Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / XmlBuffer.cs / 1 / XmlBuffer.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- #define BINARY namespace System.ServiceModel { using System.IO; using System.Xml; using System.Text; using System.Collections.Generic; using System.Diagnostics; using System.ServiceModel.Channels; class XmlBuffer { Listsections; byte[] buffer; int offset; BufferedOutputStream stream; BufferState bufferState; XmlDictionaryWriter writer; XmlDictionaryReaderQuotas quotas; enum BufferState { Created, Writing, Reading, } struct Section { int offset; int size; XmlDictionaryReaderQuotas quotas; public Section(int offset, int size, XmlDictionaryReaderQuotas quotas) { this.offset = offset; this.size = size; this.quotas = quotas; } public int Offset { get { return this.offset; } } public int Size { get { return this.size; } } public XmlDictionaryReaderQuotas Quotas { get { return this.quotas; } } } public XmlBuffer(int maxBufferSize) { if (maxBufferSize < 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("maxBufferSize", maxBufferSize, SR.GetString(SR.ValueMustBeNonNegative))); int initialBufferSize = Math.Min(512, maxBufferSize); stream = new BufferedOutputStream(SR.XmlBufferQuotaExceeded, initialBufferSize, maxBufferSize, BufferManager.CreateBufferManager(0, int.MaxValue)); sections = new List (1); } public int BufferSize { get { DiagnosticUtility.DebugAssert(bufferState == BufferState.Reading, "Buffer size shuold only be retrieved during Reading state"); return buffer.Length; } } public int SectionCount { get { return this.sections.Count; } } public XmlDictionaryWriter OpenSection(XmlDictionaryReaderQuotas quotas) { if (bufferState != BufferState.Created) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(CreateInvalidStateException()); bufferState = BufferState.Writing; this.quotas = new XmlDictionaryReaderQuotas(); quotas.CopyTo(this.quotas); if (this.writer == null) { this.writer = XmlDictionaryWriter.CreateBinaryWriter(stream, XD.Dictionary, null, true); } else { ((IXmlBinaryWriterInitializer)this.writer).SetOutput(stream, XD.Dictionary, null, true); } return this.writer; } public void CloseSection() { if (bufferState != BufferState.Writing) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(CreateInvalidStateException()); this.writer.Close(); bufferState = BufferState.Created; int size = (int)stream.Length - offset; sections.Add(new Section(offset, size, this.quotas)); offset += size; } public void Close() { if (bufferState != BufferState.Created) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(CreateInvalidStateException()); bufferState = BufferState.Reading; int bufferSize; buffer = stream.ToArray(out bufferSize); writer = null; stream = null; } Exception CreateInvalidStateException() { return new InvalidOperationException(SR.GetString(SR.XmlBufferInInvalidState)); } public XmlDictionaryReader GetReader(int sectionIndex) { if (bufferState != BufferState.Reading) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(CreateInvalidStateException()); Section section = sections[sectionIndex]; XmlDictionaryReader reader = XmlDictionaryReader.CreateBinaryReader(buffer, section.Offset, section.Size, XD.Dictionary, section.Quotas, null, null); reader.MoveToContent(); return reader; } public void WriteTo(int sectionIndex, XmlWriter writer) { if (bufferState != BufferState.Reading) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(CreateInvalidStateException()); XmlDictionaryReader reader = GetReader(sectionIndex); try { writer.WriteNode(reader, false); } finally { reader.Close(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
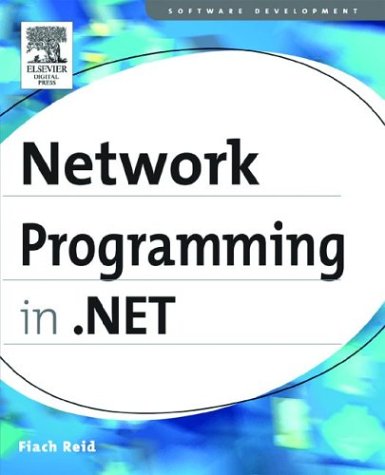
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PeerMessageDispatcher.cs
- HttpCachePolicyBase.cs
- ListViewGroup.cs
- ProgressBar.cs
- InvalidTimeZoneException.cs
- AnnotationAuthorChangedEventArgs.cs
- ResourceDescriptionAttribute.cs
- XPathSelectionIterator.cs
- DispatcherOperation.cs
- GenericWebPart.cs
- DetailsViewPageEventArgs.cs
- InstanceCreationEditor.cs
- XPathException.cs
- StoreContentChangedEventArgs.cs
- ColorContext.cs
- webbrowsersite.cs
- ViewStateException.cs
- XslException.cs
- RegistrySecurity.cs
- WmlCalendarAdapter.cs
- TemplateControlBuildProvider.cs
- ChannelServices.cs
- JavaScriptObjectDeserializer.cs
- _ConnectOverlappedAsyncResult.cs
- VScrollProperties.cs
- ChildDocumentBlock.cs
- SafeProcessHandle.cs
- VirtualPathProvider.cs
- Pair.cs
- UpdateManifestForBrowserApplication.cs
- EventDescriptor.cs
- MappingItemCollection.cs
- SoapAttributeAttribute.cs
- ProcessProtocolHandler.cs
- ContractAdapter.cs
- BamlTreeUpdater.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- NavigationPropertyEmitter.cs
- IProvider.cs
- MembershipValidatePasswordEventArgs.cs
- DecimalAnimationBase.cs
- TagElement.cs
- CancelEventArgs.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- DirtyTextRange.cs
- InkPresenterAutomationPeer.cs
- VarInfo.cs
- OrderedEnumerableRowCollection.cs
- CompilerError.cs
- HttpWriter.cs
- StringWriter.cs
- DefaultTypeArgumentAttribute.cs
- RectangleF.cs
- __Filters.cs
- TableStyle.cs
- ConfigXmlWhitespace.cs
- XmlSchema.cs
- DataGridViewCellMouseEventArgs.cs
- RegexGroupCollection.cs
- Roles.cs
- MessageQueueException.cs
- Context.cs
- ValueTypePropertyReference.cs
- SmtpMail.cs
- OutputCacheSettings.cs
- RenderTargetBitmap.cs
- CodeGotoStatement.cs
- RoleManagerSection.cs
- UnknownBitmapDecoder.cs
- PassportPrincipal.cs
- DependencyObjectType.cs
- CustomValidator.cs
- DecoderFallback.cs
- HostingEnvironmentSection.cs
- ScriptHandlerFactory.cs
- RenderContext.cs
- DaylightTime.cs
- DrawingContext.cs
- PageFunction.cs
- CompilerScopeManager.cs
- TransformerInfo.cs
- UrlMappingCollection.cs
- UpdateCommandGenerator.cs
- ResourceAttributes.cs
- RootCodeDomSerializer.cs
- XmlSigningNodeWriter.cs
- HttpClientCertificate.cs
- MembershipValidatePasswordEventArgs.cs
- ToolStripHighContrastRenderer.cs
- ObjectConverter.cs
- InvokeGenerator.cs
- AttributeTable.cs
- _NativeSSPI.cs
- ToolBarButtonDesigner.cs
- SystemUdpStatistics.cs
- SafeTimerHandle.cs
- DataGridDesigner.cs
- BuildProvider.cs
- BigInt.cs
- WindowPattern.cs