Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / ClientRuntime.cs / 1 / ClientRuntime.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.Collections.ObjectModel; using System.Collections.Generic; using System.Diagnostics; using System.ServiceModel.Channels; using System.ServiceModel.Security; public sealed class ClientRuntime { bool addTransactionFlowProperties = true; Type callbackProxyType; ProxyBehaviorCollectionchannelInitializers; string contractName; string contractNamespace; Type contractProxyType; DispatchRuntime dispatchRuntime; IdentityVerifier identityVerifier; ProxyBehaviorCollection interactiveChannelInitializers; ProxyBehaviorCollection messageInspectors; OperationCollection operations; IClientOperationSelector operationSelector; ImmutableClientRuntime runtime; ClientOperation unhandled; bool useSynchronizationContext = true; Uri via; SharedRuntimeState shared; int maxFaultSize; internal ClientRuntime(DispatchRuntime dispatchRuntime, SharedRuntimeState shared) : this(dispatchRuntime.EndpointDispatcher.ContractName, dispatchRuntime.EndpointDispatcher.ContractNamespace, shared) { if (dispatchRuntime == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("dispatchRuntime"); this.dispatchRuntime = dispatchRuntime; this.shared = shared; DiagnosticUtility.DebugAssert(shared.IsOnServer, "Server constructor called on client?"); } internal ClientRuntime(string contractName, string contractNamespace) : this(contractName, contractNamespace, new SharedRuntimeState(false)) { DiagnosticUtility.DebugAssert(!shared.IsOnServer, "Client constructor called on server?"); } ClientRuntime(string contractName, string contractNamespace, SharedRuntimeState shared) { this.contractName = contractName; this.contractNamespace = contractNamespace; this.shared = shared; this.operations = new OperationCollection(this); this.channelInitializers = new ProxyBehaviorCollection (this); this.messageInspectors = new ProxyBehaviorCollection (this); this.interactiveChannelInitializers = new ProxyBehaviorCollection (this); this.unhandled = new ClientOperation(this, "*", MessageHeaders.WildcardAction, MessageHeaders.WildcardAction); this.unhandled.InternalFormatter = new MessageOperationFormatter(); this.maxFaultSize = TransportDefaults.MaxFaultSize; } internal bool AddTransactionFlowProperties { get { return this.addTransactionFlowProperties; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.addTransactionFlowProperties = value; } } } public Type CallbackClientType { get { return this.callbackProxyType; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.callbackProxyType = value; } } } public SynchronizedCollection ChannelInitializers { get { return this.channelInitializers; } } public string ContractName { get { return this.contractName; } } public string ContractNamespace { get { return this.contractNamespace; } } public Type ContractClientType { get { return this.contractProxyType; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.contractProxyType = value; } } } internal IdentityVerifier IdentityVerifier { get { if (this.identityVerifier == null) { this.identityVerifier = IdentityVerifier.CreateDefault(); } return this.identityVerifier; } set { if (value == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); } this.InvalidateRuntime(); this.identityVerifier = value; } } public Uri Via { get { return this.via;} set { lock (this.ThisLock) { this.InvalidateRuntime(); this.via = value; } } } public bool ValidateMustUnderstand { get { return this.shared.ValidateMustUnderstand; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.shared.ValidateMustUnderstand = value; } } } internal DispatchRuntime DispatchRuntime { get { return this.dispatchRuntime; } } public DispatchRuntime CallbackDispatchRuntime { get { if (this.dispatchRuntime == null) this.dispatchRuntime = new DispatchRuntime(this, this.shared); return this.dispatchRuntime; } } internal bool EnableFaults { get { if (this.IsOnServer) { return this.dispatchRuntime.EnableFaults; } else { return this.shared.EnableFaults; } } set { lock (this.ThisLock) { if (this.IsOnServer) { string text = SR.GetString(SR.SFxSetEnableFaultsOnChannelDispatcher0); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(text)); } else { this.InvalidateRuntime(); this.shared.EnableFaults = value; } } } } public SynchronizedCollection InteractiveChannelInitializers { get { return this.interactiveChannelInitializers; } } public int MaxFaultSize { get { return this.maxFaultSize; } set { this.InvalidateRuntime(); this.maxFaultSize = value; } } internal bool IsOnServer { get { return this.shared.IsOnServer; } } public bool ManualAddressing { get { if (this.IsOnServer) { return this.dispatchRuntime.ManualAddressing; } else { return this.shared.ManualAddressing; } } set { lock (this.ThisLock) { if (this.IsOnServer) { string text = SR.GetString(SR.SFxSetManualAddresssingOnChannelDispatcher0); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(text)); } else { this.InvalidateRuntime(); this.shared.ManualAddressing = value; } } } } internal int MaxParameterInspectors { get { lock (this.ThisLock) { int max = 0; for (int i=0; i MessageInspectors { get { return this.messageInspectors; } } public SynchronizedKeyedCollection Operations { get { return this.operations; } } public IClientOperationSelector OperationSelector { get { return this.operationSelector; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.operationSelector = value; } } } internal object ThisLock { get { return this.shared; } } public ClientOperation UnhandledClientOperation { get { return this.unhandled; } } internal bool UseSynchronizationContext { get { return this.useSynchronizationContext; } set { lock (this.ThisLock) { this.InvalidateRuntime(); this.useSynchronizationContext = value; } } } internal T[] GetArray (SynchronizedCollection collection) { lock (collection.SyncRoot) { if (collection.Count == 0) { return EmptyArray .Instance; } else { T[] array = new T[collection.Count]; collection.CopyTo(array, 0); return array; } } } internal ImmutableClientRuntime GetRuntime() { lock (this.ThisLock) { if (this.runtime == null) this.runtime = new ImmutableClientRuntime(this); return this.runtime; } } internal void InvalidateRuntime() { lock (this.ThisLock) { this.shared.ThrowIfImmutable(); this.runtime = null; } } internal void LockDownProperties() { this.shared.LockDownProperties(); } internal SynchronizedCollection NewBehaviorCollection () { return new ProxyBehaviorCollection (this); } class ProxyBehaviorCollection : SynchronizedCollection { ClientRuntime outer; internal ProxyBehaviorCollection(ClientRuntime outer) : base(outer.ThisLock) { this.outer = outer; } protected override void ClearItems() { this.outer.InvalidateRuntime(); base.ClearItems(); } protected override void InsertItem(int index, T item) { if (item == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); } this.outer.InvalidateRuntime(); base.InsertItem(index, item); } protected override void RemoveItem(int index) { this.outer.InvalidateRuntime(); base.RemoveItem(index); } protected override void SetItem(int index, T item) { if (item == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); } this.outer.InvalidateRuntime(); base.SetItem(index, item); } } class OperationCollection : SynchronizedKeyedCollection { ClientRuntime outer; internal OperationCollection(ClientRuntime outer) : base(outer.ThisLock) { this.outer = outer; } protected override void ClearItems() { this.outer.InvalidateRuntime(); base.ClearItems(); } protected override string GetKeyForItem(ClientOperation item) { return item.Name; } protected override void InsertItem(int index, ClientOperation item) { if (item == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); if (item.Parent != this.outer) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.SFxMismatchedOperationParent)); this.outer.InvalidateRuntime(); base.InsertItem(index, item); } protected override void RemoveItem(int index) { this.outer.InvalidateRuntime(); base.RemoveItem(index); } protected override void SetItem(int index, ClientOperation item) { if (item == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); if (item.Parent != this.outer) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.SFxMismatchedOperationParent)); this.outer.InvalidateRuntime(); base.SetItem(index, item); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
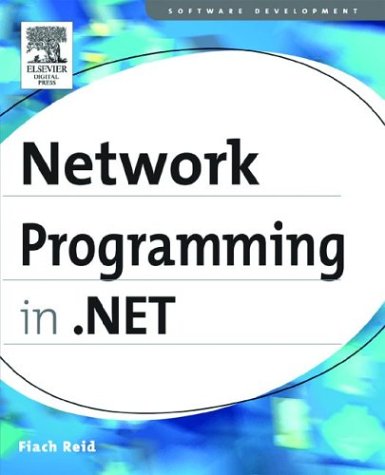
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlMobileTextWriter.cs
- GenericIdentity.cs
- GenericAuthenticationEventArgs.cs
- Stackframe.cs
- MDIControlStrip.cs
- DataSourceSelectArguments.cs
- BaseValidator.cs
- Calendar.cs
- Matrix3DConverter.cs
- InstanceHandleReference.cs
- OpenFileDialog.cs
- prompt.cs
- CellTreeNodeVisitors.cs
- XmlMemberMapping.cs
- Propagator.ExtentPlaceholderCreator.cs
- EncodingStreamWrapper.cs
- DataTableExtensions.cs
- BuilderElements.cs
- ContextMenuStrip.cs
- COM2AboutBoxPropertyDescriptor.cs
- DataGridBoolColumn.cs
- WindowsSpinner.cs
- SequentialWorkflowHeaderFooter.cs
- WhitespaceRuleLookup.cs
- CompiledRegexRunner.cs
- PasswordBoxAutomationPeer.cs
- XmlSchemaAppInfo.cs
- HttpListenerRequest.cs
- BooleanProjectedSlot.cs
- RefreshEventArgs.cs
- TransformationRules.cs
- odbcmetadatafactory.cs
- ZoneButton.cs
- CodePageUtils.cs
- AVElementHelper.cs
- ConversionValidationRule.cs
- XmlSchemaImporter.cs
- ToolStripSettings.cs
- XDRSchema.cs
- DataGridItemCollection.cs
- PathData.cs
- Certificate.cs
- XmlReflectionImporter.cs
- CancelRequestedQuery.cs
- DoWorkEventArgs.cs
- EDesignUtil.cs
- ConnectionPoolManager.cs
- NonBatchDirectoryCompiler.cs
- CatalogZone.cs
- DataColumnMappingCollection.cs
- EntityDataSourceReferenceGroup.cs
- DecoderBestFitFallback.cs
- DbProviderServices.cs
- FigureParagraph.cs
- TreeViewTemplateSelector.cs
- StreamWithDictionary.cs
- MessageFault.cs
- AssociationType.cs
- CodeAssignStatement.cs
- TraceFilter.cs
- PropertyPath.cs
- SafeEventHandle.cs
- UniqueConstraint.cs
- NativeActivity.cs
- LookupNode.cs
- ManipulationBoundaryFeedbackEventArgs.cs
- EntityContainerRelationshipSetEnd.cs
- ObjectComplexPropertyMapping.cs
- Grant.cs
- IntAverageAggregationOperator.cs
- translator.cs
- _RequestLifetimeSetter.cs
- SocketInformation.cs
- SchemaNamespaceManager.cs
- BitConverter.cs
- DropShadowEffect.cs
- BaseUriHelper.cs
- ServicePerformanceCounters.cs
- CorrelationScope.cs
- HashJoinQueryOperatorEnumerator.cs
- RecognitionResult.cs
- PlaceHolder.cs
- RegexCode.cs
- RegexNode.cs
- ItemContainerGenerator.cs
- AssemblyAttributesGoHere.cs
- RuntimeConfigLKG.cs
- Timer.cs
- PhysicalAddress.cs
- FontStyle.cs
- Fonts.cs
- TTSEngineProxy.cs
- MarkupExtensionParser.cs
- MergablePropertyAttribute.cs
- EventDescriptor.cs
- SessionEndingCancelEventArgs.cs
- StickyNoteHelper.cs
- ReflectPropertyDescriptor.cs
- HGlobalSafeHandle.cs
- TabControlToolboxItem.cs