Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / SMDiagnostics / System / ServiceModel / Diagnostics / PiiTraceSource.cs / 1 / PiiTraceSource.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Diagnostics { using System.Configuration; // using System.ServiceModel.Channels; using System.Diagnostics; using System.ServiceModel.Configuration; class PiiTraceSource : TraceSource { string eventSourceName = String.Empty; internal const string LogPii = "logKnownPii"; bool shouldLogPii = false; bool initialized = false; object localSyncObject = new object(); internal PiiTraceSource(string name, string eventSourceName) : base(name) { #pragma warning disable 618 AssertUtility.DebugAssert(!String.IsNullOrEmpty(eventSourceName), "Event log source name must be valid"); #pragma warning restore 618 this.eventSourceName = eventSourceName; } internal PiiTraceSource(string name, string eventSourceName, SourceLevels levels) : base(name, levels) { #pragma warning disable 618 AssertUtility.DebugAssert(!String.IsNullOrEmpty(eventSourceName), "Event log source name must be valid"); #pragma warning restore 618 this.eventSourceName = eventSourceName; } void Initialize() { if (!this.initialized) { lock (localSyncObject) { if (!this.initialized) { string attributeValue = this.Attributes[PiiTraceSource.LogPii]; bool shouldLogPii = false; if (!string.IsNullOrEmpty(attributeValue)) { if (!bool.TryParse(attributeValue, out shouldLogPii)) { shouldLogPii = false; } } if (shouldLogPii) { #pragma warning disable 618 EventLogger logger = new EventLogger(this.eventSourceName, null); #pragma warning restore 618 if (MachineSettingsSection.EnableLoggingKnownPii) { logger.LogEvent(TraceEventType.Information, EventLogCategory.MessageLogging, EventLogEventId.PiiLoggingOn, false); this.shouldLogPii = true; } else { logger.LogEvent(TraceEventType.Error, EventLogCategory.MessageLogging, EventLogEventId.PiiLoggingNotAllowed, false); } } this.initialized = true; } } } } protected override string[] GetSupportedAttributes() { return new string[] { PiiTraceSource.LogPii }; } internal bool ShouldLogPii { get { // ShouldLogPii is called very frequently, don't call Initialize unless we have to. if (!this.initialized) { Initialize(); } return this.shouldLogPii; } set { // If you call this, you know what you're doing this.initialized = true; this.shouldLogPii = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
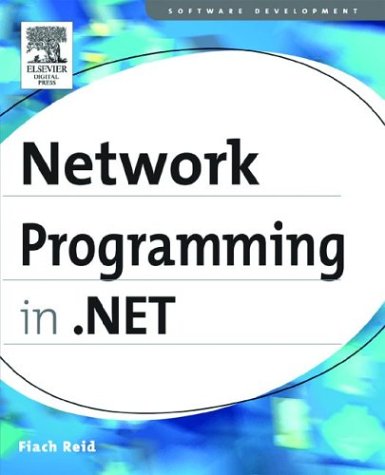
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MenuItemAutomationPeer.cs
- ThreadNeutralSemaphore.cs
- FormatterConverter.cs
- ComponentChangedEvent.cs
- StrokeNodeOperations.cs
- ListViewItem.cs
- StringInfo.cs
- KeyValueConfigurationElement.cs
- KoreanLunisolarCalendar.cs
- CompiledIdentityConstraint.cs
- StylusPointPropertyInfo.cs
- DataGridViewCell.cs
- ReferenceService.cs
- SessionStateItemCollection.cs
- DSASignatureFormatter.cs
- EdmFunction.cs
- Freezable.cs
- EpmCustomContentSerializer.cs
- SyndicationElementExtensionCollection.cs
- DiagnosticsConfiguration.cs
- DynamicArgumentDialog.cs
- FocusTracker.cs
- HtmlInputReset.cs
- DrawingAttributesDefaultValueFactory.cs
- DefaultValueAttribute.cs
- ConsoleTraceListener.cs
- Message.cs
- DiscreteKeyFrames.cs
- FileSystemWatcher.cs
- SaveFileDialog.cs
- IBuiltInEvidence.cs
- CheckableControlBaseAdapter.cs
- AnnotationDocumentPaginator.cs
- WpfWebRequestHelper.cs
- HttpCachePolicyBase.cs
- ControlAdapter.cs
- GenericIdentity.cs
- ReceiveCompletedEventArgs.cs
- Win32SafeHandles.cs
- ProviderIncompatibleException.cs
- PrintController.cs
- XmlQueryTypeFactory.cs
- CatalogPartCollection.cs
- UITypeEditor.cs
- TransactionChannel.cs
- DoubleStorage.cs
- EntityViewGenerator.cs
- CodeMemberProperty.cs
- BulletDecorator.cs
- WorkflowEnvironment.cs
- typedescriptorpermissionattribute.cs
- TextEditorParagraphs.cs
- DataSetUtil.cs
- coordinatorscratchpad.cs
- BulletedListDesigner.cs
- TextLineBreak.cs
- AutomationPropertyInfo.cs
- Missing.cs
- LocatorPart.cs
- CodeSnippetExpression.cs
- DeclarativeCatalogPartDesigner.cs
- KnownTypeHelper.cs
- UidManager.cs
- AcceptorSessionSymmetricMessageSecurityProtocol.cs
- PopupRootAutomationPeer.cs
- DateTimeFormatInfoScanner.cs
- TypeUsage.cs
- StatusBar.cs
- DataGridViewCellValueEventArgs.cs
- DataRelation.cs
- EntityTypeEmitter.cs
- LogicalExpressionTypeConverter.cs
- InputMethodStateTypeInfo.cs
- Timeline.cs
- CalendarDay.cs
- AssemblyAssociatedContentFileAttribute.cs
- DataColumn.cs
- ReaderWriterLockSlim.cs
- FontStretches.cs
- XmlILCommand.cs
- ItemsControlAutomationPeer.cs
- SafeSerializationManager.cs
- GlobalItem.cs
- EdmPropertyAttribute.cs
- StringHelper.cs
- SynchronizationLockException.cs
- BaseInfoTable.cs
- VisualTreeUtils.cs
- FormParameter.cs
- TypeFieldSchema.cs
- XsdBuilder.cs
- ProviderSettings.cs
- OneOf.cs
- CroppedBitmap.cs
- PropertySet.cs
- FileInfo.cs
- ParameterRetriever.cs
- ErrorProvider.cs
- Ops.cs
- RegexWorker.cs