDecoder { #region Constructors ////// Constructor /// ////// Critical: This code calls into BitmapDownload.BeginDownload which is critical /// [SecurityCritical] internal LateBoundBitmapDecoder( Uri baseUri, Uri uri, Stream stream, BitmapCreateOptions createOptions, BitmapCacheOption cacheOption, RequestCachePolicy requestCachePolicy ) : base(true) { _baseUri = baseUri; _uri = uri; _stream = stream; _createOptions = createOptions; _cacheOption = cacheOption; _requestCachePolicy = requestCachePolicy; // Check to see if we need to download content off thread Uri uriToDecode = (_baseUri != null) ? new Uri(_baseUri, _uri) : _uri; if (uriToDecode != null) { if (uriToDecode.Scheme == Uri.UriSchemeHttp || uriToDecode.Scheme == Uri.UriSchemeHttps) { // Begin the download BitmapDownload.BeginDownload(this, uriToDecode, _requestCachePolicy, _stream); _isDownloading = true; } } if (_stream != null && !_stream.CanSeek) { // Begin the download BitmapDownload.BeginDownload(this, uriToDecode, _requestCachePolicy, _stream); _isDownloading = true; } } #endregion #region Properties ////// If there is an palette, return it. /// Otherwise, return null. /// If the LateBoundDecoder is still downloading, the returned Palette is null. /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Getting palette data is OK /// public override BitmapPalette Palette { [SecurityCritical] get { VerifyAccess(); if (_isDownloading) { return null; } return Decoder.Palette; } } ////// If there is an embedded color profile, return it. /// Otherwise, return null. /// If the LateBoundDecoder is still downloading, the returned ColorContext is null. /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Getting colorcontext data is OK /// public override ReadOnlyCollectionColorContexts { [SecurityCritical] get { VerifyAccess(); if (_isDownloading) { return null; } return Decoder.ColorContexts; } } /// /// If there is a global thumbnail, return it. /// Otherwise, return null. The returned source is frozen. /// If the LateBoundDecoder is still downloading, the returned Thumbnail is null. /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Getting thumbnail data is OK /// public override BitmapSource Thumbnail { [SecurityCritical] get { VerifyAccess(); if (_isDownloading) { return null; } return Decoder.Thumbnail; } } ////// The info that identifies this codec. /// If the LateBoundDecoder is still downloading, the returned CodecInfo is null. /// ////// The getter demands RegistryPermission(PermissionState.Unrestricted) /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Demands RegistryPermission(PermissionState.Unrestricted) /// public override BitmapCodecInfo CodecInfo { [SecurityCritical] get { VerifyAccess(); SecurityHelper.DemandRegistryPermission(); if (_isDownloading) { return null; } return Decoder.CodecInfo; } } ////// Access to the individual frames. /// Since a LateBoundBitmapDecoder is downloaded asynchronously, /// its possible the underlying frame collection may change once /// content has been downloaded and decoded. When content is initially /// downloading, the collection will always return at least one item /// in the collection. When the download/decode is complete, the BitmapFrame /// will automatically change its underlying content. i.e. Only the collection /// object may change. The actual frame object will remain the same. /// public override ReadOnlyCollectionFrames { get { VerifyAccess(); // If the content is still being downloaded, create a collection // with 1 item that will point to an empty bitmap if (_isDownloading) { if (_readOnlyFrames == null) { _frames = new List ((int)1); _frames.Add( new BitmapFrameDecode( 0, _createOptions, _cacheOption, this ) ); _readOnlyFrames = new ReadOnlyCollection (_frames); } return _readOnlyFrames; } else { return Decoder.Frames; } } } /// /// If there is a global preview image, return it. /// Otherwise, return null. The returned source is frozen. /// If the LateBoundDecoder is still downloading, the returned Preview is null. /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Getting preview data is OK /// public override BitmapSource Preview { [SecurityCritical] get { VerifyAccess(); if (_isDownloading) { return null; } return Decoder.Preview; } } ////// Returns the underlying decoder associated with this late bound decoder. /// If the LateBoundDecoder is still downloading, the underlying decoder is null, /// otherwise the underlying decoder is created on first access. /// public BitmapDecoder Decoder { get { VerifyAccess(); if (_isDownloading || _failed) { return null; } EnsureDecoder(); return _realDecoder; } } ////// Returns if the decoder is downloading content /// public override bool IsDownloading { get { VerifyAccess(); return _isDownloading; } } #endregion #region Methods /// /// Ensure that the underlying decoder is created /// private void EnsureDecoder() { if (_realDecoder == null) { _realDecoder = BitmapDecoder.CreateFromUriOrStream( _baseUri, _uri, _stream, _createOptions & ~BitmapCreateOptions.DelayCreation, _cacheOption, _requestCachePolicy, true ); // Check to see if someone already got the frames // If so, we need to ensure that the real decoder // references the same frame as the one we already created // Creating a new object would be bad. if (_readOnlyFrames != null) { _realDecoder.SetupFrames(null, _readOnlyFrames); // // The frames have been transfered to the real decoder, so we no // longer need them. // _readOnlyFrames = null; _frames = null; } } } /// /// Called when download is complete /// internal object DownloadCallback(object arg) { Stream newStream = (Stream)arg; // Assert that we are able to seek the new stream Debug.Assert(newStream.CanSeek == true); _stream = newStream; // If we are not supposed to delay create, then ensure the decoder // otherwise it will be done on first access if ((_createOptions & BitmapCreateOptions.DelayCreation) == 0) { try { EnsureDecoder(); } catch(Exception e) { #pragma warning disable 6500 return ExceptionCallback(e); #pragma warning restore 6500 } } _isDownloading = false; _downloadEvent.InvokeEvents(this, null); return null; } /// /// Called when download progresses /// internal object ProgressCallback(object arg) { int percentComplete = (int)arg; _progressEvent.InvokeEvents(this, new DownloadProgressEventArgs(percentComplete)); return null; } /// /// Called when an exception occurs /// internal object ExceptionCallback(object arg) { _isDownloading = false; _failed = true; _failedEvent.InvokeEvents(this, new ExceptionEventArgs((Exception)arg)); return null; } #endregion #region Internal Abstract /// Need to implement this to derive from the "sealed" object internal override void SealObject() { throw new NotImplementedException(); } #endregion #region Data Members /// Is downloading data private bool _isDownloading; /// Is downloading data private bool _failed; /// Real decoder private BitmapDecoder _realDecoder; ////// the cache policy to use for web requests. /// private RequestCachePolicy _requestCachePolicy; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: LateBoundBitmapDecoder.cs // //----------------------------------------------------------------------------- #pragma warning disable 1634, 1691 // Allow suppression of certain presharp messages using System; using System.IO; using System.IO.Packaging; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Collections.ObjectModel; using System.Reflection; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Win32.PresentationCore; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Threading; using System.Windows.Threading; using System.Windows.Media.Imaging; using MS.Internal.PresentationCore; // SecurityHelper using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Net; using System.Net.Cache; namespace System.Windows.Media.Imaging { #region LateBoundBitmapDecoder ////// LateBoundBitmapDecoder is a container for bitmap frames. Each bitmap frame is an BitmapFrame. /// Any BitmapFrame it returns are frozen /// be immutable. /// public sealed class LateBoundBitmapDecoder : BitmapDecoder { #region Constructors ////// Constructor /// ////// Critical: This code calls into BitmapDownload.BeginDownload which is critical /// [SecurityCritical] internal LateBoundBitmapDecoder( Uri baseUri, Uri uri, Stream stream, BitmapCreateOptions createOptions, BitmapCacheOption cacheOption, RequestCachePolicy requestCachePolicy ) : base(true) { _baseUri = baseUri; _uri = uri; _stream = stream; _createOptions = createOptions; _cacheOption = cacheOption; _requestCachePolicy = requestCachePolicy; // Check to see if we need to download content off thread Uri uriToDecode = (_baseUri != null) ? new Uri(_baseUri, _uri) : _uri; if (uriToDecode != null) { if (uriToDecode.Scheme == Uri.UriSchemeHttp || uriToDecode.Scheme == Uri.UriSchemeHttps) { // Begin the download BitmapDownload.BeginDownload(this, uriToDecode, _requestCachePolicy, _stream); _isDownloading = true; } } if (_stream != null && !_stream.CanSeek) { // Begin the download BitmapDownload.BeginDownload(this, uriToDecode, _requestCachePolicy, _stream); _isDownloading = true; } } #endregion #region Properties ////// If there is an palette, return it. /// Otherwise, return null. /// If the LateBoundDecoder is still downloading, the returned Palette is null. /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Getting palette data is OK /// public override BitmapPalette Palette { [SecurityCritical] get { VerifyAccess(); if (_isDownloading) { return null; } return Decoder.Palette; } } ////// If there is an embedded color profile, return it. /// Otherwise, return null. /// If the LateBoundDecoder is still downloading, the returned ColorContext is null. /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Getting colorcontext data is OK /// public override ReadOnlyCollectionColorContexts { [SecurityCritical] get { VerifyAccess(); if (_isDownloading) { return null; } return Decoder.ColorContexts; } } /// /// If there is a global thumbnail, return it. /// Otherwise, return null. The returned source is frozen. /// If the LateBoundDecoder is still downloading, the returned Thumbnail is null. /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Getting thumbnail data is OK /// public override BitmapSource Thumbnail { [SecurityCritical] get { VerifyAccess(); if (_isDownloading) { return null; } return Decoder.Thumbnail; } } ////// The info that identifies this codec. /// If the LateBoundDecoder is still downloading, the returned CodecInfo is null. /// ////// The getter demands RegistryPermission(PermissionState.Unrestricted) /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Demands RegistryPermission(PermissionState.Unrestricted) /// public override BitmapCodecInfo CodecInfo { [SecurityCritical] get { VerifyAccess(); SecurityHelper.DemandRegistryPermission(); if (_isDownloading) { return null; } return Decoder.CodecInfo; } } ////// Access to the individual frames. /// Since a LateBoundBitmapDecoder is downloaded asynchronously, /// its possible the underlying frame collection may change once /// content has been downloaded and decoded. When content is initially /// downloading, the collection will always return at least one item /// in the collection. When the download/decode is complete, the BitmapFrame /// will automatically change its underlying content. i.e. Only the collection /// object may change. The actual frame object will remain the same. /// public override ReadOnlyCollectionFrames { get { VerifyAccess(); // If the content is still being downloaded, create a collection // with 1 item that will point to an empty bitmap if (_isDownloading) { if (_readOnlyFrames == null) { _frames = new List ((int)1); _frames.Add( new BitmapFrameDecode( 0, _createOptions, _cacheOption, this ) ); _readOnlyFrames = new ReadOnlyCollection (_frames); } return _readOnlyFrames; } else { return Decoder.Frames; } } } /// /// If there is a global preview image, return it. /// Otherwise, return null. The returned source is frozen. /// If the LateBoundDecoder is still downloading, the returned Preview is null. /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Getting preview data is OK /// public override BitmapSource Preview { [SecurityCritical] get { VerifyAccess(); if (_isDownloading) { return null; } return Decoder.Preview; } } ////// Returns the underlying decoder associated with this late bound decoder. /// If the LateBoundDecoder is still downloading, the underlying decoder is null, /// otherwise the underlying decoder is created on first access. /// public BitmapDecoder Decoder { get { VerifyAccess(); if (_isDownloading || _failed) { return null; } EnsureDecoder(); return _realDecoder; } } ////// Returns if the decoder is downloading content /// public override bool IsDownloading { get { VerifyAccess(); return _isDownloading; } } #endregion #region Methods /// /// Ensure that the underlying decoder is created /// private void EnsureDecoder() { if (_realDecoder == null) { _realDecoder = BitmapDecoder.CreateFromUriOrStream( _baseUri, _uri, _stream, _createOptions & ~BitmapCreateOptions.DelayCreation, _cacheOption, _requestCachePolicy, true ); // Check to see if someone already got the frames // If so, we need to ensure that the real decoder // references the same frame as the one we already created // Creating a new object would be bad. if (_readOnlyFrames != null) { _realDecoder.SetupFrames(null, _readOnlyFrames); // // The frames have been transfered to the real decoder, so we no // longer need them. // _readOnlyFrames = null; _frames = null; } } } /// /// Called when download is complete /// internal object DownloadCallback(object arg) { Stream newStream = (Stream)arg; // Assert that we are able to seek the new stream Debug.Assert(newStream.CanSeek == true); _stream = newStream; // If we are not supposed to delay create, then ensure the decoder // otherwise it will be done on first access if ((_createOptions & BitmapCreateOptions.DelayCreation) == 0) { try { EnsureDecoder(); } catch(Exception e) { #pragma warning disable 6500 return ExceptionCallback(e); #pragma warning restore 6500 } } _isDownloading = false; _downloadEvent.InvokeEvents(this, null); return null; } /// /// Called when download progresses /// internal object ProgressCallback(object arg) { int percentComplete = (int)arg; _progressEvent.InvokeEvents(this, new DownloadProgressEventArgs(percentComplete)); return null; } /// /// Called when an exception occurs /// internal object ExceptionCallback(object arg) { _isDownloading = false; _failed = true; _failedEvent.InvokeEvents(this, new ExceptionEventArgs((Exception)arg)); return null; } #endregion #region Internal Abstract /// Need to implement this to derive from the "sealed" object internal override void SealObject() { throw new NotImplementedException(); } #endregion #region Data Members /// Is downloading data private bool _isDownloading; /// Is downloading data private bool _failed; /// Real decoder private BitmapDecoder _realDecoder; ////// the cache policy to use for web requests. /// private RequestCachePolicy _requestCachePolicy; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
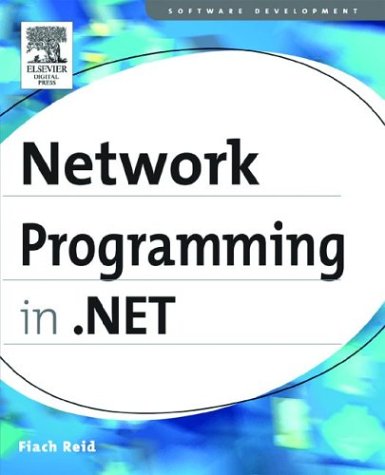
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InvalidProgramException.cs
- Journal.cs
- Image.cs
- SchemaNames.cs
- KeyGestureValueSerializer.cs
- Hex.cs
- FilteredAttributeCollection.cs
- BrowserTree.cs
- InitializerFacet.cs
- HttpWriter.cs
- XmlTextAttribute.cs
- TextBounds.cs
- SqlTriggerAttribute.cs
- ManagementObjectCollection.cs
- ResourcePermissionBase.cs
- X509Certificate.cs
- EventArgs.cs
- PathFigureCollection.cs
- ImageFormatConverter.cs
- SqlBooleanMismatchVisitor.cs
- CaseInsensitiveHashCodeProvider.cs
- GlobalItem.cs
- XmlCompatibilityReader.cs
- StylusPointPropertyInfoDefaults.cs
- UnsafeNativeMethodsTablet.cs
- GACIdentityPermission.cs
- MDIClient.cs
- ScriptMethodAttribute.cs
- FixedPageStructure.cs
- AsyncMethodInvoker.cs
- InternalConfigHost.cs
- DecoderBestFitFallback.cs
- ScriptResourceAttribute.cs
- LabelEditEvent.cs
- IdentityNotMappedException.cs
- ToolboxComponentsCreatedEventArgs.cs
- DataObjectEventArgs.cs
- VariableQuery.cs
- FrameSecurityDescriptor.cs
- HierarchicalDataBoundControl.cs
- AdvancedBindingPropertyDescriptor.cs
- ZipIOFileItemStream.cs
- WorkflowDefinitionContext.cs
- DiscoveryClientReferences.cs
- ObjectAssociationEndMapping.cs
- COM2PictureConverter.cs
- CommonGetThemePartSize.cs
- WebContext.cs
- FontWeights.cs
- BamlResourceContent.cs
- AccessibleObject.cs
- MultiPageTextView.cs
- GeometryHitTestResult.cs
- MD5CryptoServiceProvider.cs
- TableItemProviderWrapper.cs
- ContractBase.cs
- DatatypeImplementation.cs
- SmtpNegotiateAuthenticationModule.cs
- compensatingcollection.cs
- ToolStripComboBox.cs
- HasCopySemanticsAttribute.cs
- SHA256.cs
- ProtectedProviderSettings.cs
- ListSortDescription.cs
- XamlGridLengthSerializer.cs
- ZipIOExtraFieldZip64Element.cs
- BaseTemplateCodeDomTreeGenerator.cs
- ActiveDesignSurfaceEvent.cs
- XamlClipboardData.cs
- ConditionCollection.cs
- SecurityTokenTypes.cs
- X509ChainElement.cs
- AuthenticationModuleElement.cs
- HttpHandlerAction.cs
- XmlComment.cs
- SocketPermission.cs
- ButtonStandardAdapter.cs
- DbDataSourceEnumerator.cs
- PackagingUtilities.cs
- RenderingBiasValidation.cs
- UxThemeWrapper.cs
- ButtonPopupAdapter.cs
- StdValidatorsAndConverters.cs
- AutomationPropertyInfo.cs
- SingleTagSectionHandler.cs
- BinaryEditor.cs
- InstancePersistenceEvent.cs
- ConditionalAttribute.cs
- Point3DAnimationUsingKeyFrames.cs
- PartialTrustVisibleAssembly.cs
- ProbeMatchesApril2005.cs
- CheckBoxDesigner.cs
- TextCompositionEventArgs.cs
- VersionPair.cs
- WindowsStatusBar.cs
- AssemblyName.cs
- dsa.cs
- Int64Converter.cs
- RelativeSource.cs
- _ConnectionGroup.cs