Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / Collections / Specialized / StringDictionary.cs / 1 / StringDictionary.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Collections.Specialized { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Collections; using System.ComponentModel.Design.Serialization; using System.Globalization; ////// [Serializable] [DesignerSerializer("System.Diagnostics.Design.StringDictionaryCodeDomSerializer, " + AssemblyRef.SystemDesign, "System.ComponentModel.Design.Serialization.CodeDomSerializer, " + AssemblyRef.SystemDesign)] public class StringDictionary : IEnumerable { internal Hashtable contents = new Hashtable(); ///Implements a hashtable with the key strongly typed to be /// a string rather than an object. ////// public StringDictionary() { } ///Initializes a new instance of the System.Windows.Forms.StringDictionary class. ////// public virtual int Count { get { return contents.Count; } } ///Gets the number of key-and-value pairs in the System.Windows.Forms.StringDictionary. ////// public virtual bool IsSynchronized { get { return contents.IsSynchronized; } } ///Indicates whether access to the System.Windows.Forms.StringDictionary is synchronized (thread-safe). This property is /// read-only. ////// public virtual string this[string key] { get { if( key == null ) { throw new ArgumentNullException("key"); } return (string) contents[key.ToLower(CultureInfo.InvariantCulture)]; } set { if( key == null ) { throw new ArgumentNullException("key"); } contents[key.ToLower(CultureInfo.InvariantCulture)] = value; } } ///Gets or sets the value associated with the specified key. ////// public virtual ICollection Keys { get { return contents.Keys; } } ///Gets a collection of keys in the System.Windows.Forms.StringDictionary. ////// public virtual object SyncRoot { get { return contents.SyncRoot; } } ///Gets an object that can be used to synchronize access to the System.Windows.Forms.StringDictionary. ////// public virtual ICollection Values { get { return contents.Values; } } ///Gets a collection of values in the System.Windows.Forms.StringDictionary. ////// public virtual void Add(string key, string value) { if( key == null ) { throw new ArgumentNullException("key"); } contents.Add(key.ToLower(CultureInfo.InvariantCulture), value); } ///Adds an entry with the specified key and value into the System.Windows.Forms.StringDictionary. ////// public virtual void Clear() { contents.Clear(); } ///Removes all entries from the System.Windows.Forms.StringDictionary. ////// public virtual bool ContainsKey(string key) { if( key == null ) { throw new ArgumentNullException("key"); } return contents.ContainsKey(key.ToLower(CultureInfo.InvariantCulture)); } ///Determines if the string dictionary contains a specific key ////// public virtual bool ContainsValue(string value) { return contents.ContainsValue(value); } ///Determines if the System.Windows.Forms.StringDictionary contains a specific value. ////// public virtual void CopyTo(Array array, int index) { contents.CopyTo(array, index); } ///Copies the string dictionary values to a one-dimensional ///instance at the /// specified index. /// public virtual IEnumerator GetEnumerator() { return contents.GetEnumerator(); } ///Returns an enumerator that can iterate through the string dictionary. ////// public virtual void Remove(string key) { if( key == null ) { throw new ArgumentNullException("key"); } contents.Remove(key.ToLower(CultureInfo.InvariantCulture)); } } }Removes the entry with the specified key from the string dictionary. ///
Link Menu
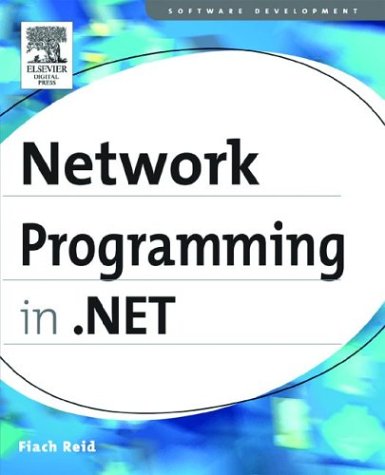
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafePEFileHandle.cs
- RtfToken.cs
- NotifyIcon.cs
- NotifyCollectionChangedEventArgs.cs
- FamilyTypeface.cs
- OrderedDictionaryStateHelper.cs
- ItemAutomationPeer.cs
- XmlArrayAttribute.cs
- SoapCodeExporter.cs
- Util.cs
- StoreContentChangedEventArgs.cs
- CodePageUtils.cs
- Icon.cs
- RC2CryptoServiceProvider.cs
- SpellerStatusTable.cs
- EventListener.cs
- ResourceSetExpression.cs
- WindowsRichEditRange.cs
- DataGridPagerStyle.cs
- ObjectDataSource.cs
- BindUriHelper.cs
- UserInitiatedRoutedEventPermissionAttribute.cs
- StreamGeometry.cs
- ISAPIApplicationHost.cs
- SapiInterop.cs
- SqlClientFactory.cs
- GeneralTransform3DGroup.cs
- MessageFormatterConverter.cs
- TakeOrSkipQueryOperator.cs
- MobileDeviceCapabilitiesSectionHandler.cs
- IProvider.cs
- ProcessModuleCollection.cs
- TypeConverters.cs
- RegistrationServices.cs
- ForwardPositionQuery.cs
- HtmlTableCell.cs
- VisualBrush.cs
- FontDifferentiator.cs
- CodeMethodReturnStatement.cs
- HttpServerUtilityWrapper.cs
- XsltFunctions.cs
- ExpressionBindingCollection.cs
- SqlDelegatedTransaction.cs
- DataRelationCollection.cs
- FixedDocumentPaginator.cs
- RightsManagementEncryptionTransform.cs
- XmlNodeChangedEventArgs.cs
- WebPartDescriptionCollection.cs
- AdapterUtil.cs
- Button.cs
- Propagator.ExtentPlaceholderCreator.cs
- CompositionAdorner.cs
- PhysicalOps.cs
- TypeReference.cs
- CuspData.cs
- ObjectReferenceStack.cs
- ScriptModule.cs
- DiffuseMaterial.cs
- MailAddress.cs
- LostFocusEventManager.cs
- StylusButton.cs
- Run.cs
- QueueException.cs
- WebSysDescriptionAttribute.cs
- FixedFindEngine.cs
- CompressionTransform.cs
- WizardStepBase.cs
- DesignerAttribute.cs
- LOSFormatter.cs
- _ConnectionGroup.cs
- ToolboxItemFilterAttribute.cs
- CodeVariableReferenceExpression.cs
- DirectoryNotFoundException.cs
- ListenerChannelContext.cs
- EFDataModelProvider.cs
- BasePropertyDescriptor.cs
- StreamWriter.cs
- DelegateBodyWriter.cs
- ProfileGroupSettings.cs
- ReadOnlyDictionary.cs
- GenericEnumConverter.cs
- OleServicesContext.cs
- ImageButton.cs
- SQLInt16.cs
- SessionIDManager.cs
- PresentationSource.cs
- SimpleType.cs
- sortedlist.cs
- PageClientProxyGenerator.cs
- HostingEnvironmentException.cs
- UnitySerializationHolder.cs
- SystemInfo.cs
- PinnedBufferMemoryStream.cs
- shaperfactoryquerycacheentry.cs
- Screen.cs
- WorkflowTraceTransfer.cs
- ProvidersHelper.cs
- DataDocumentXPathNavigator.cs
- EventLogger.cs
- DataTable.cs