Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / CqlGeneration / SlotInfo.cs / 1305376 / SlotInfo.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Data.Mapping.ViewGeneration.Structures; using System.Text; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.CqlGeneration { // A class that keeps track of slot information in a CqlBlock internal class SlotInfo : InternalBase { #region Constructor // effects: Creates a SlotInfo for a CqlBlock X with information // about whether this slot is needed by X's parent // (isRequiredByParent), whether X projects it (isProjected) along with // the slot value (slot) and the output member path (for // regular/non-boolean slots) for the slot internal SlotInfo(bool isRequiredByParent, bool isProjected, ProjectedSlot slot, MemberPath memberPath) : this(isRequiredByParent, isProjected, slot, memberPath, false /* enforceNotNull */) { } // We need to ensure that _from variables are never null since // view generation uses 2-valued boolean logic. // If enforceNotNull=true, the generated CQL adds a condition (AND slot NOT NULL) // This flag is used only for boolean slots. internal SlotInfo(bool isRequiredByParent, bool isProjected, ProjectedSlot slot, MemberPath memberPath, bool enforceNotNull) { m_isRequiredByParent = isRequiredByParent; m_isProjected = isProjected; m_slot = slot; m_memberPath = memberPath; m_enforceNotNull = enforceNotNull; Debug.Assert(false == m_isRequiredByParent || m_slot != null, "Required slots cannot be null"); Debug.Assert(m_slot is AliasedSlot || (m_slot == null && m_memberPath == null) || // unused boolean slot (m_slot is BooleanProjectedSlot) == (m_memberPath == null), "If slot is boolean slot, there is no member path for it and vice-versa"); } #endregion #region Fields private bool m_isRequiredByParent; // If slot is required by the parent private bool m_isProjected; // If the node is capable of projecting this slot private ProjectedSlot m_slot; // The slot represented by this SlotInfo private MemberPath m_memberPath; // The output member path of this slot private bool m_enforceNotNull; // Whether to add AND NOT NULL to CQL #endregion #region Properties // effects: Returns true iff this slot is required by the CqlBlock's parent internal bool IsRequiredByParent { get { return m_isRequiredByParent; } } // effects: Returns true iff this slot is projected by this CqlBlock internal bool IsProjected { get { return m_isProjected; } } // effects: Returns the output memberpath of this slot internal MemberPath MemberPath { get { return m_memberPath; } } // effects: Returns the slot corresponfing to this object internal ProjectedSlot SlotValue { get { return m_slot; } } // effects: Returns the Cql Alias for this slot, e.g., CPerson1_Pid, _from0 internal string CqlFieldAlias { get { return m_slot.CqlFieldAlias(m_memberPath); } } // effects: Returns true if CQL generated for slot needs to have an extra AND IS NOT NULL condition internal bool IsEnforcedNotNull { get { return m_enforceNotNull; } } #endregion #region Methods // effects: Sets the isrequiredbyparent to false. Note we don't have // a setter because we don't want people to set this field to true // after the object has been created internal void ResetIsRequiredByParent() { m_isRequiredByParent = false; } // effects: Modifies builder to contain a Cql representation of this // and returns the modified builder. For different slots, the result // is different, e.g., _from0, CPerson1.pid, TREAT(....) internal StringBuilder AsCql(StringBuilder builder, string blockAlias, int indentLevel) { if (m_enforceNotNull) { builder.Append('('); m_slot.AsCql(builder, m_memberPath, blockAlias, indentLevel); builder.Append(" AND "); m_slot.AsCql(builder, m_memberPath, blockAlias, indentLevel); builder.Append(" IS NOT NULL)"); } else { m_slot.AsCql(builder, m_memberPath, blockAlias, indentLevel); } return builder; } internal override void ToCompactString(StringBuilder builder) { if (m_slot != null) { builder.Append(CqlFieldAlias); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
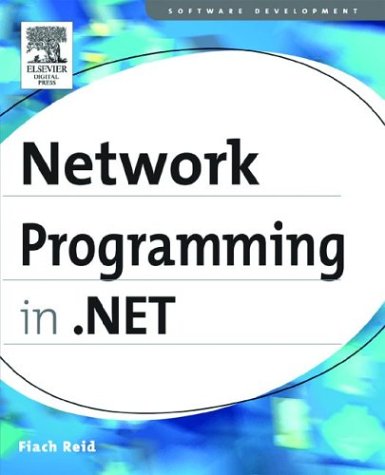
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TCPListener.cs
- WebUtil.cs
- BuildResult.cs
- NonSerializedAttribute.cs
- BuildDependencySet.cs
- SpotLight.cs
- CodeDomDecompiler.cs
- ValidationError.cs
- DLinqDataModelProvider.cs
- BindingWorker.cs
- AssociatedControlConverter.cs
- Processor.cs
- TextTreeTextNode.cs
- AppDomainAttributes.cs
- PropertyInfo.cs
- CachedFontFamily.cs
- ValuePattern.cs
- SslStream.cs
- SpecialTypeDataContract.cs
- GroupItemAutomationPeer.cs
- RegexWriter.cs
- EventSourceCreationData.cs
- OleDbReferenceCollection.cs
- InkCollectionBehavior.cs
- AppDomainGrammarProxy.cs
- StrongNamePublicKeyBlob.cs
- PopOutPanel.cs
- HMACMD5.cs
- TreeNode.cs
- EventListener.cs
- XamlWriter.cs
- MemberProjectedSlot.cs
- WebPartRestoreVerb.cs
- WebCategoryAttribute.cs
- EncoderNLS.cs
- AppSettingsReader.cs
- SessionStateModule.cs
- SqlNotificationEventArgs.cs
- XmlNotation.cs
- MarshalByValueComponent.cs
- hresults.cs
- SqlDataSourceStatusEventArgs.cs
- TriState.cs
- XmlSerializerSection.cs
- UserControl.cs
- SoapIgnoreAttribute.cs
- ImmutableObjectAttribute.cs
- WinFormsComponentEditor.cs
- CodeSnippetTypeMember.cs
- HtmlWindowCollection.cs
- RuntimeIdentifierPropertyAttribute.cs
- ProxyGenerationError.cs
- _HTTPDateParse.cs
- PageStatePersister.cs
- EnumMember.cs
- PersonalizationAdministration.cs
- AuthenticationService.cs
- WebContext.cs
- AssemblyBuilder.cs
- DateTimeValueSerializer.cs
- ZipArchive.cs
- TagNameToTypeMapper.cs
- Latin1Encoding.cs
- PeerNameResolver.cs
- SizeConverter.cs
- ProxyAttribute.cs
- FileCodeGroup.cs
- MissingManifestResourceException.cs
- CustomErrorsSection.cs
- Span.cs
- XmlILCommand.cs
- XmlSchemaException.cs
- XmlSchemaSimpleTypeUnion.cs
- HotSpot.cs
- UInt32Converter.cs
- WinEventHandler.cs
- ClosableStream.cs
- SoapEnumAttribute.cs
- Binding.cs
- OperationGenerator.cs
- ObjectConverter.cs
- RepeatBehavior.cs
- BoundField.cs
- MonitorWrapper.cs
- Processor.cs
- DataControlButton.cs
- RightsController.cs
- Descriptor.cs
- GenericTextProperties.cs
- InstalledFontCollection.cs
- StatusBarItemAutomationPeer.cs
- JsonReader.cs
- XmlSiteMapProvider.cs
- TableLayoutStyleCollection.cs
- CacheVirtualItemsEvent.cs
- RemoteX509AsymmetricSecurityKey.cs
- ElementUtil.cs
- Bits.cs
- WebEventCodes.cs
- ZoneButton.cs