Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / CredentialSelector.cs / 1 / CredentialSelector.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.IO; using System.Text; using System.Xml; using System.Xml.Serialization; using System.Xml.Schema; using System.Security.Cryptography.X509Certificates; using Microsoft.InfoCards.Diagnostics; using System.Collections.Generic; using System.Collections; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // Enumeration of known CredentialSelectors // internal enum CredentialSelectorType { InvalidSelector = -1, X509CertificateIssuerNameSelector = 0, X509CertificateIssuerSerialNoSelector = 1, X509CertificateKeyHashSelector = 2, X509CertificateKeyIdSelector = 3, UserNameSelector = 4, SpnSelector = 5, SelfIssuedCardIdSelector = 6, RsaKeyValueSelector = 7, UserPrincipalNameSelector = 8 } // // Summary: // CredentialSelector class. // internal class CredentialSelector :IXmlSerializable { CredentialSelectorType m_type; byte[ ] m_data; // // Summary: // CTOR // public CredentialSelector() { m_type = CredentialSelectorType.InvalidSelector; } // // Summary: // Gets/Sets the type of selector // public CredentialSelectorType Type { get { return m_type; } set { m_type = value; } } public bool IsComplete() { return ( CredentialSelectorType.InvalidSelector != m_type && m_data != null && m_data.Length > 0 ); } // // Summary: // returns the inner buffer as a unicode string. // public string GetStringWithoutNullTerminator() { if( null == m_data ) { return null; } string value = Encoding.Unicode.GetString( m_data ); return value.Substring( 0, value.Length - 1 ); } // // Summary: // returns a copy of the inner buffer. // public byte[ ] GetBytes() { if( null == m_data ) { return null; } byte[ ] buffer = new byte[ m_data.Length ]; Array.Copy( m_data, 0, buffer, 0, m_data.Length ); return buffer; } // // Summary: // Set the inner buffer to the Unicode bytes of the specified string // public void SetValue( string data ) { m_data = Encoding.Unicode.GetBytes( data + "\0" ); } // // Summary: // Sets the inner buffer to the sepcified data // public void SetValue( byte[ ] data, int index, int length ) { byte[ ] buffer = new byte[ length ]; Array.Copy( data, index, buffer, 0, length ); m_data = buffer; } // // Summary: // Serialized object to stream // public void Serialize( BinaryWriter writer ) { writer.Write( (Int32)m_type ); Utility.SerializeBytes( writer, m_data ); } // // Summary: // Populates members from stream. // public void Deserialize( BinaryReader reader ) { m_type = (CredentialSelectorType)reader.ReadInt32(); m_data = reader.ReadBytes( reader.ReadInt32() ); } public XmlSchema GetSchema() { return null; } // // Summary // Write the CredentialSelector to xml. // // Parameters // writer - The XmlWriter to write the data to // public void WriteXml( XmlWriter writer ) { switch( m_type ) { case CredentialSelectorType.UserNameSelector: WriteUserNameSelector( writer ); break; case CredentialSelectorType.X509CertificateIssuerNameSelector: WriteX509CertificateIssuerNameSelector( writer ); break; case CredentialSelectorType.X509CertificateIssuerSerialNoSelector: WriteX509CertificateIssuerSerialNoSelector( writer ); break; case CredentialSelectorType.X509CertificateKeyHashSelector: WriteX509CertificateKeyHashSelector( writer ); break; case CredentialSelectorType.SelfIssuedCardIdSelector: WriteSelfIssuedCardIdSelector( writer ); break; case CredentialSelectorType.UserPrincipalNameSelector: WriteUserPrincipalNameSelector( writer ); break; default: throw IDT.ThrowHelperError( new XmlException( SR.GetString( SR.UnexpectedElement ) ) ); } } // // Summary // Read the Credential from the xml. The reader should be on one of the following elements //// // // // // // Parameters // reader - The XmlReader to read data from // public void ReadXml( XmlReader reader ) { switch( reader.LocalName ) { case XmlNames.WSIdentity.UserNameElement: ReadUserNameSelector( reader ); break; case XmlNames.XmlDSig.X509IssuerNameElement: ReadX509IssuerNameSelector( reader ); break; case XmlNames.XmlDSig.X509SerialNumberElement: ReadX509IssuerSerialNumberSelector( reader ); break; case XmlNames.WSSecurityExt.KeyIdentifierElement: ReadX509KeyIdentifierSelector( reader ); break; case XmlNames.WSIdentity.PrivatePersonalIdentifierElement: ReadSelfIssuedSelector( reader ); break; case XmlNames.WSIdentity.UserPrincipalNameElement: ReadUserPrincipalNameSelector( reader ); break; default: throw IDT.ThrowHelperError( new XmlException( SR.GetString( SR.UnexpectedElement ) ) ); } } // // Summary: // Write the username selector information. // // Arguments: // writer: The XmlWriter to write the xml information to. // private void WriteUserNameSelector( XmlWriter writer ) { writer.WriteStartElement( XmlNames.WSIdentity.UserNameElement, XmlNames.WSIdentity.Namespace ); writer.WriteString( this.GetStringWithoutNullTerminator() ); writer.WriteEndElement(); } // // Summary: // Write the userprincipalname selector information. // // Arguments: // writer: The XmlWriter to write the xml information to. // private void WriteUserPrincipalNameSelector( XmlWriter writer ) { writer.WriteStartElement( XmlNames.WSIdentity.UserPrincipalNameElement, XmlNames.WSIdentity.Namespace ); writer.WriteString( this.GetStringWithoutNullTerminator() ); writer.WriteEndElement(); } // // Summary: // Write the X509IssuerName selector information. // // Arguments: // writer: The XmlWriter to write the xml information to. // private void WriteX509CertificateIssuerNameSelector( XmlWriter writer ) { writer.WriteStartElement( XmlNames.XmlDSig.X509IssuerNameElement, XmlNames.XmlDSig.Namespace ); string value = this.GetStringWithoutNullTerminator(); if( !String.IsNullOrEmpty( value ) ) { writer.WriteString( value ); } writer.WriteEndElement(); } // // Summary: // Write the X509IssuerSerialNumber selector information. // // Arguments: // writer: The XmlWriter to write the xml information to. // private void WriteX509CertificateIssuerSerialNoSelector( XmlWriter writer ) { writer.WriteStartElement( XmlNames.XmlDSig.X509SerialNumberElement, XmlNames.XmlDSig.Namespace ); BigInt bigint = new BigInt( this.GetBytes() ); writer.WriteString( bigint.ToDecimal() ); writer.WriteEndElement(); } // // Summary: // Write the X509CertificateKeyHash selector information. // // Arguments: // writer: The XmlWriter to write the xml information to. // private void WriteX509CertificateKeyHashSelector( XmlWriter writer ) { writer.WriteStartElement( XmlNames.WSSecurityExt.KeyIdentifierElement, XmlNames.WSSecurityExt.Namespace ); // // NOTE: We are still writing the incorrect SHA1 uri in order to maintain compatibility between // v1 and Orcas. However, in v2 we should change this value to the correct URI. // writer.WriteAttributeString( XmlNames.WSSecurityExt.ValueTypeAttribute, null, XmlNames.WSSecurityExt.AltSha1ThumbprintKeyTypeValue ); writer.WriteString( Convert.ToBase64String( this.GetBytes(), Base64FormattingOptions.None ) ); writer.WriteEndElement(); } // // Summary: // Write the SelfIssued selector information. // // Arguments: // writer: The XmlWriter to write the xml information to. // private void WriteSelfIssuedCardIdSelector( XmlWriter writer ) { writer.WriteStartElement( XmlNames.WSIdentity.PrivatePersonalIdentifierElement, XmlNames.WSIdentity.Namespace ); string value = this.GetStringWithoutNullTerminator(); if( !String.IsNullOrEmpty( value ) ) { writer.WriteString( value ); } writer.WriteEndElement(); } // // Summary: // Read the username selector information. // // Arguments: // reader: The XmlReader to read the xml information from. // private void ReadUserNameSelector( XmlReader reader ) { IDT.ThrowInvalidArgumentConditional( XmlNames.WSIdentity.UserNameElement != reader.LocalName || XmlNames.WSIdentity.Namespace != reader.NamespaceURI , reader.LocalName ); IDT.TraceDebug( "Reading the username information" ); // // Read the credential data // this.Type = CredentialSelectorType.UserNameSelector; String value = reader.ReadElementContentAsString().Trim(); if( !String.IsNullOrEmpty( value ) ) { this.SetValue( value ); } } // // Summary: // Read the userprinicpalname selector information. // // Arguments: // reader: The XmlReader to read the xml information from. // private void ReadUserPrincipalNameSelector( XmlReader reader ) { IDT.ThrowInvalidArgumentConditional( XmlNames.WSIdentity.UserPrincipalNameElement != reader.LocalName || XmlNames.WSIdentity.Namespace != reader.NamespaceURI , reader.LocalName ); IDT.TraceDebug( "Reading the Kerberos credential information" ); // // Read the credential data // this.Type = CredentialSelectorType.UserPrincipalNameSelector; } // // Summary: // Read the X509IssuerName selector information. // // Arguments: // reader: The XmlReader to read the xml information from. // private void ReadX509IssuerNameSelector( XmlReader reader ) { // // Read the X509IssuerName element // IDT.ThrowInvalidArgumentConditional( XmlNames.XmlDSig.X509IssuerNameElement != reader.LocalName || XmlNames.XmlDSig.Namespace != reader.NamespaceURI , reader.LocalName ); IDT.TraceDebug( "Reading the x509 issuer name information" ); this.Type = CredentialSelectorType.X509CertificateIssuerNameSelector; String value = reader.ReadElementContentAsString().Trim(); if( !String.IsNullOrEmpty( value ) ) { this.SetValue( value ); } } // // Summary: // Read the X509IssuerSerialNumber selector information. // // Arguments: // reader: The XmlReader to read the xml information from. // private void ReadX509IssuerSerialNumberSelector( XmlReader reader ) { // // Read the X509IssuerSerialNumber element // IDT.ThrowInvalidArgumentConditional( XmlNames.XmlDSig.X509SerialNumberElement != reader.LocalName || XmlNames.XmlDSig.Namespace != reader.NamespaceURI , reader.LocalName ); IDT.TraceDebug( "Reading the X509IssuerSerialNumber information" ); this.Type = CredentialSelectorType.X509CertificateIssuerSerialNoSelector; BigInt bigint = new BigInt(); String strval = reader.ReadElementContentAsString().Trim(); try { if( !String.IsNullOrEmpty( strval ) ) { bigint.FromDecimal( strval ); byte[ ] value = bigint.ToByteArray(); this.SetValue( value, 0, value.Length ); } } catch( FormatException ) { throw IDT.ThrowHelperError( new InvalidCardException( SR.GetString( SR.ServiceInvalidSerialNumber ) ) ); } } // // Summary: // Read the X509KeyIdentifier selector information. // // Arguments: // reader: The XmlReader to read the xml information from. // private void ReadX509KeyIdentifierSelector( XmlReader reader ) { // // Read the X509KeyIdentifier element // IDT.ThrowInvalidArgumentConditional( XmlNames.WSSecurityExt.KeyIdentifierElement != reader.LocalName || XmlNames.WSSecurityExt.Namespace != reader.NamespaceURI , reader.LocalName ); IDT.TraceDebug( "Reading the X509KeyIdentifier information" ); this.Type = CredentialSelectorType.X509CertificateKeyHashSelector; string hashType = reader.GetAttribute( XmlNames.WSSecurityExt.ValueTypeAttribute ); if( String.IsNullOrEmpty( hashType ) ) { hashType = reader.GetAttribute( XmlNames.WSSecurityExt.ValueTypeAttribute, XmlNames.WSSecurityExt.Namespace ); } // // For backward compability we will support both versions of the SHA1 uri // if( XmlNames.WSSecurityExt.Sha1ThumbprintKeyTypeValue != hashType && XmlNames.WSSecurityExt.AltSha1ThumbprintKeyTypeValue != hashType ) { throw IDT.ThrowHelperError( new InvalidCardException( SR.GetString( SR.ServiceUnsupportedKeyIdentifierType ) ) ); } try { MemoryStream ms = Utility.ReadByteStreamFromBase64( reader ); int length = Convert.ToInt32( ms.Length ); if( length > 0 ) { SetValue( ms.GetBuffer(), 0, length ); } } catch( FormatException ) { throw IDT.ThrowHelperError( new InvalidCardException( SR.GetString( SR.ServiceInvalidThumbPrintValue ) ) ); } } // // Summary: // Read the SelfIssued credential selector information. // // Arguments: // reader: The XmlReader to read the xml information from. // private void ReadSelfIssuedSelector( XmlReader reader ) { IDT.ThrowInvalidArgumentConditional( XmlNames.WSIdentity.PrivatePersonalIdentifierElement != reader.LocalName || XmlNames.WSIdentity.Namespace != reader.NamespaceURI , reader.LocalName ); IDT.TraceDebug( "Reading the PPID information" ); // // Read the credential data // this.Type = CredentialSelectorType.SelfIssuedCardIdSelector; string strval = reader.ReadElementContentAsString().Trim(); if( !String.IsNullOrEmpty( strval ) ) { this.SetValue( strval ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
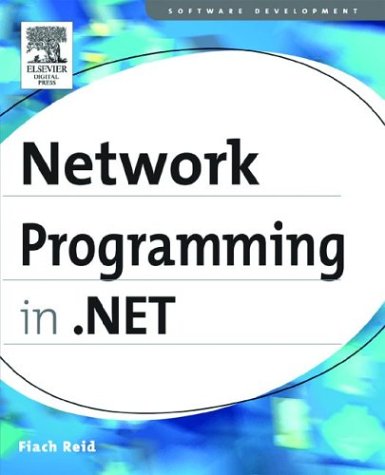
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SoapInteropTypes.cs
- ProcessStartInfo.cs
- BaseDataList.cs
- InfoCardKeyedHashAlgorithm.cs
- AutomationPropertyInfo.cs
- ListBindableAttribute.cs
- WebPartManager.cs
- ToolStripGripRenderEventArgs.cs
- TextElementEditingBehaviorAttribute.cs
- WinEventQueueItem.cs
- GridItem.cs
- DataTable.cs
- relpropertyhelper.cs
- WaitHandle.cs
- PropertyGridView.cs
- ConstructorBuilder.cs
- StylusDownEventArgs.cs
- ErrorTableItemStyle.cs
- InvokePattern.cs
- CodeParameterDeclarationExpression.cs
- TableLayoutCellPaintEventArgs.cs
- SqlServices.cs
- _NegoStream.cs
- EqualityComparer.cs
- HashStream.cs
- AssociationTypeEmitter.cs
- CommandField.cs
- Normalization.cs
- ViewCellRelation.cs
- ReferencedCollectionType.cs
- InfoCardAsymmetricCrypto.cs
- DebugView.cs
- _PooledStream.cs
- ProviderUtil.cs
- PropertyItemInternal.cs
- PeerApplication.cs
- ISAPIWorkerRequest.cs
- DocumentEventArgs.cs
- PackagingUtilities.cs
- TrustManagerPromptUI.cs
- RepeaterDesigner.cs
- StreamHelper.cs
- ResourcePermissionBase.cs
- BamlMapTable.cs
- DiscoveryViaBehavior.cs
- XmlSchemaInfo.cs
- HttpRawResponse.cs
- DataSpaceManager.cs
- DrawingCollection.cs
- VScrollProperties.cs
- SessionEndingEventArgs.cs
- BinaryExpressionHelper.cs
- WeakReferenceList.cs
- RuntimeConfigurationRecord.cs
- ObjectConverter.cs
- AppDomainGrammarProxy.cs
- DbDataAdapter.cs
- TypeCollectionDesigner.xaml.cs
- WebInvokeAttribute.cs
- BasicHttpMessageCredentialType.cs
- CapiNative.cs
- TrackBar.cs
- AnimationTimeline.cs
- DataSourceControlBuilder.cs
- StyleBamlTreeBuilder.cs
- DetailsViewDeleteEventArgs.cs
- WebBrowserDesigner.cs
- TypeInfo.cs
- grammarelement.cs
- IPGlobalProperties.cs
- MimeImporter.cs
- DecodeHelper.cs
- MultiBindingExpression.cs
- XmlSchemaGroup.cs
- CreateUserErrorEventArgs.cs
- ProjectionPruner.cs
- NamespaceDisplay.xaml.cs
- InstanceHandleReference.cs
- MessageHeaderInfoTraceRecord.cs
- EditingCoordinator.cs
- AdornerHitTestResult.cs
- CryptoApi.cs
- UndoEngine.cs
- TimelineClockCollection.cs
- XmlSchemaChoice.cs
- COM2EnumConverter.cs
- DataObject.cs
- ViewUtilities.cs
- StringInfo.cs
- ResXBuildProvider.cs
- TextInfo.cs
- ValidatorAttribute.cs
- CodeRemoveEventStatement.cs
- TraceData.cs
- DependencyPropertyKind.cs
- WmlImageAdapter.cs
- UrlAuthFailureHandler.cs
- CoreSwitches.cs
- GrammarBuilderWildcard.cs
- ElapsedEventArgs.cs