Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / FaultContractInfo.cs / 1 / FaultContractInfo.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.ServiceModel; using System.Collections.Generic; using System.ServiceModel.Description; using System.Runtime.Serialization; public class FaultContractInfo { string action; Type detail; string elementName; string ns; IListknownTypes; DataContractSerializer serializer; public FaultContractInfo(string action, Type detail):this(action, detail, null, null, null) { } internal FaultContractInfo(string action, Type detail, XmlName elementName, string ns, IList knownTypes) { if (action == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("action"); } if (detail == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("detail"); } this.action = action; this.detail = detail; if (elementName != null) this.elementName = elementName.EncodedName; this.ns = ns; this.knownTypes = knownTypes; } public string Action { get { return this.action; } } public Type Detail { get { return this.detail; } } internal string ElementName { get { return this.elementName; } } internal string ElementNamespace { get { return this.ns; } } internal IList KnownTypes { get { return this.knownTypes; } } internal DataContractSerializer Serializer { get { if (this.serializer == null) { if (this.elementName == null) { this.serializer = DataContractSerializerDefaults.CreateSerializer(this.detail, this.knownTypes, int.MaxValue /* maxItemsInObjectGraph */); } else { this.serializer = DataContractSerializerDefaults.CreateSerializer(this.detail, this.knownTypes, this.elementName, this.ns == null ? string.Empty : this.ns, int.MaxValue /* maxItemsInObjectGraph */); } } return this.serializer; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
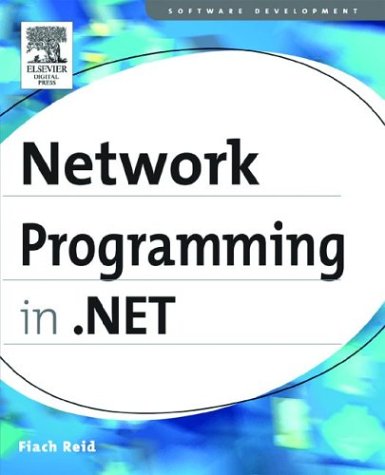
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- KernelTypeValidation.cs
- PointLightBase.cs
- IndicFontClient.cs
- PropertyGeneratedEventArgs.cs
- SourceElementsCollection.cs
- InternalEnumValidator.cs
- EventData.cs
- DbConnectionPoolCounters.cs
- Parser.cs
- JsonDataContract.cs
- QuaternionRotation3D.cs
- TypographyProperties.cs
- StylusTip.cs
- MimeFormatExtensions.cs
- BooleanSwitch.cs
- ModelItemCollectionImpl.cs
- WindowsAuthenticationEventArgs.cs
- ToolStripPanelSelectionBehavior.cs
- BorderGapMaskConverter.cs
- InteropAutomationProvider.cs
- EntityContainer.cs
- InputLanguage.cs
- SecurityKeyUsage.cs
- FontFaceLayoutInfo.cs
- WebPartConnectVerb.cs
- Vector3DCollection.cs
- InlineCollection.cs
- LambdaCompiler.cs
- URLString.cs
- ConfigurationException.cs
- Odbc32.cs
- DictionaryManager.cs
- FrameDimension.cs
- PeerDefaultCustomResolverClient.cs
- PhysicalAddress.cs
- Assert.cs
- ellipse.cs
- ZipIOExtraFieldZip64Element.cs
- HashAlgorithm.cs
- AccessText.cs
- DataControlLinkButton.cs
- WindowInteropHelper.cs
- Substitution.cs
- connectionpool.cs
- LayoutTableCell.cs
- IndexingContentUnit.cs
- XmlNullResolver.cs
- PageAdapter.cs
- SizeF.cs
- PolicyLevel.cs
- SqlBulkCopy.cs
- XPathBinder.cs
- ColumnCollection.cs
- DropShadowBitmapEffect.cs
- MenuEventArgs.cs
- DesignerSerializerAttribute.cs
- TreeView.cs
- Rss20ItemFormatter.cs
- Point3DCollection.cs
- ServiceObjectContainer.cs
- ParseElement.cs
- LexicalChunk.cs
- TableItemProviderWrapper.cs
- FormViewPagerRow.cs
- DbConnectionPoolGroupProviderInfo.cs
- SqlCharStream.cs
- ObservableDictionary.cs
- MethodExpression.cs
- Asn1Utilities.cs
- RequestQueryParser.cs
- WebConfigManager.cs
- ValidationResult.cs
- CharAnimationUsingKeyFrames.cs
- SqlUnionizer.cs
- DecimalConverter.cs
- ContentOperations.cs
- OleDbConnectionInternal.cs
- DataListItemEventArgs.cs
- StylusDevice.cs
- _HeaderInfoTable.cs
- ScrollChrome.cs
- Automation.cs
- PrimitiveXmlSerializers.cs
- TableCell.cs
- SafeRegistryHandle.cs
- ApplicationContext.cs
- EllipseGeometry.cs
- XNameTypeConverter.cs
- WorkflowRequestContext.cs
- XNameTypeConverter.cs
- Opcode.cs
- TemplateBamlRecordReader.cs
- VirtualPathProvider.cs
- FileLevelControlBuilderAttribute.cs
- Frame.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- AstTree.cs
- MemoryStream.cs
- DocumentStream.cs
- TreeViewTemplateSelector.cs