Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Documents / nulltextcontainer.cs / 1 / nulltextcontainer.cs
//---------------------------------------------------------------------------- //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // Description: // NullTextContainer is an immutable empty TextContainer that contains // single collapsed Start/End position. This is primarily used internally // so parameter check is mostly replaced with debug assert. // // History: // 07/14/2004 - Zhenbin Xu (ZhenbinX) - Created. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using System; using System.Diagnostics; using System.Windows.Threading; using System.Windows; // DependencyID etc. using MS.Internal.Documents; using MS.Internal; //===================================================================== ////// NullTextContainer is an immutable empty TextContainer that contains /// single collapsed Start/End position. This is primarily used internally /// so parameter check is mostly replaced with debug assert. /// internal sealed class NullTextContainer : ITextContainer { //------------------------------------------------------------------- // // Connstructors // //---------------------------------------------------------------------- #region Constructors internal NullTextContainer() { _start = new NullTextPointer(this, LogicalDirection.Backward); _end = new NullTextPointer(this, LogicalDirection.Forward); } #endregion Constructors //------------------------------------------------------------------- // // Public Methods // //---------------------------------------------------------------------- #region Public Methods // // This is readonly Text OM. All modification methods returns false // void ITextContainer.BeginChange() { } ////// void ITextContainer.BeginChangeNoUndo() { // We don't support undo, so follow the BeginChange codepath. ((ITextContainer)this).BeginChange(); } ////// /// void ITextContainer.EndChange() { ((ITextContainer)this).EndChange(false /* skipEvents */); } ////// void ITextContainer.EndChange(bool skipEvents) { } // Allocate a new ITextPointer at the specified offset. // Equivalent to this.Start.CreatePointer(offset), but does not // necessarily allocate this.Start. ITextPointer ITextContainer.CreatePointerAtOffset(int offset, LogicalDirection direction) { return ((ITextContainer)this).Start.CreatePointer(offset, direction); } // Not Implemented. ITextPointer ITextContainer.CreatePointerAtCharOffset(int charOffset, LogicalDirection direction) { throw new NotImplementedException(); } ITextPointer ITextContainer.CreateDynamicTextPointer(StaticTextPointer position, LogicalDirection direction) { return ((ITextPointer)position.Handle0).CreatePointer(direction); } StaticTextPointer ITextContainer.CreateStaticPointerAtOffset(int offset) { return new StaticTextPointer(this, ((ITextContainer)this).CreatePointerAtOffset(offset, LogicalDirection.Forward)); } TextPointerContext ITextContainer.GetPointerContext(StaticTextPointer pointer, LogicalDirection direction) { return ((ITextPointer)pointer.Handle0).GetPointerContext(direction); } int ITextContainer.GetOffsetToPosition(StaticTextPointer position1, StaticTextPointer position2) { return ((ITextPointer)position1.Handle0).GetOffsetToPosition((ITextPointer)position2.Handle0); } int ITextContainer.GetTextInRun(StaticTextPointer position, LogicalDirection direction, char[] textBuffer, int startIndex, int count) { return ((ITextPointer)position.Handle0).GetTextInRun(direction, textBuffer, startIndex, count); } object ITextContainer.GetAdjacentElement(StaticTextPointer position, LogicalDirection direction) { return ((ITextPointer)position.Handle0).GetAdjacentElement(direction); } DependencyObject ITextContainer.GetParent(StaticTextPointer position) { return null; } StaticTextPointer ITextContainer.CreatePointer(StaticTextPointer position, int offset) { return new StaticTextPointer(this, ((ITextPointer)position.Handle0).CreatePointer(offset)); } StaticTextPointer ITextContainer.GetNextContextPosition(StaticTextPointer position, LogicalDirection direction) { return new StaticTextPointer(this, ((ITextPointer)position.Handle0).GetNextContextPosition(direction)); } int ITextContainer.CompareTo(StaticTextPointer position1, StaticTextPointer position2) { return ((ITextPointer)position1.Handle0).CompareTo((ITextPointer)position2.Handle0); } int ITextContainer.CompareTo(StaticTextPointer position1, ITextPointer position2) { return ((ITextPointer)position1.Handle0).CompareTo(position2); } object ITextContainer.GetValue(StaticTextPointer position, DependencyProperty formattingProperty) { return ((ITextPointer)position.Handle0).GetValue(formattingProperty); } #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- #region Public Properties ////// bool ITextContainer.IsReadOnly { get { return true; } } ////// /// ITextPointer ITextContainer.Start { get { Debug.Assert(_start != null); return _start; } } ////// /// ITextPointer ITextContainer.End { get { Debug.Assert(_end != null); return _end; } } ////// /// Autoincremented counter of content changes in this TextContainer /// uint ITextContainer.Generation { get { // For read-only content, return some constant value. return 0; } } ////// Collection of highlights applied to TextContainer content. /// Highlights ITextContainer.Highlights { get { //agurcan: The following line makes it hard to use debugger on FDS code so I'm commenting it out //Debug.Assert(false, "Unexpected Highlights access on NullTextContainer!"); return null; } } ////// DependencyObject ITextContainer.Parent { get { return null; } } // Optional text selection, always null for this ITextContainer. ITextSelection ITextContainer.TextSelection { get { return null; } set { Invariant.Assert(false, "NullTextContainer is never associated with a TextEditor/TextSelection!"); } } // Optional undo manager, always null for this ITextContainer. UndoManager ITextContainer.UndoManager { get { return null; } } ///// // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // Description: // NullTextContainer is an immutable empty TextContainer that contains // single collapsed Start/End position. This is primarily used internally // so parameter check is mostly replaced with debug assert. // // History: // 07/14/2004 - Zhenbin Xu (ZhenbinX) - Created. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using System; using System.Diagnostics; using System.Windows.Threading; using System.Windows; // DependencyID etc. using MS.Internal.Documents; using MS.Internal; //===================================================================== /// /// NullTextContainer is an immutable empty TextContainer that contains /// single collapsed Start/End position. This is primarily used internally /// so parameter check is mostly replaced with debug assert. /// internal sealed class NullTextContainer : ITextContainer { //------------------------------------------------------------------- // // Connstructors // //---------------------------------------------------------------------- #region Constructors internal NullTextContainer() { _start = new NullTextPointer(this, LogicalDirection.Backward); _end = new NullTextPointer(this, LogicalDirection.Forward); } #endregion Constructors //------------------------------------------------------------------- // // Public Methods // //---------------------------------------------------------------------- #region Public Methods // // This is readonly Text OM. All modification methods returns false // void ITextContainer.BeginChange() { } ////// void ITextContainer.BeginChangeNoUndo() { // We don't support undo, so follow the BeginChange codepath. ((ITextContainer)this).BeginChange(); } ////// /// void ITextContainer.EndChange() { ((ITextContainer)this).EndChange(false /* skipEvents */); } ////// void ITextContainer.EndChange(bool skipEvents) { } // Allocate a new ITextPointer at the specified offset. // Equivalent to this.Start.CreatePointer(offset), but does not // necessarily allocate this.Start. ITextPointer ITextContainer.CreatePointerAtOffset(int offset, LogicalDirection direction) { return ((ITextContainer)this).Start.CreatePointer(offset, direction); } // Not Implemented. ITextPointer ITextContainer.CreatePointerAtCharOffset(int charOffset, LogicalDirection direction) { throw new NotImplementedException(); } ITextPointer ITextContainer.CreateDynamicTextPointer(StaticTextPointer position, LogicalDirection direction) { return ((ITextPointer)position.Handle0).CreatePointer(direction); } StaticTextPointer ITextContainer.CreateStaticPointerAtOffset(int offset) { return new StaticTextPointer(this, ((ITextContainer)this).CreatePointerAtOffset(offset, LogicalDirection.Forward)); } TextPointerContext ITextContainer.GetPointerContext(StaticTextPointer pointer, LogicalDirection direction) { return ((ITextPointer)pointer.Handle0).GetPointerContext(direction); } int ITextContainer.GetOffsetToPosition(StaticTextPointer position1, StaticTextPointer position2) { return ((ITextPointer)position1.Handle0).GetOffsetToPosition((ITextPointer)position2.Handle0); } int ITextContainer.GetTextInRun(StaticTextPointer position, LogicalDirection direction, char[] textBuffer, int startIndex, int count) { return ((ITextPointer)position.Handle0).GetTextInRun(direction, textBuffer, startIndex, count); } object ITextContainer.GetAdjacentElement(StaticTextPointer position, LogicalDirection direction) { return ((ITextPointer)position.Handle0).GetAdjacentElement(direction); } DependencyObject ITextContainer.GetParent(StaticTextPointer position) { return null; } StaticTextPointer ITextContainer.CreatePointer(StaticTextPointer position, int offset) { return new StaticTextPointer(this, ((ITextPointer)position.Handle0).CreatePointer(offset)); } StaticTextPointer ITextContainer.GetNextContextPosition(StaticTextPointer position, LogicalDirection direction) { return new StaticTextPointer(this, ((ITextPointer)position.Handle0).GetNextContextPosition(direction)); } int ITextContainer.CompareTo(StaticTextPointer position1, StaticTextPointer position2) { return ((ITextPointer)position1.Handle0).CompareTo((ITextPointer)position2.Handle0); } int ITextContainer.CompareTo(StaticTextPointer position1, ITextPointer position2) { return ((ITextPointer)position1.Handle0).CompareTo(position2); } object ITextContainer.GetValue(StaticTextPointer position, DependencyProperty formattingProperty) { return ((ITextPointer)position.Handle0).GetValue(formattingProperty); } #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- #region Public Properties ////// bool ITextContainer.IsReadOnly { get { return true; } } ////// /// ITextPointer ITextContainer.Start { get { Debug.Assert(_start != null); return _start; } } ////// /// ITextPointer ITextContainer.End { get { Debug.Assert(_end != null); return _end; } } ////// /// Autoincremented counter of content changes in this TextContainer /// uint ITextContainer.Generation { get { // For read-only content, return some constant value. return 0; } } ////// Collection of highlights applied to TextContainer content. /// Highlights ITextContainer.Highlights { get { //agurcan: The following line makes it hard to use debugger on FDS code so I'm commenting it out //Debug.Assert(false, "Unexpected Highlights access on NullTextContainer!"); return null; } } ////// DependencyObject ITextContainer.Parent { get { return null; } } // Optional text selection, always null for this ITextContainer. ITextSelection ITextContainer.TextSelection { get { return null; } set { Invariant.Assert(false, "NullTextContainer is never associated with a TextEditor/TextSelection!"); } } // Optional undo manager, always null for this ITextContainer. UndoManager ITextContainer.UndoManager { get { return null; } } /////
Link Menu
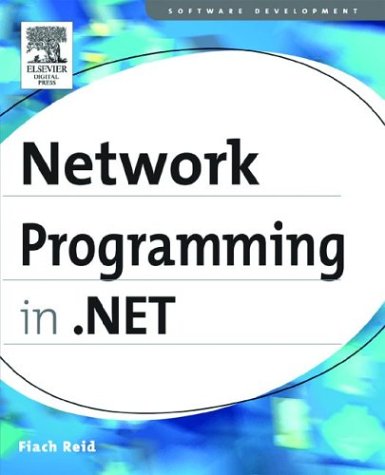
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataShape.cs
- Int64AnimationBase.cs
- graph.cs
- EnumerableCollectionView.cs
- CurrencyWrapper.cs
- ClientScriptManager.cs
- SingleSelectRootGridEntry.cs
- XmlSerializerSection.cs
- EntryPointNotFoundException.cs
- FilteredAttributeCollection.cs
- PointAnimation.cs
- SimpleWorkerRequest.cs
- Material.cs
- QilBinary.cs
- GridViewItemAutomationPeer.cs
- QilBinary.cs
- InvocationExpression.cs
- HandlerFactoryWrapper.cs
- WebPartTracker.cs
- GZipDecoder.cs
- ObjectListDataBindEventArgs.cs
- DBSchemaRow.cs
- metadatamappinghashervisitor.hashsourcebuilder.cs
- ManagementObjectSearcher.cs
- UnmanagedBitmapWrapper.cs
- QuaternionAnimation.cs
- CodeExpressionStatement.cs
- SiteMapHierarchicalDataSourceView.cs
- columnmapkeybuilder.cs
- SettingsPropertyIsReadOnlyException.cs
- CurrencyWrapper.cs
- XamlParser.cs
- WebBrowserEvent.cs
- DataServiceRequest.cs
- ConnectionPoint.cs
- Rotation3D.cs
- VectorAnimationBase.cs
- EventBindingService.cs
- basenumberconverter.cs
- Timer.cs
- EmptyStringExpandableObjectConverter.cs
- WMIInterop.cs
- CorrelationManager.cs
- GenericQueueSurrogate.cs
- MulticastIPAddressInformationCollection.cs
- XamlFilter.cs
- Grid.cs
- PropertyIDSet.cs
- CodeDomExtensionMethods.cs
- StrokeNodeEnumerator.cs
- SecuritySessionClientSettings.cs
- PropertyItem.cs
- DataBindEngine.cs
- PageRanges.cs
- PersonalizablePropertyEntry.cs
- TextTreeRootTextBlock.cs
- UInt32Converter.cs
- XmlMemberMapping.cs
- AssociationEndMember.cs
- BitmapDecoder.cs
- FormatSettings.cs
- DefaultBinder.cs
- EncoderParameters.cs
- PackUriHelper.cs
- CryptoApi.cs
- EntityDataSourceEntityTypeFilterItem.cs
- DefaultBindingPropertyAttribute.cs
- TypeResolver.cs
- CallTemplateAction.cs
- HostedElements.cs
- BackgroundWorker.cs
- ExternalException.cs
- AutomationIdentifierGuids.cs
- TransactionFilter.cs
- ExtractorMetadata.cs
- XmlArrayItemAttribute.cs
- Globals.cs
- ExtensionDataObject.cs
- EndpointAddressElementBase.cs
- AdRotator.cs
- AnonymousIdentificationSection.cs
- ContentHostHelper.cs
- DictionarySectionHandler.cs
- XmlSchemaAny.cs
- StackBuilderSink.cs
- GeneralTransform2DTo3DTo2D.cs
- CalendarItem.cs
- EmbeddedObject.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- Utility.cs
- HtmlControlAdapter.cs
- NameGenerator.cs
- ColorContext.cs
- TreeBuilderXamlTranslator.cs
- PermissionSetEnumerator.cs
- SortedDictionary.cs
- GridViewAutomationPeer.cs
- CellTreeNodeVisitors.cs
- WebPartMenu.cs
- RangeBase.cs