Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Automation / Peers / DocumentAutomationPeer.cs / 1305600 / DocumentAutomationPeer.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: DocumentAutomationPeer.cs // // Description: AutomationPeer associated with flow and fixed documents. // //--------------------------------------------------------------------------- using System.Collections.Generic; // Listusing System.Security; // SecurityCritical using System.Windows.Documents; // ITextContainer using System.Windows.Interop; // HwndSource using System.Windows.Media; // Visual using MS.Internal; // PointUtil using MS.Internal.Automation; // TextAdaptor using MS.Internal.Documents; // TextContainerHelper namespace System.Windows.Automation.Peers { /// /// AutomationPeer associated with flow and fixed documents. /// public class DocumentAutomationPeer : ContentTextAutomationPeer { ////// Constructor. /// /// Owner of the AutomationPeer. public DocumentAutomationPeer(FrameworkContentElement owner) : base(owner) { if (owner is IServiceProvider) { ITextContainer textContainer = ((IServiceProvider)owner).GetService(typeof(ITextContainer)) as ITextContainer; if (textContainer != null) { _textPattern = new TextAdaptor(this, textContainer); } } } ////// Notify the peer that it has been disconnected. /// internal void OnDisconnected() { if (_textPattern != null) { _textPattern.Dispose(); _textPattern = null; } } ////// ////// /// Since DocumentAutomationPeer gives access to its content through /// TextPattern, this method always returns null. /// protected override ListGetChildrenCore() { if (_childrenStart != null && _childrenEnd != null) { ITextContainer textContainer = ((IServiceProvider)Owner).GetService(typeof(ITextContainer)) as ITextContainer; return TextContainerHelper.GetAutomationPeersFromRange(_childrenStart, _childrenEnd, textContainer.Start); } return null; } /// /// public override object GetPattern(PatternInterface patternInterface) { object returnValue = null; if (patternInterface == PatternInterface.Text) { if (_textPattern == null) { if (Owner is IServiceProvider) { ITextContainer textContainer = ((IServiceProvider)Owner).GetService(typeof(ITextContainer)) as ITextContainer; if (textContainer != null) { _textPattern = new TextAdaptor(this, textContainer); } } } returnValue = _textPattern; } else { returnValue = base.GetPattern(patternInterface); } return returnValue; } ////// /// protected override AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Document; } ////// /// ////// protected override string GetClassNameCore() { return "Document"; } /// /// protected override bool IsControlElementCore() { return true; } ////// /// ////// /// Critical - Calls PresentationSource.CriticalFromVisual to get the source for this visual /// TreatAsSafe - The returned PresenationSource object is not exposed and is only used for converting /// co-ordinates to screen space. /// [SecurityCritical, SecurityTreatAsSafe] protected override Rect GetBoundingRectangleCore() { UIElement uiScope; Rect boundingRect = CalculateBoundingRect(false, out uiScope); if (boundingRect != Rect.Empty && uiScope != null) { HwndSource hwndSource = PresentationSource.CriticalFromVisual(uiScope) as HwndSource; if (hwndSource != null) { boundingRect = PointUtil.ElementToRoot(boundingRect, uiScope, hwndSource); boundingRect = PointUtil.RootToClient(boundingRect, hwndSource); boundingRect = PointUtil.ClientToScreen(boundingRect, hwndSource); } } return boundingRect; } ////// ////// /// Critical - Calls PresentationSource.CriticalFromVisual to get the source for this visual /// TreatAsSafe - The returned PresenationSource object is not exposed and is only used for converting /// co-ordinates to screen space. /// [SecurityCritical, SecurityTreatAsSafe] protected override Point GetClickablePointCore() { Point point = new Point(); UIElement uiScope; Rect boundingRect = CalculateBoundingRect(true, out uiScope); if (boundingRect != Rect.Empty && uiScope != null) { HwndSource hwndSource = PresentationSource.CriticalFromVisual(uiScope) as HwndSource; if (hwndSource != null) { boundingRect = PointUtil.ElementToRoot(boundingRect, uiScope, hwndSource); boundingRect = PointUtil.RootToClient(boundingRect, hwndSource); boundingRect = PointUtil.ClientToScreen(boundingRect, hwndSource); point = new Point(boundingRect.Left + boundingRect.Width * 0.1, boundingRect.Top + boundingRect.Height * 0.1); } } return point; } ////// protected override bool IsOffscreenCore() { UIElement uiScope; Rect boundingRect = CalculateBoundingRect(true, out uiScope); return (boundingRect == Rect.Empty || uiScope == null); } ////// /// Gets collection of AutomationPeers for given text range. /// internal override ListGetAutomationPeersFromRange(ITextPointer start, ITextPointer end) { _childrenStart = start.CreatePointer(); _childrenEnd = end.CreatePointer(); ResetChildrenCache(); return GetChildren(); } /// /// Calculate visible rectangle. /// private Rect CalculateBoundingRect(bool clipToVisible, out UIElement uiScope) { uiScope = null; Rect boundingRect = Rect.Empty; if (Owner is IServiceProvider) { ITextContainer textContainer = ((IServiceProvider)Owner).GetService(typeof(ITextContainer)) as ITextContainer; ITextView textView = (textContainer != null) ? textContainer.TextView : null; if (textView != null) { // Make sure TextView is updated if (!textView.IsValid) { if (!textView.Validate()) { textView = null; } if (textView != null && !textView.IsValid) { textView = null; } } // Get bounding rect from TextView. if (textView != null) { boundingRect = new Rect(textView.RenderScope.RenderSize); uiScope = textView.RenderScope; // Compute visible portion of the rectangle. if (clipToVisible) { Visual visual = textView.RenderScope; while (visual != null && boundingRect != Rect.Empty) { if (VisualTreeHelper.GetClip(visual) != null) { GeneralTransform transform = textView.RenderScope.TransformToAncestor(visual).Inverse; // Safer version of transform to descendent (doing the inverse ourself), // we want the rect inside of our space. (Which is always rectangular and much nicer to work with) if (transform != null) { Rect clipBounds = VisualTreeHelper.GetClip(visual).Bounds; clipBounds = transform.TransformBounds(clipBounds); boundingRect.Intersect(clipBounds); } else { // No visibility if non-invertable transform exists. boundingRect = Rect.Empty; } } visual = VisualTreeHelper.GetParent(visual) as Visual; } } } } } return boundingRect; } private ITextPointer _childrenStart; private ITextPointer _childrenEnd; private TextAdaptor _textPattern; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
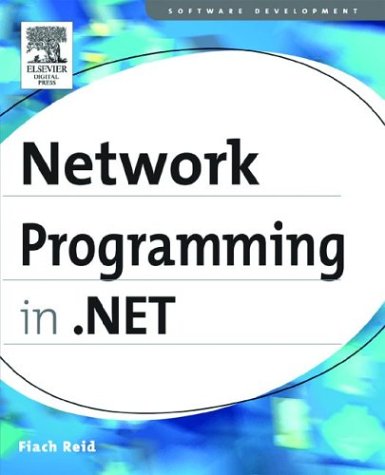
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PeerSecurityHelpers.cs
- Size3DConverter.cs
- RawTextInputReport.cs
- EndpointDiscoveryMetadata.cs
- Variant.cs
- MouseCaptureWithinProperty.cs
- XPathDocumentNavigator.cs
- TokenizerHelper.cs
- DrawingContextWalker.cs
- IntegerValidator.cs
- ColorMatrix.cs
- SelectionPattern.cs
- Debugger.cs
- EtwTrackingParticipant.cs
- MetadataItemEmitter.cs
- SystemIPInterfaceStatistics.cs
- StickyNoteHelper.cs
- WebPartEditVerb.cs
- Label.cs
- DataSourceCache.cs
- DataList.cs
- MimeWriter.cs
- ToolBarButtonClickEvent.cs
- BamlLocalizabilityResolver.cs
- WSSecurityPolicy11.cs
- smtppermission.cs
- TcpHostedTransportConfiguration.cs
- Calendar.cs
- StreamGeometryContext.cs
- TableLayoutPanel.cs
- TrustVersion.cs
- WebPartDisplayModeEventArgs.cs
- AutoCompleteStringCollection.cs
- DataGridViewCheckBoxCell.cs
- RadioButtonRenderer.cs
- InteropTrackingRecord.cs
- XmlSchemaSimpleTypeList.cs
- webbrowsersite.cs
- CodeTypeOfExpression.cs
- XpsFilter.cs
- DataError.cs
- SystemKeyConverter.cs
- XmlSchemaSimpleContent.cs
- TabItemAutomationPeer.cs
- TreeWalkHelper.cs
- SpAudioStreamWrapper.cs
- PropertyStore.cs
- OracleDataAdapter.cs
- ColumnHeaderConverter.cs
- InternalPermissions.cs
- TrustLevelCollection.cs
- DependentList.cs
- MailDefinition.cs
- DataTableMappingCollection.cs
- CalloutQueueItem.cs
- InfoCardRSAPKCS1SignatureDeformatter.cs
- Baml2006KeyRecord.cs
- StatusBarPanel.cs
- initElementDictionary.cs
- XPathDescendantIterator.cs
- DropDownList.cs
- BufferModesCollection.cs
- XmlStringTable.cs
- GetWinFXPath.cs
- SpellerStatusTable.cs
- SafeTimerHandle.cs
- TextInfo.cs
- ExclusiveTcpListener.cs
- MediaCommands.cs
- DesignTimeParseData.cs
- ChildTable.cs
- SimpleExpression.cs
- XmlSchemaSimpleTypeUnion.cs
- BaseDataListPage.cs
- TypeConverterBase.cs
- Invariant.cs
- RealizationDrawingContextWalker.cs
- ThreadExceptionDialog.cs
- CodeDefaultValueExpression.cs
- RefreshPropertiesAttribute.cs
- SessionStateItemCollection.cs
- DataViewListener.cs
- RectangleF.cs
- StringKeyFrameCollection.cs
- Vector3D.cs
- XmlReflectionImporter.cs
- WebBrowserBase.cs
- XmlArrayItemAttributes.cs
- RoutingConfiguration.cs
- OleDbErrorCollection.cs
- XmlTypeMapping.cs
- FunctionCommandText.cs
- TextEffectCollection.cs
- ListBoxDesigner.cs
- CancellationHandlerDesigner.cs
- SystemIcmpV6Statistics.cs
- SecurityDescriptor.cs
- UrlAuthFailureHandler.cs
- HtmlLink.cs
- SemanticBasicElement.cs