Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / X509CertificateTokenFactoryCredential.cs / 1 / X509CertificateTokenFactoryCredential.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.IO; using System.Security.Cryptography.X509Certificates; using System.Security.Cryptography.Xml; using System.Text; internal class X509CertificateTokenFactoryCredential : TokenFactoryCredential { X509Certificate2 m_cert; string m_contextKey; string m_portName; UIAgentRequest m_request; bool m_disposed = false; object m_sync = new object(); public X509CertificateTokenFactoryCredential( UIAgentRequest request ) : base( TokenFactoryCredentialType.X509CertificateCredential ) { m_request = request; } // // Get/Set the context key for the credential. // public string ContextKey { get{ return m_contextKey; } } // // Summary: // Get/Set the Rpc Port name // public string PortName { get{ return m_portName; } } // // Summary: // Get/Set certificate // public X509Certificate2 Certificate { get{ return m_cert; } } // // Summary: // Populate class members from reader // protected override void DeserializeData( BinaryReader reader ) { m_portName = Utility.DeserializeString( reader ); m_contextKey = Utility.DeserializeString( reader ); byte[] certBytes = new byte[ reader.ReadInt32() ]; reader.Read( certBytes, 0, certBytes.Length ); m_cert = new X509Certificate2( certBytes ); m_cert.PrivateKey = new RemoteCryptoRsaServiceProvider( this, m_request ); } public override void Dispose( bool disposing ) { if ( m_disposed ) { return; } lock( m_sync ) { if( m_disposed ) { return; } try { if( disposing ) { // // Dispose managed resources // // // This internall calls PrivateKey.Dispose which in turn closes the // smartcard context // this.m_cert.PrivateKey.Clear(); this.m_cert.PublicKey.Key.Clear(); } // // Dispose unmanaged resources // m_disposed = true; } finally { base.Dispose( disposing ); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
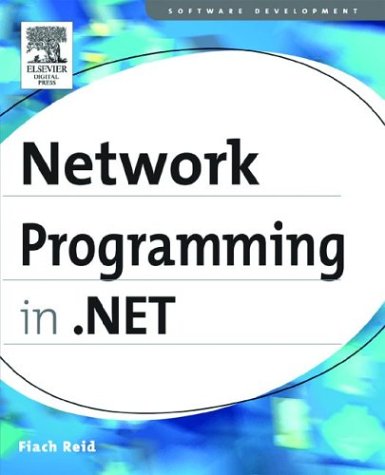
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MetafileHeaderWmf.cs
- DecimalMinMaxAggregationOperator.cs
- UnsignedPublishLicense.cs
- XmlChildNodes.cs
- HttpServerVarsCollection.cs
- CaseCqlBlock.cs
- TypeResolver.cs
- KeyPressEvent.cs
- HtmlTableRow.cs
- SemanticResolver.cs
- RegexGroupCollection.cs
- HtmlForm.cs
- XmlMemberMapping.cs
- EmptyStringExpandableObjectConverter.cs
- DataPointer.cs
- X509CertificateClaimSet.cs
- Accessors.cs
- PropVariant.cs
- HelpEvent.cs
- MenuAutomationPeer.cs
- RowUpdatedEventArgs.cs
- NameHandler.cs
- SolidColorBrush.cs
- SizeF.cs
- BatchParser.cs
- Point4DConverter.cs
- PropertyCondition.cs
- XmlSequenceWriter.cs
- ResourceAssociationTypeEnd.cs
- SmtpDigestAuthenticationModule.cs
- SqlSupersetValidator.cs
- MatrixCamera.cs
- ProbeDuplexCD1AsyncResult.cs
- columnmapfactory.cs
- DataGridColumn.cs
- ReplyChannelAcceptor.cs
- ContainerCodeDomSerializer.cs
- PtsContext.cs
- EventSinkHelperWriter.cs
- TabletDevice.cs
- BitmapEffect.cs
- GeneralTransform3DGroup.cs
- NativeWindow.cs
- Base64Stream.cs
- MaterialGroup.cs
- HostedElements.cs
- RenamedEventArgs.cs
- UnsafeNativeMethods.cs
- KernelTypeValidation.cs
- ObjectQueryState.cs
- RTLAwareMessageBox.cs
- ValidationHelper.cs
- FormsAuthenticationUserCollection.cs
- Rotation3DAnimationBase.cs
- DataGridCommandEventArgs.cs
- RectAnimationBase.cs
- DocComment.cs
- Convert.cs
- DotAtomReader.cs
- safesecurityhelperavalon.cs
- NetworkStream.cs
- Int16Converter.cs
- Directory.cs
- VisualBrush.cs
- PseudoWebRequest.cs
- LabelAutomationPeer.cs
- InvalidEnumArgumentException.cs
- KeySpline.cs
- HttpRuntime.cs
- ZoneMembershipCondition.cs
- LambdaExpression.cs
- Calendar.cs
- InstanceDataCollectionCollection.cs
- Debug.cs
- PackagePartCollection.cs
- DiagnosticTrace.cs
- DeviceContexts.cs
- InkPresenter.cs
- ConditionCollection.cs
- LambdaCompiler.Generated.cs
- MLangCodePageEncoding.cs
- ColorMatrix.cs
- Random.cs
- RectConverter.cs
- FileLoadException.cs
- HttpConfigurationSystem.cs
- ConnectionPointCookie.cs
- ADMembershipUser.cs
- TransportOutputChannel.cs
- TemplatePropertyEntry.cs
- CreateUserWizardAutoFormat.cs
- ServiceOperationParameter.cs
- BufferBuilder.cs
- ChannelListenerBase.cs
- BindUriHelper.cs
- DesignerVerb.cs
- InfoCardSymmetricCrypto.cs
- CodeTypeConstructor.cs
- ListViewItem.cs
- _ScatterGatherBuffers.cs