Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / ChannelDispatcherCollection.cs / 1 / ChannelDispatcherCollection.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Runtime.Serialization; public class ChannelDispatcherCollection : SynchronizedCollection{ ServiceHostBase service; internal ChannelDispatcherCollection(ServiceHostBase service, object syncRoot) : base(syncRoot) { if (service == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("service"); this.service = service; } protected override void ClearItems() { ChannelDispatcherBase[] array = new ChannelDispatcherBase[this.Count]; this.CopyTo(array, 0); base.ClearItems(); if (this.service != null) { foreach (ChannelDispatcherBase channelDispatcher in array) this.service.OnRemoveChannelDispatcher(channelDispatcher); } } protected override void InsertItem(int index, ChannelDispatcherBase item) { if (this.service != null) { if (this.service.State == CommunicationState.Closed) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ObjectDisposedException(this.service.GetType().ToString())); this.service.OnAddChannelDispatcher(item); } base.InsertItem(index, item); } protected override void RemoveItem(int index) { ChannelDispatcherBase channelDispatcher = this.Items[index]; base.RemoveItem(index); if (this.service != null) this.service.OnRemoveChannelDispatcher(channelDispatcher); } protected override void SetItem(int index, ChannelDispatcherBase item) { if (this.service != null) { if (this.service.State == CommunicationState.Closed) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ObjectDisposedException(this.service.GetType().ToString())); } if (this.service != null) this.service.OnAddChannelDispatcher(item); ChannelDispatcherBase old; lock (this.SyncRoot) { old = this.Items[index]; base.SetItem(index, item); } if (this.service != null) this.service.OnRemoveChannelDispatcher(old); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
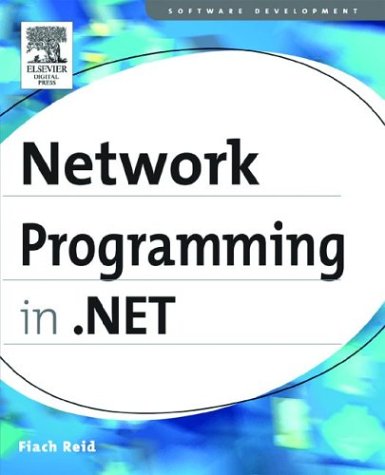
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeCloseHandleCritical.cs
- ToolStripItemEventArgs.cs
- ClientSponsor.cs
- MailWriter.cs
- CustomPopupPlacement.cs
- _TLSstream.cs
- SerializationException.cs
- ISessionStateStore.cs
- StaticSiteMapProvider.cs
- WeakEventTable.cs
- TextSegment.cs
- assertwrapper.cs
- DataExpression.cs
- SqlInternalConnectionTds.cs
- PenLineJoinValidation.cs
- DataStorage.cs
- DesignObjectWrapper.cs
- IWorkflowDebuggerService.cs
- CompilerWrapper.cs
- TraceRecords.cs
- AuthenticationService.cs
- WizardPanel.cs
- PassportAuthenticationModule.cs
- ElementProxy.cs
- PointKeyFrameCollection.cs
- ChangeConflicts.cs
- BamlResourceSerializer.cs
- QueryOptionExpression.cs
- OracleException.cs
- SchemaCollectionCompiler.cs
- Application.cs
- EntityFrameworkVersions.cs
- MainMenu.cs
- HitTestWithPointDrawingContextWalker.cs
- TextMarkerSource.cs
- DirectoryRedirect.cs
- CancellableEnumerable.cs
- DocumentPageViewAutomationPeer.cs
- SimplePropertyEntry.cs
- ServiceActivationException.cs
- TableLayoutStyle.cs
- QualifierSet.cs
- LicenseManager.cs
- EntityClientCacheEntry.cs
- ActivityInterfaces.cs
- UmAlQuraCalendar.cs
- TriggerCollection.cs
- AutomationElement.cs
- InternalControlCollection.cs
- ToolStripInSituService.cs
- EventPropertyMap.cs
- ExpandableObjectConverter.cs
- IRCollection.cs
- NegotiationTokenProvider.cs
- RepeatBehavior.cs
- SqlUdtInfo.cs
- LayoutEvent.cs
- AndCondition.cs
- Missing.cs
- HTTP_SERVICE_CONFIG_URLACL_PARAM.cs
- StructuredProperty.cs
- RelatedEnd.cs
- ValidationPropertyAttribute.cs
- HttpsTransportBindingElement.cs
- FullTextBreakpoint.cs
- FormView.cs
- NameValueCollection.cs
- BulletedList.cs
- ItemDragEvent.cs
- ErrorFormatterPage.cs
- XmlNodeComparer.cs
- DocumentGridContextMenu.cs
- ResourceExpressionEditor.cs
- DetailsViewInsertedEventArgs.cs
- embossbitmapeffect.cs
- StringDictionary.cs
- DetailsViewPagerRow.cs
- ExpressionParser.cs
- IntPtr.cs
- DataGridViewImageColumn.cs
- XmlBoundElement.cs
- XNodeSchemaApplier.cs
- VisualTarget.cs
- TextEditorCopyPaste.cs
- IdentitySection.cs
- DataGridViewAdvancedBorderStyle.cs
- SiteMapPath.cs
- NamespaceEmitter.cs
- TableLayoutCellPaintEventArgs.cs
- EnvelopedPkcs7.cs
- ClientConfigPaths.cs
- PropertyValueUIItem.cs
- ThreadExceptionDialog.cs
- XslUrlEditor.cs
- TextureBrush.cs
- TypedReference.cs
- Win32.cs
- SignatureHelper.cs
- EventManager.cs
- Selection.cs