Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Security / WSTrustDec2005.cs / 1 / WSTrustDec2005.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Security { using System; using System.ServiceModel; using System.ServiceModel.Description; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Globalization; using System.IO; using System.Text; using System.Threading; using System.Xml; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.IdentityModel.Tokens; using System.Security.Cryptography.X509Certificates; using System.ServiceModel.Security.Tokens; using HexBinary = System.Runtime.Remoting.Metadata.W3cXsd2001.SoapHexBinary; using System.ServiceModel.Channels; using System.ServiceModel.Security; using System.Runtime.Serialization; using System.ServiceModel.Dispatcher; using KeyIdentifierEntry = WSSecurityTokenSerializer.KeyIdentifierEntry; using KeyIdentifierClauseEntry = WSSecurityTokenSerializer.KeyIdentifierClauseEntry; using TokenEntry = WSSecurityTokenSerializer.TokenEntry; using StrEntry = WSSecurityTokenSerializer.StrEntry; class WSTrustDec2005 : WSTrustFeb2005 { public WSTrustDec2005(WSSecurityTokenSerializer tokenSerializer) : base(tokenSerializer) { } public override TrustDictionary SerializerDictionary { get { return DXD.TrustDec2005Dictionary; } } public class DriverDec2005 : WSTrustFeb2005.DriverFeb2005 { public DriverDec2005(SecurityStandardsManager standardsManager) : base(standardsManager) { } public override TrustDictionary DriverDictionary { get { return DXD.TrustDec2005Dictionary; } } public override XmlDictionaryString RequestSecurityTokenResponseFinalAction { get { return DXD.TrustDec2005Dictionary.RequestSecurityTokenCollectionIssuanceFinalResponse; } } public override XmlElement CreateKeyTypeElement(SecurityKeyType keyType) { if (keyType == SecurityKeyType.BearerKey) { XmlDocument doc = new XmlDocument(); XmlElement result = doc.CreateElement(this.DriverDictionary.Prefix.Value, this.DriverDictionary.KeyType.Value, this.DriverDictionary.Namespace.Value); result.AppendChild(doc.CreateTextNode(DXD.TrustDec2005Dictionary.BearerKeyType.Value)); return result; } return base.CreateKeyTypeElement(keyType); } public override bool TryParseKeyTypeElement(XmlElement element, out SecurityKeyType keyType) { if (element == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("element"); if (element.LocalName == this.DriverDictionary.KeyType.Value && element.NamespaceURI == this.DriverDictionary.Namespace.Value && element.InnerText == DXD.TrustDec2005Dictionary.BearerKeyType.Value) { keyType = SecurityKeyType.BearerKey; return true; } return base.TryParseKeyTypeElement(element, out keyType); } public override XmlElement CreateRequiredClaimsElement(IEnumerableclaimsList) { XmlElement result = base.CreateRequiredClaimsElement(claimsList); XmlAttribute dialectAttribute = result.OwnerDocument.CreateAttribute(DXD.TrustDec2005Dictionary.Dialect.Value); dialectAttribute.Value = DXD.TrustDec2005Dictionary.DialectType.Value; result.Attributes.Append(dialectAttribute); return result; } public override IChannelFactory CreateFederationProxy(EndpointAddress address, Binding binding, KeyedByTypeCollection channelBehaviors) { if (channelBehaviors == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("channelBehaviors"); ChannelFactory result = new ChannelFactory (binding, address); SetProtectionLevelForFederation(result.Endpoint.Contract.Operations); // remove the default client credentials that gets added to channel factories result.Endpoint.Behaviors.Remove (); for (int i = 0; i < channelBehaviors.Count; ++i) { result.Endpoint.Behaviors.Add(channelBehaviors[i]); } // add a behavior that removes the UI channel initializer added by the client credentials since there should be no UI // initializer popped up as part of obtaining the federation token (the UI should already have been popped up for the main channel) result.Endpoint.Behaviors.Add(new WSTrustFeb2005.DriverFeb2005.InteractiveInitializersRemovingBehavior()); return new WSTrustFeb2005.DriverFeb2005.RequestChannelFactory (result); } public override Collection ProcessUnknownRequestParameters(Collection unknownRequestParameters, Collection originalRequestParameters) { // For WS-Trust 1.3 we want everything in the requestSecurityTokenTemplate parameters to endup as Addtional parameters. // The parameters will appear as a child element under a XmlElement named secondaryParameters. if (originalRequestParameters == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("originalRequestParameters"); if (originalRequestParameters.Count > 0 && originalRequestParameters[0] != null && originalRequestParameters[0].OwnerDocument != null) { XmlElement secondaryParamElement = originalRequestParameters[0].OwnerDocument.CreateElement(DXD.TrustDec2005Dictionary.Prefix.Value, DXD.TrustDec2005Dictionary.SecondaryParameters.Value, DXD.TrustDec2005Dictionary.Namespace.Value); for (int i = 0; i < originalRequestParameters.Count; ++i) { secondaryParamElement.AppendChild(originalRequestParameters[i]); } Collection tempCollection = new Collection (); tempCollection.Add(secondaryParamElement); return tempCollection; } return originalRequestParameters; } internal virtual bool IsSecondaryParametersElement(XmlElement element) { return ((element.LocalName == DXD.TrustDec2005Dictionary.SecondaryParameters.Value) && (element.NamespaceURI == DXD.TrustDec2005Dictionary.Namespace.Value)); } public virtual XmlElement CreateKeyWrapAlgorithmElement(string keyWrapAlgorithm) { if (keyWrapAlgorithm == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyWrapAlgorithm"); } XmlDocument doc = new XmlDocument(); XmlElement result = doc.CreateElement(DXD.TrustDec2005Dictionary.Prefix.Value, DXD.TrustDec2005Dictionary.KeyWrapAlgorithm.Value, DXD.TrustDec2005Dictionary.Namespace.Value); result.AppendChild(doc.CreateTextNode(keyWrapAlgorithm)); return result; } internal override bool IsKeyWrapAlgorithmElement(XmlElement element, out string keyWrapAlgorithm) { return CheckElement(element, DXD.TrustDec2005Dictionary.KeyWrapAlgorithm.Value, DXD.TrustDec2005Dictionary.Namespace.Value, out keyWrapAlgorithm); } [ServiceContract] internal interface IWsTrustDec2005SecurityTokenService { [OperationContract(IsOneWay = false, Action = TrustDec2005Strings.RequestSecurityTokenIssuance, ReplyAction = TrustDec2005Strings.RequestSecurityTokenCollectionIssuanceFinalResponse)] [FaultContract(typeof(string), Action = "*", ProtectionLevel = System.Net.Security.ProtectionLevel.Sign)] Message RequestToken(Message message); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
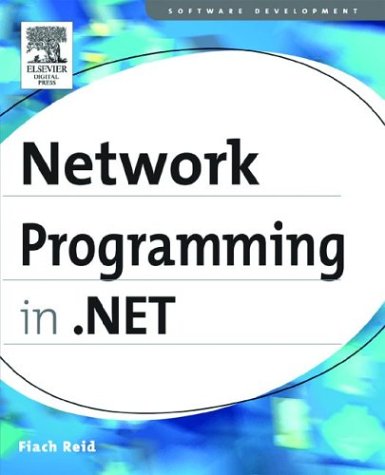
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewSelectedRowCollection.cs
- ImplicitInputBrush.cs
- TypeContext.cs
- GridViewColumnHeader.cs
- HierarchicalDataSourceControl.cs
- GeneralTransform3DCollection.cs
- ValidateNames.cs
- WebPartDisplayModeEventArgs.cs
- _NegotiateClient.cs
- DriveNotFoundException.cs
- ConfigurationPropertyAttribute.cs
- OdbcCommand.cs
- XmlSchemaCollection.cs
- TextDecorationCollection.cs
- XpsManager.cs
- LayoutUtils.cs
- SQLByte.cs
- SoapAttributeOverrides.cs
- UndoManager.cs
- RelationalExpressions.cs
- SelectionUIService.cs
- TextServicesCompartmentEventSink.cs
- CompatibleIComparer.cs
- SupportsEventValidationAttribute.cs
- StringOutput.cs
- EdmMember.cs
- OleDbParameterCollection.cs
- InvalidPropValue.cs
- AssemblyUtil.cs
- DirectoryLocalQuery.cs
- PixelShader.cs
- UserValidatedEventArgs.cs
- LockCookie.cs
- UpdateCommand.cs
- RectangleHotSpot.cs
- ExcludePathInfo.cs
- IgnorePropertiesAttribute.cs
- JournalEntryStack.cs
- SqlDependency.cs
- ReversePositionQuery.cs
- CultureSpecificStringDictionary.cs
- EntityDesignPluralizationHandler.cs
- EntityTypeBase.cs
- HashHelper.cs
- ImageBrush.cs
- InkSerializer.cs
- SendContent.cs
- BooleanProjectedSlot.cs
- LookupNode.cs
- ExtendLockAsyncResult.cs
- BitConverter.cs
- TextParagraphCache.cs
- CultureInfoConverter.cs
- ObjectCache.cs
- SqlRetyper.cs
- Identifier.cs
- CodeAttributeArgumentCollection.cs
- XmlDataContract.cs
- ConsoleEntryPoint.cs
- DataGridViewTextBoxColumn.cs
- DataKeyArray.cs
- GiveFeedbackEventArgs.cs
- XPathArrayIterator.cs
- PropertyGeneratedEventArgs.cs
- AutoResetEvent.cs
- AssemblyNameProxy.cs
- File.cs
- IPEndPointCollection.cs
- ListViewTableCell.cs
- Rectangle.cs
- _KerberosClient.cs
- Rotation3DAnimationUsingKeyFrames.cs
- QueryStatement.cs
- OperandQuery.cs
- Tuple.cs
- TargetException.cs
- ConfigXmlSignificantWhitespace.cs
- DependencyPropertyHelper.cs
- UICuesEvent.cs
- XmlEventCache.cs
- SqlGenerator.cs
- Content.cs
- ControlEvent.cs
- SafeSecurityHelper.cs
- ListViewSortEventArgs.cs
- TextDecorationCollection.cs
- StringInfo.cs
- WmpBitmapDecoder.cs
- CodeSnippetExpression.cs
- DocumentPaginator.cs
- DiscreteKeyFrames.cs
- DataGridToolTip.cs
- RankException.cs
- ADConnectionHelper.cs
- SQlBooleanStorage.cs
- ValidationSummary.cs
- DynamicVirtualDiscoSearcher.cs
- TrackingRecordPreFilter.cs
- SqlParameterizer.cs
- SpecularMaterial.cs