Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / GetLedgerEntryForRecipientRequest.cs / 1 / GetLedgerEntryForRecipientRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.IO; using System.Text; using System.Collections; using System.Collections.Generic; using Microsoft.InfoCards.Diagnostics; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // This class handles the request from the UI Agent to get the Ledger information // for a specific recipient. // class GetLedgerEntryForRecipientRequest : UIAgentRequest { Uri m_cardId; string m_recipientId; byte[] m_bytes; // // Summary: // Constructs a new SetUserPreferenceRequest instance. // // Parameters: // rpcHandle - Handle to the RPC call from the UI Agent // inArgs - Stream for the incoming information. Null in this case. // outArgs - Stream used to collect the outbound data. // parent - Client UI Request. // public GetLedgerEntryForRecipientRequest( IntPtr rpcHandle, Stream inArgs, Stream outArgs, ClientUIRequest parent ) : base( rpcHandle, inArgs, outArgs, parent ) { } // // Summary // Event for marshalling the request information // protected override void OnMarshalInArgs() { IDT.Assert( InArgs.Length > 0, "The user preferences must be specified" ); BinaryReader br = new InfoCardBinaryReader( InArgs, Encoding.Unicode ); m_cardId = Utility.DeserializeUri( br ); m_recipientId = Utility.DeserializeString( br ); } // // Summary // Event for processing the user request // protected override void OnProcess() { IDT.Assert( null != m_cardId, "The card Id must not be null" ); IDT.Assert( false == String.IsNullOrEmpty( m_recipientId ), "The thumbPrint must be specified" ); ListparamList = new List (); QueryParameter query = new QueryParameter( SecondaryIndexDefinition.ObjectTypeIndex, (Int32)StorableObjectType.LedgerEntry ); paramList.Add( query ); query = new QueryParameter( SecondaryIndexDefinition.ParentIdIndex, GlobalId.DeriveFrom( m_cardId.ToString() ) ); paramList.Add( query ); paramList.Add( new QueryParameter( SecondaryIndexDefinition.RecipientIdIndex, m_recipientId ) ); StoreConnection connection = StoreConnection.GetConnection(); try { // // There should be only one row of this type for each recipient. So just return the first one. // DataRow row = connection.GetSingleRow( paramList.ToArray() ); if( null != row ) { m_bytes = row.GetDataField(); } } finally { connection.Close(); } } // // Summary // Event for marshalling the response information // // Remarks // Write nothing if the ledger entry is not found. // protected override void OnMarshalOutArgs() { if( null != m_bytes ) { OutArgs.Write( m_bytes, 0, m_bytes.Length ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
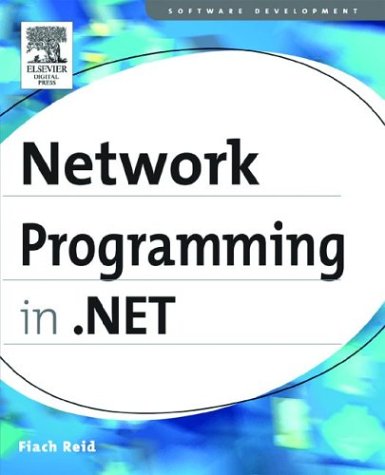
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridTableCollection.cs
- Operand.cs
- _SecureChannel.cs
- TableRowCollection.cs
- ColumnCollectionEditor.cs
- SystemColors.cs
- EntityFunctions.cs
- CachedCompositeFamily.cs
- DataColumnMappingCollection.cs
- SqlDataAdapter.cs
- ControlCachePolicy.cs
- RotateTransform3D.cs
- _SslSessionsCache.cs
- ListDataBindEventArgs.cs
- BamlBinaryReader.cs
- ELinqQueryState.cs
- IntranetCredentialPolicy.cs
- LogAppendAsyncResult.cs
- NetMsmqBindingCollectionElement.cs
- CultureTable.cs
- Tuple.cs
- SamlAssertion.cs
- DynamicEndpointElement.cs
- InternalBufferOverflowException.cs
- AssemblyNameUtility.cs
- GridToolTip.cs
- AddInControllerImpl.cs
- ProxyGenerationError.cs
- SystemResources.cs
- LinkConverter.cs
- QilSortKey.cs
- NotifyParentPropertyAttribute.cs
- HostProtectionPermission.cs
- ScriptRegistrationManager.cs
- BCLDebug.cs
- ServiceElement.cs
- SqlRowUpdatedEvent.cs
- TypeLibConverter.cs
- MediaTimeline.cs
- PersonalizableTypeEntry.cs
- EventLogReader.cs
- WebPartRestoreVerb.cs
- SchemaMerger.cs
- DocumentApplication.cs
- Operator.cs
- PrintingPermissionAttribute.cs
- PointLight.cs
- StatusBarAutomationPeer.cs
- TextCompositionEventArgs.cs
- Point3D.cs
- TypeConverters.cs
- SourceLineInfo.cs
- DiscoveryDocumentSerializer.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- Error.cs
- SHA512Cng.cs
- AsymmetricAlgorithm.cs
- CultureInfo.cs
- Vars.cs
- ProvidersHelper.cs
- HuffmanTree.cs
- FlagsAttribute.cs
- GroupBox.cs
- EncodedStreamFactory.cs
- PolicyException.cs
- UnmanagedMemoryStream.cs
- HttpException.cs
- MatrixTransform.cs
- SingleObjectCollection.cs
- BevelBitmapEffect.cs
- FormsAuthenticationConfiguration.cs
- FontStyles.cs
- ObjectKeyFrameCollection.cs
- NullRuntimeConfig.cs
- QilParameter.cs
- HttpResponseInternalWrapper.cs
- ContentPropertyAttribute.cs
- SchemaDeclBase.cs
- CssStyleCollection.cs
- Light.cs
- XmlSerializerVersionAttribute.cs
- PrintingPermission.cs
- ControlCommandSet.cs
- RemotingSurrogateSelector.cs
- BlockCollection.cs
- DoubleLink.cs
- QueryReaderSettings.cs
- MultipleViewPattern.cs
- safelink.cs
- DropSource.cs
- SafeBitVector32.cs
- NameValuePair.cs
- ObjectStateEntry.cs
- SelectionWordBreaker.cs
- CustomErrorCollection.cs
- ActivationServices.cs
- ColumnCollection.cs
- ObjectReferenceStack.cs
- BooleanProjectedSlot.cs
- __ComObject.cs