Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / MS / Internal / Automation / EventMap.cs / 1 / EventMap.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // Accessibility event map classes are used to determine if, and how many // listeners there are for events and property changes. // // History: // 07/23/2003 : [....] Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Windows; using System.Windows.Automation; using System.Windows.Automation.Peers; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.Automation { // Manages the event map that is used to determine if there are Automation // clients interested in specific events. internal static class EventMap { private class EventInfo { internal EventInfo() { NumberOfListeners = 1; } internal int NumberOfListeners; } // Never inline, as we don't want to unnecessarily link the automation DLL. [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] private static bool IsKnownEvent(int id) { if ( id == AutomationElementIdentifiers.ToolTipOpenedEvent.Id || id == AutomationElementIdentifiers.ToolTipClosedEvent.Id || id == AutomationElementIdentifiers.MenuOpenedEvent.Id || id == AutomationElementIdentifiers.MenuClosedEvent.Id || id == AutomationElementIdentifiers.AutomationFocusChangedEvent.Id || id == InvokePatternIdentifiers.InvokedEvent.Id || id == SelectionItemPatternIdentifiers.ElementAddedToSelectionEvent.Id || id == SelectionItemPatternIdentifiers.ElementRemovedFromSelectionEvent.Id || id == SelectionItemPatternIdentifiers.ElementSelectedEvent.Id || id == SelectionPatternIdentifiers.InvalidatedEvent.Id || id == TextPatternIdentifiers.TextSelectionChangedEvent.Id || id == TextPatternIdentifiers.TextChangedEvent.Id || id == AutomationElementIdentifiers.AsyncContentLoadedEvent.Id || id == AutomationElementIdentifiers.AutomationPropertyChangedEvent.Id || id == AutomationElementIdentifiers.StructureChangedEvent.Id ) { return true; } return false; } // Never inline, as we don't want to unnecessarily link the automation DLL. [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] private static AutomationEvent GetRegisteredEventObjectHelper(AutomationEvents eventId) { AutomationEvent eventObject = null; switch(eventId) { case AutomationEvents.ToolTipOpened: eventObject = AutomationElementIdentifiers.ToolTipOpenedEvent; break; case AutomationEvents.ToolTipClosed: eventObject = AutomationElementIdentifiers.ToolTipClosedEvent; break; case AutomationEvents.MenuOpened: eventObject = AutomationElementIdentifiers.MenuOpenedEvent; break; case AutomationEvents.MenuClosed: eventObject = AutomationElementIdentifiers.MenuClosedEvent; break; case AutomationEvents.AutomationFocusChanged: eventObject = AutomationElementIdentifiers.AutomationFocusChangedEvent; break; case AutomationEvents.InvokePatternOnInvoked: eventObject = InvokePatternIdentifiers.InvokedEvent; break; case AutomationEvents.SelectionItemPatternOnElementAddedToSelection: eventObject = SelectionItemPatternIdentifiers.ElementAddedToSelectionEvent; break; case AutomationEvents.SelectionItemPatternOnElementRemovedFromSelection: eventObject = SelectionItemPatternIdentifiers.ElementRemovedFromSelectionEvent; break; case AutomationEvents.SelectionItemPatternOnElementSelected: eventObject = SelectionItemPatternIdentifiers.ElementSelectedEvent; break; case AutomationEvents.SelectionPatternOnInvalidated: eventObject = SelectionPatternIdentifiers.InvalidatedEvent; break; case AutomationEvents.TextPatternOnTextSelectionChanged: eventObject = TextPatternIdentifiers.TextSelectionChangedEvent; break; case AutomationEvents.TextPatternOnTextChanged: eventObject = TextPatternIdentifiers.TextChangedEvent; break; case AutomationEvents.AsyncContentLoaded: eventObject = AutomationElementIdentifiers.AsyncContentLoadedEvent; break; case AutomationEvents.PropertyChanged: eventObject = AutomationElementIdentifiers.AutomationPropertyChangedEvent; break; case AutomationEvents.StructureChanged: eventObject = AutomationElementIdentifiers.StructureChangedEvent; break; default: throw new ArgumentException(SR.Get(SRID.Automation_InvalidEventId), "eventId"); } if (!_eventsTable.ContainsKey(eventObject.Id)) { eventObject = null; } return (eventObject); } internal static void AddEvent(int idEvent) { if (_eventsTable == null) _eventsTable = new Hashtable(20, .1f); if (_eventsTable.ContainsKey(idEvent)) { EventInfo info = (EventInfo)_eventsTable[idEvent]; info.NumberOfListeners++; } // to avoid unbound memory allocations, // register only events that we recognize else if (IsKnownEvent(idEvent)) { _eventsTable[idEvent] = new EventInfo(); } } internal static void RemoveEvent(int idEvent) { if (_eventsTable != null) { // Decrement the count of listeners for this event if (_eventsTable.ContainsKey(idEvent)) { EventInfo info = (EventInfo)_eventsTable[idEvent]; // Update or remove the entry based on remaining listeners info.NumberOfListeners--; if (info.NumberOfListeners <= 0) { _eventsTable.Remove(idEvent); // If no more entries exist kill the table if (_eventsTable.Count == 0) { _eventsTable = null; } } } } } // Unlike GetRegisteredEvent below, // HasRegisteredEvent does NOT cause automation DLLs loading internal static bool HasRegisteredEvent(AutomationEvents eventId) { if (_eventsTable != null && _eventsTable.Count != 0) { return (GetRegisteredEventObjectHelper(eventId) != null); } return (false); } internal static AutomationEvent GetRegisteredEvent(AutomationEvents eventId) { if (_eventsTable != null && _eventsTable.Count != 0) { return (GetRegisteredEventObjectHelper(eventId)); } return (null); } private static Hashtable _eventsTable; // key=event id, data=listener count } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
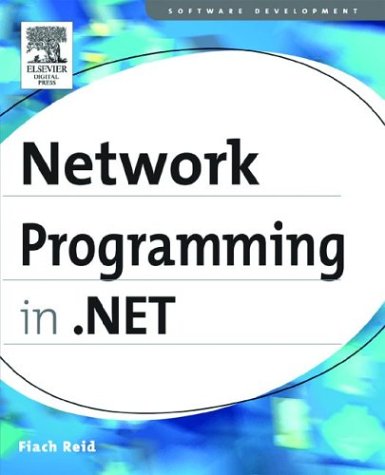
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FloaterParagraph.cs
- SqlDataSourceCache.cs
- Task.cs
- UserControlCodeDomTreeGenerator.cs
- IUnknownConstantAttribute.cs
- FactoryMaker.cs
- RelatedView.cs
- PromptEventArgs.cs
- DataGridHeaderBorder.cs
- FileAuthorizationModule.cs
- FrameAutomationPeer.cs
- HttpRuntime.cs
- DataTrigger.cs
- ListViewUpdateEventArgs.cs
- FormViewUpdateEventArgs.cs
- DictionarySurrogate.cs
- ProxySimple.cs
- DataGridViewSortCompareEventArgs.cs
- _AcceptOverlappedAsyncResult.cs
- FrameworkReadOnlyPropertyMetadata.cs
- TaskHelper.cs
- DesignerAutoFormat.cs
- RuleCache.cs
- TransactionCache.cs
- FlowNode.cs
- CatalogZone.cs
- ObjectTokenCategory.cs
- DeclarativeCatalogPart.cs
- StandardBindingImporter.cs
- AssociationTypeEmitter.cs
- TagMapInfo.cs
- EdmError.cs
- LineSegment.cs
- BCLDebug.cs
- bindurihelper.cs
- FlatButtonAppearance.cs
- CompatibleIComparer.cs
- ProcessHostMapPath.cs
- PtsPage.cs
- StringUtil.cs
- ToolStripItemTextRenderEventArgs.cs
- ContainerParaClient.cs
- CapiHashAlgorithm.cs
- RowSpanVector.cs
- TableLayoutColumnStyleCollection.cs
- ObjectSet.cs
- ToolStripItemImageRenderEventArgs.cs
- PropertyEmitter.cs
- CommandID.cs
- CookielessHelper.cs
- HScrollProperties.cs
- XmlEnumAttribute.cs
- IxmlLineInfo.cs
- EventRecord.cs
- PropertyCondition.cs
- DefaultBindingPropertyAttribute.cs
- FrameworkElementAutomationPeer.cs
- IssuedTokenClientElement.cs
- StopStoryboard.cs
- UIEndRequest.cs
- AppSettings.cs
- TimeSpanMinutesConverter.cs
- OutputCacheProfileCollection.cs
- SiteMapNode.cs
- WebException.cs
- XPathChildIterator.cs
- Int32.cs
- RectangleGeometry.cs
- MulticastIPAddressInformationCollection.cs
- ParserExtension.cs
- RadioButton.cs
- XmlEntityReference.cs
- TreeNode.cs
- TableItemStyle.cs
- SocketManager.cs
- ContravarianceAdapter.cs
- OutOfProcStateClientManager.cs
- CaseInsensitiveOrdinalStringComparer.cs
- HttpWrapper.cs
- SynchronizedRandom.cs
- PassportIdentity.cs
- CodeLabeledStatement.cs
- WebPartDisplayModeCancelEventArgs.cs
- ControlValuePropertyAttribute.cs
- BaseParser.cs
- ByteStorage.cs
- RepeatBehaviorConverter.cs
- XmlNodeComparer.cs
- DataGridColumnHeadersPresenterAutomationPeer.cs
- ProviderConnectionPoint.cs
- TraceHwndHost.cs
- Parser.cs
- tibetanshape.cs
- UnknownBitmapEncoder.cs
- PerfService.cs
- GeneralTransform.cs
- ButtonRenderer.cs
- TransformerConfigurationWizardBase.cs
- SiteOfOriginContainer.cs
- SystemPens.cs