Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / PtsHost / TableParagraph.cs / 1305600 / TableParagraph.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Implementation of the PTS paragraph corresponding to table. // // History: // 05/30/2003 : olego - Created // //--------------------------------------------------------------------------- #pragma warning disable 1634, 1691 // avoid generating warnings about unknown // message numbers and unknown pragmas for PRESharp contol using MS.Internal.Documents; using MS.Internal.PtsTable; using MS.Internal.Text; using MS.Utility; using System; using System.Collections; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using System.Windows.Media; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { ////// Table paragraph PTS object implementation /// internal sealed class TableParagraph : BaseParagraph { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal TableParagraph(DependencyObject element, StructuralCache structuralCache) : base(element, structuralCache) { Table.TableStructureChanged += new System.EventHandler(TableStructureChanged); } // ------------------------------------------------------------------ // IDisposable.Dispose // ----------------------------------------------------------------- public override void Dispose() { Table.TableStructureChanged -= new System.EventHandler(TableStructureChanged); BaseParagraph paraChild = _firstChild; while (paraChild != null) { BaseParagraph para = paraChild; paraChild = paraChild.Next; para.Dispose(); para.Next = null; para.Previous = null; } _firstChild = null; base.Dispose(); } #endregion Constructors //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion Protected Methods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Collapse Margins /// /// Para client /// input margin collapsing state /// current direction (of the track, in which margin collapsing is happening) /// suppress empty space at the top of page /// dvr, calculated based on margin collapsing state internal override void CollapseMargin( BaseParaClient paraClient, // IN: MarginCollapsingState mcs, // IN: input margin collapsing state uint fswdir, // IN: current direction (of the track, in which margin collapsing is happening) bool suppressTopSpace, // IN: suppress empty space at the top of page out int dvr) // OUT: dvr, calculated based on margin collapsing state { if (suppressTopSpace && (StructuralCache.CurrentFormatContext.FinitePage || mcs == null)) dvr = 0; else { MbpInfo mbp = MbpInfo.FromElement(Table); MarginCollapsingState mcsOut = null; MarginCollapsingState.CollapseTopMargin(PtsContext, mbp, mcs, out mcsOut, out dvr); if (mcsOut != null) { dvr = mcsOut.Margin; mcsOut.Dispose(); mcsOut = null; } } } ////// GetParaProperties. /// /// paragraph properties internal override void GetParaProperties( ref PTS.FSPAP fspap) // OUT: paragraph properties { fspap.idobj = PtsHost.TableParagraphId; // fspap.fKeepWithNext = PTS.False; fspap.fBreakPageBefore = PTS.False; fspap.fBreakColumnBefore = PTS.False; } ////// CreateParaclient /// /// Opaque to PTS paragraph client internal override void CreateParaclient( out IntPtr pfsparaclient) // OUT: opaque to PTS paragraph client { #pragma warning disable 6518 // Disable PRESharp warning 6518. TableParaClient is an UnmamangedHandle, that adds itself // to HandleMapper that holds a reference to it. PTS manages lifetime of this object, and // calls DestroyParaclient to get rid of it. DestroyParaclient will call Dispose() on the object // and remove it from HandleMapper. TableParaClient paraClient = new TableParaClient(this); pfsparaclient = paraClient.Handle; #pragma warning restore 6518 } ////// GetTableProperties /// /// Direction of Track /// Properties of the table internal void GetTableProperties( uint fswdirTrack, // IN: direction of Track out PTS.FSTABLEOBJPROPS fstableobjprops)// OUT: properties of the table { fstableobjprops = new PTS.FSTABLEOBJPROPS(); fstableobjprops.fskclear = PTS.FSKCLEAR.fskclearNone; fstableobjprops.ktablealignment = PTS.FSKTABLEOBJALIGNMENT.fsktableobjAlignLeft; fstableobjprops.fFloat = PTS.False; fstableobjprops.fskwr = PTS.FSKWRAP.fskwrBoth; fstableobjprops.fDelayNoProgress = PTS.False; fstableobjprops.dvrCaptionTop = 0; fstableobjprops.dvrCaptionBottom = 0; fstableobjprops.durCaptionLeft = 0; fstableobjprops.durCaptionRight = 0; fstableobjprops.fswdirTable = PTS.FlowDirectionToFswdir((FlowDirection)Element.GetValue(FrameworkElement.FlowDirectionProperty)); } ////// GetMCSClientAfterTable /// /// Direction of Track /// Margin collapsing state /// Margin collapsing state internal void GetMCSClientAfterTable( uint fswdirTrack, IntPtr pmcsclientIn, out IntPtr ppmcsclientOut) { ppmcsclientOut = IntPtr.Zero; MbpInfo mbp = MbpInfo.FromElement(Table); MarginCollapsingState mcs = null; if (pmcsclientIn != IntPtr.Zero) mcs = PtsContext.HandleToObject(pmcsclientIn) as MarginCollapsingState; MarginCollapsingState mcsNew = null; int margin; MarginCollapsingState.CollapseBottomMargin(PtsContext, mbp, mcs, out mcsNew, out margin); if (mcsNew != null) ppmcsclientOut = mcsNew.Handle; } ////// GetFirstHeaderRow /// /// Repeated header flag /// Indication that first header row is found /// First header row name internal void GetFirstHeaderRow( int fRepeatedHeader, out int fFound, out IntPtr pnmFirstHeaderRow) { fFound = PTS.False; pnmFirstHeaderRow = IntPtr.Zero; } ////// GetNextHeaderRow /// /// Repeated header flag /// Previous header row /// Indication that header row is found /// Header row name internal void GetNextHeaderRow( int fRepeatedHeader, IntPtr nmHeaderRow, out int fFound, out IntPtr pnmNextHeaderRow) { fFound = PTS.False; pnmNextHeaderRow = IntPtr.Zero; } ////// GetFirstFooterRow /// /// Repeated footer flag /// Indication that first header row is found /// First footer row name internal void GetFirstFooterRow( int fRepeatedFooter, out int fFound, out IntPtr pnmFirstFooterRow) { fFound = PTS.False; pnmFirstFooterRow = IntPtr.Zero; } ////// GetNextFooterRow /// /// Repeated footer flag /// Previous footer row /// Indication that header row is found /// Footer row name internal void GetNextFooterRow( int fRepeatedFooter, IntPtr nmFooterRow, out int fFound, out IntPtr pnmNextFooterRow) { fFound = PTS.False; pnmNextFooterRow = IntPtr.Zero; } ////// GetFirstRow /// /// Indication that first body row is found /// First body row name internal void GetFirstRow( out int fFound, out IntPtr pnmFirstRow) { if(_firstChild == null) { TableRow tableRow = null; for(int rowGroupIndex = 0; rowGroupIndex < Table.RowGroups.Count && tableRow == null; rowGroupIndex++) { TableRowGroup rowGroup = Table.RowGroups[rowGroupIndex]; if(rowGroup.Rows.Count > 0) { tableRow = rowGroup.Rows[0]; Invariant.Assert(tableRow.Index != -1); } } if(tableRow != null) { _firstChild = new RowParagraph(tableRow, StructuralCache); ((RowParagraph)_firstChild).CalculateRowSpans(); } } if(_firstChild != null) { fFound = PTS.True; pnmFirstRow = _firstChild.Handle; } else { fFound = PTS.False; pnmFirstRow = IntPtr.Zero; } } ////// GetNextRow /// /// Previous body row name /// Indication that body row is found /// Body row name internal void GetNextRow( IntPtr nmRow, out int fFound, out IntPtr pnmNextRow) { Debug.Assert(Table.RowGroups.Count > 0); BaseParagraph prevParagraph = ((RowParagraph)PtsContext.HandleToObject(nmRow)); BaseParagraph nextParagraph = prevParagraph.Next; if(nextParagraph == null) { TableRow currentRow = ((RowParagraph)prevParagraph).Row; TableRowGroup currentRowGroup = currentRow.RowGroup; TableRow tableRow = null; int nextRowIndex = currentRow.Index + 1; int nextRowGroupIndex = currentRowGroup.Index + 1; if (nextRowIndex < currentRowGroup.Rows.Count) { Debug.Assert(currentRowGroup.Rows[nextRowIndex].Index != -1, "Row is not in a table"); tableRow = currentRowGroup.Rows[nextRowIndex]; } while(tableRow == null) { if(nextRowGroupIndex == Table.RowGroups.Count) { break; } TableRowCollection Rows = Table.RowGroups[nextRowGroupIndex].Rows; if (Rows.Count > 0) { Debug.Assert(Rows[0].Index != -1, "Row is not in a table"); tableRow = Rows[0]; } nextRowGroupIndex++; } if(tableRow != null) { nextParagraph = new RowParagraph(tableRow, StructuralCache); prevParagraph.Next = nextParagraph; nextParagraph.Previous = prevParagraph; ((RowParagraph)nextParagraph).CalculateRowSpans(); } } if(nextParagraph != null) { fFound = PTS.True; pnmNextRow = nextParagraph.Handle; } else { fFound = PTS.False; pnmNextRow = IntPtr.Zero; } } ////// UpdFChangeInHeaderFooter /// /// Header changed? /// Footer changed? /// Repeated header changed? /// Repeated footer changed? internal void UpdFChangeInHeaderFooter( // we don't do update in header/footer out int fHeaderChanged, // OUT: out int fFooterChanged, // OUT: out int fRepeatedHeaderChanged, // OUT: unneeded for bottomless page, but... out int fRepeatedFooterChanged) // OUT: unneeded for bottomless page, but... { fHeaderChanged = PTS.False; fRepeatedHeaderChanged = PTS.False; fFooterChanged = PTS.False; fRepeatedFooterChanged = PTS.False; } ////// UpdGetFirstChangeInTable /// /// Indication that changed body row is found /// Is the change in the first body row? /// The last unchanged body row internal void UpdGetFirstChangeInTable( out int fFound, // OUT: out int fChangeFirst, // OUT: out IntPtr pnmRowBeforeChange) // OUT: { // No incremental update for table. fFound = PTS.True; fChangeFirst = PTS.True; pnmRowBeforeChange = IntPtr.Zero; } ////// GetDistributionKind /// /// Direction of the Table /// Height distribution kind internal void GetDistributionKind( uint fswdirTable, // IN: direction of the Table out PTS.FSKTABLEHEIGHTDISTRIBUTION tabledistr) // OUT: height distribution kind { tabledistr = PTS.FSKTABLEHEIGHTDISTRIBUTION.fskdistributeUnchanged; } // ----------------------------------------------------------------- // UpdGetParaChange // ----------------------------------------------------------------- internal override void UpdGetParaChange( out PTS.FSKCHANGE fskch, // OUT: kind of change out int fNoFurtherChanges) // OUT: no changes after? { base.UpdGetParaChange(out fskch, out fNoFurtherChanges); fskch = PTS.FSKCHANGE.fskchNew; } // ----------------------------------------------------------------- // Invalidate content's structural cache. // // startPosition - Position to start invalidation from. // // Returns: 'true' if entire paragraph is invalid. // ------------------------------------------------------------------ internal override bool InvalidateStructure(int startPosition) { bool isEntireTableInvalid = true; RowParagraph currentParagraph = _firstChild as RowParagraph; while(currentParagraph != null) { if(!InvalidateRowStructure(currentParagraph, startPosition)) { isEntireTableInvalid = false; } currentParagraph = currentParagraph.Next as RowParagraph; } return isEntireTableInvalid; } // ----------------------------------------------------------------- // Invalidate accumulated format caches for the table. // ------------------------------------------------------------------ internal override void InvalidateFormatCache() { RowParagraph currentParagraph = _firstChild as RowParagraph; while(currentParagraph != null) { InvalidateRowFormatCache(currentParagraph); currentParagraph = currentParagraph.Next as RowParagraph; } } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Table owner accessor /// internal Table Table { get { return (Table)Element; } } #endregion Internal Properties //------------------------------------------------------ // // Private methods // //----------------------------------------------------- #region Private Methods // ----------------------------------------------------------------- // Invalidate accumulated format caches for the row. // ----------------------------------------------------------------- private bool InvalidateRowStructure(RowParagraph rowParagraph, int startPosition) { bool isEntireTableInvalid = true; for(int iCell = 0; iCell < rowParagraph.Cells.Length; iCell++) { CellParagraph cellParagraph = rowParagraph.Cells[iCell]; if(cellParagraph.ParagraphEndCharacterPosition < startPosition || !cellParagraph.InvalidateStructure(startPosition)) { isEntireTableInvalid = false; } } return isEntireTableInvalid; } // ------------------------------------------------------------------ // Invalidate accumulated format caches for the row. // ----------------------------------------------------------------- private void InvalidateRowFormatCache(RowParagraph rowParagraph) { for(int iCell = 0; iCell < rowParagraph.Cells.Length; iCell++) { rowParagraph.Cells[iCell].InvalidateFormatCache(); } } // ------------------------------------------------------------------ // Table has been structurally altered // ------------------------------------------------------------------ private void TableStructureChanged(object sender, EventArgs e) { // Disconnect obsolete paragraphs. BaseParagraph paraInvalid = _firstChild; while (paraInvalid != null) { paraInvalid.Dispose(); paraInvalid = paraInvalid.Next; } _firstChild = null; // // Since whole table content is disposed, we need // - to create dirty text range corresponding to the Table content // - notify formatter that Table's content is changed. // int charCount = Table.SymbolCount - 2;// This is equivalent to (ContentEndOffset – ContentStartOffset) but is more performant. if (charCount > 0) { DirtyTextRange dtr = new DirtyTextRange(Table.ContentStartOffset, charCount, charCount); StructuralCache.AddDirtyTextRange(dtr); } if (StructuralCache.FormattingOwner.Formatter != null) { StructuralCache.FormattingOwner.Formatter.OnContentInvalidated(true, Table.ContentStart, Table.ContentEnd); } } #endregion Private Methods //----------------------------------------------------- // // Private Properties // //------------------------------------------------------ #region Private Properties #endregion Private Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields BaseParagraph _firstChild; #endregion Private Fields //----------------------------------------------------- // // Private Structures / Classes // //------------------------------------------------------ #region Private Structures Classes #endregion Private Structures Classes } } #pragma warning enable 1634, 1691 // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Implementation of the PTS paragraph corresponding to table. // // History: // 05/30/2003 : olego - Created // //--------------------------------------------------------------------------- #pragma warning disable 1634, 1691 // avoid generating warnings about unknown // message numbers and unknown pragmas for PRESharp contol using MS.Internal.Documents; using MS.Internal.PtsTable; using MS.Internal.Text; using MS.Utility; using System; using System.Collections; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using System.Windows.Media; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { ////// Table paragraph PTS object implementation /// internal sealed class TableParagraph : BaseParagraph { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal TableParagraph(DependencyObject element, StructuralCache structuralCache) : base(element, structuralCache) { Table.TableStructureChanged += new System.EventHandler(TableStructureChanged); } // ------------------------------------------------------------------ // IDisposable.Dispose // ----------------------------------------------------------------- public override void Dispose() { Table.TableStructureChanged -= new System.EventHandler(TableStructureChanged); BaseParagraph paraChild = _firstChild; while (paraChild != null) { BaseParagraph para = paraChild; paraChild = paraChild.Next; para.Dispose(); para.Next = null; para.Previous = null; } _firstChild = null; base.Dispose(); } #endregion Constructors //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion Protected Methods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Collapse Margins /// /// Para client /// input margin collapsing state /// current direction (of the track, in which margin collapsing is happening) /// suppress empty space at the top of page /// dvr, calculated based on margin collapsing state internal override void CollapseMargin( BaseParaClient paraClient, // IN: MarginCollapsingState mcs, // IN: input margin collapsing state uint fswdir, // IN: current direction (of the track, in which margin collapsing is happening) bool suppressTopSpace, // IN: suppress empty space at the top of page out int dvr) // OUT: dvr, calculated based on margin collapsing state { if (suppressTopSpace && (StructuralCache.CurrentFormatContext.FinitePage || mcs == null)) dvr = 0; else { MbpInfo mbp = MbpInfo.FromElement(Table); MarginCollapsingState mcsOut = null; MarginCollapsingState.CollapseTopMargin(PtsContext, mbp, mcs, out mcsOut, out dvr); if (mcsOut != null) { dvr = mcsOut.Margin; mcsOut.Dispose(); mcsOut = null; } } } ////// GetParaProperties. /// /// paragraph properties internal override void GetParaProperties( ref PTS.FSPAP fspap) // OUT: paragraph properties { fspap.idobj = PtsHost.TableParagraphId; // fspap.fKeepWithNext = PTS.False; fspap.fBreakPageBefore = PTS.False; fspap.fBreakColumnBefore = PTS.False; } ////// CreateParaclient /// /// Opaque to PTS paragraph client internal override void CreateParaclient( out IntPtr pfsparaclient) // OUT: opaque to PTS paragraph client { #pragma warning disable 6518 // Disable PRESharp warning 6518. TableParaClient is an UnmamangedHandle, that adds itself // to HandleMapper that holds a reference to it. PTS manages lifetime of this object, and // calls DestroyParaclient to get rid of it. DestroyParaclient will call Dispose() on the object // and remove it from HandleMapper. TableParaClient paraClient = new TableParaClient(this); pfsparaclient = paraClient.Handle; #pragma warning restore 6518 } ////// GetTableProperties /// /// Direction of Track /// Properties of the table internal void GetTableProperties( uint fswdirTrack, // IN: direction of Track out PTS.FSTABLEOBJPROPS fstableobjprops)// OUT: properties of the table { fstableobjprops = new PTS.FSTABLEOBJPROPS(); fstableobjprops.fskclear = PTS.FSKCLEAR.fskclearNone; fstableobjprops.ktablealignment = PTS.FSKTABLEOBJALIGNMENT.fsktableobjAlignLeft; fstableobjprops.fFloat = PTS.False; fstableobjprops.fskwr = PTS.FSKWRAP.fskwrBoth; fstableobjprops.fDelayNoProgress = PTS.False; fstableobjprops.dvrCaptionTop = 0; fstableobjprops.dvrCaptionBottom = 0; fstableobjprops.durCaptionLeft = 0; fstableobjprops.durCaptionRight = 0; fstableobjprops.fswdirTable = PTS.FlowDirectionToFswdir((FlowDirection)Element.GetValue(FrameworkElement.FlowDirectionProperty)); } ////// GetMCSClientAfterTable /// /// Direction of Track /// Margin collapsing state /// Margin collapsing state internal void GetMCSClientAfterTable( uint fswdirTrack, IntPtr pmcsclientIn, out IntPtr ppmcsclientOut) { ppmcsclientOut = IntPtr.Zero; MbpInfo mbp = MbpInfo.FromElement(Table); MarginCollapsingState mcs = null; if (pmcsclientIn != IntPtr.Zero) mcs = PtsContext.HandleToObject(pmcsclientIn) as MarginCollapsingState; MarginCollapsingState mcsNew = null; int margin; MarginCollapsingState.CollapseBottomMargin(PtsContext, mbp, mcs, out mcsNew, out margin); if (mcsNew != null) ppmcsclientOut = mcsNew.Handle; } ////// GetFirstHeaderRow /// /// Repeated header flag /// Indication that first header row is found /// First header row name internal void GetFirstHeaderRow( int fRepeatedHeader, out int fFound, out IntPtr pnmFirstHeaderRow) { fFound = PTS.False; pnmFirstHeaderRow = IntPtr.Zero; } ////// GetNextHeaderRow /// /// Repeated header flag /// Previous header row /// Indication that header row is found /// Header row name internal void GetNextHeaderRow( int fRepeatedHeader, IntPtr nmHeaderRow, out int fFound, out IntPtr pnmNextHeaderRow) { fFound = PTS.False; pnmNextHeaderRow = IntPtr.Zero; } ////// GetFirstFooterRow /// /// Repeated footer flag /// Indication that first header row is found /// First footer row name internal void GetFirstFooterRow( int fRepeatedFooter, out int fFound, out IntPtr pnmFirstFooterRow) { fFound = PTS.False; pnmFirstFooterRow = IntPtr.Zero; } ////// GetNextFooterRow /// /// Repeated footer flag /// Previous footer row /// Indication that header row is found /// Footer row name internal void GetNextFooterRow( int fRepeatedFooter, IntPtr nmFooterRow, out int fFound, out IntPtr pnmNextFooterRow) { fFound = PTS.False; pnmNextFooterRow = IntPtr.Zero; } ////// GetFirstRow /// /// Indication that first body row is found /// First body row name internal void GetFirstRow( out int fFound, out IntPtr pnmFirstRow) { if(_firstChild == null) { TableRow tableRow = null; for(int rowGroupIndex = 0; rowGroupIndex < Table.RowGroups.Count && tableRow == null; rowGroupIndex++) { TableRowGroup rowGroup = Table.RowGroups[rowGroupIndex]; if(rowGroup.Rows.Count > 0) { tableRow = rowGroup.Rows[0]; Invariant.Assert(tableRow.Index != -1); } } if(tableRow != null) { _firstChild = new RowParagraph(tableRow, StructuralCache); ((RowParagraph)_firstChild).CalculateRowSpans(); } } if(_firstChild != null) { fFound = PTS.True; pnmFirstRow = _firstChild.Handle; } else { fFound = PTS.False; pnmFirstRow = IntPtr.Zero; } } ////// GetNextRow /// /// Previous body row name /// Indication that body row is found /// Body row name internal void GetNextRow( IntPtr nmRow, out int fFound, out IntPtr pnmNextRow) { Debug.Assert(Table.RowGroups.Count > 0); BaseParagraph prevParagraph = ((RowParagraph)PtsContext.HandleToObject(nmRow)); BaseParagraph nextParagraph = prevParagraph.Next; if(nextParagraph == null) { TableRow currentRow = ((RowParagraph)prevParagraph).Row; TableRowGroup currentRowGroup = currentRow.RowGroup; TableRow tableRow = null; int nextRowIndex = currentRow.Index + 1; int nextRowGroupIndex = currentRowGroup.Index + 1; if (nextRowIndex < currentRowGroup.Rows.Count) { Debug.Assert(currentRowGroup.Rows[nextRowIndex].Index != -1, "Row is not in a table"); tableRow = currentRowGroup.Rows[nextRowIndex]; } while(tableRow == null) { if(nextRowGroupIndex == Table.RowGroups.Count) { break; } TableRowCollection Rows = Table.RowGroups[nextRowGroupIndex].Rows; if (Rows.Count > 0) { Debug.Assert(Rows[0].Index != -1, "Row is not in a table"); tableRow = Rows[0]; } nextRowGroupIndex++; } if(tableRow != null) { nextParagraph = new RowParagraph(tableRow, StructuralCache); prevParagraph.Next = nextParagraph; nextParagraph.Previous = prevParagraph; ((RowParagraph)nextParagraph).CalculateRowSpans(); } } if(nextParagraph != null) { fFound = PTS.True; pnmNextRow = nextParagraph.Handle; } else { fFound = PTS.False; pnmNextRow = IntPtr.Zero; } } ////// UpdFChangeInHeaderFooter /// /// Header changed? /// Footer changed? /// Repeated header changed? /// Repeated footer changed? internal void UpdFChangeInHeaderFooter( // we don't do update in header/footer out int fHeaderChanged, // OUT: out int fFooterChanged, // OUT: out int fRepeatedHeaderChanged, // OUT: unneeded for bottomless page, but... out int fRepeatedFooterChanged) // OUT: unneeded for bottomless page, but... { fHeaderChanged = PTS.False; fRepeatedHeaderChanged = PTS.False; fFooterChanged = PTS.False; fRepeatedFooterChanged = PTS.False; } ////// UpdGetFirstChangeInTable /// /// Indication that changed body row is found /// Is the change in the first body row? /// The last unchanged body row internal void UpdGetFirstChangeInTable( out int fFound, // OUT: out int fChangeFirst, // OUT: out IntPtr pnmRowBeforeChange) // OUT: { // No incremental update for table. fFound = PTS.True; fChangeFirst = PTS.True; pnmRowBeforeChange = IntPtr.Zero; } ////// GetDistributionKind /// /// Direction of the Table /// Height distribution kind internal void GetDistributionKind( uint fswdirTable, // IN: direction of the Table out PTS.FSKTABLEHEIGHTDISTRIBUTION tabledistr) // OUT: height distribution kind { tabledistr = PTS.FSKTABLEHEIGHTDISTRIBUTION.fskdistributeUnchanged; } // ----------------------------------------------------------------- // UpdGetParaChange // ----------------------------------------------------------------- internal override void UpdGetParaChange( out PTS.FSKCHANGE fskch, // OUT: kind of change out int fNoFurtherChanges) // OUT: no changes after? { base.UpdGetParaChange(out fskch, out fNoFurtherChanges); fskch = PTS.FSKCHANGE.fskchNew; } // ----------------------------------------------------------------- // Invalidate content's structural cache. // // startPosition - Position to start invalidation from. // // Returns: 'true' if entire paragraph is invalid. // ------------------------------------------------------------------ internal override bool InvalidateStructure(int startPosition) { bool isEntireTableInvalid = true; RowParagraph currentParagraph = _firstChild as RowParagraph; while(currentParagraph != null) { if(!InvalidateRowStructure(currentParagraph, startPosition)) { isEntireTableInvalid = false; } currentParagraph = currentParagraph.Next as RowParagraph; } return isEntireTableInvalid; } // ----------------------------------------------------------------- // Invalidate accumulated format caches for the table. // ------------------------------------------------------------------ internal override void InvalidateFormatCache() { RowParagraph currentParagraph = _firstChild as RowParagraph; while(currentParagraph != null) { InvalidateRowFormatCache(currentParagraph); currentParagraph = currentParagraph.Next as RowParagraph; } } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Table owner accessor /// internal Table Table { get { return (Table)Element; } } #endregion Internal Properties //------------------------------------------------------ // // Private methods // //----------------------------------------------------- #region Private Methods // ----------------------------------------------------------------- // Invalidate accumulated format caches for the row. // ----------------------------------------------------------------- private bool InvalidateRowStructure(RowParagraph rowParagraph, int startPosition) { bool isEntireTableInvalid = true; for(int iCell = 0; iCell < rowParagraph.Cells.Length; iCell++) { CellParagraph cellParagraph = rowParagraph.Cells[iCell]; if(cellParagraph.ParagraphEndCharacterPosition < startPosition || !cellParagraph.InvalidateStructure(startPosition)) { isEntireTableInvalid = false; } } return isEntireTableInvalid; } // ------------------------------------------------------------------ // Invalidate accumulated format caches for the row. // ----------------------------------------------------------------- private void InvalidateRowFormatCache(RowParagraph rowParagraph) { for(int iCell = 0; iCell < rowParagraph.Cells.Length; iCell++) { rowParagraph.Cells[iCell].InvalidateFormatCache(); } } // ------------------------------------------------------------------ // Table has been structurally altered // ------------------------------------------------------------------ private void TableStructureChanged(object sender, EventArgs e) { // Disconnect obsolete paragraphs. BaseParagraph paraInvalid = _firstChild; while (paraInvalid != null) { paraInvalid.Dispose(); paraInvalid = paraInvalid.Next; } _firstChild = null; // // Since whole table content is disposed, we need // - to create dirty text range corresponding to the Table content // - notify formatter that Table's content is changed. // int charCount = Table.SymbolCount - 2;// This is equivalent to (ContentEndOffset – ContentStartOffset) but is more performant. if (charCount > 0) { DirtyTextRange dtr = new DirtyTextRange(Table.ContentStartOffset, charCount, charCount); StructuralCache.AddDirtyTextRange(dtr); } if (StructuralCache.FormattingOwner.Formatter != null) { StructuralCache.FormattingOwner.Formatter.OnContentInvalidated(true, Table.ContentStart, Table.ContentEnd); } } #endregion Private Methods //----------------------------------------------------- // // Private Properties // //------------------------------------------------------ #region Private Properties #endregion Private Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields BaseParagraph _firstChild; #endregion Private Fields //----------------------------------------------------- // // Private Structures / Classes // //------------------------------------------------------ #region Private Structures Classes #endregion Private Structures Classes } } #pragma warning enable 1634, 1691 // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
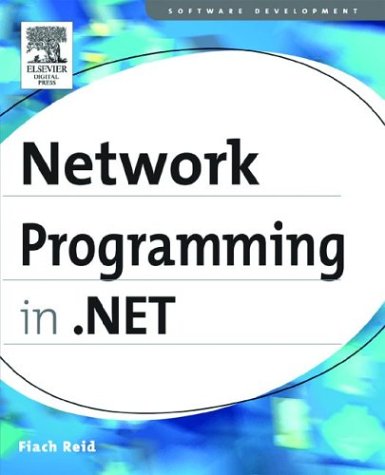
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventToken.cs
- PageAsyncTask.cs
- PiiTraceSource.cs
- TerminatorSinks.cs
- UserControlParser.cs
- EditingCommands.cs
- CommandID.cs
- SerializationException.cs
- MediaElementAutomationPeer.cs
- ExecutionEngineException.cs
- ObjectToken.cs
- DataServiceQueryException.cs
- URI.cs
- TemplateColumn.cs
- PackagingUtilities.cs
- ToolStripCodeDomSerializer.cs
- ListViewGroupItemCollection.cs
- SecurityCriticalDataForSet.cs
- DataServiceRequest.cs
- TypeElementCollection.cs
- FilterQuery.cs
- RectAnimation.cs
- ObjectListSelectEventArgs.cs
- FocusChangedEventArgs.cs
- AbstractExpressions.cs
- TraversalRequest.cs
- Stylesheet.cs
- LinqDataSourceHelper.cs
- SiteMapNode.cs
- WSHttpBindingBase.cs
- ProfessionalColorTable.cs
- CellConstant.cs
- WebPartCloseVerb.cs
- TypeInfo.cs
- DecimalKeyFrameCollection.cs
- DesignerLoader.cs
- RoleGroupCollection.cs
- ModelChangedEventArgsImpl.cs
- WebPartCatalogCloseVerb.cs
- ActivityDesignerHighlighter.cs
- ControlPager.cs
- OciHandle.cs
- EventProxy.cs
- PointCollection.cs
- Rect3D.cs
- SiteMapPath.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- ConfigurationStrings.cs
- FileDialog.cs
- Missing.cs
- QilGeneratorEnv.cs
- CommonRemoteMemoryBlock.cs
- Environment.cs
- TransformerInfo.cs
- Help.cs
- VBIdentifierName.cs
- WebPartZoneBase.cs
- HttpFileCollectionWrapper.cs
- ButtonBaseAutomationPeer.cs
- LightweightEntityWrapper.cs
- LightweightCodeGenerator.cs
- PathNode.cs
- XmlDocumentSurrogate.cs
- DataServices.cs
- QueryCacheManager.cs
- BaseDataList.cs
- EntityContainer.cs
- SQlBooleanStorage.cs
- BrowserCapabilitiesFactoryBase.cs
- SoapElementAttribute.cs
- ExtentKey.cs
- AssemblyName.cs
- XmlUTF8TextWriter.cs
- BeginStoryboard.cs
- HttpConfigurationContext.cs
- HandlerFactoryCache.cs
- PersonalizationAdministration.cs
- CodeDOMProvider.cs
- StringCollectionEditor.cs
- PathFigureCollection.cs
- EncryptedPackage.cs
- ButtonFieldBase.cs
- AssertUtility.cs
- SortedDictionary.cs
- _NestedMultipleAsyncResult.cs
- DataStreams.cs
- CodeNamespaceImport.cs
- ContractValidationHelper.cs
- WorkflowNamespace.cs
- AttributeQuery.cs
- Currency.cs
- LocalClientSecuritySettings.cs
- PenCursorManager.cs
- CharacterBufferReference.cs
- WebAdminConfigurationHelper.cs
- TemplateApplicationHelper.cs
- VectorKeyFrameCollection.cs
- LinkedResource.cs
- Brush.cs
- HttpRuntimeSection.cs