Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / MouseEventArgs.cs / 1305600 / MouseEventArgs.cs
using System.Collections; using System; namespace System.Windows.Input { ////// The MouseEventArgs class provides access to the logical /// Mouse device for all derived event args. /// public class MouseEventArgs : InputEventArgs { ////// Initializes a new instance of the MouseEventArgs class. /// /// /// The logical Mouse device associated with this event. /// /// /// The time when the input occured. /// public MouseEventArgs(MouseDevice mouse, int timestamp) : base(mouse, timestamp) { if( mouse == null ) { throw new System.ArgumentNullException("mouse"); } _stylusDevice = null; } ////// Initializes a new instance of the MouseEventArgs class. /// /// /// The logical Mouse device associated with this event. /// /// /// The time when the input occured. /// /// /// The stylus device that was involved with this event. /// public MouseEventArgs(MouseDevice mouse, int timestamp, StylusDevice stylusDevice) : base(mouse, timestamp) { if( mouse == null ) { throw new System.ArgumentNullException("mouse"); } _stylusDevice = stylusDevice; } ////// Read-only access to the mouse device associated with this /// event. /// public MouseDevice MouseDevice { get {return (MouseDevice) this.Device;} } ////// Read-only access to the stylus Mouse associated with this event. /// public StylusDevice StylusDevice { get {return _stylusDevice;} } ////// Calculates the position of the mouse relative to /// a particular element. /// public Point GetPosition(IInputElement relativeTo) { return this.MouseDevice.GetPosition(relativeTo); } ////// The state of the left button. /// public MouseButtonState LeftButton { get { return this.MouseDevice.LeftButton; } } ////// The state of the right button. /// public MouseButtonState RightButton { get { return this.MouseDevice.RightButton; } } ////// The state of the middle button. /// public MouseButtonState MiddleButton { get { return this.MouseDevice.MiddleButton; } } ////// The state of the first extended button. /// public MouseButtonState XButton1 { get { return this.MouseDevice.XButton1; } } ////// The state of the second extended button. /// public MouseButtonState XButton2 { get { return this.MouseDevice.XButton2; } } ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { MouseEventHandler handler = (MouseEventHandler) genericHandler; handler(genericTarget, this); } private StylusDevice _stylusDevice; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Collections; using System; namespace System.Windows.Input { ////// The MouseEventArgs class provides access to the logical /// Mouse device for all derived event args. /// public class MouseEventArgs : InputEventArgs { ////// Initializes a new instance of the MouseEventArgs class. /// /// /// The logical Mouse device associated with this event. /// /// /// The time when the input occured. /// public MouseEventArgs(MouseDevice mouse, int timestamp) : base(mouse, timestamp) { if( mouse == null ) { throw new System.ArgumentNullException("mouse"); } _stylusDevice = null; } ////// Initializes a new instance of the MouseEventArgs class. /// /// /// The logical Mouse device associated with this event. /// /// /// The time when the input occured. /// /// /// The stylus device that was involved with this event. /// public MouseEventArgs(MouseDevice mouse, int timestamp, StylusDevice stylusDevice) : base(mouse, timestamp) { if( mouse == null ) { throw new System.ArgumentNullException("mouse"); } _stylusDevice = stylusDevice; } ////// Read-only access to the mouse device associated with this /// event. /// public MouseDevice MouseDevice { get {return (MouseDevice) this.Device;} } ////// Read-only access to the stylus Mouse associated with this event. /// public StylusDevice StylusDevice { get {return _stylusDevice;} } ////// Calculates the position of the mouse relative to /// a particular element. /// public Point GetPosition(IInputElement relativeTo) { return this.MouseDevice.GetPosition(relativeTo); } ////// The state of the left button. /// public MouseButtonState LeftButton { get { return this.MouseDevice.LeftButton; } } ////// The state of the right button. /// public MouseButtonState RightButton { get { return this.MouseDevice.RightButton; } } ////// The state of the middle button. /// public MouseButtonState MiddleButton { get { return this.MouseDevice.MiddleButton; } } ////// The state of the first extended button. /// public MouseButtonState XButton1 { get { return this.MouseDevice.XButton1; } } ////// The state of the second extended button. /// public MouseButtonState XButton2 { get { return this.MouseDevice.XButton2; } } ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { MouseEventHandler handler = (MouseEventHandler) genericHandler; handler(genericTarget, this); } private StylusDevice _stylusDevice; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
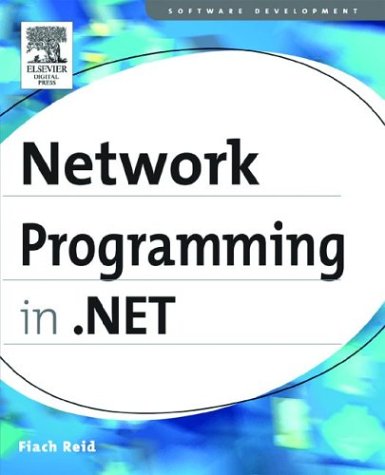
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ISAPIWorkerRequest.cs
- RawMouseInputReport.cs
- xmlsaver.cs
- ISAPIWorkerRequest.cs
- DataTableCollection.cs
- Hash.cs
- BitmapSizeOptions.cs
- TemplateControlCodeDomTreeGenerator.cs
- InputBuffer.cs
- Cursors.cs
- FixedSOMGroup.cs
- QilSortKey.cs
- CapabilitiesState.cs
- SecureEnvironment.cs
- RC2CryptoServiceProvider.cs
- Nodes.cs
- Site.cs
- ModelFactory.cs
- Utils.cs
- DataTemplateSelector.cs
- TripleDESCryptoServiceProvider.cs
- HasActivatableWorkflowEvent.cs
- ObjectReaderCompiler.cs
- Automation.cs
- SqlNamer.cs
- SqlDataReaderSmi.cs
- XmlDataSourceView.cs
- StringExpressionSet.cs
- DefaultExpressionVisitor.cs
- BoundField.cs
- CompressEmulationStream.cs
- HtmlElement.cs
- AsyncDataRequest.cs
- MgmtResManager.cs
- GlyphCollection.cs
- ScriptBehaviorDescriptor.cs
- HttpCachePolicy.cs
- Crc32Helper.cs
- EditorZoneBase.cs
- HierarchicalDataTemplate.cs
- ParagraphVisual.cs
- LogWriteRestartAreaState.cs
- GridItemPatternIdentifiers.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- ConfigXmlElement.cs
- Marshal.cs
- ReturnEventArgs.cs
- ThrowOnMultipleAssignment.cs
- TrackingProfileManager.cs
- ObjectToIdCache.cs
- recordstatefactory.cs
- StateMachine.cs
- QilVisitor.cs
- MouseActionConverter.cs
- AccessDataSource.cs
- NativeCppClassAttribute.cs
- OdbcConnectionFactory.cs
- Int64AnimationBase.cs
- CursorConverter.cs
- FixedPosition.cs
- MarkupCompiler.cs
- WebPartDeleteVerb.cs
- DbSetClause.cs
- SingleStorage.cs
- MSAANativeProvider.cs
- SelectionEditingBehavior.cs
- SoapIgnoreAttribute.cs
- XmlnsDictionary.cs
- BitmapDownload.cs
- ImageListUtils.cs
- ToolStripPanel.cs
- SqlUserDefinedAggregateAttribute.cs
- BamlLocalizabilityResolver.cs
- ProtectedConfigurationSection.cs
- TextServicesDisplayAttributePropertyRanges.cs
- OrderablePartitioner.cs
- PointConverter.cs
- NativeCppClassAttribute.cs
- XXXOnTypeBuilderInstantiation.cs
- ComNativeDescriptor.cs
- FixedDocumentSequencePaginator.cs
- ContextBase.cs
- DrawingAttributesDefaultValueFactory.cs
- SafeIUnknown.cs
- DataProtection.cs
- MILUtilities.cs
- PathData.cs
- GPPOINT.cs
- EventSource.cs
- NumberSubstitution.cs
- CacheAxisQuery.cs
- BaseParagraph.cs
- XmlAttributeHolder.cs
- Dispatcher.cs
- Content.cs
- PropertyDescriptor.cs
- XmlArrayItemAttribute.cs
- AccessDataSourceDesigner.cs
- TemplateBindingExpressionConverter.cs
- FileInfo.cs