Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / InfoCardSchemas.cs / 1 / InfoCardSchemas.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using System.IO; using System.Reflection; using System.Text; using System.Xml; using System.Xml.Schema; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; class InfoCardSchemas { // // A list of resources names that will be serialized to XmlSchema objects // and added directly to the XmlSchemaSet // static readonly string[] s_primarySchemas = new string[] { XmlNames.WSIdentity.LocalSchemaLocation, XmlNames.WSIdentity07.LocalSchemaLocation }; // // A list of SchemaLocation and ResourceName entries that will be // resolved on demand as needed. // static readonly SchemaLocationResourceMapping[] s_supportingSchemas = new SchemaLocationResourceMapping[] { new SchemaLocationResourceMapping( XmlNames.WSAddressing.LocalSchemaLocation, XmlNames.WSAddressing.SchemaLocation ), new SchemaLocationResourceMapping( XmlNames.WSAddressing04.LocalSchemaLocation, XmlNames.WSAddressing04.SchemaLocation ), new SchemaLocationResourceMapping( XmlNames.XmlDSig.LocalSchemaLocation, XmlNames.XmlDSig.SchemaLocation ), new SchemaLocationResourceMapping( XmlNames.XmlSchema.LocalSchemaLocation, XmlNames.XmlSchema.SchemaLocation ), new SchemaLocationResourceMapping( XmlNames.XmlEnc.LocalSchemaLocation, XmlNames.XmlEnc.SchemaLocation ), new SchemaLocationResourceMapping( XmlNames.WSSecurityExt.LocalSchemaLocation, XmlNames.WSSecurityExt.SchemaLocation ), new SchemaLocationResourceMapping( XmlNames.WSSecurityUtility.LocalSchemaLocation, XmlNames.WSSecurityUtility.SchemaLocation ), new SchemaLocationResourceMapping( XmlNames.WSPolicyXmlSoap2004.Instance.LocalSchemaLocation, XmlNames.WSPolicyXmlSoap2004.Instance.SchemaLocation ), new SchemaLocationResourceMapping( XmlNames.WSPolicyW32007.Instance.LocalSchemaLocation, XmlNames.WSPolicyW32007.Instance.SchemaLocation ), new SchemaLocationResourceMapping( XmlNames.WSSecurityPolicyXmlSoap2005.Instance.LocalSchemaLocation, XmlNames.WSSecurityPolicyXmlSoap2005.Instance.SchemaLocation ), new SchemaLocationResourceMapping( XmlNames.WSSecurityPolicyOasis2007.Instance.LocalSchemaLocation, XmlNames.WSSecurityPolicyOasis2007.Instance.SchemaLocation ), new SchemaLocationResourceMapping( XmlNames.WSTrustXmlSoap2005.Instance.LocalSchemaLocation, XmlNames.WSTrustXmlSoap2005.Instance.SchemaLocation ), new SchemaLocationResourceMapping( XmlNames.WSTrustOasis2007.Instance.LocalSchemaLocation, XmlNames.WSTrustOasis2007.Instance.SchemaLocation ), }; static InfoCardSchemas s_current = new InfoCardSchemas( Assembly.GetExecutingAssembly() ); LocalCachingResolver m_resolver; Assembly m_assembly; XmlSchemaSet m_schemas; object m_sync; // // Private CTOR for singleton // private InfoCardSchemas( Assembly sourceAssembly ) { m_sync = new object(); m_resolver = new LocalCachingResolver(); m_assembly = sourceAssembly; } // // Summary: // Returns the XmlSchemaSet that can be used for schema validation. // public static XmlSchemaSet GetSchemaSet() { if( null == s_current.m_schemas ) { lock( s_current.m_sync ) { if( null == s_current.m_schemas ) { s_current.InitializeSchemas(); } } } return s_current.m_schemas; } public static XmlReader CreateReader( string fragment ) { return InfoCardSchemas.CreateReader( Utility.CreateReaderWithQuotas( fragment ) ); } #if DEBUG // // Summary: // Creates a new validating XmlReader wrapping the specified stream. // // Remarks: // Only invoked by ExportFileRequest.cs -- Need to wrap in #if DEBUG // to pass FXCOP AvoidUncalledPrivateCode rule. // public static XmlReader CreateReader( FileStream stream ) { return CreateReader( Utility.CreateReaderWithQuotas( stream ) ); } #endif // // Summary: // Creates a new validating XmlReader wrapping the specified stream. // public static XmlReader CreateReader( Stream stream, XmlReaderSettings settings ) { return CreateReader( Utility.CreateReaderWithQuotas( stream ), settings ); } // // Summary: // Creates a new validating XmlReader wrapping the specified reader. // public static XmlReader CreateReader( XmlDictionaryReader reader, XmlReaderSettings settings ) { // // Same as XmlReader.Create because XmlDictionaryReader.Create is not overriden. // However, it is just more explicit. // return XmlDictionaryReader.Create( reader, settings ); } // // Summary: // Creates a new validating XmlReader wrapping the specified reader. // public static XmlReader CreateReader( XmlDictionaryReader reader ) { XmlReaderSettings settings = CreateDefaultReaderSettings(); // // Same as XmlReader.Create because XmlDictionaryReader.Create is not overriden. // However, it is just more explicit. // return XmlDictionaryReader.Create( reader, settings ); } // // Summary: // Creates the default validating reader setting that infocard uses // public static XmlReaderSettings CreateDefaultReaderSettings() { XmlReaderSettings settings = new XmlReaderSettings(); settings.CloseInput = false; settings.ValidationType = ValidationType.Schema; settings.ValidationFlags = XmlSchemaValidationFlags.ReportValidationWarnings; settings.ConformanceLevel = ConformanceLevel.Auto; settings.Schemas = GetSchemaSet(); settings.ValidationEventHandler += new ValidationEventHandler( ErrorHandler ); settings.XmlResolver = s_current.m_resolver; settings.IgnoreComments = true; settings.IgnoreWhitespace = true; settings.IgnoreProcessingInstructions = true; return settings; } // // Summary: // Initialize and load all schemas // void InitializeSchemas() { XmlSchema currentSchema = null; XmlSchemaSet set = null; LocalCachingResolver resolver = null; Stream manifestStream = null; Uri schemaLocation = null; resolver = new LocalCachingResolver(); // // Load all supporting schemas in to the local caching resolver. // for( int i = 0 ; i < s_supportingSchemas.Length ; i++ ) { schemaLocation = new Uri( s_supportingSchemas[ i ].SchemaLocation ); manifestStream = m_assembly.GetManifestResourceStream( s_supportingSchemas[ i ].ResourceName ); currentSchema = XmlSchema.Read( manifestStream, new ValidationEventHandler( ErrorHandler ) ); resolver.AddLocalSchema( schemaLocation, currentSchema ); } // // Load all of the primary schemsa into the schema set. // set = new XmlSchemaSet(); set.XmlResolver = resolver; for( int i = 0; i < s_primarySchemas.Length; i++ ) { manifestStream = m_assembly.GetManifestResourceStream( s_primarySchemas[ i ] ); currentSchema = XmlSchema.Read( manifestStream, new ValidationEventHandler( ErrorHandler ) ); set.Add( currentSchema ); } set.ValidationEventHandler += new ValidationEventHandler( ErrorHandler ); set.Compile(); m_resolver = resolver; m_schemas = set; } // // Summary: // Error handler for all Schema validations, including loading and processing of schema files. // static void ErrorHandler( object sender, ValidationEventArgs args ) { IDT.TraceDebug( "SCHEMA: {0}", args.Exception.Message ); if( XmlSeverityType.Error == args.Severity ) { // // The stack trace is NOT included with args.Exception.Message so we should // not be leaking that information. // throw IDT.ThrowHelperCritical( new XmlSchemaValidationException( SR.GetString( SR.SchemaValidationError, args.Exception.Message ), args.Exception ) ); } } // // Summary: // XmlUrlResolver that keeps a cache of XmlSchems that can be populated to prevent // off box travel. // class LocalCachingResolver : XmlUrlResolver { Dictionarym_table; public LocalCachingResolver() { m_table = new Dictionary (); } // // Summary: // Add the specified schema to be resolved at the specified location. // public void AddLocalSchema( Uri location, XmlSchema schema ) { m_table.Add( location, schema ); } // // Summary: // Resolve the uri. // public override Uri ResolveUri( Uri baseUri, string relativeUri ) { return base.ResolveUri( baseUri, relativeUri ); } // // Summary: // Map the XmlSchema object to the aboluteUri. // public override object GetEntity( Uri absoluteUri, string role, Type ofObjectToReturn ) { return ( XmlSchema )m_table[ absoluteUri ]; } } // // Summary: // Mapping class to map a internal resource name to a schema location url. // class SchemaLocationResourceMapping { string m_resourceName; string m_schemaLocation; public SchemaLocationResourceMapping( string resourceName, string schemaLocation ) { m_resourceName = resourceName; m_schemaLocation = schemaLocation; } public string ResourceName { get{ return m_resourceName; } } public string SchemaLocation { get{ return m_schemaLocation; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
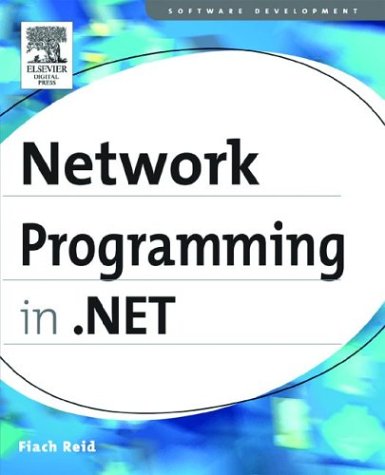
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TimeoutConverter.cs
- ApplicationInterop.cs
- StagingAreaInputItem.cs
- RunInstallerAttribute.cs
- SqlError.cs
- AutoFocusStyle.xaml.cs
- WindowProviderWrapper.cs
- SwitchExpression.cs
- ListControl.cs
- __TransparentProxy.cs
- PageThemeParser.cs
- CharConverter.cs
- Environment.cs
- XamlStyleSerializer.cs
- AxHost.cs
- CfgParser.cs
- XmlConverter.cs
- XmlAttributeCollection.cs
- DataViewListener.cs
- CharacterShapingProperties.cs
- PageAction.cs
- CheckBoxStandardAdapter.cs
- StrongNamePublicKeyBlob.cs
- DoubleCollection.cs
- GenericPrincipal.cs
- ServiceNotStartedException.cs
- FullTrustAssemblyCollection.cs
- ResourceCodeDomSerializer.cs
- ICollection.cs
- UnsettableComboBox.cs
- ProcessThread.cs
- XmlSchemaType.cs
- FunctionQuery.cs
- WindowsComboBox.cs
- SplitContainerDesigner.cs
- MouseButton.cs
- WorkflowFormatterBehavior.cs
- PolicyManager.cs
- SimpleHandlerFactory.cs
- NumberAction.cs
- WindowInteractionStateTracker.cs
- ConstNode.cs
- ObjectQueryExecutionPlan.cs
- DataGrid.cs
- ConnectionInterfaceCollection.cs
- StyleSheet.cs
- DataGridTextBox.cs
- ProviderSettingsCollection.cs
- Button.cs
- FontUnit.cs
- ControlUtil.cs
- DiffuseMaterial.cs
- BulletDecorator.cs
- WorkflowQueue.cs
- __Filters.cs
- BulletedList.cs
- __ConsoleStream.cs
- Literal.cs
- SpecialFolderEnumConverter.cs
- sqlpipe.cs
- LinqDataSourceContextEventArgs.cs
- PersistenceTypeAttribute.cs
- TransactionBehavior.cs
- PartitionerStatic.cs
- ColumnMap.cs
- PointConverter.cs
- odbcmetadatacolumnnames.cs
- PeerName.cs
- ApplicationServiceManager.cs
- ValidationEventArgs.cs
- TextSpanModifier.cs
- WebPartConnectionsCancelVerb.cs
- EntityDataSourceWizardForm.cs
- DataFormats.cs
- EntitySetBaseCollection.cs
- WebPartExportVerb.cs
- StatusBarItem.cs
- CodeVariableReferenceExpression.cs
- Point4DValueSerializer.cs
- MultipartContentParser.cs
- XNodeSchemaApplier.cs
- List.cs
- FontFamily.cs
- PackWebResponse.cs
- milexports.cs
- XmlSerializerVersionAttribute.cs
- RootBuilder.cs
- Cursor.cs
- CredentialCache.cs
- BindingOperations.cs
- TextView.cs
- WriteLineDesigner.xaml.cs
- FormClosedEvent.cs
- Stack.cs
- IsolatedStorageFileStream.cs
- EventMappingSettingsCollection.cs
- RuntimeHandles.cs
- ClientTarget.cs
- FileAuthorizationModule.cs
- ProxyDataContractResolver.cs