Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / Advanced / EncoderParameters.cs / 1305376 / EncoderParameters.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Text; using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Drawing.Internal; using Marshal = System.Runtime.InteropServices.Marshal; using System.Drawing; //[StructLayout(LayoutKind.Sequential)] ////// /// public sealed class EncoderParameters : IDisposable { EncoderParameter[] param; ///[To be supplied.] ////// /// public EncoderParameters(int count) { param = new EncoderParameter[count]; } ///[To be supplied.] ////// /// public EncoderParameters() { param = new EncoderParameter[1]; } ///[To be supplied.] ////// /// public EncoderParameter[] Param { // get { return param; } set { param = value; } } ///[To be supplied.] ////// Copy the EncoderParameters data into a chunk of memory to be consumed by native GDI+ code. /// /// We need to marshal the EncoderParameters info from/to native GDI+ ourselve since the definition of the managed/unmanaged classes /// are different and the native class is a bit weird. The native EncoderParameters class is defined in GDI+ as follows: /// /// class EncoderParameters { /// UINT Count; // Number of parameters in this structure /// EncoderParameter Parameter[1]; // Parameter values /// }; /// /// We don't have the 'Count' field since the managed array contains it. In order for this structure to work with more than one /// EncoderParameter we need to preallocate memory for the extra n-1 elements, something like this: /// /// EncoderParameters* pEncoderParameters = (EncoderParameters*) malloc(sizeof(EncoderParameters) + (n-1) * sizeof(EncoderParameter)); /// /// Also, in 64-bit platforms, 'Count' is aligned in 8 bytes (4 extra padding bytes) so we use IntPtr instead of Int32 to account for /// that (See VSW#451333). /// internal IntPtr ConvertToMemory() { int size = Marshal.SizeOf(typeof(EncoderParameter)); IntPtr memory = Marshal.AllocHGlobal(param.Length * size + Marshal.SizeOf(typeof(IntPtr))); if (memory == IntPtr.Zero){ throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); } Marshal.WriteIntPtr(memory, (IntPtr) param.Length); long arrayOffset = (long) memory + Marshal.SizeOf(typeof(IntPtr)); for (int i=0; i/// Copy the native GDI+ EncoderParameters data from a chunk of memory into a managed EncoderParameters object. /// See ConvertToMemory for more info. /// [SuppressMessage("Microsoft.Performance", "CA1808:AvoidCallsThatBoxValueTypes")] internal static EncoderParameters ConvertFromMemory(IntPtr memory) { if (memory == IntPtr.Zero) { throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); } int count = Marshal.ReadIntPtr(memory).ToInt32(); EncoderParameters p = new EncoderParameters(count); int size = Marshal.SizeOf(typeof(EncoderParameter)); long arrayOffset = (long)memory + Marshal.SizeOf(typeof(IntPtr)); for (int i=0; i public void Dispose() { foreach (EncoderParameter p in param) { if( p != null ){ p.Dispose(); } } param = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Text; using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Drawing.Internal; using Marshal = System.Runtime.InteropServices.Marshal; using System.Drawing; //[StructLayout(LayoutKind.Sequential)] ////// /// public sealed class EncoderParameters : IDisposable { EncoderParameter[] param; ///[To be supplied.] ////// /// public EncoderParameters(int count) { param = new EncoderParameter[count]; } ///[To be supplied.] ////// /// public EncoderParameters() { param = new EncoderParameter[1]; } ///[To be supplied.] ////// /// public EncoderParameter[] Param { // get { return param; } set { param = value; } } ///[To be supplied.] ////// Copy the EncoderParameters data into a chunk of memory to be consumed by native GDI+ code. /// /// We need to marshal the EncoderParameters info from/to native GDI+ ourselve since the definition of the managed/unmanaged classes /// are different and the native class is a bit weird. The native EncoderParameters class is defined in GDI+ as follows: /// /// class EncoderParameters { /// UINT Count; // Number of parameters in this structure /// EncoderParameter Parameter[1]; // Parameter values /// }; /// /// We don't have the 'Count' field since the managed array contains it. In order for this structure to work with more than one /// EncoderParameter we need to preallocate memory for the extra n-1 elements, something like this: /// /// EncoderParameters* pEncoderParameters = (EncoderParameters*) malloc(sizeof(EncoderParameters) + (n-1) * sizeof(EncoderParameter)); /// /// Also, in 64-bit platforms, 'Count' is aligned in 8 bytes (4 extra padding bytes) so we use IntPtr instead of Int32 to account for /// that (See VSW#451333). /// internal IntPtr ConvertToMemory() { int size = Marshal.SizeOf(typeof(EncoderParameter)); IntPtr memory = Marshal.AllocHGlobal(param.Length * size + Marshal.SizeOf(typeof(IntPtr))); if (memory == IntPtr.Zero){ throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); } Marshal.WriteIntPtr(memory, (IntPtr) param.Length); long arrayOffset = (long) memory + Marshal.SizeOf(typeof(IntPtr)); for (int i=0; i/// Copy the native GDI+ EncoderParameters data from a chunk of memory into a managed EncoderParameters object. /// See ConvertToMemory for more info. /// [SuppressMessage("Microsoft.Performance", "CA1808:AvoidCallsThatBoxValueTypes")] internal static EncoderParameters ConvertFromMemory(IntPtr memory) { if (memory == IntPtr.Zero) { throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); } int count = Marshal.ReadIntPtr(memory).ToInt32(); EncoderParameters p = new EncoderParameters(count); int size = Marshal.SizeOf(typeof(EncoderParameter)); long arrayOffset = (long)memory + Marshal.SizeOf(typeof(IntPtr)); for (int i=0; i public void Dispose() { foreach (EncoderParameter p in param) { if( p != null ){ p.Dispose(); } } param = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
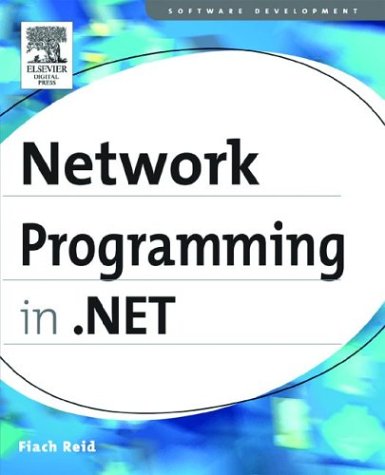
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LookupBindingPropertiesAttribute.cs
- TextTrailingCharacterEllipsis.cs
- ClientConfigurationHost.cs
- OraclePermission.cs
- Package.cs
- HostedHttpContext.cs
- Guid.cs
- BaseWebProxyFinder.cs
- RegistryPermission.cs
- ShaderEffect.cs
- AssemblyAssociatedContentFileAttribute.cs
- HierarchicalDataSourceDesigner.cs
- CommandHelpers.cs
- QilInvokeLateBound.cs
- PriorityChain.cs
- OpenCollectionAsyncResult.cs
- WebFaultClientMessageInspector.cs
- Style.cs
- SQLMoneyStorage.cs
- DesignBinding.cs
- Events.cs
- Function.cs
- HttpCacheParams.cs
- ObjectDataSource.cs
- TextDecorationUnitValidation.cs
- CompareValidator.cs
- PathSegment.cs
- ServicePointManagerElement.cs
- DispatchChannelSink.cs
- XamlHttpHandlerFactory.cs
- WmlCalendarAdapter.cs
- QueryGenerator.cs
- FixedHyperLink.cs
- WindowsListViewGroup.cs
- StatusCommandUI.cs
- QueryOperationResponseOfT.cs
- UpdateTracker.cs
- PrincipalPermission.cs
- WriterOutput.cs
- COM2ComponentEditor.cs
- TextChangedEventArgs.cs
- Symbol.cs
- QuaternionAnimation.cs
- XPathBinder.cs
- DrawListViewSubItemEventArgs.cs
- XmlBindingWorker.cs
- ProtectedConfiguration.cs
- MetadataFile.cs
- SerializationStore.cs
- SerialStream.cs
- SweepDirectionValidation.cs
- MemoryPressure.cs
- DefaultMergeHelper.cs
- RequestChannelBinder.cs
- SmtpReplyReaderFactory.cs
- UpdateTranslator.cs
- HtmlString.cs
- CalendarButtonAutomationPeer.cs
- ExpressionUtilities.cs
- ExpressionBuilder.cs
- ImageSourceValueSerializer.cs
- RadioButton.cs
- LockedActivityGlyph.cs
- Configuration.cs
- EventLogPermissionEntryCollection.cs
- InspectionWorker.cs
- InfoCardAsymmetricCrypto.cs
- MetadataItemCollectionFactory.cs
- StrokeDescriptor.cs
- AuthorizationSection.cs
- MoveSizeWinEventHandler.cs
- ConfigurationStrings.cs
- DataServiceRequest.cs
- LiteralDesigner.cs
- VerticalAlignConverter.cs
- RolePrincipal.cs
- BufferModeSettings.cs
- MdiWindowListStrip.cs
- TextModifierScope.cs
- DBSqlParserTableCollection.cs
- ColorInterpolationModeValidation.cs
- BaseDataBoundControl.cs
- BeginEvent.cs
- ReverseComparer.cs
- _SslStream.cs
- MappableObjectManager.cs
- HierarchicalDataSourceControl.cs
- GiveFeedbackEventArgs.cs
- SQLByte.cs
- UInt16.cs
- ConnectionStringSettings.cs
- FilteredSchemaElementLookUpTable.cs
- DataGridCell.cs
- ObjectRef.cs
- NullableLongAverageAggregationOperator.cs
- CodeNamespace.cs
- OracleCommandBuilder.cs
- TransformPattern.cs
- DataBindingsDialog.cs
- XmlUrlResolver.cs