Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Data / System / Data / DataViewManagerListItemTypeDescriptor.cs / 1305376 / DataViewManagerListItemTypeDescriptor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.ComponentModel; ////// internal sealed class DataViewManagerListItemTypeDescriptor : ICustomTypeDescriptor { private DataViewManager dataViewManager; private PropertyDescriptorCollection propsCollection; internal DataViewManagerListItemTypeDescriptor(DataViewManager dataViewManager) { this.dataViewManager = dataViewManager; } internal void Reset() { propsCollection = null; } internal DataView GetDataView(DataTable table) { DataView dataView = new DataView(table); dataView.SetDataViewManager(dataViewManager); return dataView; } ///[To be supplied.] ////// Retrieves an array of member attributes for the given object. /// AttributeCollection ICustomTypeDescriptor.GetAttributes() { return new AttributeCollection((Attribute[])null); } ////// Retrieves the class name for this object. If null is returned, /// the type name is used. /// string ICustomTypeDescriptor.GetClassName() { return null; } ////// Retrieves the name for this object. If null is returned, /// the default is used. /// string ICustomTypeDescriptor.GetComponentName() { return null; } ////// Retrieves the type converter for this object. /// TypeConverter ICustomTypeDescriptor.GetConverter() { return null; } ////// Retrieves the default event. /// EventDescriptor ICustomTypeDescriptor.GetDefaultEvent() { return null; } ////// Retrieves the default property. /// PropertyDescriptor ICustomTypeDescriptor.GetDefaultProperty() { return null; } ////// Retrieves the an editor for this object. /// object ICustomTypeDescriptor.GetEditor(Type editorBaseType) { return null; } ////// Retrieves an array of events that the given component instance /// provides. This may differ from the set of events the class /// provides. If the component is sited, the site may add or remove /// additional events. /// EventDescriptorCollection ICustomTypeDescriptor.GetEvents() { return new EventDescriptorCollection(null); } ////// Retrieves an array of events that the given component instance /// provides. This may differ from the set of events the class /// provides. If the component is sited, the site may add or remove /// additional events. The returned array of events will be /// filtered by the given set of attributes. /// EventDescriptorCollection ICustomTypeDescriptor.GetEvents(Attribute[] attributes) { return new EventDescriptorCollection(null); } ////// Retrieves an array of properties that the given component instance /// provides. This may differ from the set of properties the class /// provides. If the component is sited, the site may add or remove /// additional properties. /// PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties() { return((ICustomTypeDescriptor)this).GetProperties(null); } ////// Retrieves an array of properties that the given component instance /// provides. This may differ from the set of properties the class /// provides. If the component is sited, the site may add or remove /// additional properties. The returned array of properties will be /// filtered by the given set of attributes. /// PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties(Attribute[] attributes) { if (propsCollection == null) { PropertyDescriptor[] props = null; DataSet dataSet = dataViewManager.DataSet; if (dataSet != null) { int tableCount = dataSet.Tables.Count; props = new PropertyDescriptor[tableCount]; for (int i = 0; i < tableCount; i++) { props[i] = new DataTablePropertyDescriptor(dataSet.Tables[i]); } } propsCollection = new PropertyDescriptorCollection(props); } return propsCollection; } ////// Retrieves the object that directly depends on this value being edited. This is /// generally the object that is required for the PropertyDescriptor's GetValue and SetValue /// methods. If 'null' is passed for the PropertyDescriptor, the ICustomComponent /// descripotor implemementation should return the default object, that is the main /// object that exposes the properties and attributes, /// object ICustomTypeDescriptor.GetPropertyOwner(PropertyDescriptor pd) { return this; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.ComponentModel; ////// internal sealed class DataViewManagerListItemTypeDescriptor : ICustomTypeDescriptor { private DataViewManager dataViewManager; private PropertyDescriptorCollection propsCollection; internal DataViewManagerListItemTypeDescriptor(DataViewManager dataViewManager) { this.dataViewManager = dataViewManager; } internal void Reset() { propsCollection = null; } internal DataView GetDataView(DataTable table) { DataView dataView = new DataView(table); dataView.SetDataViewManager(dataViewManager); return dataView; } ///[To be supplied.] ////// Retrieves an array of member attributes for the given object. /// AttributeCollection ICustomTypeDescriptor.GetAttributes() { return new AttributeCollection((Attribute[])null); } ////// Retrieves the class name for this object. If null is returned, /// the type name is used. /// string ICustomTypeDescriptor.GetClassName() { return null; } ////// Retrieves the name for this object. If null is returned, /// the default is used. /// string ICustomTypeDescriptor.GetComponentName() { return null; } ////// Retrieves the type converter for this object. /// TypeConverter ICustomTypeDescriptor.GetConverter() { return null; } ////// Retrieves the default event. /// EventDescriptor ICustomTypeDescriptor.GetDefaultEvent() { return null; } ////// Retrieves the default property. /// PropertyDescriptor ICustomTypeDescriptor.GetDefaultProperty() { return null; } ////// Retrieves the an editor for this object. /// object ICustomTypeDescriptor.GetEditor(Type editorBaseType) { return null; } ////// Retrieves an array of events that the given component instance /// provides. This may differ from the set of events the class /// provides. If the component is sited, the site may add or remove /// additional events. /// EventDescriptorCollection ICustomTypeDescriptor.GetEvents() { return new EventDescriptorCollection(null); } ////// Retrieves an array of events that the given component instance /// provides. This may differ from the set of events the class /// provides. If the component is sited, the site may add or remove /// additional events. The returned array of events will be /// filtered by the given set of attributes. /// EventDescriptorCollection ICustomTypeDescriptor.GetEvents(Attribute[] attributes) { return new EventDescriptorCollection(null); } ////// Retrieves an array of properties that the given component instance /// provides. This may differ from the set of properties the class /// provides. If the component is sited, the site may add or remove /// additional properties. /// PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties() { return((ICustomTypeDescriptor)this).GetProperties(null); } ////// Retrieves an array of properties that the given component instance /// provides. This may differ from the set of properties the class /// provides. If the component is sited, the site may add or remove /// additional properties. The returned array of properties will be /// filtered by the given set of attributes. /// PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties(Attribute[] attributes) { if (propsCollection == null) { PropertyDescriptor[] props = null; DataSet dataSet = dataViewManager.DataSet; if (dataSet != null) { int tableCount = dataSet.Tables.Count; props = new PropertyDescriptor[tableCount]; for (int i = 0; i < tableCount; i++) { props[i] = new DataTablePropertyDescriptor(dataSet.Tables[i]); } } propsCollection = new PropertyDescriptorCollection(props); } return propsCollection; } ////// Retrieves the object that directly depends on this value being edited. This is /// generally the object that is required for the PropertyDescriptor's GetValue and SetValue /// methods. If 'null' is passed for the PropertyDescriptor, the ICustomComponent /// descripotor implemementation should return the default object, that is the main /// object that exposes the properties and attributes, /// object ICustomTypeDescriptor.GetPropertyOwner(PropertyDescriptor pd) { return this; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
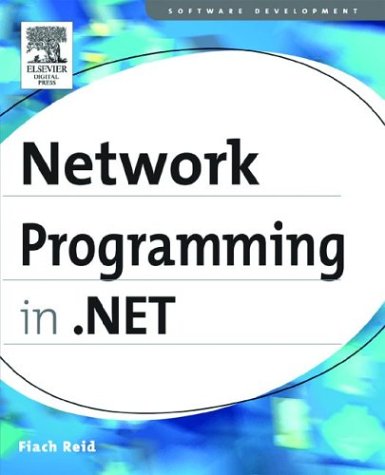
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NonceCache.cs
- RefType.cs
- ListViewContainer.cs
- UnhandledExceptionEventArgs.cs
- EntityType.cs
- SerializableAttribute.cs
- DataGridViewRowStateChangedEventArgs.cs
- DataBoundControl.cs
- ConsoleKeyInfo.cs
- WebPartRestoreVerb.cs
- ParameterSubsegment.cs
- PerfCounters.cs
- DynamicDiscoveryDocument.cs
- ObjectConverter.cs
- FixUpCollection.cs
- CombinedGeometry.cs
- Span.cs
- COM2PropertyDescriptor.cs
- InputDevice.cs
- ObjectStateEntryDbDataRecord.cs
- PermissionSet.cs
- Mapping.cs
- X509Utils.cs
- DllNotFoundException.cs
- ComplusEndpointConfigContainer.cs
- XamlWrapperReaders.cs
- InvalidOleVariantTypeException.cs
- EntityContainerAssociationSet.cs
- AsymmetricKeyExchangeDeformatter.cs
- AttributedMetaModel.cs
- XmlArrayAttribute.cs
- RbTree.cs
- AnnotationObservableCollection.cs
- ServerValidateEventArgs.cs
- StylusShape.cs
- RequestCacheManager.cs
- PropertyDescriptorComparer.cs
- TimeSpanValidator.cs
- DesignTimeVisibleAttribute.cs
- DataGridColumnHeadersPresenter.cs
- JapaneseLunisolarCalendar.cs
- LocalBuilder.cs
- SerializableAttribute.cs
- X509Utils.cs
- DescriptionAttribute.cs
- BuilderInfo.cs
- TypeConverterValueSerializer.cs
- InvalidOperationException.cs
- WorkflowMarkupSerializationProvider.cs
- TextFormatterHost.cs
- PagesSection.cs
- Aggregates.cs
- TreeNodeCollection.cs
- PreviewPrintController.cs
- RegularExpressionValidator.cs
- ResourcePool.cs
- Helpers.cs
- DataGridPagerStyle.cs
- listitem.cs
- UIElementCollection.cs
- ListBox.cs
- WebPartCollection.cs
- BStrWrapper.cs
- UiaCoreTypesApi.cs
- PublishLicense.cs
- SimpleWorkerRequest.cs
- WindowsEditBox.cs
- _SslSessionsCache.cs
- RegexReplacement.cs
- ClientFormsIdentity.cs
- ProfilePropertySettings.cs
- PrinterSettings.cs
- EnterpriseServicesHelper.cs
- XmlAttributeAttribute.cs
- WeakReferenceKey.cs
- SoapInteropTypes.cs
- SynchronizedReadOnlyCollection.cs
- XmlSchemaValidationException.cs
- Vector3DAnimation.cs
- xmlsaver.cs
- WindowsUpDown.cs
- ObjectViewEntityCollectionData.cs
- XslCompiledTransform.cs
- EdmRelationshipRoleAttribute.cs
- CheckBox.cs
- SplineKeyFrames.cs
- Typeface.cs
- SelectionListDesigner.cs
- AssemblyBuilder.cs
- DataGridParentRows.cs
- CodeVariableDeclarationStatement.cs
- TextElementCollection.cs
- OpCodes.cs
- DomNameTable.cs
- PhysicalOps.cs
- CheckBoxRenderer.cs
- RepeaterItem.cs
- JapaneseCalendar.cs
- CryptoApi.cs
- IconBitmapDecoder.cs