Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Diagnostics / PerformanceCounters.cs / 1 / PerformanceCounters.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Diagnostics { using System; using System.ServiceModel; using System.ServiceModel.Dispatcher; using System.ServiceModel.Description; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Reflection; using System.Runtime.InteropServices; using System.ServiceModel.Channels; using System.ServiceModel.Configuration; using System.ServiceModel.Diagnostics; using System.Security; internal static class PerformanceCounters { static PerformanceCounterScope scope = PerformanceCounterScope.Default; static object perfCounterDictionarySyncObject = new object(); internal const int MaxInstanceNameLength = 127; static bool serviceOOM = false; static bool endpointOOM = false; static bool operationOOM = false; //we need a couple of ways of accessing the same performance counters. Normally, we know which endpoint //for which we need to update a perf counter. In some cases (e.g. RM), we only have a base uri. In those //cases, we update all the perf counters associated with the base uri. These two dictionaries point to //the same underlying perf counters, but in different ways. static DictionaryperformanceCounters = null; static Dictionary performanceCountersBaseUri = null; static internal PerformanceCounterScope Scope { get { return PerformanceCounters.scope; } } static internal bool PerformanceCountersEnabled { get { return (PerformanceCounters.scope != PerformanceCounterScope.Off) && (PerformanceCounters.scope != PerformanceCounterScope.Default); } } static internal bool MinimalPerformanceCountersEnabled { get { return (PerformanceCounters.scope == PerformanceCounterScope.Default); } } static PerformanceCounters() { PerformanceCounterScope scope = GetPerformanceCountersFromConfig(); if (PerformanceCounterScope.Off != scope) { try { AppDomain.CurrentDomain.UnhandledException += new UnhandledExceptionEventHandler(ExitOrUnloadEventHandler); AppDomain.CurrentDomain.DomainUnload += new EventHandler(ExitOrUnloadEventHandler); AppDomain.CurrentDomain.ProcessExit += new EventHandler(ExitOrUnloadEventHandler); PerformanceCounters.scope = scope; } catch (SecurityException securityException) { //switch off the counters - not supported in PT PerformanceCounters.scope = PerformanceCounterScope.Off; // not re-throwing on purpose if (DiagnosticUtility.ShouldTraceWarning) { DiagnosticUtility.ExceptionUtility.TraceHandledException(securityException, TraceEventType.Warning); DiagnosticUtility.DiagnosticTrace.TraceEvent(System.Diagnostics.TraceEventType.Warning, TraceCode.PerformanceCounterFailedToLoad, SR.GetString(SR.PartialTrustPerformanceCountersNotEnabled)); } } } else { PerformanceCounters.scope = PerformanceCounterScope.Off; } } /// /// Critical - calls SecurityCritical method UnsafeGetSection which elevates in order to load config /// Safe - does not leak any config objects /// [SecurityCritical, SecurityTreatAsSafe] static PerformanceCounterScope GetPerformanceCountersFromConfig() { return DiagnosticSection.UnsafeGetSection().PerformanceCounters; } static internal PerformanceCounter GetOperationPerformanceCounter(string perfCounterName, string instanceName) { return PerformanceCounters.GetPerformanceCounter( PerformanceCounterStrings.SERVICEMODELOPERATION.OperationPerfCounters, perfCounterName, instanceName, PerformanceCounterInstanceLifetime.Process); } static internal PerformanceCounter GetEndpointPerformanceCounter(string perfCounterName, string instanceName) { return PerformanceCounters.GetPerformanceCounter( PerformanceCounterStrings.SERVICEMODELENDPOINT.EndpointPerfCounters, perfCounterName, instanceName, PerformanceCounterInstanceLifetime.Process); } static internal PerformanceCounter GetServicePerformanceCounter(string perfCounterName, string instanceName) { return PerformanceCounters.GetPerformanceCounter( PerformanceCounterStrings.SERVICEMODELSERVICE.ServicePerfCounters, perfCounterName, instanceName, PerformanceCounterInstanceLifetime.Process); } static internal PerformanceCounter GetDefaultPerformanceCounter(string perfCounterName, string instanceName) { return PerformanceCounters.GetPerformanceCounter( PerformanceCounterStrings.SERVICEMODELSERVICE.ServicePerfCounters, perfCounterName, instanceName, PerformanceCounterInstanceLifetime.Global); } static internal PerformanceCounter GetPerformanceCounter(string categoryName, string perfCounterName, string instanceName, PerformanceCounterInstanceLifetime instanceLifetime) { PerformanceCounter counter = null; if (PerformanceCounters.PerformanceCountersEnabled || PerformanceCounters.MinimalPerformanceCountersEnabled) { counter = PerformanceCounters.GetPerformanceCounterInternal(categoryName, perfCounterName, instanceName, instanceLifetime); } return counter; } static internal PerformanceCounter GetPerformanceCounterInternal(string categoryName, string perfCounterName, string instanceName, PerformanceCounterInstanceLifetime instanceLifetime) { PerformanceCounter counter = null; try { counter = new PerformanceCounter(); counter.CategoryName = categoryName; counter.CounterName = perfCounterName; counter.InstanceName = instanceName; counter.ReadOnly = false; counter.InstanceLifetime = instanceLifetime; // We now need to access the counter raw data to // force the counter object to be initialized. This // will force any exceptions due to mis-installation // of counters to occur here and be traced appropriately. try { long rawValue = counter.RawValue; } catch (InvalidOperationException) { counter = null; throw; } catch (SecurityException securityException) { // Cannot access performance counter due to partial trust scenarios // Disable the default performance counters' access otherwise // in PT the service will be broken by default if (PerformanceCounters.MinimalPerformanceCountersEnabled) { PerformanceCounters.scope = PerformanceCounterScope.Off; } if (DiagnosticUtility.ShouldTraceWarning) { DiagnosticUtility.ExceptionUtility.TraceHandledException(new SecurityException(SR.GetString( SR.PartialTrustPerformanceCountersNotEnabled), securityException), TraceEventType.Warning); } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SecurityException(SR.GetString( SR.PartialTrustPerformanceCountersNotEnabled))); } } #pragma warning suppress 56500 // covered by FxCOP catch (Exception e) { if (ExceptionUtility.IsFatal(e)) throw; if (null != counter) { if (!counter.ReadOnly) { try { counter.RemoveInstance(); } // Already inside a catch block for a failure case // ok to ignore any exceptions here and trace the // original failure. #pragma warning suppress 56500 // covered by FxCOP catch (Exception e1) { if (ExceptionUtility.IsFatal(e1)) throw; } } counter = null; } bool logEvent = true; if (categoryName == PerformanceCounterStrings.SERVICEMODELSERVICE.ServicePerfCounters) { if (serviceOOM == false) { serviceOOM = true; } else { logEvent = false; } } else if (categoryName == PerformanceCounterStrings.SERVICEMODELOPERATION.OperationPerfCounters) { if (operationOOM == false) { operationOOM = true; } else { logEvent = false; } } else if (categoryName == PerformanceCounterStrings.SERVICEMODELENDPOINT.EndpointPerfCounters) { if (endpointOOM == false) { endpointOOM = true; } else { logEvent = false; } } if (logEvent) { DiagnosticUtility.EventLog.LogEvent(TraceEventType.Error, EventLogCategory.PerformanceCounter, EventLogEventId.FailedToLoadPerformanceCounter, categoryName, perfCounterName, e.ToString()); } } return counter; } internal static DictionaryPerformanceCountersForEndpoint { get { if (PerformanceCounters.performanceCounters == null) { lock (PerformanceCounters.perfCounterDictionarySyncObject) { if (PerformanceCounters.performanceCounters == null) { PerformanceCounters.performanceCounters = new Dictionary (); } } } return PerformanceCounters.performanceCounters; } } internal static Dictionary PerformanceCountersForBaseUri { get { if (PerformanceCounters.performanceCountersBaseUri == null) { lock (PerformanceCounters.perfCounterDictionarySyncObject) { if (PerformanceCounters.performanceCountersBaseUri == null) { PerformanceCounters.performanceCountersBaseUri = new Dictionary (); } } } return PerformanceCounters.performanceCountersBaseUri; } } internal static void AddPerformanceCountersForEndpoint( ServiceHostBase serviceHost, ContractDescription contractDescription, EndpointDispatcher endpointDispatcher) { if (PerformanceCounters.PerformanceCountersEnabled || PerformanceCounters.MinimalPerformanceCountersEnabled) { if (endpointDispatcher.SetPerfCounterId()) { ServiceModelPerformanceCounters counters; lock (PerformanceCounters.perfCounterDictionarySyncObject) { if (!PerformanceCounters.PerformanceCountersForEndpoint.TryGetValue(endpointDispatcher.PerfCounterId, out counters)) { counters = new ServiceModelPerformanceCounters(serviceHost, contractDescription, endpointDispatcher); if (counters.Initialized) { PerformanceCounters.PerformanceCountersForEndpoint.Add(endpointDispatcher.PerfCounterId, counters); } else { return; } } } ServiceModelPerformanceCountersEntry countersEntry; lock (PerformanceCounters.perfCounterDictionarySyncObject) { if (!PerformanceCounters.PerformanceCountersForBaseUri.TryGetValue(endpointDispatcher.PerfCounterBaseId, out countersEntry)) { if (PerformanceCounters.PerformanceCountersEnabled) { countersEntry = new ServiceModelPerformanceCountersEntry(serviceHost.Counters); } else if (PerformanceCounters.MinimalPerformanceCountersEnabled) { countersEntry = new ServiceModelPerformanceCountersEntry(serviceHost.DefaultCounters); } PerformanceCounters.PerformanceCountersForBaseUri.Add(endpointDispatcher.PerfCounterBaseId, countersEntry); } countersEntry.Add(counters); } } } } internal static void ReleasePerformanceCountersForEndpoint(string id, string baseId) { if (PerformanceCounters.PerformanceCountersEnabled) { lock (PerformanceCounters.perfCounterDictionarySyncObject) { if (!String.IsNullOrEmpty(id)) { ServiceModelPerformanceCounters counters; if (PerformanceCounters.PerformanceCountersForEndpoint.TryGetValue(id, out counters)) { counters.ReleasePerformanceCounters(); PerformanceCounters.PerformanceCountersForEndpoint.Remove(id); } } if (!String.IsNullOrEmpty(baseId)) { ServiceModelPerformanceCountersEntry counters2; if (PerformanceCounters.PerformanceCountersForBaseUri.TryGetValue(baseId, out counters2)) { counters2.Remove(id); } } } } } internal static void ReleasePerformanceCountersForService(PerformanceCountersBase counters, bool isDefaultCounters) { if ((PerformanceCounters.PerformanceCountersEnabled || PerformanceCounters.MinimalPerformanceCountersEnabled) && counters != null) { List counterKeys = null; bool released = false; lock (PerformanceCounters.perfCounterDictionarySyncObject) { try { IDictionaryEnumerator e = PerformanceCounters.PerformanceCountersForBaseUri.GetEnumerator(); while (e.MoveNext()) { ServiceModelPerformanceCountersEntry entry = (ServiceModelPerformanceCountersEntry)e.Value; PerformanceCountersBase serviceCounters = null; if (isDefaultCounters) { serviceCounters = entry.DefaultPerformanceCounters; } else { serviceCounters = entry.ServicePerformanceCounters; } if (serviceCounters != null && serviceCounters.InstanceName == counters.InstanceName) { if (counterKeys == null) { counterKeys = new List (); } counterKeys.Add((string)e.Key); if (released == false) { counters.ReleasePerformanceCounters(); released = true; } } } } #pragma warning suppress 56500 // covered by FxCOP catch (Exception e) { if (ExceptionUtility.IsFatal(e)) throw; if (DiagnosticUtility.ShouldTraceError) TraceUtility.TraceEvent(TraceEventType.Error, TraceCode.PerformanceCountersFailedOnRelease, null, e); } if (counterKeys != null) { foreach (string key in counterKeys) { PerformanceCounters.PerformanceCountersForBaseUri.Remove(key); } } } } } internal static void ReleasePerformanceCounter(ref PerformanceCounter counter) { if (counter != null) { try { counter.RemoveInstance(); counter = null; } #pragma warning suppress 56500 // covered by FxCOP catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) throw; } } } internal static void TxFlowed(EndpointDispatcher el, string operation) { if (null != el) { ServicePerformanceCounters sCounters = PerformanceCounters.GetServicePerformanceCounters(el.PerfCounterId); if (null != sCounters) { sCounters.TxFlowed(); } if (PerformanceCounters.Scope == PerformanceCounterScope.All) { OperationPerformanceCounters oCounters = PerformanceCounters.GetOperationPerformanceCounters(el.PerfCounterId, operation); if (null != oCounters) { oCounters.TxFlowed(); } EndpointPerformanceCounters eCounters = PerformanceCounters.GetEndpointPerformanceCounters(el.PerfCounterId); if (null != sCounters) { eCounters.TxFlowed(); } } } } internal static void TxAborted(EndpointDispatcher el, long count) { if (PerformanceCounters.PerformanceCountersEnabled) { if (null != el) { ServicePerformanceCounters sCounters = PerformanceCounters.GetServicePerformanceCounters(el.PerfCounterId); if (null != sCounters) { sCounters.TxAborted(count); } } } } internal static void TxCommitted(EndpointDispatcher el, long count) { if (PerformanceCounters.PerformanceCountersEnabled) { if (null != el) { ServicePerformanceCounters sCounters = PerformanceCounters.GetServicePerformanceCounters(el.PerfCounterId); if (null != sCounters) { sCounters.TxCommitted(count); } } } } internal static void TxInDoubt(EndpointDispatcher el, long count) { if (PerformanceCounters.PerformanceCountersEnabled) { if (null != el) { ServicePerformanceCounters sCounters = PerformanceCounters.GetServicePerformanceCounters(el.PerfCounterId); if (null != sCounters) { sCounters.TxInDoubt(count); } } } } internal static void MethodCalled(string operationName) { EndpointDispatcher el = GetEndpointDispatcher(); if (null != el) { string uri = el.PerfCounterId; if (PerformanceCounters.Scope == PerformanceCounterScope.All) { OperationPerformanceCounters opCounters = PerformanceCounters.GetOperationPerformanceCounters(uri, operationName); if (null != opCounters) { opCounters.MethodCalled(); } EndpointPerformanceCounters eCounters = PerformanceCounters.GetEndpointPerformanceCounters(uri); if (null != eCounters) { eCounters.MethodCalled(); } } ServicePerformanceCounters sCounters = PerformanceCounters.GetServicePerformanceCounters(uri); if (null != sCounters) { sCounters.MethodCalled(); } } } internal static void MethodReturnedSuccess(string operationName) { PerformanceCounters.MethodReturnedSuccess(operationName, -1); } internal static void MethodReturnedSuccess(string operationName, long time) { EndpointDispatcher el = GetEndpointDispatcher(); if (null != el) { string uri = el.PerfCounterId; if (PerformanceCounters.Scope == PerformanceCounterScope.All) { OperationPerformanceCounters counters = PerformanceCounters.GetOperationPerformanceCounters(uri, operationName); if (null != counters) { counters.MethodReturnedSuccess(); if (time > 0) { counters.SaveCallDuration(time); } } EndpointPerformanceCounters eCounters = PerformanceCounters.GetEndpointPerformanceCounters(uri); if (null != eCounters) { eCounters.MethodReturnedSuccess(); if (time > 0) { eCounters.SaveCallDuration(time); } } } ServicePerformanceCounters sCounters = PerformanceCounters.GetServicePerformanceCounters(el.PerfCounterId); if (null != sCounters) { sCounters.MethodReturnedSuccess(); if (time > 0) { sCounters.SaveCallDuration(time); } } } } internal static void MethodReturnedFault(string operationName) { EndpointDispatcher el = GetEndpointDispatcher(); if (null != el) { string uri = el.PerfCounterId; if (PerformanceCounters.Scope == PerformanceCounterScope.All) { OperationPerformanceCounters counters = PerformanceCounters.GetOperationPerformanceCounters(uri, operationName); if (null != counters) { counters.MethodReturnedFault(); } EndpointPerformanceCounters eCounters = PerformanceCounters.GetEndpointPerformanceCounters(uri); if (null != eCounters) { eCounters.MethodReturnedFault(); } } ServicePerformanceCounters sCounters = PerformanceCounters.GetServicePerformanceCounters(el.PerfCounterId); if (null != sCounters) { sCounters.MethodReturnedFault(); } } } internal static void MethodReturnedError(string operationName) { EndpointDispatcher el = GetEndpointDispatcher(); if (null != el) { string uri = el.PerfCounterId; if (PerformanceCounters.Scope == PerformanceCounterScope.All) { OperationPerformanceCounters counters = PerformanceCounters.GetOperationPerformanceCounters(uri, operationName); if (null != counters) { counters.MethodReturnedError(); } EndpointPerformanceCounters eCounters = PerformanceCounters.GetEndpointPerformanceCounters(uri); if (null != eCounters) { eCounters.MethodReturnedError(); } } ServicePerformanceCounters sCounters = PerformanceCounters.GetServicePerformanceCounters(el.PerfCounterId); if (null != sCounters) { sCounters.MethodReturnedError(); } } } static void InvokeMethod(object o, string methodName) { DiagnosticUtility.DebugAssert(null != o, "object must not be null"); MethodInfo method = o.GetType().GetMethod(methodName, BindingFlags.NonPublic | BindingFlags.Instance); DiagnosticUtility.DebugAssert(null != method, o.GetType().ToString() + " must have method " + methodName); method.Invoke(o, null); } static void CallOnAllCounters(string methodName, Message message, Uri listenUri, bool includeOperations) { DiagnosticUtility.DebugAssert(null != message, "message must not be null"); DiagnosticUtility.DebugAssert(null != listenUri, "listenUri must not be null"); if (null != message && null != message.Headers && null != message.Headers.To && null != listenUri) { string uri = listenUri.AbsoluteUri.ToUpperInvariant(); ServiceModelPerformanceCountersEntry counters = PerformanceCounters.GetServiceModelPerformanceCountersBaseUri(uri); if (null != counters) { DiagnosticUtility.DebugAssert(null != counters.ServicePerformanceCounters, "counters.ServicePerformanceCounters must not be null"); PerformanceCounters.InvokeMethod(counters.ServicePerformanceCounters, methodName); if (PerformanceCounters.Scope == PerformanceCounterScope.All) { List counters2 = counters.CounterList; foreach (ServiceModelPerformanceCounters sCounters in counters2) { if (sCounters.EndpointPerformanceCounters != null) { PerformanceCounters.InvokeMethod(sCounters.EndpointPerformanceCounters, methodName); } if (includeOperations) { OperationPerformanceCounters oCounters = sCounters.GetOperationPerformanceCountersFromMessage(message); if (oCounters != null) { PerformanceCounters.InvokeMethod(oCounters, methodName); } } } } } } } static internal void AuthenticationFailed(Message message, Uri listenUri) { PerformanceCounters.CallOnAllCounters("AuthenticationFailed", message, listenUri, true); } static internal void AuthorizationFailed(string operationName) { EndpointDispatcher el = GetEndpointDispatcher(); if (null != el) { string uri = el.PerfCounterId; if (PerformanceCounters.Scope == PerformanceCounterScope.All) { OperationPerformanceCounters counters = PerformanceCounters.GetOperationPerformanceCounters(uri, operationName); if (null != counters) { counters.AuthorizationFailed(); } EndpointPerformanceCounters eCounters = PerformanceCounters.GetEndpointPerformanceCounters(uri); if (null != eCounters) { eCounters.AuthorizationFailed(); } } ServicePerformanceCounters sCounters = PerformanceCounters.GetServicePerformanceCounters(el.PerfCounterId); if (null != sCounters) { sCounters.AuthorizationFailed(); } } } internal static void SessionFaulted(string uri) { ServiceModelPerformanceCountersEntry counters = PerformanceCounters.GetServiceModelPerformanceCountersBaseUri(uri); if (null != counters) { counters.ServicePerformanceCounters.SessionFaulted(); if (PerformanceCounters.Scope == PerformanceCounterScope.All) { List counters2 = counters.CounterList; foreach (ServiceModelPerformanceCounters sCounters in counters2) { if (sCounters.EndpointPerformanceCounters != null) { sCounters.EndpointPerformanceCounters.SessionFaulted(); } } } } } internal static void MessageDropped(string uri) { ServiceModelPerformanceCountersEntry counters = PerformanceCounters.GetServiceModelPerformanceCountersBaseUri(uri); if (null != counters) { counters.ServicePerformanceCounters.MessageDropped(); if (PerformanceCounters.Scope == PerformanceCounterScope.All) { List counters2 = counters.CounterList; foreach (ServiceModelPerformanceCounters sCounters in counters2) { if (sCounters.EndpointPerformanceCounters != null) { sCounters.EndpointPerformanceCounters.MessageDropped(); } } } } } internal static void MsmqDroppedMessage(string uri) { if (PerformanceCounters.Scope == PerformanceCounterScope.All) { ServiceModelPerformanceCountersEntry counters = PerformanceCounters.GetServiceModelPerformanceCountersBaseUri(uri); if (null != counters) { counters.ServicePerformanceCounters.MsmqDroppedMessage(); } } } internal static void MsmqPoisonMessage(string uri) { if (PerformanceCounters.Scope == PerformanceCounterScope.All) { ServiceModelPerformanceCountersEntry counters = PerformanceCounters.GetServiceModelPerformanceCountersBaseUri(uri); if (null != counters) { counters.ServicePerformanceCounters.MsmqPoisonMessage(); } } } internal static void MsmqRejectedMessage(string uri) { if (PerformanceCounters.Scope == PerformanceCounterScope.All) { ServiceModelPerformanceCountersEntry counters = PerformanceCounters.GetServiceModelPerformanceCountersBaseUri(uri); if (null != counters) { counters.ServicePerformanceCounters.MsmqRejectedMessage(); } } } static internal EndpointDispatcher GetEndpointDispatcher() { EndpointDispatcher endpointDispatcher = null; if (null != OperationContext.Current && OperationContext.Current.InternalServiceChannel != null) { endpointDispatcher = OperationContext.Current.EndpointDispatcher; } return endpointDispatcher; } static ServiceModelPerformanceCounters GetServiceModelPerformanceCounters(string uri) { ServiceModelPerformanceCounters counters = null; if (!String.IsNullOrEmpty(uri)) { PerformanceCounters.PerformanceCountersForEndpoint.TryGetValue(uri, out counters); } return counters; } static ServiceModelPerformanceCountersEntry GetServiceModelPerformanceCountersBaseUri(string uri) { ServiceModelPerformanceCountersEntry counters = null; if (!String.IsNullOrEmpty(uri)) { PerformanceCounters.PerformanceCountersForBaseUri.TryGetValue(uri, out counters); } return counters; } static OperationPerformanceCounters GetOperationPerformanceCounters(string uri, string operation) { ServiceModelPerformanceCounters counters = PerformanceCounters.GetServiceModelPerformanceCounters(uri); if (counters != null) { return counters.GetOperationPerformanceCounters(operation); } return null; } static EndpointPerformanceCounters GetEndpointPerformanceCounters(string uri) { ServiceModelPerformanceCounters counters = PerformanceCounters.GetServiceModelPerformanceCounters(uri); if (counters != null) { return counters.EndpointPerformanceCounters; } return null; } static ServicePerformanceCounters GetServicePerformanceCounters(string uri) { ServiceModelPerformanceCounters counters = PerformanceCounters.GetServiceModelPerformanceCounters(uri); if (counters != null) { return counters.ServicePerformanceCounters; } return null; } static internal void TracePerformanceCounterUpdateFailure(string instanceName, string perfCounterName) { if (DiagnosticUtility.ShouldTraceError) { DiagnosticUtility.DiagnosticTrace.TraceEvent( System.Diagnostics.TraceEventType.Error, TraceCode.PerformanceCountersFailedDuringUpdate, SR.GetString(SR.TraceCodePerformanceCountersFailedDuringUpdate, perfCounterName + "::" + instanceName)); } } static void ExitOrUnloadEventHandler(object sender, EventArgs e) { Dictionary countersToRemove = null; if (PerformanceCounters.performanceCounters != null) { lock (perfCounterDictionarySyncObject) { if (PerformanceCounters.performanceCounters != null) { countersToRemove = PerformanceCounters.performanceCounters; PerformanceCounters.performanceCounters = null; PerformanceCounters.performanceCountersBaseUri = null; } } } if (null != countersToRemove) { IDictionaryEnumerator enumerator = countersToRemove.GetEnumerator(); while (enumerator.MoveNext()) { ServiceModelPerformanceCounters counters = (ServiceModelPerformanceCounters)enumerator.Value; ServicePerformanceCounters serviceCounters = counters.ServicePerformanceCounters; DefaultPerformanceCounters defaultCounters = counters.DefaultPerformanceCounters; counters.ReleasePerformanceCounters(); if (serviceCounters != null) { serviceCounters.ReleasePerformanceCounters(); } if (defaultCounters != null) { defaultCounters.ReleasePerformanceCounters(); } } countersToRemove.Clear(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
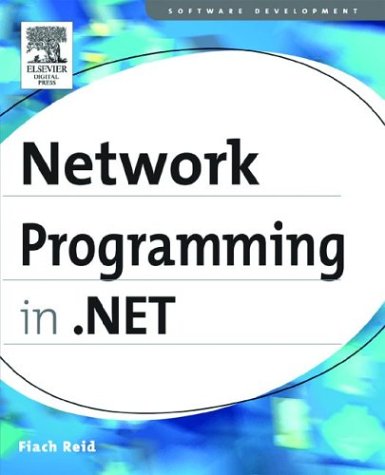
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SecurityHelper.cs
- IntegrationExceptionEventArgs.cs
- FixedSOMTable.cs
- ReflectPropertyDescriptor.cs
- ScriptRegistrationManager.cs
- JsonServiceDocumentSerializer.cs
- Array.cs
- GroupBox.cs
- KeyedCollection.cs
- PointKeyFrameCollection.cs
- MdbDataFileEditor.cs
- StringOutput.cs
- TypeToken.cs
- XmlExtensionFunction.cs
- LoginCancelEventArgs.cs
- FixedDocument.cs
- TabControl.cs
- BamlLocalizableResource.cs
- UmAlQuraCalendar.cs
- DataGridViewRowPostPaintEventArgs.cs
- XPathNavigatorKeyComparer.cs
- FixedElement.cs
- PartDesigner.cs
- Helper.cs
- Panel.cs
- DocumentApplicationJournalEntry.cs
- InstanceDescriptor.cs
- ResourceDictionaryCollection.cs
- SequentialOutput.cs
- BuiltInExpr.cs
- OracleString.cs
- ObfuscationAttribute.cs
- SecurityStateEncoder.cs
- _ProxyChain.cs
- ManipulationCompletedEventArgs.cs
- BinaryMessageEncoder.cs
- DesignerAttribute.cs
- SHA256CryptoServiceProvider.cs
- BroadcastEventHelper.cs
- ResourceAssociationSetEnd.cs
- ColumnMap.cs
- SQLSingleStorage.cs
- ResourcePermissionBaseEntry.cs
- Visitor.cs
- ValidatorCompatibilityHelper.cs
- WindowsButton.cs
- TraceUtility.cs
- GacUtil.cs
- AssertValidation.cs
- _SslState.cs
- UserControl.cs
- COAUTHIDENTITY.cs
- FigureParagraph.cs
- SessionStateContainer.cs
- DesignerCategoryAttribute.cs
- DockProviderWrapper.cs
- ResourceDisplayNameAttribute.cs
- AutomationPatternInfo.cs
- WindowsAuthenticationModule.cs
- Brush.cs
- ClipboardProcessor.cs
- XhtmlBasicPageAdapter.cs
- LinearGradientBrush.cs
- precedingsibling.cs
- sqlser.cs
- FragmentQueryProcessor.cs
- FtpWebRequest.cs
- DataGridViewRowStateChangedEventArgs.cs
- GeneratedView.cs
- BuildManager.cs
- LinqDataSource.cs
- Options.cs
- ModulesEntry.cs
- SqlInfoMessageEvent.cs
- Profiler.cs
- XmlDocument.cs
- ExpressionBuilder.cs
- Journaling.cs
- ComplusTypeValidator.cs
- CompilerGeneratedAttribute.cs
- IisTraceListener.cs
- TypeConverterHelper.cs
- DesignerSerializationOptionsAttribute.cs
- InvalidCastException.cs
- FrameworkRichTextComposition.cs
- CapiSafeHandles.cs
- DbConnectionOptions.cs
- UnsignedPublishLicense.cs
- ApplicationServiceManager.cs
- CodeTypeParameterCollection.cs
- PageScaling.cs
- ExternalCalls.cs
- _DigestClient.cs
- CodeTypeParameterCollection.cs
- AlternationConverter.cs
- Axis.cs
- PageHandlerFactory.cs
- DataFormats.cs
- ItemCollection.cs
- LayoutManager.cs