Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Activation / MetabaseReader.cs / 1 / MetabaseReader.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Activation { using System; using System.Globalization; using System.Text; using System.Collections.Generic; using System.Runtime.InteropServices; using System.ServiceModel.Security; using System.Threading; using Microsoft.Win32.SafeHandles; using System.Security; using System.Security.Permissions; // These values are copied from %SDXROOT%\public\sdk\inc\iiscnfg.h. enum MetabasePropertyType { ServerBindings = 1023, SecureBindings = 2021, AuthFlags = 6000, Realm = 6001, AnonymousUserName = 6020, AnonymousPassword = 6021, AccessSslFlags = 6030, AuthPersistence = 6031, AuthProviders = 6032, } ////// Critical - does a bunch of unsafe and native access /// caller should guard MetabaseReader instance as well as any results /// [SecurityCritical(SecurityCriticalScope.Everything)] class MetabaseReader : IDisposable { internal const string LMPath = "/LM"; const uint E_INSUFFICIENT_BUFFER = 0x8007007A; const uint E_PATH_NOT_FOUND = 0x80070003; const uint E_DATA_NOT_FOUND = 0x800CC801; static IMSAdminBase adminBase; static object syncRoot = new object(); int mdHandle = 0; METADATA_RECORD record; SafeHandle bufferHandle; uint currentBufferSize = 1024; bool disposed; public MetabaseReader() { lock (syncRoot) { if (adminBase == null) { adminBase = (IMSAdminBase)new MSAdminBase(); } } uint handle; uint hResult = adminBase.OpenKey(MSAdminBase.METADATA_MASTER_ROOT_HANDLE, LMPath, MSAdminBase.METADATA_PERMISSION_READ, MSAdminBase.DEFAULT_METABASE_TIMEOUT, out handle); mdHandle = (int) handle; if (hResult != 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new COMException(SR.GetString(SR.Hosting_MetabaseAccessError), (int)hResult)); } bufferHandle = SafeHGlobalHandleWrapper.AllocHGlobal(currentBufferSize); } ~MetabaseReader() { Dispose(false); } public object GetData(string path, MetabasePropertyType propertyType) { return GetData(path, (uint)propertyType); } public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } void EnsureRecordBuffer(uint bytes) { if (bytes <= currentBufferSize) { return; } SafeHGlobalHandleWrapper.CloseHandle(bufferHandle); currentBufferSize = bytes; bufferHandle = SafeHGlobalHandleWrapper.AllocHGlobal(currentBufferSize); record.pbMDData = bufferHandle.DangerousGetHandle(); record.dwMDDataLen = currentBufferSize; } object GetData(string path, uint type) { uint bytes = currentBufferSize; record.dwMDAttributes = MSAdminBase.METADATA_INHERIT; record.dwMDUserType = MSAdminBase.IIS_MD_UT_SERVER; record.dwMDDataType = MSAdminBase.ALL_METADATA; record.dwMDIdentifier = type; record.pbMDData = bufferHandle.DangerousGetHandle(); record.dwMDDataLen = currentBufferSize; uint hResult = adminBase.GetData((uint) mdHandle, path, ref record, ref bytes); if (hResult == E_INSUFFICIENT_BUFFER) { EnsureRecordBuffer(bytes); hResult = adminBase.GetData((uint) mdHandle, path, ref record, ref bytes); } if (hResult == E_PATH_NOT_FOUND || hResult == E_DATA_NOT_FOUND) { return null; } else if (hResult != 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new COMException(SR.GetString(SR.Hosting_MetabaseAccessError), (int)hResult)); } return ConvertData(); } object ConvertData() { switch (record.dwMDDataType) { case MSAdminBase.DWORD_METADATA: return (UInt32)Marshal.ReadInt32(record.pbMDData); case MSAdminBase.EXPANDSZ_METADATA: case MSAdminBase.STRING_METADATA: return Marshal.PtrToStringUni(record.pbMDData); case MSAdminBase.MULTISZ_METADATA: return RecordToStringArray(); default: throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException(SR.GetString( SR.Hosting_MetabaseDataTypeUnsupported, record.dwMDDataType.ToString(NumberFormatInfo.CurrentInfo), record.dwMDIdentifier.ToString(NumberFormatInfo.CurrentInfo)))); } } string[] RecordToStringArray() { Listlist = new List (); if (record.dwMDDataType == MSAdminBase.MULTISZ_METADATA) { // Ensure that the data is an array of double-byte unicode chars. if ((record.dwMDDataLen & 1) != 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new DataMisalignedException(SR.GetString( SR.Hosting_MetabaseDataStringsTerminate, record.dwMDIdentifier.ToString(NumberFormatInfo.CurrentInfo)))); } int startPos = 0; int endPos = 0; while (record.dwMDDataLen > 0) { // Scan for a null terminator. while (endPos < record.dwMDDataLen && Marshal.ReadInt16(record.pbMDData, endPos) != 0) { endPos += 2; } if (endPos == record.dwMDDataLen && Marshal.ReadInt16(record.pbMDData, endPos - 2) != 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new DataMisalignedException(SR.GetString( SR.Hosting_MetabaseDataStringsTerminate, record.dwMDIdentifier.ToString(NumberFormatInfo.CurrentInfo)))); } // End of the string. if (endPos == startPos) { break; } // Convert to string. list.Add(Marshal.PtrToStringUni(new IntPtr(record.pbMDData.ToInt64() + startPos), (endPos - startPos)/2)); // Go to next string startPos = endPos += 2; } } return list.ToArray(); } void Dispose(bool disposing) { if (!disposed) { if (disposing) { record.pbMDData = IntPtr.Zero; currentBufferSize = 0; if (bufferHandle != null) { SafeHGlobalHandleWrapper.CloseHandle(bufferHandle); } } int handleToClose = Interlocked.Exchange(ref mdHandle, 0); if (handleToClose != 0) { adminBase.CloseKey((uint) handleToClose); } //Notice we cannot assign adminBase to NULL in Dispose, because //it could be shared by multiple instances of MetabaseReader. disposed = true; } } static class SafeHGlobalHandleWrapper { [SecurityPermission(SecurityAction.Assert, UnmanagedCode=true)] public static SafeHandle AllocHGlobal(uint cb) { return System.IdentityModel.SafeHGlobalHandle.AllocHGlobal(cb); } [SecurityPermission(SecurityAction.Assert, UnmanagedCode = true)] public static void CloseHandle(SafeHandle handle) { handle.Close(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
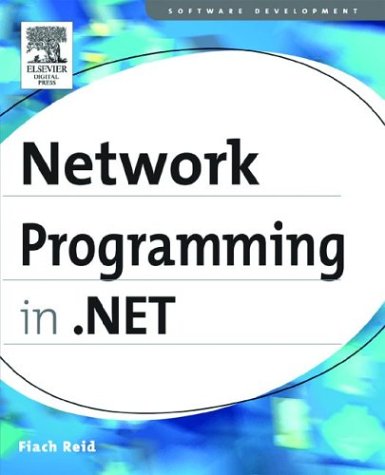
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- counter.cs
- UIPermission.cs
- OdbcDataAdapter.cs
- HitTestParameters.cs
- ConfigXmlDocument.cs
- Vector3DAnimationBase.cs
- CorrelationToken.cs
- InternalConfirm.cs
- ResourceDictionaryCollection.cs
- RawStylusInput.cs
- HtmlInputImage.cs
- StringPropertyBuilder.cs
- SettingsBindableAttribute.cs
- TreeNodeSelectionProcessor.cs
- OrderedDictionary.cs
- DocumentCollection.cs
- ReaderContextStackData.cs
- MaskInputRejectedEventArgs.cs
- PhysicalOps.cs
- ViewStateException.cs
- EventBuilder.cs
- MissingSatelliteAssemblyException.cs
- Speller.cs
- SqlClientMetaDataCollectionNames.cs
- GridViewColumnHeaderAutomationPeer.cs
- ControlCodeDomSerializer.cs
- ResXDataNode.cs
- Regex.cs
- CharacterHit.cs
- MimeMapping.cs
- TdsEnums.cs
- HtmlTable.cs
- ControlHelper.cs
- GroupItemAutomationPeer.cs
- EnumerableRowCollection.cs
- HMACMD5.cs
- MultiDataTrigger.cs
- DrawingBrush.cs
- ObjectItemCollection.cs
- CodeTypeReferenceExpression.cs
- BypassElement.cs
- CachedCompositeFamily.cs
- ArrayWithOffset.cs
- CombinedGeometry.cs
- SiteMapNode.cs
- HwndSource.cs
- TextRangeEditTables.cs
- WebConfigManager.cs
- AssemblyEvidenceFactory.cs
- SchemaTypeEmitter.cs
- CatchBlock.cs
- DateRangeEvent.cs
- Serializer.cs
- WebBrowserHelper.cs
- Keyboard.cs
- PropertyConverter.cs
- BitmapEffectGroup.cs
- AttributeQuery.cs
- FontFamily.cs
- CodeGenerator.cs
- TargetInvocationException.cs
- WindowsListBox.cs
- AccessText.cs
- LazyInitializer.cs
- LambdaCompiler.Generated.cs
- HttpSocketManager.cs
- ConfigurationLockCollection.cs
- GeometryCollection.cs
- UiaCoreApi.cs
- TransformerConfigurationWizardBase.cs
- FileCodeGroup.cs
- MediaTimeline.cs
- TextTreeRootTextBlock.cs
- SourceFilter.cs
- RegularExpressionValidator.cs
- BamlBinaryWriter.cs
- MenuItemBinding.cs
- DoWorkEventArgs.cs
- DynamicObject.cs
- GatewayIPAddressInformationCollection.cs
- NullableBoolConverter.cs
- DataGridViewCellPaintingEventArgs.cs
- PreloadedPackages.cs
- ControlPropertyNameConverter.cs
- TextRunProperties.cs
- ItemsControl.cs
- IsolatedStorageException.cs
- XmlText.cs
- TaiwanCalendar.cs
- TableLayoutSettingsTypeConverter.cs
- ConnectionManagementElement.cs
- ThreadStaticAttribute.cs
- TextParaLineResult.cs
- OneOf.cs
- ApplicationDirectoryMembershipCondition.cs
- ProfileModule.cs
- StylusEventArgs.cs
- AudioFormatConverter.cs
- ListView.cs
- ColorAnimationBase.cs