Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / Primitives / CalendarButton.cs / 1305600 / CalendarButton.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows; using System.Windows.Automation.Peers; using System.Windows.Controls; using System.Windows.Data; namespace System.Windows.Controls.Primitives { ////// Represents a button control used in Calendar Control, which reacts to the Click event. /// public sealed class CalendarButton : Button { ////// Static constructor /// static CalendarButton() { DefaultStyleKeyProperty.OverrideMetadata(typeof(CalendarButton), new FrameworkPropertyMetadata(typeof(CalendarButton))); } ////// Represents the CalendarButton that is used in Calendar Control. /// public CalendarButton() : base() { } #region Public Properties #region HasSelectedDays internal static readonly DependencyPropertyKey HasSelectedDaysPropertyKey = DependencyProperty.RegisterReadOnly( "HasSelectedDays", typeof(bool), typeof(CalendarButton), new FrameworkPropertyMetadata(false, new PropertyChangedCallback(OnVisualStatePropertyChanged))); ////// Dependency property field for HasSelectedDays property /// public static readonly DependencyProperty HasSelectedDaysProperty = HasSelectedDaysPropertyKey.DependencyProperty; ////// True if the CalendarButton represents a date range containing the display date /// public bool HasSelectedDays { get { return (bool)GetValue(HasSelectedDaysProperty); } internal set { SetValue(HasSelectedDaysPropertyKey, value); } } #endregion HasSelectedDays #region IsInactive internal static readonly DependencyPropertyKey IsInactivePropertyKey = DependencyProperty.RegisterReadOnly( "IsInactive", typeof(bool), typeof(CalendarButton), new FrameworkPropertyMetadata(false, new PropertyChangedCallback(OnVisualStatePropertyChanged))); ////// Dependency property field for IsInactive property /// public static readonly DependencyProperty IsInactiveProperty = IsInactivePropertyKey.DependencyProperty; ////// True if the CalendarButton represents /// a month that falls outside the current year /// or /// a year that falls outside the current decade /// public bool IsInactive { get { return (bool)GetValue(IsInactiveProperty); } internal set { SetValue(IsInactivePropertyKey, value); } } #endregion IsInactive #endregion Public Properties #region Internal Properties internal Calendar Owner { get; set; } #endregion Internal Properties #region Public Methods #endregion Public Methods #region Protected Methods ////// Change to the correct visual state for the button. /// /// /// true to use transitions when updating the visual state, false to /// snap directly to the new visual state. /// internal override void ChangeVisualState(bool useTransitions) { // Update the SelectionStates group if (HasSelectedDays) { VisualStates.GoToState(this, useTransitions, VisualStates.StateSelected, VisualStates.StateUnselected); } else { VisualStateManager.GoToState(this, VisualStates.StateUnselected, useTransitions); } // Update the ActiveStates group if (!IsInactive) { VisualStates.GoToState(this, useTransitions, VisualStates.StateActive, VisualStates.StateInactive); } else { VisualStateManager.GoToState(this, VisualStates.StateInactive, useTransitions); } // Update the FocusStates group if (IsKeyboardFocused) { VisualStates.GoToState(this, useTransitions, VisualStates.StateCalendarButtonFocused, VisualStates.StateCalendarButtonUnfocused); } else { VisualStateManager.GoToState(this, VisualStates.StateCalendarButtonUnfocused, useTransitions); } base.ChangeVisualState(useTransitions); } ////// Creates the automation peer for the DayButton. /// ///protected override AutomationPeer OnCreateAutomationPeer() { return new CalendarButtonAutomationPeer(this); } #endregion Protected Methods #region Internal Methods internal void SetContentInternal(string value) { SetCurrentValueInternal(ContentControl.ContentProperty, value); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows; using System.Windows.Automation.Peers; using System.Windows.Controls; using System.Windows.Data; namespace System.Windows.Controls.Primitives { /// /// Represents a button control used in Calendar Control, which reacts to the Click event. /// public sealed class CalendarButton : Button { ////// Static constructor /// static CalendarButton() { DefaultStyleKeyProperty.OverrideMetadata(typeof(CalendarButton), new FrameworkPropertyMetadata(typeof(CalendarButton))); } ////// Represents the CalendarButton that is used in Calendar Control. /// public CalendarButton() : base() { } #region Public Properties #region HasSelectedDays internal static readonly DependencyPropertyKey HasSelectedDaysPropertyKey = DependencyProperty.RegisterReadOnly( "HasSelectedDays", typeof(bool), typeof(CalendarButton), new FrameworkPropertyMetadata(false, new PropertyChangedCallback(OnVisualStatePropertyChanged))); ////// Dependency property field for HasSelectedDays property /// public static readonly DependencyProperty HasSelectedDaysProperty = HasSelectedDaysPropertyKey.DependencyProperty; ////// True if the CalendarButton represents a date range containing the display date /// public bool HasSelectedDays { get { return (bool)GetValue(HasSelectedDaysProperty); } internal set { SetValue(HasSelectedDaysPropertyKey, value); } } #endregion HasSelectedDays #region IsInactive internal static readonly DependencyPropertyKey IsInactivePropertyKey = DependencyProperty.RegisterReadOnly( "IsInactive", typeof(bool), typeof(CalendarButton), new FrameworkPropertyMetadata(false, new PropertyChangedCallback(OnVisualStatePropertyChanged))); ////// Dependency property field for IsInactive property /// public static readonly DependencyProperty IsInactiveProperty = IsInactivePropertyKey.DependencyProperty; ////// True if the CalendarButton represents /// a month that falls outside the current year /// or /// a year that falls outside the current decade /// public bool IsInactive { get { return (bool)GetValue(IsInactiveProperty); } internal set { SetValue(IsInactivePropertyKey, value); } } #endregion IsInactive #endregion Public Properties #region Internal Properties internal Calendar Owner { get; set; } #endregion Internal Properties #region Public Methods #endregion Public Methods #region Protected Methods ////// Change to the correct visual state for the button. /// /// /// true to use transitions when updating the visual state, false to /// snap directly to the new visual state. /// internal override void ChangeVisualState(bool useTransitions) { // Update the SelectionStates group if (HasSelectedDays) { VisualStates.GoToState(this, useTransitions, VisualStates.StateSelected, VisualStates.StateUnselected); } else { VisualStateManager.GoToState(this, VisualStates.StateUnselected, useTransitions); } // Update the ActiveStates group if (!IsInactive) { VisualStates.GoToState(this, useTransitions, VisualStates.StateActive, VisualStates.StateInactive); } else { VisualStateManager.GoToState(this, VisualStates.StateInactive, useTransitions); } // Update the FocusStates group if (IsKeyboardFocused) { VisualStates.GoToState(this, useTransitions, VisualStates.StateCalendarButtonFocused, VisualStates.StateCalendarButtonUnfocused); } else { VisualStateManager.GoToState(this, VisualStates.StateCalendarButtonUnfocused, useTransitions); } base.ChangeVisualState(useTransitions); } ////// Creates the automation peer for the DayButton. /// ///protected override AutomationPeer OnCreateAutomationPeer() { return new CalendarButtonAutomationPeer(this); } #endregion Protected Methods #region Internal Methods internal void SetContentInternal(string value) { SetCurrentValueInternal(ContentControl.ContentProperty, value); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
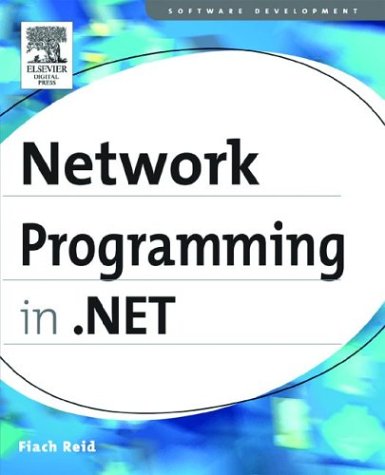
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HitTestWithGeometryDrawingContextWalker.cs
- DataGridViewTopLeftHeaderCell.cs
- EntityDataSourceDesignerHelper.cs
- ConditionalAttribute.cs
- UpDownEvent.cs
- CodeCommentStatementCollection.cs
- ValidationHelper.cs
- LambdaCompiler.Statements.cs
- LinqDataSource.cs
- DbConnectionClosed.cs
- QilReference.cs
- TdsParameterSetter.cs
- PointUtil.cs
- Component.cs
- BackStopAuthenticationModule.cs
- MailWebEventProvider.cs
- DataGridSortCommandEventArgs.cs
- DoWorkEventArgs.cs
- DbParameterHelper.cs
- CodeTypeReferenceCollection.cs
- HostingEnvironmentException.cs
- WorkflowTransactionService.cs
- QilTargetType.cs
- InstancePersistenceException.cs
- LinqDataSourceContextEventArgs.cs
- ChildDocumentBlock.cs
- mda.cs
- ToolboxItemFilterAttribute.cs
- DoubleAnimationUsingKeyFrames.cs
- XdrBuilder.cs
- UniqueConstraint.cs
- IntSecurity.cs
- StandardOleMarshalObject.cs
- DataTemplate.cs
- Rect3D.cs
- PropertyItem.cs
- AggregatePushdown.cs
- GlobalizationSection.cs
- XmlQualifiedName.cs
- DockPattern.cs
- IdentityReference.cs
- InputLanguageCollection.cs
- DataGridViewComboBoxColumn.cs
- ShellProvider.cs
- ReadOnlyActivityGlyph.cs
- RegisteredDisposeScript.cs
- SignedXml.cs
- Sequence.cs
- XslCompiledTransform.cs
- DesignerSerializationManager.cs
- SByteStorage.cs
- GuidelineSet.cs
- ComponentGuaranteesAttribute.cs
- JsonClassDataContract.cs
- TableItemPattern.cs
- TextDocumentView.cs
- GPPOINTF.cs
- future.cs
- sqlstateclientmanager.cs
- XmlImplementation.cs
- TagPrefixInfo.cs
- BoolExpressionVisitors.cs
- SchemaAttDef.cs
- OdbcTransaction.cs
- TransformerConfigurationWizardBase.cs
- FileDialog.cs
- HandleRef.cs
- DocumentViewerHelper.cs
- GeneralTransform3D.cs
- ContainsSearchOperator.cs
- FrameworkContentElement.cs
- UIAgentInitializationException.cs
- DateTimeValueSerializer.cs
- SecurityProtocolCorrelationState.cs
- UsernameTokenFactoryCredential.cs
- MsmqOutputSessionChannel.cs
- CapiHashAlgorithm.cs
- _IPv6Address.cs
- XmlSchemaComplexContentRestriction.cs
- CatalogZone.cs
- NameTable.cs
- OleServicesContext.cs
- DataGridViewLinkCell.cs
- Sentence.cs
- SID.cs
- EdmProperty.cs
- LogLogRecord.cs
- LateBoundBitmapDecoder.cs
- EnumBuilder.cs
- RadioButtonFlatAdapter.cs
- ItemsChangedEventArgs.cs
- ProbeMatches11.cs
- BindingMAnagerBase.cs
- DragEvent.cs
- ColorTranslator.cs
- OutputCacheProfile.cs
- RequestBringIntoViewEventArgs.cs
- IndexOutOfRangeException.cs
- ByteStream.cs
- XmlQualifiedNameTest.cs