Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / OutputChannelBinder.cs / 1 / OutputChannelBinder.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.ServiceModel.Channels; using System.ServiceModel; using System.ServiceModel.Diagnostics; class OutputChannelBinder : IChannelBinder { IOutputChannel channel; internal OutputChannelBinder(IOutputChannel channel) { if (!((channel != null))) { DiagnosticUtility.DebugAssert("OutputChannelBinder.OutputChannelBinder: (channel != null)"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("channel"); } this.channel = channel; } public IChannel Channel { get { return this.channel; } } public bool HasSession { get { return this.channel is ISessionChannel; } } public Uri ListenUri { get { return null; } } public EndpointAddress LocalAddress { get { #pragma warning suppress 56503 // [....], the property is really not implemented, cannot lie, API not public throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } } public EndpointAddress RemoteAddress { get { return this.channel.RemoteAddress; } } public void Abort() { this.channel.Abort(); } public void CloseAfterFault(TimeSpan timeout) { this.channel.Close(timeout); } public IAsyncResult BeginTryReceive(TimeSpan timeout, AsyncCallback callback, object state) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public bool EndTryReceive(IAsyncResult result, out RequestContext requestContext) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public IAsyncResult BeginSend(Message message, TimeSpan timeout, AsyncCallback callback, object state) { return this.channel.BeginSend(message, timeout, callback, state); } public void EndSend(IAsyncResult result) { this.channel.EndSend(result); } public void Send(Message message, TimeSpan timeout) { this.channel.Send(message, timeout); } public IAsyncResult BeginRequest(Message message, TimeSpan timeout, AsyncCallback callback, object state) { throw TraceUtility.ThrowHelperError(new NotImplementedException(), message); } public Message EndRequest(IAsyncResult result) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public bool TryReceive(TimeSpan timeout, out RequestContext requestContext) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public Message Request(Message message, TimeSpan timeout) { throw TraceUtility.ThrowHelperError(new NotImplementedException(), message); } public bool WaitForMessage(TimeSpan timeout) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public IAsyncResult BeginWaitForMessage(TimeSpan timeout, AsyncCallback callback, object state) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public bool EndWaitForMessage(IAsyncResult result) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
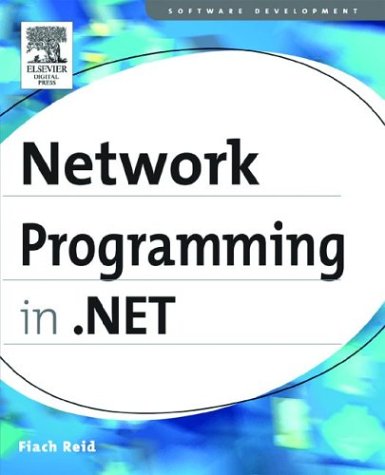
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClientScriptItemCollection.cs
- HttpRequest.cs
- MenuCommandService.cs
- RenderingEventArgs.cs
- ThousandthOfEmRealPoints.cs
- ToolStripDropDownItem.cs
- StylusPlugin.cs
- XmlWriterDelegator.cs
- DBParameter.cs
- NamespaceCollection.cs
- SqlErrorCollection.cs
- ConfigurationValue.cs
- DesignerAdapterUtil.cs
- ImageDrawing.cs
- OutOfProcStateClientManager.cs
- ConnectivityStatus.cs
- ConfigurationHelpers.cs
- ModelPropertyDescriptor.cs
- MetadataUtilsSmi.cs
- UserControl.cs
- ConstrainedDataObject.cs
- InkCanvasAutomationPeer.cs
- Attributes.cs
- DebuggerAttributes.cs
- RelationalExpressions.cs
- BoundColumn.cs
- ResourceSetExpression.cs
- StyleSelector.cs
- UrlPath.cs
- HttpRequest.cs
- DefaultCommandExtensionCallback.cs
- AttributeCollection.cs
- LinkClickEvent.cs
- CorrelationManager.cs
- NavigationWindow.cs
- RelationshipNavigation.cs
- XmlNullResolver.cs
- ArraySubsetEnumerator.cs
- TextServicesCompartment.cs
- TableLayoutSettings.cs
- NextPreviousPagerField.cs
- OdbcConnectionFactory.cs
- CollectionViewGroup.cs
- DesignerOptionService.cs
- DbConnectionClosed.cs
- ComplusEndpointConfigContainer.cs
- TextLine.cs
- ProcessInfo.cs
- StyleCollection.cs
- InvokePatternIdentifiers.cs
- TypedAsyncResult.cs
- ContainerSelectorBehavior.cs
- Item.cs
- _FixedSizeReader.cs
- RealizationContext.cs
- TriggerActionCollection.cs
- AppDomainManager.cs
- EnvironmentPermission.cs
- SystemColors.cs
- EllipseGeometry.cs
- AppDomainEvidenceFactory.cs
- XpsImage.cs
- InheritablePropertyChangeInfo.cs
- ConfigurationPropertyCollection.cs
- MimeMultiPart.cs
- MDIWindowDialog.cs
- MemberProjectionIndex.cs
- OSEnvironmentHelper.cs
- EncoderParameters.cs
- DefaultParameterValueAttribute.cs
- CodePrimitiveExpression.cs
- SQLMembershipProvider.cs
- DataSourceView.cs
- SqlMethodAttribute.cs
- CompilerLocalReference.cs
- ObjectHelper.cs
- TableCellCollection.cs
- EnumBuilder.cs
- PageAdapter.cs
- PropertyToken.cs
- EdmFunction.cs
- XPathNodeHelper.cs
- ButtonField.cs
- TextRunCacheImp.cs
- SoapRpcServiceAttribute.cs
- CssTextWriter.cs
- FlowLayoutPanel.cs
- ContractValidationHelper.cs
- SQLBoolean.cs
- DataGridViewRowEventArgs.cs
- OdbcHandle.cs
- SqlParameterCollection.cs
- IdentitySection.cs
- ResourceAttributes.cs
- WindowsUpDown.cs
- COSERVERINFO.cs
- DelimitedListTraceListener.cs
- PointLightBase.cs
- AuthenticationModulesSection.cs
- ClientApiGenerator.cs