Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / UriTemplatePathSegment.cs / 2 / UriTemplatePathSegment.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System { using System.Collections.Specialized; using System.Diagnostics; using System.ServiceModel.Web; using System.Text; // This represents a Path segment, which can either be a Literal, a Variable or a Compound [DebuggerDisplay("Segment={originalSegment} Nature={nature}")] abstract class UriTemplatePathSegment { readonly bool endsWithSlash; readonly UriTemplatePartType nature; readonly string originalSegment; protected UriTemplatePathSegment(string originalSegment, UriTemplatePartType nature, bool endsWithSlash) { this.originalSegment = originalSegment; this.nature = nature; this.endsWithSlash = endsWithSlash; } public bool EndsWithSlash { get { return this.endsWithSlash; } } public UriTemplatePartType Nature { get { return this.nature; } } public string OriginalSegment { get { return this.originalSegment; } } public static UriTemplatePathSegment CreateFromUriTemplate(string segment, UriTemplate template) { // Identifying the type of segment - Literal|Compound|Variable switch (UriTemplateHelpers.IdentifyPartType(segment)) { case UriTemplatePartType.Literal: return UriTemplateLiteralPathSegment.CreateFromUriTemplate(segment, template); case UriTemplatePartType.Compound: return UriTemplateCompoundPathSegment.CreateFromUriTemplate(segment, template); case UriTemplatePartType.Variable: if (segment.EndsWith("/", StringComparison.Ordinal)) { string varName = template.AddPathVariable(UriTemplatePartType.Variable, segment.Substring(1, segment.Length - 3)); return new UriTemplateVariablePathSegment(segment, true, varName); } else { string varName = template.AddPathVariable(UriTemplatePartType.Variable, segment.Substring(1, segment.Length - 2)); return new UriTemplateVariablePathSegment(segment, false, varName); } default: Fx.Assert("Invalid value from IdentifyStringNature"); return null; } } public abstract void Bind(string[] values, ref int valueIndex, StringBuilder path); public abstract bool IsEquivalentTo(UriTemplatePathSegment other, bool ignoreTrailingSlash); public bool IsMatch(UriTemplateLiteralPathSegment segment) { return IsMatch(segment, false); } public abstract bool IsMatch(UriTemplateLiteralPathSegment segment, bool ignoreTrailingSlash); public abstract void Lookup(string segment, NameValueCollection boundParameters); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
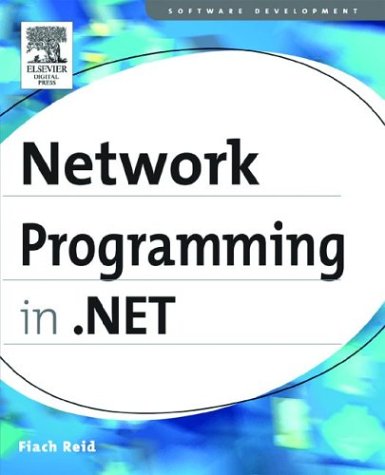
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TraceContext.cs
- ItemAutomationPeer.cs
- AppDomainAttributes.cs
- DESCryptoServiceProvider.cs
- InvalidPropValue.cs
- InfiniteIntConverter.cs
- Stroke2.cs
- CodeMethodInvokeExpression.cs
- Parameter.cs
- BindingsCollection.cs
- RSAPKCS1KeyExchangeFormatter.cs
- SqlProfileProvider.cs
- GroupLabel.cs
- NullableIntMinMaxAggregationOperator.cs
- DataSourceNameHandler.cs
- DataGridViewDataConnection.cs
- DrawingImage.cs
- recordstatescratchpad.cs
- PersistNameAttribute.cs
- DataBindingValueUIHandler.cs
- CustomErrorCollection.cs
- ReturnType.cs
- ComboBoxItem.cs
- Unit.cs
- TypeUtils.cs
- InvalidPrinterException.cs
- TileBrush.cs
- GroupDescription.cs
- CompiledQueryCacheKey.cs
- TextFormatter.cs
- RuntimeHelpers.cs
- ConnectionInterfaceCollection.cs
- HttpRequest.cs
- StringSource.cs
- IntersectQueryOperator.cs
- Misc.cs
- DiscardableAttribute.cs
- DecimalFormatter.cs
- InternalConfigEventArgs.cs
- Matrix3DStack.cs
- DefinitionUpdate.cs
- Inline.cs
- ProgressBar.cs
- ListItemParagraph.cs
- Timeline.cs
- SingleObjectCollection.cs
- DecimalSumAggregationOperator.cs
- TextDecorationCollection.cs
- FixedBufferAttribute.cs
- BasicHttpMessageSecurityElement.cs
- SimpleTextLine.cs
- PropertyRecord.cs
- TextWriter.cs
- HwndSubclass.cs
- ElementProxy.cs
- Aes.cs
- UserPreferenceChangingEventArgs.cs
- DefaultHttpHandler.cs
- HttpClientCertificate.cs
- Emitter.cs
- MsmqIntegrationProcessProtocolHandler.cs
- ZipIOModeEnforcingStream.cs
- FunctionQuery.cs
- BamlBinaryReader.cs
- WmlPageAdapter.cs
- MenuEventArgs.cs
- WebHttpElement.cs
- HttpCacheVaryByContentEncodings.cs
- JsonMessageEncoderFactory.cs
- DBAsyncResult.cs
- TraceHandler.cs
- LayoutManager.cs
- ChtmlTextWriter.cs
- WebRequestModulesSection.cs
- IPCCacheManager.cs
- ListSortDescription.cs
- PropertyOverridesTypeEditor.cs
- __Filters.cs
- FontConverter.cs
- CodeTypeMemberCollection.cs
- HttpClientCertificate.cs
- SystemDiagnosticsSection.cs
- SByte.cs
- InheritanceAttribute.cs
- EventSource.cs
- IteratorFilter.cs
- SystemInformation.cs
- BufferedWebEventProvider.cs
- MergeFilterQuery.cs
- NativeMethods.cs
- CustomAttributeFormatException.cs
- ProviderConnectionPoint.cs
- SerialStream.cs
- PageContent.cs
- NameValueConfigurationCollection.cs
- CodeDOMUtility.cs
- ObjectIDGenerator.cs
- DataGridViewRowConverter.cs
- LogicalExpr.cs
- ErasingStroke.cs