Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / CustomTokenProvider.cs / 1 / CustomTokenProvider.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // // Presharp uses the c# pragma mechanism to supress its warnings. // These are not recognised by the base compiler so we need to explictly // disable the following warnings. See http://winweb/cse/Tools/PREsharp/userguide/default.asp // for details. // #pragma warning disable 1634, 1691 // unknown message, unknown pragma namespace Microsoft.InfoCards { using System; using System.IdentityModel.Selectors; using System.IdentityModel.Tokens; using System.ServiceModel; using System.ServiceModel.Security; using System.ServiceModel.Security.Tokens; using System.Runtime.InteropServices; using System.Security.Cryptography; using System.IdentityModel; using System.Security.Cryptography.Xml; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; using System.Collections.ObjectModel; using System.Collections.Generic; // // This class is used to provide a custom token provider for self issued card authentication // internal class CustomTokenProvider : SecurityTokenProvider, IDisposable { InfoCardPolicy m_policy; InfoCard m_card; IssuedSecurityTokenParameters m_parameters; TokenDescriptor m_token; EndpointAddress m_target; SelfIssuedSamlTokenFactory m_tokenFactory; bool m_isSelfIssuedCreds; ProtocolProfile m_protocolProfile; SelfIssuedAuthProofToken m_proofToken; public CustomTokenProvider( IssuedSecurityTokenParameters parameters, InfoCard card, EndpointAddress target, bool isSelfIssuedCreds, ProtocolProfile profile ) { m_isSelfIssuedCreds = isSelfIssuedCreds; m_card = card; m_parameters = parameters; m_target = target; m_protocolProfile = profile; ValidatePolicy(); m_tokenFactory = new SelfIssuedSamlTokenFactory(); } public void Dispose() { // // We must dispose this handle explicitly. // if ( null != m_token ) { m_token.Dispose(); m_token = null; } // // We must dispose this proof token explicitly. // if ( null != m_proofToken ) { m_proofToken.Dispose(); m_proofToken = null; } } void ValidatePolicy() { try { m_policy = PolicyFactory.CreatePolicyForCustomTokenProvider( m_target, m_parameters, m_protocolProfile ); // // For customTokenProvider, the party we're encrypting the token to, an IP/STS, is also our relying party // for the self-issued or X509 token. So ImmediateTokenRecipient == Recipient in this case. // Manually set the recipient cert to allow us to create the RPID, OrgId etc. subsequently // when Validate is called. // m_policy.SetRecipientInfo( m_policy.ImmediateTokenRecipient, null, 0 ); // // The custom token provider is also used in X509 creds code path // so need to have m_isSelfIssuedCreds to distinguish the two. // if ( m_isSelfIssuedCreds ) { m_policy.ThrowIfNonPpidClaimsPresent(); } m_policy.Validate(); } catch ( Exception e ) { if ( IDT.IsFatal( e ) ) { throw; } // // We need to throw here. // We do not want any other providers to // to be able to handle this request. // IDT.TraceDebug( "Falied to read IP STS policy: {0}", e.ToString() ); throw IDT.ThrowHelperError( new TrustExchangeException( SR.GetString( SR.FailedReadingIPSTSPolicy ), e ) ); } } // // Summary // Retrieves a token from the system // // Parameters // address - The address of the recipient // timeout - The time span till the call times out // // Returns // The security token. // protected override SecurityToken GetTokenCore( TimeSpan timeout ) { // // Retrieve a connection for the card (may be need to fetch ledger entries) // StoreConnection connection = StoreConnection.GetConnection(); m_card.Connection = connection; try { m_token = m_tokenFactory.CreateToken( m_card, null, m_policy, false ); if ( null == m_token.SymmetricProof ) { // // Private because we need the private key for proof of possesion. // The regular self-issued case does not need the private key // in the proof crypto because we hand out the CryptoSession handle // to do the proof of possession for us. // m_proofToken = new SelfIssuedAuthProofToken( m_card.GetPrivateCryptography( m_policy.Recipient.GetIdentifier() ), m_token.ExpirationTime ); } else { m_proofToken = new SelfIssuedAuthProofToken( new InMemorySymmetricSecurityKey( m_token.SymmetricProof.Key ), m_token.ExpirationTime ); } return new GenericXmlSecurityToken( m_token.ProtectedToken, m_proofToken, m_token.EffectiveTime, m_token.ExpirationTime, new SamlAssertionKeyIdentifierClause( m_token.TokenId ), null, null ); } finally { connection.Close(); m_card.Connection = null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
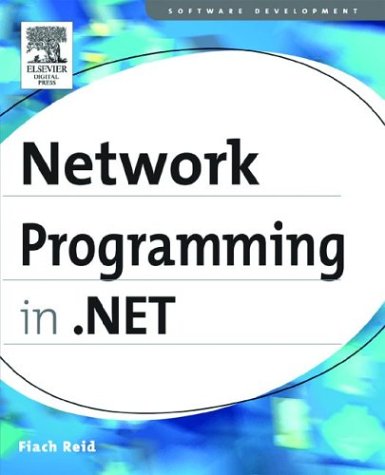
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QueryOptionExpression.cs
- TraceLog.cs
- XmlEncodedRawTextWriter.cs
- ColorIndependentAnimationStorage.cs
- TableStyle.cs
- AttributeUsageAttribute.cs
- NativeMethods.cs
- DesignerEventService.cs
- PrintPageEvent.cs
- Storyboard.cs
- ExceptionNotification.cs
- StreamResourceInfo.cs
- ToolStripArrowRenderEventArgs.cs
- MimeTypeAttribute.cs
- OdbcTransaction.cs
- DynamicControl.cs
- ExpressionBuilder.cs
- ProtocolElement.cs
- AsmxEndpointPickerExtension.cs
- SAPIEngineTypes.cs
- Renderer.cs
- SqlBuilder.cs
- HttpCachePolicy.cs
- TrackingMemoryStreamFactory.cs
- SafeRightsManagementHandle.cs
- _CookieModule.cs
- HierarchicalDataSourceDesigner.cs
- CodeAttributeDeclarationCollection.cs
- AudioFormatConverter.cs
- SamlAction.cs
- ToolboxItemFilterAttribute.cs
- MarkupWriter.cs
- DecimalConstantAttribute.cs
- ImpersonationContext.cs
- ExceptionTrace.cs
- Model3D.cs
- LogReserveAndAppendState.cs
- UrlMapping.cs
- CapabilitiesRule.cs
- DataGridState.cs
- DockProviderWrapper.cs
- MarshalByRefObject.cs
- PointConverter.cs
- UriScheme.cs
- CFGGrammar.cs
- DataGridColumnHeader.cs
- SimpleBitVector32.cs
- NotSupportedException.cs
- IpcManager.cs
- ClockGroup.cs
- Sequence.cs
- UndirectedGraph.cs
- XPathNavigatorReader.cs
- PrintPreviewDialog.cs
- MessageProperties.cs
- SyndicationFeed.cs
- SpeechAudioFormatInfo.cs
- EdmProperty.cs
- TableProviderWrapper.cs
- SoapIncludeAttribute.cs
- MemoryFailPoint.cs
- EmbeddedMailObject.cs
- TypedTableBase.cs
- EncoderExceptionFallback.cs
- XmlArrayItemAttributes.cs
- ImportContext.cs
- UiaCoreTypesApi.cs
- HttpWebResponse.cs
- ToolStripItem.cs
- ComplexPropertyEntry.cs
- ObjectParameter.cs
- WebPartConnectionsDisconnectVerb.cs
- SizeAnimationUsingKeyFrames.cs
- SystemNetworkInterface.cs
- Int32RectConverter.cs
- ImageListImageEditor.cs
- HebrewCalendar.cs
- ExceptionRoutedEventArgs.cs
- SmtpFailedRecipientsException.cs
- MetadataPropertyCollection.cs
- Convert.cs
- CompilerGeneratedAttribute.cs
- DesignerTransactionCloseEvent.cs
- DataGridViewTopRowAccessibleObject.cs
- ConfigurationManagerInternal.cs
- QilStrConcat.cs
- Figure.cs
- WorkflowServiceHost.cs
- TripleDESCryptoServiceProvider.cs
- WorkflowViewStateService.cs
- WebPartCollection.cs
- CodeParameterDeclarationExpressionCollection.cs
- DesignerSerializationOptionsAttribute.cs
- MaskDesignerDialog.cs
- RenderTargetBitmap.cs
- XmlBoundElement.cs
- Parsers.cs
- PropertyInformation.cs
- DetailsViewUpdateEventArgs.cs
- CreateUserWizardStep.cs