Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Controls / ProgressBar.cs / 1 / ProgressBar.cs
//---------------------------------------------------------------------------- // File: ProgressBar.cs // // Description: // Implementation of ProgressBar control. // // History: // 06/28/2004 - [....] - Created // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.Specialized; using System.Threading; using System.Windows.Automation.Peers; using System.Windows.Automation.Provider; using System.Windows; using System.Windows.Controls.Primitives; using System.Windows.Media; using MS.Internal.KnownBoxes; namespace System.Windows.Controls { ////// The ProgressBar class /// ///[TemplatePart(Name = "PART_Track", Type = typeof(FrameworkElement))] [TemplatePart(Name = "PART_Indicator", Type = typeof(FrameworkElement))] public class ProgressBar : RangeBase { #region Constructors static ProgressBar() { FocusableProperty.OverrideMetadata(typeof(ProgressBar), new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); DefaultStyleKeyProperty.OverrideMetadata(typeof(ProgressBar), new FrameworkPropertyMetadata(typeof(ProgressBar))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(ProgressBar)); // Set default to 100.0 RangeBase.MaximumProperty.OverrideMetadata(typeof(ProgressBar), new FrameworkPropertyMetadata(100.0)); } /// /// Instantiates a new instance of Progressbar without Dispatcher. /// public ProgressBar() : base() { } #endregion Constructors #region Properties ////// The DependencyProperty for the IsIndeterminate property. /// Flags: none /// DefaultValue: false /// public static readonly DependencyProperty IsIndeterminateProperty = DependencyProperty.Register( "IsIndeterminate", typeof(bool), typeof(ProgressBar), new FrameworkPropertyMetadata( false, new PropertyChangedCallback(OnIsIndeterminateChanged))); ////// Determines if ProgressBar shows actual values (false) /// or generic, continuous progress feedback (true). /// ///public bool IsIndeterminate { get { return (bool) GetValue(IsIndeterminateProperty); } set { SetValue(IsIndeterminateProperty, value); } } /// /// Called when IsIndeterminateProperty is changed on "d". /// private static void OnIsIndeterminateChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { ProgressBar progressBar = (ProgressBar)d; // Invalidate automation peer ProgressBarAutomationPeer peer = UIElementAutomationPeer.FromElement(progressBar) as ProgressBarAutomationPeer; if (peer != null) { peer.InvalidatePeer(); } progressBar.SetProgressBarIndicatorLength(); } ////// DependencyProperty for public static readonly DependencyProperty OrientationProperty = DependencyProperty.Register( "Orientation", typeof(Orientation), typeof(ProgressBar), new FrameworkPropertyMetadata( Orientation.Horizontal, FrameworkPropertyMetadataOptions.AffectsMeasure), new ValidateValueCallback(IsValidOrientation)); ///property. /// /// Specifies orientation of the ProgressBar. /// public Orientation Orientation { get { return (Orientation) GetValue(OrientationProperty); } set { SetValue(OrientationProperty, value); } } internal static bool IsValidOrientation(object o) { Orientation value = (Orientation)o; return value == Orientation.Horizontal || value == Orientation.Vertical; } #endregion Properties #region Event Handler // Set the width/height of the contract parts private void SetProgressBarIndicatorLength() { if (_track != null && _indicator != null) { double min = Minimum; double max = Maximum; double val = Value; // When indeterminate or maximum == minimum, have the indicator stretch the // whole length of track double percent = IsIndeterminate || max == min ? 1.0 : (val - min) / (max - min); if (Orientation == Orientation.Horizontal) { _indicator.Width = percent * _track.ActualWidth; } else // Oriention == Vertical { _indicator.Height = percent * _track.ActualHeight; } } } #endregion #region Method Overrides ////// Creates AutomationPeer ( protected override AutomationPeer OnCreateAutomationPeer() { return new ProgressBarAutomationPeer(this); } ///) /// /// This method is invoked when the Value property changes. /// ProgressBar updates its style parts when Value changes. /// /// The old value of the Value property. /// The new value of the Value property. protected override void OnValueChanged(double oldValue, double newValue) { base.OnValueChanged(oldValue, newValue); SetProgressBarIndicatorLength(); } ////// Called when the Template's tree has been generated /// public override void OnApplyTemplate() { base.OnApplyTemplate(); if (_track != null) { _track.SizeChanged -= OnTrackSizeChanged; } _track = GetTemplateChild(TrackTemplateName) as FrameworkElement; _indicator = GetTemplateChild(IndicatorTemplateName) as FrameworkElement; if (_track != null) { _track.SizeChanged += OnTrackSizeChanged; } } private void OnTrackSizeChanged(object sender, SizeChangedEventArgs e) { SetProgressBarIndicatorLength(); } #endregion #region Data private const string TrackTemplateName = "PART_Track"; private const string IndicatorTemplateName = "PART_Indicator"; private FrameworkElement _track; private FrameworkElement _indicator; #endregion Data #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
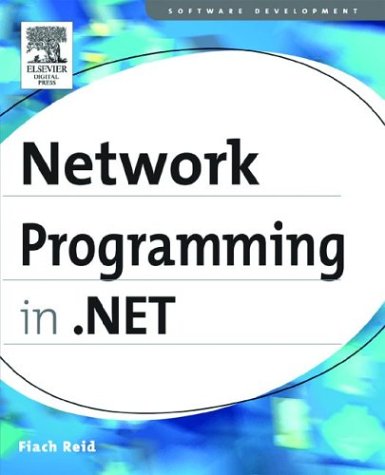
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PreviewKeyDownEventArgs.cs
- Italic.cs
- PasswordBox.cs
- Bitmap.cs
- ColumnMap.cs
- XPathAncestorIterator.cs
- ConfigXmlText.cs
- DataTableMappingCollection.cs
- BulletedList.cs
- GridViewSelectEventArgs.cs
- HttpPostProtocolImporter.cs
- FormsAuthenticationUser.cs
- ITreeGenerator.cs
- MenuAutomationPeer.cs
- SafePEFileHandle.cs
- QilStrConcat.cs
- TemplateBamlRecordReader.cs
- ToggleProviderWrapper.cs
- Errors.cs
- Button.cs
- QilInvokeEarlyBound.cs
- DependencyObjectProvider.cs
- loginstatus.cs
- XamlReaderConstants.cs
- DictionaryMarkupSerializer.cs
- TextTreeRootTextBlock.cs
- HttpModuleActionCollection.cs
- MappingException.cs
- FormViewInsertEventArgs.cs
- ProxyHwnd.cs
- SpotLight.cs
- WrappedReader.cs
- GlyphsSerializer.cs
- ReverseQueryOperator.cs
- ManagementException.cs
- GenericUI.cs
- SerializationEventsCache.cs
- GZipDecoder.cs
- XmlDataImplementation.cs
- DataGridLinkButton.cs
- ZipIOLocalFileDataDescriptor.cs
- RequestSecurityTokenForRemoteTokenFactory.cs
- TabControlDesigner.cs
- JpegBitmapDecoder.cs
- WebRequestModulesSection.cs
- Rotation3DAnimationUsingKeyFrames.cs
- PlaceHolder.cs
- COM2PropertyPageUITypeConverter.cs
- PerfCounters.cs
- UriSection.cs
- ConnectionInterfaceCollection.cs
- MiniLockedBorderGlyph.cs
- CellQuery.cs
- Brush.cs
- Source.cs
- Utils.cs
- NativeBuffer.cs
- _RequestLifetimeSetter.cs
- CustomBindingElement.cs
- System.Data_BID.cs
- PreProcessor.cs
- OracleCommandBuilder.cs
- KeyValueConfigurationElement.cs
- SqlRemoveConstantOrderBy.cs
- XmlCodeExporter.cs
- EmptyImpersonationContext.cs
- Brush.cs
- CheckBox.cs
- precedingsibling.cs
- ObjectSpanRewriter.cs
- RequestNavigateEventArgs.cs
- AdjustableArrowCap.cs
- TemplatedWizardStep.cs
- StorageConditionPropertyMapping.cs
- RelationshipWrapper.cs
- ToolStripItemRenderEventArgs.cs
- StyleBamlRecordReader.cs
- GeometryModel3D.cs
- TextHidden.cs
- ContextProperty.cs
- DiagnosticTraceSource.cs
- ChannelManager.cs
- CompositeScriptReferenceEventArgs.cs
- InheritanceContextHelper.cs
- HighlightComponent.cs
- CodeAccessSecurityEngine.cs
- DbConnectionPoolIdentity.cs
- QilReference.cs
- ElementsClipboardData.cs
- path.cs
- LicenseManager.cs
- ResourceDescriptionAttribute.cs
- SQLInt16.cs
- PolicyException.cs
- BulletChrome.cs
- WindowsListViewItemStartMenu.cs
- Comparer.cs
- DefaultTextStore.cs
- DataGridHelper.cs
- XmlUtil.cs