Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Automation / Peers / TreeViewItemAutomationPeer.cs / 1 / TreeViewItemAutomationPeer.cs
using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class TreeViewItemAutomationPeer : FrameworkElementAutomationPeer, IExpandCollapseProvider, ISelectionItemProvider, IScrollItemProvider { /// public TreeViewItemAutomationPeer(TreeViewItem owner): base(owner) { } /// override protected string GetClassNameCore() { return "TreeViewItem"; } /// override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.TreeItem; } /// override public object GetPattern(PatternInterface patternInterface) { if (patternInterface == PatternInterface.ExpandCollapse) { return this; } else if (patternInterface == PatternInterface.SelectionItem) { return this; } else if (patternInterface == PatternInterface.ScrollItem) { return this; } return null; } /// void IExpandCollapseProvider.Expand() { if(!IsEnabled()) throw new ElementNotEnabledException(); TreeViewItem treeViewItem = (TreeViewItem)Owner; if (!treeViewItem.HasItems) { throw new InvalidOperationException(SR.Get(SRID.UIA_OperationCannotBePerformed)); } treeViewItem.IsExpanded = true; } /// void IExpandCollapseProvider.Collapse() { if(!IsEnabled()) throw new ElementNotEnabledException(); TreeViewItem treeViewItem = (TreeViewItem)Owner; if (!treeViewItem.HasItems) { throw new InvalidOperationException(SR.Get(SRID.UIA_OperationCannotBePerformed)); } treeViewItem.IsExpanded = false; } ExpandCollapseState IExpandCollapseProvider.ExpandCollapseState { get { TreeViewItem treeViewItem = (TreeViewItem)Owner; if (treeViewItem.HasItems) return treeViewItem.IsExpanded ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed; else return ExpandCollapseState.LeafNode; } } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseExpandCollapseAutomationEvent(bool oldValue, bool newValue) { RaisePropertyChangedEvent( ExpandCollapsePatternIdentifiers.ExpandCollapseStateProperty, oldValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed, newValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed); } #region ISelectionItemProvider ////// Selects this element, removing any other element from the selection. /// void ISelectionItemProvider.Select() { ((TreeViewItem)Owner).IsSelected = true; } ////// Selects this item. /// void ISelectionItemProvider.AddToSelection() { TreeView treeView = ((TreeViewItem)Owner).ParentTreeView; // If TreeView already has a selected item different from current - we cannot add to selection and throw if (treeView == null || (treeView.SelectedItem != null && treeView.SelectedContainer != Owner)) { throw new InvalidOperationException(SR.Get(SRID.UIA_OperationCannotBePerformed)); } ((TreeViewItem)Owner).IsSelected = true; } ////// Unselects this item. /// void ISelectionItemProvider.RemoveFromSelection() { ((TreeViewItem)Owner).IsSelected = false; } ////// Returns whether the item is selected. /// bool ISelectionItemProvider.IsSelected { get { return ((TreeViewItem)Owner).IsSelected; } } ////// The logical element that supports the SelectionPattern for this item. /// IRawElementProviderSimple ISelectionItemProvider.SelectionContainer { get { ItemsControl parent = ((TreeViewItem)Owner).ParentItemsControl; if (parent != null) { AutomationPeer peer = UIElementAutomationPeer.FromElement(parent); if (peer != null) return ProviderFromPeer(peer); } return null; } } void IScrollItemProvider.ScrollIntoView() { ((TreeViewItem)Owner).BringIntoView(); } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseAutomationIsSelectedChanged(bool isSelected) { RaisePropertyChangedEvent( SelectionItemPatternIdentifiers.IsSelectedProperty, !isSelected, isSelected); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
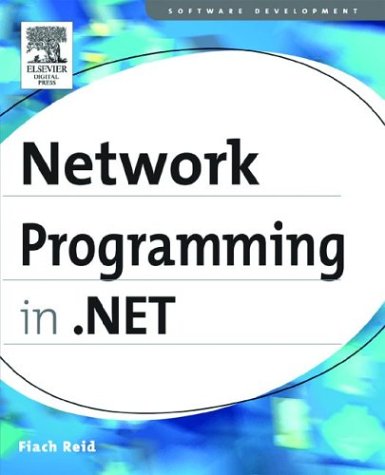
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesigntimeLicenseContextSerializer.cs
- PeerName.cs
- PointF.cs
- TimeSpanStorage.cs
- SapiRecoContext.cs
- HtmlInputControl.cs
- MediaPlayerState.cs
- EncryptedKeyIdentifierClause.cs
- XmlNamedNodeMap.cs
- CompiledELinqQueryState.cs
- ResourceDescriptionAttribute.cs
- FtpRequestCacheValidator.cs
- Helper.cs
- QilVisitor.cs
- AssociationTypeEmitter.cs
- WindowsScrollBarBits.cs
- HTMLTextWriter.cs
- InteropAutomationProvider.cs
- UrlMappingsSection.cs
- SharedPersonalizationStateInfo.cs
- ProxyHwnd.cs
- PageCache.cs
- ToolStripMenuItemDesigner.cs
- TextEditorParagraphs.cs
- DataSourceConverter.cs
- HtmlInputSubmit.cs
- HtmlInputFile.cs
- BitStack.cs
- PeerNameResolver.cs
- XamlTreeBuilder.cs
- CanExecuteRoutedEventArgs.cs
- SocketPermission.cs
- SqlGenericUtil.cs
- XmlTypeMapping.cs
- HttpProcessUtility.cs
- XmlArrayItemAttributes.cs
- ListViewGroup.cs
- SeekStoryboard.cs
- TemplateControlParser.cs
- CapabilitiesRule.cs
- RC2CryptoServiceProvider.cs
- WebEvents.cs
- TabControl.cs
- SerializationException.cs
- TypeBuilderInstantiation.cs
- XPathAncestorIterator.cs
- HttpClientCertificate.cs
- ChtmlTextWriter.cs
- TextParaLineResult.cs
- PolicyStatement.cs
- Int32Rect.cs
- SmtpNegotiateAuthenticationModule.cs
- XmlSchemaDocumentation.cs
- HierarchicalDataSourceControl.cs
- HttpConfigurationSystem.cs
- RegularExpressionValidator.cs
- ExpressionWriter.cs
- CurrentTimeZone.cs
- ProtocolsSection.cs
- SafeSystemMetrics.cs
- XsltOutput.cs
- NativeMethods.cs
- ConfigurationSchemaErrors.cs
- FactoryGenerator.cs
- StylusShape.cs
- SID.cs
- ReturnValue.cs
- altserialization.cs
- ButtonColumn.cs
- ImageBrush.cs
- ConstraintStruct.cs
- XsdCachingReader.cs
- ChannelTracker.cs
- NativeMethods.cs
- AutomationIdentifierGuids.cs
- SingleAnimationBase.cs
- GC.cs
- SHA512.cs
- WindowsEditBox.cs
- LayoutManager.cs
- FigureHelper.cs
- KeyValuePair.cs
- ThumbAutomationPeer.cs
- CodeVariableDeclarationStatement.cs
- ActivationService.cs
- ParseElement.cs
- DocumentViewer.cs
- SynchronousChannelMergeEnumerator.cs
- SolidColorBrush.cs
- ToolboxComponentsCreatedEventArgs.cs
- InstanceLockQueryResult.cs
- Buffer.cs
- RuntimeHandles.cs
- ShapingEngine.cs
- DictationGrammar.cs
- ExceptionUtil.cs
- UrlPath.cs
- PolicyImporterElement.cs
- SmiContext.cs
- Assert.cs