Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Effects / Generated / OuterGlowBitmapEffect.cs / 1305600 / OuterGlowBitmapEffect.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.KnownBoxes; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Effects { sealed partial class OuterGlowBitmapEffect : BitmapEffect { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new OuterGlowBitmapEffect Clone() { return (OuterGlowBitmapEffect)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new OuterGlowBitmapEffect CloneCurrentValue() { return (OuterGlowBitmapEffect)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void GlowColorPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { OuterGlowBitmapEffect target = ((OuterGlowBitmapEffect) d); target.PropertyChanged(GlowColorProperty); } private static void GlowSizePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { OuterGlowBitmapEffect target = ((OuterGlowBitmapEffect) d); target.PropertyChanged(GlowSizeProperty); } private static void NoisePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { OuterGlowBitmapEffect target = ((OuterGlowBitmapEffect) d); target.PropertyChanged(NoiseProperty); } private static void OpacityPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { OuterGlowBitmapEffect target = ((OuterGlowBitmapEffect) d); target.PropertyChanged(OpacityProperty); } #region Public Properties ////// GlowColor - Color. Default value is Colors.Gold. /// public Color GlowColor { get { return (Color) GetValue(GlowColorProperty); } set { SetValueInternal(GlowColorProperty, value); } } ////// GlowSize - double. Default value is 5.0. /// public double GlowSize { get { return (double) GetValue(GlowSizeProperty); } set { SetValueInternal(GlowSizeProperty, value); } } ////// Noise - double. Default value is 0.0. /// public double Noise { get { return (double) GetValue(NoiseProperty); } set { SetValueInternal(NoiseProperty, value); } } ////// Opacity - double. Default value is 1.0. /// public double Opacity { get { return (double) GetValue(OpacityProperty); } set { SetValueInternal(OpacityProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new OuterGlowBitmapEffect(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the OuterGlowBitmapEffect.GlowColor property. /// public static readonly DependencyProperty GlowColorProperty; ////// The DependencyProperty for the OuterGlowBitmapEffect.GlowSize property. /// public static readonly DependencyProperty GlowSizeProperty; ////// The DependencyProperty for the OuterGlowBitmapEffect.Noise property. /// public static readonly DependencyProperty NoiseProperty; ////// The DependencyProperty for the OuterGlowBitmapEffect.Opacity property. /// public static readonly DependencyProperty OpacityProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal static Color s_GlowColor = Colors.Gold; internal const double c_GlowSize = 5.0; internal const double c_Noise = 0.0; internal const double c_Opacity = 1.0; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static OuterGlowBitmapEffect() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(OuterGlowBitmapEffect); GlowColorProperty = RegisterProperty("GlowColor", typeof(Color), typeofThis, Colors.Gold, new PropertyChangedCallback(GlowColorPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); GlowSizeProperty = RegisterProperty("GlowSize", typeof(double), typeofThis, 5.0, new PropertyChangedCallback(GlowSizePropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); NoiseProperty = RegisterProperty("Noise", typeof(double), typeofThis, 0.0, new PropertyChangedCallback(NoisePropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); OpacityProperty = RegisterProperty("Opacity", typeof(double), typeofThis, 1.0, new PropertyChangedCallback(OpacityPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.KnownBoxes; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Effects { sealed partial class OuterGlowBitmapEffect : BitmapEffect { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new OuterGlowBitmapEffect Clone() { return (OuterGlowBitmapEffect)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new OuterGlowBitmapEffect CloneCurrentValue() { return (OuterGlowBitmapEffect)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void GlowColorPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { OuterGlowBitmapEffect target = ((OuterGlowBitmapEffect) d); target.PropertyChanged(GlowColorProperty); } private static void GlowSizePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { OuterGlowBitmapEffect target = ((OuterGlowBitmapEffect) d); target.PropertyChanged(GlowSizeProperty); } private static void NoisePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { OuterGlowBitmapEffect target = ((OuterGlowBitmapEffect) d); target.PropertyChanged(NoiseProperty); } private static void OpacityPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { OuterGlowBitmapEffect target = ((OuterGlowBitmapEffect) d); target.PropertyChanged(OpacityProperty); } #region Public Properties ////// GlowColor - Color. Default value is Colors.Gold. /// public Color GlowColor { get { return (Color) GetValue(GlowColorProperty); } set { SetValueInternal(GlowColorProperty, value); } } ////// GlowSize - double. Default value is 5.0. /// public double GlowSize { get { return (double) GetValue(GlowSizeProperty); } set { SetValueInternal(GlowSizeProperty, value); } } ////// Noise - double. Default value is 0.0. /// public double Noise { get { return (double) GetValue(NoiseProperty); } set { SetValueInternal(NoiseProperty, value); } } ////// Opacity - double. Default value is 1.0. /// public double Opacity { get { return (double) GetValue(OpacityProperty); } set { SetValueInternal(OpacityProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new OuterGlowBitmapEffect(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the OuterGlowBitmapEffect.GlowColor property. /// public static readonly DependencyProperty GlowColorProperty; ////// The DependencyProperty for the OuterGlowBitmapEffect.GlowSize property. /// public static readonly DependencyProperty GlowSizeProperty; ////// The DependencyProperty for the OuterGlowBitmapEffect.Noise property. /// public static readonly DependencyProperty NoiseProperty; ////// The DependencyProperty for the OuterGlowBitmapEffect.Opacity property. /// public static readonly DependencyProperty OpacityProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal static Color s_GlowColor = Colors.Gold; internal const double c_GlowSize = 5.0; internal const double c_Noise = 0.0; internal const double c_Opacity = 1.0; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static OuterGlowBitmapEffect() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(OuterGlowBitmapEffect); GlowColorProperty = RegisterProperty("GlowColor", typeof(Color), typeofThis, Colors.Gold, new PropertyChangedCallback(GlowColorPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); GlowSizeProperty = RegisterProperty("GlowSize", typeof(double), typeofThis, 5.0, new PropertyChangedCallback(GlowSizePropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); NoiseProperty = RegisterProperty("Noise", typeof(double), typeofThis, 0.0, new PropertyChangedCallback(NoisePropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); OpacityProperty = RegisterProperty("Opacity", typeof(double), typeofThis, 1.0, new PropertyChangedCallback(OpacityPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
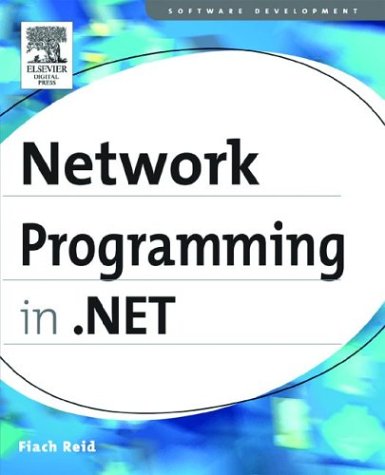
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CharEntityEncoderFallback.cs
- CollectionViewGroupInternal.cs
- DataRecord.cs
- SelectionPattern.cs
- AlignmentYValidation.cs
- ProfileGroupSettingsCollection.cs
- WebPartUserCapability.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- DataGridViewAutoSizeModeEventArgs.cs
- ThreadStateException.cs
- SqlCacheDependencySection.cs
- RegexStringValidator.cs
- EndpointAddressMessageFilter.cs
- RequestCacheEntry.cs
- TextBlock.cs
- HostProtectionException.cs
- TypeUsage.cs
- EntityDataReader.cs
- HttpDigestClientElement.cs
- MarkedHighlightComponent.cs
- InputBuffer.cs
- Single.cs
- XmlComment.cs
- ObjectListCommandEventArgs.cs
- DataSysAttribute.cs
- DiscardableAttribute.cs
- ReadOnlyHierarchicalDataSourceView.cs
- ThemeConfigurationDialog.cs
- TextHidden.cs
- ConfigXmlAttribute.cs
- PageRequestManager.cs
- StartUpEventArgs.cs
- GACMembershipCondition.cs
- DataServiceProcessingPipeline.cs
- COSERVERINFO.cs
- X509Chain.cs
- SmtpDigestAuthenticationModule.cs
- GlobalEventManager.cs
- TableItemProviderWrapper.cs
- ExceptionUtil.cs
- DataGridRelationshipRow.cs
- HttpResponseInternalWrapper.cs
- SHA1CryptoServiceProvider.cs
- SmtpDigestAuthenticationModule.cs
- StrongTypingException.cs
- ZipIOLocalFileHeader.cs
- UidManager.cs
- FileUpload.cs
- Selection.cs
- CompilerScopeManager.cs
- EnumerableValidator.cs
- WebScriptEndpointElement.cs
- BoundsDrawingContextWalker.cs
- SingleStorage.cs
- WindowsFormsLinkLabel.cs
- Transform3D.cs
- LineBreakRecord.cs
- PropertyCollection.cs
- AutomationElementIdentifiers.cs
- FileLoadException.cs
- BatchParser.cs
- SemaphoreSecurity.cs
- PersianCalendar.cs
- RoleGroup.cs
- ConnectionStringsExpressionBuilder.cs
- DesignerCategoryAttribute.cs
- ResetableIterator.cs
- TagMapInfo.cs
- DataGridViewColumnStateChangedEventArgs.cs
- SQLMoneyStorage.cs
- XmlImplementation.cs
- CodeIdentifiers.cs
- XmlUnspecifiedAttribute.cs
- ImmComposition.cs
- DecimalConstantAttribute.cs
- SerializationSectionGroup.cs
- ListItemParagraph.cs
- MsmqTransportReceiveParameters.cs
- MetadataCache.cs
- ToolStripGripRenderEventArgs.cs
- PartialArray.cs
- EmptyCollection.cs
- SmtpException.cs
- Native.cs
- KeyManager.cs
- PeerCollaboration.cs
- ConsoleCancelEventArgs.cs
- RangeContentEnumerator.cs
- TreeNodeCollection.cs
- FrameworkElementFactoryMarkupObject.cs
- DataDesignUtil.cs
- NoPersistHandle.cs
- SiteMapDataSourceDesigner.cs
- DataComponentGenerator.cs
- BamlLocalizableResource.cs
- InputScope.cs
- MarshalDirectiveException.cs
- MemberAccessException.cs
- NavigationProperty.cs
- Part.cs