Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Animation / WeakRefEnumerator.cs / 1 / WeakRefEnumerator.cs
//------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2003 // // File: WeakRefEnumerator.cs //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; namespace System.Windows.Media.Animation { ////// An enumerator for collections of weak references. /// ////// The enumerator returns only live objects and removes dead /// references from the list as it walks. /// internal struct WeakRefEnumerator{ #region Internal implementation #region Construction /// /// Creates an enumerator for the given list. /// /// /// The list to enumerate. /// internal WeakRefEnumerator(Listlist) { _list = list; _readIndex = 0; _writeIndex = 0; _current = default(T); #if DEBUG _valid = false; #endif // DEBUG } #endregion // Construction #region Properties /// /// Gets the current element in the collection. /// ////// The current element in the collection. /// internal T Current { get { #if DEBUG Debug.Assert(_valid); #endif // DEBUG return _current; } } ////// Gets the index of the current element in the collection. /// ////// The index of the current element in the collection. /// internal int CurrentIndex { get { #if DEBUG Debug.Assert(_valid); #endif // DEBUG return _writeIndex - 1; } } #endregion // Properties #region Methods ////// This method must be called when the enumerator is no longer /// in use, if the last call to MoveNext returned true. The /// enumerator is no longer valid after this call. /// ////// When MoveNext returns false, the collection being enumerated is /// left in a good, known state, so nothing additional needs to be /// done. However, before MoveNext returns false the collection is /// in an intermediate state, so if the enumerator isn't advanced /// all the way to the end then this method must be called to clean /// up the collection and make sure that it ends in a known state. /// Calling this method in the case where MoveNext has returned /// false is OK. In that case, the method is a no-op. /// internal void Dispose() { // Remove only those elements that we've previously seen. // This means removing the range between the read and write // indices, as that's dead space in the collection. if (_readIndex != _writeIndex) { _list.RemoveRange(_writeIndex, _readIndex - _writeIndex); _readIndex = _writeIndex = _list.Count; } _current = default(T); #if DEBUG _valid = false; #endif // DEBUG } ////// Advances the enumerator to the next element of the collection. /// ////// true if the enumerator was successfully advanced to the next /// element; false if the enumerator has passed the end of the /// collection. /// ////// Elements that have been GC'ed are removed from the list. /// internal bool MoveNext() { // Get a reference to the next element that has not yet been // garbage-collected while (_readIndex < _list.Count) { WeakReference currentRef = _list[_readIndex]; _current = (T)currentRef.Target; if ( (object)_current != null) { // Found a live object. First compress the list, which // is necessary if we've previously seen GC'ed objects. if (_writeIndex != _readIndex) { _list[_writeIndex] = currentRef; } // Update internal state and return the found element _readIndex++; _writeIndex++; #if DEBUG _valid = true; #endif // DEBUG return true; } else { // This object was garbage-collected, so keep looking _readIndex++; } } // If we get here we didn't have any more live elements in the // collection, so we should return false. This is also a good // opportunity to clean up the list as necessary. Dispose(); return false; } #endregion // Methods #region Data private List_list; private T _current; private int _readIndex; private int _writeIndex; #if DEBUG private bool _valid; #endif // DEBUG #endregion // Data #endregion // Internal implementation } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2003 // // File: WeakRefEnumerator.cs //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; namespace System.Windows.Media.Animation { /// /// An enumerator for collections of weak references. /// ////// The enumerator returns only live objects and removes dead /// references from the list as it walks. /// internal struct WeakRefEnumerator{ #region Internal implementation #region Construction /// /// Creates an enumerator for the given list. /// /// /// The list to enumerate. /// internal WeakRefEnumerator(Listlist) { _list = list; _readIndex = 0; _writeIndex = 0; _current = default(T); #if DEBUG _valid = false; #endif // DEBUG } #endregion // Construction #region Properties /// /// Gets the current element in the collection. /// ////// The current element in the collection. /// internal T Current { get { #if DEBUG Debug.Assert(_valid); #endif // DEBUG return _current; } } ////// Gets the index of the current element in the collection. /// ////// The index of the current element in the collection. /// internal int CurrentIndex { get { #if DEBUG Debug.Assert(_valid); #endif // DEBUG return _writeIndex - 1; } } #endregion // Properties #region Methods ////// This method must be called when the enumerator is no longer /// in use, if the last call to MoveNext returned true. The /// enumerator is no longer valid after this call. /// ////// When MoveNext returns false, the collection being enumerated is /// left in a good, known state, so nothing additional needs to be /// done. However, before MoveNext returns false the collection is /// in an intermediate state, so if the enumerator isn't advanced /// all the way to the end then this method must be called to clean /// up the collection and make sure that it ends in a known state. /// Calling this method in the case where MoveNext has returned /// false is OK. In that case, the method is a no-op. /// internal void Dispose() { // Remove only those elements that we've previously seen. // This means removing the range between the read and write // indices, as that's dead space in the collection. if (_readIndex != _writeIndex) { _list.RemoveRange(_writeIndex, _readIndex - _writeIndex); _readIndex = _writeIndex = _list.Count; } _current = default(T); #if DEBUG _valid = false; #endif // DEBUG } ////// Advances the enumerator to the next element of the collection. /// ////// true if the enumerator was successfully advanced to the next /// element; false if the enumerator has passed the end of the /// collection. /// ////// Elements that have been GC'ed are removed from the list. /// internal bool MoveNext() { // Get a reference to the next element that has not yet been // garbage-collected while (_readIndex < _list.Count) { WeakReference currentRef = _list[_readIndex]; _current = (T)currentRef.Target; if ( (object)_current != null) { // Found a live object. First compress the list, which // is necessary if we've previously seen GC'ed objects. if (_writeIndex != _readIndex) { _list[_writeIndex] = currentRef; } // Update internal state and return the found element _readIndex++; _writeIndex++; #if DEBUG _valid = true; #endif // DEBUG return true; } else { // This object was garbage-collected, so keep looking _readIndex++; } } // If we get here we didn't have any more live elements in the // collection, so we should return false. This is also a good // opportunity to clean up the list as necessary. Dispose(); return false; } #endregion // Methods #region Data private List_list; private T _current; private int _readIndex; private int _writeIndex; #if DEBUG private bool _valid; #endif // DEBUG #endregion // Data #endregion // Internal implementation } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
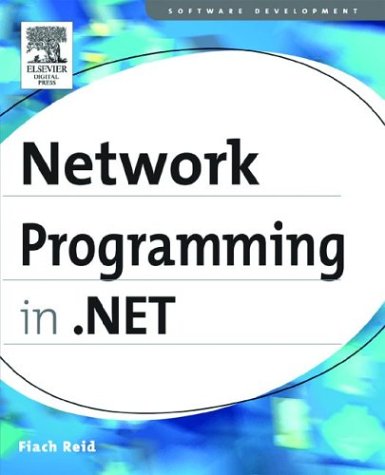
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NetworkInterface.cs
- SqlFunctionAttribute.cs
- TextControlDesigner.cs
- CssTextWriter.cs
- Imaging.cs
- XmlChildNodes.cs
- GroupItemAutomationPeer.cs
- Helper.cs
- AuthenticateEventArgs.cs
- MeshGeometry3D.cs
- CuspData.cs
- CryptoConfig.cs
- MarkupExtensionParser.cs
- ObjectDataSourceChooseMethodsPanel.cs
- EncodingDataItem.cs
- ConfigDefinitionUpdates.cs
- UnsignedPublishLicense.cs
- StoragePropertyMapping.cs
- XmlUrlResolver.cs
- InstanceKeyCollisionException.cs
- MetadataFile.cs
- SecurityDescriptor.cs
- RotateTransform3D.cs
- ConfigXmlComment.cs
- XmlSchemaCollection.cs
- PropertyMappingExceptionEventArgs.cs
- TemplateContainer.cs
- AspCompat.cs
- WebPartConnectionsCancelEventArgs.cs
- peernodeimplementation.cs
- QueryCorrelationInitializer.cs
- ContentPropertyAttribute.cs
- CommandField.cs
- ProxyGenerationError.cs
- NegotiateStream.cs
- DrawingBrush.cs
- DoubleLink.cs
- LocationSectionRecord.cs
- WindowsFormsSectionHandler.cs
- OutputScopeManager.cs
- InteropExecutor.cs
- StrongName.cs
- PointCollection.cs
- CategoryValueConverter.cs
- CustomLineCap.cs
- ImageSource.cs
- HostedBindingBehavior.cs
- WebSysDescriptionAttribute.cs
- PropertyMetadata.cs
- AutomationElement.cs
- AdCreatedEventArgs.cs
- ProxyWebPartConnectionCollection.cs
- IndentTextWriter.cs
- ResourcePermissionBaseEntry.cs
- ToolBar.cs
- Parser.cs
- AnimationStorage.cs
- ImageMapEventArgs.cs
- VariableQuery.cs
- LinqExpressionNormalizer.cs
- FacetChecker.cs
- DPCustomTypeDescriptor.cs
- ObjectQuery_EntitySqlExtensions.cs
- CodeIdentifier.cs
- CookielessHelper.cs
- UriTemplateVariablePathSegment.cs
- Encoder.cs
- NullToBooleanConverter.cs
- DockPanel.cs
- SmiXetterAccessMap.cs
- ParseElement.cs
- ImageClickEventArgs.cs
- FlowDocumentPage.cs
- AccessText.cs
- TypeValidationEventArgs.cs
- XsltSettings.cs
- SoapAttributeOverrides.cs
- TTSVoice.cs
- DataSpaceManager.cs
- Int32AnimationBase.cs
- CaretElement.cs
- QuaternionRotation3D.cs
- Environment.cs
- GraphicsState.cs
- PassportAuthentication.cs
- SafeWaitHandle.cs
- ExtendedPropertyDescriptor.cs
- StateFinalizationDesigner.cs
- ClipboardProcessor.cs
- FloaterParaClient.cs
- dataprotectionpermission.cs
- XmlAttributeHolder.cs
- TypeToArgumentTypeConverter.cs
- RewritingPass.cs
- Size.cs
- Trace.cs
- IsolatedStorageFile.cs
- FieldBuilder.cs
- MetadataWorkspace.cs
- ChunkedMemoryStream.cs