Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Base / System / IO / Packaging / ZipPackagePart.cs / 1 / ZipPackagePart.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This is a subclass for the abstract PackagePart class. // This implementation is specific to Zip file format. // // History: // 12/28/2004: SarjanaS: Initial creation. [BruceMac provided some of the // initial code] // //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using MS.Internal.IO.Zip; using MS.Internal.IO.Packaging; using MS.Internal; // for Invariant namespace System.IO.Packaging { ////// This class represents a Part within a Zip container. /// This is a part of the Packaging Layer APIs /// public sealed class ZipPackagePart : PackagePart { //----------------------------------------------------- // // Public Constructors // //----------------------------------------------------- // None //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- // None //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Custom Implementation for the GetStream Method /// /// Mode in which the stream should be opened /// Access with which the stream should be opened ///Stream Corresponding to this part protected override Stream GetStreamCore(FileMode mode, FileAccess access) { if (Package.InStreamingCreation) { // Convert Metro CompressionOption to Zip CompressionMethodEnum. CompressionMethodEnum compressionMethod; DeflateOptionEnum deflateOption; ZipPackage.GetZipCompressionMethodFromOpcCompressionOption(this.CompressionOption, out compressionMethod, out deflateOption); // Mode and access get validated in StreamingZipPartStream(). return new StreamingZipPartStream( PackUriHelper.GetStringForPartUri(this.Uri), _zipArchive, compressionMethod, deflateOption, mode, access); } else if (_zipFileInfo != null) { // Case of an atomic part. return _zipFileInfo.GetStream(mode, access); } else { // Case of an interleaved part. Invariant.Assert(_pieces != null); return new InterleavedZipPartStream(this, mode, access); } } #endregion Public Methods //----------------------------------------------------- // // Public Events // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Constructors // //----------------------------------------------------- #region Internal Constructors ////// Constructs a ZipPackagePart for an atomic (i.e. non-interleaved) part. /// This is called from the ZipPackage class as a result of GetPartCore, /// GetPartsCore or CreatePartCore methods /// /// /// /// /// /// internal ZipPackagePart(ZipPackage container, ZipFileInfo zipFileInfo, PackUriHelper.ValidatedPartUri partUri, string contentType, CompressionOption compressionOption) :base(container, partUri, contentType, compressionOption) { _zipArchive = zipFileInfo.ZipArchive; _zipFileInfo = zipFileInfo; } ////// Constructs a ZipPackagePart. This is called from ZipPackage.CreatePartCore in streaming /// production. /// No piece is created until the first write operation on the associated stream. Therefore /// this constructor does not take a ZipFileInfo. /// /// /// /// /// /// internal ZipPackagePart(ZipPackage container, ZipArchive zipArchive, PackUriHelper.ValidatedPartUri partUri, string contentType, CompressionOption compressionOption) :base(container, partUri, contentType, compressionOption) { _zipArchive = zipArchive; } ////// Constructs a ZipPackagePart for an interleaved part. This is called outside of streaming /// production when an interleaved part is encountered in the package. /// /// /// /// /// /// /// internal ZipPackagePart(ZipPackage container, ZipArchive zipArchive, Listpieces, PackUriHelper.ValidatedPartUri partUri, string contentType, CompressionOption compressionOption) :base(container, partUri, contentType, compressionOption) { _zipArchive = zipArchive; _pieces = pieces; } #endregion Internal Constructors //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties /// /// Obtain the sorted array of piece descriptors for an interleaved part. /// internal ListPieceDescriptors { get { return _pieces; } } /// /// Obtain the ZipFileInfo descriptor of an atomic part. /// internal ZipFileInfo ZipFileInfo { get { return _zipFileInfo; } } #endregion Internal Properties //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ // None //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- // None //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- // None //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Variables // Zip item info for an atomic part. private ZipFileInfo _zipFileInfo; // Piece descriptors for an interleaved part. private List_pieces; //ZipArhive private ZipArchive _zipArchive; #endregion Private Variables //------------------------------------------------------ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This is a subclass for the abstract PackagePart class. // This implementation is specific to Zip file format. // // History: // 12/28/2004: SarjanaS: Initial creation. [BruceMac provided some of the // initial code] // //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using MS.Internal.IO.Zip; using MS.Internal.IO.Packaging; using MS.Internal; // for Invariant namespace System.IO.Packaging { ////// This class represents a Part within a Zip container. /// This is a part of the Packaging Layer APIs /// public sealed class ZipPackagePart : PackagePart { //----------------------------------------------------- // // Public Constructors // //----------------------------------------------------- // None //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- // None //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Custom Implementation for the GetStream Method /// /// Mode in which the stream should be opened /// Access with which the stream should be opened ///Stream Corresponding to this part protected override Stream GetStreamCore(FileMode mode, FileAccess access) { if (Package.InStreamingCreation) { // Convert Metro CompressionOption to Zip CompressionMethodEnum. CompressionMethodEnum compressionMethod; DeflateOptionEnum deflateOption; ZipPackage.GetZipCompressionMethodFromOpcCompressionOption(this.CompressionOption, out compressionMethod, out deflateOption); // Mode and access get validated in StreamingZipPartStream(). return new StreamingZipPartStream( PackUriHelper.GetStringForPartUri(this.Uri), _zipArchive, compressionMethod, deflateOption, mode, access); } else if (_zipFileInfo != null) { // Case of an atomic part. return _zipFileInfo.GetStream(mode, access); } else { // Case of an interleaved part. Invariant.Assert(_pieces != null); return new InterleavedZipPartStream(this, mode, access); } } #endregion Public Methods //----------------------------------------------------- // // Public Events // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Constructors // //----------------------------------------------------- #region Internal Constructors ////// Constructs a ZipPackagePart for an atomic (i.e. non-interleaved) part. /// This is called from the ZipPackage class as a result of GetPartCore, /// GetPartsCore or CreatePartCore methods /// /// /// /// /// /// internal ZipPackagePart(ZipPackage container, ZipFileInfo zipFileInfo, PackUriHelper.ValidatedPartUri partUri, string contentType, CompressionOption compressionOption) :base(container, partUri, contentType, compressionOption) { _zipArchive = zipFileInfo.ZipArchive; _zipFileInfo = zipFileInfo; } ////// Constructs a ZipPackagePart. This is called from ZipPackage.CreatePartCore in streaming /// production. /// No piece is created until the first write operation on the associated stream. Therefore /// this constructor does not take a ZipFileInfo. /// /// /// /// /// /// internal ZipPackagePart(ZipPackage container, ZipArchive zipArchive, PackUriHelper.ValidatedPartUri partUri, string contentType, CompressionOption compressionOption) :base(container, partUri, contentType, compressionOption) { _zipArchive = zipArchive; } ////// Constructs a ZipPackagePart for an interleaved part. This is called outside of streaming /// production when an interleaved part is encountered in the package. /// /// /// /// /// /// /// internal ZipPackagePart(ZipPackage container, ZipArchive zipArchive, Listpieces, PackUriHelper.ValidatedPartUri partUri, string contentType, CompressionOption compressionOption) :base(container, partUri, contentType, compressionOption) { _zipArchive = zipArchive; _pieces = pieces; } #endregion Internal Constructors //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties /// /// Obtain the sorted array of piece descriptors for an interleaved part. /// internal ListPieceDescriptors { get { return _pieces; } } /// /// Obtain the ZipFileInfo descriptor of an atomic part. /// internal ZipFileInfo ZipFileInfo { get { return _zipFileInfo; } } #endregion Internal Properties //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ // None //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- // None //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- // None //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Variables // Zip item info for an atomic part. private ZipFileInfo _zipFileInfo; // Piece descriptors for an interleaved part. private List_pieces; //ZipArhive private ZipArchive _zipArchive; #endregion Private Variables //------------------------------------------------------ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
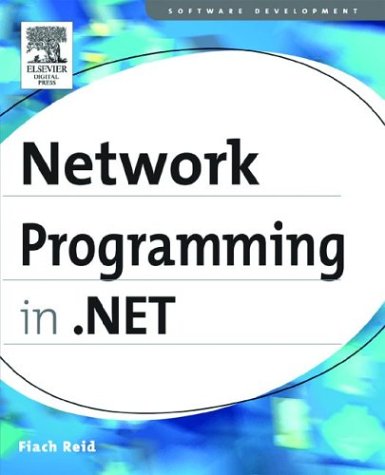
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Screen.cs
- SharedPerformanceCounter.cs
- DiscoveryInnerClientAdhoc11.cs
- QualifierSet.cs
- storagemappingitemcollection.viewdictionary.cs
- DataServiceConfiguration.cs
- PeerNearMe.cs
- PerfService.cs
- MemberPath.cs
- SymDocumentType.cs
- TextEmbeddedObject.cs
- ParentUndoUnit.cs
- PlainXmlWriter.cs
- OptionUsage.cs
- MimeXmlImporter.cs
- EasingQuaternionKeyFrame.cs
- MembershipSection.cs
- FontFamilyIdentifier.cs
- FrameSecurityDescriptor.cs
- RemoteWebConfigurationHostStream.cs
- BuildManagerHost.cs
- HttpListenerPrefixCollection.cs
- MsmqTransportSecurityElement.cs
- localization.cs
- IndexedEnumerable.cs
- KnownAssemblyEntry.cs
- RepeaterItem.cs
- DeploymentExceptionMapper.cs
- UidPropertyAttribute.cs
- PropertyMetadata.cs
- cookieexception.cs
- MimeXmlReflector.cs
- RotateTransform.cs
- SqlColumnizer.cs
- EntityClientCacheEntry.cs
- ChangeConflicts.cs
- AdCreatedEventArgs.cs
- CodeMethodReturnStatement.cs
- FlatButtonAppearance.cs
- DataStreams.cs
- dsa.cs
- QueryReaderSettings.cs
- ADConnectionHelper.cs
- XmlHierarchyData.cs
- ObjectListFieldCollection.cs
- regiisutil.cs
- WorkflowViewService.cs
- Encoder.cs
- WindowsListViewGroup.cs
- StructuralObject.cs
- PathFigure.cs
- RelationshipConverter.cs
- TabControl.cs
- AlgoModule.cs
- TemplateKey.cs
- ScaleTransform3D.cs
- CodeTypeConstructor.cs
- ButtonFlatAdapter.cs
- ToolStripProgressBar.cs
- ScaleTransform3D.cs
- PtsCache.cs
- DataServiceHost.cs
- BaseContextMenu.cs
- XPathNavigator.cs
- SqlDataSourceCache.cs
- AssemblyBuilderData.cs
- AutomationEventArgs.cs
- EventQueueState.cs
- ObjectDataSource.cs
- ValidatingReaderNodeData.cs
- DynamicPropertyReader.cs
- PropertyItem.cs
- ClassValidator.cs
- Base64Decoder.cs
- MaskedTextBoxDesignerActionList.cs
- WinEventTracker.cs
- SQLInt16Storage.cs
- TypeConverterMarkupExtension.cs
- UserValidatedEventArgs.cs
- DataGridCellItemAutomationPeer.cs
- CompilationRelaxations.cs
- ColumnMapProcessor.cs
- CatalogZone.cs
- PeerService.cs
- Window.cs
- SimpleWebHandlerParser.cs
- MobileContainerDesigner.cs
- PassportAuthenticationEventArgs.cs
- HtmlInputHidden.cs
- LoginName.cs
- DefaultHttpHandler.cs
- PartialTrustVisibleAssembliesSection.cs
- TraceSection.cs
- MDIWindowDialog.cs
- PathFigureCollectionConverter.cs
- TypefaceMetricsCache.cs
- BamlLocalizableResourceKey.cs
- X509AsymmetricSecurityKey.cs
- ColumnResizeUndoUnit.cs
- Repeater.cs