Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / ComIntegration / SecurityCookieModeValidator.cs / 1 / SecurityCookieModeValidator.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.ComIntegration { using System; using System.ServiceModel.Channels; using System.ServiceModel.Description; using System.ServiceModel.Dispatcher; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.ServiceModel; using System.ServiceModel.Security.Tokens; class SecurityCookieModeValidator : IServiceBehavior { void CheckForCookie(SecurityTokenParameters tokenParameters, ServiceEndpoint endpoint) { bool cookie = false; SecureConversationSecurityTokenParameters sc = tokenParameters as SecureConversationSecurityTokenParameters; if (sc != null && sc.RequireCancellation == false) cookie = true; SspiSecurityTokenParameters sspi = tokenParameters as SspiSecurityTokenParameters; if (sspi != null && sspi.RequireCancellation == false) cookie = true; SspiSecurityTokenParameters ssl = tokenParameters as SspiSecurityTokenParameters; if (ssl != null && ssl.RequireCancellation == false) cookie = true; if (cookie) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.RequireNonCookieMode, endpoint.Binding.Name, endpoint.Binding.Namespace))); } void IServiceBehavior.AddBindingParameters(ServiceDescription description, ServiceHostBase serviceHostBase, Collectionendpoints, BindingParameterCollection parameters) { } void IServiceBehavior.Validate(ServiceDescription service, ServiceHostBase serviceHostBase) { } void IServiceBehavior.ApplyDispatchBehavior(ServiceDescription service, ServiceHostBase serviceHostBase) { // The philosophy here is to respect settings obtained from the // service surrogate class' attributes, as written by the user, // while rejecting those that contradict our requirements. // We never want to silently overwrite a user's attributes. // So we either accept overrides or reject them. // // If you're changing this code, you'll probably also want to change // ComPlusServiceLoader.AddBehaviors foreach (ServiceEndpoint endpoint in service.Endpoints) { ICollection bindingElements = endpoint.Binding.CreateBindingElements(); foreach (BindingElement element in bindingElements) { SymmetricSecurityBindingElement sbe = (element as SymmetricSecurityBindingElement); if (sbe != null) { this.CheckForCookie(sbe.ProtectionTokenParameters, endpoint); foreach (SecurityTokenParameters p in sbe.EndpointSupportingTokenParameters.Endorsing) this.CheckForCookie(p, endpoint); break; } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
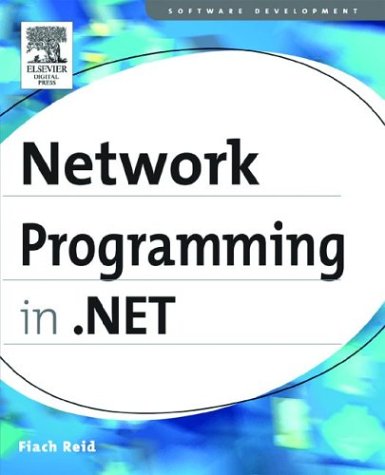
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnsafeNativeMethods.cs
- SoapException.cs
- COM2EnumConverter.cs
- Semaphore.cs
- AddInAttribute.cs
- ExtenderProviderService.cs
- NotFiniteNumberException.cs
- BuildDependencySet.cs
- MultiSelectRootGridEntry.cs
- PathSegmentCollection.cs
- CompilerErrorCollection.cs
- TextWriterEngine.cs
- DataGridViewToolTip.cs
- ComplexType.cs
- AdapterUtil.cs
- RTLAwareMessageBox.cs
- EntityDataSourceEntitySetNameItem.cs
- SQLBinary.cs
- DataGridCellsPresenter.cs
- PropertyGridEditorPart.cs
- TableColumnCollectionInternal.cs
- ArgumentDirectionHelper.cs
- DataTableReaderListener.cs
- PeerMessageDispatcher.cs
- EditorBrowsableAttribute.cs
- ProfessionalColorTable.cs
- WindowsFont.cs
- RepeaterItemCollection.cs
- URLAttribute.cs
- GroupQuery.cs
- ExceptionHandlersDesigner.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- RowToFieldTransformer.cs
- GridViewHeaderRowPresenter.cs
- ViewStateChangedEventArgs.cs
- InputElement.cs
- AnimationClockResource.cs
- CollectionEditor.cs
- XPathSelfQuery.cs
- CodeSnippetCompileUnit.cs
- ZipPackagePart.cs
- ChtmlPageAdapter.cs
- DataBoundLiteralControl.cs
- BrowserCapabilitiesCodeGenerator.cs
- ScrollViewer.cs
- WorkflowElementDialog.cs
- BaseHashHelper.cs
- BamlBinaryWriter.cs
- SqlBinder.cs
- CharEnumerator.cs
- PathGradientBrush.cs
- MemberAssignment.cs
- X509Utils.cs
- PropertyOverridesDialog.cs
- ItemCollectionEditor.cs
- StorageTypeMapping.cs
- SupportingTokenSecurityTokenResolver.cs
- MobileComponentEditorPage.cs
- StyleXamlParser.cs
- StoragePropertyMapping.cs
- DocumentSequence.cs
- TraceHandlerErrorFormatter.cs
- LogPolicy.cs
- Stack.cs
- CodeStatement.cs
- TableHeaderCell.cs
- CommandBindingCollection.cs
- ThrowHelper.cs
- ListDictionaryInternal.cs
- CopyOfAction.cs
- wgx_render.cs
- PropagatorResult.cs
- ByteAnimationBase.cs
- ParentControlDesigner.cs
- AccessKeyManager.cs
- BinaryOperationBinder.cs
- DateTimeParse.cs
- PathFigureCollectionValueSerializer.cs
- NetStream.cs
- StyleModeStack.cs
- ClientConvert.cs
- ReadContentAsBinaryHelper.cs
- _UncName.cs
- DBSchemaTable.cs
- PointLightBase.cs
- DataGridViewToolTip.cs
- InputProcessorProfiles.cs
- WindowManager.cs
- SchemaSetCompiler.cs
- PenContexts.cs
- ResourceKey.cs
- ObjectQuery.cs
- NameNode.cs
- UniqueIdentifierService.cs
- RelatedCurrencyManager.cs
- Parser.cs
- PlanCompiler.cs
- ListControl.cs
- AxisAngleRotation3D.cs
- LogEntrySerializationException.cs