Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / ComIntegration / EmitterCache.cs / 1 / EmitterCache.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.ComIntegration { using System; using System.Reflection; using System.Reflection.Emit; using System.Collections.Generic; using System.Threading; using System.Runtime.InteropServices; internal class EmitterCache { private static EmitterCache Provider = null; private static object initLock = new object (); internal static EmitterCache TypeEmitter { get { lock (initLock) { if (Provider == null) { EmitterCache localProvider = new EmitterCache(); Thread.MemoryBarrier(); Provider = localProvider; } } if (Provider == null) { DiagnosticUtility.DebugAssert("Provider should not be null"); #pragma warning suppress 56503 // throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(true); } return Provider; } } ModuleBuilder DynamicModule; AssemblyBuilder assemblyBuilder; DictionaryinterfaceToClassMap ; private EmitterCache () { AssemblyName assemblyName = new AssemblyName(); assemblyName.Name = Guid.NewGuid().ToString (); assemblyBuilder = Thread.GetDomain().DefineDynamicAssembly(assemblyName, AssemblyBuilderAccess.Run); DynamicModule = assemblyBuilder.DefineDynamicModule (Guid.NewGuid().ToString () ); interfaceToClassMap = new Dictionary (); } private Type [] GetParameterTypes (MethodInfo mInfo) { ParameterInfo [] parameters = mInfo.GetParameters (); Type [] typeArray = new Type [parameters.Length ]; int index = 0; for (; index < parameters.Length; index++) { typeArray [index] = parameters [index].ParameterType ; } return typeArray; } internal Type FindOrCreateType (Type interfaceType) { if (!interfaceType.IsInterface) { DiagnosticUtility.DebugAssert("Passed in type should be an Interface"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } Type classType = null; lock(this) { interfaceToClassMap.TryGetValue (interfaceType, out classType); if (classType == null) { TypeBuilder typeBuilder = DynamicModule.DefineType(interfaceType.Name + "MarshalByRefObject", TypeAttributes.Public | TypeAttributes.Class | TypeAttributes.Abstract, typeof(MarshalByRefObject), new Type[] { interfaceType }); Type[] ctorParams = new Type[] { typeof(ClassInterfaceType) }; ConstructorInfo classCtorInfo = typeof(ClassInterfaceAttribute).GetConstructor(ctorParams); CustomAttributeBuilder attributeBuilder = new CustomAttributeBuilder(classCtorInfo, new object[] { ClassInterfaceType.None }); typeBuilder.SetCustomAttribute(attributeBuilder); typeBuilder.AddInterfaceImplementation(interfaceType); foreach (MethodInfo mInfo in interfaceType.GetMethods()) { MethodBuilder methodInClass = null; methodInClass = typeBuilder.DefineMethod(mInfo.Name, MethodAttributes.Public | MethodAttributes.Virtual | MethodAttributes.Abstract | MethodAttributes.Abstract | MethodAttributes.HideBySig | MethodAttributes.NewSlot, mInfo.ReturnType, GetParameterTypes(mInfo)); } classType = typeBuilder.CreateType(); interfaceToClassMap[interfaceType] = classType; } } if (classType == null) { DiagnosticUtility.DebugAssert("Class Type should not be null at this point"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } return classType; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
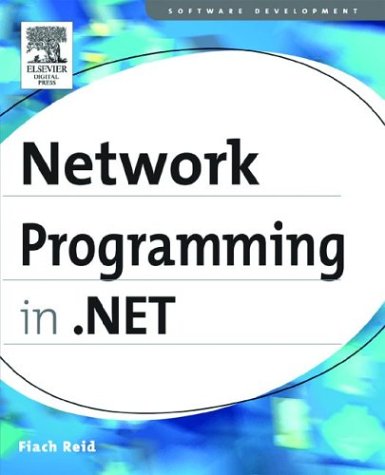
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridRelationshipRow.cs
- AudioLevelUpdatedEventArgs.cs
- XamlToRtfParser.cs
- X509Certificate.cs
- PointKeyFrameCollection.cs
- CardSpacePolicyElement.cs
- LogReservationCollection.cs
- DBDataPermissionAttribute.cs
- SplineKeyFrames.cs
- VectorCollectionConverter.cs
- SuppressMessageAttribute.cs
- DbMetaDataCollectionNames.cs
- WindowsFont.cs
- MetricEntry.cs
- HyperLinkDataBindingHandler.cs
- DataTableMappingCollection.cs
- ToolStripSplitStackLayout.cs
- ServicePoint.cs
- CorrelationManager.cs
- ComponentEvent.cs
- WebServicesInteroperability.cs
- WebPartConnectionsCancelEventArgs.cs
- GridErrorDlg.cs
- DataGridTextBox.cs
- MultiPageTextView.cs
- XmlFormatExtensionPrefixAttribute.cs
- SoundPlayer.cs
- UnsafeNativeMethods.cs
- ReflectionTypeLoadException.cs
- HebrewNumber.cs
- IPipelineRuntime.cs
- BufferedReadStream.cs
- AppDomainInfo.cs
- FileStream.cs
- MapPathBasedVirtualPathProvider.cs
- Typeface.cs
- TransformerInfoCollection.cs
- StaticExtension.cs
- ListSurrogate.cs
- BeginEvent.cs
- SmtpAuthenticationManager.cs
- HtmlPanelAdapter.cs
- SetStateDesigner.cs
- SqlGatherProducedAliases.cs
- DataServiceKeyAttribute.cs
- ValidationError.cs
- ValidationUtility.cs
- DefaultEventAttribute.cs
- _NestedSingleAsyncResult.cs
- DataSourceControlBuilder.cs
- NullableDecimalAverageAggregationOperator.cs
- ToolStripSplitStackLayout.cs
- SparseMemoryStream.cs
- FrameworkContentElementAutomationPeer.cs
- SqlDataSourceConfigureFilterForm.cs
- ComponentSerializationService.cs
- SignatureConfirmationElement.cs
- MaskedTextBox.cs
- ImageFormatConverter.cs
- SingleObjectCollection.cs
- IntSecurity.cs
- Executor.cs
- Base64Stream.cs
- _BasicClient.cs
- autovalidator.cs
- ContentWrapperAttribute.cs
- Version.cs
- WebHttpDispatchOperationSelectorData.cs
- BamlLocalizer.cs
- SafeIUnknown.cs
- AutoCompleteStringCollection.cs
- Parser.cs
- BreakRecordTable.cs
- EntityContainerEntitySetDefiningQuery.cs
- ImpersonateTokenRef.cs
- ImmComposition.cs
- DateBoldEvent.cs
- Select.cs
- ReadWriteSpinLock.cs
- RuntimeWrappedException.cs
- HttpUnhandledOperationInvoker.cs
- BehaviorEditorPart.cs
- BitmapCodecInfoInternal.cs
- HttpWebRequestElement.cs
- ToolStripHighContrastRenderer.cs
- ContainsRowNumberChecker.cs
- HttpResponse.cs
- AlgoModule.cs
- UrlMappingsModule.cs
- StyleXamlParser.cs
- ObjectQueryState.cs
- CompilationSection.cs
- ConfigurationException.cs
- LateBoundBitmapDecoder.cs
- PathFigureCollection.cs
- DocumentPageTextView.cs
- ReflectionTypeLoadException.cs
- ArrayList.cs
- ProfilePropertySettingsCollection.cs
- NextPreviousPagerField.cs