Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / Configuration / BrowserCapabilitiesFactory.cs / 5 / BrowserCapabilitiesFactory.cs
//------------------------------------------------------------------------------ //// This code was generated by a tool. // Runtime Version:2.0.50804.0 // // Changes to this file may cause incorrect behavior and will be lost if // the code is regenerated. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Web; using System.Web.Configuration; using System.Reflection; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal)] public class BrowserCapabilitiesFactory : System.Web.Configuration.BrowserCapabilitiesFactoryBase { public override void ConfigureBrowserCapabilities(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { this.DefaultProcess(headers, browserCaps); if ((this.IsBrowserUnknown(browserCaps) == false)) { return; } this.DefaultDefaultProcess(headers, browserCaps); } protected virtual void IeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void IeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool IeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Mozilla[^(]*\\([C|c]ompatible;\\s*MSIE (?\'version\'(?\'major\'\\d+)(?\'minor\'\\.\\d+)(?\'l" + "etters\'\\w*))(?\'extra\'[^)]*)"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "Opera|Go\\.Web|Windows CE|EudoraWeb"); if ((result == true)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "IE"; dictionary["extra"] = regexWorker["${extra}"]; dictionary["isColor"] = "true"; dictionary["letters"] = regexWorker["${letters}"]; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["screenBitDepth"] = "8"; dictionary["type"] = regexWorker["IE${major}"]; dictionary["version"] = regexWorker["${version}"]; browserCaps.AddBrowser("IE"); this.IeProcessGateways(headers, browserCaps); // gateway, parent=IE this.IeaolProcess(headers, browserCaps); // gateway, parent=IE this.IebetaProcess(headers, browserCaps); // gateway, parent=IE this.IeupdateProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=IE if (this.Ie5to9Process(headers, browserCaps)) { } else { if (this.Ie4Process(headers, browserCaps)) { } else { if (this.Ie3Process(headers, browserCaps)) { } else { if (this.Ie2Process(headers, browserCaps)) { } else { if (this.Ie1minor5Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } this.IeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie5to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie5to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie5to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^[5-9]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["activexcontrols"] = "true"; dictionary["backgroundsounds"] = "true"; dictionary["cookies"] = "true"; dictionary["css1"] = "true"; dictionary["css2"] = "true"; dictionary["ecmascriptversion"] = "1.2"; dictionary["frames"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["jscriptversion"] = "5.0"; dictionary["msdomversion"] = regexWorker["${majorversion}${minorversion}"]; dictionary["supportsCallback"] = "true"; dictionary["supportsFileUpload"] = "true"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["supportsMaintainScrollPositionOnPostback"] = "true"; dictionary["supportsVCard"] = "true"; dictionary["supportsXmlHttp"] = "true"; dictionary["tables"] = "true"; dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["vbscript"] = "true"; dictionary["w3cdomversion"] = "1.0"; dictionary["xml"] = "true"; browserCaps.AddBrowser("IE5to9"); this.Ie5to9ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=IE5to9 if (this.Ie6to9Process(headers, browserCaps)) { } else { if (this.Ie5Process(headers, browserCaps)) { } else { if (this.Ie5to9macProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.Ie5to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie6to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie6to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie6to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "[6-9]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["jscriptversion"] = "5.6"; dictionary["ExchangeOmaSupported"] = "true"; browserCaps.AddBrowser("IE6to9"); this.Ie6to9ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=IE6to9 if (this.Treo600Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Ie6to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Treo600ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Treo600ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Treo600Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "PalmSource; Blazer"); if ((result == false)) { return false; } // Capture: header values regexWorker.ProcessRegex(((string)(browserCaps[string.Empty])), "PalmSource; Blazer 3\\.0\\)\\s\\d+;(?\'screenPixelsHeight\'\\d+)x(?\'screenPixelsWidth\'\\d" + "+)$"); // Capabilities: set capabilities dictionary["browser"] = "Blazer 3.0"; dictionary["cachesAllResponsesWithExpires"] = "false"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canRenderEmptySelects"] = "true"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["ecmascriptversion"] = "1.1"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "false"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["javascript"] = "true"; dictionary["jscriptversion"] = "0.0"; dictionary["maximumHrefLength"] = "10000"; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["mobileDeviceManufacturer"] = ""; dictionary["mobileDeviceModel"] = ""; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "html32"; dictionary["preferredRequestEncoding"] = "utf-8"; dictionary["preferredResponseEncoding"] = "utf-8"; dictionary["rendersBreaksAfterHtmlLists"] = "true"; dictionary["requiredMetaTagNameValue"] = "PalmComputingPlatform"; dictionary["requiresAttributeColonSubstitution"] = "false"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["requiresControlStateInSession"] = "false"; dictionary["requiresDBCSCharacter"] = "false"; dictionary["requiresFullyQualifiedRedirectUrl"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["requiresLeadingPageBreak"] = "false"; dictionary["requiresNoBreakInFormatting"] = "false"; dictionary["requiresOutputOptimization"] = "false"; dictionary["requiresPostRedirectionHandling"] = "false"; dictionary["requiresPragmaNoCacheHeader"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["requiresUniqueHtmlCheckboxNames"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "13"; dictionary["screenCharactersWidth"] = "32"; dictionary["screenPixelsHeight"] = regexWorker["${screenPixelsHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenPixelsWidth}"]; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyColor"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCharacterEntityEncoding"] = "true"; dictionary["supportsCss"] = "false"; dictionary["supportsDivAlign"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsEmptyStringInCookieValue"] = "true"; dictionary["supportsFileUpload"] = "false"; dictionary["supportsFontColor"] = "true"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsIModeSymbols"] = "false"; dictionary["supportsInputIStyle"] = "false"; dictionary["supportsInputMode"] = "false"; dictionary["supportsItalic"] = "true"; dictionary["supportsJPhoneMultiMediaAttributes"] = "false"; dictionary["supportsJPhoneSymbols"] = "false"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["supportsQueryStringInFormAction"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectMultiple"] = "true"; dictionary["supportsUncheck"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "Handspring Treo 600"; browserCaps.AddBrowser("Treo600"); this.Treo600ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Treo600ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^5$"); if ((result == false)) { return false; } // Capabilities: set capabilities browserCaps.AddBrowser("IE5"); this.Ie5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=IE5 if (this.Ie50Process(headers, browserCaps)) { } else { if (this.Ie55Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.Ie5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie50ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie50ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie50Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["minorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^\\.0"); if ((result == false)) { return false; } // Capabilities: set capabilities browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("IE50"); this.Ie50ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie50ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie55ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie55ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie55Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["minorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^\\.5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["jscriptversion"] = "5.5"; dictionary["ExchangeOmaSupported"] = "true"; browserCaps.AddBrowser("IE55"); this.Ie55ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie55ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie5to9macProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie5to9macProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie5to9macProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["platform"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(MacPPC|Mac68K)"); if ((result == false)) { return false; } // Capabilities: set capabilities browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("IE5to9Mac"); this.Ie5to9macProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie5to9macProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MSIE 4"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["activexcontrols"] = "true"; dictionary["backgroundsounds"] = "true"; dictionary["cdf"] = "true"; dictionary["cookies"] = "true"; dictionary["css1"] = "true"; dictionary["ecmascriptversion"] = "1.2"; dictionary["frames"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["jscriptversion"] = "3.0"; dictionary["msdomversion"] = "4.0"; dictionary["supportsFileUpload"] = "true"; dictionary["supportsMultilineTextBoxDisplay"] = "false"; dictionary["supportsMaintainScrollPositionOnPostback"] = "true"; dictionary["tables"] = "true"; dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["vbscript"] = "true"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("IE4"); this.Ie4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie3ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie3ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie3Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^3"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["activexcontrols"] = "true"; dictionary["backgroundsounds"] = "true"; dictionary["cookies"] = "true"; dictionary["css1"] = "true"; dictionary["ecmascriptversion"] = "1.0"; dictionary["frames"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["jscriptversion"] = "1.0"; dictionary["supportsMultilineTextBoxDisplay"] = "false"; dictionary["tables"] = "true"; dictionary["vbscript"] = "true"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("IE3"); this.Ie3ProcessGateways(headers, browserCaps); // gateway, parent=IE3 this.Ie3akProcess(headers, browserCaps); // gateway, parent=IE3 this.Ie3skProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=IE3 if (this.Ie3win16Process(headers, browserCaps)) { } else { if (this.Ie3macProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.Ie3ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie3win16ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie3win16ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie3win16Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "16bit|Win(dows 3\\.1|16)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["activexcontrols"] = "false"; dictionary["javaapplets"] = "false"; browserCaps.AddBrowser("IE3win16"); this.Ie3win16ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=IE3win16 if (this.Ie3win16aProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Ie3win16ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie3win16aProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie3win16aProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie3win16aProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["extra"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^a"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; dictionary["javascript"] = "false"; dictionary["vbscript"] = "false"; browserCaps.AddBrowser("IE3win16a"); this.Ie3win16aProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie3win16aProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie3macProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie3macProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie3macProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "PPC Mac|Macintosh.*(68K|PPC)|Mac_(PowerPC|PPC|68(K|000))"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["activexcontrols"] = "false"; dictionary["vbscript"] = "false"; browserCaps.AddBrowser("IE3Mac"); this.Ie3macProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie3macProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie3akProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie3akProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie3akProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["extra"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "; AK;"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ak"] = "true"; this.Ie3akProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie3akProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie3skProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie3skProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie3skProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["extra"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "; SK;"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["sk"] = "true"; this.Ie3skProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie3skProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["backgroundsounds"] = "true"; dictionary["cookies"] = "true"; dictionary["tables"] = "true"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("IE2"); this.Ie2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=IE2 if (this.WebtvProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Ie2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie1minor5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie1minor5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie1minor5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^1\\.5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "true"; dictionary["tables"] = "true"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("IE1minor5"); this.Ie1minor5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie1minor5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void IeaolProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void IeaolProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool IeaolProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["extra"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "; AOL"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["aol"] = "true"; dictionary["frames"] = "true"; this.IeaolProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.IeaolProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void IebetaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void IebetaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool IebetaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["letters"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^([bB]|ab)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; this.IebetaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.IebetaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void IeupdateProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void IeupdateProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool IeupdateProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["extra"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "; Update a;"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["authenticodeupdate"] = "true"; this.IeupdateProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.IeupdateProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MozillaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MozillaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MozillaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Mozilla"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "MME|Opera"); if ((result == true)) { return false; } // Capture: header values regexWorker.ProcessRegex(((string)(browserCaps[string.Empty])), "Mozilla/(?\'version\'(?\'major\'\\d+)(?\'minor\'\\.\\d+)\\w*)"); regexWorker.ProcessRegex(((string)(browserCaps[string.Empty])), " (?\'screenWidth\'\\d*)x(?\'screenHeight\'\\d*)"); // Capabilities: set capabilities dictionary["browser"] = "Mozilla"; dictionary["cookies"] = "false"; dictionary["defaultScreenCharactersHeight"] = "40"; dictionary["defaultScreenCharactersWidth"] = "80"; dictionary["defaultScreenPixelsHeight"] = "480"; dictionary["defaultScreenPixelsWidth"] = "640"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "false"; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["screenBitDepth"] = "8"; dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsDivNoWrap"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["type"] = "Mozilla"; dictionary["version"] = regexWorker["${version}"]; browserCaps.AddBrowser("Mozilla"); this.MozillaProcessGateways(headers, browserCaps); // gateway, parent=Mozilla this.MozillabetaProcess(headers, browserCaps); // gateway, parent=Mozilla this.MozillagoldProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Mozilla if (this.IeProcess(headers, browserCaps)) { } else { if (this.PowerbrowserProcess(headers, browserCaps)) { } else { if (this.GeckoProcess(headers, browserCaps)) { } else { if (this.AvantgoProcess(headers, browserCaps)) { } else { if (this.GoamericaProcess(headers, browserCaps)) { } else { if (this.Netscape3Process(headers, browserCaps)) { } else { if (this.Netscape4Process(headers, browserCaps)) { } else { if (this.MypalmProcess(headers, browserCaps)) { } else { if (this.EudorawebProcess(headers, browserCaps)) { } else { if (this.WinceProcess(headers, browserCaps)) { } else { if (this.MspieProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } } } this.MozillaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MozillabetaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MozillabetaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MozillabetaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Mozilla/\\d+\\.\\d+b"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; this.MozillabetaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MozillabetaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MozillagoldProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MozillagoldProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MozillagoldProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Mozilla/\\d+\\.\\d+\\w*Gold"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["gold"] = "true"; this.MozillagoldProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MozillagoldProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PowerbrowserProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PowerbrowserProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PowerbrowserProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Mozilla/2\\.01 \\(Compatible\\) Oracle\\(tm\\) PowerBrowser\\(tm\\)/1\\.0a"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "PowerBrowser"; dictionary["cookies"] = "true"; dictionary["ecmascriptversion"] = "1.0"; dictionary["frames"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["majorversion"] = "1"; dictionary["minorversion"] = ".5"; dictionary["platform"] = "Win95"; dictionary["tables"] = "true"; dictionary["vbscript"] = "true"; dictionary["version"] = "1.5"; dictionary["type"] = "PowerBrowser1"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("PowerBrowser"); this.PowerbrowserProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PowerbrowserProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GeckoProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GeckoProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GeckoProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Gecko"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Mozilla"; dictionary["css1"] = "true"; dictionary["css2"] = "true"; dictionary["cookies"] = "true"; dictionary["ecmascriptversion"] = "1.5"; dictionary["frames"] = "true"; dictionary["isColor"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["maximumRenderedPageSize"] = "20000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["screenBitDepth"] = "32"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFileUpload"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "desktop"; dictionary["version"] = regexWorker["${version}"]; dictionary["w3cdomversion"] = "1.0"; browserCaps.AddBrowser("Gecko"); this.GeckoProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Gecko if (this.MozillarvProcess(headers, browserCaps)) { } else { if (this.SafariProcess(headers, browserCaps)) { } else { if (this.Netscape5Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.GeckoProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MozillarvProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MozillarvProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MozillarvProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "rv\\:(?\'version\'(?\'major\'\\d+)(?\'minor\'\\.[.\\d]*))"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "Netscape"); if ((result == true)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.4"; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["preferredImageMime"] = "image/gif"; dictionary["supportsCallback"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsMaintainScrollPositionOnPostback"] = "true"; dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["type"] = regexWorker["Mozilla${major}"]; dictionary["version"] = regexWorker["${version}"]; dictionary["w3cdomversion"] = "1.0"; browserCaps.AddBrowser("MozillaRV"); this.MozillarvProcessGateways(headers, browserCaps); // gateway, parent=MozillaRV this.Mozillav14plusProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=MozillaRV if (this.MozillafirebirdProcess(headers, browserCaps)) { } else { if (this.MozillafirefoxProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.MozillarvProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mozillav14plusProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mozillav14plusProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mozillav14plusProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^1$"); if ((result == false)) { return false; } headerValue = ((string)(dictionary["minorversion"])); result = regexWorker.ProcessRegex(headerValue, "^\\.[4-9]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["backgroundsounds"] = "true"; dictionary["css1"] = "true"; dictionary["css2"] = "true"; dictionary["javaapplets"] = "true"; dictionary["maximumRenderedPageSize"] = "2000000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["screenBitDepth"] = "32"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["type"] = regexWorker["Mozilla${version}"]; dictionary["xml"] = "true"; this.Mozillav14plusProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mozillav14plusProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MozillafirebirdProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MozillafirebirdProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MozillafirebirdProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Gecko\\/\\d+ Firebird\\/(?\'version\'(?\'major\'\\d+)(?\'minor\'\\.[.\\d]*))"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Firebird"; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["version"] = regexWorker["${version}"]; dictionary["type"] = regexWorker["Firebird${version}"]; browserCaps.AddBrowser("MozillaFirebird"); this.MozillafirebirdProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MozillafirebirdProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MozillafirefoxProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MozillafirefoxProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MozillafirefoxProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Gecko\\/\\d+ Firefox\\/(?\'version\'(?\'major\'\\d+)(?\'minor\'\\.[.\\d]*))"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Firefox"; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["version"] = regexWorker["${version}"]; dictionary["type"] = regexWorker["Firefox${version}"]; browserCaps.AddBrowser("MozillaFirefox"); this.MozillafirefoxProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MozillafirefoxProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SafariProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SafariProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SafariProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "AppleWebKit/(?\'webversion\'\\d+)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["appleWebTechnologyVersion"] = regexWorker["${webversion}"]; dictionary["backgroundsounds"] = "true"; dictionary["browser"] = "AppleMAC-Safari"; dictionary["css1"] = "true"; dictionary["css2"] = "true"; dictionary["ecmascriptversion"] = "0.0"; dictionary["futureBrowser"] = "Apple Safari"; dictionary["screenBitDepth"] = "24"; dictionary["tables"] = "true"; dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["type"] = "Desktop"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("Safari"); this.SafariProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Safari if (this.Safari60Process(headers, browserCaps)) { } else { if (this.Safari85Process(headers, browserCaps)) { } else { if (this.Safari1plusProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.SafariProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Safari60ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Safari60ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Safari60Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["appleWebTechnologyVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "60"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.0"; browserCaps.AddBrowser("Safari60"); this.Safari60ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Safari60ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Safari85ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Safari85ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Safari85Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["appleWebTechnologyVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "85"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.4"; browserCaps.AddBrowser("Safari85"); this.Safari85ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Safari85ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Safari1plusProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Safari1plusProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Safari1plusProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["appleWebTechnologyVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "\\d\\d\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.4"; dictionary["w3cdomversion"] = "1.0"; dictionary["supportsCallback"] = "true"; browserCaps.AddBrowser("Safari1Plus"); this.Safari1plusProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Safari1plusProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void AvantgoProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void AvantgoProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool AvantgoProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "\\(compatible; AvantGo .*\\)"); if ((result == false)) { return false; } // Capture: header values regexWorker.ProcessRegex(((string)(headers["X-AVANTGO-VERSION"])), "(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*)"); // Capabilities: set capabilities dictionary["browser"] = "AvantGo"; dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "true"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "12"; dictionary["defaultScreenPixelsHeight"] = "72"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["javascript"] = "false"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "2560"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiredMetaTagNameValue"] = "HandheldFriendly"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["requiresUniqueHtmlCheckboxNames"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "13"; dictionary["screenCharactersWidth"] = "30"; dictionary["screenPixelsHeight"] = "150"; dictionary["screenPixelsWidth"] = "150"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "false"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "false"; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["AvantGo${browserMajorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("AvantGo"); this.AvantgoProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=AvantGo if (this.TmobilesidekickProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.AvantgoProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void TmobilesidekickProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void TmobilesidekickProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool TmobilesidekickProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Danger hiptop"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["css1"] = "true"; dictionary["ecmaScriptVersion"] = "1.3"; dictionary["frames"] = "true"; dictionary["inputType"] = "telephoneKeypad"; dictionary["javaapplets"] = "true"; dictionary["majorVersion"] = "5"; dictionary["maximumRenderedPageSize"] = "7000"; dictionary["minorVersion"] = ".0"; dictionary["mobileDeviceManufacturer"] = "T-Mobile"; dictionary["mobileDeviceModel"] = "SideKick"; dictionary["preferredRenderingType"] = "html32"; dictionary["requiresLeadingPageBreak"] = "false"; dictionary["requiresUniqueHtmlCheckboxNames"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "11"; dictionary["screenCharactersWidth"] = "57"; dictionary["screenPixelsHeight"] = "136"; dictionary["screenPixelsWidth"] = "236"; dictionary["supportsCss"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["type"] = "AvantGo 3"; dictionary["version"] = "5.0"; browserCaps.AddBrowser("TMobileSidekick"); this.TmobilesidekickProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.TmobilesidekickProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void CasiopeiaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void CasiopeiaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool CasiopeiaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "CASSIOPEIA BE"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "CASSIOPEIA"; dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["cookies"] = "true"; dictionary["css1"] = "false"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "12"; dictionary["defaultScreenPixelsHeight"] = "72"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["ecmascriptversion"] = "1.3"; dictionary["frames"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isMobileDevice"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["mobileDeviceManufacturer"] = "Casio"; dictionary["mobileDeviceModel"] = "Casio BE-500"; dictionary["preferredImageMime"] = "image/gif"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresContentTypeMetaTag"] = "true"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["requiresNoBreakInFormatting"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "50"; dictionary["screenCharactersWidth"] = "38"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "240"; dictionary["supportsBold"] = "true"; dictionary["supportsCharacterEntityEncoding"] = "false"; dictionary["supportsCss"] = "false"; dictionary["supportsDivNoWrap"] = "true"; dictionary["supportsFileUpload"] = "false"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "false"; dictionary["supportsItalic"] = "false"; dictionary["tables"] = "true"; dictionary["type"] = "CASSIOPEIA"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("Casiopeia"); this.CasiopeiaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.CasiopeiaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void DefaultProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void DefaultProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool DefaultProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Capabilities: set capabilities dictionary["activexcontrols"] = "false"; dictionary["ak"] = "false"; dictionary["aol"] = "false"; dictionary["authenticodeupdate"] = "false"; dictionary["backgroundsounds"] = "false"; dictionary["beta"] = "false"; dictionary["browser"] = "Unknown"; dictionary["cachesAllResponsesWithExpires"] = "false"; dictionary["canCombineFormsInDeck"] = "true"; dictionary["canInitiateVoiceCall"] = "false"; dictionary["canRenderAfterInputOrSelectElement"] = "true"; dictionary["canRenderEmptySelects"] = "true"; dictionary["canRenderInputAndSelectElementsTogether"] = "true"; dictionary["canRenderMixedSelects"] = "true"; dictionary["canRenderOneventAndPrevElementsTogether"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["canRenderSetvarZeroWithMultiSelectionList"] = "true"; dictionary["canSendMail"] = "true"; dictionary["cdf"] = "false"; dictionary["cookies"] = "true"; dictionary["crawler"] = "false"; dictionary["css1"] = "false"; dictionary["css2"] = "false"; dictionary["defaultCharacterHeight"] = "12"; dictionary["defaultCharacterWidth"] = "8"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "12"; dictionary["defaultScreenPixelsHeight"] = "72"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["defaultSubmitButtonLimit"] = "1"; dictionary["ecmascriptversion"] = "0.0"; dictionary["frames"] = "false"; dictionary["gatewayMajorVersion"] = "0"; dictionary["gatewayMinorVersion"] = "0"; dictionary["gatewayVersion"] = "None"; dictionary["gold"] = "false"; dictionary["hasBackButton"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "false"; dictionary["inputType"] = "telephoneKeypad"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "false"; dictionary["javaapplets"] = "false"; dictionary["javascript"] = "false"; dictionary["jscriptversion"] = "0.0"; dictionary["majorversion"] = "0"; dictionary["maximumHrefLength"] = "10000"; dictionary["maximumRenderedPageSize"] = "2000"; dictionary["maximumSoftkeyLabelLength"] = "5"; dictionary["minorversion"] = "0"; dictionary["mobileDeviceManufacturer"] = "Unknown"; dictionary["mobileDeviceModel"] = "Unknown"; dictionary["msdomversion"] = "0.0"; dictionary["numberOfSoftkeys"] = "0"; dictionary["platform"] = "Unknown"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "html32"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["rendersBreaksAfterHtmlLists"] = "true"; dictionary["rendersBreaksAfterWmlAnchor"] = "false"; dictionary["rendersBreaksAfterWmlInput"] = "false"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["rendersWmlSelectsAsMenuCards"] = "false"; dictionary["requiredMetaTagNameValue"] = ""; dictionary["requiresAdaptiveErrorReporting"] = "false"; dictionary["requiresAttributeColonSubstitution"] = "false"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["requiresDBCSCharacter"] = "false"; dictionary["requiresFullyQualifiedRedirectUrl"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["requiresLeadingPageBreak"] = "false"; dictionary["requiresNoBreakInFormatting"] = "false"; dictionary["requiresNoescapedPostUrl"] = "true"; dictionary["requiresNoSoftkeyLabels"] = "false"; dictionary["requiresOutputOptimization"] = "false"; dictionary["requiresPhoneNumbersAsPlainText"] = "false"; dictionary["requiresPostRedirectionHandling"] = "false"; dictionary["requiresSpecialViewStateEncoding"] = "false"; dictionary["requiresUniqueFilePathSuffix"] = "false"; dictionary["requiresUniqueHtmlCheckboxNames"] = "false"; dictionary["requiresUniqueHtmlInputNames"] = "false"; dictionary["requiresUrlEncodedPostfieldValues"] = "false"; dictionary["screenBitDepth"] = "1"; dictionary["sk"] = "false"; dictionary["supportsAccesskeyAttribute"] = "false"; dictionary["supportsBodyColor"] = "true"; dictionary["supportsBold"] = "false"; dictionary["supportsCacheControlMetaTag"] = "true"; dictionary["supportsCharacterEntityEncoding"] = "true"; dictionary["supportsCss"] = "false"; dictionary["supportsDivAlign"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsEmptyStringInCookieValue"] = "true"; dictionary["supportsFileUpload"] = "false"; dictionary["supportsFontColor"] = "true"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["supportsImageSubmit"] = "false"; dictionary["supportsIModeSymbols"] = "false"; dictionary["supportsInputIStyle"] = "false"; dictionary["supportsInputMode"] = "false"; dictionary["supportsItalic"] = "false"; dictionary["supportsJPhoneMultiMediaAttributes"] = "false"; dictionary["supportsJPhoneSymbols"] = "false"; dictionary["supportsMaintainScrollPositionOnPostback"] = "false"; dictionary["supportsMultilineTextBoxDisplay"] = "false"; dictionary["supportsQueryStringInFormAction"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectMultiple"] = "true"; dictionary["supportsUncheck"] = "true"; dictionary["supportsVCard"] = "false"; dictionary["tables"] = "false"; dictionary["tagwriter"] = "System.Web.UI.Html32TextWriter"; dictionary["type"] = "Unknown"; dictionary["vbscript"] = "false"; dictionary["version"] = "0.0"; dictionary["w3cdomversion"] = "0.0"; dictionary["win16"] = "false"; dictionary["win32"] = "false"; dictionary["xml"] = "false"; browserCaps.AddBrowser("Default"); this.DefaultProcessGateways(headers, browserCaps); // gateway, parent=Default this.NokiagatewayProcess(headers, browserCaps); // gateway, parent=Default this.UpgatewayProcess(headers, browserCaps); // gateway, parent=Default this.CrawlerProcess(headers, browserCaps); // gateway, parent=Default this.ColorProcess(headers, browserCaps); // gateway, parent=Default this.MonoProcess(headers, browserCaps); // gateway, parent=Default this.PixelsProcess(headers, browserCaps); // gateway, parent=Default this.VoiceProcess(headers, browserCaps); // gateway, parent=Default this.CharsetProcess(headers, browserCaps); // gateway, parent=Default this.PlatformProcess(headers, browserCaps); // gateway, parent=Default this.WinProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Default if (this.MozillaProcess(headers, browserCaps)) { } else { if (this.DocomoProcess(headers, browserCaps)) { } else { if (this.Ericssonr380Process(headers, browserCaps)) { } else { if (this.EricssonProcess(headers, browserCaps)) { } else { if (this.EzwapProcess(headers, browserCaps)) { } else { if (this.GenericdownlevelProcess(headers, browserCaps)) { } else { if (this.JataayuProcess(headers, browserCaps)) { } else { if (this.JphoneProcess(headers, browserCaps)) { } else { if (this.LegendProcess(headers, browserCaps)) { } else { if (this.MmeProcess(headers, browserCaps)) { } else { if (this.NokiaProcess(headers, browserCaps)) { } else { if (this.NokiamobilebrowserrainbowProcess(headers, browserCaps)) { } else { if (this.Nokiaepoc32wtlProcess(headers, browserCaps)) { } else { if (this.UpProcess(headers, browserCaps)) { } else { if (this.OperaProcess(headers, browserCaps)) { } else { if (this.PalmscapeProcess(headers, browserCaps)) { } else { if (this.AuspalmProcess(headers, browserCaps)) { } else { if (this.SharppdaProcess(headers, browserCaps)) { } else { if (this.PanasonicProcess(headers, browserCaps)) { } else { if (this.Mspie06Process(headers, browserCaps)) { } else { if (this.SktdevicesProcess(headers, browserCaps)) { } else { if (this.WinwapProcess(headers, browserCaps)) { } else { if (this.XiinoProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } } } } } } } } } } } } } } } this.DefaultProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void DocomoProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void DocomoProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool DocomoProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^DoCoMo/"); if ((result == false)) { return false; } // Capture: header values regexWorker.ProcessRegex(((string)(browserCaps[string.Empty])), "^DoCoMo/(?\'httpVersion\'[^/ ]*)[/ ](?\'deviceID\'[^/\\x28]*)"); // Capabilities: set capabilities dictionary["browser"] = "i-mode"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["cookies"] = "false"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "16"; dictionary["defaultScreenPixelsHeight"] = "70"; dictionary["defaultScreenPixelsWidth"] = "90"; dictionary["deviceID"] = regexWorker["${deviceID}"]; dictionary["inputType"] = "telephoneKeypad"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["javaapplets"] = "false"; dictionary["javascript"] = "false"; dictionary["maximumHrefLength"] = "524"; dictionary["maximumRenderedPageSize"] = "5120"; dictionary["mobileDeviceModel"] = regexWorker["${deviceID}"]; dictionary["optimumPageWeight"] = "700"; dictionary["preferredRenderingType"] = "chtml10"; dictionary["preferredRequestEncoding"] = "shift_jis"; dictionary["preferredResponseEncoding"] = "shift_jis"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresFullyQualifiedRedirectUrl"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOutputOptimization"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["supportsAccesskeyAttribute"] = "true"; dictionary["supportsCharacterEntityEncoding"] = "false"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsIModeSymbols"] = "true"; dictionary["supportsInputIStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["tables"] = "false"; dictionary["type"] = "i-mode"; dictionary["vbscript"] = "false"; browserCaps.HtmlTextWriter = "System.Web.UI.ChtmlTextWriter"; browserCaps.AddBrowser("Docomo"); this.DocomoProcessGateways(headers, browserCaps); // gateway, parent=Docomo this.DocomorenderingsizeProcess(headers, browserCaps); // gateway, parent=Docomo this.DocomodefaultrenderingsizeProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Docomo if (this.Docomosh251iProcess(headers, browserCaps)) { } else { if (this.Docomon251iProcess(headers, browserCaps)) { } else { if (this.Docomop211iProcess(headers, browserCaps)) { } else { if (this.Docomof212iProcess(headers, browserCaps)) { } else { if (this.Docomod501iProcess(headers, browserCaps)) { } else { if (this.Docomof501iProcess(headers, browserCaps)) { } else { if (this.Docomon501iProcess(headers, browserCaps)) { } else { if (this.Docomop501iProcess(headers, browserCaps)) { } else { if (this.Docomod502iProcess(headers, browserCaps)) { } else { if (this.Docomof502iProcess(headers, browserCaps)) { } else { if (this.Docomon502iProcess(headers, browserCaps)) { } else { if (this.Docomop502iProcess(headers, browserCaps)) { } else { if (this.Docomonm502iProcess(headers, browserCaps)) { } else { if (this.Docomoso502iProcess(headers, browserCaps)) { } else { if (this.Docomof502itProcess(headers, browserCaps)) { } else { if (this.Docomon502itProcess(headers, browserCaps)) { } else { if (this.Docomoso502iwmProcess(headers, browserCaps)) { } else { if (this.Docomof504iProcess(headers, browserCaps)) { } else { if (this.Docomon504iProcess(headers, browserCaps)) { } else { if (this.Docomop504iProcess(headers, browserCaps)) { } else { if (this.Docomon821iProcess(headers, browserCaps)) { } else { if (this.Docomop821iProcess(headers, browserCaps)) { } else { if (this.Docomod209iProcess(headers, browserCaps)) { } else { if (this.Docomoer209iProcess(headers, browserCaps)) { } else { if (this.Docomof209iProcess(headers, browserCaps)) { } else { if (this.Docomoko209iProcess(headers, browserCaps)) { } else { if (this.Docomon209iProcess(headers, browserCaps)) { } else { if (this.Docomop209iProcess(headers, browserCaps)) { } else { if (this.Docomop209isProcess(headers, browserCaps)) { } else { if (this.Docomor209iProcess(headers, browserCaps)) { } else { if (this.Docomor691iProcess(headers, browserCaps)) { } else { if (this.Docomof503iProcess(headers, browserCaps)) { } else { if (this.Docomof503isProcess(headers, browserCaps)) { } else { if (this.Docomod503iProcess(headers, browserCaps)) { } else { if (this.Docomod503isProcess(headers, browserCaps)) { } else { if (this.Docomod210iProcess(headers, browserCaps)) { } else { if (this.Docomof210iProcess(headers, browserCaps)) { } else { if (this.Docomon210iProcess(headers, browserCaps)) { } else { if (this.Docomon2001Process(headers, browserCaps)) { } else { if (this.Docomod211iProcess(headers, browserCaps)) { } else { if (this.Docomon211iProcess(headers, browserCaps)) { } else { if (this.Docomop210iProcess(headers, browserCaps)) { } else { if (this.Docomoko210iProcess(headers, browserCaps)) { } else { if (this.Docomop2101vProcess(headers, browserCaps)) { } else { if (this.Docomop2102vProcess(headers, browserCaps)) { } else { if (this.Docomof211iProcess(headers, browserCaps)) { } else { if (this.Docomof671iProcess(headers, browserCaps)) { } else { if (this.Docomon503isProcess(headers, browserCaps)) { } else { if (this.Docomon503iProcess(headers, browserCaps)) { } else { if (this.Docomoso503iProcess(headers, browserCaps)) { } else { if (this.Docomop503isProcess(headers, browserCaps)) { } else { if (this.Docomop503iProcess(headers, browserCaps)) { } else { if (this.Docomoso210iProcess(headers, browserCaps)) { } else { if (this.Docomoso503isProcess(headers, browserCaps)) { } else { if (this.Docomosh821iProcess(headers, browserCaps)) { } else { if (this.Docomon2002Process(headers, browserCaps)) { } else { if (this.Docomoso505iProcess(headers, browserCaps)) { } else { if (this.Docomop505iProcess(headers, browserCaps)) { } else { if (this.Docomon505iProcess(headers, browserCaps)) { } else { if (this.Docomod505iProcess(headers, browserCaps)) { } else { if (this.Docomoisim60Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } this.DocomoProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void DocomorenderingsizeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void DocomorenderingsizeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool DocomorenderingsizeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^DoCoMo/([^/ ]*)[/ ]([^/\\x28]*)([/\\x28]c(?\'cacheSize\'\\d+))"); if ((result == false)) { return false; } // Identification: check capability matches headerValue = ((string)(dictionary["maximumRenderedPageSize"])); result = regexWorker.ProcessRegex(headerValue, "^5120$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = regexWorker["${cacheSize}000"]; this.DocomorenderingsizeProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.DocomorenderingsizeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void DocomodefaultrenderingsizeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void DocomodefaultrenderingsizeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool DocomodefaultrenderingsizeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["maximumRenderedPageSize"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^(0|00|000)$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "800"; this.DocomodefaultrenderingsizeProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.DocomodefaultrenderingsizeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomosh251iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomosh251iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomosh251iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SH251i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Sharp"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoSH251i"); this.Docomosh251iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=DocomoSH251i if (this.Docomosh251isProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Docomosh251iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomosh251isProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomosh251isProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomosh251isProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SH251iS"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["requiresLeadingPageBreak"] = "false"; dictionary["screenCharactersHeight"] = "11"; dictionary["screenCharactersWidth"] = "22"; dictionary["screenPixelsHeight"] = "220"; dictionary["screenPixelsWidth"] = "176"; browserCaps.AddBrowser("DocomoSH251iS"); this.Docomosh251isProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomosh251isProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon251iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon251iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon251iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N251i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "22"; dictionary["screenPixelsHeight"] = "140"; dictionary["screenPixelsWidth"] = "132"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoN251i"); this.Docomon251iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=DocomoN251i if (this.Docomon251isProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Docomon251iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon251isProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon251isProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon251isProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N251iS"); if ((result == false)) { return false; } // Capabilities: set capabilities browserCaps.AddBrowser("DocomoN251iS"); this.Docomon251isProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon251isProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop211iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop211iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop211iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P211i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["MobileDeviceManufacturer"] = "Panasonic"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoP211i"); this.Docomop211iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop211iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof212iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof212iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof212iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F212i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenPixelsHeight"] = "136"; dictionary["screenPixelsWidth"] = "132"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoF212i"); this.Docomof212iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof212iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomod501iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomod501iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomod501iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D501i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoD501i"); this.Docomod501iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomod501iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof501iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof501iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof501iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F501i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "84"; dictionary["screenPixelsWidth"] = "112"; browserCaps.AddBrowser("DocomoF501i"); this.Docomof501iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof501iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon501iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon501iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon501iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N501i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; browserCaps.AddBrowser("DocomoN501i"); this.Docomon501iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon501iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop501iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop501iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop501iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P501i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "120"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoP501i"); this.Docomop501iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop501iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomod502iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomod502iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomod502iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D502i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "90"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoD502i"); this.Docomod502iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomod502iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof502iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof502iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof502iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F502i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "91"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoF502i"); this.Docomof502iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof502iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon502iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon502iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon502iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N502i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; browserCaps.AddBrowser("DocomoN502i"); this.Docomon502iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon502iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop502iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop502iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop502iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P502i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderEmptySelects"] = "false"; dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "117"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoP502i"); this.Docomop502iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop502iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomonm502iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomonm502iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomonm502iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "NM502i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "Nokia"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "106"; dictionary["screenPixelsWidth"] = "111"; browserCaps.AddBrowser("DocomoNm502i"); this.Docomonm502iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomonm502iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoso502iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoso502iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoso502iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SO502i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "120"; dictionary["screenPixelsWidth"] = "120"; browserCaps.AddBrowser("DocomoSo502i"); this.Docomoso502iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoso502iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof502itProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof502itProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof502itProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F502it"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "91"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoF502it"); this.Docomof502itProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof502itProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon502itProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon502itProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon502itProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N502it"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; browserCaps.AddBrowser("DocomoN502it"); this.Docomon502itProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon502itProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoso502iwmProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoso502iwmProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoso502iwmProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SO502iWM"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "113"; dictionary["screenPixelsWidth"] = "120"; browserCaps.AddBrowser("DocomoSo502iwm"); this.Docomoso502iwmProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoso502iwmProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof504iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof504iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof504iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F504i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "136"; dictionary["screenPixelsWidth"] = "132"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoF504i"); this.Docomof504iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof504iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon504iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon504iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon504iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N504i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "180"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoN504i"); this.Docomon504iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon504iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop504iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop504iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop504iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P504i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "9"; dictionary["screenCharactersWidth"] = "22"; dictionary["screenPixelsHeight"] = "144"; dictionary["screenPixelsWidth"] = "132"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoP504i"); this.Docomop504iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop504iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon821iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon821iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon821iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N821i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "119"; browserCaps.AddBrowser("DocomoN821i"); this.Docomon821iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon821iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop821iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop821iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop821iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P821i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["maximumRenderedPageSize"] = "5000"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoP821i"); this.Docomop821iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop821iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomod209iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomod209iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomod209iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D209i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "90"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoD209i"); this.Docomod209iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomod209iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoer209iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoer209iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoer209iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "ER209i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "Ericsson"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "120"; browserCaps.AddBrowser("DocomoEr209i"); this.Docomoer209iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoer209iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof209iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof209iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof209iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F209i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "91"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoF209i"); this.Docomof209iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof209iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoko209iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoko209iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoko209iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KO209i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Kokusai"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "96"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoKo209i"); this.Docomoko209iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoko209iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon209iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon209iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon209iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N209i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "82"; dictionary["screenPixelsWidth"] = "108"; browserCaps.AddBrowser("DocomoN209i"); this.Docomon209iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon209iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop209iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop209iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop209iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P209i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "87"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoP209i"); this.Docomop209iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop209iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop209isProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop209isProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop209isProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P209iS"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "87"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoP209is"); this.Docomop209isProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop209isProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomor209iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomor209iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomor209iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R209i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "JRC"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoR209i"); this.Docomor209iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomor209iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomor691iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomor691iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomor691iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R691i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "JRC"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoR691i"); this.Docomor691iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomor691iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof503iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof503iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof503iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F503i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "120"; browserCaps.AddBrowser("DocomoF503i"); this.Docomof503iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof503iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof503isProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof503isProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof503isProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F503iS"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "24"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "120"; browserCaps.AddBrowser("DocomoF503is"); this.Docomof503isProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof503isProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomod503iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomod503iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomod503iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D503i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "126"; dictionary["screenPixelsWidth"] = "132"; browserCaps.AddBrowser("DocomoD503i"); this.Docomod503iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomod503iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomod503isProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomod503isProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomod503isProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D503iS$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "126"; dictionary["screenPixelsWidth"] = "132"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoD503is"); this.Docomod503isProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomod503isProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomod210iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomod210iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomod210iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D210i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "91"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoD210i"); this.Docomod210iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomod210iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof210iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof210iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof210iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F210i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "113"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoF210i"); this.Docomof210iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof210iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon210iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon210iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon210iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N210i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "113"; dictionary["screenPixelsWidth"] = "118"; browserCaps.AddBrowser("DocomoN210i"); this.Docomon210iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon210iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon2001ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon2001ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon2001Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N2001"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoN2001"); this.Docomon2001ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon2001ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomod211iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomod211iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomod211iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D211i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "91"; dictionary["screenPixelsWidth"] = "100"; browserCaps.AddBrowser("DocomoD211i"); this.Docomod211iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomod211iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon211iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon211iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon211iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N211i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoN211i"); this.Docomon211iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon211iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop210iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop210iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop210iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P210i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "91"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoP210i"); this.Docomop210iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop210iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoko210iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoko210iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoko210iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KO210i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Kokusai"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "96"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoKo210i"); this.Docomoko210iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoko210iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop2101vProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop2101vProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop2101vProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P2101V"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["screenBitDepth"] = "18"; dictionary["screenCharactersHeight"] = "9"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "182"; dictionary["screenPixelsWidth"] = "163"; browserCaps.AddBrowser("DocomoP2101v"); this.Docomop2101vProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop2101vProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop2102vProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop2102vProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop2102vProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P2102V"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; browserCaps.AddBrowser("DocomoP2102v"); this.Docomop2102vProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop2102vProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof211iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof211iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof211iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F211i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "113"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoF211i"); this.Docomof211iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof211iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof671iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof671iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof671iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F671i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "9"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "126"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoF671i"); this.Docomof671iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof671iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon503isProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon503isProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon503isProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N503iS"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoN503is"); this.Docomon503isProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon503isProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon503iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon503iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon503iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N503i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoN503i"); this.Docomon503iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon503iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoso503iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoso503iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoso503iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SO503i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "113"; dictionary["screenPixelsWidth"] = "120"; browserCaps.AddBrowser("DocomoSo503i"); this.Docomoso503iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoso503iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop503isProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop503isProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop503isProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P503iS"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderEmptySelects"] = "false"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoP503is"); this.Docomop503isProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop503isProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop503iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop503iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop503iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P503i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderEmptySelects"] = "false"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["rendersBreaksAfterHtmlLists"] = "false"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsFontSize"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoP503i"); this.Docomop503iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop503iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoso210iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoso210iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoso210iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SO210i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "17"; dictionary["screenPixelsHeight"] = "113"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoSo210i"); this.Docomoso210iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoso210iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoso503isProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoso503isProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoso503isProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SO503iS"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "17"; dictionary["screenPixelsHeight"] = "113"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoSo503is"); this.Docomoso503isProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoso503isProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomosh821iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomosh821iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomosh821iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SH821i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderEmptySelects"] = "false"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Sharp"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "78"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoSh821i"); this.Docomosh821iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomosh821iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon2002ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon2002ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon2002Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N2002"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoN2002"); this.Docomon2002ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon2002ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoso505iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoso505iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoso505iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SO505i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "SonyEricsson"; dictionary["screenBitDepth"] = "18"; dictionary["screenCharactersHeight"] = "9"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "240"; dictionary["screenPixelsWidth"] = "256"; browserCaps.AddBrowser("DocomoSo505i"); this.Docomoso505iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoso505iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop505iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop505iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop505iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P505i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; browserCaps.AddBrowser("DocomoP505i"); this.Docomop505iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop505iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon505iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon505iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon505iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N505i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "NEC"; browserCaps.AddBrowser("DocomoN505i"); this.Docomon505iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon505iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomod505iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomod505iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomod505iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D505i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["screenBitDepth"] = "18"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "270"; dictionary["screenPixelsWidth"] = "240"; browserCaps.AddBrowser("DocomoD505i"); this.Docomod505iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomod505iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoisim60ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoisim60ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoisim60Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "ISIM60"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "false"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "true"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NTT DoCoMo"; dictionary["mobileDeviceModel"] = "i-mode HTML Simulator"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "180"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsEmptyStringInCookieValue"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectMultiple"] = "false"; browserCaps.AddBrowser("DocomoISIM60"); this.Docomoisim60ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoisim60ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssonr380ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssonr380ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssonr380Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R380 (?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*) WAP1\\.1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Ericsson"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["cookies"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "3000"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceManufacturer"] = "Ericsson"; dictionary["mobileDeviceModel"] = "R380"; dictionary["requiresNoescapedPostUrl"] = "false"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml11"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenPixelsHeight"] = "100"; dictionary["screenPixelsWidth"] = "310"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["type"] = "Ericsson R380"; dictionary["version"] = regexWorker["${browserMajorVersion}.${browserMinorVersion}"]; browserCaps.AddBrowser("EricssonR380"); this.Ericssonr380ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssonr380ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void EricssonProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void EricssonProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool EricssonProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Ericsson(?\'deviceID\'[^/]+)/(?\'deviceVer\'.*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Ericsson"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["cookies"] = "false"; dictionary["defaultScreenCharactersHeight"] = "4"; dictionary["defaultScreenCharactersWidth"] = "20"; dictionary["defaultScreenPixelsHeight"] = "52"; dictionary["defaultScreenPixelsWidth"] = "101"; dictionary["inputType"] = "telephoneKeypad"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["maximumRenderedPageSize"] = "1600"; dictionary["mobileDeviceManufacturer"] = "Ericsson"; dictionary["mobileDeviceModel"] = regexWorker["${deviceID}"]; dictionary["mobileDeviceVersion"] = regexWorker["${deviceVer}"]; dictionary["numberOfSoftkeys"] = "2"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml11"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["type"] = regexWorker["Ericsson ${deviceID}"]; browserCaps.HtmlTextWriter = "System.Web.UI.XhtmlTextWriter"; browserCaps.AddBrowser("Ericsson"); this.EricssonProcessGateways(headers, browserCaps); // gateway, parent=Ericsson this.SonyericssonProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Ericsson if (this.Ericssonr320Process(headers, browserCaps)) { } else { if (this.Ericssont20Process(headers, browserCaps)) { } else { if (this.Ericssont65Process(headers, browserCaps)) { } else { if (this.Ericssont68Process(headers, browserCaps)) { } else { if (this.Ericssont300Process(headers, browserCaps)) { } else { if (this.Ericssonp800Process(headers, browserCaps)) { } else { if (this.Ericssont61Process(headers, browserCaps)) { } else { if (this.Ericssont31Process(headers, browserCaps)) { } else { if (this.Ericssonr520Process(headers, browserCaps)) { } else { if (this.Ericssona2628Process(headers, browserCaps)) { } else { if (this.Ericssont39Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } } } this.EricssonProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SonyericssonProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SonyericssonProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SonyericssonProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^SonyEricsson"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Sony Ericsson"; dictionary["mobileDeviceManufacturer"] = "Sony Ericsson"; dictionary["type"] = regexWorker["Sony Ericsson ${mobileDeviceModel}"]; this.SonyericssonProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SonyericssonProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssonr320ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssonr320ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssonr320Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R320"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "3000"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "52"; dictionary["screenPixelsWidth"] = "101"; browserCaps.AddBrowser("EricssonR320"); this.Ericssonr320ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssonr320ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont20ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont20ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont20Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "T20"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "false"; dictionary["maximumRenderedPageSize"] = "1400"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["mobileDeviceModel"] = "T20, T20e, T29s"; dictionary["numberOfSoftkeys"] = "1"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenCharactersHeight"] = "3"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "33"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("EricssonT20"); this.Ericssont20ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont20ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont65ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont65ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont65Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "T65"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "3000"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["mobileDeviceModel"] = "Ericsson T65"; dictionary["numberOfSoftkeys"] = "1"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingType"] = "wml12"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "67"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("EricssonT65"); this.Ericssont65ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont65ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont68ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont68ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont68Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "T68"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "false"; dictionary["isColor"] = "true"; dictionary["numberOfSoftkeys"] = "1"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "false"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "80"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; browserCaps.AddBrowser("EricssonT68"); this.Ericssont68ProcessGateways(headers, browserCaps); // gateway, parent=EricssonT68 this.Ericssont68upgatewayProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=EricssonT68 if (this.Ericsson301aProcess(headers, browserCaps)) { } else { if (this.Ericssont68r1aProcess(headers, browserCaps)) { } else { if (this.Ericssont68r101Process(headers, browserCaps)) { } else { if (this.Ericssont68r201aProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } this.Ericssont68ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont68upgatewayProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont68upgatewayProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont68upgatewayProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "UP\\.Link"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "true"; this.Ericssont68upgatewayProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont68upgatewayProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericsson301aProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericsson301aProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericsson301aProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R301A"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canSendMail"] = "true"; dictionary["displaysAccessKeysAutomatically"] = "true"; dictionary["maximumRenderedPageSize"] = "2800"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresNewLineSuppression"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsBold"] = "false"; dictionary["supportsDivAlign"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "false"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["usePOverDiv"] = "true"; browserCaps.AddBrowser("Ericsson301A"); this.Ericsson301aProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericsson301aProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont300ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont300ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont300Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "T300"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnBlockElements"] = "false"; dictionary["breaksOnInlineElements"] = "false"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["cookies"] = "true"; dictionary["displaysAccessKeysAutomatically"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "2800"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "15"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsDivAlign"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "false"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["supportsWtai"] = "true"; dictionary["tables"] = "false"; browserCaps.AddBrowser("EricssonT300"); this.Ericssont300ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont300ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssonp800ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssonp800ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssonp800Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P800"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Sony Ericsson"; dictionary["cookies"] = "true"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Sony Ericsson"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "16"; dictionary["screenCharactersWidth"] = "28"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "208"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("EricssonP800"); this.Ericssonp800ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=EricssonP800 if (this.Ericssonp800r101Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Ericssonp800ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssonp800r101ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssonp800r101ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssonp800r101Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R101"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "10000"; browserCaps.AddBrowser("EricssonP800R101"); this.Ericssonp800r101ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssonp800r101ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont61ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont61ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont61Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "T610|T616|T618"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["cookies"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "9800"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "true"; dictionary["requiredOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "128"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsSelectFollowingTable"] = "false"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; browserCaps.AddBrowser("EricssonT61"); this.Ericssont61ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont61ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont31ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont31ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont31Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "T310|T312|T316"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnBlockElements"] = "false"; dictionary["breaksOnInlineElements"] = "false"; dictionary["canInitiateVoiceCall"] = "false"; dictionary["cookies"] = "true"; dictionary["displaysAccessKeysAutomatically"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "2800"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "15"; dictionary["screenPixelsHeight"] = "80"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsDivAlign"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "false"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; browserCaps.AddBrowser("EricssonT31"); this.Ericssont31ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont31ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont68r1aProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont68r1aProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont68r1aProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R1A"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "false"; dictionary["maximumRenderedPageSize"] = "5000"; dictionary["maximumSoftkeyLabelLength"] = "14"; dictionary["preferredImageMime"] = "image/gif"; dictionary["rendersWmlDoAcceptsInline"] = "true"; browserCaps.AddBrowser("EricssonT68R1A"); this.Ericssont68r1aProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont68r1aProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont68r101ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont68r101ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont68r101Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R101"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2900"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["preferredImageMime"] = "image/gif"; dictionary["requiresNoSoftkeyLabels"] = "true"; browserCaps.AddBrowser("EricssonT68R101"); this.Ericssont68r101ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont68r101ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont68r201aProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont68r201aProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont68r201aProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R201A"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["maximumRenderedPageSize"] = "2900"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingType"] = "wml12"; dictionary["screenBitDepth"] = "24"; browserCaps.AddBrowser("EricssonT68R201A"); this.Ericssont68r201aProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont68r201aProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssonr520ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssonr520ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssonr520Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R520"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "1600"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "67"; dictionary["screenPixelsWidth"] = "101"; browserCaps.AddBrowser("EricssonR520"); this.Ericssonr520ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssonr520ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssona2628ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssona2628ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssona2628Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "A2628"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "1600"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "54"; dictionary["screenPixelsWidth"] = "101"; browserCaps.AddBrowser("EricssonA2628"); this.Ericssona2628ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssona2628ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont39ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont39ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont39Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "T39"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "true"; dictionary["inputType"] = "telephoneKeypad"; dictionary["maximumRenderedPageSize"] = "3000"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["mobileDeviceManufacturer"] = "Ericsson"; dictionary["mobileDeviceModel"] = "Ericsson T39"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml12"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "3"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "54"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("EricssonT39"); this.Ericssont39ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont39ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void EzwapProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void EzwapProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool EzwapProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "EzWAP (?\'browserMajorVersion\'\\d+)(?\'browserMinorVersion\'\\.\\d+)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "EzWAP"; dictionary["canSendMail"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresXhtmlCssSuppression"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "33"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "240"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsDivAlign"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsStyleElement"] = "false"; dictionary["supportsUrlAttributeEncoding"] = "false"; dictionary["tables"] = "true"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.AddBrowser("EzWAP"); this.EzwapProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.EzwapProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void NokiagatewayProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void NokiagatewayProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool NokiagatewayProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["VIA"])); if (string.IsNullOrEmpty(headerValue)) { return false; } headerValue = ((string)(headers["VIA"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'nokiaVersion\'Nokia\\D*(?\'gatewayMajorVersion\'\\d+)(?\'gatewayMinorVersion\'\\.\\d+)[" + "^,]*)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["gatewayMajorVersion"] = regexWorker["${gatewayMajorVersion}"]; dictionary["gatewayMinorVersion"] = regexWorker["${gatewayMinorVersion}"]; dictionary["gatewayVersion"] = regexWorker["${nokiaVersion}"]; this.NokiagatewayProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.NokiagatewayProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpgatewayProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpgatewayProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpgatewayProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "UP\\.Link/"); if ((result == false)) { return false; } // Capture: header values regexWorker.ProcessRegex(((string)(browserCaps[string.Empty])), "(?\'goWebUPGateway\'Go\\.Web)"); // Capabilities: set capabilities dictionary["isGoWebUpGateway"] = regexWorker["${goWebUPGateway}"]; this.UpgatewayProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=UPGateway if (this.UpnongogatewayProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.UpgatewayProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpnongogatewayProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpnongogatewayProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpnongogatewayProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "UP\\.Link/(?\'gatewayMajorVersion\'\\d*)(?\'gatewayMinorVersion\'\\.\\d*)(?\'other\'\\S*)"); if ((result == false)) { return false; } // Identification: check capability matches headerValue = ((string)(dictionary["isGoWebUpGateway"])); result = regexWorker.ProcessRegex(headerValue, "^$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["gatewayMajorVersion"] = regexWorker["${gatewayMajorVersion}"]; dictionary["gatewayMinorVersion"] = regexWorker["${gatewayMinorVersion}"]; dictionary["gatewayVersion"] = regexWorker["UP.Link/${gatewayMajorVersion}${gatewayMinorVersion}${other}"]; this.UpnongogatewayProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpnongogatewayProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void CrawlerProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void CrawlerProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool CrawlerProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "crawler|Crawler|Googlebot|msnbot"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["crawler"] = "true"; this.CrawlerProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.CrawlerProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void ColorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void ColorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool ColorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["UA-COLOR"])); if (string.IsNullOrEmpty(headerValue)) { return false; } headerValue = ((string)(headers["UA-COLOR"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "color(?\'colorDepth\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["screenBitDepth"] = regexWorker["${colorDepth}"]; this.ColorProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.ColorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MonoProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MonoProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MonoProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["UA-COLOR"])); if (string.IsNullOrEmpty(headerValue)) { return false; } headerValue = ((string)(headers["UA-COLOR"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "mono(?\'colorDepth\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["screenBitDepth"] = regexWorker["${colorDepth}"]; this.MonoProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MonoProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PixelsProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PixelsProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PixelsProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["UA-PIXELS"])); if (string.IsNullOrEmpty(headerValue)) { return false; } headerValue = ((string)(headers["UA-PIXELS"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'screenWidth\'\\d+)x(?\'screenHeight\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; this.PixelsProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PixelsProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void VoiceProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void VoiceProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool VoiceProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["UA-VOICE"])); if (string.IsNullOrEmpty(headerValue)) { return false; } headerValue = ((string)(headers["UA-VOICE"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?i:TRUE)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "true"; this.VoiceProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.VoiceProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void CharsetProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void CharsetProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool CharsetProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-CHARSET"])); if (string.IsNullOrEmpty(headerValue)) { return false; } headerValue = ((string)(headers["X-UP-DEVCAP-CHARSET"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?i)^Shift_JIS$"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "(UP/\\S* UP\\.Browser/3\\.\\[3-9]d*)|(UP\\.Browser/3\\.\\[3-9]d*)|(UP\\.Browser/3\\.\\[3-9]" + "d*)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["canSendMail"] = "true"; this.CharsetProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.CharsetProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PlatformProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PlatformProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PlatformProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); if (string.IsNullOrEmpty(headerValue)) { return false; } // Capabilities: set capabilities this.PlatformProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Platform if (this.PlatformwinntProcess(headers, browserCaps)) { } else { if (this.Platformwin2000bProcess(headers, browserCaps)) { } else { if (this.Platformwin95Process(headers, browserCaps)) { } else { if (this.Platformwin98Process(headers, browserCaps)) { } else { if (this.Platformwin16Process(headers, browserCaps)) { } else { if (this.PlatformwinceProcess(headers, browserCaps)) { } else { if (this.Platformmac68kProcess(headers, browserCaps)) { } else { if (this.PlatformmacppcProcess(headers, browserCaps)) { } else { if (this.PlatformunixProcess(headers, browserCaps)) { } else { if (this.PlatformwebtvProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } } this.PlatformProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PlatformwinntProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PlatformwinntProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PlatformwinntProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Windows NT|WinNT|Windows XP"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "WinCE|Windows CE"); if ((result == true)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "WinNT"; this.PlatformwinntProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=PlatformWinnt if (this.PlatformwinxpProcess(headers, browserCaps)) { } else { if (this.Platformwin2000aProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.PlatformwinntProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PlatformwinxpProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PlatformwinxpProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PlatformwinxpProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Windows (NT 5\\.1|XP)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "WinXP"; this.PlatformwinxpProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PlatformwinxpProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Platformwin2000aProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Platformwin2000aProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Platformwin2000aProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Windows NT 5\\.0"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "Win2000"; this.Platformwin2000aProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Platformwin2000aProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Platformwin2000bProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Platformwin2000bProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Platformwin2000bProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Windows 2000"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "Win2000"; this.Platformwin2000bProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Platformwin2000bProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Platformwin95ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Platformwin95ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Platformwin95Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Win(dows )?95"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "Win95"; this.Platformwin95ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Platformwin95ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Platformwin98ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Platformwin98ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Platformwin98Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Win(dows )?98"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "Win98"; this.Platformwin98ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Platformwin98ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Platformwin16ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Platformwin16ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Platformwin16Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Win(dows 3\\.1|16)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "Win16"; this.Platformwin16ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Platformwin16ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PlatformwinceProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PlatformwinceProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PlatformwinceProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Win(dows )?CE"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "WinCE"; this.PlatformwinceProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PlatformwinceProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Platformmac68kProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Platformmac68kProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Platformmac68kProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Mac(_68(000|K)|intosh.*68K)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "Mac68K"; this.Platformmac68kProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Platformmac68kProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PlatformmacppcProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PlatformmacppcProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PlatformmacppcProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Mac(_PowerPC|intosh.*PPC|_PPC)|PPC Mac"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "MacPPC"; this.PlatformmacppcProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PlatformmacppcProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PlatformunixProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PlatformunixProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PlatformunixProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "X11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "UNIX"; this.PlatformunixProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PlatformunixProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PlatformwebtvProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PlatformwebtvProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PlatformwebtvProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "WebTV"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "WebTV"; this.PlatformwebtvProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PlatformwebtvProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void WinProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void WinProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool WinProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); if (string.IsNullOrEmpty(headerValue)) { return false; } // Capabilities: set capabilities this.WinProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Win if (this.Win32Process(headers, browserCaps)) { } else { if (this.Win16Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.WinProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Win32ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Win32ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Win32Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Win(dows )?(9[58]|NT|32)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["win32"] = "true"; this.Win32ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Win32ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Win16ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Win16ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Win16Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "16bit|Win(dows 3\\.1|16)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["win16"] = "true"; this.Win16ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Win16ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GenericdownlevelProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GenericdownlevelProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GenericdownlevelProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Generic Downlevel$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "false"; dictionary["ecmascriptversion"] = "1.0"; dictionary["tables"] = "true"; dictionary["type"] = "Downlevel"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("GenericDownlevel"); this.GenericdownlevelProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.GenericdownlevelProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GoamericaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GoamericaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GoamericaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Go\\.Web/(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["BackgroundSounds"] = "true"; dictionary["browser"] = "Go.Web"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "true"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "12"; dictionary["defaultScreenPixelsHeight"] = "72"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["isMobileDevice"] = "true"; dictionary["javaapplets"] = "false"; dictionary["javascript"] = "false"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "6000"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["rendersBreaksAfterHtmlLists"] = "false"; dictionary["requiredMetaTagNameValue"] = "HandheldFriendly"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresNoBreakInFormatting"] = "true"; dictionary["requiresUniqueHtmlCheckboxNames"] = "true"; dictionary["screenBitDepth"] = "2"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "false"; dictionary["supportsDivAlign"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["SupportsDivNoWrap"] = "false"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "false"; dictionary["supportsSelectMultiple"] = "false"; dictionary["type"] = "Go.Web"; dictionary["vbscript"] = "false"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("GoAmerica"); this.GoamericaProcessGateways(headers, browserCaps); // gateway, parent=GoAmerica this.GoamericaupProcess(headers, browserCaps); // gateway, parent=GoAmerica this.GatableProcess(headers, browserCaps); // gateway, parent=GoAmerica this.MaxpagesizeProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=GoAmerica if (this.GoamericawinceProcess(headers, browserCaps)) { } else { if (this.GoamericapalmProcess(headers, browserCaps)) { } else { if (this.GoamericarimProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.GoamericaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GoamericaupProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GoamericaupProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GoamericaupProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "UP\\.Browser"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "0.0"; dictionary["frames"] = "false"; this.GoamericaupProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.GoamericaupProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GoamericawinceProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GoamericawinceProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GoamericawinceProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "WinCE"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["defaultScreenCharactersHeight"] = "14"; dictionary["defaultScreenCharactersWidth"] = "30"; dictionary["defaultScreenPixelsHeight"] = "320"; dictionary["defaultScreenPixelsWidth"] = "240"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["mobileDeviceModel"] = "Pocket PC"; dictionary["platform"] = "WinCE"; dictionary["screenBitDepth"] = "16"; dictionary["supportsDivAlign"] = "true"; dictionary["supportsFontColor"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsSelectMultiple"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("GoAmericaWinCE"); this.GoamericawinceProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.GoamericawinceProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GoamericapalmProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GoamericapalmProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GoamericapalmProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Palm"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "PalmOS-licensee"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "36"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsUncheck"] = "false"; browserCaps.AddBrowser("GoAmericaPalm"); this.GoamericapalmProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.GoamericapalmProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GoamericarimProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GoamericarimProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GoamericarimProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "RIM"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "RIM"; dictionary["screenBitDepth"] = "1"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsCss"] = "false"; dictionary["supportsDivAlign"] = "false"; dictionary["supportsDivWrap"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["supportsFontItalic"] = "false"; browserCaps.AddBrowser("GoAmericaRIM"); this.GoamericarimProcessGateways(headers, browserCaps); // gateway, parent=GoAmericaRIM this.GoamericanonuprimProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=GoAmericaRIM if (this.Goamericarim950Process(headers, browserCaps)) { } else { if (this.Goamericarim850Process(headers, browserCaps)) { } else { if (this.Goamericarim957Process(headers, browserCaps)) { } else { if (this.Goamericarim857Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } this.GoamericarimProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GoamericanonuprimProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GoamericanonuprimProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GoamericanonuprimProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "UP\\.Browser"); if ((result == true)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.1"; dictionary["frames"] = "true"; this.GoamericanonuprimProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.GoamericanonuprimProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Goamericarim950ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Goamericarim950ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Goamericarim950Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "RIM950"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["BackgroundSounds"] = "false"; dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["mobileDeviceModel"] = "950"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "25"; dictionary["screenPixelsHeight"] = "64"; dictionary["screenPixelsWidth"] = "132"; browserCaps.AddBrowser("GoAmericaRIM950"); this.Goamericarim950ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Goamericarim950ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Goamericarim850ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Goamericarim850ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Goamericarim850Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "RIM850"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceModel"] = "850"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "25"; dictionary["screenPixelsHeight"] = "64"; dictionary["screenPixelsWidth"] = "132"; browserCaps.AddBrowser("GoAmericaRIM850"); this.Goamericarim850ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Goamericarim850ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Goamericarim957ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Goamericarim957ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Goamericarim957Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "RIM957"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["BackgroundSounds"] = "false"; dictionary["mobileDeviceModel"] = "957"; dictionary["screenCharactersHeight"] = "15"; dictionary["screenCharactersWidth"] = "32"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "160"; browserCaps.AddBrowser("GoAmericaRIM957"); this.Goamericarim957ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=GoAmericaRIM957 if (this.Goamericarim957major6minor2Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Goamericarim957ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Goamericarim957major6minor2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Goamericarim957major6minor2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Goamericarim957major6minor2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "6\\.2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["maximumRenderedPageSize"] = "7168"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["requiresUniqueHtmlCheckboxNames"] = "true"; dictionary["screenCharactersHeight"] = "13"; dictionary["screenCharactersWidth"] = "30"; dictionary["supportsSelectMultiple"] = "true"; dictionary["supportsUncheck"] = "true"; browserCaps.AddBrowser("GoAmericaRIM957major6minor2"); this.Goamericarim957major6minor2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Goamericarim957major6minor2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Goamericarim857ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Goamericarim857ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Goamericarim857Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "RIM857"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["BackgroundSounds"] = "false"; dictionary["ecmascriptversion"] = "0.0"; dictionary["frames"] = "false"; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["mobileDeviceModel"] = "857"; dictionary["screenCharactersHeight"] = "15"; dictionary["screenCharactersWidth"] = "32"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "160"; browserCaps.AddBrowser("GoAmericaRIM857"); this.Goamericarim857ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=GoAmericaRIM857 if (this.Goamericarim857major6Process(headers, browserCaps)) { } else { if (this.Goamerica7to9Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.Goamericarim857ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Goamericarim857major6ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Goamericarim857major6ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Goamericarim857major6Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "6\\."); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.1"; dictionary["frames"] = "true"; browserCaps.AddBrowser("GoAmericaRIM857major6"); this.Goamericarim857major6ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=GoAmericaRIM857major6 if (this.Goamericarim857major6minor2to9Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Goamericarim857major6ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Goamericarim857major6minor2to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Goamericarim857major6minor2to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Goamericarim857major6minor2to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "6\\.[2-9]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["requiresAttributeColonSubstitution"] = "false"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenCharactersHeight"] = "16"; dictionary["screenCharactersWidth"] = "31"; dictionary["supportsUncheck"] = "false"; browserCaps.AddBrowser("GoAmericaRIM857major6minor2to9"); this.Goamericarim857major6minor2to9ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Goamericarim857major6minor2to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Goamerica7to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Goamerica7to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Goamerica7to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^[7-9]$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["requiresAttributeColonSubstitution"] = "false"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenCharactersHeight"] = "16"; dictionary["screenCharactersWidth"] = "31"; dictionary["supportsUncheck"] = "false"; browserCaps.AddBrowser("GoAmerica7to9"); this.Goamerica7to9ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Goamerica7to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GatableProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GatableProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GatableProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-GA-TABLES"])); if (string.IsNullOrEmpty(headerValue)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities this.GatableProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=GaTable if (this.GatablefalseProcess(headers, browserCaps)) { } else { if (this.GatabletrueProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.GatableProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GatablefalseProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GatablefalseProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GatablefalseProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-GA-TABLES"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?i:FALSE)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["tables"] = "false"; this.GatablefalseProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.GatablefalseProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GatabletrueProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GatabletrueProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GatabletrueProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-GA-TABLES"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?i:TRUE)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["tables"] = "true"; this.GatabletrueProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.GatabletrueProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MaxpagesizeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MaxpagesizeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MaxpagesizeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-GA-MAX-TRANSFER"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'maxPageSize\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = regexWorker["${maxPageSize}"]; this.MaxpagesizeProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MaxpagesizeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JataayuProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JataayuProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JataayuProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^jBrowser"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Jataayu jBrowser"; dictionary["canSendMail"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceManufacturer"] = "Jataayu"; dictionary["mobileDeviceModel"] = "jBrowser"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "wml20"; dictionary["requiresCommentInStyleElement"] = "true"; dictionary["requiresHiddenFieldValues"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "true"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "17"; dictionary["screenCharactersWidth"] = "42"; dictionary["screenPixelsHeight"] = "265"; dictionary["screenPixelsWidth"] = "248"; dictionary["supportsBodyClassAttribute"] = "false"; dictionary["supportsBold"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["tables"] = "true"; dictionary["type"] = "Jataayu jBrowser"; browserCaps.AddBrowser("Jataayu"); this.JataayuProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Jataayu if (this.JataayuppcProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.JataayuProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JataayuppcProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JataayuppcProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JataayuppcProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(PPC)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "true"; dictionary["screenCharactersHeight"] = "14"; dictionary["screenCharactersWidth"] = "31"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "240"; dictionary["supportsStyleElement"] = "true"; browserCaps.AddBrowser("JataayuPPC"); this.JataayuppcProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JataayuppcProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphoneProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphoneProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphoneProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-PHONE/"); if ((result == false)) { return false; } // Capture: header values regexWorker.ProcessRegex(((string)(browserCaps[string.Empty])), "J-PHONE/(?\'majorVersion\'\\d+)(?\'minorVersion\'\\.\\d+)/(?\'deviceModel\'.*)"); // Capabilities: set capabilities dictionary["browser"] = "J-Phone"; dictionary["cookies"] = "false"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["defaultCharacterHeight"] = "12"; dictionary["defaultCharacterWidth"] = "12"; dictionary["defaultScreenCharactersHeight"] = "7"; dictionary["defaultScreenCharactersWidth"] = "16"; dictionary["defaultScreenPixelsHeight"] = "84"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["javaapplets"] = "false"; dictionary["javascript"] = "false"; dictionary["majorVersion"] = regexWorker["${majorVersion}"]; dictionary["maximumRenderedPageSize"] = "6000"; dictionary["minorVersion"] = regexWorker["${minorVersion}"]; dictionary["mobileDeviceModel"] = regexWorker["${deviceModel}"]; dictionary["optimumPageWeight"] = "700"; dictionary["preferredImageMime"] = "image/png"; dictionary["preferredRenderingType"] = "html32"; dictionary["preferredRequestEncoding"] = "shift_jis"; dictionary["preferredResponseEncoding"] = "shift_jis"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresContentTypeMetaTag"] = "true"; dictionary["requiresFullyQualifiedRedirectUrl"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOutputOptimization"] = "true"; dictionary["screenBitDepth"] = "2"; dictionary["supportsAccesskeyAttribute"] = "true"; dictionary["supportsBodyColor"] = "true"; dictionary["supportsBold"] = "false"; dictionary["supportsCharacterEntityEncoding"] = "false"; dictionary["supportsDivAlign"] = "true"; dictionary["supportsDivNoWrap"] = "true"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsFontColor"] = "true"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["supportsInputMode"] = "true"; dictionary["supportsItalic"] = "false"; dictionary["supportsJPhoneMultiMediaAttributes"] = "true"; dictionary["supportsJPhoneSymbols"] = "true"; dictionary["supportsQueryStringInFormAction"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["tables"] = "true"; dictionary["type"] = "J-Phone"; dictionary["vbscript"] = "false"; dictionary["version"] = regexWorker["${majorVersion}${minorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.ChtmlTextWriter"; browserCaps.AddBrowser("Jphone"); this.JphoneProcessGateways(headers, browserCaps); // gateway, parent=Jphone this.Jphone4Process(headers, browserCaps); // gateway, parent=Jphone this.JphonecolorProcess(headers, browserCaps); // gateway, parent=Jphone this.JphonedisplayProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Jphone if (this.JphonemitsubishiProcess(headers, browserCaps)) { } else { if (this.JphonedensoProcess(headers, browserCaps)) { } else { if (this.JphonekenwoodProcess(headers, browserCaps)) { } else { if (this.JphonenecProcess(headers, browserCaps)) { } else { if (this.JphonepanasonicProcess(headers, browserCaps)) { } else { if (this.JphonepioneerProcess(headers, browserCaps)) { } else { if (this.JphonesanyoProcess(headers, browserCaps)) { } else { if (this.JphonesharpProcess(headers, browserCaps)) { } else { if (this.JphonetoshibaProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } this.JphoneProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonemitsubishiProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonemitsubishiProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonemitsubishiProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-D\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; browserCaps.AddBrowser("JphoneMitsubishi"); this.JphonemitsubishiProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JphonemitsubishiProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonedensoProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonedensoProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonedensoProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-DN\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Denso"; browserCaps.AddBrowser("JphoneDenso"); this.JphonedensoProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JphonedensoProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonekenwoodProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonekenwoodProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonekenwoodProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-K\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kenwood"; browserCaps.AddBrowser("JphoneKenwood"); this.JphonekenwoodProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JphonekenwoodProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonenecProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonenecProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonenecProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-N\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "NEC"; browserCaps.AddBrowser("JphoneNec"); this.JphonenecProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=JphoneNec if (this.Jphonenecn51Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.JphonenecProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonenecn51ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonenecn51ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonenecn51Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-N51"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "12000"; dictionary["mobileDeviceModel"] = "J-N51"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["supportsCharacterEntityEncoding"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsInputIStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneNecN51"); this.Jphonenecn51ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonenecn51ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonepanasonicProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonepanasonicProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonepanasonicProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-P\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; browserCaps.AddBrowser("JphonePanasonic"); this.JphonepanasonicProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JphonepanasonicProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonepioneerProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonepioneerProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonepioneerProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-PE\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Pioneer"; browserCaps.AddBrowser("JphonePioneer"); this.JphonepioneerProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JphonepioneerProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonesanyoProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonesanyoProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonesanyoProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-SA\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; browserCaps.AddBrowser("JphoneSanyo"); this.JphonesanyoProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=JphoneSanyo if (this.Jphonesa51Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.JphonesanyoProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonesa51ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonesa51ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonesa51Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-SA51\\D*"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "12000"; dictionary["mobileDeviceModel"] = "J-SA51"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenCharactersWidth"] = "22"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsInputIStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneSA51"); this.Jphonesa51ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonesa51ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonesharpProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonesharpProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonesharpProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-SH\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sharp"; browserCaps.AddBrowser("JphoneSharp"); this.JphonesharpProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=JphoneSharp if (this.Jphonesharpsh53Process(headers, browserCaps)) { } else { if (this.Jphonesharpsh07Process(headers, browserCaps)) { } else { if (this.Jphonesharpsh08Process(headers, browserCaps)) { } else { if (this.Jphonesharpsh51Process(headers, browserCaps)) { } else { if (this.Jphonesharpsh52Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } this.JphonesharpProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonesharpsh53ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonesharpsh53ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonesharpsh53Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-SH53"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "12000"; dictionary["mobileDeviceModel"] = "J-SH53"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["screenBitDepth"] = "18"; dictionary["screenCharactersHeight"] = "13"; dictionary["screenCharactersWidth"] = "24"; dictionary["supportsCharacterEntityEncoding"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsInputIStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneSharpSh53"); this.Jphonesharpsh53ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonesharpsh53ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonesharpsh07ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonesharpsh07ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonesharpsh07Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-SH07"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderEmptySelects"] = "false"; dictionary["maximumRenderedPageSize"] = "12000"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["requiresLeadingPageBreak"] = "false"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneSharpSh07"); this.Jphonesharpsh07ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonesharpsh07ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonesharpsh08ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonesharpsh08ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonesharpsh08Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-SH08"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["supportsInputIStyle"] = "false"; dictionary["requiresLeadingPageBreak"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "117"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneSharpSh08"); this.Jphonesharpsh08ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonesharpsh08ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonesharpsh51ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonesharpsh51ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonesharpsh51Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-SH51"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "12000"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["supportsInputIStyle"] = "true"; dictionary["requiresLeadingPageBreak"] = "false"; dictionary["requiresUniqueHtmlCheckboxNames"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneSharpSh51"); this.Jphonesharpsh51ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonesharpsh51ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonesharpsh52ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonesharpsh52ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonesharpsh52Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-SH52\\D*"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "12000"; dictionary["mobileDeviceModel"] = "J-SH52"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenCharactersWidth"] = "20"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsInputIStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneSharpSh52"); this.Jphonesharpsh52ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonesharpsh52ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonetoshibaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonetoshibaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonetoshibaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-T\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; browserCaps.AddBrowser("JphoneToshiba"); this.JphonetoshibaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=JphoneToshiba if (this.Jphonetoshibat06aProcess(headers, browserCaps)) { } else { if (this.Jphonetoshibat08Process(headers, browserCaps)) { } else { if (this.Jphonetoshibat51Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.JphonetoshibaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonetoshibat06aProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonetoshibat06aProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonetoshibat06aProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-T06_a"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "12000"; dictionary["mobileDeviceModel"] = "J-T06"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "20"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneToshibaT06a"); this.Jphonetoshibat06aProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonetoshibat06aProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonetoshibat08ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonetoshibat08ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonetoshibat08Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-T08\\D*"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "22"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneToshibaT08"); this.Jphonetoshibat08ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonetoshibat08ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonetoshibat51ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonetoshibat51ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonetoshibat51Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-T51"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "12000"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["requiresLeadingPageBreak"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "24"; dictionary["screenPixelsHeight"] = "144"; dictionary["screenPixelsWidth"] = "144"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsInputIStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneToshibaT51"); this.Jphonetoshibat51ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonetoshibat51ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphone4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphone4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphone4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "4"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["supportsQueryStringInFormAction"] = "true"; this.Jphone4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphone4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonecolorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonecolorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonecolorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-JPHONE-COLOR"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'colorIndicator\'[CG])(?\'bitDepth\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["bitDepth"] = regexWorker["${bitDepth}"]; dictionary["colorIndicator"] = regexWorker["${colorIndicator}"]; this.JphonecolorProcessGateways(headers, browserCaps); // gateway, parent=JphoneColor this.JphonecoloriscolorProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=JphoneColor if (this.Jphone16bitcolorProcess(headers, browserCaps)) { } else { if (this.Jphone8bitcolorProcess(headers, browserCaps)) { } else { if (this.Jphone2bitcolorProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.JphonecolorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonecoloriscolorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonecoloriscolorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonecoloriscolorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["colorIndicator"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "C"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; this.JphonecoloriscolorProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JphonecoloriscolorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphone16bitcolorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphone16bitcolorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphone16bitcolorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["bitDepth"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^65536$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenBitDepth"] = "16"; this.Jphone16bitcolorProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphone16bitcolorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphone8bitcolorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphone8bitcolorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphone8bitcolorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["bitDepth"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^256$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenBitDepth"] = "8"; this.Jphone8bitcolorProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphone8bitcolorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphone2bitcolorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphone2bitcolorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphone2bitcolorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["bitDepth"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^4$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenBitDepth"] = "2"; this.Jphone2bitcolorProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphone2bitcolorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonedisplayProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonedisplayProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonedisplayProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-JPHONE-DISPLAY"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'screenWidth\'\\d+)\\*(?\'screenHeight\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; this.JphonedisplayProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JphonedisplayProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void LegendProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void LegendProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool LegendProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^(?\'deviceID\'LG\\S*) AU\\/(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*)." + "*"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "AU-System"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "false"; dictionary["hasBackButton"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["numberOfSoftkeys"] = "2"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "false"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["type"] = regexWorker["AU ${browserMajorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.AddBrowser("Legend"); this.LegendProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Legend if (this.Lgg5200Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.LegendProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lgg5200ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lgg5200ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lgg5200Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^LG-G5200"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "3500"; dictionary["maximumSoftkeyLabelLength"] = "6"; dictionary["mobileDeviceManufacturer"] = "LEGEND"; dictionary["mobileDeviceModel"] = "G808"; dictionary["screenBitDepth"] = "4"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "17"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "128"; browserCaps.AddBrowser("LGG5200"); this.Lgg5200ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lgg5200ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MmeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MmeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MmeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MMEF"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "Mozilla/(?\'version\'(?\'major\'\\d+)(?\'minor\'\\.\\d+)\\w*).*"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Microsoft Mobile Explorer"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["inputType"] = "telephoneKeypad"; dictionary["isMobileDevice"] = "true"; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["mobileDeviceManufacturer"] = "Microsoft"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["type"] = "Microsoft Mobile Explorer"; dictionary["version"] = regexWorker["${version}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("MME"); this.MmeProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=MME if (this.Mmef20Process(headers, browserCaps)) { } else { if (this.MmemobileexplorerProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.MmeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mmef20ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mmef20ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mmef20Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MMEF20"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderSetvarZeroWithMultiSelectionList"] = "false"; dictionary["defaultCharacterHeight"] = "15"; dictionary["defaultCharacterWidth"] = "5"; dictionary["defaultScreenPixelsHeight"] = "160"; dictionary["defaultScreenPixelsWidth"] = "120"; dictionary["isColor"] = "false"; dictionary["maximumRenderedPageSize"] = "4000"; dictionary["mobileDeviceModel"] = "Simulator"; dictionary["numberOfSoftkeys"] = "2"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml11"; dictionary["screenBitDepth"] = "1"; browserCaps.AddBrowser("MMEF20"); this.Mmef20ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=MMEF20 if (this.MmecellphoneProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Mmef20ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MmemobileexplorerProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MmemobileexplorerProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MmemobileexplorerProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^MobileExplorer/(?\'majorVersion\'\\d*)(?\'minorVersion\'\\.\\d*) \\(Mozilla/1\\.22; compa" + "tible; MMEF\\d+; (?\'manufacturer\'[^;]*); (?\'model\'[^;)]*)(; (?\'deviceID\'[^)]*))?"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderSetvarZeroWithMultiSelectionList"] = "false"; dictionary["defaultCharacterHeight"] = "15"; dictionary["defaultCharacterWidth"] = "5"; dictionary["defaultScreenPixelsHeight"] = "160"; dictionary["defaultScreenPixelsWidth"] = "120"; dictionary["deviceID"] = regexWorker["${deviceID}"]; dictionary["isColor"] = "true"; dictionary["majorVersion"] = regexWorker["${majorVersion}"]; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["minorVersion"] = regexWorker["${minorVersion}"]; dictionary["mobileDeviceManufacturer"] = regexWorker["${manufacturer}"]; dictionary["mobileDeviceModel"] = regexWorker["${model}"]; dictionary["numberOfSoftkeys"] = "2"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "html32"; dictionary["screenBitDepth"] = "8"; dictionary["supportsBodyColor"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsDivAlign"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFontColor"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["version"] = regexWorker["${majorVersion}${minorVersion}"]; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("MMEMobileExplorer"); this.MmemobileexplorerProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MmemobileexplorerProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MmecellphoneProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MmecellphoneProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MmecellphoneProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Mozilla/.*\\(compatible; MMEF(?\'majorVersion\'\\d)(?\'minorVersion\'\\d); Cell[pP]hone(" + "([;,] (?\'deviceID\'[^;]*))(;(?\'buildInfo\'.*))*)*\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canCombineFormsInDeck"] = "false"; dictionary["canRenderPostBackCards"] = "false"; dictionary["deviceID"] = regexWorker["${deviceID}"]; dictionary["majorVersion"] = regexWorker["${majorVersion}"]; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["minorVersion"] = regexWorker[".${minorVersion}"]; dictionary["version"] = regexWorker["${majorVersion}.${minorVersion}"]; browserCaps.AddBrowser("MMECellphone"); this.MmecellphoneProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=MMECellphone if (this.MmebenefonqProcess(headers, browserCaps)) { } else { if (this.Mmesonycmdz5Process(headers, browserCaps)) { } else { if (this.Mmesonycmdj5Process(headers, browserCaps)) { } else { if (this.Mmesonycmdj7Process(headers, browserCaps)) { } else { if (this.MmegenericsmallProcess(headers, browserCaps)) { } else { if (this.MmegenericlargeProcess(headers, browserCaps)) { } else { if (this.MmegenericflipProcess(headers, browserCaps)) { } else { if (this.Mmegeneric3dProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } this.MmecellphoneProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MmebenefonqProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MmebenefonqProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MmebenefonqProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Benefon Q"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Benefon"; dictionary["mobileDeviceModel"] = "Q"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "48"; dictionary["screenPixelsWidth"] = "100"; browserCaps.AddBrowser("MMEBenefonQ"); this.MmebenefonqProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MmebenefonqProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mmesonycmdz5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mmesonycmdz5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mmesonycmdz5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Sony CMD-Z5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "CMD-Z5"; dictionary["requiresOutputOptimization"] = "true"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "60"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("MMESonyCMDZ5"); this.Mmesonycmdz5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=MMESonyCMDZ5 if (this.Mmesonycmdz5pj020eProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Mmesonycmdz5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mmesonycmdz5pj020eProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mmesonycmdz5pj020eProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mmesonycmdz5pj020eProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Pj020e"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenPixelsHeight"] = "65"; browserCaps.AddBrowser("MMESonyCMDZ5Pj020e"); this.Mmesonycmdz5pj020eProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mmesonycmdz5pj020eProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mmesonycmdj5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mmesonycmdj5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mmesonycmdj5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Sony CMD-J5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "CMD-J5"; dictionary["requiresOutputOptimization"] = "true"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "65"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("MMESonyCMDJ5"); this.Mmesonycmdj5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mmesonycmdj5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mmesonycmdj7ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mmesonycmdj7ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mmesonycmdj7Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Sony CMD-J7/J70"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canCombineFormsInDeck"] = "true"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["canRenderSetvarZeroWithMultiSelectionList"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "2900"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["mobileDeviceManufacturer"] = "Ericsson"; dictionary["mobileDeviceModel"] = "T68"; dictionary["numberOfSoftkeys"] = "1"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "false"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "80"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; browserCaps.AddBrowser("MMESonyCMDJ7"); this.Mmesonycmdj7ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mmesonycmdj7ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MmegenericsmallProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MmegenericsmallProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MmegenericsmallProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "GenericSmall"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Microsoft"; dictionary["mobileDeviceModel"] = "Generic Small Skin"; dictionary["screenBitDepth"] = "1"; dictionary["screenPixelsHeight"] = "60"; dictionary["screenPixelsWidth"] = "100"; browserCaps.AddBrowser("MMEGenericSmall"); this.MmegenericsmallProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MmegenericsmallProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MmegenericlargeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MmegenericlargeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MmegenericlargeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "GenericLarge"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Microsoft"; dictionary["mobileDeviceModel"] = "Generic Large Skin"; dictionary["screenBitDepth"] = "1"; dictionary["screenPixelsHeight"] = "240"; dictionary["screenPixelsWidth"] = "160"; browserCaps.AddBrowser("MMEGenericLarge"); this.MmegenericlargeProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MmegenericlargeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MmegenericflipProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MmegenericflipProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MmegenericflipProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "GenericFlip"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Microsoft"; dictionary["mobileDeviceModel"] = "Generic Flip Skin"; dictionary["screenBitDepth"] = "1"; dictionary["screenPixelsHeight"] = "200"; dictionary["screenPixelsWidth"] = "160"; browserCaps.AddBrowser("MMEGenericFlip"); this.MmegenericflipProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MmegenericflipProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mmegeneric3dProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mmegeneric3dProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mmegeneric3dProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Generic3D"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Microsoft"; dictionary["mobileDeviceModel"] = "Generic 3D Skin"; dictionary["screenBitDepth"] = "1"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "128"; browserCaps.AddBrowser("MMEGeneric3D"); this.Mmegeneric3dProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mmegeneric3dProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Netscape3ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Netscape3ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Netscape3Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Mozilla/3"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "Opera"); if ((result == true)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "AvantGo"); if ((result == true)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "MSIE"); if ((result == true)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Netscape"; dictionary["cookies"] = "true"; dictionary["css1"] = "true"; dictionary["ecmascriptversion"] = "1.1"; dictionary["frames"] = "true"; dictionary["isColor"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["supportsCss"] = "false"; dictionary["supportsFileUpload"] = "true"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "Netscape3"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("Netscape3"); this.Netscape3ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Netscape3ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Netscape4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Netscape4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Netscape4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Mozilla/4"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "Opera"); if ((result == true)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "MSIE"); if ((result == true)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Netscape"; dictionary["cookies"] = "true"; dictionary["css1"] = "true"; dictionary["ecmascriptversion"] = "1.3"; dictionary["frames"] = "true"; dictionary["isColor"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["supportsCss"] = "false"; dictionary["supportsFileUpload"] = "true"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "Netscape4"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("Netscape4"); this.Netscape4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Netscape4 if (this.CasiopeiaProcess(headers, browserCaps)) { } else { if (this.PalmwebproProcess(headers, browserCaps)) { } else { if (this.NetfrontProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.Netscape4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Netscape5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Netscape5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Netscape5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Mozilla/5\\.0 \\([^)]*\\) (Gecko/[-\\d]+ )?Netscape\\d?/(?\'version\'(?\'major\'\\d+)(?\'mi" + "nor\'\\.\\d+)(?\'letters\'\\w*))"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Netscape"; dictionary["cookies"] = "true"; dictionary["css1"] = "true"; dictionary["css2"] = "true"; dictionary["ecmascriptversion"] = "1.5"; dictionary["frames"] = "true"; dictionary["isColor"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["letters"] = regexWorker["${letters}"]; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["preferredImageMime"] = "image/gif"; dictionary["screenBitDepth"] = "8"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["supportsVCard"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["Netscape${major}"]; dictionary["version"] = regexWorker["${version}"]; dictionary["w3cdomversion"] = "1.0"; dictionary["xml"] = "true"; browserCaps.AddBrowser("Netscape5"); this.Netscape5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Netscape5 if (this.Netscape6to9Process(headers, browserCaps)) { } else { if (this.NetscapebetaProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.Netscape5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Netscape6to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Netscape6to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Netscape6to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "[6-9]\\."); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsXmlHttp"] = "true"; dictionary["supportsCallback"] = "true"; dictionary["supportsMaintainScrollPositionOnPostback"] = "true"; browserCaps.AddBrowser("Netscape6to9"); this.Netscape6to9ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Netscape6to9 if (this.Netscape6to9betaProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Netscape6to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Netscape6to9betaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Netscape6to9betaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Netscape6to9betaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["letters"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^b"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; browserCaps.AddBrowser("Netscape6to9Beta"); this.Netscape6to9betaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Netscape6to9betaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void NetscapebetaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void NetscapebetaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool NetscapebetaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["letters"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^b"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; browserCaps.AddBrowser("NetscapeBeta"); this.NetscapebetaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.NetscapebetaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void NokiaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void NokiaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool NokiaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Nokia"; dictionary["cookies"] = "false"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canRenderOneventAndPrevElementsTogether"] = "false"; dictionary["canRenderPostBackCards"] = "false"; dictionary["canSendMail"] = "false"; dictionary["defaultScreenCharactersHeight"] = "4"; dictionary["defaultScreenCharactersWidth"] = "20"; dictionary["defaultScreenPixelsHeight"] = "40"; dictionary["defaultScreenPixelsWidth"] = "90"; dictionary["hasBackButton"] = "false"; dictionary["inputType"] = "telephoneKeypad"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["maximumRenderedPageSize"] = "1397"; dictionary["mobileDeviceManufacturer"] = "Nokia"; dictionary["numberOfSoftkeys"] = "2"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml11"; dictionary["rendersBreaksAfterWmlAnchor"] = "true"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "false"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresPhoneNumbersAsPlainText"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["type"] = "Nokia"; browserCaps.AddBrowser("Nokia"); this.NokiaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Nokia if (this.NokiablueprintProcess(headers, browserCaps)) { } else { if (this.NokiawapsimulatorProcess(headers, browserCaps)) { } else { if (this.NokiamobilebrowserProcess(headers, browserCaps)) { } else { if (this.Nokia7110Process(headers, browserCaps)) { } else { if (this.Nokia6220Process(headers, browserCaps)) { } else { if (this.Nokia6250Process(headers, browserCaps)) { } else { if (this.Nokia6310Process(headers, browserCaps)) { } else { if (this.Nokia6510Process(headers, browserCaps)) { } else { if (this.Nokia8310Process(headers, browserCaps)) { } else { if (this.Nokia9110iProcess(headers, browserCaps)) { } else { if (this.Nokia9110Process(headers, browserCaps)) { } else { if (this.Nokia3330Process(headers, browserCaps)) { } else { if (this.Nokia9210Process(headers, browserCaps)) { } else { if (this.Nokia9210htmlProcess(headers, browserCaps)) { } else { if (this.Nokia3590Process(headers, browserCaps)) { } else { if (this.Nokia3595Process(headers, browserCaps)) { } else { if (this.Nokia3560Process(headers, browserCaps)) { } else { if (this.Nokia3650Process(headers, browserCaps)) { } else { if (this.Nokia5100Process(headers, browserCaps)) { } else { if (this.Nokia6200Process(headers, browserCaps)) { } else { if (this.Nokia6590Process(headers, browserCaps)) { } else { if (this.Nokia6800Process(headers, browserCaps)) { } else { if (this.Nokia7650Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } } } } } } } } } } } } } } } this.NokiaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void NokiablueprintProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void NokiablueprintProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool NokiablueprintProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia\\-WAP\\-Toolkit\\/(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "false"; dictionary["cookies"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "65536"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "Blueprint Simulator"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreaksAfterWmlAnchor"] = "false"; dictionary["type"] = "Nokia WAP Toolkit"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.AddBrowser("NokiaBlueprint"); this.NokiablueprintProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.NokiablueprintProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void NokiawapsimulatorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void NokiawapsimulatorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool NokiawapsimulatorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia\\-MIT\\-Browser\\/(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderOnEventAndPrevElementsTogether"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["cookies"] = "true"; dictionary["hasBackButton"] = "true"; dictionary["inputType"] = "virtualKeyboard"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "3584"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "WAP Simulator"; dictionary["rendersBreaksAfterWmlAnchor"] = "false"; dictionary["requiresPhoneNumbersAsPlainText"] = "false"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "25"; dictionary["screenCharactersWidth"] = "32"; dictionary["screenPixelsHeight"] = "512"; dictionary["screenPixelsWidth"] = "384"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["type"] = "Nokia Mobile Internet Toolkit"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.AddBrowser("NokiaWapSimulator"); this.NokiawapsimulatorProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.NokiawapsimulatorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void NokiamobilebrowserProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void NokiamobilebrowserProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool NokiamobilebrowserProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia Mobile Browser (?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canInitiateVoiceCall"] = "false"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "25000"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = regexWorker["Mobile Browser ${browserMajorVersion}${browserMinorVersion}"]; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "application/vnd.wap.xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "true"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresPostRedirectionHandling"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "14"; dictionary["screenCharactersWidth"] = "24"; dictionary["screenPixelsHeight"] = "255"; dictionary["screenPixelsWidth"] = "180"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["supportsStyleElement"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["Nokia Mobile Browser ${browserMajorVersion}${browserMinorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.HtmlTextWriter = "System.Web.UI.XhtmlTextWriter"; browserCaps.AddBrowser("NokiaMobileBrowser"); this.NokiamobilebrowserProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.NokiamobilebrowserProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia7110ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia7110ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia7110Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia7110/1\\.0 \\((?\'versionString\'(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersi" + "on\'\\.\\w*).*)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "7110"; dictionary["optimumPageWeight"] = "800"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "22"; dictionary["screenPixelsHeight"] = "44"; dictionary["screenPixelsWidth"] = "96"; dictionary["type"] = "Nokia 7110"; dictionary["version"] = regexWorker["${versionString}"]; browserCaps.AddBrowser("Nokia7110"); this.Nokia7110ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia7110ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia6220ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia6220ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia6220Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia6210/1\\.0 \\((?\'versionString\'(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersi" + "on\'\\.\\w*).*)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "6210"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "22"; dictionary["screenPixelsHeight"] = "41"; dictionary["screenPixelsWidth"] = "96"; dictionary["type"] = "Nokia 6210"; dictionary["version"] = regexWorker["${versionString}"]; browserCaps.AddBrowser("Nokia6220"); this.Nokia6220ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia6220ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia6250ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia6250ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia6250Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia6250/1\\.0 \\((?\'versionString\'(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersi" + "on\'\\.\\w*).*)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "6250"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "22"; dictionary["screenPixelsHeight"] = "41"; dictionary["screenPixelsWidth"] = "96"; dictionary["type"] = "Nokia 6250"; dictionary["version"] = regexWorker["${versionString}"]; browserCaps.AddBrowser("Nokia6250"); this.Nokia6250ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia6250ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia6310ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia6310ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia6310Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia6310/1\\.0 \\((?\'versionString\'(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersi" + "on\'\\.\\w*).*)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderOneventAndPrevElementsTogether"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["cookies"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "2800"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "6310"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreaksAfterWmlAnchor"] = "false"; dictionary["rendersBreaksAfterWmlInput"] = "false"; dictionary["requiresPhoneNumbersAsPlainText"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "45"; dictionary["screenPixelsWidth"] = "92"; dictionary["type"] = "Nokia 6310"; dictionary["version"] = regexWorker["${versionString}"]; browserCaps.AddBrowser("Nokia6310"); this.Nokia6310ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia6310ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia6510ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia6510ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia6510Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia6510/1\\.0 \\((?\'versionString\'(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersi" + "on\'\\.\\w*).*)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderOnEventAndPrevElementsTogether"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["cookies"] = "true"; dictionary["hasBackButton"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "2800"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "6510"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingType"] = "wml12"; dictionary["requiresPhoneNumbersAsPlainText"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "45"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["type"] = "Nokia 6510"; dictionary["version"] = regexWorker["${versionString}"]; browserCaps.AddBrowser("Nokia6510"); this.Nokia6510ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia6510ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia8310ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia8310ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia8310Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia8310/1\\.0 \\((?\'versionString\'(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersi" + "on\'\\.\\w*).*)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderOneventAndPrevElementsTogether"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "2700"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "8310"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreaksAfterWmlAnchor"] = "false"; dictionary["rendersBreaksAfterWmlInput"] = "false"; dictionary["requiresPhoneNumbersAsPlainText"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "3"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "39"; dictionary["screenPixelsWidth"] = "78"; dictionary["type"] = "Nokia 8310"; dictionary["version"] = regexWorker["${versionString}"]; browserCaps.AddBrowser("Nokia8310"); this.Nokia8310ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia8310ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia9110iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia9110iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia9110iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia9110/1\\.0"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["maximumRenderedPageSize"] = "8192"; dictionary["mobileDeviceModel"] = "9110i Communicator"; dictionary["rendersBreaksAfterWmlAnchor"] = "false"; dictionary["screenBitDepth"] = "4"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "60"; dictionary["screenPixelsHeight"] = "180"; dictionary["screenPixelsWidth"] = "400"; dictionary["type"] = "Nokia 9110"; browserCaps.AddBrowser("Nokia9110i"); this.Nokia9110iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia9110iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia9110ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia9110ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia9110Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia-9110"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "false"; dictionary["canSendMail"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["maximumRenderedPageSize"] = "150000"; dictionary["mobileDeviceModel"] = "Nokia 9110"; dictionary["numberOfSoftkeys"] = "0"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "html32"; dictionary["rendersBreaksAfterHtmlLists"] = "false"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "11"; dictionary["screenCharactersWidth"] = "57"; dictionary["screenPixelsHeight"] = "200"; dictionary["screenPixelsWidth"] = "540"; dictionary["supportsAccesskeyAttribute"] = "true"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsBold"] = "true"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsSelectMultiple"] = "false"; dictionary["tables"] = "true"; dictionary["type"] = "Nokia 9110"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("Nokia9110"); this.Nokia9110ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia9110ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia3330ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia3330ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia3330Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia3330/1\\.0 \\((?\'versionString\'(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersi" + "on\'\\.\\w*).*)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hasBackButton"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "2800"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "3330"; dictionary["screenCharactersHeight"] = "3"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "39"; dictionary["screenPixelsWidth"] = "78"; dictionary["type"] = "Nokia 3330"; dictionary["version"] = regexWorker["${versionString}"]; browserCaps.AddBrowser("Nokia3330"); this.Nokia3330ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia3330ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia9210ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia9210ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia9210Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia9210/1\\.0"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "8192"; dictionary["mobileDeviceModel"] = "9210 Communicator"; dictionary["rendersBreaksAfterWmlAnchor"] = "false"; dictionary["rendersBreaksAfterWmlInput"] = "false"; dictionary["requiresNoSoftkeyLabels"] = "true"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "75"; dictionary["screenPixelsHeight"] = "165"; dictionary["screenPixelsWidth"] = "490"; dictionary["supportsCacheControlMetaTag"] = "false"; dictionary["type"] = "Nokia 9210"; browserCaps.AddBrowser("Nokia9210"); this.Nokia9210ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia9210ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia9210htmlProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia9210htmlProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia9210htmlProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "EPOC32-WTL/2\\.2 Crystal/6\\.0 STNC-WTL/"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; browserCaps.AddBrowser("Nokia9210HTML"); this.Nokia9210htmlProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia9210htmlProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia3590ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia3590ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia3590Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Nokia3590/(?\'version\'(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*)\\S*" + ")"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "3200"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "3590"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "true"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsBold"] = "true"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "Nokia 3590"; dictionary["version"] = regexWorker["${version}"]; browserCaps.AddBrowser("Nokia3590"); this.Nokia3590ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Nokia3590 if (this.Nokia3590v1Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Nokia3590ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia3590v1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia3590v1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia3590v1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia3590/1\\.0\\(7\\."); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "8020"; dictionary["preferredRequestEncoding"] = "iso-8859-1"; dictionary["preferredResponseEncoding"] = "utf-8"; dictionary["screenPixelsHeight"] = "65"; dictionary["supportsCss"] = "true"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsTitleElement"] = "true"; dictionary["tables"] = "false"; browserCaps.AddBrowser("Nokia3590V1"); this.Nokia3590v1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia3590v1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia3595ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia3595ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia3595Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia3595/(?\'version\'(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*)\\S*)" + ""); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "15700"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "3595"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["preferredRequestEncoding"] = "iso-8859-1"; dictionary["preferredResponseEncoding"] = "utf-8"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersWidth"] = "17"; dictionary["screenPixelsHeight"] = "132"; dictionary["screenPixelsWidth"] = "176"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "Nokia 3595"; dictionary["version"] = regexWorker["${version}"]; browserCaps.AddBrowser("Nokia3595"); this.Nokia3595ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia3595ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia3560ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia3560ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia3560Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia3560"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "28"; dictionary["screenPixelsHeight"] = "176"; dictionary["screenPixelsWidth"] = "208"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("Nokia3560"); this.Nokia3560ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia3560ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia3650ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia3650ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia3650Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia3650/(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*).* Series60/(?\'" + "platformVersion\'\\S*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "3650"; dictionary["mobilePlatformVersion"] = regexWorker["${platformVersion}"]; dictionary["type"] = "Nokia 3650"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.AddBrowser("Nokia3650"); this.Nokia3650ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Nokia3650 if (this.Nokia3650p12plusProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Nokia3650ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia3650p12plusProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia3650p12plusProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia3650p12plusProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobilePlatformVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "[^01]\\.|1\\.[^01]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "28"; dictionary["screenPixelsHeight"] = "176"; dictionary["screenPixelsWidth"] = "208"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; dictionary["tagwriter"] = "System.Web.UI.XhtmlTextWriter"; browserCaps.AddBrowser("Nokia3650P12Plus"); this.Nokia3650p12plusProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia3650p12plusProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia5100ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia5100ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia5100Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia5100/(?\'version\'(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*)\\S*)" + ""); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderOnEventAndPrevElementsTogether"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["isColor"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "3500"; dictionary["maximumSoftkeyLabelLength"] = "14"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "5100"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["rendersWmlSelectsAsMenuCards"] = "true"; dictionary["requiresPhoneNumbersAsPlainText"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "15"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "128"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["type"] = "Nokia 5100"; dictionary["version"] = regexWorker["${version}"]; browserCaps.AddBrowser("Nokia5100"); this.Nokia5100ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia5100ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia6200ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia6200ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia6200Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia6200/(?\'version\'(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*)\\S*)" + ""); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "6200"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["preferredRequestEncoding"] = "iso-8859-1"; dictionary["preferredResponseEncoding"] = "utf-8"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "19"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "128"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsNoWrapStyle"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "Nokia 6200"; dictionary["version"] = regexWorker["${version}"]; browserCaps.AddBrowser("Nokia6200"); this.Nokia6200ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia6200ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia6590ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia6590ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia6590Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Nokia6590/(?\'versionString\'(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\" + "d*)\\S*) "); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "9800"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "6590"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "true"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsBold"] = "true"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "Nokia 6590"; dictionary["version"] = regexWorker["${versionString}"]; browserCaps.AddBrowser("Nokia6590"); this.Nokia6590ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia6590ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia6800ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia6800ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia6800Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia6800/(?\'version\'(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*)\\S*)" + ""); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderOnEventAndPrevElementsTogether"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["hasBackButton"] = "true"; dictionary["isColor"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "3500"; dictionary["maximumSoftkeyLabelLength"] = "14"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "6800"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingType"] = "wml12"; dictionary["requiresPhoneNumbersAsPlainText"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "15"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "128"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["type"] = "Nokia 6800"; dictionary["version"] = regexWorker["${version}"]; browserCaps.AddBrowser("Nokia6800"); this.Nokia6800ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia6800ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia7650ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia7650ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia7650Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Nokia7650/(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*).*"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderOnEventAndPrevElementsTogether"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["cookies"] = "true"; dictionary["hasBackButton"] = "true"; dictionary["isColor"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "3500"; dictionary["maximumSoftkeyLabelLength"] = "18"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "7650"; dictionary["numberOfSoftkeys"] = "3"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingType"] = "wml12"; dictionary["requiresPhoneNumbersAsPlainText"] = "false"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "28"; dictionary["screenPixelsHeight"] = "208"; dictionary["screenPixelsWidth"] = "176"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["type"] = "Nokia 7650"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.AddBrowser("Nokia7650"); this.Nokia7650ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia7650ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void NokiamobilebrowserrainbowProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void NokiamobilebrowserrainbowProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool NokiamobilebrowserrainbowProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Rainbow/(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Nokia"; dictionary["canInitiateVoiceCall"] = "false"; dictionary["canSendMail"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["javascript"] = "false"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "25000"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceManufacturer"] = "Nokia"; dictionary["mobileDeviceModel"] = regexWorker["Mobile Browser ${browserMajorVersion}${browserMinorVersion}"]; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "application/vnd.wap.xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresInputTypeAttribute"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "14"; dictionary["screenCharactersWidth"] = "24"; dictionary["screenPixelsHeight"] = "255"; dictionary["screenPixelsWidth"] = "180"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyColor"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsDivAlign"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFontColor"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsQueryStringInFormAction"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["supportsStyleElement"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["Nokia Mobile Browser ${browserMajorVersion}${browserMinorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.XhtmlTextWriter"; browserCaps.AddBrowser("NokiaMobileBrowserRainbow"); this.NokiamobilebrowserrainbowProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.NokiamobilebrowserrainbowProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokiaepoc32wtlProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokiaepoc32wtlProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokiaepoc32wtlProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "EPOC32-WTL/(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "EPOC"; dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["canSendMail"] = "false"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["maximumRenderedPageSize"] = "150000"; dictionary["mobileDeviceManufacturer"] = "Nokia"; dictionary["mobileDeviceModel"] = "Nokia 9210"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["rendersBreaksAfterHtmlLists"] = "false"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "54"; dictionary["screenPixelsHeight"] = "170"; dictionary["screenPixelsWidth"] = "478"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsSelectMultiple"] = "false"; dictionary["supportsEmptyStringInCookieValue"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "Nokia Epoc"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("NokiaEpoc32wtl"); this.Nokiaepoc32wtlProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=NokiaEpoc32wtl if (this.Nokiaepoc32wtl20Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Nokiaepoc32wtlProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokiaepoc32wtl20ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokiaepoc32wtl20ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokiaepoc32wtl20Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "2\\.0"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderEmptySelects"] = "false"; dictionary["canSendMail"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "false"; dictionary["maximumRenderedPageSize"] = "7168"; dictionary["mobileDeviceManufacturer"] = "Psion"; dictionary["mobileDeviceModel"] = "Series 7"; dictionary["rendersBreaksAfterHtmlLists"] = "true"; dictionary["requiresAttributeColonSubstitution"] = "false"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["requiresUniqueHtmlCheckboxNames"] = "true"; dictionary["screenCharactersHeight"] = "31"; dictionary["screenCharactersWidth"] = "69"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; dictionary["SupportsEmptyStringInCookieValue"] = "true"; browserCaps.AddBrowser("NokiaEpoc32wtl20"); this.Nokiaepoc32wtl20ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokiaepoc32wtl20ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(UP\\.Browser)|(UP/)"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "Go\\.Web"); if ((result == true)) { return false; } // Capture: header values regexWorker.ProcessRegex(((string)(browserCaps[string.Empty])), @"((?'deviceID'\S*) UP/\S* UP\.Browser/((?'browserMajorVersion'\d*)(?'browserMinorVersion'\.\d*)\S*) UP\.Link/)|((?'deviceID'\S*)/\S* UP(\.Browser)*/((?'browserMajorVersion'\d*)(?'browserMinorVersion'\.\d*)\S*))|(UP\.Browser/((?'browserMajorVersion'\d*)(?'browserMinorVersion'\.\d*)\S*)-(?'deviceID'\S*) UP\.Link/)|((?'deviceID'\S*) UP\.Browser/((?'browserMajorVersion'\d*)(?'browserMinorVersion'\.\d*)\S*) UP\.Link/)|((?'deviceID'\S*)/(?'DeviceVersion'\S*) UP/((?'browserMajorVersion'\d*)(?'browserMinorVersion'\.\d*)\S*))|((?'deviceID'\S*)/(?'DeviceVersion'\S*) UP.Browser/((?'browserMajorVersion'\d*)(?'browserMinorVersion'\.\d*)\S*))|((?'deviceID'\S*) UP.Browser/((?'browserMajorVersion'\d*)(?'browserMinorVersion'\.\d*)\S*))"); // Capabilities: set capabilities dictionary["browser"] = "Phone.com"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canSendMail"] = "false"; dictionary["deviceID"] = regexWorker["${deviceID}"]; dictionary["deviceVersion"] = regexWorker["${deviceVersion}"]; dictionary["inputType"] = "telephoneKeypad"; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "1492"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["numberOfSoftkeys"] = "2"; dictionary["optimumPageWeight"] = "700"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml11"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "false"; dictionary["rendersWmlSelectsAsMenuCards"] = "true"; dictionary["requiresFullyQualifiedRedirectUrl"] = "true"; dictionary["requiresNoescapedPostUrl"] = "true"; dictionary["requiresPostRedirectionHandling"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["type"] = regexWorker["Phone.com${browserMajorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.AddBrowser("Up"); this.UpProcessGateways(headers, browserCaps); // gateway, parent=Up this.UpdefaultscreencharactersProcess(headers, browserCaps); // gateway, parent=Up this.UpdefaultscreenpixelsProcess(headers, browserCaps); // gateway, parent=Up this.UpscreendepthProcess(headers, browserCaps); // gateway, parent=Up this.UpscreencharsProcess(headers, browserCaps); // gateway, parent=Up this.UpscreenpixelsProcess(headers, browserCaps); // gateway, parent=Up this.UpmsizeProcess(headers, browserCaps); // gateway, parent=Up this.IscolorProcess(headers, browserCaps); // gateway, parent=Up this.UpnumsoftkeysProcess(headers, browserCaps); // gateway, parent=Up this.UpsoftkeysizeProcess(headers, browserCaps); // gateway, parent=Up this.UpmaxpduProcess(headers, browserCaps); // gateway, parent=Up this.UpversionProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Up if (this.AumicProcess(headers, browserCaps)) { } else { if (this.Alcatelbe4Process(headers, browserCaps)) { } else { if (this.Alcatelbe5Process(headers, browserCaps)) { } else { if (this.Alcatelbe3Process(headers, browserCaps)) { } else { if (this.Alcatelbf3Process(headers, browserCaps)) { } else { if (this.Alcatelbf4Process(headers, browserCaps)) { } else { if (this.MotcbProcess(headers, browserCaps)) { } else { if (this.Motf5Process(headers, browserCaps)) { } else { if (this.Motd8Process(headers, browserCaps)) { } else { if (this.MotcfProcess(headers, browserCaps)) { } else { if (this.Motf6Process(headers, browserCaps)) { } else { if (this.MotbcProcess(headers, browserCaps)) { } else { if (this.MotdcProcess(headers, browserCaps)) { } else { if (this.MotpancProcess(headers, browserCaps)) { } else { if (this.Motc4Process(headers, browserCaps)) { } else { if (this.MccaProcess(headers, browserCaps)) { } else { if (this.Mot2000Process(headers, browserCaps)) { } else { if (this.Motp2kcProcess(headers, browserCaps)) { } else { if (this.MotafProcess(headers, browserCaps)) { } else { if (this.Motc2Process(headers, browserCaps)) { } else { if (this.XeniumProcess(headers, browserCaps)) { } else { if (this.Sagem959Process(headers, browserCaps)) { } else { if (this.Sgha300Process(headers, browserCaps)) { } else { if (this.Sghn100Process(headers, browserCaps)) { } else { if (this.C304saProcess(headers, browserCaps)) { } else { if (this.Sy11Process(headers, browserCaps)) { } else { if (this.St12Process(headers, browserCaps)) { } else { if (this.Sy14Process(headers, browserCaps)) { } else { if (this.Sies40Process(headers, browserCaps)) { } else { if (this.Siesl45Process(headers, browserCaps)) { } else { if (this.Sies35Process(headers, browserCaps)) { } else { if (this.Sieme45Process(headers, browserCaps)) { } else { if (this.Sies45Process(headers, browserCaps)) { } else { if (this.Gm832Process(headers, browserCaps)) { } else { if (this.Gm910iProcess(headers, browserCaps)) { } else { if (this.Mot32Process(headers, browserCaps)) { } else { if (this.Mot28Process(headers, browserCaps)) { } else { if (this.D2Process(headers, browserCaps)) { } else { if (this.PpatProcess(headers, browserCaps)) { } else { if (this.AlazProcess(headers, browserCaps)) { } else { if (this.Cdm9100Process(headers, browserCaps)) { } else { if (this.Cdm135Process(headers, browserCaps)) { } else { if (this.Cdm9000Process(headers, browserCaps)) { } else { if (this.C303caProcess(headers, browserCaps)) { } else { if (this.C311caProcess(headers, browserCaps)) { } else { if (this.C202deProcess(headers, browserCaps)) { } else { if (this.C409caProcess(headers, browserCaps)) { } else { if (this.C402deProcess(headers, browserCaps)) { } else { if (this.Ds15Process(headers, browserCaps)) { } else { if (this.Tp2200Process(headers, browserCaps)) { } else { if (this.Tp120Process(headers, browserCaps)) { } else { if (this.Ds10Process(headers, browserCaps)) { } else { if (this.R280Process(headers, browserCaps)) { } else { if (this.C201hProcess(headers, browserCaps)) { } else { if (this.S71Process(headers, browserCaps)) { } else { if (this.C302hProcess(headers, browserCaps)) { } else { if (this.C309hProcess(headers, browserCaps)) { } else { if (this.C407hProcess(headers, browserCaps)) { } else { if (this.C451hProcess(headers, browserCaps)) { } else { if (this.R201Process(headers, browserCaps)) { } else { if (this.P21Process(headers, browserCaps)) { } else { if (this.Kyocera702gProcess(headers, browserCaps)) { } else { if (this.Kyocera703gProcess(headers, browserCaps)) { } else { if (this.Kyocerac307kProcess(headers, browserCaps)) { } else { if (this.Tk01Process(headers, browserCaps)) { } else { if (this.Tk02Process(headers, browserCaps)) { } else { if (this.Tk03Process(headers, browserCaps)) { } else { if (this.Tk04Process(headers, browserCaps)) { } else { if (this.Tk05Process(headers, browserCaps)) { } else { if (this.D303kProcess(headers, browserCaps)) { } else { if (this.D304kProcess(headers, browserCaps)) { } else { if (this.Qcp2035Process(headers, browserCaps)) { } else { if (this.Qcp3035Process(headers, browserCaps)) { } else { if (this.D512Process(headers, browserCaps)) { } else { if (this.Dm110Process(headers, browserCaps)) { } else { if (this.Tm510Process(headers, browserCaps)) { } else { if (this.Lg13Process(headers, browserCaps)) { } else { if (this.P100Process(headers, browserCaps)) { } else { if (this.Lgc875fProcess(headers, browserCaps)) { } else { if (this.Lgp680fProcess(headers, browserCaps)) { } else { if (this.Lgp7800fProcess(headers, browserCaps)) { } else { if (this.Lgc840fProcess(headers, browserCaps)) { } else { if (this.Lgi2100Process(headers, browserCaps)) { } else { if (this.Lgp7300fProcess(headers, browserCaps)) { } else { if (this.Sd500Process(headers, browserCaps)) { } else { if (this.Tp1100Process(headers, browserCaps)) { } else { if (this.Tp3000Process(headers, browserCaps)) { } else { if (this.T250Process(headers, browserCaps)) { } else { if (this.Mo01Process(headers, browserCaps)) { } else { if (this.Mo02Process(headers, browserCaps)) { } else { if (this.Mc01Process(headers, browserCaps)) { } else { if (this.McccProcess(headers, browserCaps)) { } else { if (this.Mcc9Process(headers, browserCaps)) { } else { if (this.Nk00Process(headers, browserCaps)) { } else { if (this.Mai12Process(headers, browserCaps)) { } else { if (this.Ma112Process(headers, browserCaps)) { } else { if (this.Ma13Process(headers, browserCaps)) { } else { if (this.Mac1Process(headers, browserCaps)) { } else { if (this.Mat1Process(headers, browserCaps)) { } else { if (this.Sc01Process(headers, browserCaps)) { } else { if (this.Sc03Process(headers, browserCaps)) { } else { if (this.Sc02Process(headers, browserCaps)) { } else { if (this.Sc04Process(headers, browserCaps)) { } else { if (this.Sg08Process(headers, browserCaps)) { } else { if (this.Sc13Process(headers, browserCaps)) { } else { if (this.Sc11Process(headers, browserCaps)) { } else { if (this.Sec01Process(headers, browserCaps)) { } else { if (this.Sc10Process(headers, browserCaps)) { } else { if (this.Sy12Process(headers, browserCaps)) { } else { if (this.St11Process(headers, browserCaps)) { } else { if (this.Sy13Process(headers, browserCaps)) { } else { if (this.Syc1Process(headers, browserCaps)) { } else { if (this.Sy01Process(headers, browserCaps)) { } else { if (this.Syt1Process(headers, browserCaps)) { } else { if (this.Sty2Process(headers, browserCaps)) { } else { if (this.Sy02Process(headers, browserCaps)) { } else { if (this.Sy03Process(headers, browserCaps)) { } else { if (this.Si01Process(headers, browserCaps)) { } else { if (this.Sni1Process(headers, browserCaps)) { } else { if (this.Sn11Process(headers, browserCaps)) { } else { if (this.Sn12Process(headers, browserCaps)) { } else { if (this.Sn134Process(headers, browserCaps)) { } else { if (this.Sn156Process(headers, browserCaps)) { } else { if (this.Snc1Process(headers, browserCaps)) { } else { if (this.Tsc1Process(headers, browserCaps)) { } else { if (this.Tsi1Process(headers, browserCaps)) { } else { if (this.Ts11Process(headers, browserCaps)) { } else { if (this.Ts12Process(headers, browserCaps)) { } else { if (this.Ts13Process(headers, browserCaps)) { } else { if (this.Tst1Process(headers, browserCaps)) { } else { if (this.Tst2Process(headers, browserCaps)) { } else { if (this.Tst3Process(headers, browserCaps)) { } else { if (this.Ig01Process(headers, browserCaps)) { } else { if (this.Ig02Process(headers, browserCaps)) { } else { if (this.Ig03Process(headers, browserCaps)) { } else { if (this.Qc31Process(headers, browserCaps)) { } else { if (this.Qc12Process(headers, browserCaps)) { } else { if (this.Qc32Process(headers, browserCaps)) { } else { if (this.Sp01Process(headers, browserCaps)) { } else { if (this.ShProcess(headers, browserCaps)) { } else { if (this.Upg1Process(headers, browserCaps)) { } else { if (this.Opwv1Process(headers, browserCaps)) { } else { if (this.AlavProcess(headers, browserCaps)) { } else { if (this.Im1kProcess(headers, browserCaps)) { } else { if (this.Nt95Process(headers, browserCaps)) { } else { if (this.Mot2001Process(headers, browserCaps)) { } else { if (this.Motv200Process(headers, browserCaps)) { } else { if (this.Mot72Process(headers, browserCaps)) { } else { if (this.Mot76Process(headers, browserCaps)) { } else { if (this.Scp6000Process(headers, browserCaps)) { } else { if (this.Motd5Process(headers, browserCaps)) { } else { if (this.Motf0Process(headers, browserCaps)) { } else { if (this.Sgha400Process(headers, browserCaps)) { } else { if (this.Sec03Process(headers, browserCaps)) { } else { if (this.Siec3iProcess(headers, browserCaps)) { } else { if (this.Sn17Process(headers, browserCaps)) { } else { if (this.Scp4700Process(headers, browserCaps)) { } else { if (this.Sec02Process(headers, browserCaps)) { } else { if (this.Sy15Process(headers, browserCaps)) { } else { if (this.Db520Process(headers, browserCaps)) { } else { if (this.L430v03j02Process(headers, browserCaps)) { } else { if (this.OpwvsdkProcess(headers, browserCaps)) { } else { if (this.Kddica21Process(headers, browserCaps)) { } else { if (this.Kddits21Process(headers, browserCaps)) { } else { if (this.Kddisa21Process(headers, browserCaps)) { } else { if (this.Km100Process(headers, browserCaps)) { } else { if (this.Lgelx5350Process(headers, browserCaps)) { } else { if (this.Hitachip300Process(headers, browserCaps)) { } else { if (this.Sies46Process(headers, browserCaps)) { } else { if (this.Motorolav60gProcess(headers, browserCaps)) { } else { if (this.Motorolav708Process(headers, browserCaps)) { } else { if (this.Motorolav708aProcess(headers, browserCaps)) { } else { if (this.Motorolae360Process(headers, browserCaps)) { } else { if (this.Sonyericssona1101sProcess(headers, browserCaps)) { } else { if (this.Philipsfisio820Process(headers, browserCaps)) { } else { if (this.Casioa5302Process(headers, browserCaps)) { } else { if (this.Tcll668Process(headers, browserCaps)) { } else { if (this.Kddits24Process(headers, browserCaps)) { } else { if (this.Sies55Process(headers, browserCaps)) { } else { if (this.Sharpgx10Process(headers, browserCaps)) { } else { if (this.BenqathenaProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } this.UpProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpdefaultscreencharactersProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpdefaultscreencharactersProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpdefaultscreencharactersProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-SCREENCHARS"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^$"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["defaultScreenCharactersWidth"] = "15"; dictionary["defaultScreenCharactersHeight"] = "4"; this.UpdefaultscreencharactersProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpdefaultscreencharactersProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpdefaultscreenpixelsProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpdefaultscreenpixelsProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpdefaultscreenpixelsProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-SCREENPIXELS"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^$"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["defaultScreenPixelsWidth"] = "120"; dictionary["defaultScreenPixelsHeight"] = "40"; this.UpdefaultscreenpixelsProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpdefaultscreenpixelsProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpscreendepthProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpscreendepthProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpscreendepthProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-SCREENDEPTH"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'screenDepth\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["screenBitDepth"] = regexWorker["${screenDepth}"]; this.UpscreendepthProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpscreendepthProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpscreencharsProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpscreencharsProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpscreencharsProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-SCREENCHARS"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'screenCharsWidth\'[1-9]\\d*),(?\'screenCharsHeight\'[1-9]\\d*)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["screenCharactersHeight"] = regexWorker["${screenCharsHeight}"]; dictionary["screenCharactersWidth"] = regexWorker["${screenCharsWidth}"]; this.UpscreencharsProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpscreencharsProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpscreenpixelsProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpscreenpixelsProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpscreenpixelsProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-SCREENPIXELS"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'screenPixWidth\'[1-9]\\d*),(?\'screenPixHeight\'[1-9]\\d*)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["screenPixelsHeight"] = regexWorker["${screenPixHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenPixWidth}"]; this.UpscreenpixelsProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpscreenpixelsProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpmsizeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpmsizeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpmsizeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-MSIZE"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'width\'\\d+),(?\'height\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["characterHeight"] = regexWorker["${height}"]; dictionary["characterWidth"] = regexWorker["${width}"]; this.UpmsizeProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpmsizeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void IscolorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void IscolorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool IscolorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-ISCOLOR"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, ".+"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities this.IscolorProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=IsColor if (this.IscolortrueProcess(headers, browserCaps)) { } else { if (this.IscolorfalseProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.IscolorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void IscolortrueProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void IscolortrueProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool IscolortrueProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-ISCOLOR"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "1"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["isColor"] = "true"; this.IscolortrueProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.IscolortrueProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void IscolorfalseProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void IscolorfalseProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool IscolorfalseProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-ISCOLOR"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "0"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["isColor"] = "false"; this.IscolorfalseProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.IscolorfalseProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpnumsoftkeysProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpnumsoftkeysProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpnumsoftkeysProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-NUMSOFTKEYS"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'softkeys\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["numberOfSoftkeys"] = regexWorker["${softkeys}"]; this.UpnumsoftkeysProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpnumsoftkeysProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpsoftkeysizeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpsoftkeysizeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpsoftkeysizeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-SOFTKEYSIZE"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'softkeySize\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["maximumSoftkeyLabelLength"] = regexWorker["${softkeySize}"]; this.UpsoftkeysizeProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpsoftkeysizeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpmaxpduProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpmaxpduProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpmaxpduProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-MAX-PDU"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'maxDeckSize\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = regexWorker["${maxDeckSize}"]; this.UpmaxpduProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpmaxpduProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpversionProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpversionProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpversionProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches // Capabilities: set capabilities RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); dictionary["type"] = regexWorker["Phone.com ${majorVersion}.x Browser"]; this.UpversionProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=UpVersion if (this.BlazerProcess(headers, browserCaps)) { } else { if (this.Up4Process(headers, browserCaps)) { } else { if (this.Up5Process(headers, browserCaps)) { } else { if (this.Up6Process(headers, browserCaps)) { } else { if (this.Up3Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } this.UpversionProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void AumicProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void AumicProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool AumicProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "AU-MIC/(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*).*"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "AU MIC"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "true"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "17"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsSelectFollowingTable"] = "false"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["AU MIC ${browserMajorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.AddBrowser("AuMic"); this.AumicProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=AuMic if (this.Aumicv2Process(headers, browserCaps)) { } else { if (this.A500Process(headers, browserCaps)) { } else { if (this.N400Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.AumicProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Aumicv2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Aumicv2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Aumicv2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "8600"; dictionary["screenCharactersHeight"] = "9"; dictionary["supportsBold"] = "false"; browserCaps.AddBrowser("AuMicV2"); this.Aumicv2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Aumicv2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void A500ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void A500ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool A500Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["HTTP_X_WAP_PROFILE"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "\\/SPH-A500\\/"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SPH-A500"; dictionary["maximumRenderedPageSize"] = "8850"; dictionary["preferredREnderingType"] = "xhtml-basic"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsTitleElement"] = "true"; browserCaps.AddBrowser("a500"); this.A500ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.A500ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void N400ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void N400ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool N400Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["HTTP_X_WAP_PROFILE"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "\\/SPH-N400\\/"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "N400"; dictionary["maximumRenderedPageSize"] = "46750"; dictionary["preferredREnderingType"] = "xhtml-basic"; dictionary["screenBitDepth"] = "24"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsTitleElement"] = "false"; browserCaps.AddBrowser("n400"); this.N400ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.N400ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void BlazerProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void BlazerProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool BlazerProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Blazer"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Handspring Blazer"; dictionary["canInitiateVoiceCall"] = "false"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "12"; dictionary["defaultScreenPixelsHeight"] = "72"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["maximumRenderedPageSize"] = "7000"; dictionary["mobileDeviceManufacturer"] = "PalmOS-licensee"; dictionary["numberOfSoftkeys"] = "0"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "html32"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["rendersWmlSelectsAsMenuCards"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "36"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsBold"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["type"] = "Handspring Blazer"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; this.BlazerProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Blazer if (this.Blazerupg1Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.BlazerProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Blazerupg1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Blazerupg1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Blazerupg1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "UPG1 UP/\\S* \\(compatible; Blazer (?\'browserMajorVersion\'\\d+)(?\'browserMinorVersio" + "n\'\\.\\d+)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; this.Blazerupg1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Blazerupg1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Up4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Up4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Up4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "4"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["requiresAbsolutePostbackUrl"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; this.Up4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Up4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Up5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Up5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Up5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["requiresUniqueFilePathSuffix"] = "true"; this.Up5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Up5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Up6ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Up6ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Up6Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "6"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "true"; dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["supportsStyleElement"] = "true"; dictionary["type"] = regexWorker["Openwave ${majorVersion}.x Browser"]; browserCaps.HtmlTextWriter = "System.Web.UI.XhtmlTextWriter"; this.Up6ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Up6 if (this.Up61plusProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Up6ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Up61plusProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Up61plusProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Up61plusProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["minorVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^\\.[^0]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["preferredRenderingMime"] = "application/xhtml+xml"; this.Up61plusProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Up61plusProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Up3ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Up3ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Up3Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "3"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderInputAndSelectElementsTogether"] = "false"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["requiresAbsolutePostbackUrl"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["requiresUrlEncodedPostfieldValues"] = "true"; dictionary["type"] = "Phone.com 3.x Browser"; this.Up3ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Up3ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Alcatelbe4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Alcatelbe4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Alcatelbe4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Alcatel-BE4"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Alcatel"; dictionary["mobileDeviceModel"] = "301"; browserCaps.AddBrowser("AlcatelBe4"); this.Alcatelbe4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Alcatelbe4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Alcatelbe5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Alcatelbe5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Alcatelbe5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Alcatel-BE5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Alcatel"; dictionary["mobileDeviceModel"] = "501, 701"; browserCaps.AddBrowser("AlcatelBe5"); this.Alcatelbe5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=AlcatelBe5 if (this.Alcatelbe5v2Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Alcatelbe5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Alcatelbe5v2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Alcatelbe5v2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Alcatelbe5v2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "2\\.0"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "1900"; dictionary["maximumSoftkeyLabelLength"] = "0"; dictionary["mobileDeviceModel"] = "Alcatel One Touch 501"; dictionary["numberOfSoftkeys"] = "10"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["requiresNoSoftkeyLabels"] = "true"; dictionary["screenBitDepth"] = "0"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "14"; dictionary["screenPixelsHeight"] = "60"; dictionary["screenPixelsWidth"] = "91"; dictionary["supportsBold"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("AlcatelBe5v2"); this.Alcatelbe5v2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Alcatelbe5v2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Alcatelbe3ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Alcatelbe3ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Alcatelbe3Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Alcatel-BE3"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Alcatel"; dictionary["mobileDeviceModel"] = "OneTouchDB"; browserCaps.AddBrowser("AlcatelBe3"); this.Alcatelbe3ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Alcatelbe3ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Alcatelbf3ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Alcatelbf3ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Alcatelbf3Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Alcatel-BF3"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "1900"; dictionary["maximumSoftkeyLabelLength"] = "13"; dictionary["mobileDeviceManufacturer"] = "Alcatel"; dictionary["mobileDeviceModel"] = "Alcatel One Touch 311"; dictionary["numberOfSoftkeys"] = "10"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "11"; dictionary["screenPixelsHeight"] = "65"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("AlcatelBf3"); this.Alcatelbf3ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Alcatelbf3ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Alcatelbf4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Alcatelbf4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Alcatelbf4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Alcatel-BF4"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "1900"; dictionary["maximumSoftkeyLabelLength"] = "13"; dictionary["mobileDeviceManufacturer"] = "Alcatel"; dictionary["mobileDeviceModel"] = "Alcatel One Touch 511"; dictionary["numberOfSoftkeys"] = "10"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "11"; dictionary["screenPixelsHeight"] = "60"; dictionary["screenPixelsWidth"] = "89"; browserCaps.AddBrowser("AlcatelBf4"); this.Alcatelbf4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Alcatelbf4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MotcbProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MotcbProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MotcbProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-CB"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Timeport P7389"; dictionary["numberOfSoftkeys"] = "1"; browserCaps.AddBrowser("MotCb"); this.MotcbProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MotcbProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motf5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motf5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motf5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-F5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "false"; dictionary["maximumRenderedPageSize"] = "1900"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Talkabout 192"; dictionary["numberOfSoftkeys"] = "3"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "40"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("MotF5"); this.Motf5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motf5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motd8ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motd8ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motd8Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-D8"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Timeport 250/P7689"; browserCaps.AddBrowser("MotD8"); this.Motd8ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motd8ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MotcfProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MotcfProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MotcfProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-CF"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Accompli 6188"; browserCaps.AddBrowser("MotCf"); this.MotcfProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MotcfProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motf6ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motf6ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motf6Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-F6"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["maximumRenderedPageSize"] = "5000"; dictionary["maximumSoftkeyLabelLength"] = "6"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Accompli 008/6288"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlSelectsAsMenuCards"] = "false"; dictionary["screenBitDepth"] = "4"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "240"; browserCaps.AddBrowser("MotF6"); this.Motf6ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motf6ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MotbcProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MotbcProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MotbcProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-BC"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["inputType"] = "keyboard"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Accompli 009"; browserCaps.AddBrowser("MotBc"); this.MotbcProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MotbcProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MotdcProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MotdcProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MotdcProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-DC"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "V3682, V50"; browserCaps.AddBrowser("MotDc"); this.MotdcProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MotdcProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MotpancProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MotpancProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MotpancProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-PAN-C"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Timeport 270c"; browserCaps.AddBrowser("MotPanC"); this.MotpancProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MotpancProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motc4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motc4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motc4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-C4"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderMixedSelects"] = "false"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "V2288, V2282"; dictionary["supportsCacheControlMetaTag"] = "false"; browserCaps.AddBrowser("MotC4"); this.Motc4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motc4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MccaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MccaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MccaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MCCA"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Timeport 8767/ST7868"; browserCaps.AddBrowser("Mcca"); this.MccaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MccaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mot2000ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mot2000ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mot2000Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-2000"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "V60c"; browserCaps.AddBrowser("Mot2000"); this.Mot2000ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mot2000ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motp2kcProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motp2kcProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motp2kcProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-P2K-C"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "V120c"; browserCaps.AddBrowser("MotP2kC"); this.Motp2kcProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motp2kcProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MotafProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MotafProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MotafProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-AF"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Timeport 260/P7382i/P7389i"; dictionary["screenCharactersHeight"] = "4"; browserCaps.AddBrowser("MotAf"); this.MotafProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=MotAf if (this.Motaf418Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.MotafProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motaf418ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motaf418ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motaf418Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["DeviceVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "4\\.1\\.8"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["maximumRenderedPageSize"] = "1900"; dictionary["maximumSoftkeyLabelLength"] = "5"; dictionary["mobileDeviceModel"] = "Timeport 260"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "64"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsCacheControlMetaTag"] = "false"; dictionary["tables"] = "true"; browserCaps.AddBrowser("MotAf418"); this.Motaf418ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motaf418ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motc2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motc2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motc2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-C2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["inputType"] = "keyboard"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "V100, V.Box"; browserCaps.AddBrowser("MotC2"); this.Motc2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motc2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void XeniumProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void XeniumProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool XeniumProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Philips-Xenium9@9"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Philips"; dictionary["mobileDeviceModel"] = "Xenium 9"; browserCaps.AddBrowser("Xenium"); this.XeniumProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.XeniumProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sagem959ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sagem959ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sagem959Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Sagem-959"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sagem"; dictionary["mobileDeviceModel"] = "MW-959"; browserCaps.AddBrowser("Sagem959"); this.Sagem959ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sagem959ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sgha300ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sgha300ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sgha300Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SAMSUNG-SGH-A300"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2000"; dictionary["maximumSoftkeyLabelLength"] = "19"; dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SGH-A300"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "13"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "128"; browserCaps.AddBrowser("SghA300"); this.Sgha300ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sgha300ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sghn100ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sghn100ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sghn100Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Samsung-SGH-N100/"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SGH-N100"; browserCaps.AddBrowser("SghN100"); this.Sghn100ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sghn100ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C304saProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C304saProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C304saProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Sanyo-C304SA/"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "C304SA"; browserCaps.AddBrowser("C304sa"); this.C304saProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C304saProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sy11ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sy11ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sy11Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SY11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "C304SA"; browserCaps.AddBrowser("Sy11"); this.Sy11ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sy11ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void St12ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void St12ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool St12Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "ST12"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "C411ST"; browserCaps.AddBrowser("St12"); this.St12ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.St12ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sy14ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sy14ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sy14Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SY14"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "C412SA"; browserCaps.AddBrowser("Sy14"); this.Sy14ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sy14ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sies40ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sies40ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sies40Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SIE-S40"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["maximumRenderedPageSize"] = "2048"; dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "S40, S42"; browserCaps.AddBrowser("SieS40"); this.Sies40ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sies40ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Siesl45ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Siesl45ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Siesl45Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SIE-SL45"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "SL-45"; browserCaps.AddBrowser("SieSl45"); this.Siesl45ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Siesl45ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sies35ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sies35ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sies35Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SIE-S35"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderMixedSelects"] = "false"; dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "S35"; browserCaps.AddBrowser("SieS35"); this.Sies35ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sies35ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sieme45ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sieme45ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sieme45Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SIE-ME45"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2800"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "ME45"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "65"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; browserCaps.AddBrowser("SieMe45"); this.Sieme45ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sieme45ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sies45ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sies45ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sies45Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SIE-S45"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2765"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "S45"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "64"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; browserCaps.AddBrowser("SieS45"); this.Sies45ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sies45ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Gm832ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Gm832ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Gm832Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "GM832"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Telit"; dictionary["mobileDeviceModel"] = "GM832"; browserCaps.AddBrowser("Gm832"); this.Gm832ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Gm832ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Gm910iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Gm910iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Gm910iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Telit-GM910i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Telit"; dictionary["mobileDeviceModel"] = "GM910i"; browserCaps.AddBrowser("Gm910i"); this.Gm910iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Gm910iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mot32ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mot32ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mot32Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-32"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "i85s, i50sx"; browserCaps.AddBrowser("Mot32"); this.Mot32ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mot32ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mot28ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mot28ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mot28Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-28"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "i700+, i1000+"; browserCaps.AddBrowser("Mot28"); this.Mot28ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mot28ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void D2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void D2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool D2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["mobileDeviceModel"] = "D2"; browserCaps.AddBrowser("D2"); this.D2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.D2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PpatProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PpatProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PpatProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P-PAT"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["mobileDeviceModel"] = "P-PAT"; browserCaps.AddBrowser("PPat"); this.PpatProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PpatProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void AlazProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void AlazProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool AlazProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "ALAZ"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Alcatel"; dictionary["mobileDeviceModel"] = "OneTouch"; browserCaps.AddBrowser("Alaz"); this.AlazProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.AlazProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Cdm9100ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Cdm9100ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Cdm9100Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "AUDIOVOX-CDM9100"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Audiovox"; dictionary["mobileDeviceModel"] = "CDM-9100"; browserCaps.AddBrowser("Cdm9100"); this.Cdm9100ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Cdm9100ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Cdm135ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Cdm135ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Cdm135Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HD-MMD1010"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Audiovox"; dictionary["mobileDeviceModel"] = "CDM-135"; browserCaps.AddBrowser("Cdm135"); this.Cdm135ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Cdm135ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Cdm9000ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Cdm9000ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Cdm9000Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TSCA"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Audiovox"; dictionary["mobileDeviceModel"] = "CDM-9000"; browserCaps.AddBrowser("Cdm9000"); this.Cdm9000ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Cdm9000ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C303caProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C303caProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C303caProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "CA11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Casio"; dictionary["mobileDeviceModel"] = "C303CA"; browserCaps.AddBrowser("C303ca"); this.C303caProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C303caProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C311caProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C311caProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C311caProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "CA12"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Casio"; dictionary["mobileDeviceModel"] = "C311CA"; browserCaps.AddBrowser("C311ca"); this.C311caProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C311caProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C202deProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C202deProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C202deProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "DN01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Casio"; dictionary["mobileDeviceModel"] = "C202DE"; browserCaps.AddBrowser("C202de"); this.C202deProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C202deProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C409caProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C409caProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C409caProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "CA13"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Casio"; dictionary["mobileDeviceModel"] = "C409CA"; browserCaps.AddBrowser("C409ca"); this.C409caProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C409caProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C402deProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C402deProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C402deProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "DN11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Denso"; dictionary["mobileDeviceModel"] = "C402DE"; browserCaps.AddBrowser("C402de"); this.C402deProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C402deProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ds15ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ds15ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ds15Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "DS15"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Denso"; dictionary["mobileDeviceModel"] = "Touchpoint DS15"; browserCaps.AddBrowser("Ds15"); this.Ds15ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ds15ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tp2200ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tp2200ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tp2200Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "DS1[34]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Denso"; dictionary["mobileDeviceModel"] = "TouchPoint TP2200"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "15"; browserCaps.AddBrowser("Tp2200"); this.Tp2200ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tp2200ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tp120ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tp120ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tp120Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "DS12"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Denso"; dictionary["mobileDeviceModel"] = "TouchPoint TP120"; browserCaps.AddBrowser("Tp120"); this.Tp120ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tp120ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ds10ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ds10ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ds10Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "DS10"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Denso"; dictionary["mobileDeviceModel"] = "Eagle 10"; browserCaps.AddBrowser("Ds10"); this.Ds10ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ds10ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void R280ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void R280ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool R280Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "ERK0"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Ericsson"; dictionary["mobileDeviceModel"] = "R280"; browserCaps.AddBrowser("R280"); this.R280ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.R280ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C201hProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C201hProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C201hProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HI01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hitachi"; dictionary["mobileDeviceModel"] = "C201H"; browserCaps.AddBrowser("C201h"); this.C201hProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C201hProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void S71ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void S71ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool S71Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HW01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hanwha"; dictionary["mobileDeviceModel"] = "S71"; browserCaps.AddBrowser("S71"); this.S71ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.S71ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C302hProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C302hProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C302hProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HI11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hitachi"; dictionary["mobileDeviceModel"] = "C302H"; browserCaps.AddBrowser("C302h"); this.C302hProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C302hProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C309hProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C309hProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C309hProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HI12"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hitachi"; dictionary["mobileDeviceModel"] = "C309H"; browserCaps.AddBrowser("C309h"); this.C309hProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C309hProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C407hProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C407hProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C407hProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HI13"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["mobileDeviceManufacturer"] = "Hitachi"; dictionary["mobileDeviceModel"] = "C407H"; browserCaps.AddBrowser("C407h"); this.C407hProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C407hProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C451hProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C451hProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C451hProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HI14"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hitachi"; dictionary["mobileDeviceModel"] = "C451H"; browserCaps.AddBrowser("C451h"); this.C451hProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C451hProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void R201ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void R201ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool R201Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HD03"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hyundai"; dictionary["mobileDeviceModel"] = "HGC-R201"; browserCaps.AddBrowser("R201"); this.R201ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.R201ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void P21ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void P21ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool P21Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HD02"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hyundai"; dictionary["mobileDeviceModel"] = "P-21"; browserCaps.AddBrowser("P21"); this.P21ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.P21ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Kyocera702gProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Kyocera702gProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Kyocera702gProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCI1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "702G"; browserCaps.AddBrowser("Kyocera702g"); this.Kyocera702gProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Kyocera702gProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Kyocera703gProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Kyocera703gProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Kyocera703gProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCI2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "703G"; browserCaps.AddBrowser("Kyocera703g"); this.Kyocera703gProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Kyocera703gProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Kyocerac307kProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Kyocerac307kProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Kyocerac307kProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KC11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "C307K"; browserCaps.AddBrowser("KyoceraC307k"); this.Kyocerac307kProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Kyocerac307kProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tk01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tk01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tk01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCT1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "TK01"; browserCaps.AddBrowser("Tk01"); this.Tk01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tk01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tk02ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tk02ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tk02Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCT2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "TK02"; browserCaps.AddBrowser("Tk02"); this.Tk02ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tk02ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tk03ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tk03ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tk03Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCT4"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "TK03"; browserCaps.AddBrowser("Tk03"); this.Tk03ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tk03ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tk04ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tk04ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tk04Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCT5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "TK04"; browserCaps.AddBrowser("Tk04"); this.Tk04ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tk04ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tk05ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tk05ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tk05Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCT6"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "TK05"; browserCaps.AddBrowser("Tk05"); this.Tk05ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tk05ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void D303kProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void D303kProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool D303kProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCC1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "D303K"; browserCaps.AddBrowser("D303k"); this.D303kProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.D303kProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void D304kProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void D304kProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool D304kProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCC2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "D304K"; browserCaps.AddBrowser("D304k"); this.D304kProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.D304kProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Qcp2035ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Qcp2035ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Qcp2035Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "QC06"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "QCP2035/2037"; browserCaps.AddBrowser("Qcp2035"); this.Qcp2035ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Qcp2035ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Qcp3035ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Qcp3035ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Qcp3035Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "QC07"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "QCP3035"; browserCaps.AddBrowser("Qcp3035"); this.Qcp3035ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Qcp3035ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void D512ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void D512ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool D512Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG22"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "D-512"; browserCaps.AddBrowser("D512"); this.D512ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.D512ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Dm110ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Dm110ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Dm110Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG05"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "DM-110"; browserCaps.AddBrowser("Dm110"); this.Dm110ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Dm110ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tm510ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tm510ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tm510Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG21"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderPostBackCards"] = "false"; dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "TM-510"; browserCaps.AddBrowser("Tm510"); this.Tm510ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tm510ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lg13ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lg13ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lg13Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG13"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "DM-510"; browserCaps.AddBrowser("Lg13"); this.Lg13ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lg13ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void P100ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void P100ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool P100Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "P-100"; browserCaps.AddBrowser("P100"); this.P100ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.P100ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lgc875fProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lgc875fProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lgc875fProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG07"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "LGC-875F"; browserCaps.AddBrowser("Lgc875f"); this.Lgc875fProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lgc875fProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lgp680fProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lgp680fProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lgp680fProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG03"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "LGP-6800F"; browserCaps.AddBrowser("Lgp680f"); this.Lgp680fProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lgp680fProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lgp7800fProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lgp7800fProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lgp7800fProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG04"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "LGP-7800F"; browserCaps.AddBrowser("Lgp7800f"); this.Lgp7800fProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lgp7800fProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lgc840fProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lgc840fProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lgc840fProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG09"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "LGC-840F"; browserCaps.AddBrowser("Lgc840f"); this.Lgc840fProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lgc840fProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lgi2100ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lgi2100ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lgi2100Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG02"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "LGI-2100"; browserCaps.AddBrowser("Lgi2100"); this.Lgi2100ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lgi2100ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lgp7300fProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lgp7300fProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lgp7300fProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "LGP-7300F"; browserCaps.AddBrowser("Lgp7300f"); this.Lgp7300fProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lgp7300fProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sd500ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sd500ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sd500Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG10"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "SD-500"; browserCaps.AddBrowser("Sd500"); this.Sd500ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sd500ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tp1100ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tp1100ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tp1100Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG06"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "Touchpoint TP1100"; browserCaps.AddBrowser("Tp1100"); this.Tp1100ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tp1100ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tp3000ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tp3000ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tp3000Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG08"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderAfterInputOrSelectElement"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "Touchpoint TP3000"; browserCaps.AddBrowser("Tp3000"); this.Tp3000ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tp3000ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void T250ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void T250ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool T250Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "T250"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["mobileDeviceModel"] = "T250"; browserCaps.AddBrowser("T250"); this.T250ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.T250ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mo01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mo01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mo01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MO01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "i500+, i700+, i1000+"; browserCaps.AddBrowser("Mo01"); this.Mo01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mo01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mo02ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mo02ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mo02Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MO02"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "i2000+"; browserCaps.AddBrowser("Mo02"); this.Mo02ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mo02ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mc01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mc01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mc01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MC01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "StarTac ST786x, Talkabout T816x, Timeport P816x"; browserCaps.AddBrowser("Mc01"); this.Mc01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mc01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void McccProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void McccProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool McccProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MCCC"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Talkabout V2267"; browserCaps.AddBrowser("Mccc"); this.McccProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.McccProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mcc9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mcc9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mcc9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MCC9"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Talkabout V8162"; browserCaps.AddBrowser("Mcc9"); this.Mcc9ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mcc9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nk00ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nk00ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nk00Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "NK00"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2252"; dictionary["maximumSoftkeyLabelLength"] = "6"; dictionary["mobileDeviceManufacturer"] = "Nokia"; dictionary["mobileDeviceModel"] = "nokia 3285"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["screenCharactersWidth"] = "15"; dictionary["supportsBold"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Nk00"); this.Nk00ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nk00ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mai12ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mai12ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mai12Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MAI[12]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["mobileDeviceModel"] = "704G"; browserCaps.AddBrowser("Mai12"); this.Mai12ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mai12ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ma112ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ma112ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ma112Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MA1[12]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["mobileDeviceModel"] = "C308P"; browserCaps.AddBrowser("Ma112"); this.Ma112ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ma112ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ma13ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ma13ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ma13Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MA13"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["mobileDeviceModel"] = "C408P"; browserCaps.AddBrowser("Ma13"); this.Ma13ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ma13ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mac1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mac1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mac1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MAC1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["mobileDeviceModel"] = "D305P"; browserCaps.AddBrowser("Mac1"); this.Mac1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mac1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mat1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mat1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mat1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MAT1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["mobileDeviceModel"] = "TP01"; browserCaps.AddBrowser("Mat1"); this.Mat1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mat1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sc01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sc01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sc01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SC01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SCH-3500"; browserCaps.AddBrowser("Sc01"); this.Sc01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sc01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sc03ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sc03ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sc03Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SC03"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SCH-6100"; browserCaps.AddBrowser("Sc03"); this.Sc03ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sc03ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sc02ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sc02ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sc02Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SC02"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SCH-8500"; browserCaps.AddBrowser("Sc02"); this.Sc02ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sc02ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sc04ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sc04ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sc04Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SC04"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SCH-850"; browserCaps.AddBrowser("Sc04"); this.Sc04ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sc04ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sg08ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sg08ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sg08Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SG08"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SGH-800"; browserCaps.AddBrowser("Sg08"); this.Sg08ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sg08ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sc13ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sc13ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sc13Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SC13"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "Uproar M100"; browserCaps.AddBrowser("Sc13"); this.Sc13ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sc13ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sc11ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sc11ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sc11Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SC11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SCH-N105"; browserCaps.AddBrowser("Sc11"); this.Sc11ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sc11ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sec01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sec01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sec01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SEC01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SCH-U03"; browserCaps.AddBrowser("Sec01"); this.Sec01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sec01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sc10ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sc10ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sc10Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SC10"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SCH-U02"; browserCaps.AddBrowser("Sc10"); this.Sc10ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sc10ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sy12ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sy12ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sy12Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SY12"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "C401SA"; browserCaps.AddBrowser("Sy12"); this.Sy12ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sy12ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void St11ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void St11ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool St11Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "ST11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "C403ST"; browserCaps.AddBrowser("St11"); this.St11ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.St11ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sy13ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sy13ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sy13Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SY13"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "C405SA"; browserCaps.AddBrowser("Sy13"); this.Sy13ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sy13ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Syc1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Syc1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Syc1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SYC1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "D301SA"; browserCaps.AddBrowser("Syc1"); this.Syc1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Syc1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sy01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sy01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sy01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SY01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "SCP-4000"; browserCaps.AddBrowser("Sy01"); this.Sy01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sy01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Syt1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Syt1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Syt1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SYT1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "TS01"; browserCaps.AddBrowser("Syt1"); this.Syt1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Syt1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sty2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sty2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sty2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SYT2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "TS02"; browserCaps.AddBrowser("Sty2"); this.Sty2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sty2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sy02ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sy02ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sy02Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SY02"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "SCP-4500"; browserCaps.AddBrowser("Sy02"); this.Sy02ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sy02ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sy03ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sy03ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sy03Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SY03"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "SCP-5000"; browserCaps.AddBrowser("Sy03"); this.Sy03ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sy03ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Si01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Si01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Si01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SI01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "S25"; browserCaps.AddBrowser("Si01"); this.Si01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Si01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sni1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sni1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sni1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SNI1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "705G"; browserCaps.AddBrowser("Sni1"); this.Sni1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sni1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sn11ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sn11ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sn11Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SN11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "C305SN"; browserCaps.AddBrowser("Sn11"); this.Sn11ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sn11ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sn12ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sn12ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sn12Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SN12"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "C404S"; browserCaps.AddBrowser("Sn12"); this.Sn12ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sn12ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sn134ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sn134ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sn134Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SN1[34]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "C406S"; browserCaps.AddBrowser("Sn134"); this.Sn134ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sn134ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sn156ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sn156ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sn156Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SN1[56]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "C413S"; browserCaps.AddBrowser("Sn156"); this.Sn156ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sn156ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Snc1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Snc1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Snc1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SNC1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "D306S"; browserCaps.AddBrowser("Snc1"); this.Snc1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Snc1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tsc1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tsc1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tsc1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TSC1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "D302T"; browserCaps.AddBrowser("Tsc1"); this.Tsc1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tsc1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tsi1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tsi1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tsi1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TSI1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "701G"; browserCaps.AddBrowser("Tsi1"); this.Tsi1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tsi1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ts11ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ts11ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ts11Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TS11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "C301T"; browserCaps.AddBrowser("Ts11"); this.Ts11ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ts11ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ts12ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ts12ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ts12Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TS12"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "C310T"; browserCaps.AddBrowser("Ts12"); this.Ts12ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ts12ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ts13ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ts13ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ts13Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TS13"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "C410T"; browserCaps.AddBrowser("Ts13"); this.Ts13ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ts13ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tst1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tst1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tst1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TST1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "TT01"; browserCaps.AddBrowser("Tst1"); this.Tst1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tst1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tst2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tst2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tst2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TST2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "TT02"; browserCaps.AddBrowser("Tst2"); this.Tst2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tst2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tst3ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tst3ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tst3Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TST3"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "TT03"; browserCaps.AddBrowser("Tst3"); this.Tst3ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tst3ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ig01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ig01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ig01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "IG01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "NeoPoint"; dictionary["mobileDeviceModel"] = "NP1000"; browserCaps.AddBrowser("Ig01"); this.Ig01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ig01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ig02ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ig02ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ig02Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "IG02"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "NeoPoint"; dictionary["mobileDeviceModel"] = "NP1660"; browserCaps.AddBrowser("Ig02"); this.Ig02ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ig02ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ig03ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ig03ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ig03Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "IG03"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "NeoPoint"; dictionary["mobileDeviceModel"] = "NP2000"; browserCaps.AddBrowser("Ig03"); this.Ig03ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ig03ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Qc31ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Qc31ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Qc31Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "QC31"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Qualcomm"; dictionary["mobileDeviceModel"] = "QCP-860, QCP-1960"; browserCaps.AddBrowser("Qc31"); this.Qc31ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Qc31ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Qc12ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Qc12ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Qc12Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "QC12"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Qualcomm"; dictionary["mobileDeviceModel"] = "QCP-1900, QCP-2700"; browserCaps.AddBrowser("Qc12"); this.Qc12ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Qc12ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Qc32ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Qc32ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Qc32Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "QC32"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Qualcomm"; dictionary["mobileDeviceModel"] = "QCP-2760"; browserCaps.AddBrowser("Qc32"); this.Qc32ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Qc32ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sp01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sp01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sp01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SP01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["mobileDeviceModel"] = "MA120"; browserCaps.AddBrowser("Sp01"); this.Sp01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sp01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void ShProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void ShProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool ShProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SH"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "Duette"; browserCaps.AddBrowser("Sh"); this.ShProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.ShProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Upg1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Upg1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Upg1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "UPG1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "false"; dictionary["mobileDeviceManufacturer"] = "OpenWave"; dictionary["mobileDeviceModel"] = "Generic Simulator"; browserCaps.AddBrowser("Upg1"); this.Upg1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Upg1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opwv1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opwv1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opwv1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "OPWV1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "false"; dictionary["inputType"] = "keyboard"; dictionary["maximumRenderedPageSize"] = "3584"; dictionary["maximumSoftkeyLabelLength"] = "9"; dictionary["mobileDeviceManufacturer"] = "Openwave"; dictionary["mobileDeviceModel"] = "Openwave 5.0 emulator"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "19"; dictionary["screenPixelsHeight"] = "188"; dictionary["screenPixelsWidth"] = "144"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; browserCaps.AddBrowser("Opwv1"); this.Opwv1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Opwv1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void AlavProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void AlavProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool AlavProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "ALAV"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Alcatel"; dictionary["mobileDeviceModel"] = "OneTouch"; browserCaps.AddBrowser("Alav"); this.AlavProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.AlavProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Im1kProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Im1kProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Im1kProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "IM1K"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "iDEN"; browserCaps.AddBrowser("Im1k"); this.Im1kProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Im1kProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nt95ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nt95ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nt95Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "NT95"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "cdmaOne"; browserCaps.AddBrowser("Nt95"); this.Nt95ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nt95ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mot2001ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mot2001ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mot2001Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-2001"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "1946"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Timeport 270c"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["requiresUrlEncodedPostfieldValues"] = "true"; dictionary["screenCharactersWidth"] = "19"; browserCaps.AddBrowser("Mot2001"); this.Mot2001ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mot2001ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motv200ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motv200ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motv200Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-v200"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hasBackButton"] = "false"; dictionary["inputType"] = "keyboard"; dictionary["maximumRenderedPageSize"] = "2000"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Motorola v200"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["requiresUrlEncodedPostfieldValues"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Motv200"); this.Motv200ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motv200ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mot72ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mot72ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mot72Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-72"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hasBackButton"] = "false"; dictionary["maximumRenderedPageSize"] = "2900"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Motorola i80s"; dictionary["numberOfSoftkeys"] = "4"; dictionary["rendersBreaksAfterWmlAnchor"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["requiresUrlEncodedPostfieldValues"] = "true"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "13"; browserCaps.AddBrowser("Mot72"); this.Mot72ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mot72ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mot76ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mot76ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mot76Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-76"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2969"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Motorola i90c"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["screenCharactersWidth"] = "14"; browserCaps.AddBrowser("Mot76"); this.Mot76ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mot76ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Scp6000ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Scp6000ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Scp6000Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Sanyo-SCP6000"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderInputAndSelectElementsTogether"] = "false"; dictionary["hasBackButton"] = "false"; dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "Sanyo SCP-6000"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["screenBitDepth"] = "1"; dictionary["screenPixelsHeight"] = "120"; dictionary["screenPixelsWidth"] = "128"; dictionary["supportsBold"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Scp6000"); this.Scp6000ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Scp6000ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motd5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motd5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motd5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-D5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2000"; dictionary["maximumSoftkeyLabelLength"] = "6"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Motorola Talkabout 191/192/193"; dictionary["numberOfSoftkeys"] = "3"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "13"; dictionary["screenPixelsHeight"] = "51"; dictionary["screenPixelsWidth"] = "91"; browserCaps.AddBrowser("MotD5"); this.Motd5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motd5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motf0ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motf0ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motf0Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-F0"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2000"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Motorola v50"; dictionary["numberOfSoftkeys"] = "3"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["requiresUrlEncodedPostfieldValues"] = "true"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "40"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("MotF0"); this.Motf0ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motf0ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sgha400ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sgha400ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sgha400Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SAMSUNG-SGH-A400"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2000"; dictionary["maximumSoftkeyLabelLength"] = "6"; dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "Samsung SGH-A400"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["requiresNoSoftkeyLabels"] = "true"; dictionary["screenCharactersHeight"] = "3"; dictionary["screenCharactersWidth"] = "13"; dictionary["screenPixelsHeight"] = "96"; dictionary["screenPixelsWidth"] = "128"; browserCaps.AddBrowser("SghA400"); this.Sgha400ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sgha400ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sec03ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sec03ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sec03Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SEC03"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "false"; dictionary["maximumRenderedPageSize"] = "3000"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "Samsung SPH-i300"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "38"; dictionary["screenPixelsHeight"] = "240"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsBold"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Sec03"); this.Sec03ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sec03ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Siec3iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Siec3iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Siec3iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SIE-C3I"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderMixedSelects"] = "false"; dictionary["maximumRenderedPageSize"] = "1900"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "C35/M35"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "false"; dictionary["requiresSpecialViewStateEncoding"] = "false"; dictionary["requiresUrlEncodedPostfieldValues"] = "false"; dictionary["screenCharactersHeight"] = "3"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "54"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsBold"] = "true"; browserCaps.AddBrowser("SieC3i"); this.Siec3iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Siec3iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sn17ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sn17ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sn17Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SN17"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["maximumRenderedPageSize"] = "12000"; dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "C1002S"; dictionary["numberOfSoftkeys"] = "3"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "120"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Sn17"); this.Sn17ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sn17ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Scp4700ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Scp4700ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Scp4700Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Sanyo-SCP4700"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "3072"; dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "Sanyo SCP 4700"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "15"; dictionary["screenPixelsHeight"] = "32"; dictionary["screenPixelsWidth"] = "91"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Scp4700"); this.Scp4700ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Scp4700ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sec02ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sec02ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sec02Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SEC02"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2867"; dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "Samsung SPH-N200"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["rendersBreaksAfterWmlAnchor"] = "true"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "15"; dictionary["screenPixelsHeight"] = "96"; dictionary["screenPixelsWidth"] = "128"; dictionary["supportsItalic"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Sec02"); this.Sec02ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sec02ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sy15ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sy15ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sy15Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SY15"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["maximumRenderedPageSize"] = "7500"; dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "Sanyo C1001SA"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "8"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Sy15"); this.Sy15ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sy15ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Db520ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Db520ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Db520Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LGE-DB520"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "3072"; dictionary["mobileDeviceManufacturer"] = "Sprint"; dictionary["mobileDeviceModel"] = "TP5200"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/wnd.wap.wml"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Db520"); this.Db520ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Db520ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void L430v03j02ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void L430v03j02ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool L430v03j02Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LGE-L430V03J02"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2900"; dictionary["maximumSoftkeyLabelLength"] = "10"; dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "LG-LP9000"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["requiresNoSoftkeyLabels"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "19"; dictionary["screenPixelsHeight"] = "133"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsBold"] = "true"; browserCaps.AddBrowser("L430V03J02"); this.L430v03j02ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.L430v03j02ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void OpwvsdkProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void OpwvsdkProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool OpwvsdkProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^OPWV-SDK"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "false"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceManufacturer"] = "Openwave"; dictionary["mobileDeviceModel"] = "Mobile Browser"; dictionary["preferredImageMime"] = "image/gif"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "9"; dictionary["screenCharactersWidth"] = "19"; dictionary["screenPixelsHeight"] = "188"; dictionary["screenPixelsWidth"] = "144"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("OPWVSDK"); this.OpwvsdkProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=OPWVSDK if (this.Opwvsdk6Process(headers, browserCaps)) { } else { if (this.Opwvsdk6plusProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.OpwvsdkProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opwvsdk6ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opwvsdk6ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opwvsdk6Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^OPWV-SDK/61"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "UP.Browser"; dictionary["canSendMail"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["mobileDeviceModel"] = "WAP Simulator 6.1"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "15"; dictionary["screenPixelsHeight"] = "108"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; browserCaps.AddBrowser("OPWVSDK6"); this.Opwvsdk6ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Opwvsdk6ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opwvsdk6plusProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opwvsdk6plusProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opwvsdk6plusProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^OPWV-SDK/(?\'modelVersion\'[7-9]|6[^01])"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Openwave"; dictionary["canSendMail"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["maximumRenderedPageSize"] = "66000"; dictionary["mobileDeviceModel"] = regexWorker["Mobile Browser ${version}"]; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresPragmaNoCacheHeader"] = "true"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsNoWrapStyle"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; browserCaps.AddBrowser("OPWVSDK6Plus"); this.Opwvsdk6plusProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Opwvsdk6plusProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Kddica21ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Kddica21ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Kddica21Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^KDDI-CA21$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "9000"; dictionary["mobileDeviceManufacturer"] = "Casio"; dictionary["mobileDeviceModel"] = "A3012CA"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "147"; dictionary["screenPixelsWidth"] = "125"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsCss"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("KDDICA21"); this.Kddica21ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Kddica21ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Kddits21ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Kddits21ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Kddits21Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KDDI-TS21"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "9000"; dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "C5001T"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "135"; dictionary["screenPixelsWidth"] = "144"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsCss"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("KDDITS21"); this.Kddits21ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Kddits21ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Kddisa21ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Kddisa21ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Kddisa21Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KDDI-SA21"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "9000"; dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "A3011SA"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "176"; dictionary["screenPixelsWidth"] = "132"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsItalic"] = "false"; dictionary["tables"] = "true"; browserCaps.AddBrowser("KDDISA21"); this.Kddisa21ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Kddisa21ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Km100ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Km100ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Km100Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KM100"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "false"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "2900"; dictionary["maximumSoftkeyLabelLength"] = "12"; dictionary["mobileDeviceManufacturer"] = "OKWap"; dictionary["mobileDeviceModel"] = "i108"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["rendersWmlSelectsAsMenuCards"] = "false"; dictionary["requiresNoSoftkeyLabels"] = "true"; dictionary["requiresPhoneNumbersAsPlainText"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("KM100"); this.Km100ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Km100ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lgelx5350ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lgelx5350ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lgelx5350Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LGE-LX5350"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "LX5350"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "108"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("LGELX5350"); this.Lgelx5350ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lgelx5350ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Hitachip300ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Hitachip300ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Hitachip300Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Hitachi-P300"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceManufacturer"] = "Hitachi"; dictionary["mobileDeviceModel"] = "SH-P300"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "17"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsFontSize"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("HitachiP300"); this.Hitachip300ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Hitachip300ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sies46ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sies46ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sies46Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SIE-S46"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hasBackButton"] = "false"; dictionary["maximumRenderedPageSize"] = "2700"; dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "S46"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "19"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsBold"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("SIES46"); this.Sies46ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sies46ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motorolav60gProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motorolav60gProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motorolav60gProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-PHX4_"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2000"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "V60G"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["requiresUrlEncodedPostfieldValues"] = "true"; dictionary["screenCharactersHeight"] = "3"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "36"; dictionary["screenPixelsWidth"] = "90"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("MotorolaV60G"); this.Motorolav60gProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motorolav60gProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motorolav708ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motorolav708ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motorolav708Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-V708_"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["maximumRenderedPageSize"] = "1900"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "V70"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "2"; dictionary["screenCharactersWidth"] = "15"; dictionary["screenPixelsHeight"] = "34"; dictionary["screenPixelsWidth"] = "90"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("MotorolaV708"); this.Motorolav708ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motorolav708ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motorolav708aProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motorolav708aProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motorolav708aProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-V708A"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderInputAndSelectElementsTogether"] = "false"; dictionary["hasBackButton"] = "false"; dictionary["maximumRenderedPageSize"] = "2000"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "V70"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["screenCharactersHeight"] = "3"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "94"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("MotorolaV708A"); this.Motorolav708aProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motorolav708aProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motorolae360ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motorolae360ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motorolae360Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Motorola-T33"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["hasBackButton"] = "false"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "3500"; dictionary["maximumSoftkeyLabelLength"] = "8"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "E360"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "27"; dictionary["screenPixelsHeight"] = "96"; dictionary["screenPixelsWidth"] = "128"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("MotorolaE360"); this.Motorolae360ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motorolae360ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sonyericssona1101sProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sonyericssona1101sProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sonyericssona1101sProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KDDI-SN22"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "9000"; dictionary["mobileDeviceManufacturer"] = "SonyEricsson"; dictionary["mobileDeviceModel"] = "A1101S"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "9"; dictionary["screenPixelsHeight"] = "123"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("SonyericssonA1101S"); this.Sonyericssona1101sProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sonyericssona1101sProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Philipsfisio820ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Philipsfisio820ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Philipsfisio820Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "PHILIPS-FISIO 820"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "3000"; dictionary["maximumSoftkeyLabelLength"] = "6"; dictionary["mobileDeviceManufacturer"] = "Philips"; dictionary["mobileDeviceModel"] = "Fisio 820"; dictionary["numberOfSoftkeys"] = "3"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["requiresNoSoftkeyLabels"] = "true"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "112"; dictionary["screenPixelsWidth"] = "112"; dictionary["supportsBold"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("PhilipsFisio820"); this.Philipsfisio820ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Philipsfisio820ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Casioa5302ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Casioa5302ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Casioa5302Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KDDI-CA22"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "9000"; dictionary["mobileDeviceManufacturer"] = "Casio"; dictionary["mobileDeviceModel"] = "A5302CA"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenPixelsHeight"] = "147"; dictionary["screenPixelsWidth"] = "132"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("CasioA5302"); this.Casioa5302ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Casioa5302ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tcll668ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tcll668ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tcll668Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Compal-Seville"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Openwave"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "3800"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "TCL"; dictionary["mobileDeviceModel"] = "L668"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "14"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "128"; dictionary["supportsBold"] = "true"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsItalic"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["type"] = regexWorker["Openwave ${majorVersion}.x Browser"]; browserCaps.AddBrowser("TCLL668"); this.Tcll668ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tcll668ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Kddits24ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Kddits24ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Kddits24Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KDDI-TS24"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Openwave"; dictionary["canSendMail"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "9000"; dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "A5304T"; dictionary["PreferredImageMime"] = "image/jpeg"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenPixelsHeight"] = "176"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsNoWrapStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsTitleElement"] = "false"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("KDDITS24"); this.Kddits24ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Kddits24ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sies55ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sies55ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sies55Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SIE-S55"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Openwave"; dictionary["canSendMail"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumHrefLength"] = "980"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "S55"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "19"; dictionary["screenPixelsHeight"] = "80"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "false"; dictionary["supportsNoWrapStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("SIES55"); this.Sies55ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sies55ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sharpgx10ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sharpgx10ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sharpgx10Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^SHARP\\-TQ\\-GX10"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Openwave"; dictionary["canSendMail"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceManufacturer"] = "Sharp"; dictionary["mobileDeviceModel"] = "GX10"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "9"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsNoWrapStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsTitleElement"] = "false"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("SHARPGx10"); this.Sharpgx10ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sharpgx10ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void BenqathenaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void BenqathenaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool BenqathenaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^BENQ\\-Athena"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Openwave"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceManufacturer"] = "BenQ"; dictionary["mobileDeviceModel"] = "S830c"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "0"; dictionary["screenPixelsWidth"] = "0"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsNoWrapStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("BenQAthena"); this.BenqathenaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.BenqathenaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void OperaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void OperaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool OperaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Opera[ /](?\'version\'(?\'major\'\\d+)(?\'minor\'\\.\\d+)(?\'letters\'\\w*))"); if ((result == false)) { return false; } // Capture: header values regexWorker.ProcessRegex(((string)(browserCaps[string.Empty])), " (?\'screenWidth\'\\d*)x(?\'screenHeight\'\\d*)"); // Capabilities: set capabilities dictionary["browser"] = "Opera"; dictionary["cookies"] = "true"; dictionary["css1"] = "true"; dictionary["css2"] = "true"; dictionary["defaultScreenCharactersHeight"] = "40"; dictionary["defaultScreenCharactersWidth"] = "80"; dictionary["defaultScreenPixelsHeight"] = "480"; dictionary["defaultScreenPixelsWidth"] = "640"; dictionary["ecmascriptversion"] = "1.1"; dictionary["frames"] = "true"; dictionary["javascript"] = "true"; dictionary["letters"] = regexWorker["${letters}"]; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "false"; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["screenBitDepth"] = "8"; dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsDivNoWrap"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["tables"] = "true"; dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["type"] = regexWorker["Opera${major}"]; dictionary["version"] = regexWorker["${version}"]; browserCaps.Adapters["System.Web.UI.WebControls.CheckBox, System.Web, Version=2.0.0.0, Culture=neutral," + " PublicKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.HideDisabledControlAdapter"; browserCaps.Adapters["System.Web.UI.WebControls.RadioButton, System.Web, Version=2.0.0.0, Culture=neutr" + "al, PublicKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.HideDisabledControlAdapter"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("Opera"); this.OperaProcessGateways(headers, browserCaps); // gateway, parent=Opera this.OperamobileProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Opera if (this.Opera1to3betaProcess(headers, browserCaps)) { } else { if (this.Opera4Process(headers, browserCaps)) { } else { if (this.Opera5to9Process(headers, browserCaps)) { } else { if (this.OperapsionProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } this.OperaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void OperamobileProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void OperamobileProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool OperamobileProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Linux \\S+embedix"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isMobileDevice"] = "true"; this.OperamobileProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.OperamobileProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opera1to3betaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opera1to3betaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opera1to3betaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["letters"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^b"); if ((result == false)) { return false; } headerValue = ((string)(dictionary["majorversion"])); result = regexWorker.ProcessRegex(headerValue, "[1-3]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; browserCaps.AddBrowser("Opera1to3beta"); this.Opera1to3betaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Opera1to3betaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opera4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opera4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opera4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "4"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.3"; dictionary["supportsFileUpload"] = "true"; dictionary["xml"] = "true"; browserCaps.AddBrowser("Opera4"); this.Opera4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Opera4 if (this.Opera4betaProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Opera4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opera4betaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opera4betaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opera4betaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["letters"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^b"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; browserCaps.AddBrowser("Opera4beta"); this.Opera4betaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Opera4betaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opera5to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opera5to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opera5to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "[5-9]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.3"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "7000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["screenBitDepth"] = "24"; dictionary["supportsFileUpload"] = "true"; dictionary["supportsFontName"] = "false"; dictionary["supportsItalic"] = "false"; dictionary["w3cdomversion"] = "1.0"; dictionary["xml"] = "true"; browserCaps.AddBrowser("Opera5to9"); this.Opera5to9ProcessGateways(headers, browserCaps); // gateway, parent=Opera5to9 this.Opera5to9betaProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Opera5to9 if (this.Opera6to9Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Opera5to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opera6to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opera6to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opera6to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "[6-9]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["backgroundsounds"] = "true"; dictionary["css1"] = "true"; dictionary["css2"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["javaapplets"] = "true"; dictionary["maximumRenderedPageSize"] = "2000000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["screenBitDepth"] = "32"; dictionary["supportsFontName"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["xml"] = "false"; browserCaps.Adapters["System.Web.UI.WebControls.CheckBox, System.Web, Version=2.0.0.0, Culture=neutral," + " PublicKeyToken=b03f5f7f11d50a3a"] = ""; browserCaps.Adapters["System.Web.UI.WebControls.RadioButton, System.Web, Version=2.0.0.0, Culture=neutr" + "al, PublicKeyToken=b03f5f7f11d50a3a"] = ""; browserCaps.Adapters["System.Web.UI.WebControls.TextBox, System.Web, Version=2.0.0.0, Culture=neutral, " + "PublicKeyToken=b03f5f7f11d50a3a"] = ""; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = ""; browserCaps.HtmlTextWriter = ""; browserCaps.AddBrowser("Opera6to9"); this.Opera6to9ProcessGateways(headers, browserCaps); // gateway, parent=Opera6to9 this.OperamobilebrowserProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Opera6to9 if (this.Opera7to9Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Opera6to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opera7to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opera7to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opera7to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "[7-9]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.4"; dictionary["supportsMaintainScrollPositionOnPostback"] = "true"; browserCaps.AddBrowser("Opera7to9"); this.Opera7to9ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Opera7to9 if (this.Opera8to9Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Opera7to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opera8to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opera8to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opera8to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "[8-9]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["supportsCallback"] = "true"; browserCaps.AddBrowser("Opera8to9"); this.Opera8to9ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Opera8to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void OperamobilebrowserProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void OperamobilebrowserProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool OperamobilebrowserProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "; (?\'screenWidth\'\\d+)x(?\'screenHeight\'\\d+)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["backgroundSounds"] = "false"; dictionary["canSendMail"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["JavaApplets"] = "false"; dictionary["maximumRenderedPageSize"] = "200000"; dictionary["msDomVersion"] = "0.0"; dictionary["preferredImageMime"] = "image/gif"; dictionary["requiredMetaTagNameValue"] = "HandheldFriendly"; dictionary["requiresPragmaNoCacheHeader"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsItalic"] = "true"; dictionary["tagwriter"] = "System.Web.UI.Html32TextWriter"; this.OperamobilebrowserProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.OperamobilebrowserProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opera5to9betaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opera5to9betaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opera5to9betaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["letters"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^b"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; this.Opera5to9betaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Opera5to9betaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void OperapsionProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void OperapsionProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool OperapsionProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Mozilla/.* \\(compatible; Opera .*; EPOC; (?\'screenWidth\'\\d*)x(?\'screenHeight\'\\d*)" + "\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["canRenderEmptySelects"] = "false"; dictionary["canSendMail"] = "false"; dictionary["css1"] = "false"; dictionary["css2"] = "false"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["maximumRenderedPageSize"] = "2560"; dictionary["mobileDeviceManufacturer"] = "Psion"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "50"; dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; dictionary["supportsBodyColor"] = "false"; dictionary["supportsCss"] = "false"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsSelectMultiple"] = "false"; dictionary["tagwriter"] = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("OperaPsion"); this.OperapsionProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.OperapsionProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MypalmProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MypalmProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MypalmProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Mozilla/2\\.0 \\(compatible; Elaine/(?\'gatewayMajorVersion\'\\w*)(?\'gatewayMinorVersi" + "on\'\\.\\w*)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "MyPalm"; dictionary["cookies"] = "false"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "12"; dictionary["defaultScreenPixelsHeight"] = "72"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["ecmascriptversion"] = "1.1"; dictionary["frames"] = "true"; dictionary["gatewayMajorVersion"] = regexWorker["${gatewayMajorVersion}"]; dictionary["gatewayMinorVersion"] = regexWorker["${gatewayMinorVersion}"]; dictionary["gatewayVersion"] = regexWorker["${gatewayMajorVersion}${gatewayMinorVersion}"]; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["javaapplets"] = "false"; dictionary["javascript"] = "false"; dictionary["mobileDeviceManufacturer"] = "PalmOS-licensee"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiredMetaTagNameValue"] = "PalmComputingPlatform"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "36"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "false"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsImageSubmit"] = "false"; dictionary["tables"] = "false"; dictionary["type"] = "MyPalm"; dictionary["vbscript"] = "false"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("Mypalm"); this.MypalmProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Mypalm if (this.Mypalm1Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.MypalmProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mypalm1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mypalm1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mypalm1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["gatewayMajorVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^1"); if ((result == false)) { return false; } headerValue = ((string)(dictionary["gatewayMinorVersion"])); result = regexWorker.ProcessRegex(headerValue, "\\.0$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "EarthLink"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "false"; dictionary["maximumRenderedPageSize"] = "7000"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["rendersBreaksAfterHtmlLists"] = "false"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["requiresUniqueHtmlCheckboxNames"] = "true"; dictionary["requiresUniqueHtmlInputNames"] = "true"; dictionary["screenBitDepth"] = "4"; dictionary["screenCharactersHeight"] = "13"; dictionary["screenCharactersWidth"] = "30"; dictionary["supportsFontSize"] = "false"; dictionary["tables"] = "true"; dictionary["type"] = "EarthLink"; browserCaps.AddBrowser("MyPalm1"); this.Mypalm1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mypalm1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PalmwebproProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PalmwebproProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PalmwebproProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "PalmOS.*WebPro"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Palm WebPro"; dictionary["canSendMail"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["javascript"] = "false"; dictionary["maximumRenderedPageSize"] = "7000"; dictionary["mobileDeviceManufacturer"] = "Palm"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiredMetaTagNameValue"] = "PalmComputingPlatform"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOutputOptimization"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "30"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "320"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsCss"] = "false"; dictionary["supportsDivAlign"] = "false"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFileUpload"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["tables"] = "false"; dictionary["type"] = "Palm WebPro"; browserCaps.AddBrowser("PalmWebPro"); this.PalmwebproProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=PalmWebPro if (this.Palmwebpro3Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.PalmwebproProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Palmwebpro3ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Palmwebpro3ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Palmwebpro3Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "WebPro3\\."); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["CanSendMail"] = "true"; dictionary["InputType"] = "keyboard"; dictionary["JavaScript"] = "true"; dictionary["MaximumRenderedPageSize"] = "520000"; dictionary["maximumHrefLength"] = "2000"; dictionary["preferredRequestEncoding"] = "ISO-8859-1"; dictionary["preferredResponseEncoding"] = "ISO-8859-1"; dictionary["RequiresControlStateInSession"] = "false"; dictionary["RequiresOutputOptimization"] = "false"; dictionary["RequiresPragmaNoCacheHeader"] = "false"; dictionary["RequiresUniqueFilePathSuffix"] = "false"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["Tables"] = "true"; browserCaps.AddBrowser("PalmWebPro3"); this.Palmwebpro3ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Palmwebpro3ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void EudorawebProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void EudorawebProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool EudorawebProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "EudoraWeb (?\'browserMajorVersion\'\\d+)(?\'browserMinorVersion\'\\.\\d+)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "EudoraWeb"; dictionary["canInitiateVoiceCall"] = "false"; dictionary["cookies"] = "true"; dictionary["isColor"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isMobileDevice"] = "true"; dictionary["javaapplets"] = "false"; dictionary["javascript"] = "false"; dictionary["maximumRenderedPageSize"] = "30000"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceManufacturer"] = "PalmOS-licensee"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "36"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "false"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsImageSubmit"] = "false"; dictionary["supportsItalic"] = "true"; dictionary["tables"] = "false"; dictionary["type"] = "EudoraWeb"; dictionary["vbscript"] = "false"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("Eudoraweb"); this.EudorawebProcessGateways(headers, browserCaps); // gateway, parent=Eudoraweb this.EudorawebmsieProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Eudoraweb if (this.PdqbrowserProcess(headers, browserCaps)) { } else { if (this.Eudoraweb21plusProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.EudorawebProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void EudorawebmsieProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void EudorawebmsieProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool EudorawebmsieProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MSIE (?\'version\'(?\'msMajorVersion\'\\d+)(?\'msMinorVersion\'\\.\\d+)(?\'letters\'\\w*))(?\'" + "extra\'[^)]*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["activexcontrols"] = "true"; dictionary["backgroundsounds"] = "true"; dictionary["ecmaScriptVersion"] = "1.2"; dictionary["frames"] = "true"; dictionary["msdomversion"] = regexWorker["${msMajorVersion}${msMinorVersion}"]; dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["w3cdomversion"] = "1.0"; this.EudorawebmsieProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.EudorawebmsieProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PdqbrowserProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PdqbrowserProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PdqbrowserProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "pdQbrowser"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "true"; dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "QCP 6035"; dictionary["supportsFontSize"] = "false"; browserCaps.AddBrowser("PdQbrowser"); this.PdqbrowserProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PdqbrowserProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Eudoraweb21plusProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Eudoraweb21plusProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Eudoraweb21plusProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "([3-9]\\.\\d+)|(2\\.[1-9]\\d*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["requiresUniqueHtmlCheckboxNames"] = "true"; dictionary["screenCharactersHeight"] = "11"; dictionary["screenCharactersWidth"] = "30"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["tables"] = "true"; browserCaps.AddBrowser("Eudoraweb21Plus"); this.Eudoraweb21plusProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Eudoraweb21plusProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PalmscapeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PalmscapeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PalmscapeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Palmscape/.*\\(v. (?\'version\'[^;]+);"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Palmscape"; dictionary["cookies"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isMobileDevice"] = "true"; dictionary["mobileDeviceManufacturer"] = "PalmOS-licensee"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "36"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsCharacterEntityEncodign"] = "false"; dictionary["type"] = "Palmscape"; dictionary["version"] = regexWorker["${version}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("Palmscape"); this.PalmscapeProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Palmscape if (this.PalmscapeversionProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.PalmscapeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PalmscapeversionProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PalmscapeversionProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PalmscapeversionProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'browserMajorVersion\'\\d+)(?\'browserMinorVersion\'\\.\\d+)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; browserCaps.AddBrowser("PalmscapeVersion"); this.PalmscapeversionProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PalmscapeversionProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void AuspalmProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void AuspalmProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool AuspalmProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "AUS PALM WAPPER"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "AU-System Demo WAP Browser"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isMobileDevice"] = "true"; dictionary["mobileDeviceManufacturer"] = "PalmOS-licensee"; dictionary["optimumPageWeight"] = "900"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml11"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "36"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsCharacterEntityEncoding"] = "true"; dictionary["type"] = "AU-System"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("AusPalm"); this.AuspalmProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.AuspalmProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SharppdaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SharppdaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SharppdaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "sharp pda browser/(?\'browserMajorVersion\'\\d+)(?\'browserMinorVersion\'\\.\\d+)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Sharp PDA Browser"; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceManufacturer"] = "Sharp"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["supportsCharacterEntityEncoding"] = "false"; dictionary["type"] = "Sharp PDA Browser"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("SharpPda"); this.SharppdaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=SharpPda if (this.Zaurusmie1Process(headers, browserCaps)) { } else { if (this.Zaurusmie21Process(headers, browserCaps)) { } else { if (this.Zaurusmie25Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.SharppdaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Zaurusmie1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Zaurusmie1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Zaurusmie1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MI-E1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["defaultCharacterHeight"] = "18"; dictionary["defaultCharacterWidth"] = "7"; dictionary["frames"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["javascript"] = "false"; dictionary["mobileDeviceModel"] = "Zaurus MI-E1"; dictionary["requiresDBCSCharacter"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenPixelsHeight"] = "240"; dictionary["screenPixelsWidth"] = "320"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("ZaurusMiE1"); this.Zaurusmie1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Zaurusmie1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Zaurusmie21ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Zaurusmie21ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Zaurusmie21Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MI-E21"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["canRenderEmptySelects"] = "false"; dictionary["cookies"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "60000"; dictionary["mobileDeviceModel"] = "Zaurus MI-E21"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresDBCSCharacter"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "18"; dictionary["screenCharactersWidth"] = "40"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "240"; dictionary["supportsFontSize"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("ZaurusMiE21"); this.Zaurusmie21ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Zaurusmie21ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Zaurusmie25ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Zaurusmie25ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Zaurusmie25Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MI-E25"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["cookies"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "60000"; dictionary["mobileDeviceModel"] = "Zaurus MI-E25DC"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["requiresDBCSCharacter"] = "true"; dictionary["requiresOutputOptimization"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "11"; dictionary["screenCharactersWidth"] = "50"; dictionary["screenPixelsHeight"] = "240"; dictionary["screenPixelsWidth"] = "320"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("ZaurusMiE25"); this.Zaurusmie25ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Zaurusmie25ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PanasonicProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PanasonicProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PanasonicProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Panasonic-(?\'deviceModel\'.*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Panasonic"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["maximumSoftkeyLabelLength"] = "16"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["mobileDeviceModel"] = regexWorker["${deviceModel}"]; dictionary["numberOfSoftkeys"] = "2"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersWmlDoAcceptsInline"] = "false"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "100"; dictionary["supportsCacheControlMetaTag"] = "false"; dictionary["tables"] = "false"; dictionary["type"] = "Panasonic"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("Panasonic"); this.PanasonicProcessGateways(headers, browserCaps); // gateway, parent=Panasonic this.PanasonicexchangesupporteddeviceProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Panasonic if (this.Panasonicgad95Process(headers, browserCaps)) { } else { if (this.Panasonicgad87Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.PanasonicProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Panasonicgad95ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Panasonicgad95ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Panasonicgad95Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "GAD95"); if ((result == false)) { return false; } // Capabilities: set capabilities browserCaps.AddBrowser("PanasonicGAD95"); this.Panasonicgad95ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Panasonicgad95ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Panasonicgad87ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Panasonicgad87ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Panasonicgad87Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "GAD87"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Panasonic"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "12000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenPixelsHeight"] = "176"; dictionary["screenPixelsWidth"] = "132"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "false"; dictionary["type"] = "Panasonic"; browserCaps.AddBrowser("PanasonicGAD87"); this.Panasonicgad87ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=PanasonicGAD87 if (this.Panasonicgad87a39Process(headers, browserCaps)) { } else { if (this.Panasonicgad87a38Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.Panasonicgad87ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PanasonicexchangesupporteddeviceProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PanasonicexchangesupporteddeviceProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PanasonicexchangesupporteddeviceProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "\\/(A3[89]|A[4-9]\\d|A\\d{3,})"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; this.PanasonicexchangesupporteddeviceProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PanasonicexchangesupporteddeviceProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Panasonicgad87a39ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Panasonicgad87a39ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Panasonicgad87a39Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "GAD87\\/A39"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceModel"] = "GD87"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "14"; dictionary["tables"] = "false"; browserCaps.AddBrowser("PanasonicGAD87A39"); this.Panasonicgad87a39ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Panasonicgad87a39ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Panasonicgad87a38ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Panasonicgad87a38ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Panasonicgad87a38Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "GAD87\\/A38"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "12155"; dictionary["mobileDeviceModel"] = "GU87"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; browserCaps.AddBrowser("PanasonicGAD87A38"); this.Panasonicgad87a38ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Panasonicgad87a38ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void WinceProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void WinceProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool WinceProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Mozilla/\\S* \\(compatible; MSIE (?\'majorVersion\'\\d*)(?\'minorVersion\'\\.\\d*);\\D* Wi" + "ndows CE(;(?\'deviceID\' \\D\\w*))?(; (?\'screenWidth\'\\d+)x(?\'screenHeight\'\\d+))?"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["activexcontrols"] = "true"; dictionary["backgroundsounds"] = "true"; dictionary["browser"] = "WinCE"; dictionary["cookies"] = "true"; dictionary["css1"] = "true"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "12"; dictionary["defaultScreenPixelsHeight"] = "72"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["deviceID"] = regexWorker["${deviceID}"]; dictionary["ecmascriptversion"] = "1.0"; dictionary["frames"] = "true"; dictionary["inputType"] = "telephoneKeypad"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["javascript"] = "true"; dictionary["jscriptversion"] = "1.0"; dictionary["majorVersion"] = regexWorker["${majorVersion}"]; dictionary["minorVersion"] = regexWorker["${minorVersion}"]; dictionary["platform"] = "WinCE"; dictionary["screenBitDepth"] = "1"; dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["tables"] = "true"; dictionary["version"] = regexWorker["${majorVersion}${minorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("WinCE"); this.WinceProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=WinCE if (this.PieProcess(headers, browserCaps)) { } else { if (this.Pie4Process(headers, browserCaps)) { } else { if (this.Pie5plusProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.WinceProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PieProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PieProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PieProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^3\\.02$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Pocket IE"; dictionary["defaultCharacterHeight"] = "18"; dictionary["defaultCharacterWidth"] = "7"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["javascript"] = "true"; dictionary["majorVersion"] = "4"; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["minorVersion"] = ".0"; dictionary["mobileDeviceModel"] = "Pocket PC"; dictionary["optimumPageWeight"] = "4000"; dictionary["requiresContentTypeMetaTag"] = "true"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["supportsBodyColor"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "false"; dictionary["supportsDivAlign"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFontColor"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["type"] = "Pocket IE"; dictionary["version"] = "4.0"; browserCaps.AddBrowser("PIE"); this.PieProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=PIE if (this.PieppcProcess(headers, browserCaps)) { } else { if (this.PienodeviceidProcess(headers, browserCaps)) { } else { if (this.PiesmartphoneProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.PieProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PieppcProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PieppcProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PieppcProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, " PPC;"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["minorVersion"] = ".1"; dictionary["version"] = "4.1"; browserCaps.AddBrowser("PIEPPC"); this.PieppcProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PieppcProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Pie4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Pie4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Pie4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MSIE 4(\\.\\d*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["activexcontrols"] = "true"; dictionary["browser"] = "Pocket IE"; dictionary["cdf"] = "true"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "12"; dictionary["defaultScreenPixelsHeight"] = "72"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["ecmascriptversion"] = "1.2"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["javaapplets"] = "true"; dictionary["maximumRenderedPageSize"] = "7000"; dictionary["msdomversion"] = "4.0"; dictionary["platform"] = "WinCE"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "9"; dictionary["screenCharactersWidth"] = "50"; dictionary["screenPixelsHeight"] = "240"; dictionary["screenPixelsWidth"] = "640"; dictionary["supportsCss"] = "true"; dictionary["supportsDivNoWrap"] = "true"; dictionary["supportsFileUpload"] = "false"; dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["type"] = "Pocket IE"; dictionary["vbscript"] = "true"; browserCaps.AddBrowser("PIE4"); this.Pie4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=PIE4 if (this.Pie4ppcProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Pie4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Pie5plusProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Pie5plusProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Pie5plusProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MSIE 5(\\.\\d*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "IE"; dictionary["ecmascriptversion"] = "1.2"; dictionary["javaapplets"] = "true"; dictionary["msdomversion"] = "5.5"; dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["type"] = "Pocket IE"; dictionary["vbscript"] = "true"; dictionary["w3cdomversion"] = "1.0"; dictionary["xml"] = "true"; browserCaps.AddBrowser("PIE5Plus"); this.Pie5plusProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=PIE5Plus if (this.Sigmarion3Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Pie5plusProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PienodeviceidProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PienodeviceidProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PienodeviceidProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["supportsQueryStringInFormAction"] = "false"; browserCaps.AddBrowser("PIEnoDeviceID"); this.PienodeviceidProcessGateways(headers, browserCaps); // gateway, parent=PIEnoDeviceID this.PiescreenbitdepthProcess(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PienodeviceidProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PiescreenbitdepthProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PiescreenbitdepthProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PiescreenbitdepthProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["UA-COLOR"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "color32"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["screenBitDepth"] = "32"; this.PiescreenbitdepthProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PiescreenbitdepthProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void NetfrontProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void NetfrontProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool NetfrontProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "\\((?\'deviceID\'.*)\\) NetFront\\/(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\" + ".\\d*).*"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Compact NetFront"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canSendMail"] = "false"; dictionary["inputType"] = "telephoneKeypad"; dictionary["isMobileDevice"] = "true"; dictionary["javascript"] = "false"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresFullyQualifiedRedirectUrl"] = "true"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFileUpload"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsImageSubmit"] = "false"; dictionary["supportsItalic"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["type"] = regexWorker["Compact NetFront ${browserMajorVersion}"]; browserCaps.AddBrowser("NetFront"); this.NetfrontProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=NetFront if (this.Slb500Process(headers, browserCaps)) { } else { if (this.VrnaProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.NetfrontProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Slb500ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Slb500ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Slb500Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SL-B500"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "NetFront"; dictionary["canInitiateVoiceCall"] = "false"; dictionary["canSendMail"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "60000"; dictionary["mobileDeviceManufacturer"] = "Sharp"; dictionary["mobileDeviceModel"] = "SL-B500"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "html32"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["requiresNoBreakInFormatting"] = "true"; dictionary["requiresOutputOptimization"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "21"; dictionary["screenCharactersWidth"] = "47"; dictionary["screenPixelsHeight"] = "240"; dictionary["screenPixelsWidth"] = "320"; dictionary["supportsAccessKeyAttribute"] = "false"; dictionary["supportsBold"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsMultilineTextboxDisplay"] = "true"; browserCaps.AddBrowser("SLB500"); this.Slb500ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Slb500ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void VrnaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void VrnaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool VrnaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "sony/model vrna"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "CLIE PEG-TG50"; dictionary["canInitiateVoiceCall"] = "false"; dictionary["canSendMail"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["javascript"] = "true"; dictionary["maximumRenderedPageSize"] = "65000"; dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "html32"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOutputOptimization"] = "true"; dictionary["requiresPragmaNoCacheHeader"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "31"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "320"; dictionary["supportsAccessKeyAttribute"] = "false"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsMultilineTextboxDisplay"] = "true"; browserCaps.AddBrowser("VRNA"); this.VrnaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.VrnaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Pie4ppcProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Pie4ppcProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Pie4ppcProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "PPC(; (?\'screenWidth\'\\d+)x(?\'screenHeight\'\\d+))?"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "MSIE"; dictionary["ecmascriptversion"] = "1.0"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["maximumRenderedPageSize"] = "800000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingType"] = "html32"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "16"; dictionary["screenCharactersWidth"] = "32"; dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "false"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["type"] = regexWorker["MSIE ${majorVersion}"]; browserCaps.AddBrowser("PIE4PPC"); this.Pie4ppcProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Pie4ppcProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PiesmartphoneProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PiesmartphoneProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PiesmartphoneProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Smartphone"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "true"; dictionary["frames"] = "false"; dictionary["inputType"] = "telephoneKeypad"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["minorVersion"] = ".1"; dictionary["mobileDeviceModel"] = "Smartphone"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["screenCharactersHeight"] = "13"; dictionary["screenCharactersWidth"] = "28"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsFontName"] = "false"; dictionary["version"] = "4.1"; browserCaps.AddBrowser("PIESmartphone"); this.PiesmartphoneProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PiesmartphoneProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sigmarion3ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sigmarion3ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sigmarion3Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "sigmarion3"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "64000"; dictionary["mobileDeviceManufacturer"] = "NTT DoCoMo"; dictionary["MobileDeviceModel"] = "Sigmarion III"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRequestEncoding"] = "shift_jis"; dictionary["preferredResponseEncoding"] = "shift_jis"; dictionary["requiresOutputOptimization"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "19"; dictionary["screenCharactersWidth"] = "94"; dictionary["screenPixelsHeight"] = "480"; dictionary["screenPixelsWidth"] = "800"; browserCaps.AddBrowser("sigmarion3"); this.Sigmarion3ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sigmarion3ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mspie06ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mspie06ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mspie06Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Microsoft Pocket Internet Explorer/0\\.6"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["backgroundsounds"] = "true"; dictionary["browser"] = "PIE"; dictionary["cookies"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["majorversion"] = "1"; dictionary["minorversion"] = "0"; dictionary["platform"] = "WinCE"; dictionary["tables"] = "true"; dictionary["type"] = "PIE"; dictionary["version"] = "1.0"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("MSPIE06"); this.Mspie06ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mspie06ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MspieProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MspieProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MspieProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Mozilla[^(]*\\(compatible; MSPIE (?\'version\'(?\'major\'\\d+)(?\'minor\'\\.\\d+)(?\'letter" + "s\'\\w*))(?\'extra\'.*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["backgroundsounds"] = "true"; dictionary["browser"] = "PIE"; dictionary["cookies"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["PIE${major}"]; dictionary["version"] = regexWorker["${version}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("MSPIE"); this.MspieProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=MSPIE if (this.Mspie2Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.MspieProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mspie2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mspie2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mspie2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "2\\."); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["frames"] = "true"; browserCaps.AddBrowser("MSPIE2"); this.Mspie2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mspie2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, @"^(?'carrier'\S{3})(?'serviceType'\d)(?'deviceType'\d)(?'deviceManufacturer'\S{2})(?'deviceID'\S{2})(?'browserType'\d{2})(?'majorVersion'\d)(?'minorVersion'\d)(?'screenWidth'\d{3})(?'screenHeight'\d{3})(?'screenColumn'\d{2})(?'screenRow'\d{2})(?'colorDepth'\d{2})(?'MINNumber'\d{8})"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browserType"] = regexWorker["${browserType}"]; dictionary["colorDepth"] = regexWorker["${colorDepth}"]; dictionary["cookies"] = "false"; dictionary["deviceManufacturer"] = regexWorker["${deviceManufacturer}"]; dictionary["deviceID"] = regexWorker["${deviceID}"]; dictionary["deviceType"] = regexWorker["${deviceType}"]; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${majorVersion}"]; dictionary["minorVersion"] = regexWorker["${minorVersion}"]; dictionary["screenColumn"] = regexWorker["${screenColumn}"]; dictionary["screenHeight"] = regexWorker["${screenHeight}"]; dictionary["screenWidth"] = regexWorker["${screenWidth}"]; dictionary["screenRow"] = regexWorker["${screenRow}"]; dictionary["version"] = regexWorker["${majorVersion}.${minorVersion}"]; browserCaps.AddBrowser("SKTDevices"); this.SktdevicesProcessGateways(headers, browserCaps); // gateway, parent=SKTDevices this.SktdevicescolordepthProcess(headers, browserCaps); // gateway, parent=SKTDevices this.SktdevicesscreenrowProcess(headers, browserCaps); // gateway, parent=SKTDevices this.SktdevicesscreencolumnProcess(headers, browserCaps); // gateway, parent=SKTDevices this.SktdevicesscreenheightProcess(headers, browserCaps); // gateway, parent=SKTDevices this.SktdevicesscreenwidthProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=SKTDevices if (this.SktdeviceshyundaiProcess(headers, browserCaps)) { } else { if (this.SktdeviceshanhwaProcess(headers, browserCaps)) { } else { if (this.SktdevicesjtelProcess(headers, browserCaps)) { } else { if (this.SktdeviceslgProcess(headers, browserCaps)) { } else { if (this.SktdevicesmotorolaProcess(headers, browserCaps)) { } else { if (this.SktdevicesnokiaProcess(headers, browserCaps)) { } else { if (this.SktdevicesskttProcess(headers, browserCaps)) { } else { if (this.SktdevicessamsungProcess(headers, browserCaps)) { } else { if (this.SktdevicesericssonProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } this.SktdevicesProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicescolordepthProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicescolordepthProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicescolordepthProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["colorDepth"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^(\\d[1-9])|([1-9]0)$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenBitDepth"] = regexWorker["${colorDepth}"]; this.SktdevicescolordepthProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=SKTDevicesColorDepth if (this.SktdevicesiscolorProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.SktdevicescolordepthProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesiscolorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesiscolorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesiscolorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["colorDepth"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^(0[5-9])|([1-9]\\d)$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; this.SktdevicesiscolorProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdevicesiscolorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesscreenrowProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesscreenrowProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesscreenrowProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["screenRow"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^(\\d[1-9])|([1-9]0)$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenCharactersHeight"] = regexWorker["${screenRow}"]; this.SktdevicesscreenrowProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdevicesscreenrowProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesscreencolumnProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesscreencolumnProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesscreencolumnProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["screenColumn"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^(\\d[1-9])|([1-9]0)$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenCharactersWidth"] = regexWorker["${screenColumn}"]; this.SktdevicesscreencolumnProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdevicesscreencolumnProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesscreenheightProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesscreenheightProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesscreenheightProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["screenHeight"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^(\\d\\d[1-9])|(((\\d[1-9])|([1-9]0))0)$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; this.SktdevicesscreenheightProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdevicesscreenheightProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesscreenwidthProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesscreenwidthProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesscreenwidthProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["screenWidth"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^(\\d\\d[1-9])|(((\\d[1-9])|([1-9]0))0)$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; this.SktdevicesscreenwidthProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdevicesscreenwidthProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdeviceshyundaiProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdeviceshyundaiProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdeviceshyundaiProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceManufacturer"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HD"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hyundai"; browserCaps.AddBrowser("SKTDevicesHyundai"); this.SktdeviceshyundaiProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=SKTDevicesHyundai if (this.Pse200Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.SktdeviceshyundaiProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Pse200ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Pse200ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Pse200Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "10"); if ((result == false)) { return false; } headerValue = ((string)(dictionary["browserType"])); result = regexWorker.ProcessRegex(headerValue, "15"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Infraware"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceManufacturer"] = "Pantech&Curitel"; dictionary["mobileDeviceModel"] = "PS-E200"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "true"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenPixelsHeight"] = "80"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsNoWrapStyle"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["${browser} ${majorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.XhtmlTextWriter"; browserCaps.AddBrowser("PSE200"); this.Pse200ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Pse200ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdeviceshanhwaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdeviceshanhwaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdeviceshanhwaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceManufacturer"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HH"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hanhwa"; browserCaps.AddBrowser("SKTDevicesHanhwa"); this.SktdeviceshanhwaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdeviceshanhwaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesjtelProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesjtelProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesjtelProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceManufacturer"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "JT"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "JTEL"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.ChtmlTextWriter"; browserCaps.AddBrowser("SKTDevicesJTEL"); this.SktdevicesjtelProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=SKTDevicesJTEL if (this.Jtel01Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.SktdevicesjtelProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jtel01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jtel01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jtel01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["inputType"] = "virtualKeyboard"; dictionary["mobileDeviceModel"] = "Cellvic XG"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "12"; browserCaps.AddBrowser("JTEL01"); this.Jtel01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=JTEL01 if (this.JtelnateProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Jtel01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JtelnateProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JtelnateProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JtelnateProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["browserType"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "03"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "NATE"; dictionary["cookies"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsBold"] = "true"; dictionary["supportsFontColor"] = "false"; dictionary["tables"] = "true"; dictionary["type"] = "NATE 1"; browserCaps.AddBrowser("JTELNate"); this.JtelnateProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JtelnateProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdeviceslgProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdeviceslgProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdeviceslgProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceManufacturer"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; browserCaps.AddBrowser("SKTDevicesLG"); this.SktdeviceslgProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdeviceslgProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesmotorolaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesmotorolaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesmotorolaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceManufacturer"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MT"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; browserCaps.AddBrowser("SKTDevicesMotorola"); this.SktdevicesmotorolaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=SKTDevicesMotorola if (this.Sktdevicesv730Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.SktdevicesmotorolaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sktdevicesv730ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sktdevicesv730ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sktdevicesv730Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "07"); if ((result == false)) { return false; } headerValue = ((string)(dictionary["browserType"])); result = regexWorker.ProcessRegex(headerValue, "00"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "AU-System"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "false"; dictionary["maximumRenderedPageSize"] = "2900"; dictionary["mobileDeviceModel"] = "V730"; dictionary["numberOfSoftkeys"] = "2"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml11"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "true"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlSelectsAsMenuCards"] = "true"; dictionary["requiresNoSoftkeyLabels"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["type"] = regexWorker["${browser} ${majorVersion}"]; browserCaps.AddBrowser("SKTDevicesV730"); this.Sktdevicesv730ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sktdevicesv730ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesnokiaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesnokiaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesnokiaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceManufacturer"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "NO"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Nokia"; browserCaps.AddBrowser("SKTDevicesNokia"); this.SktdevicesnokiaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdevicesnokiaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesskttProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesskttProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesskttProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceManufacturer"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SK"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "SKTT"; browserCaps.AddBrowser("SKTDevicesSKTT"); this.SktdevicesskttProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdevicesskttProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicessamsungProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicessamsungProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicessamsungProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceManufacturer"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SS"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; browserCaps.AddBrowser("SKTDevicesSamSung"); this.SktdevicessamsungProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=SKTDevicesSamSung if (this.Sche150Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.SktdevicessamsungProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sche150ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sche150ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sche150Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["browserType"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "15"); if ((result == false)) { return false; } headerValue = ((string)(dictionary["deviceID"])); result = regexWorker.ProcessRegex(headerValue, "50"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Infraware"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceModel"] = "SCH-E150"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenPixelsHeight"] = "80"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsNoWrapStyle"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["${browser} ${majorVersion}"]; browserCaps.AddBrowser("SCHE150"); this.Sche150ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sche150ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesericssonProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesericssonProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesericssonProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["browserType"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Ericsson"; browserCaps.AddBrowser("SKTDevicesEricsson"); this.SktdevicesericssonProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdevicesericssonProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void WebtvProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void WebtvProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool WebtvProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "WebTV/(?\'version\'(?\'major\'\\d+)(?\'minor\'\\.\\d+)(?\'letters\'\\w*))"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["backgroundsounds"] = "true"; dictionary["browser"] = "WebTV"; dictionary["cookies"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["letters"] = regexWorker["${letters}"]; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["WebTV${major}"]; dictionary["version"] = regexWorker["${version}"]; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("WebTV"); this.WebtvProcessGateways(headers, browserCaps); // gateway, parent=WebTV this.WebtvbetaProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=WebTV if (this.Webtv2Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.WebtvProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Webtv2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Webtv2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Webtv2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["minorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["css1"] = "true"; dictionary["ecmascriptversion"] = "1.0"; dictionary["isMobileDevice"] = "false"; dictionary["javascript"] = "true"; dictionary["supportsBold"] = "false"; dictionary["supportsCss"] = "false"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["supportsImageSubmit"] = "false"; dictionary["supportsItalic"] = "false"; browserCaps.AddBrowser("WebTV2"); this.Webtv2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Webtv2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void WebtvbetaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void WebtvbetaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool WebtvbetaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["letters"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^b"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; this.WebtvbetaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.WebtvbetaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void WinwapProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void WinwapProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool WinwapProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^WinWAP-(?\'platform\'\\w*)/(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*)" + ""); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "WinWAP"; dictionary["canRenderAfterInputOrSelectElement"] = "false"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "false"; dictionary["hasBackButton"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "3500"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["platform"] = regexWorker["${platform}"]; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreaksAfterWmlInput"] = "false"; dictionary["rendersWmlSelectsAsMenuCards"] = "false"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["requiresUniqueFilepathSuffix"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "16"; dictionary["screenCharactersWidth"] = "43"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "240"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["type"] = regexWorker["WinWAP ${browserMajorVersion}${browserMinorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("WinWap"); this.WinwapProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.WinwapProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void XiinoProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void XiinoProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool XiinoProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Xiino/(?\'browserMajorVersion\'\\d+)(?\'browserMinorVersion\'\\.\\d+).* (?\'screenWidth\'\\" + "d+)x(?\'screenHeight\'\\d+);"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Xiino"; dictionary["canRenderEmptySelects"] = "true"; dictionary["canSendMail"] = "false"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "65000"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresAttributeColonSubstitution"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "30"; dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; dictionary["supportsBold"] = "true"; dictionary["supportsCharacterEntityEncoding"] = "false"; dictionary["supportsFontSize"] = "true"; dictionary["type"] = "Xiino"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("Xiino"); this.XiinoProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Xiino if (this.Xiinov2Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.XiinoProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Xiinov2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Xiinov2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Xiinov2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^2\\.0$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresOutputOptimization"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["supportsItalic"] = "true"; browserCaps.AddBrowser("XiinoV2"); this.Xiinov2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Xiinov2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void DefaultDefaultProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool DefaultDefaultProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Capabilities: set capabilities dictionary["ecmascriptversion"] = "0.0"; dictionary["javascript"] = "false"; dictionary["jscriptversion"] = "0.0"; bool ignoreApplicationBrowsers = true; // browser, parent=Default if (this.DefaultWmlProcess(headers, browserCaps)) { } else { if (this.DefaultXhtmlmpProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.DefaultDefaultProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void DefaultWmlProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool DefaultWmlProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["Accept"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "text/vnd\\.wap\\.wml|text/hdml"); if ((result == false)) { return false; } headerValue = ((string)(headers["Accept"])); result = regexWorker.ProcessRegex(headerValue, "application/xhtml\\+xml; profile|application/vnd\\.wap\\.xhtml\\+xml"); if ((result == true)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml11"; bool ignoreApplicationBrowsers = false; this.DefaultWmlProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void DefaultXhtmlmpProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool DefaultXhtmlmpProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["Accept"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "application/xhtml\\+xml; profile|application/vnd\\.wap\\.xhtml\\+xml"); if ((result == false)) { return false; } headerValue = ((string)(headers["Accept"])); result = regexWorker.ProcessRegex(headerValue, "text/hdml"); if ((result == true)) { return false; } headerValue = ((string)(headers["Accept"])); result = regexWorker.ProcessRegex(headerValue, "text/vnd\\.wap\\.wml"); if ((result == true)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "xhtml-mp"; browserCaps.HtmlTextWriter = "System.Web.UI.XhtmlTextWriter"; bool ignoreApplicationBrowsers = false; this.DefaultXhtmlmpProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected override void PopulateMatchedHeaders(System.Collections.IDictionary dictionary) { base.PopulateMatchedHeaders(dictionary); dictionary["X-UP-DEVCAP-NUMSOFTKEYS"] = null; dictionary["UA-COLOR"] = null; dictionary["UA-PIXELS"] = null; dictionary["HTTP_X_WAP_PROFILE"] = null; dictionary["X-UP-DEVCAP-SCREENDEPTH"] = null; dictionary["X-UP-DEVCAP-MSIZE"] = null; dictionary["X-UP-DEVCAP-MAX-PDU"] = null; dictionary["X-UP-DEVCAP-CHARSET"] = null; dictionary["X-GA-TABLES"] = null; dictionary["X-AVANTGO-VERSION"] = null; dictionary["X-UP-DEVCAP-SOFTKEYSIZE"] = null; dictionary["UA-VOICE"] = null; dictionary["X-UP-DEVCAP-ISCOLOR"] = null; dictionary["X-JPHONE-DISPLAY"] = null; dictionary["X-UP-DEVCAP-SCREENPIXELS"] = null; dictionary["X-JPHONE-COLOR"] = null; dictionary["X-UP-DEVCAP-SCREENCHARS"] = null; dictionary["Accept"] = null; dictionary[""] = null; dictionary["VIA"] = null; dictionary["X-GA-MAX-TRANSFER"] = null; } protected override void PopulateBrowserElements(System.Collections.IDictionary dictionary) { base.PopulateBrowserElements(dictionary); dictionary["Default"] = new System.Web.UI.Triplet(null, string.Empty, 0); dictionary["Mozilla"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["IE"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["IE5to9"] = new System.Web.UI.Triplet("Ie", string.Empty, 3); dictionary["IE6to9"] = new System.Web.UI.Triplet("Ie5to9", string.Empty, 4); dictionary["Treo600"] = new System.Web.UI.Triplet("Ie6to9", string.Empty, 5); dictionary["IE5"] = new System.Web.UI.Triplet("Ie5to9", string.Empty, 4); dictionary["IE50"] = new System.Web.UI.Triplet("Ie5", string.Empty, 5); dictionary["IE55"] = new System.Web.UI.Triplet("Ie5", string.Empty, 5); dictionary["IE5to9Mac"] = new System.Web.UI.Triplet("Ie5to9", string.Empty, 4); dictionary["IE4"] = new System.Web.UI.Triplet("Ie", string.Empty, 3); dictionary["IE3"] = new System.Web.UI.Triplet("Ie", string.Empty, 3); dictionary["IE3win16"] = new System.Web.UI.Triplet("Ie3", string.Empty, 4); dictionary["IE3win16a"] = new System.Web.UI.Triplet("Ie3win16", string.Empty, 5); dictionary["IE3Mac"] = new System.Web.UI.Triplet("Ie3", string.Empty, 4); dictionary["IE2"] = new System.Web.UI.Triplet("Ie", string.Empty, 3); dictionary["WebTV"] = new System.Web.UI.Triplet("Ie2", string.Empty, 4); dictionary["WebTV2"] = new System.Web.UI.Triplet("Webtv", string.Empty, 5); dictionary["IE1minor5"] = new System.Web.UI.Triplet("Ie", string.Empty, 3); dictionary["PowerBrowser"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["Gecko"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["MozillaRV"] = new System.Web.UI.Triplet("Gecko", string.Empty, 3); dictionary["MozillaFirebird"] = new System.Web.UI.Triplet("Mozillarv", string.Empty, 4); dictionary["MozillaFirefox"] = new System.Web.UI.Triplet("Mozillarv", string.Empty, 4); dictionary["Safari"] = new System.Web.UI.Triplet("Gecko", string.Empty, 3); dictionary["Safari60"] = new System.Web.UI.Triplet("Safari", string.Empty, 4); dictionary["Safari85"] = new System.Web.UI.Triplet("Safari", string.Empty, 4); dictionary["Safari1Plus"] = new System.Web.UI.Triplet("Safari", string.Empty, 4); dictionary["Netscape5"] = new System.Web.UI.Triplet("Gecko", string.Empty, 3); dictionary["Netscape6to9"] = new System.Web.UI.Triplet("Netscape5", string.Empty, 4); dictionary["Netscape6to9Beta"] = new System.Web.UI.Triplet("Netscape6to9", string.Empty, 5); dictionary["NetscapeBeta"] = new System.Web.UI.Triplet("Netscape5", string.Empty, 4); dictionary["AvantGo"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["TMobileSidekick"] = new System.Web.UI.Triplet("Avantgo", string.Empty, 3); dictionary["GoAmerica"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["GoAmericaWinCE"] = new System.Web.UI.Triplet("Goamerica", string.Empty, 3); dictionary["GoAmericaPalm"] = new System.Web.UI.Triplet("Goamerica", string.Empty, 3); dictionary["GoAmericaRIM"] = new System.Web.UI.Triplet("Goamerica", string.Empty, 3); dictionary["GoAmericaRIM950"] = new System.Web.UI.Triplet("Goamericarim", string.Empty, 4); dictionary["GoAmericaRIM850"] = new System.Web.UI.Triplet("Goamericarim", string.Empty, 4); dictionary["GoAmericaRIM957"] = new System.Web.UI.Triplet("Goamericarim", string.Empty, 4); dictionary["GoAmericaRIM957major6minor2"] = new System.Web.UI.Triplet("Goamericarim957", string.Empty, 5); dictionary["GoAmericaRIM857"] = new System.Web.UI.Triplet("Goamericarim", string.Empty, 4); dictionary["GoAmericaRIM857major6"] = new System.Web.UI.Triplet("Goamericarim857", string.Empty, 5); dictionary["GoAmericaRIM857major6minor2to9"] = new System.Web.UI.Triplet("Goamericarim857major6", string.Empty, 6); dictionary["GoAmerica7to9"] = new System.Web.UI.Triplet("Goamericarim857", string.Empty, 5); dictionary["Netscape3"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["Netscape4"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["Casiopeia"] = new System.Web.UI.Triplet("Netscape4", string.Empty, 3); dictionary["PalmWebPro"] = new System.Web.UI.Triplet("Netscape4", string.Empty, 3); dictionary["PalmWebPro3"] = new System.Web.UI.Triplet("Palmwebpro", string.Empty, 4); dictionary["NetFront"] = new System.Web.UI.Triplet("Netscape4", string.Empty, 3); dictionary["SLB500"] = new System.Web.UI.Triplet("Netfront", string.Empty, 4); dictionary["VRNA"] = new System.Web.UI.Triplet("Netfront", string.Empty, 4); dictionary["Mypalm"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["MyPalm1"] = new System.Web.UI.Triplet("Mypalm", string.Empty, 3); dictionary["Eudoraweb"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["PdQbrowser"] = new System.Web.UI.Triplet("Eudoraweb", string.Empty, 3); dictionary["Eudoraweb21Plus"] = new System.Web.UI.Triplet("Eudoraweb", string.Empty, 3); dictionary["WinCE"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["PIE"] = new System.Web.UI.Triplet("Wince", string.Empty, 3); dictionary["PIEPPC"] = new System.Web.UI.Triplet("Pie", string.Empty, 4); dictionary["PIEnoDeviceID"] = new System.Web.UI.Triplet("Pie", string.Empty, 4); dictionary["PIESmartphone"] = new System.Web.UI.Triplet("Pie", string.Empty, 4); dictionary["PIE4"] = new System.Web.UI.Triplet("Wince", string.Empty, 3); dictionary["PIE4PPC"] = new System.Web.UI.Triplet("Pie4", string.Empty, 4); dictionary["PIE5Plus"] = new System.Web.UI.Triplet("Wince", string.Empty, 3); dictionary["sigmarion3"] = new System.Web.UI.Triplet("Pie5plus", string.Empty, 4); dictionary["MSPIE"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["MSPIE2"] = new System.Web.UI.Triplet("Mspie", string.Empty, 3); dictionary["Docomo"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["DocomoSH251i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoSH251iS"] = new System.Web.UI.Triplet("Docomosh251i", string.Empty, 3); dictionary["DocomoN251i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN251iS"] = new System.Web.UI.Triplet("Docomon251i", string.Empty, 3); dictionary["DocomoP211i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF212i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoD501i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF501i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN501i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP501i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoD502i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF502i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN502i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP502i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoNm502i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoSo502i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF502it"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN502it"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoSo502iwm"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF504i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN504i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP504i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN821i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP821i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoD209i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoEr209i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF209i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoKo209i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN209i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP209i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP209is"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoR209i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoR691i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF503i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF503is"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoD503i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoD503is"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoD210i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF210i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN210i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN2001"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoD211i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN211i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP210i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoKo210i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP2101v"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP2102v"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF211i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF671i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN503is"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN503i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoSo503i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP503is"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP503i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoSo210i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoSo503is"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoSh821i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN2002"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoSo505i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP505i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN505i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoD505i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoISIM60"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["EricssonR380"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["Ericsson"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["EricssonR320"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonT20"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonT65"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonT68"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["Ericsson301A"] = new System.Web.UI.Triplet("Ericssont68", string.Empty, 3); dictionary["EricssonT68R1A"] = new System.Web.UI.Triplet("Ericssont68", string.Empty, 3); dictionary["EricssonT68R101"] = new System.Web.UI.Triplet("Ericssont68", string.Empty, 3); dictionary["EricssonT68R201A"] = new System.Web.UI.Triplet("Ericssont68", string.Empty, 3); dictionary["EricssonT300"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonP800"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonP800R101"] = new System.Web.UI.Triplet("Ericssonp800", string.Empty, 3); dictionary["EricssonT61"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonT31"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonR520"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonA2628"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonT39"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EzWAP"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["GenericDownlevel"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["Jataayu"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["JataayuPPC"] = new System.Web.UI.Triplet("Jataayu", string.Empty, 2); dictionary["Jphone"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["JphoneMitsubishi"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphoneDenso"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphoneKenwood"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphoneNec"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphoneNecN51"] = new System.Web.UI.Triplet("Jphonenec", string.Empty, 3); dictionary["JphonePanasonic"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphonePioneer"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphoneSanyo"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphoneSA51"] = new System.Web.UI.Triplet("Jphonesanyo", string.Empty, 3); dictionary["JphoneSharp"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphoneSharpSh53"] = new System.Web.UI.Triplet("Jphonesharp", string.Empty, 3); dictionary["JphoneSharpSh07"] = new System.Web.UI.Triplet("Jphonesharp", string.Empty, 3); dictionary["JphoneSharpSh08"] = new System.Web.UI.Triplet("Jphonesharp", string.Empty, 3); dictionary["JphoneSharpSh51"] = new System.Web.UI.Triplet("Jphonesharp", string.Empty, 3); dictionary["JphoneSharpSh52"] = new System.Web.UI.Triplet("Jphonesharp", string.Empty, 3); dictionary["JphoneToshiba"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphoneToshibaT06a"] = new System.Web.UI.Triplet("Jphonetoshiba", string.Empty, 3); dictionary["JphoneToshibaT08"] = new System.Web.UI.Triplet("Jphonetoshiba", string.Empty, 3); dictionary["JphoneToshibaT51"] = new System.Web.UI.Triplet("Jphonetoshiba", string.Empty, 3); dictionary["Legend"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["LGG5200"] = new System.Web.UI.Triplet("Legend", string.Empty, 2); dictionary["MME"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["MMEF20"] = new System.Web.UI.Triplet("Mme", string.Empty, 2); dictionary["MMECellphone"] = new System.Web.UI.Triplet("Mmef20", string.Empty, 3); dictionary["MMEBenefonQ"] = new System.Web.UI.Triplet("Mmecellphone", string.Empty, 4); dictionary["MMESonyCMDZ5"] = new System.Web.UI.Triplet("Mmecellphone", string.Empty, 4); dictionary["MMESonyCMDZ5Pj020e"] = new System.Web.UI.Triplet("Mmesonycmdz5", string.Empty, 5); dictionary["MMESonyCMDJ5"] = new System.Web.UI.Triplet("Mmecellphone", string.Empty, 4); dictionary["MMESonyCMDJ7"] = new System.Web.UI.Triplet("Mmecellphone", string.Empty, 4); dictionary["MMEGenericSmall"] = new System.Web.UI.Triplet("Mmecellphone", string.Empty, 4); dictionary["MMEGenericLarge"] = new System.Web.UI.Triplet("Mmecellphone", string.Empty, 4); dictionary["MMEGenericFlip"] = new System.Web.UI.Triplet("Mmecellphone", string.Empty, 4); dictionary["MMEGeneric3D"] = new System.Web.UI.Triplet("Mmecellphone", string.Empty, 4); dictionary["MMEMobileExplorer"] = new System.Web.UI.Triplet("Mme", string.Empty, 2); dictionary["Nokia"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["NokiaBlueprint"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["NokiaWapSimulator"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["NokiaMobileBrowser"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia7110"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia6220"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia6250"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia6310"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia6510"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia8310"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia9110i"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia9110"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia3330"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia9210"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia9210HTML"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia3590"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia3590V1"] = new System.Web.UI.Triplet("Nokia3590", string.Empty, 3); dictionary["Nokia3595"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia3560"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia3650"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia3650P12Plus"] = new System.Web.UI.Triplet("Nokia3650", string.Empty, 3); dictionary["Nokia5100"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia6200"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia6590"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia6800"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia7650"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["NokiaMobileBrowserRainbow"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["NokiaEpoc32wtl"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["NokiaEpoc32wtl20"] = new System.Web.UI.Triplet("Nokiaepoc32wtl", string.Empty, 2); dictionary["Up"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["AuMic"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["AuMicV2"] = new System.Web.UI.Triplet("Aumic", string.Empty, 3); dictionary["a500"] = new System.Web.UI.Triplet("Aumic", string.Empty, 3); dictionary["n400"] = new System.Web.UI.Triplet("Aumic", string.Empty, 3); dictionary["AlcatelBe4"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["AlcatelBe5"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["AlcatelBe5v2"] = new System.Web.UI.Triplet("Alcatelbe5", string.Empty, 3); dictionary["AlcatelBe3"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["AlcatelBf3"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["AlcatelBf4"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotCb"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotF5"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotD8"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotCf"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotF6"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotBc"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotDc"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotPanC"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotC4"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mcca"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mot2000"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotP2kC"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotAf"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotAf418"] = new System.Web.UI.Triplet("Motaf", string.Empty, 3); dictionary["MotC2"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Xenium"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sagem959"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SghA300"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SghN100"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C304sa"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sy11"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["St12"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sy14"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SieS40"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SieSl45"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SieS35"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SieMe45"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SieS45"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Gm832"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Gm910i"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mot32"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mot28"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["D2"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["PPat"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Alaz"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Cdm9100"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Cdm135"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Cdm9000"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C303ca"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C311ca"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C202de"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C409ca"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C402de"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ds15"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tp2200"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tp120"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ds10"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["R280"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C201h"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["S71"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C302h"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C309h"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C407h"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C451h"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["R201"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["P21"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Kyocera702g"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Kyocera703g"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["KyoceraC307k"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tk01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tk02"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tk03"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tk04"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tk05"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["D303k"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["D304k"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Qcp2035"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Qcp3035"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["D512"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Dm110"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tm510"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Lg13"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["P100"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Lgc875f"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Lgp680f"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Lgp7800f"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Lgc840f"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Lgi2100"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Lgp7300f"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sd500"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tp1100"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tp3000"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["T250"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mo01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mo02"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mc01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mccc"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mcc9"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Nk00"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mai12"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ma112"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ma13"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mac1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mat1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sc01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sc03"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sc02"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sc04"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sg08"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sc13"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sc11"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sec01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sc10"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sy12"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["St11"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sy13"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Syc1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sy01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Syt1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sty2"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sy02"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sy03"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Si01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sni1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sn11"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sn12"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sn134"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sn156"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Snc1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tsc1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tsi1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ts11"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ts12"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ts13"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tst1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tst2"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tst3"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ig01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ig02"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ig03"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Qc31"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Qc12"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Qc32"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sp01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sh"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Upg1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Opwv1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Alav"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Im1k"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Nt95"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mot2001"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Motv200"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mot72"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mot76"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Scp6000"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotD5"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotF0"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SghA400"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sec03"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SieC3i"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sn17"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Scp4700"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sec02"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sy15"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Db520"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["L430V03J02"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["OPWVSDK"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["OPWVSDK6"] = new System.Web.UI.Triplet("Opwvsdk", string.Empty, 3); dictionary["OPWVSDK6Plus"] = new System.Web.UI.Triplet("Opwvsdk", string.Empty, 3); dictionary["KDDICA21"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["KDDITS21"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["KDDISA21"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["KM100"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["LGELX5350"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["HitachiP300"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SIES46"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotorolaV60G"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotorolaV708"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotorolaV708A"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotorolaE360"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SonyericssonA1101S"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["PhilipsFisio820"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["CasioA5302"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["TCLL668"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["KDDITS24"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SIES55"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SHARPGx10"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["BenQAthena"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Opera"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["Opera1to3beta"] = new System.Web.UI.Triplet("Opera", string.Empty, 2); dictionary["Opera4"] = new System.Web.UI.Triplet("Opera", string.Empty, 2); dictionary["Opera4beta"] = new System.Web.UI.Triplet("Opera4", string.Empty, 3); dictionary["Opera5to9"] = new System.Web.UI.Triplet("Opera", string.Empty, 2); dictionary["Opera6to9"] = new System.Web.UI.Triplet("Opera5to9", string.Empty, 3); dictionary["Opera7to9"] = new System.Web.UI.Triplet("Opera6to9", string.Empty, 4); dictionary["Opera8to9"] = new System.Web.UI.Triplet("Opera7to9", string.Empty, 5); dictionary["OperaPsion"] = new System.Web.UI.Triplet("Opera", string.Empty, 2); dictionary["Palmscape"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["PalmscapeVersion"] = new System.Web.UI.Triplet("Palmscape", string.Empty, 2); dictionary["AusPalm"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["SharpPda"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["ZaurusMiE1"] = new System.Web.UI.Triplet("Sharppda", string.Empty, 2); dictionary["ZaurusMiE21"] = new System.Web.UI.Triplet("Sharppda", string.Empty, 2); dictionary["ZaurusMiE25"] = new System.Web.UI.Triplet("Sharppda", string.Empty, 2); dictionary["Panasonic"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["PanasonicGAD95"] = new System.Web.UI.Triplet("Panasonic", string.Empty, 2); dictionary["PanasonicGAD87"] = new System.Web.UI.Triplet("Panasonic", string.Empty, 2); dictionary["PanasonicGAD87A39"] = new System.Web.UI.Triplet("Panasonicgad87", string.Empty, 3); dictionary["PanasonicGAD87A38"] = new System.Web.UI.Triplet("Panasonicgad87", string.Empty, 3); dictionary["MSPIE06"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["SKTDevices"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["SKTDevicesHyundai"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["PSE200"] = new System.Web.UI.Triplet("Sktdeviceshyundai", string.Empty, 3); dictionary["SKTDevicesHanhwa"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["SKTDevicesJTEL"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["JTEL01"] = new System.Web.UI.Triplet("Sktdevicesjtel", string.Empty, 3); dictionary["JTELNate"] = new System.Web.UI.Triplet("Jtel01", string.Empty, 4); dictionary["SKTDevicesLG"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["SKTDevicesMotorola"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["SKTDevicesV730"] = new System.Web.UI.Triplet("Sktdevicesmotorola", string.Empty, 3); dictionary["SKTDevicesNokia"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["SKTDevicesSKTT"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["SKTDevicesSamSung"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["SCHE150"] = new System.Web.UI.Triplet("Sktdevicessamsung", string.Empty, 3); dictionary["SKTDevicesEricsson"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["WinWap"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["Xiino"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["XiinoV2"] = new System.Web.UI.Triplet("Xiino", string.Empty, 2); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// This code was generated by a tool. // Runtime Version:2.0.50804.0 // // Changes to this file may cause incorrect behavior and will be lost if // the code is regenerated. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Web; using System.Web.Configuration; using System.Reflection; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal)] public class BrowserCapabilitiesFactory : System.Web.Configuration.BrowserCapabilitiesFactoryBase { public override void ConfigureBrowserCapabilities(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { this.DefaultProcess(headers, browserCaps); if ((this.IsBrowserUnknown(browserCaps) == false)) { return; } this.DefaultDefaultProcess(headers, browserCaps); } protected virtual void IeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void IeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool IeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Mozilla[^(]*\\([C|c]ompatible;\\s*MSIE (?\'version\'(?\'major\'\\d+)(?\'minor\'\\.\\d+)(?\'l" + "etters\'\\w*))(?\'extra\'[^)]*)"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "Opera|Go\\.Web|Windows CE|EudoraWeb"); if ((result == true)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "IE"; dictionary["extra"] = regexWorker["${extra}"]; dictionary["isColor"] = "true"; dictionary["letters"] = regexWorker["${letters}"]; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["screenBitDepth"] = "8"; dictionary["type"] = regexWorker["IE${major}"]; dictionary["version"] = regexWorker["${version}"]; browserCaps.AddBrowser("IE"); this.IeProcessGateways(headers, browserCaps); // gateway, parent=IE this.IeaolProcess(headers, browserCaps); // gateway, parent=IE this.IebetaProcess(headers, browserCaps); // gateway, parent=IE this.IeupdateProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=IE if (this.Ie5to9Process(headers, browserCaps)) { } else { if (this.Ie4Process(headers, browserCaps)) { } else { if (this.Ie3Process(headers, browserCaps)) { } else { if (this.Ie2Process(headers, browserCaps)) { } else { if (this.Ie1minor5Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } this.IeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie5to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie5to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie5to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^[5-9]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["activexcontrols"] = "true"; dictionary["backgroundsounds"] = "true"; dictionary["cookies"] = "true"; dictionary["css1"] = "true"; dictionary["css2"] = "true"; dictionary["ecmascriptversion"] = "1.2"; dictionary["frames"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["jscriptversion"] = "5.0"; dictionary["msdomversion"] = regexWorker["${majorversion}${minorversion}"]; dictionary["supportsCallback"] = "true"; dictionary["supportsFileUpload"] = "true"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["supportsMaintainScrollPositionOnPostback"] = "true"; dictionary["supportsVCard"] = "true"; dictionary["supportsXmlHttp"] = "true"; dictionary["tables"] = "true"; dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["vbscript"] = "true"; dictionary["w3cdomversion"] = "1.0"; dictionary["xml"] = "true"; browserCaps.AddBrowser("IE5to9"); this.Ie5to9ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=IE5to9 if (this.Ie6to9Process(headers, browserCaps)) { } else { if (this.Ie5Process(headers, browserCaps)) { } else { if (this.Ie5to9macProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.Ie5to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie6to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie6to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie6to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "[6-9]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["jscriptversion"] = "5.6"; dictionary["ExchangeOmaSupported"] = "true"; browserCaps.AddBrowser("IE6to9"); this.Ie6to9ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=IE6to9 if (this.Treo600Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Ie6to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Treo600ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Treo600ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Treo600Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "PalmSource; Blazer"); if ((result == false)) { return false; } // Capture: header values regexWorker.ProcessRegex(((string)(browserCaps[string.Empty])), "PalmSource; Blazer 3\\.0\\)\\s\\d+;(?\'screenPixelsHeight\'\\d+)x(?\'screenPixelsWidth\'\\d" + "+)$"); // Capabilities: set capabilities dictionary["browser"] = "Blazer 3.0"; dictionary["cachesAllResponsesWithExpires"] = "false"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canRenderEmptySelects"] = "true"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["ecmascriptversion"] = "1.1"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "false"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["javascript"] = "true"; dictionary["jscriptversion"] = "0.0"; dictionary["maximumHrefLength"] = "10000"; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["mobileDeviceManufacturer"] = ""; dictionary["mobileDeviceModel"] = ""; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "html32"; dictionary["preferredRequestEncoding"] = "utf-8"; dictionary["preferredResponseEncoding"] = "utf-8"; dictionary["rendersBreaksAfterHtmlLists"] = "true"; dictionary["requiredMetaTagNameValue"] = "PalmComputingPlatform"; dictionary["requiresAttributeColonSubstitution"] = "false"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["requiresControlStateInSession"] = "false"; dictionary["requiresDBCSCharacter"] = "false"; dictionary["requiresFullyQualifiedRedirectUrl"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["requiresLeadingPageBreak"] = "false"; dictionary["requiresNoBreakInFormatting"] = "false"; dictionary["requiresOutputOptimization"] = "false"; dictionary["requiresPostRedirectionHandling"] = "false"; dictionary["requiresPragmaNoCacheHeader"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["requiresUniqueHtmlCheckboxNames"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "13"; dictionary["screenCharactersWidth"] = "32"; dictionary["screenPixelsHeight"] = regexWorker["${screenPixelsHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenPixelsWidth}"]; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyColor"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCharacterEntityEncoding"] = "true"; dictionary["supportsCss"] = "false"; dictionary["supportsDivAlign"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsEmptyStringInCookieValue"] = "true"; dictionary["supportsFileUpload"] = "false"; dictionary["supportsFontColor"] = "true"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsIModeSymbols"] = "false"; dictionary["supportsInputIStyle"] = "false"; dictionary["supportsInputMode"] = "false"; dictionary["supportsItalic"] = "true"; dictionary["supportsJPhoneMultiMediaAttributes"] = "false"; dictionary["supportsJPhoneSymbols"] = "false"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["supportsQueryStringInFormAction"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectMultiple"] = "true"; dictionary["supportsUncheck"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "Handspring Treo 600"; browserCaps.AddBrowser("Treo600"); this.Treo600ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Treo600ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^5$"); if ((result == false)) { return false; } // Capabilities: set capabilities browserCaps.AddBrowser("IE5"); this.Ie5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=IE5 if (this.Ie50Process(headers, browserCaps)) { } else { if (this.Ie55Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.Ie5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie50ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie50ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie50Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["minorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^\\.0"); if ((result == false)) { return false; } // Capabilities: set capabilities browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("IE50"); this.Ie50ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie50ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie55ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie55ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie55Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["minorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^\\.5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["jscriptversion"] = "5.5"; dictionary["ExchangeOmaSupported"] = "true"; browserCaps.AddBrowser("IE55"); this.Ie55ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie55ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie5to9macProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie5to9macProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie5to9macProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["platform"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(MacPPC|Mac68K)"); if ((result == false)) { return false; } // Capabilities: set capabilities browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("IE5to9Mac"); this.Ie5to9macProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie5to9macProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MSIE 4"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["activexcontrols"] = "true"; dictionary["backgroundsounds"] = "true"; dictionary["cdf"] = "true"; dictionary["cookies"] = "true"; dictionary["css1"] = "true"; dictionary["ecmascriptversion"] = "1.2"; dictionary["frames"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["jscriptversion"] = "3.0"; dictionary["msdomversion"] = "4.0"; dictionary["supportsFileUpload"] = "true"; dictionary["supportsMultilineTextBoxDisplay"] = "false"; dictionary["supportsMaintainScrollPositionOnPostback"] = "true"; dictionary["tables"] = "true"; dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["vbscript"] = "true"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("IE4"); this.Ie4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie3ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie3ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie3Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^3"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["activexcontrols"] = "true"; dictionary["backgroundsounds"] = "true"; dictionary["cookies"] = "true"; dictionary["css1"] = "true"; dictionary["ecmascriptversion"] = "1.0"; dictionary["frames"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["jscriptversion"] = "1.0"; dictionary["supportsMultilineTextBoxDisplay"] = "false"; dictionary["tables"] = "true"; dictionary["vbscript"] = "true"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("IE3"); this.Ie3ProcessGateways(headers, browserCaps); // gateway, parent=IE3 this.Ie3akProcess(headers, browserCaps); // gateway, parent=IE3 this.Ie3skProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=IE3 if (this.Ie3win16Process(headers, browserCaps)) { } else { if (this.Ie3macProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.Ie3ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie3win16ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie3win16ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie3win16Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "16bit|Win(dows 3\\.1|16)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["activexcontrols"] = "false"; dictionary["javaapplets"] = "false"; browserCaps.AddBrowser("IE3win16"); this.Ie3win16ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=IE3win16 if (this.Ie3win16aProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Ie3win16ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie3win16aProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie3win16aProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie3win16aProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["extra"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^a"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; dictionary["javascript"] = "false"; dictionary["vbscript"] = "false"; browserCaps.AddBrowser("IE3win16a"); this.Ie3win16aProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie3win16aProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie3macProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie3macProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie3macProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "PPC Mac|Macintosh.*(68K|PPC)|Mac_(PowerPC|PPC|68(K|000))"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["activexcontrols"] = "false"; dictionary["vbscript"] = "false"; browserCaps.AddBrowser("IE3Mac"); this.Ie3macProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie3macProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie3akProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie3akProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie3akProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["extra"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "; AK;"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ak"] = "true"; this.Ie3akProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie3akProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie3skProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie3skProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie3skProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["extra"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "; SK;"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["sk"] = "true"; this.Ie3skProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie3skProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["backgroundsounds"] = "true"; dictionary["cookies"] = "true"; dictionary["tables"] = "true"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("IE2"); this.Ie2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=IE2 if (this.WebtvProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Ie2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ie1minor5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ie1minor5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ie1minor5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^1\\.5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "true"; dictionary["tables"] = "true"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("IE1minor5"); this.Ie1minor5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ie1minor5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void IeaolProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void IeaolProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool IeaolProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["extra"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "; AOL"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["aol"] = "true"; dictionary["frames"] = "true"; this.IeaolProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.IeaolProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void IebetaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void IebetaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool IebetaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["letters"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^([bB]|ab)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; this.IebetaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.IebetaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void IeupdateProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void IeupdateProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool IeupdateProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["extra"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "; Update a;"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["authenticodeupdate"] = "true"; this.IeupdateProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.IeupdateProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MozillaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MozillaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MozillaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Mozilla"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "MME|Opera"); if ((result == true)) { return false; } // Capture: header values regexWorker.ProcessRegex(((string)(browserCaps[string.Empty])), "Mozilla/(?\'version\'(?\'major\'\\d+)(?\'minor\'\\.\\d+)\\w*)"); regexWorker.ProcessRegex(((string)(browserCaps[string.Empty])), " (?\'screenWidth\'\\d*)x(?\'screenHeight\'\\d*)"); // Capabilities: set capabilities dictionary["browser"] = "Mozilla"; dictionary["cookies"] = "false"; dictionary["defaultScreenCharactersHeight"] = "40"; dictionary["defaultScreenCharactersWidth"] = "80"; dictionary["defaultScreenPixelsHeight"] = "480"; dictionary["defaultScreenPixelsWidth"] = "640"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "false"; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["screenBitDepth"] = "8"; dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsDivNoWrap"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["type"] = "Mozilla"; dictionary["version"] = regexWorker["${version}"]; browserCaps.AddBrowser("Mozilla"); this.MozillaProcessGateways(headers, browserCaps); // gateway, parent=Mozilla this.MozillabetaProcess(headers, browserCaps); // gateway, parent=Mozilla this.MozillagoldProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Mozilla if (this.IeProcess(headers, browserCaps)) { } else { if (this.PowerbrowserProcess(headers, browserCaps)) { } else { if (this.GeckoProcess(headers, browserCaps)) { } else { if (this.AvantgoProcess(headers, browserCaps)) { } else { if (this.GoamericaProcess(headers, browserCaps)) { } else { if (this.Netscape3Process(headers, browserCaps)) { } else { if (this.Netscape4Process(headers, browserCaps)) { } else { if (this.MypalmProcess(headers, browserCaps)) { } else { if (this.EudorawebProcess(headers, browserCaps)) { } else { if (this.WinceProcess(headers, browserCaps)) { } else { if (this.MspieProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } } } this.MozillaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MozillabetaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MozillabetaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MozillabetaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Mozilla/\\d+\\.\\d+b"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; this.MozillabetaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MozillabetaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MozillagoldProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MozillagoldProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MozillagoldProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Mozilla/\\d+\\.\\d+\\w*Gold"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["gold"] = "true"; this.MozillagoldProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MozillagoldProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PowerbrowserProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PowerbrowserProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PowerbrowserProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Mozilla/2\\.01 \\(Compatible\\) Oracle\\(tm\\) PowerBrowser\\(tm\\)/1\\.0a"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "PowerBrowser"; dictionary["cookies"] = "true"; dictionary["ecmascriptversion"] = "1.0"; dictionary["frames"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["majorversion"] = "1"; dictionary["minorversion"] = ".5"; dictionary["platform"] = "Win95"; dictionary["tables"] = "true"; dictionary["vbscript"] = "true"; dictionary["version"] = "1.5"; dictionary["type"] = "PowerBrowser1"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("PowerBrowser"); this.PowerbrowserProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PowerbrowserProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GeckoProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GeckoProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GeckoProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Gecko"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Mozilla"; dictionary["css1"] = "true"; dictionary["css2"] = "true"; dictionary["cookies"] = "true"; dictionary["ecmascriptversion"] = "1.5"; dictionary["frames"] = "true"; dictionary["isColor"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["maximumRenderedPageSize"] = "20000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["screenBitDepth"] = "32"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFileUpload"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "desktop"; dictionary["version"] = regexWorker["${version}"]; dictionary["w3cdomversion"] = "1.0"; browserCaps.AddBrowser("Gecko"); this.GeckoProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Gecko if (this.MozillarvProcess(headers, browserCaps)) { } else { if (this.SafariProcess(headers, browserCaps)) { } else { if (this.Netscape5Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.GeckoProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MozillarvProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MozillarvProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MozillarvProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "rv\\:(?\'version\'(?\'major\'\\d+)(?\'minor\'\\.[.\\d]*))"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "Netscape"); if ((result == true)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.4"; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["preferredImageMime"] = "image/gif"; dictionary["supportsCallback"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsMaintainScrollPositionOnPostback"] = "true"; dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["type"] = regexWorker["Mozilla${major}"]; dictionary["version"] = regexWorker["${version}"]; dictionary["w3cdomversion"] = "1.0"; browserCaps.AddBrowser("MozillaRV"); this.MozillarvProcessGateways(headers, browserCaps); // gateway, parent=MozillaRV this.Mozillav14plusProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=MozillaRV if (this.MozillafirebirdProcess(headers, browserCaps)) { } else { if (this.MozillafirefoxProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.MozillarvProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mozillav14plusProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mozillav14plusProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mozillav14plusProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^1$"); if ((result == false)) { return false; } headerValue = ((string)(dictionary["minorversion"])); result = regexWorker.ProcessRegex(headerValue, "^\\.[4-9]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["backgroundsounds"] = "true"; dictionary["css1"] = "true"; dictionary["css2"] = "true"; dictionary["javaapplets"] = "true"; dictionary["maximumRenderedPageSize"] = "2000000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["screenBitDepth"] = "32"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["type"] = regexWorker["Mozilla${version}"]; dictionary["xml"] = "true"; this.Mozillav14plusProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mozillav14plusProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MozillafirebirdProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MozillafirebirdProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MozillafirebirdProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Gecko\\/\\d+ Firebird\\/(?\'version\'(?\'major\'\\d+)(?\'minor\'\\.[.\\d]*))"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Firebird"; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["version"] = regexWorker["${version}"]; dictionary["type"] = regexWorker["Firebird${version}"]; browserCaps.AddBrowser("MozillaFirebird"); this.MozillafirebirdProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MozillafirebirdProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MozillafirefoxProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MozillafirefoxProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MozillafirefoxProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Gecko\\/\\d+ Firefox\\/(?\'version\'(?\'major\'\\d+)(?\'minor\'\\.[.\\d]*))"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Firefox"; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["version"] = regexWorker["${version}"]; dictionary["type"] = regexWorker["Firefox${version}"]; browserCaps.AddBrowser("MozillaFirefox"); this.MozillafirefoxProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MozillafirefoxProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SafariProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SafariProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SafariProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "AppleWebKit/(?\'webversion\'\\d+)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["appleWebTechnologyVersion"] = regexWorker["${webversion}"]; dictionary["backgroundsounds"] = "true"; dictionary["browser"] = "AppleMAC-Safari"; dictionary["css1"] = "true"; dictionary["css2"] = "true"; dictionary["ecmascriptversion"] = "0.0"; dictionary["futureBrowser"] = "Apple Safari"; dictionary["screenBitDepth"] = "24"; dictionary["tables"] = "true"; dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["type"] = "Desktop"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("Safari"); this.SafariProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Safari if (this.Safari60Process(headers, browserCaps)) { } else { if (this.Safari85Process(headers, browserCaps)) { } else { if (this.Safari1plusProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.SafariProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Safari60ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Safari60ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Safari60Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["appleWebTechnologyVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "60"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.0"; browserCaps.AddBrowser("Safari60"); this.Safari60ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Safari60ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Safari85ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Safari85ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Safari85Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["appleWebTechnologyVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "85"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.4"; browserCaps.AddBrowser("Safari85"); this.Safari85ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Safari85ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Safari1plusProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Safari1plusProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Safari1plusProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["appleWebTechnologyVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "\\d\\d\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.4"; dictionary["w3cdomversion"] = "1.0"; dictionary["supportsCallback"] = "true"; browserCaps.AddBrowser("Safari1Plus"); this.Safari1plusProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Safari1plusProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void AvantgoProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void AvantgoProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool AvantgoProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "\\(compatible; AvantGo .*\\)"); if ((result == false)) { return false; } // Capture: header values regexWorker.ProcessRegex(((string)(headers["X-AVANTGO-VERSION"])), "(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*)"); // Capabilities: set capabilities dictionary["browser"] = "AvantGo"; dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "true"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "12"; dictionary["defaultScreenPixelsHeight"] = "72"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["javascript"] = "false"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "2560"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiredMetaTagNameValue"] = "HandheldFriendly"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["requiresUniqueHtmlCheckboxNames"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "13"; dictionary["screenCharactersWidth"] = "30"; dictionary["screenPixelsHeight"] = "150"; dictionary["screenPixelsWidth"] = "150"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "false"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "false"; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["AvantGo${browserMajorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("AvantGo"); this.AvantgoProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=AvantGo if (this.TmobilesidekickProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.AvantgoProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void TmobilesidekickProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void TmobilesidekickProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool TmobilesidekickProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Danger hiptop"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["css1"] = "true"; dictionary["ecmaScriptVersion"] = "1.3"; dictionary["frames"] = "true"; dictionary["inputType"] = "telephoneKeypad"; dictionary["javaapplets"] = "true"; dictionary["majorVersion"] = "5"; dictionary["maximumRenderedPageSize"] = "7000"; dictionary["minorVersion"] = ".0"; dictionary["mobileDeviceManufacturer"] = "T-Mobile"; dictionary["mobileDeviceModel"] = "SideKick"; dictionary["preferredRenderingType"] = "html32"; dictionary["requiresLeadingPageBreak"] = "false"; dictionary["requiresUniqueHtmlCheckboxNames"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "11"; dictionary["screenCharactersWidth"] = "57"; dictionary["screenPixelsHeight"] = "136"; dictionary["screenPixelsWidth"] = "236"; dictionary["supportsCss"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["type"] = "AvantGo 3"; dictionary["version"] = "5.0"; browserCaps.AddBrowser("TMobileSidekick"); this.TmobilesidekickProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.TmobilesidekickProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void CasiopeiaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void CasiopeiaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool CasiopeiaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "CASSIOPEIA BE"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "CASSIOPEIA"; dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["cookies"] = "true"; dictionary["css1"] = "false"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "12"; dictionary["defaultScreenPixelsHeight"] = "72"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["ecmascriptversion"] = "1.3"; dictionary["frames"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isMobileDevice"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["mobileDeviceManufacturer"] = "Casio"; dictionary["mobileDeviceModel"] = "Casio BE-500"; dictionary["preferredImageMime"] = "image/gif"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresContentTypeMetaTag"] = "true"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["requiresNoBreakInFormatting"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "50"; dictionary["screenCharactersWidth"] = "38"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "240"; dictionary["supportsBold"] = "true"; dictionary["supportsCharacterEntityEncoding"] = "false"; dictionary["supportsCss"] = "false"; dictionary["supportsDivNoWrap"] = "true"; dictionary["supportsFileUpload"] = "false"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "false"; dictionary["supportsItalic"] = "false"; dictionary["tables"] = "true"; dictionary["type"] = "CASSIOPEIA"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("Casiopeia"); this.CasiopeiaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.CasiopeiaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void DefaultProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void DefaultProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool DefaultProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Capabilities: set capabilities dictionary["activexcontrols"] = "false"; dictionary["ak"] = "false"; dictionary["aol"] = "false"; dictionary["authenticodeupdate"] = "false"; dictionary["backgroundsounds"] = "false"; dictionary["beta"] = "false"; dictionary["browser"] = "Unknown"; dictionary["cachesAllResponsesWithExpires"] = "false"; dictionary["canCombineFormsInDeck"] = "true"; dictionary["canInitiateVoiceCall"] = "false"; dictionary["canRenderAfterInputOrSelectElement"] = "true"; dictionary["canRenderEmptySelects"] = "true"; dictionary["canRenderInputAndSelectElementsTogether"] = "true"; dictionary["canRenderMixedSelects"] = "true"; dictionary["canRenderOneventAndPrevElementsTogether"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["canRenderSetvarZeroWithMultiSelectionList"] = "true"; dictionary["canSendMail"] = "true"; dictionary["cdf"] = "false"; dictionary["cookies"] = "true"; dictionary["crawler"] = "false"; dictionary["css1"] = "false"; dictionary["css2"] = "false"; dictionary["defaultCharacterHeight"] = "12"; dictionary["defaultCharacterWidth"] = "8"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "12"; dictionary["defaultScreenPixelsHeight"] = "72"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["defaultSubmitButtonLimit"] = "1"; dictionary["ecmascriptversion"] = "0.0"; dictionary["frames"] = "false"; dictionary["gatewayMajorVersion"] = "0"; dictionary["gatewayMinorVersion"] = "0"; dictionary["gatewayVersion"] = "None"; dictionary["gold"] = "false"; dictionary["hasBackButton"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "false"; dictionary["inputType"] = "telephoneKeypad"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "false"; dictionary["javaapplets"] = "false"; dictionary["javascript"] = "false"; dictionary["jscriptversion"] = "0.0"; dictionary["majorversion"] = "0"; dictionary["maximumHrefLength"] = "10000"; dictionary["maximumRenderedPageSize"] = "2000"; dictionary["maximumSoftkeyLabelLength"] = "5"; dictionary["minorversion"] = "0"; dictionary["mobileDeviceManufacturer"] = "Unknown"; dictionary["mobileDeviceModel"] = "Unknown"; dictionary["msdomversion"] = "0.0"; dictionary["numberOfSoftkeys"] = "0"; dictionary["platform"] = "Unknown"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "html32"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["rendersBreaksAfterHtmlLists"] = "true"; dictionary["rendersBreaksAfterWmlAnchor"] = "false"; dictionary["rendersBreaksAfterWmlInput"] = "false"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["rendersWmlSelectsAsMenuCards"] = "false"; dictionary["requiredMetaTagNameValue"] = ""; dictionary["requiresAdaptiveErrorReporting"] = "false"; dictionary["requiresAttributeColonSubstitution"] = "false"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["requiresDBCSCharacter"] = "false"; dictionary["requiresFullyQualifiedRedirectUrl"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["requiresLeadingPageBreak"] = "false"; dictionary["requiresNoBreakInFormatting"] = "false"; dictionary["requiresNoescapedPostUrl"] = "true"; dictionary["requiresNoSoftkeyLabels"] = "false"; dictionary["requiresOutputOptimization"] = "false"; dictionary["requiresPhoneNumbersAsPlainText"] = "false"; dictionary["requiresPostRedirectionHandling"] = "false"; dictionary["requiresSpecialViewStateEncoding"] = "false"; dictionary["requiresUniqueFilePathSuffix"] = "false"; dictionary["requiresUniqueHtmlCheckboxNames"] = "false"; dictionary["requiresUniqueHtmlInputNames"] = "false"; dictionary["requiresUrlEncodedPostfieldValues"] = "false"; dictionary["screenBitDepth"] = "1"; dictionary["sk"] = "false"; dictionary["supportsAccesskeyAttribute"] = "false"; dictionary["supportsBodyColor"] = "true"; dictionary["supportsBold"] = "false"; dictionary["supportsCacheControlMetaTag"] = "true"; dictionary["supportsCharacterEntityEncoding"] = "true"; dictionary["supportsCss"] = "false"; dictionary["supportsDivAlign"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsEmptyStringInCookieValue"] = "true"; dictionary["supportsFileUpload"] = "false"; dictionary["supportsFontColor"] = "true"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["supportsImageSubmit"] = "false"; dictionary["supportsIModeSymbols"] = "false"; dictionary["supportsInputIStyle"] = "false"; dictionary["supportsInputMode"] = "false"; dictionary["supportsItalic"] = "false"; dictionary["supportsJPhoneMultiMediaAttributes"] = "false"; dictionary["supportsJPhoneSymbols"] = "false"; dictionary["supportsMaintainScrollPositionOnPostback"] = "false"; dictionary["supportsMultilineTextBoxDisplay"] = "false"; dictionary["supportsQueryStringInFormAction"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectMultiple"] = "true"; dictionary["supportsUncheck"] = "true"; dictionary["supportsVCard"] = "false"; dictionary["tables"] = "false"; dictionary["tagwriter"] = "System.Web.UI.Html32TextWriter"; dictionary["type"] = "Unknown"; dictionary["vbscript"] = "false"; dictionary["version"] = "0.0"; dictionary["w3cdomversion"] = "0.0"; dictionary["win16"] = "false"; dictionary["win32"] = "false"; dictionary["xml"] = "false"; browserCaps.AddBrowser("Default"); this.DefaultProcessGateways(headers, browserCaps); // gateway, parent=Default this.NokiagatewayProcess(headers, browserCaps); // gateway, parent=Default this.UpgatewayProcess(headers, browserCaps); // gateway, parent=Default this.CrawlerProcess(headers, browserCaps); // gateway, parent=Default this.ColorProcess(headers, browserCaps); // gateway, parent=Default this.MonoProcess(headers, browserCaps); // gateway, parent=Default this.PixelsProcess(headers, browserCaps); // gateway, parent=Default this.VoiceProcess(headers, browserCaps); // gateway, parent=Default this.CharsetProcess(headers, browserCaps); // gateway, parent=Default this.PlatformProcess(headers, browserCaps); // gateway, parent=Default this.WinProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Default if (this.MozillaProcess(headers, browserCaps)) { } else { if (this.DocomoProcess(headers, browserCaps)) { } else { if (this.Ericssonr380Process(headers, browserCaps)) { } else { if (this.EricssonProcess(headers, browserCaps)) { } else { if (this.EzwapProcess(headers, browserCaps)) { } else { if (this.GenericdownlevelProcess(headers, browserCaps)) { } else { if (this.JataayuProcess(headers, browserCaps)) { } else { if (this.JphoneProcess(headers, browserCaps)) { } else { if (this.LegendProcess(headers, browserCaps)) { } else { if (this.MmeProcess(headers, browserCaps)) { } else { if (this.NokiaProcess(headers, browserCaps)) { } else { if (this.NokiamobilebrowserrainbowProcess(headers, browserCaps)) { } else { if (this.Nokiaepoc32wtlProcess(headers, browserCaps)) { } else { if (this.UpProcess(headers, browserCaps)) { } else { if (this.OperaProcess(headers, browserCaps)) { } else { if (this.PalmscapeProcess(headers, browserCaps)) { } else { if (this.AuspalmProcess(headers, browserCaps)) { } else { if (this.SharppdaProcess(headers, browserCaps)) { } else { if (this.PanasonicProcess(headers, browserCaps)) { } else { if (this.Mspie06Process(headers, browserCaps)) { } else { if (this.SktdevicesProcess(headers, browserCaps)) { } else { if (this.WinwapProcess(headers, browserCaps)) { } else { if (this.XiinoProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } } } } } } } } } } } } } } } this.DefaultProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void DocomoProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void DocomoProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool DocomoProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^DoCoMo/"); if ((result == false)) { return false; } // Capture: header values regexWorker.ProcessRegex(((string)(browserCaps[string.Empty])), "^DoCoMo/(?\'httpVersion\'[^/ ]*)[/ ](?\'deviceID\'[^/\\x28]*)"); // Capabilities: set capabilities dictionary["browser"] = "i-mode"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["cookies"] = "false"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "16"; dictionary["defaultScreenPixelsHeight"] = "70"; dictionary["defaultScreenPixelsWidth"] = "90"; dictionary["deviceID"] = regexWorker["${deviceID}"]; dictionary["inputType"] = "telephoneKeypad"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["javaapplets"] = "false"; dictionary["javascript"] = "false"; dictionary["maximumHrefLength"] = "524"; dictionary["maximumRenderedPageSize"] = "5120"; dictionary["mobileDeviceModel"] = regexWorker["${deviceID}"]; dictionary["optimumPageWeight"] = "700"; dictionary["preferredRenderingType"] = "chtml10"; dictionary["preferredRequestEncoding"] = "shift_jis"; dictionary["preferredResponseEncoding"] = "shift_jis"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresFullyQualifiedRedirectUrl"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOutputOptimization"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["supportsAccesskeyAttribute"] = "true"; dictionary["supportsCharacterEntityEncoding"] = "false"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsIModeSymbols"] = "true"; dictionary["supportsInputIStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["tables"] = "false"; dictionary["type"] = "i-mode"; dictionary["vbscript"] = "false"; browserCaps.HtmlTextWriter = "System.Web.UI.ChtmlTextWriter"; browserCaps.AddBrowser("Docomo"); this.DocomoProcessGateways(headers, browserCaps); // gateway, parent=Docomo this.DocomorenderingsizeProcess(headers, browserCaps); // gateway, parent=Docomo this.DocomodefaultrenderingsizeProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Docomo if (this.Docomosh251iProcess(headers, browserCaps)) { } else { if (this.Docomon251iProcess(headers, browserCaps)) { } else { if (this.Docomop211iProcess(headers, browserCaps)) { } else { if (this.Docomof212iProcess(headers, browserCaps)) { } else { if (this.Docomod501iProcess(headers, browserCaps)) { } else { if (this.Docomof501iProcess(headers, browserCaps)) { } else { if (this.Docomon501iProcess(headers, browserCaps)) { } else { if (this.Docomop501iProcess(headers, browserCaps)) { } else { if (this.Docomod502iProcess(headers, browserCaps)) { } else { if (this.Docomof502iProcess(headers, browserCaps)) { } else { if (this.Docomon502iProcess(headers, browserCaps)) { } else { if (this.Docomop502iProcess(headers, browserCaps)) { } else { if (this.Docomonm502iProcess(headers, browserCaps)) { } else { if (this.Docomoso502iProcess(headers, browserCaps)) { } else { if (this.Docomof502itProcess(headers, browserCaps)) { } else { if (this.Docomon502itProcess(headers, browserCaps)) { } else { if (this.Docomoso502iwmProcess(headers, browserCaps)) { } else { if (this.Docomof504iProcess(headers, browserCaps)) { } else { if (this.Docomon504iProcess(headers, browserCaps)) { } else { if (this.Docomop504iProcess(headers, browserCaps)) { } else { if (this.Docomon821iProcess(headers, browserCaps)) { } else { if (this.Docomop821iProcess(headers, browserCaps)) { } else { if (this.Docomod209iProcess(headers, browserCaps)) { } else { if (this.Docomoer209iProcess(headers, browserCaps)) { } else { if (this.Docomof209iProcess(headers, browserCaps)) { } else { if (this.Docomoko209iProcess(headers, browserCaps)) { } else { if (this.Docomon209iProcess(headers, browserCaps)) { } else { if (this.Docomop209iProcess(headers, browserCaps)) { } else { if (this.Docomop209isProcess(headers, browserCaps)) { } else { if (this.Docomor209iProcess(headers, browserCaps)) { } else { if (this.Docomor691iProcess(headers, browserCaps)) { } else { if (this.Docomof503iProcess(headers, browserCaps)) { } else { if (this.Docomof503isProcess(headers, browserCaps)) { } else { if (this.Docomod503iProcess(headers, browserCaps)) { } else { if (this.Docomod503isProcess(headers, browserCaps)) { } else { if (this.Docomod210iProcess(headers, browserCaps)) { } else { if (this.Docomof210iProcess(headers, browserCaps)) { } else { if (this.Docomon210iProcess(headers, browserCaps)) { } else { if (this.Docomon2001Process(headers, browserCaps)) { } else { if (this.Docomod211iProcess(headers, browserCaps)) { } else { if (this.Docomon211iProcess(headers, browserCaps)) { } else { if (this.Docomop210iProcess(headers, browserCaps)) { } else { if (this.Docomoko210iProcess(headers, browserCaps)) { } else { if (this.Docomop2101vProcess(headers, browserCaps)) { } else { if (this.Docomop2102vProcess(headers, browserCaps)) { } else { if (this.Docomof211iProcess(headers, browserCaps)) { } else { if (this.Docomof671iProcess(headers, browserCaps)) { } else { if (this.Docomon503isProcess(headers, browserCaps)) { } else { if (this.Docomon503iProcess(headers, browserCaps)) { } else { if (this.Docomoso503iProcess(headers, browserCaps)) { } else { if (this.Docomop503isProcess(headers, browserCaps)) { } else { if (this.Docomop503iProcess(headers, browserCaps)) { } else { if (this.Docomoso210iProcess(headers, browserCaps)) { } else { if (this.Docomoso503isProcess(headers, browserCaps)) { } else { if (this.Docomosh821iProcess(headers, browserCaps)) { } else { if (this.Docomon2002Process(headers, browserCaps)) { } else { if (this.Docomoso505iProcess(headers, browserCaps)) { } else { if (this.Docomop505iProcess(headers, browserCaps)) { } else { if (this.Docomon505iProcess(headers, browserCaps)) { } else { if (this.Docomod505iProcess(headers, browserCaps)) { } else { if (this.Docomoisim60Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } this.DocomoProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void DocomorenderingsizeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void DocomorenderingsizeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool DocomorenderingsizeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^DoCoMo/([^/ ]*)[/ ]([^/\\x28]*)([/\\x28]c(?\'cacheSize\'\\d+))"); if ((result == false)) { return false; } // Identification: check capability matches headerValue = ((string)(dictionary["maximumRenderedPageSize"])); result = regexWorker.ProcessRegex(headerValue, "^5120$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = regexWorker["${cacheSize}000"]; this.DocomorenderingsizeProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.DocomorenderingsizeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void DocomodefaultrenderingsizeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void DocomodefaultrenderingsizeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool DocomodefaultrenderingsizeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["maximumRenderedPageSize"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^(0|00|000)$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "800"; this.DocomodefaultrenderingsizeProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.DocomodefaultrenderingsizeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomosh251iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomosh251iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomosh251iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SH251i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Sharp"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoSH251i"); this.Docomosh251iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=DocomoSH251i if (this.Docomosh251isProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Docomosh251iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomosh251isProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomosh251isProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomosh251isProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SH251iS"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["requiresLeadingPageBreak"] = "false"; dictionary["screenCharactersHeight"] = "11"; dictionary["screenCharactersWidth"] = "22"; dictionary["screenPixelsHeight"] = "220"; dictionary["screenPixelsWidth"] = "176"; browserCaps.AddBrowser("DocomoSH251iS"); this.Docomosh251isProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomosh251isProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon251iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon251iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon251iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N251i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "22"; dictionary["screenPixelsHeight"] = "140"; dictionary["screenPixelsWidth"] = "132"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoN251i"); this.Docomon251iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=DocomoN251i if (this.Docomon251isProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Docomon251iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon251isProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon251isProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon251isProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N251iS"); if ((result == false)) { return false; } // Capabilities: set capabilities browserCaps.AddBrowser("DocomoN251iS"); this.Docomon251isProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon251isProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop211iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop211iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop211iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P211i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["MobileDeviceManufacturer"] = "Panasonic"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoP211i"); this.Docomop211iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop211iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof212iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof212iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof212iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F212i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenPixelsHeight"] = "136"; dictionary["screenPixelsWidth"] = "132"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoF212i"); this.Docomof212iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof212iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomod501iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomod501iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomod501iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D501i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoD501i"); this.Docomod501iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomod501iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof501iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof501iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof501iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F501i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "84"; dictionary["screenPixelsWidth"] = "112"; browserCaps.AddBrowser("DocomoF501i"); this.Docomof501iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof501iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon501iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon501iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon501iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N501i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; browserCaps.AddBrowser("DocomoN501i"); this.Docomon501iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon501iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop501iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop501iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop501iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P501i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "120"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoP501i"); this.Docomop501iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop501iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomod502iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomod502iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomod502iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D502i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "90"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoD502i"); this.Docomod502iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomod502iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof502iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof502iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof502iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F502i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "91"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoF502i"); this.Docomof502iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof502iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon502iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon502iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon502iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N502i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; browserCaps.AddBrowser("DocomoN502i"); this.Docomon502iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon502iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop502iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop502iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop502iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P502i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderEmptySelects"] = "false"; dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "117"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoP502i"); this.Docomop502iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop502iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomonm502iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomonm502iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomonm502iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "NM502i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "Nokia"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "106"; dictionary["screenPixelsWidth"] = "111"; browserCaps.AddBrowser("DocomoNm502i"); this.Docomonm502iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomonm502iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoso502iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoso502iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoso502iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SO502i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "120"; dictionary["screenPixelsWidth"] = "120"; browserCaps.AddBrowser("DocomoSo502i"); this.Docomoso502iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoso502iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof502itProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof502itProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof502itProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F502it"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "91"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoF502it"); this.Docomof502itProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof502itProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon502itProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon502itProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon502itProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N502it"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; browserCaps.AddBrowser("DocomoN502it"); this.Docomon502itProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon502itProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoso502iwmProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoso502iwmProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoso502iwmProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SO502iWM"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "113"; dictionary["screenPixelsWidth"] = "120"; browserCaps.AddBrowser("DocomoSo502iwm"); this.Docomoso502iwmProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoso502iwmProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof504iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof504iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof504iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F504i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "136"; dictionary["screenPixelsWidth"] = "132"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoF504i"); this.Docomof504iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof504iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon504iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon504iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon504iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N504i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "180"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoN504i"); this.Docomon504iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon504iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop504iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop504iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop504iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P504i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "9"; dictionary["screenCharactersWidth"] = "22"; dictionary["screenPixelsHeight"] = "144"; dictionary["screenPixelsWidth"] = "132"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoP504i"); this.Docomop504iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop504iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon821iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon821iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon821iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N821i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "119"; browserCaps.AddBrowser("DocomoN821i"); this.Docomon821iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon821iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop821iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop821iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop821iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P821i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["maximumRenderedPageSize"] = "5000"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoP821i"); this.Docomop821iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop821iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomod209iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomod209iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomod209iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D209i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "90"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoD209i"); this.Docomod209iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomod209iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoer209iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoer209iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoer209iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "ER209i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "Ericsson"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "120"; browserCaps.AddBrowser("DocomoEr209i"); this.Docomoer209iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoer209iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof209iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof209iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof209iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F209i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "91"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoF209i"); this.Docomof209iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof209iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoko209iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoko209iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoko209iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KO209i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Kokusai"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "96"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoKo209i"); this.Docomoko209iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoko209iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon209iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon209iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon209iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N209i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "82"; dictionary["screenPixelsWidth"] = "108"; browserCaps.AddBrowser("DocomoN209i"); this.Docomon209iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon209iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop209iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop209iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop209iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P209i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "87"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoP209i"); this.Docomop209iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop209iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop209isProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop209isProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop209isProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P209iS"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "87"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoP209is"); this.Docomop209isProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop209isProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomor209iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomor209iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomor209iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R209i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "JRC"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoR209i"); this.Docomor209iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomor209iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomor691iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomor691iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomor691iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R691i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "JRC"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoR691i"); this.Docomor691iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomor691iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof503iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof503iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof503iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F503i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "120"; browserCaps.AddBrowser("DocomoF503i"); this.Docomof503iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof503iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof503isProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof503isProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof503isProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F503iS"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "24"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "120"; browserCaps.AddBrowser("DocomoF503is"); this.Docomof503isProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof503isProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomod503iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomod503iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomod503iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D503i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "126"; dictionary["screenPixelsWidth"] = "132"; browserCaps.AddBrowser("DocomoD503i"); this.Docomod503iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomod503iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomod503isProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomod503isProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomod503isProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D503iS$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "126"; dictionary["screenPixelsWidth"] = "132"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoD503is"); this.Docomod503isProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomod503isProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomod210iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomod210iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomod210iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D210i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "91"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoD210i"); this.Docomod210iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomod210iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof210iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof210iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof210iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F210i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "113"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoF210i"); this.Docomof210iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof210iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon210iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon210iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon210iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N210i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "113"; dictionary["screenPixelsWidth"] = "118"; browserCaps.AddBrowser("DocomoN210i"); this.Docomon210iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon210iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon2001ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon2001ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon2001Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N2001"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoN2001"); this.Docomon2001ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon2001ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomod211iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomod211iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomod211iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D211i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "91"; dictionary["screenPixelsWidth"] = "100"; browserCaps.AddBrowser("DocomoD211i"); this.Docomod211iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomod211iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon211iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon211iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon211iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N211i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoN211i"); this.Docomon211iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon211iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop210iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop210iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop210iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P210i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "91"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoP210i"); this.Docomop210iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop210iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoko210iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoko210iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoko210iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KO210i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Kokusai"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "96"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("DocomoKo210i"); this.Docomoko210iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoko210iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop2101vProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop2101vProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop2101vProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P2101V"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["screenBitDepth"] = "18"; dictionary["screenCharactersHeight"] = "9"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "182"; dictionary["screenPixelsWidth"] = "163"; browserCaps.AddBrowser("DocomoP2101v"); this.Docomop2101vProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop2101vProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop2102vProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop2102vProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop2102vProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P2102V"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; browserCaps.AddBrowser("DocomoP2102v"); this.Docomop2102vProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop2102vProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof211iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof211iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof211iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F211i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "113"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoF211i"); this.Docomof211iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof211iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomof671iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomof671iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomof671iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "F671i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Fujitsu"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "9"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "126"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoF671i"); this.Docomof671iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomof671iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon503isProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon503isProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon503isProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N503iS"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoN503is"); this.Docomon503isProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon503isProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon503iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon503iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon503iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N503i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoN503i"); this.Docomon503iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon503iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoso503iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoso503iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoso503iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SO503i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "113"; dictionary["screenPixelsWidth"] = "120"; browserCaps.AddBrowser("DocomoSo503i"); this.Docomoso503iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoso503iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop503isProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop503isProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop503isProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P503iS"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderEmptySelects"] = "false"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoP503is"); this.Docomop503isProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop503isProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop503iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop503iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop503iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P503i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderEmptySelects"] = "false"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["rendersBreaksAfterHtmlLists"] = "false"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsFontSize"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoP503i"); this.Docomop503iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop503iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoso210iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoso210iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoso210iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SO210i$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "17"; dictionary["screenPixelsHeight"] = "113"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoSo210i"); this.Docomoso210iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoso210iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoso503isProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoso503isProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoso503isProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SO503iS"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "17"; dictionary["screenPixelsHeight"] = "113"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoSo503is"); this.Docomoso503isProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoso503isProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomosh821iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomosh821iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomosh821iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SH821i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderEmptySelects"] = "false"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Sharp"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "78"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoSh821i"); this.Docomosh821iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomosh821iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon2002ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon2002ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon2002Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N2002"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NEC"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "118"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("DocomoN2002"); this.Docomon2002ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon2002ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoso505iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoso505iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoso505iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SO505i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "SonyEricsson"; dictionary["screenBitDepth"] = "18"; dictionary["screenCharactersHeight"] = "9"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "240"; dictionary["screenPixelsWidth"] = "256"; browserCaps.AddBrowser("DocomoSo505i"); this.Docomoso505iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoso505iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomop505iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomop505iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomop505iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P505i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; browserCaps.AddBrowser("DocomoP505i"); this.Docomop505iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomop505iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomon505iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomon505iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomon505iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "N505i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "NEC"; browserCaps.AddBrowser("DocomoN505i"); this.Docomon505iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomon505iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomod505iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomod505iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomod505iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D505i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["screenBitDepth"] = "18"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "270"; dictionary["screenPixelsWidth"] = "240"; browserCaps.AddBrowser("DocomoD505i"); this.Docomod505iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomod505iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Docomoisim60ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Docomoisim60ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Docomoisim60Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "ISIM60"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "false"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "true"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "NTT DoCoMo"; dictionary["mobileDeviceModel"] = "i-mode HTML Simulator"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "180"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsEmptyStringInCookieValue"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectMultiple"] = "false"; browserCaps.AddBrowser("DocomoISIM60"); this.Docomoisim60ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Docomoisim60ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssonr380ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssonr380ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssonr380Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R380 (?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*) WAP1\\.1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Ericsson"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["cookies"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "3000"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceManufacturer"] = "Ericsson"; dictionary["mobileDeviceModel"] = "R380"; dictionary["requiresNoescapedPostUrl"] = "false"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml11"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenPixelsHeight"] = "100"; dictionary["screenPixelsWidth"] = "310"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["type"] = "Ericsson R380"; dictionary["version"] = regexWorker["${browserMajorVersion}.${browserMinorVersion}"]; browserCaps.AddBrowser("EricssonR380"); this.Ericssonr380ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssonr380ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void EricssonProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void EricssonProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool EricssonProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Ericsson(?\'deviceID\'[^/]+)/(?\'deviceVer\'.*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Ericsson"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["cookies"] = "false"; dictionary["defaultScreenCharactersHeight"] = "4"; dictionary["defaultScreenCharactersWidth"] = "20"; dictionary["defaultScreenPixelsHeight"] = "52"; dictionary["defaultScreenPixelsWidth"] = "101"; dictionary["inputType"] = "telephoneKeypad"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["maximumRenderedPageSize"] = "1600"; dictionary["mobileDeviceManufacturer"] = "Ericsson"; dictionary["mobileDeviceModel"] = regexWorker["${deviceID}"]; dictionary["mobileDeviceVersion"] = regexWorker["${deviceVer}"]; dictionary["numberOfSoftkeys"] = "2"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml11"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["type"] = regexWorker["Ericsson ${deviceID}"]; browserCaps.HtmlTextWriter = "System.Web.UI.XhtmlTextWriter"; browserCaps.AddBrowser("Ericsson"); this.EricssonProcessGateways(headers, browserCaps); // gateway, parent=Ericsson this.SonyericssonProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Ericsson if (this.Ericssonr320Process(headers, browserCaps)) { } else { if (this.Ericssont20Process(headers, browserCaps)) { } else { if (this.Ericssont65Process(headers, browserCaps)) { } else { if (this.Ericssont68Process(headers, browserCaps)) { } else { if (this.Ericssont300Process(headers, browserCaps)) { } else { if (this.Ericssonp800Process(headers, browserCaps)) { } else { if (this.Ericssont61Process(headers, browserCaps)) { } else { if (this.Ericssont31Process(headers, browserCaps)) { } else { if (this.Ericssonr520Process(headers, browserCaps)) { } else { if (this.Ericssona2628Process(headers, browserCaps)) { } else { if (this.Ericssont39Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } } } this.EricssonProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SonyericssonProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SonyericssonProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SonyericssonProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^SonyEricsson"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Sony Ericsson"; dictionary["mobileDeviceManufacturer"] = "Sony Ericsson"; dictionary["type"] = regexWorker["Sony Ericsson ${mobileDeviceModel}"]; this.SonyericssonProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SonyericssonProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssonr320ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssonr320ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssonr320Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R320"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "3000"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "52"; dictionary["screenPixelsWidth"] = "101"; browserCaps.AddBrowser("EricssonR320"); this.Ericssonr320ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssonr320ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont20ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont20ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont20Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "T20"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "false"; dictionary["maximumRenderedPageSize"] = "1400"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["mobileDeviceModel"] = "T20, T20e, T29s"; dictionary["numberOfSoftkeys"] = "1"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenCharactersHeight"] = "3"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "33"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("EricssonT20"); this.Ericssont20ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont20ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont65ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont65ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont65Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "T65"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "3000"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["mobileDeviceModel"] = "Ericsson T65"; dictionary["numberOfSoftkeys"] = "1"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingType"] = "wml12"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "67"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("EricssonT65"); this.Ericssont65ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont65ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont68ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont68ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont68Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "T68"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "false"; dictionary["isColor"] = "true"; dictionary["numberOfSoftkeys"] = "1"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "false"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "80"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; browserCaps.AddBrowser("EricssonT68"); this.Ericssont68ProcessGateways(headers, browserCaps); // gateway, parent=EricssonT68 this.Ericssont68upgatewayProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=EricssonT68 if (this.Ericsson301aProcess(headers, browserCaps)) { } else { if (this.Ericssont68r1aProcess(headers, browserCaps)) { } else { if (this.Ericssont68r101Process(headers, browserCaps)) { } else { if (this.Ericssont68r201aProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } this.Ericssont68ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont68upgatewayProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont68upgatewayProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont68upgatewayProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "UP\\.Link"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "true"; this.Ericssont68upgatewayProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont68upgatewayProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericsson301aProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericsson301aProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericsson301aProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R301A"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canSendMail"] = "true"; dictionary["displaysAccessKeysAutomatically"] = "true"; dictionary["maximumRenderedPageSize"] = "2800"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresNewLineSuppression"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsBold"] = "false"; dictionary["supportsDivAlign"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "false"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["usePOverDiv"] = "true"; browserCaps.AddBrowser("Ericsson301A"); this.Ericsson301aProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericsson301aProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont300ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont300ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont300Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "T300"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnBlockElements"] = "false"; dictionary["breaksOnInlineElements"] = "false"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["cookies"] = "true"; dictionary["displaysAccessKeysAutomatically"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "2800"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "15"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsDivAlign"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "false"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["supportsWtai"] = "true"; dictionary["tables"] = "false"; browserCaps.AddBrowser("EricssonT300"); this.Ericssont300ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont300ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssonp800ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssonp800ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssonp800Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P800"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Sony Ericsson"; dictionary["cookies"] = "true"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["mobileDeviceManufacturer"] = "Sony Ericsson"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "16"; dictionary["screenCharactersWidth"] = "28"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "208"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("EricssonP800"); this.Ericssonp800ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=EricssonP800 if (this.Ericssonp800r101Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Ericssonp800ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssonp800r101ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssonp800r101ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssonp800r101Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R101"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "10000"; browserCaps.AddBrowser("EricssonP800R101"); this.Ericssonp800r101ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssonp800r101ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont61ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont61ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont61Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "T610|T616|T618"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["cookies"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "9800"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "true"; dictionary["requiredOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "128"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsSelectFollowingTable"] = "false"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; browserCaps.AddBrowser("EricssonT61"); this.Ericssont61ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont61ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont31ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont31ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont31Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "T310|T312|T316"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnBlockElements"] = "false"; dictionary["breaksOnInlineElements"] = "false"; dictionary["canInitiateVoiceCall"] = "false"; dictionary["cookies"] = "true"; dictionary["displaysAccessKeysAutomatically"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "2800"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "15"; dictionary["screenPixelsHeight"] = "80"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsDivAlign"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "false"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; browserCaps.AddBrowser("EricssonT31"); this.Ericssont31ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont31ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont68r1aProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont68r1aProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont68r1aProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R1A"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "false"; dictionary["maximumRenderedPageSize"] = "5000"; dictionary["maximumSoftkeyLabelLength"] = "14"; dictionary["preferredImageMime"] = "image/gif"; dictionary["rendersWmlDoAcceptsInline"] = "true"; browserCaps.AddBrowser("EricssonT68R1A"); this.Ericssont68r1aProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont68r1aProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont68r101ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont68r101ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont68r101Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R101"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2900"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["preferredImageMime"] = "image/gif"; dictionary["requiresNoSoftkeyLabels"] = "true"; browserCaps.AddBrowser("EricssonT68R101"); this.Ericssont68r101ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont68r101ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont68r201aProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont68r201aProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont68r201aProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R201A"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["maximumRenderedPageSize"] = "2900"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingType"] = "wml12"; dictionary["screenBitDepth"] = "24"; browserCaps.AddBrowser("EricssonT68R201A"); this.Ericssont68r201aProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont68r201aProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssonr520ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssonr520ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssonr520Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "R520"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "1600"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "67"; dictionary["screenPixelsWidth"] = "101"; browserCaps.AddBrowser("EricssonR520"); this.Ericssonr520ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssonr520ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssona2628ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssona2628ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssona2628Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "A2628"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "1600"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "54"; dictionary["screenPixelsWidth"] = "101"; browserCaps.AddBrowser("EricssonA2628"); this.Ericssona2628ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssona2628ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ericssont39ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ericssont39ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ericssont39Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "T39"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "true"; dictionary["inputType"] = "telephoneKeypad"; dictionary["maximumRenderedPageSize"] = "3000"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["mobileDeviceManufacturer"] = "Ericsson"; dictionary["mobileDeviceModel"] = "Ericsson T39"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml12"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "3"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "54"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("EricssonT39"); this.Ericssont39ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ericssont39ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void EzwapProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void EzwapProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool EzwapProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "EzWAP (?\'browserMajorVersion\'\\d+)(?\'browserMinorVersion\'\\.\\d+)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "EzWAP"; dictionary["canSendMail"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresXhtmlCssSuppression"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "33"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "240"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsDivAlign"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsStyleElement"] = "false"; dictionary["supportsUrlAttributeEncoding"] = "false"; dictionary["tables"] = "true"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.AddBrowser("EzWAP"); this.EzwapProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.EzwapProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void NokiagatewayProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void NokiagatewayProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool NokiagatewayProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["VIA"])); if (string.IsNullOrEmpty(headerValue)) { return false; } headerValue = ((string)(headers["VIA"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'nokiaVersion\'Nokia\\D*(?\'gatewayMajorVersion\'\\d+)(?\'gatewayMinorVersion\'\\.\\d+)[" + "^,]*)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["gatewayMajorVersion"] = regexWorker["${gatewayMajorVersion}"]; dictionary["gatewayMinorVersion"] = regexWorker["${gatewayMinorVersion}"]; dictionary["gatewayVersion"] = regexWorker["${nokiaVersion}"]; this.NokiagatewayProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.NokiagatewayProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpgatewayProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpgatewayProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpgatewayProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "UP\\.Link/"); if ((result == false)) { return false; } // Capture: header values regexWorker.ProcessRegex(((string)(browserCaps[string.Empty])), "(?\'goWebUPGateway\'Go\\.Web)"); // Capabilities: set capabilities dictionary["isGoWebUpGateway"] = regexWorker["${goWebUPGateway}"]; this.UpgatewayProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=UPGateway if (this.UpnongogatewayProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.UpgatewayProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpnongogatewayProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpnongogatewayProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpnongogatewayProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "UP\\.Link/(?\'gatewayMajorVersion\'\\d*)(?\'gatewayMinorVersion\'\\.\\d*)(?\'other\'\\S*)"); if ((result == false)) { return false; } // Identification: check capability matches headerValue = ((string)(dictionary["isGoWebUpGateway"])); result = regexWorker.ProcessRegex(headerValue, "^$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["gatewayMajorVersion"] = regexWorker["${gatewayMajorVersion}"]; dictionary["gatewayMinorVersion"] = regexWorker["${gatewayMinorVersion}"]; dictionary["gatewayVersion"] = regexWorker["UP.Link/${gatewayMajorVersion}${gatewayMinorVersion}${other}"]; this.UpnongogatewayProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpnongogatewayProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void CrawlerProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void CrawlerProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool CrawlerProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "crawler|Crawler|Googlebot|msnbot"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["crawler"] = "true"; this.CrawlerProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.CrawlerProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void ColorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void ColorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool ColorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["UA-COLOR"])); if (string.IsNullOrEmpty(headerValue)) { return false; } headerValue = ((string)(headers["UA-COLOR"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "color(?\'colorDepth\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["screenBitDepth"] = regexWorker["${colorDepth}"]; this.ColorProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.ColorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MonoProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MonoProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MonoProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["UA-COLOR"])); if (string.IsNullOrEmpty(headerValue)) { return false; } headerValue = ((string)(headers["UA-COLOR"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "mono(?\'colorDepth\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["screenBitDepth"] = regexWorker["${colorDepth}"]; this.MonoProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MonoProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PixelsProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PixelsProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PixelsProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["UA-PIXELS"])); if (string.IsNullOrEmpty(headerValue)) { return false; } headerValue = ((string)(headers["UA-PIXELS"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'screenWidth\'\\d+)x(?\'screenHeight\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; this.PixelsProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PixelsProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void VoiceProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void VoiceProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool VoiceProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["UA-VOICE"])); if (string.IsNullOrEmpty(headerValue)) { return false; } headerValue = ((string)(headers["UA-VOICE"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?i:TRUE)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "true"; this.VoiceProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.VoiceProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void CharsetProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void CharsetProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool CharsetProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-CHARSET"])); if (string.IsNullOrEmpty(headerValue)) { return false; } headerValue = ((string)(headers["X-UP-DEVCAP-CHARSET"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?i)^Shift_JIS$"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "(UP/\\S* UP\\.Browser/3\\.\\[3-9]d*)|(UP\\.Browser/3\\.\\[3-9]d*)|(UP\\.Browser/3\\.\\[3-9]" + "d*)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["canSendMail"] = "true"; this.CharsetProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.CharsetProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PlatformProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PlatformProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PlatformProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); if (string.IsNullOrEmpty(headerValue)) { return false; } // Capabilities: set capabilities this.PlatformProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Platform if (this.PlatformwinntProcess(headers, browserCaps)) { } else { if (this.Platformwin2000bProcess(headers, browserCaps)) { } else { if (this.Platformwin95Process(headers, browserCaps)) { } else { if (this.Platformwin98Process(headers, browserCaps)) { } else { if (this.Platformwin16Process(headers, browserCaps)) { } else { if (this.PlatformwinceProcess(headers, browserCaps)) { } else { if (this.Platformmac68kProcess(headers, browserCaps)) { } else { if (this.PlatformmacppcProcess(headers, browserCaps)) { } else { if (this.PlatformunixProcess(headers, browserCaps)) { } else { if (this.PlatformwebtvProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } } this.PlatformProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PlatformwinntProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PlatformwinntProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PlatformwinntProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Windows NT|WinNT|Windows XP"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "WinCE|Windows CE"); if ((result == true)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "WinNT"; this.PlatformwinntProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=PlatformWinnt if (this.PlatformwinxpProcess(headers, browserCaps)) { } else { if (this.Platformwin2000aProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.PlatformwinntProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PlatformwinxpProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PlatformwinxpProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PlatformwinxpProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Windows (NT 5\\.1|XP)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "WinXP"; this.PlatformwinxpProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PlatformwinxpProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Platformwin2000aProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Platformwin2000aProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Platformwin2000aProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Windows NT 5\\.0"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "Win2000"; this.Platformwin2000aProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Platformwin2000aProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Platformwin2000bProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Platformwin2000bProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Platformwin2000bProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Windows 2000"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "Win2000"; this.Platformwin2000bProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Platformwin2000bProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Platformwin95ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Platformwin95ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Platformwin95Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Win(dows )?95"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "Win95"; this.Platformwin95ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Platformwin95ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Platformwin98ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Platformwin98ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Platformwin98Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Win(dows )?98"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "Win98"; this.Platformwin98ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Platformwin98ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Platformwin16ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Platformwin16ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Platformwin16Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Win(dows 3\\.1|16)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "Win16"; this.Platformwin16ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Platformwin16ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PlatformwinceProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PlatformwinceProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PlatformwinceProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Win(dows )?CE"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "WinCE"; this.PlatformwinceProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PlatformwinceProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Platformmac68kProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Platformmac68kProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Platformmac68kProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Mac(_68(000|K)|intosh.*68K)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "Mac68K"; this.Platformmac68kProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Platformmac68kProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PlatformmacppcProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PlatformmacppcProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PlatformmacppcProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Mac(_PowerPC|intosh.*PPC|_PPC)|PPC Mac"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "MacPPC"; this.PlatformmacppcProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PlatformmacppcProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PlatformunixProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PlatformunixProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PlatformunixProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "X11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "UNIX"; this.PlatformunixProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PlatformunixProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PlatformwebtvProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PlatformwebtvProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PlatformwebtvProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "WebTV"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["platform"] = "WebTV"; this.PlatformwebtvProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PlatformwebtvProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void WinProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void WinProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool WinProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); if (string.IsNullOrEmpty(headerValue)) { return false; } // Capabilities: set capabilities this.WinProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Win if (this.Win32Process(headers, browserCaps)) { } else { if (this.Win16Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.WinProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Win32ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Win32ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Win32Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Win(dows )?(9[58]|NT|32)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["win32"] = "true"; this.Win32ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Win32ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Win16ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Win16ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Win16Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "16bit|Win(dows 3\\.1|16)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["win16"] = "true"; this.Win16ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Win16ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GenericdownlevelProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GenericdownlevelProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GenericdownlevelProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Generic Downlevel$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "false"; dictionary["ecmascriptversion"] = "1.0"; dictionary["tables"] = "true"; dictionary["type"] = "Downlevel"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("GenericDownlevel"); this.GenericdownlevelProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.GenericdownlevelProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GoamericaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GoamericaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GoamericaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Go\\.Web/(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["BackgroundSounds"] = "true"; dictionary["browser"] = "Go.Web"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "true"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "12"; dictionary["defaultScreenPixelsHeight"] = "72"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["isMobileDevice"] = "true"; dictionary["javaapplets"] = "false"; dictionary["javascript"] = "false"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "6000"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["rendersBreaksAfterHtmlLists"] = "false"; dictionary["requiredMetaTagNameValue"] = "HandheldFriendly"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresNoBreakInFormatting"] = "true"; dictionary["requiresUniqueHtmlCheckboxNames"] = "true"; dictionary["screenBitDepth"] = "2"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "false"; dictionary["supportsDivAlign"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["SupportsDivNoWrap"] = "false"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "false"; dictionary["supportsSelectMultiple"] = "false"; dictionary["type"] = "Go.Web"; dictionary["vbscript"] = "false"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("GoAmerica"); this.GoamericaProcessGateways(headers, browserCaps); // gateway, parent=GoAmerica this.GoamericaupProcess(headers, browserCaps); // gateway, parent=GoAmerica this.GatableProcess(headers, browserCaps); // gateway, parent=GoAmerica this.MaxpagesizeProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=GoAmerica if (this.GoamericawinceProcess(headers, browserCaps)) { } else { if (this.GoamericapalmProcess(headers, browserCaps)) { } else { if (this.GoamericarimProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.GoamericaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GoamericaupProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GoamericaupProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GoamericaupProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "UP\\.Browser"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "0.0"; dictionary["frames"] = "false"; this.GoamericaupProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.GoamericaupProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GoamericawinceProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GoamericawinceProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GoamericawinceProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "WinCE"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["defaultScreenCharactersHeight"] = "14"; dictionary["defaultScreenCharactersWidth"] = "30"; dictionary["defaultScreenPixelsHeight"] = "320"; dictionary["defaultScreenPixelsWidth"] = "240"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["mobileDeviceModel"] = "Pocket PC"; dictionary["platform"] = "WinCE"; dictionary["screenBitDepth"] = "16"; dictionary["supportsDivAlign"] = "true"; dictionary["supportsFontColor"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsSelectMultiple"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("GoAmericaWinCE"); this.GoamericawinceProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.GoamericawinceProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GoamericapalmProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GoamericapalmProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GoamericapalmProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Palm"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "PalmOS-licensee"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "36"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsUncheck"] = "false"; browserCaps.AddBrowser("GoAmericaPalm"); this.GoamericapalmProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.GoamericapalmProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GoamericarimProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GoamericarimProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GoamericarimProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "RIM"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "false"; dictionary["mobileDeviceManufacturer"] = "RIM"; dictionary["screenBitDepth"] = "1"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsCss"] = "false"; dictionary["supportsDivAlign"] = "false"; dictionary["supportsDivWrap"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["supportsFontItalic"] = "false"; browserCaps.AddBrowser("GoAmericaRIM"); this.GoamericarimProcessGateways(headers, browserCaps); // gateway, parent=GoAmericaRIM this.GoamericanonuprimProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=GoAmericaRIM if (this.Goamericarim950Process(headers, browserCaps)) { } else { if (this.Goamericarim850Process(headers, browserCaps)) { } else { if (this.Goamericarim957Process(headers, browserCaps)) { } else { if (this.Goamericarim857Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } this.GoamericarimProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GoamericanonuprimProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GoamericanonuprimProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GoamericanonuprimProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "UP\\.Browser"); if ((result == true)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.1"; dictionary["frames"] = "true"; this.GoamericanonuprimProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.GoamericanonuprimProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Goamericarim950ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Goamericarim950ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Goamericarim950Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "RIM950"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["BackgroundSounds"] = "false"; dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["mobileDeviceModel"] = "950"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "25"; dictionary["screenPixelsHeight"] = "64"; dictionary["screenPixelsWidth"] = "132"; browserCaps.AddBrowser("GoAmericaRIM950"); this.Goamericarim950ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Goamericarim950ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Goamericarim850ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Goamericarim850ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Goamericarim850Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "RIM850"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceModel"] = "850"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "25"; dictionary["screenPixelsHeight"] = "64"; dictionary["screenPixelsWidth"] = "132"; browserCaps.AddBrowser("GoAmericaRIM850"); this.Goamericarim850ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Goamericarim850ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Goamericarim957ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Goamericarim957ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Goamericarim957Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "RIM957"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["BackgroundSounds"] = "false"; dictionary["mobileDeviceModel"] = "957"; dictionary["screenCharactersHeight"] = "15"; dictionary["screenCharactersWidth"] = "32"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "160"; browserCaps.AddBrowser("GoAmericaRIM957"); this.Goamericarim957ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=GoAmericaRIM957 if (this.Goamericarim957major6minor2Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Goamericarim957ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Goamericarim957major6minor2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Goamericarim957major6minor2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Goamericarim957major6minor2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "6\\.2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["maximumRenderedPageSize"] = "7168"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["requiresUniqueHtmlCheckboxNames"] = "true"; dictionary["screenCharactersHeight"] = "13"; dictionary["screenCharactersWidth"] = "30"; dictionary["supportsSelectMultiple"] = "true"; dictionary["supportsUncheck"] = "true"; browserCaps.AddBrowser("GoAmericaRIM957major6minor2"); this.Goamericarim957major6minor2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Goamericarim957major6minor2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Goamericarim857ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Goamericarim857ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Goamericarim857Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "RIM857"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["BackgroundSounds"] = "false"; dictionary["ecmascriptversion"] = "0.0"; dictionary["frames"] = "false"; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["mobileDeviceModel"] = "857"; dictionary["screenCharactersHeight"] = "15"; dictionary["screenCharactersWidth"] = "32"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "160"; browserCaps.AddBrowser("GoAmericaRIM857"); this.Goamericarim857ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=GoAmericaRIM857 if (this.Goamericarim857major6Process(headers, browserCaps)) { } else { if (this.Goamerica7to9Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.Goamericarim857ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Goamericarim857major6ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Goamericarim857major6ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Goamericarim857major6Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "6\\."); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.1"; dictionary["frames"] = "true"; browserCaps.AddBrowser("GoAmericaRIM857major6"); this.Goamericarim857major6ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=GoAmericaRIM857major6 if (this.Goamericarim857major6minor2to9Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Goamericarim857major6ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Goamericarim857major6minor2to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Goamericarim857major6minor2to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Goamericarim857major6minor2to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "6\\.[2-9]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["requiresAttributeColonSubstitution"] = "false"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenCharactersHeight"] = "16"; dictionary["screenCharactersWidth"] = "31"; dictionary["supportsUncheck"] = "false"; browserCaps.AddBrowser("GoAmericaRIM857major6minor2to9"); this.Goamericarim857major6minor2to9ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Goamericarim857major6minor2to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Goamerica7to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Goamerica7to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Goamerica7to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^[7-9]$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["requiresAttributeColonSubstitution"] = "false"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenCharactersHeight"] = "16"; dictionary["screenCharactersWidth"] = "31"; dictionary["supportsUncheck"] = "false"; browserCaps.AddBrowser("GoAmerica7to9"); this.Goamerica7to9ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Goamerica7to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GatableProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GatableProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GatableProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-GA-TABLES"])); if (string.IsNullOrEmpty(headerValue)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities this.GatableProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=GaTable if (this.GatablefalseProcess(headers, browserCaps)) { } else { if (this.GatabletrueProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.GatableProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GatablefalseProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GatablefalseProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GatablefalseProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-GA-TABLES"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?i:FALSE)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["tables"] = "false"; this.GatablefalseProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.GatablefalseProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void GatabletrueProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void GatabletrueProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool GatabletrueProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-GA-TABLES"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?i:TRUE)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["tables"] = "true"; this.GatabletrueProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.GatabletrueProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MaxpagesizeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MaxpagesizeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MaxpagesizeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-GA-MAX-TRANSFER"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'maxPageSize\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = regexWorker["${maxPageSize}"]; this.MaxpagesizeProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MaxpagesizeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JataayuProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JataayuProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JataayuProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^jBrowser"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Jataayu jBrowser"; dictionary["canSendMail"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceManufacturer"] = "Jataayu"; dictionary["mobileDeviceModel"] = "jBrowser"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "wml20"; dictionary["requiresCommentInStyleElement"] = "true"; dictionary["requiresHiddenFieldValues"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "true"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "17"; dictionary["screenCharactersWidth"] = "42"; dictionary["screenPixelsHeight"] = "265"; dictionary["screenPixelsWidth"] = "248"; dictionary["supportsBodyClassAttribute"] = "false"; dictionary["supportsBold"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["tables"] = "true"; dictionary["type"] = "Jataayu jBrowser"; browserCaps.AddBrowser("Jataayu"); this.JataayuProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Jataayu if (this.JataayuppcProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.JataayuProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JataayuppcProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JataayuppcProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JataayuppcProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(PPC)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "true"; dictionary["screenCharactersHeight"] = "14"; dictionary["screenCharactersWidth"] = "31"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "240"; dictionary["supportsStyleElement"] = "true"; browserCaps.AddBrowser("JataayuPPC"); this.JataayuppcProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JataayuppcProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphoneProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphoneProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphoneProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-PHONE/"); if ((result == false)) { return false; } // Capture: header values regexWorker.ProcessRegex(((string)(browserCaps[string.Empty])), "J-PHONE/(?\'majorVersion\'\\d+)(?\'minorVersion\'\\.\\d+)/(?\'deviceModel\'.*)"); // Capabilities: set capabilities dictionary["browser"] = "J-Phone"; dictionary["cookies"] = "false"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["defaultCharacterHeight"] = "12"; dictionary["defaultCharacterWidth"] = "12"; dictionary["defaultScreenCharactersHeight"] = "7"; dictionary["defaultScreenCharactersWidth"] = "16"; dictionary["defaultScreenPixelsHeight"] = "84"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["javaapplets"] = "false"; dictionary["javascript"] = "false"; dictionary["majorVersion"] = regexWorker["${majorVersion}"]; dictionary["maximumRenderedPageSize"] = "6000"; dictionary["minorVersion"] = regexWorker["${minorVersion}"]; dictionary["mobileDeviceModel"] = regexWorker["${deviceModel}"]; dictionary["optimumPageWeight"] = "700"; dictionary["preferredImageMime"] = "image/png"; dictionary["preferredRenderingType"] = "html32"; dictionary["preferredRequestEncoding"] = "shift_jis"; dictionary["preferredResponseEncoding"] = "shift_jis"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresContentTypeMetaTag"] = "true"; dictionary["requiresFullyQualifiedRedirectUrl"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOutputOptimization"] = "true"; dictionary["screenBitDepth"] = "2"; dictionary["supportsAccesskeyAttribute"] = "true"; dictionary["supportsBodyColor"] = "true"; dictionary["supportsBold"] = "false"; dictionary["supportsCharacterEntityEncoding"] = "false"; dictionary["supportsDivAlign"] = "true"; dictionary["supportsDivNoWrap"] = "true"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsFontColor"] = "true"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["supportsInputMode"] = "true"; dictionary["supportsItalic"] = "false"; dictionary["supportsJPhoneMultiMediaAttributes"] = "true"; dictionary["supportsJPhoneSymbols"] = "true"; dictionary["supportsQueryStringInFormAction"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["tables"] = "true"; dictionary["type"] = "J-Phone"; dictionary["vbscript"] = "false"; dictionary["version"] = regexWorker["${majorVersion}${minorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.ChtmlTextWriter"; browserCaps.AddBrowser("Jphone"); this.JphoneProcessGateways(headers, browserCaps); // gateway, parent=Jphone this.Jphone4Process(headers, browserCaps); // gateway, parent=Jphone this.JphonecolorProcess(headers, browserCaps); // gateway, parent=Jphone this.JphonedisplayProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Jphone if (this.JphonemitsubishiProcess(headers, browserCaps)) { } else { if (this.JphonedensoProcess(headers, browserCaps)) { } else { if (this.JphonekenwoodProcess(headers, browserCaps)) { } else { if (this.JphonenecProcess(headers, browserCaps)) { } else { if (this.JphonepanasonicProcess(headers, browserCaps)) { } else { if (this.JphonepioneerProcess(headers, browserCaps)) { } else { if (this.JphonesanyoProcess(headers, browserCaps)) { } else { if (this.JphonesharpProcess(headers, browserCaps)) { } else { if (this.JphonetoshibaProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } this.JphoneProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonemitsubishiProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonemitsubishiProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonemitsubishiProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-D\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; browserCaps.AddBrowser("JphoneMitsubishi"); this.JphonemitsubishiProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JphonemitsubishiProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonedensoProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonedensoProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonedensoProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-DN\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Denso"; browserCaps.AddBrowser("JphoneDenso"); this.JphonedensoProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JphonedensoProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonekenwoodProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonekenwoodProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonekenwoodProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-K\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kenwood"; browserCaps.AddBrowser("JphoneKenwood"); this.JphonekenwoodProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JphonekenwoodProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonenecProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonenecProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonenecProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-N\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "NEC"; browserCaps.AddBrowser("JphoneNec"); this.JphonenecProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=JphoneNec if (this.Jphonenecn51Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.JphonenecProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonenecn51ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonenecn51ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonenecn51Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-N51"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "12000"; dictionary["mobileDeviceModel"] = "J-N51"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["supportsCharacterEntityEncoding"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsInputIStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneNecN51"); this.Jphonenecn51ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonenecn51ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonepanasonicProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonepanasonicProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonepanasonicProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-P\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; browserCaps.AddBrowser("JphonePanasonic"); this.JphonepanasonicProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JphonepanasonicProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonepioneerProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonepioneerProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonepioneerProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-PE\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Pioneer"; browserCaps.AddBrowser("JphonePioneer"); this.JphonepioneerProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JphonepioneerProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonesanyoProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonesanyoProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonesanyoProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-SA\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; browserCaps.AddBrowser("JphoneSanyo"); this.JphonesanyoProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=JphoneSanyo if (this.Jphonesa51Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.JphonesanyoProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonesa51ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonesa51ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonesa51Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-SA51\\D*"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "12000"; dictionary["mobileDeviceModel"] = "J-SA51"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenCharactersWidth"] = "22"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsInputIStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneSA51"); this.Jphonesa51ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonesa51ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonesharpProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonesharpProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonesharpProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-SH\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sharp"; browserCaps.AddBrowser("JphoneSharp"); this.JphonesharpProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=JphoneSharp if (this.Jphonesharpsh53Process(headers, browserCaps)) { } else { if (this.Jphonesharpsh07Process(headers, browserCaps)) { } else { if (this.Jphonesharpsh08Process(headers, browserCaps)) { } else { if (this.Jphonesharpsh51Process(headers, browserCaps)) { } else { if (this.Jphonesharpsh52Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } this.JphonesharpProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonesharpsh53ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonesharpsh53ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonesharpsh53Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-SH53"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "12000"; dictionary["mobileDeviceModel"] = "J-SH53"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["screenBitDepth"] = "18"; dictionary["screenCharactersHeight"] = "13"; dictionary["screenCharactersWidth"] = "24"; dictionary["supportsCharacterEntityEncoding"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsInputIStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneSharpSh53"); this.Jphonesharpsh53ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonesharpsh53ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonesharpsh07ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonesharpsh07ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonesharpsh07Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-SH07"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderEmptySelects"] = "false"; dictionary["maximumRenderedPageSize"] = "12000"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["requiresLeadingPageBreak"] = "false"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneSharpSh07"); this.Jphonesharpsh07ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonesharpsh07ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonesharpsh08ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonesharpsh08ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonesharpsh08Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-SH08"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["supportsInputIStyle"] = "false"; dictionary["requiresLeadingPageBreak"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "117"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneSharpSh08"); this.Jphonesharpsh08ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonesharpsh08ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonesharpsh51ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonesharpsh51ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonesharpsh51Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-SH51"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "12000"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["supportsInputIStyle"] = "true"; dictionary["requiresLeadingPageBreak"] = "false"; dictionary["requiresUniqueHtmlCheckboxNames"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneSharpSh51"); this.Jphonesharpsh51ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonesharpsh51ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonesharpsh52ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonesharpsh52ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonesharpsh52Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-SH52\\D*"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "12000"; dictionary["mobileDeviceModel"] = "J-SH52"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenCharactersWidth"] = "20"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsInputIStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneSharpSh52"); this.Jphonesharpsh52ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonesharpsh52ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonetoshibaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonetoshibaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonetoshibaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "J-T\\d"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; browserCaps.AddBrowser("JphoneToshiba"); this.JphonetoshibaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=JphoneToshiba if (this.Jphonetoshibat06aProcess(headers, browserCaps)) { } else { if (this.Jphonetoshibat08Process(headers, browserCaps)) { } else { if (this.Jphonetoshibat51Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.JphonetoshibaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonetoshibat06aProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonetoshibat06aProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonetoshibat06aProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-T06_a"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "12000"; dictionary["mobileDeviceModel"] = "J-T06"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "20"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneToshibaT06a"); this.Jphonetoshibat06aProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonetoshibat06aProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonetoshibat08ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonetoshibat08ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonetoshibat08Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-T08\\D*"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "22"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneToshibaT08"); this.Jphonetoshibat08ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonetoshibat08ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphonetoshibat51ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphonetoshibat51ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphonetoshibat51Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^J-T51"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "12000"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "false"; dictionary["requiresLeadingPageBreak"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "24"; dictionary["screenPixelsHeight"] = "144"; dictionary["screenPixelsWidth"] = "144"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsInputIStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; browserCaps.AddBrowser("JphoneToshibaT51"); this.Jphonetoshibat51ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphonetoshibat51ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphone4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphone4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphone4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "4"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["supportsQueryStringInFormAction"] = "true"; this.Jphone4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphone4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonecolorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonecolorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonecolorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-JPHONE-COLOR"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'colorIndicator\'[CG])(?\'bitDepth\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["bitDepth"] = regexWorker["${bitDepth}"]; dictionary["colorIndicator"] = regexWorker["${colorIndicator}"]; this.JphonecolorProcessGateways(headers, browserCaps); // gateway, parent=JphoneColor this.JphonecoloriscolorProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=JphoneColor if (this.Jphone16bitcolorProcess(headers, browserCaps)) { } else { if (this.Jphone8bitcolorProcess(headers, browserCaps)) { } else { if (this.Jphone2bitcolorProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.JphonecolorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonecoloriscolorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonecoloriscolorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonecoloriscolorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["colorIndicator"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "C"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; this.JphonecoloriscolorProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JphonecoloriscolorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphone16bitcolorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphone16bitcolorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphone16bitcolorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["bitDepth"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^65536$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenBitDepth"] = "16"; this.Jphone16bitcolorProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphone16bitcolorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphone8bitcolorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphone8bitcolorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphone8bitcolorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["bitDepth"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^256$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenBitDepth"] = "8"; this.Jphone8bitcolorProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphone8bitcolorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jphone2bitcolorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jphone2bitcolorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jphone2bitcolorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["bitDepth"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^4$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenBitDepth"] = "2"; this.Jphone2bitcolorProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Jphone2bitcolorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JphonedisplayProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JphonedisplayProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JphonedisplayProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-JPHONE-DISPLAY"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'screenWidth\'\\d+)\\*(?\'screenHeight\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; this.JphonedisplayProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JphonedisplayProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void LegendProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void LegendProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool LegendProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^(?\'deviceID\'LG\\S*) AU\\/(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*)." + "*"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "AU-System"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "false"; dictionary["hasBackButton"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["numberOfSoftkeys"] = "2"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "false"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["type"] = regexWorker["AU ${browserMajorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.AddBrowser("Legend"); this.LegendProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Legend if (this.Lgg5200Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.LegendProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lgg5200ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lgg5200ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lgg5200Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^LG-G5200"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "3500"; dictionary["maximumSoftkeyLabelLength"] = "6"; dictionary["mobileDeviceManufacturer"] = "LEGEND"; dictionary["mobileDeviceModel"] = "G808"; dictionary["screenBitDepth"] = "4"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "17"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "128"; browserCaps.AddBrowser("LGG5200"); this.Lgg5200ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lgg5200ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MmeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MmeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MmeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MMEF"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "Mozilla/(?\'version\'(?\'major\'\\d+)(?\'minor\'\\.\\d+)\\w*).*"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Microsoft Mobile Explorer"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["inputType"] = "telephoneKeypad"; dictionary["isMobileDevice"] = "true"; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["mobileDeviceManufacturer"] = "Microsoft"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["type"] = "Microsoft Mobile Explorer"; dictionary["version"] = regexWorker["${version}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("MME"); this.MmeProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=MME if (this.Mmef20Process(headers, browserCaps)) { } else { if (this.MmemobileexplorerProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.MmeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mmef20ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mmef20ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mmef20Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MMEF20"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderSetvarZeroWithMultiSelectionList"] = "false"; dictionary["defaultCharacterHeight"] = "15"; dictionary["defaultCharacterWidth"] = "5"; dictionary["defaultScreenPixelsHeight"] = "160"; dictionary["defaultScreenPixelsWidth"] = "120"; dictionary["isColor"] = "false"; dictionary["maximumRenderedPageSize"] = "4000"; dictionary["mobileDeviceModel"] = "Simulator"; dictionary["numberOfSoftkeys"] = "2"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml11"; dictionary["screenBitDepth"] = "1"; browserCaps.AddBrowser("MMEF20"); this.Mmef20ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=MMEF20 if (this.MmecellphoneProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Mmef20ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MmemobileexplorerProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MmemobileexplorerProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MmemobileexplorerProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^MobileExplorer/(?\'majorVersion\'\\d*)(?\'minorVersion\'\\.\\d*) \\(Mozilla/1\\.22; compa" + "tible; MMEF\\d+; (?\'manufacturer\'[^;]*); (?\'model\'[^;)]*)(; (?\'deviceID\'[^)]*))?"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderSetvarZeroWithMultiSelectionList"] = "false"; dictionary["defaultCharacterHeight"] = "15"; dictionary["defaultCharacterWidth"] = "5"; dictionary["defaultScreenPixelsHeight"] = "160"; dictionary["defaultScreenPixelsWidth"] = "120"; dictionary["deviceID"] = regexWorker["${deviceID}"]; dictionary["isColor"] = "true"; dictionary["majorVersion"] = regexWorker["${majorVersion}"]; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["minorVersion"] = regexWorker["${minorVersion}"]; dictionary["mobileDeviceManufacturer"] = regexWorker["${manufacturer}"]; dictionary["mobileDeviceModel"] = regexWorker["${model}"]; dictionary["numberOfSoftkeys"] = "2"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "html32"; dictionary["screenBitDepth"] = "8"; dictionary["supportsBodyColor"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsDivAlign"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFontColor"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["version"] = regexWorker["${majorVersion}${minorVersion}"]; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("MMEMobileExplorer"); this.MmemobileexplorerProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MmemobileexplorerProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MmecellphoneProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MmecellphoneProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MmecellphoneProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Mozilla/.*\\(compatible; MMEF(?\'majorVersion\'\\d)(?\'minorVersion\'\\d); Cell[pP]hone(" + "([;,] (?\'deviceID\'[^;]*))(;(?\'buildInfo\'.*))*)*\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canCombineFormsInDeck"] = "false"; dictionary["canRenderPostBackCards"] = "false"; dictionary["deviceID"] = regexWorker["${deviceID}"]; dictionary["majorVersion"] = regexWorker["${majorVersion}"]; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["minorVersion"] = regexWorker[".${minorVersion}"]; dictionary["version"] = regexWorker["${majorVersion}.${minorVersion}"]; browserCaps.AddBrowser("MMECellphone"); this.MmecellphoneProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=MMECellphone if (this.MmebenefonqProcess(headers, browserCaps)) { } else { if (this.Mmesonycmdz5Process(headers, browserCaps)) { } else { if (this.Mmesonycmdj5Process(headers, browserCaps)) { } else { if (this.Mmesonycmdj7Process(headers, browserCaps)) { } else { if (this.MmegenericsmallProcess(headers, browserCaps)) { } else { if (this.MmegenericlargeProcess(headers, browserCaps)) { } else { if (this.MmegenericflipProcess(headers, browserCaps)) { } else { if (this.Mmegeneric3dProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } this.MmecellphoneProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MmebenefonqProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MmebenefonqProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MmebenefonqProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Benefon Q"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Benefon"; dictionary["mobileDeviceModel"] = "Q"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "48"; dictionary["screenPixelsWidth"] = "100"; browserCaps.AddBrowser("MMEBenefonQ"); this.MmebenefonqProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MmebenefonqProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mmesonycmdz5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mmesonycmdz5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mmesonycmdz5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Sony CMD-Z5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "CMD-Z5"; dictionary["requiresOutputOptimization"] = "true"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "60"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("MMESonyCMDZ5"); this.Mmesonycmdz5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=MMESonyCMDZ5 if (this.Mmesonycmdz5pj020eProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Mmesonycmdz5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mmesonycmdz5pj020eProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mmesonycmdz5pj020eProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mmesonycmdz5pj020eProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Pj020e"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenPixelsHeight"] = "65"; browserCaps.AddBrowser("MMESonyCMDZ5Pj020e"); this.Mmesonycmdz5pj020eProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mmesonycmdz5pj020eProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mmesonycmdj5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mmesonycmdj5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mmesonycmdj5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Sony CMD-J5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "CMD-J5"; dictionary["requiresOutputOptimization"] = "true"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "65"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("MMESonyCMDJ5"); this.Mmesonycmdj5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mmesonycmdj5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mmesonycmdj7ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mmesonycmdj7ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mmesonycmdj7Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Sony CMD-J7/J70"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canCombineFormsInDeck"] = "true"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["canRenderSetvarZeroWithMultiSelectionList"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "2900"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["mobileDeviceManufacturer"] = "Ericsson"; dictionary["mobileDeviceModel"] = "T68"; dictionary["numberOfSoftkeys"] = "1"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "false"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "80"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; browserCaps.AddBrowser("MMESonyCMDJ7"); this.Mmesonycmdj7ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mmesonycmdj7ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MmegenericsmallProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MmegenericsmallProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MmegenericsmallProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "GenericSmall"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Microsoft"; dictionary["mobileDeviceModel"] = "Generic Small Skin"; dictionary["screenBitDepth"] = "1"; dictionary["screenPixelsHeight"] = "60"; dictionary["screenPixelsWidth"] = "100"; browserCaps.AddBrowser("MMEGenericSmall"); this.MmegenericsmallProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MmegenericsmallProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MmegenericlargeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MmegenericlargeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MmegenericlargeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "GenericLarge"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Microsoft"; dictionary["mobileDeviceModel"] = "Generic Large Skin"; dictionary["screenBitDepth"] = "1"; dictionary["screenPixelsHeight"] = "240"; dictionary["screenPixelsWidth"] = "160"; browserCaps.AddBrowser("MMEGenericLarge"); this.MmegenericlargeProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MmegenericlargeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MmegenericflipProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MmegenericflipProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MmegenericflipProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "GenericFlip"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Microsoft"; dictionary["mobileDeviceModel"] = "Generic Flip Skin"; dictionary["screenBitDepth"] = "1"; dictionary["screenPixelsHeight"] = "200"; dictionary["screenPixelsWidth"] = "160"; browserCaps.AddBrowser("MMEGenericFlip"); this.MmegenericflipProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MmegenericflipProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mmegeneric3dProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mmegeneric3dProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mmegeneric3dProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Generic3D"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Microsoft"; dictionary["mobileDeviceModel"] = "Generic 3D Skin"; dictionary["screenBitDepth"] = "1"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "128"; browserCaps.AddBrowser("MMEGeneric3D"); this.Mmegeneric3dProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mmegeneric3dProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Netscape3ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Netscape3ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Netscape3Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Mozilla/3"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "Opera"); if ((result == true)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "AvantGo"); if ((result == true)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "MSIE"); if ((result == true)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Netscape"; dictionary["cookies"] = "true"; dictionary["css1"] = "true"; dictionary["ecmascriptversion"] = "1.1"; dictionary["frames"] = "true"; dictionary["isColor"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["supportsCss"] = "false"; dictionary["supportsFileUpload"] = "true"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "Netscape3"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("Netscape3"); this.Netscape3ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Netscape3ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Netscape4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Netscape4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Netscape4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Mozilla/4"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "Opera"); if ((result == true)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "MSIE"); if ((result == true)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Netscape"; dictionary["cookies"] = "true"; dictionary["css1"] = "true"; dictionary["ecmascriptversion"] = "1.3"; dictionary["frames"] = "true"; dictionary["isColor"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["supportsCss"] = "false"; dictionary["supportsFileUpload"] = "true"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "Netscape4"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("Netscape4"); this.Netscape4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Netscape4 if (this.CasiopeiaProcess(headers, browserCaps)) { } else { if (this.PalmwebproProcess(headers, browserCaps)) { } else { if (this.NetfrontProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.Netscape4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Netscape5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Netscape5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Netscape5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Mozilla/5\\.0 \\([^)]*\\) (Gecko/[-\\d]+ )?Netscape\\d?/(?\'version\'(?\'major\'\\d+)(?\'mi" + "nor\'\\.\\d+)(?\'letters\'\\w*))"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Netscape"; dictionary["cookies"] = "true"; dictionary["css1"] = "true"; dictionary["css2"] = "true"; dictionary["ecmascriptversion"] = "1.5"; dictionary["frames"] = "true"; dictionary["isColor"] = "true"; dictionary["javaapplets"] = "true"; dictionary["javascript"] = "true"; dictionary["letters"] = regexWorker["${letters}"]; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["preferredImageMime"] = "image/gif"; dictionary["screenBitDepth"] = "8"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["supportsVCard"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["Netscape${major}"]; dictionary["version"] = regexWorker["${version}"]; dictionary["w3cdomversion"] = "1.0"; dictionary["xml"] = "true"; browserCaps.AddBrowser("Netscape5"); this.Netscape5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Netscape5 if (this.Netscape6to9Process(headers, browserCaps)) { } else { if (this.NetscapebetaProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.Netscape5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Netscape6to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Netscape6to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Netscape6to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "[6-9]\\."); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsXmlHttp"] = "true"; dictionary["supportsCallback"] = "true"; dictionary["supportsMaintainScrollPositionOnPostback"] = "true"; browserCaps.AddBrowser("Netscape6to9"); this.Netscape6to9ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Netscape6to9 if (this.Netscape6to9betaProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Netscape6to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Netscape6to9betaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Netscape6to9betaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Netscape6to9betaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["letters"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^b"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; browserCaps.AddBrowser("Netscape6to9Beta"); this.Netscape6to9betaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Netscape6to9betaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void NetscapebetaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void NetscapebetaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool NetscapebetaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["letters"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^b"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; browserCaps.AddBrowser("NetscapeBeta"); this.NetscapebetaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.NetscapebetaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void NokiaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void NokiaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool NokiaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Nokia"; dictionary["cookies"] = "false"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canRenderOneventAndPrevElementsTogether"] = "false"; dictionary["canRenderPostBackCards"] = "false"; dictionary["canSendMail"] = "false"; dictionary["defaultScreenCharactersHeight"] = "4"; dictionary["defaultScreenCharactersWidth"] = "20"; dictionary["defaultScreenPixelsHeight"] = "40"; dictionary["defaultScreenPixelsWidth"] = "90"; dictionary["hasBackButton"] = "false"; dictionary["inputType"] = "telephoneKeypad"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["maximumRenderedPageSize"] = "1397"; dictionary["mobileDeviceManufacturer"] = "Nokia"; dictionary["numberOfSoftkeys"] = "2"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml11"; dictionary["rendersBreaksAfterWmlAnchor"] = "true"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "false"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresPhoneNumbersAsPlainText"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["type"] = "Nokia"; browserCaps.AddBrowser("Nokia"); this.NokiaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Nokia if (this.NokiablueprintProcess(headers, browserCaps)) { } else { if (this.NokiawapsimulatorProcess(headers, browserCaps)) { } else { if (this.NokiamobilebrowserProcess(headers, browserCaps)) { } else { if (this.Nokia7110Process(headers, browserCaps)) { } else { if (this.Nokia6220Process(headers, browserCaps)) { } else { if (this.Nokia6250Process(headers, browserCaps)) { } else { if (this.Nokia6310Process(headers, browserCaps)) { } else { if (this.Nokia6510Process(headers, browserCaps)) { } else { if (this.Nokia8310Process(headers, browserCaps)) { } else { if (this.Nokia9110iProcess(headers, browserCaps)) { } else { if (this.Nokia9110Process(headers, browserCaps)) { } else { if (this.Nokia3330Process(headers, browserCaps)) { } else { if (this.Nokia9210Process(headers, browserCaps)) { } else { if (this.Nokia9210htmlProcess(headers, browserCaps)) { } else { if (this.Nokia3590Process(headers, browserCaps)) { } else { if (this.Nokia3595Process(headers, browserCaps)) { } else { if (this.Nokia3560Process(headers, browserCaps)) { } else { if (this.Nokia3650Process(headers, browserCaps)) { } else { if (this.Nokia5100Process(headers, browserCaps)) { } else { if (this.Nokia6200Process(headers, browserCaps)) { } else { if (this.Nokia6590Process(headers, browserCaps)) { } else { if (this.Nokia6800Process(headers, browserCaps)) { } else { if (this.Nokia7650Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } } } } } } } } } } } } } } } this.NokiaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void NokiablueprintProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void NokiablueprintProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool NokiablueprintProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia\\-WAP\\-Toolkit\\/(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "false"; dictionary["cookies"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "65536"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "Blueprint Simulator"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreaksAfterWmlAnchor"] = "false"; dictionary["type"] = "Nokia WAP Toolkit"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.AddBrowser("NokiaBlueprint"); this.NokiablueprintProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.NokiablueprintProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void NokiawapsimulatorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void NokiawapsimulatorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool NokiawapsimulatorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia\\-MIT\\-Browser\\/(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderOnEventAndPrevElementsTogether"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["cookies"] = "true"; dictionary["hasBackButton"] = "true"; dictionary["inputType"] = "virtualKeyboard"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "3584"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "WAP Simulator"; dictionary["rendersBreaksAfterWmlAnchor"] = "false"; dictionary["requiresPhoneNumbersAsPlainText"] = "false"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "25"; dictionary["screenCharactersWidth"] = "32"; dictionary["screenPixelsHeight"] = "512"; dictionary["screenPixelsWidth"] = "384"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["type"] = "Nokia Mobile Internet Toolkit"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.AddBrowser("NokiaWapSimulator"); this.NokiawapsimulatorProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.NokiawapsimulatorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void NokiamobilebrowserProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void NokiamobilebrowserProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool NokiamobilebrowserProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia Mobile Browser (?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canInitiateVoiceCall"] = "false"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "25000"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = regexWorker["Mobile Browser ${browserMajorVersion}${browserMinorVersion}"]; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "application/vnd.wap.xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "true"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresPostRedirectionHandling"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "14"; dictionary["screenCharactersWidth"] = "24"; dictionary["screenPixelsHeight"] = "255"; dictionary["screenPixelsWidth"] = "180"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["supportsStyleElement"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["Nokia Mobile Browser ${browserMajorVersion}${browserMinorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.HtmlTextWriter = "System.Web.UI.XhtmlTextWriter"; browserCaps.AddBrowser("NokiaMobileBrowser"); this.NokiamobilebrowserProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.NokiamobilebrowserProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia7110ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia7110ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia7110Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia7110/1\\.0 \\((?\'versionString\'(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersi" + "on\'\\.\\w*).*)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "7110"; dictionary["optimumPageWeight"] = "800"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "22"; dictionary["screenPixelsHeight"] = "44"; dictionary["screenPixelsWidth"] = "96"; dictionary["type"] = "Nokia 7110"; dictionary["version"] = regexWorker["${versionString}"]; browserCaps.AddBrowser("Nokia7110"); this.Nokia7110ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia7110ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia6220ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia6220ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia6220Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia6210/1\\.0 \\((?\'versionString\'(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersi" + "on\'\\.\\w*).*)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "6210"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "22"; dictionary["screenPixelsHeight"] = "41"; dictionary["screenPixelsWidth"] = "96"; dictionary["type"] = "Nokia 6210"; dictionary["version"] = regexWorker["${versionString}"]; browserCaps.AddBrowser("Nokia6220"); this.Nokia6220ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia6220ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia6250ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia6250ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia6250Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia6250/1\\.0 \\((?\'versionString\'(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersi" + "on\'\\.\\w*).*)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "6250"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "22"; dictionary["screenPixelsHeight"] = "41"; dictionary["screenPixelsWidth"] = "96"; dictionary["type"] = "Nokia 6250"; dictionary["version"] = regexWorker["${versionString}"]; browserCaps.AddBrowser("Nokia6250"); this.Nokia6250ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia6250ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia6310ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia6310ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia6310Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia6310/1\\.0 \\((?\'versionString\'(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersi" + "on\'\\.\\w*).*)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderOneventAndPrevElementsTogether"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["cookies"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "2800"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "6310"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreaksAfterWmlAnchor"] = "false"; dictionary["rendersBreaksAfterWmlInput"] = "false"; dictionary["requiresPhoneNumbersAsPlainText"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "45"; dictionary["screenPixelsWidth"] = "92"; dictionary["type"] = "Nokia 6310"; dictionary["version"] = regexWorker["${versionString}"]; browserCaps.AddBrowser("Nokia6310"); this.Nokia6310ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia6310ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia6510ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia6510ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia6510Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia6510/1\\.0 \\((?\'versionString\'(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersi" + "on\'\\.\\w*).*)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderOnEventAndPrevElementsTogether"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["cookies"] = "true"; dictionary["hasBackButton"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "2800"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "6510"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingType"] = "wml12"; dictionary["requiresPhoneNumbersAsPlainText"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "45"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["type"] = "Nokia 6510"; dictionary["version"] = regexWorker["${versionString}"]; browserCaps.AddBrowser("Nokia6510"); this.Nokia6510ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia6510ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia8310ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia8310ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia8310Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia8310/1\\.0 \\((?\'versionString\'(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersi" + "on\'\\.\\w*).*)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderOneventAndPrevElementsTogether"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "2700"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "8310"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreaksAfterWmlAnchor"] = "false"; dictionary["rendersBreaksAfterWmlInput"] = "false"; dictionary["requiresPhoneNumbersAsPlainText"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "3"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "39"; dictionary["screenPixelsWidth"] = "78"; dictionary["type"] = "Nokia 8310"; dictionary["version"] = regexWorker["${versionString}"]; browserCaps.AddBrowser("Nokia8310"); this.Nokia8310ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia8310ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia9110iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia9110iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia9110iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia9110/1\\.0"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["maximumRenderedPageSize"] = "8192"; dictionary["mobileDeviceModel"] = "9110i Communicator"; dictionary["rendersBreaksAfterWmlAnchor"] = "false"; dictionary["screenBitDepth"] = "4"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "60"; dictionary["screenPixelsHeight"] = "180"; dictionary["screenPixelsWidth"] = "400"; dictionary["type"] = "Nokia 9110"; browserCaps.AddBrowser("Nokia9110i"); this.Nokia9110iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia9110iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia9110ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia9110ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia9110Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia-9110"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "false"; dictionary["canSendMail"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["maximumRenderedPageSize"] = "150000"; dictionary["mobileDeviceModel"] = "Nokia 9110"; dictionary["numberOfSoftkeys"] = "0"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "html32"; dictionary["rendersBreaksAfterHtmlLists"] = "false"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "11"; dictionary["screenCharactersWidth"] = "57"; dictionary["screenPixelsHeight"] = "200"; dictionary["screenPixelsWidth"] = "540"; dictionary["supportsAccesskeyAttribute"] = "true"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsBold"] = "true"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsSelectMultiple"] = "false"; dictionary["tables"] = "true"; dictionary["type"] = "Nokia 9110"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("Nokia9110"); this.Nokia9110ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia9110ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia3330ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia3330ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia3330Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia3330/1\\.0 \\((?\'versionString\'(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersi" + "on\'\\.\\w*).*)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hasBackButton"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "2800"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "3330"; dictionary["screenCharactersHeight"] = "3"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "39"; dictionary["screenPixelsWidth"] = "78"; dictionary["type"] = "Nokia 3330"; dictionary["version"] = regexWorker["${versionString}"]; browserCaps.AddBrowser("Nokia3330"); this.Nokia3330ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia3330ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia9210ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia9210ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia9210Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia9210/1\\.0"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "8192"; dictionary["mobileDeviceModel"] = "9210 Communicator"; dictionary["rendersBreaksAfterWmlAnchor"] = "false"; dictionary["rendersBreaksAfterWmlInput"] = "false"; dictionary["requiresNoSoftkeyLabels"] = "true"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "75"; dictionary["screenPixelsHeight"] = "165"; dictionary["screenPixelsWidth"] = "490"; dictionary["supportsCacheControlMetaTag"] = "false"; dictionary["type"] = "Nokia 9210"; browserCaps.AddBrowser("Nokia9210"); this.Nokia9210ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia9210ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia9210htmlProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia9210htmlProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia9210htmlProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "EPOC32-WTL/2\\.2 Crystal/6\\.0 STNC-WTL/"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; browserCaps.AddBrowser("Nokia9210HTML"); this.Nokia9210htmlProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia9210htmlProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia3590ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia3590ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia3590Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Nokia3590/(?\'version\'(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*)\\S*" + ")"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "3200"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "3590"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "true"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsBold"] = "true"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "Nokia 3590"; dictionary["version"] = regexWorker["${version}"]; browserCaps.AddBrowser("Nokia3590"); this.Nokia3590ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Nokia3590 if (this.Nokia3590v1Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Nokia3590ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia3590v1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia3590v1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia3590v1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia3590/1\\.0\\(7\\."); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "8020"; dictionary["preferredRequestEncoding"] = "iso-8859-1"; dictionary["preferredResponseEncoding"] = "utf-8"; dictionary["screenPixelsHeight"] = "65"; dictionary["supportsCss"] = "true"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsTitleElement"] = "true"; dictionary["tables"] = "false"; browserCaps.AddBrowser("Nokia3590V1"); this.Nokia3590v1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia3590v1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia3595ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia3595ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia3595Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia3595/(?\'version\'(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*)\\S*)" + ""); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "15700"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "3595"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["preferredRequestEncoding"] = "iso-8859-1"; dictionary["preferredResponseEncoding"] = "utf-8"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersWidth"] = "17"; dictionary["screenPixelsHeight"] = "132"; dictionary["screenPixelsWidth"] = "176"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "Nokia 3595"; dictionary["version"] = regexWorker["${version}"]; browserCaps.AddBrowser("Nokia3595"); this.Nokia3595ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia3595ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia3560ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia3560ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia3560Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia3560"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "28"; dictionary["screenPixelsHeight"] = "176"; dictionary["screenPixelsWidth"] = "208"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("Nokia3560"); this.Nokia3560ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia3560ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia3650ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia3650ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia3650Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia3650/(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*).* Series60/(?\'" + "platformVersion\'\\S*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "3650"; dictionary["mobilePlatformVersion"] = regexWorker["${platformVersion}"]; dictionary["type"] = "Nokia 3650"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.AddBrowser("Nokia3650"); this.Nokia3650ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Nokia3650 if (this.Nokia3650p12plusProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Nokia3650ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia3650p12plusProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia3650p12plusProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia3650p12plusProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobilePlatformVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "[^01]\\.|1\\.[^01]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "28"; dictionary["screenPixelsHeight"] = "176"; dictionary["screenPixelsWidth"] = "208"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; dictionary["tagwriter"] = "System.Web.UI.XhtmlTextWriter"; browserCaps.AddBrowser("Nokia3650P12Plus"); this.Nokia3650p12plusProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia3650p12plusProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia5100ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia5100ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia5100Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia5100/(?\'version\'(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*)\\S*)" + ""); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderOnEventAndPrevElementsTogether"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["isColor"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "3500"; dictionary["maximumSoftkeyLabelLength"] = "14"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "5100"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["rendersWmlSelectsAsMenuCards"] = "true"; dictionary["requiresPhoneNumbersAsPlainText"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "15"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "128"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["type"] = "Nokia 5100"; dictionary["version"] = regexWorker["${version}"]; browserCaps.AddBrowser("Nokia5100"); this.Nokia5100ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia5100ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia6200ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia6200ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia6200Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia6200/(?\'version\'(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*)\\S*)" + ""); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "6200"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["preferredRequestEncoding"] = "iso-8859-1"; dictionary["preferredResponseEncoding"] = "utf-8"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "19"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "128"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsNoWrapStyle"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "Nokia 6200"; dictionary["version"] = regexWorker["${version}"]; browserCaps.AddBrowser("Nokia6200"); this.Nokia6200ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia6200ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia6590ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia6590ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia6590Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Nokia6590/(?\'versionString\'(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\" + "d*)\\S*) "); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "9800"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "6590"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "true"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsBold"] = "true"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "Nokia 6590"; dictionary["version"] = regexWorker["${versionString}"]; browserCaps.AddBrowser("Nokia6590"); this.Nokia6590ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia6590ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia6800ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia6800ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia6800Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Nokia6800/(?\'version\'(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*)\\S*)" + ""); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderOnEventAndPrevElementsTogether"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["hasBackButton"] = "true"; dictionary["isColor"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "3500"; dictionary["maximumSoftkeyLabelLength"] = "14"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "6800"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingType"] = "wml12"; dictionary["requiresPhoneNumbersAsPlainText"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "15"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "128"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["type"] = "Nokia 6800"; dictionary["version"] = regexWorker["${version}"]; browserCaps.AddBrowser("Nokia6800"); this.Nokia6800ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia6800ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokia7650ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokia7650ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokia7650Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Nokia7650/(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*).*"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderOnEventAndPrevElementsTogether"] = "true"; dictionary["canRenderPostBackCards"] = "true"; dictionary["cookies"] = "true"; dictionary["hasBackButton"] = "true"; dictionary["isColor"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "3500"; dictionary["maximumSoftkeyLabelLength"] = "18"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceModel"] = "7650"; dictionary["numberOfSoftkeys"] = "3"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingType"] = "wml12"; dictionary["requiresPhoneNumbersAsPlainText"] = "false"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "28"; dictionary["screenPixelsHeight"] = "208"; dictionary["screenPixelsWidth"] = "176"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["type"] = "Nokia 7650"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.AddBrowser("Nokia7650"); this.Nokia7650ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokia7650ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void NokiamobilebrowserrainbowProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void NokiamobilebrowserrainbowProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool NokiamobilebrowserrainbowProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Rainbow/(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Nokia"; dictionary["canInitiateVoiceCall"] = "false"; dictionary["canSendMail"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["javascript"] = "false"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "25000"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceManufacturer"] = "Nokia"; dictionary["mobileDeviceModel"] = regexWorker["Mobile Browser ${browserMajorVersion}${browserMinorVersion}"]; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "application/vnd.wap.xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresInputTypeAttribute"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "14"; dictionary["screenCharactersWidth"] = "24"; dictionary["screenPixelsHeight"] = "255"; dictionary["screenPixelsWidth"] = "180"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyColor"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsDivAlign"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFontColor"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsQueryStringInFormAction"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["supportsStyleElement"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["Nokia Mobile Browser ${browserMajorVersion}${browserMinorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.XhtmlTextWriter"; browserCaps.AddBrowser("NokiaMobileBrowserRainbow"); this.NokiamobilebrowserrainbowProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.NokiamobilebrowserrainbowProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokiaepoc32wtlProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokiaepoc32wtlProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokiaepoc32wtlProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "EPOC32-WTL/(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "EPOC"; dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["canSendMail"] = "false"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["maximumRenderedPageSize"] = "150000"; dictionary["mobileDeviceManufacturer"] = "Nokia"; dictionary["mobileDeviceModel"] = "Nokia 9210"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["rendersBreaksAfterHtmlLists"] = "false"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "54"; dictionary["screenPixelsHeight"] = "170"; dictionary["screenPixelsWidth"] = "478"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsSelectMultiple"] = "false"; dictionary["supportsEmptyStringInCookieValue"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = "Nokia Epoc"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("NokiaEpoc32wtl"); this.Nokiaepoc32wtlProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=NokiaEpoc32wtl if (this.Nokiaepoc32wtl20Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Nokiaepoc32wtlProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nokiaepoc32wtl20ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nokiaepoc32wtl20ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nokiaepoc32wtl20Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "2\\.0"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderEmptySelects"] = "false"; dictionary["canSendMail"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "false"; dictionary["maximumRenderedPageSize"] = "7168"; dictionary["mobileDeviceManufacturer"] = "Psion"; dictionary["mobileDeviceModel"] = "Series 7"; dictionary["rendersBreaksAfterHtmlLists"] = "true"; dictionary["requiresAttributeColonSubstitution"] = "false"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["requiresUniqueHtmlCheckboxNames"] = "true"; dictionary["screenCharactersHeight"] = "31"; dictionary["screenCharactersWidth"] = "69"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; dictionary["SupportsEmptyStringInCookieValue"] = "true"; browserCaps.AddBrowser("NokiaEpoc32wtl20"); this.Nokiaepoc32wtl20ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nokiaepoc32wtl20ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(UP\\.Browser)|(UP/)"); if ((result == false)) { return false; } headerValue = ((string)(browserCaps[string.Empty])); result = regexWorker.ProcessRegex(headerValue, "Go\\.Web"); if ((result == true)) { return false; } // Capture: header values regexWorker.ProcessRegex(((string)(browserCaps[string.Empty])), @"((?'deviceID'\S*) UP/\S* UP\.Browser/((?'browserMajorVersion'\d*)(?'browserMinorVersion'\.\d*)\S*) UP\.Link/)|((?'deviceID'\S*)/\S* UP(\.Browser)*/((?'browserMajorVersion'\d*)(?'browserMinorVersion'\.\d*)\S*))|(UP\.Browser/((?'browserMajorVersion'\d*)(?'browserMinorVersion'\.\d*)\S*)-(?'deviceID'\S*) UP\.Link/)|((?'deviceID'\S*) UP\.Browser/((?'browserMajorVersion'\d*)(?'browserMinorVersion'\.\d*)\S*) UP\.Link/)|((?'deviceID'\S*)/(?'DeviceVersion'\S*) UP/((?'browserMajorVersion'\d*)(?'browserMinorVersion'\.\d*)\S*))|((?'deviceID'\S*)/(?'DeviceVersion'\S*) UP.Browser/((?'browserMajorVersion'\d*)(?'browserMinorVersion'\.\d*)\S*))|((?'deviceID'\S*) UP.Browser/((?'browserMajorVersion'\d*)(?'browserMinorVersion'\.\d*)\S*))"); // Capabilities: set capabilities dictionary["browser"] = "Phone.com"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canSendMail"] = "false"; dictionary["deviceID"] = regexWorker["${deviceID}"]; dictionary["deviceVersion"] = regexWorker["${deviceVersion}"]; dictionary["inputType"] = "telephoneKeypad"; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "1492"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["numberOfSoftkeys"] = "2"; dictionary["optimumPageWeight"] = "700"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml11"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "false"; dictionary["rendersWmlSelectsAsMenuCards"] = "true"; dictionary["requiresFullyQualifiedRedirectUrl"] = "true"; dictionary["requiresNoescapedPostUrl"] = "true"; dictionary["requiresPostRedirectionHandling"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["type"] = regexWorker["Phone.com${browserMajorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.AddBrowser("Up"); this.UpProcessGateways(headers, browserCaps); // gateway, parent=Up this.UpdefaultscreencharactersProcess(headers, browserCaps); // gateway, parent=Up this.UpdefaultscreenpixelsProcess(headers, browserCaps); // gateway, parent=Up this.UpscreendepthProcess(headers, browserCaps); // gateway, parent=Up this.UpscreencharsProcess(headers, browserCaps); // gateway, parent=Up this.UpscreenpixelsProcess(headers, browserCaps); // gateway, parent=Up this.UpmsizeProcess(headers, browserCaps); // gateway, parent=Up this.IscolorProcess(headers, browserCaps); // gateway, parent=Up this.UpnumsoftkeysProcess(headers, browserCaps); // gateway, parent=Up this.UpsoftkeysizeProcess(headers, browserCaps); // gateway, parent=Up this.UpmaxpduProcess(headers, browserCaps); // gateway, parent=Up this.UpversionProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Up if (this.AumicProcess(headers, browserCaps)) { } else { if (this.Alcatelbe4Process(headers, browserCaps)) { } else { if (this.Alcatelbe5Process(headers, browserCaps)) { } else { if (this.Alcatelbe3Process(headers, browserCaps)) { } else { if (this.Alcatelbf3Process(headers, browserCaps)) { } else { if (this.Alcatelbf4Process(headers, browserCaps)) { } else { if (this.MotcbProcess(headers, browserCaps)) { } else { if (this.Motf5Process(headers, browserCaps)) { } else { if (this.Motd8Process(headers, browserCaps)) { } else { if (this.MotcfProcess(headers, browserCaps)) { } else { if (this.Motf6Process(headers, browserCaps)) { } else { if (this.MotbcProcess(headers, browserCaps)) { } else { if (this.MotdcProcess(headers, browserCaps)) { } else { if (this.MotpancProcess(headers, browserCaps)) { } else { if (this.Motc4Process(headers, browserCaps)) { } else { if (this.MccaProcess(headers, browserCaps)) { } else { if (this.Mot2000Process(headers, browserCaps)) { } else { if (this.Motp2kcProcess(headers, browserCaps)) { } else { if (this.MotafProcess(headers, browserCaps)) { } else { if (this.Motc2Process(headers, browserCaps)) { } else { if (this.XeniumProcess(headers, browserCaps)) { } else { if (this.Sagem959Process(headers, browserCaps)) { } else { if (this.Sgha300Process(headers, browserCaps)) { } else { if (this.Sghn100Process(headers, browserCaps)) { } else { if (this.C304saProcess(headers, browserCaps)) { } else { if (this.Sy11Process(headers, browserCaps)) { } else { if (this.St12Process(headers, browserCaps)) { } else { if (this.Sy14Process(headers, browserCaps)) { } else { if (this.Sies40Process(headers, browserCaps)) { } else { if (this.Siesl45Process(headers, browserCaps)) { } else { if (this.Sies35Process(headers, browserCaps)) { } else { if (this.Sieme45Process(headers, browserCaps)) { } else { if (this.Sies45Process(headers, browserCaps)) { } else { if (this.Gm832Process(headers, browserCaps)) { } else { if (this.Gm910iProcess(headers, browserCaps)) { } else { if (this.Mot32Process(headers, browserCaps)) { } else { if (this.Mot28Process(headers, browserCaps)) { } else { if (this.D2Process(headers, browserCaps)) { } else { if (this.PpatProcess(headers, browserCaps)) { } else { if (this.AlazProcess(headers, browserCaps)) { } else { if (this.Cdm9100Process(headers, browserCaps)) { } else { if (this.Cdm135Process(headers, browserCaps)) { } else { if (this.Cdm9000Process(headers, browserCaps)) { } else { if (this.C303caProcess(headers, browserCaps)) { } else { if (this.C311caProcess(headers, browserCaps)) { } else { if (this.C202deProcess(headers, browserCaps)) { } else { if (this.C409caProcess(headers, browserCaps)) { } else { if (this.C402deProcess(headers, browserCaps)) { } else { if (this.Ds15Process(headers, browserCaps)) { } else { if (this.Tp2200Process(headers, browserCaps)) { } else { if (this.Tp120Process(headers, browserCaps)) { } else { if (this.Ds10Process(headers, browserCaps)) { } else { if (this.R280Process(headers, browserCaps)) { } else { if (this.C201hProcess(headers, browserCaps)) { } else { if (this.S71Process(headers, browserCaps)) { } else { if (this.C302hProcess(headers, browserCaps)) { } else { if (this.C309hProcess(headers, browserCaps)) { } else { if (this.C407hProcess(headers, browserCaps)) { } else { if (this.C451hProcess(headers, browserCaps)) { } else { if (this.R201Process(headers, browserCaps)) { } else { if (this.P21Process(headers, browserCaps)) { } else { if (this.Kyocera702gProcess(headers, browserCaps)) { } else { if (this.Kyocera703gProcess(headers, browserCaps)) { } else { if (this.Kyocerac307kProcess(headers, browserCaps)) { } else { if (this.Tk01Process(headers, browserCaps)) { } else { if (this.Tk02Process(headers, browserCaps)) { } else { if (this.Tk03Process(headers, browserCaps)) { } else { if (this.Tk04Process(headers, browserCaps)) { } else { if (this.Tk05Process(headers, browserCaps)) { } else { if (this.D303kProcess(headers, browserCaps)) { } else { if (this.D304kProcess(headers, browserCaps)) { } else { if (this.Qcp2035Process(headers, browserCaps)) { } else { if (this.Qcp3035Process(headers, browserCaps)) { } else { if (this.D512Process(headers, browserCaps)) { } else { if (this.Dm110Process(headers, browserCaps)) { } else { if (this.Tm510Process(headers, browserCaps)) { } else { if (this.Lg13Process(headers, browserCaps)) { } else { if (this.P100Process(headers, browserCaps)) { } else { if (this.Lgc875fProcess(headers, browserCaps)) { } else { if (this.Lgp680fProcess(headers, browserCaps)) { } else { if (this.Lgp7800fProcess(headers, browserCaps)) { } else { if (this.Lgc840fProcess(headers, browserCaps)) { } else { if (this.Lgi2100Process(headers, browserCaps)) { } else { if (this.Lgp7300fProcess(headers, browserCaps)) { } else { if (this.Sd500Process(headers, browserCaps)) { } else { if (this.Tp1100Process(headers, browserCaps)) { } else { if (this.Tp3000Process(headers, browserCaps)) { } else { if (this.T250Process(headers, browserCaps)) { } else { if (this.Mo01Process(headers, browserCaps)) { } else { if (this.Mo02Process(headers, browserCaps)) { } else { if (this.Mc01Process(headers, browserCaps)) { } else { if (this.McccProcess(headers, browserCaps)) { } else { if (this.Mcc9Process(headers, browserCaps)) { } else { if (this.Nk00Process(headers, browserCaps)) { } else { if (this.Mai12Process(headers, browserCaps)) { } else { if (this.Ma112Process(headers, browserCaps)) { } else { if (this.Ma13Process(headers, browserCaps)) { } else { if (this.Mac1Process(headers, browserCaps)) { } else { if (this.Mat1Process(headers, browserCaps)) { } else { if (this.Sc01Process(headers, browserCaps)) { } else { if (this.Sc03Process(headers, browserCaps)) { } else { if (this.Sc02Process(headers, browserCaps)) { } else { if (this.Sc04Process(headers, browserCaps)) { } else { if (this.Sg08Process(headers, browserCaps)) { } else { if (this.Sc13Process(headers, browserCaps)) { } else { if (this.Sc11Process(headers, browserCaps)) { } else { if (this.Sec01Process(headers, browserCaps)) { } else { if (this.Sc10Process(headers, browserCaps)) { } else { if (this.Sy12Process(headers, browserCaps)) { } else { if (this.St11Process(headers, browserCaps)) { } else { if (this.Sy13Process(headers, browserCaps)) { } else { if (this.Syc1Process(headers, browserCaps)) { } else { if (this.Sy01Process(headers, browserCaps)) { } else { if (this.Syt1Process(headers, browserCaps)) { } else { if (this.Sty2Process(headers, browserCaps)) { } else { if (this.Sy02Process(headers, browserCaps)) { } else { if (this.Sy03Process(headers, browserCaps)) { } else { if (this.Si01Process(headers, browserCaps)) { } else { if (this.Sni1Process(headers, browserCaps)) { } else { if (this.Sn11Process(headers, browserCaps)) { } else { if (this.Sn12Process(headers, browserCaps)) { } else { if (this.Sn134Process(headers, browserCaps)) { } else { if (this.Sn156Process(headers, browserCaps)) { } else { if (this.Snc1Process(headers, browserCaps)) { } else { if (this.Tsc1Process(headers, browserCaps)) { } else { if (this.Tsi1Process(headers, browserCaps)) { } else { if (this.Ts11Process(headers, browserCaps)) { } else { if (this.Ts12Process(headers, browserCaps)) { } else { if (this.Ts13Process(headers, browserCaps)) { } else { if (this.Tst1Process(headers, browserCaps)) { } else { if (this.Tst2Process(headers, browserCaps)) { } else { if (this.Tst3Process(headers, browserCaps)) { } else { if (this.Ig01Process(headers, browserCaps)) { } else { if (this.Ig02Process(headers, browserCaps)) { } else { if (this.Ig03Process(headers, browserCaps)) { } else { if (this.Qc31Process(headers, browserCaps)) { } else { if (this.Qc12Process(headers, browserCaps)) { } else { if (this.Qc32Process(headers, browserCaps)) { } else { if (this.Sp01Process(headers, browserCaps)) { } else { if (this.ShProcess(headers, browserCaps)) { } else { if (this.Upg1Process(headers, browserCaps)) { } else { if (this.Opwv1Process(headers, browserCaps)) { } else { if (this.AlavProcess(headers, browserCaps)) { } else { if (this.Im1kProcess(headers, browserCaps)) { } else { if (this.Nt95Process(headers, browserCaps)) { } else { if (this.Mot2001Process(headers, browserCaps)) { } else { if (this.Motv200Process(headers, browserCaps)) { } else { if (this.Mot72Process(headers, browserCaps)) { } else { if (this.Mot76Process(headers, browserCaps)) { } else { if (this.Scp6000Process(headers, browserCaps)) { } else { if (this.Motd5Process(headers, browserCaps)) { } else { if (this.Motf0Process(headers, browserCaps)) { } else { if (this.Sgha400Process(headers, browserCaps)) { } else { if (this.Sec03Process(headers, browserCaps)) { } else { if (this.Siec3iProcess(headers, browserCaps)) { } else { if (this.Sn17Process(headers, browserCaps)) { } else { if (this.Scp4700Process(headers, browserCaps)) { } else { if (this.Sec02Process(headers, browserCaps)) { } else { if (this.Sy15Process(headers, browserCaps)) { } else { if (this.Db520Process(headers, browserCaps)) { } else { if (this.L430v03j02Process(headers, browserCaps)) { } else { if (this.OpwvsdkProcess(headers, browserCaps)) { } else { if (this.Kddica21Process(headers, browserCaps)) { } else { if (this.Kddits21Process(headers, browserCaps)) { } else { if (this.Kddisa21Process(headers, browserCaps)) { } else { if (this.Km100Process(headers, browserCaps)) { } else { if (this.Lgelx5350Process(headers, browserCaps)) { } else { if (this.Hitachip300Process(headers, browserCaps)) { } else { if (this.Sies46Process(headers, browserCaps)) { } else { if (this.Motorolav60gProcess(headers, browserCaps)) { } else { if (this.Motorolav708Process(headers, browserCaps)) { } else { if (this.Motorolav708aProcess(headers, browserCaps)) { } else { if (this.Motorolae360Process(headers, browserCaps)) { } else { if (this.Sonyericssona1101sProcess(headers, browserCaps)) { } else { if (this.Philipsfisio820Process(headers, browserCaps)) { } else { if (this.Casioa5302Process(headers, browserCaps)) { } else { if (this.Tcll668Process(headers, browserCaps)) { } else { if (this.Kddits24Process(headers, browserCaps)) { } else { if (this.Sies55Process(headers, browserCaps)) { } else { if (this.Sharpgx10Process(headers, browserCaps)) { } else { if (this.BenqathenaProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } this.UpProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpdefaultscreencharactersProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpdefaultscreencharactersProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpdefaultscreencharactersProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-SCREENCHARS"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^$"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["defaultScreenCharactersWidth"] = "15"; dictionary["defaultScreenCharactersHeight"] = "4"; this.UpdefaultscreencharactersProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpdefaultscreencharactersProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpdefaultscreenpixelsProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpdefaultscreenpixelsProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpdefaultscreenpixelsProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-SCREENPIXELS"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^$"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["defaultScreenPixelsWidth"] = "120"; dictionary["defaultScreenPixelsHeight"] = "40"; this.UpdefaultscreenpixelsProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpdefaultscreenpixelsProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpscreendepthProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpscreendepthProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpscreendepthProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-SCREENDEPTH"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'screenDepth\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["screenBitDepth"] = regexWorker["${screenDepth}"]; this.UpscreendepthProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpscreendepthProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpscreencharsProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpscreencharsProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpscreencharsProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-SCREENCHARS"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'screenCharsWidth\'[1-9]\\d*),(?\'screenCharsHeight\'[1-9]\\d*)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["screenCharactersHeight"] = regexWorker["${screenCharsHeight}"]; dictionary["screenCharactersWidth"] = regexWorker["${screenCharsWidth}"]; this.UpscreencharsProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpscreencharsProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpscreenpixelsProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpscreenpixelsProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpscreenpixelsProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-SCREENPIXELS"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'screenPixWidth\'[1-9]\\d*),(?\'screenPixHeight\'[1-9]\\d*)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["screenPixelsHeight"] = regexWorker["${screenPixHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenPixWidth}"]; this.UpscreenpixelsProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpscreenpixelsProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpmsizeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpmsizeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpmsizeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-MSIZE"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'width\'\\d+),(?\'height\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["characterHeight"] = regexWorker["${height}"]; dictionary["characterWidth"] = regexWorker["${width}"]; this.UpmsizeProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpmsizeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void IscolorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void IscolorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool IscolorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-ISCOLOR"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, ".+"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities this.IscolorProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=IsColor if (this.IscolortrueProcess(headers, browserCaps)) { } else { if (this.IscolorfalseProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.IscolorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void IscolortrueProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void IscolortrueProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool IscolortrueProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-ISCOLOR"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "1"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["isColor"] = "true"; this.IscolortrueProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.IscolortrueProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void IscolorfalseProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void IscolorfalseProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool IscolorfalseProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-ISCOLOR"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "0"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["isColor"] = "false"; this.IscolorfalseProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.IscolorfalseProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpnumsoftkeysProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpnumsoftkeysProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpnumsoftkeysProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-NUMSOFTKEYS"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'softkeys\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["numberOfSoftkeys"] = regexWorker["${softkeys}"]; this.UpnumsoftkeysProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpnumsoftkeysProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpsoftkeysizeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpsoftkeysizeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpsoftkeysizeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-SOFTKEYSIZE"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'softkeySize\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["maximumSoftkeyLabelLength"] = regexWorker["${softkeySize}"]; this.UpsoftkeysizeProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpsoftkeysizeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpmaxpduProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpmaxpduProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpmaxpduProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["X-UP-DEVCAP-MAX-PDU"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'maxDeckSize\'\\d+)"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = regexWorker["${maxDeckSize}"]; this.UpmaxpduProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.UpmaxpduProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void UpversionProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void UpversionProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool UpversionProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches // Capabilities: set capabilities RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); dictionary["type"] = regexWorker["Phone.com ${majorVersion}.x Browser"]; this.UpversionProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=UpVersion if (this.BlazerProcess(headers, browserCaps)) { } else { if (this.Up4Process(headers, browserCaps)) { } else { if (this.Up5Process(headers, browserCaps)) { } else { if (this.Up6Process(headers, browserCaps)) { } else { if (this.Up3Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } this.UpversionProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void AumicProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void AumicProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool AumicProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "AU-MIC/(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\.\\d*).*"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "AU MIC"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "true"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "true"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "17"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsSelectFollowingTable"] = "false"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["AU MIC ${browserMajorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.AddBrowser("AuMic"); this.AumicProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=AuMic if (this.Aumicv2Process(headers, browserCaps)) { } else { if (this.A500Process(headers, browserCaps)) { } else { if (this.N400Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.AumicProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Aumicv2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Aumicv2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Aumicv2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "8600"; dictionary["screenCharactersHeight"] = "9"; dictionary["supportsBold"] = "false"; browserCaps.AddBrowser("AuMicV2"); this.Aumicv2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Aumicv2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void A500ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void A500ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool A500Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["HTTP_X_WAP_PROFILE"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "\\/SPH-A500\\/"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SPH-A500"; dictionary["maximumRenderedPageSize"] = "8850"; dictionary["preferredREnderingType"] = "xhtml-basic"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsTitleElement"] = "true"; browserCaps.AddBrowser("a500"); this.A500ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.A500ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void N400ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void N400ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool N400Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["HTTP_X_WAP_PROFILE"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "\\/SPH-N400\\/"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "N400"; dictionary["maximumRenderedPageSize"] = "46750"; dictionary["preferredREnderingType"] = "xhtml-basic"; dictionary["screenBitDepth"] = "24"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsTitleElement"] = "false"; browserCaps.AddBrowser("n400"); this.N400ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.N400ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void BlazerProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void BlazerProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool BlazerProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Blazer"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Handspring Blazer"; dictionary["canInitiateVoiceCall"] = "false"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "12"; dictionary["defaultScreenPixelsHeight"] = "72"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["maximumRenderedPageSize"] = "7000"; dictionary["mobileDeviceManufacturer"] = "PalmOS-licensee"; dictionary["numberOfSoftkeys"] = "0"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "html32"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["rendersWmlSelectsAsMenuCards"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "36"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsBold"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["type"] = "Handspring Blazer"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; this.BlazerProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Blazer if (this.Blazerupg1Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.BlazerProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Blazerupg1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Blazerupg1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Blazerupg1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "UPG1 UP/\\S* \\(compatible; Blazer (?\'browserMajorVersion\'\\d+)(?\'browserMinorVersio" + "n\'\\.\\d+)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; this.Blazerupg1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Blazerupg1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Up4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Up4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Up4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "4"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["requiresAbsolutePostbackUrl"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; this.Up4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Up4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Up5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Up5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Up5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["requiresUniqueFilePathSuffix"] = "true"; this.Up5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Up5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Up6ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Up6ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Up6Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "6"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "true"; dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["supportsStyleElement"] = "true"; dictionary["type"] = regexWorker["Openwave ${majorVersion}.x Browser"]; browserCaps.HtmlTextWriter = "System.Web.UI.XhtmlTextWriter"; this.Up6ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Up6 if (this.Up61plusProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Up6ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Up61plusProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Up61plusProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Up61plusProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["minorVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^\\.[^0]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["preferredRenderingMime"] = "application/xhtml+xml"; this.Up61plusProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Up61plusProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Up3ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Up3ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Up3Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "3"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderInputAndSelectElementsTogether"] = "false"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["requiresAbsolutePostbackUrl"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["requiresUrlEncodedPostfieldValues"] = "true"; dictionary["type"] = "Phone.com 3.x Browser"; this.Up3ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Up3ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Alcatelbe4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Alcatelbe4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Alcatelbe4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Alcatel-BE4"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Alcatel"; dictionary["mobileDeviceModel"] = "301"; browserCaps.AddBrowser("AlcatelBe4"); this.Alcatelbe4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Alcatelbe4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Alcatelbe5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Alcatelbe5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Alcatelbe5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Alcatel-BE5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Alcatel"; dictionary["mobileDeviceModel"] = "501, 701"; browserCaps.AddBrowser("AlcatelBe5"); this.Alcatelbe5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=AlcatelBe5 if (this.Alcatelbe5v2Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Alcatelbe5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Alcatelbe5v2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Alcatelbe5v2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Alcatelbe5v2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "2\\.0"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "1900"; dictionary["maximumSoftkeyLabelLength"] = "0"; dictionary["mobileDeviceModel"] = "Alcatel One Touch 501"; dictionary["numberOfSoftkeys"] = "10"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["requiresNoSoftkeyLabels"] = "true"; dictionary["screenBitDepth"] = "0"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "14"; dictionary["screenPixelsHeight"] = "60"; dictionary["screenPixelsWidth"] = "91"; dictionary["supportsBold"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("AlcatelBe5v2"); this.Alcatelbe5v2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Alcatelbe5v2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Alcatelbe3ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Alcatelbe3ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Alcatelbe3Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Alcatel-BE3"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Alcatel"; dictionary["mobileDeviceModel"] = "OneTouchDB"; browserCaps.AddBrowser("AlcatelBe3"); this.Alcatelbe3ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Alcatelbe3ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Alcatelbf3ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Alcatelbf3ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Alcatelbf3Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Alcatel-BF3"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "1900"; dictionary["maximumSoftkeyLabelLength"] = "13"; dictionary["mobileDeviceManufacturer"] = "Alcatel"; dictionary["mobileDeviceModel"] = "Alcatel One Touch 311"; dictionary["numberOfSoftkeys"] = "10"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "11"; dictionary["screenPixelsHeight"] = "65"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("AlcatelBf3"); this.Alcatelbf3ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Alcatelbf3ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Alcatelbf4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Alcatelbf4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Alcatelbf4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Alcatel-BF4"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "1900"; dictionary["maximumSoftkeyLabelLength"] = "13"; dictionary["mobileDeviceManufacturer"] = "Alcatel"; dictionary["mobileDeviceModel"] = "Alcatel One Touch 511"; dictionary["numberOfSoftkeys"] = "10"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "11"; dictionary["screenPixelsHeight"] = "60"; dictionary["screenPixelsWidth"] = "89"; browserCaps.AddBrowser("AlcatelBf4"); this.Alcatelbf4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Alcatelbf4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MotcbProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MotcbProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MotcbProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-CB"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Timeport P7389"; dictionary["numberOfSoftkeys"] = "1"; browserCaps.AddBrowser("MotCb"); this.MotcbProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MotcbProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motf5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motf5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motf5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-F5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "false"; dictionary["maximumRenderedPageSize"] = "1900"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Talkabout 192"; dictionary["numberOfSoftkeys"] = "3"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "40"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("MotF5"); this.Motf5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motf5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motd8ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motd8ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motd8Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-D8"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Timeport 250/P7689"; browserCaps.AddBrowser("MotD8"); this.Motd8ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motd8ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MotcfProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MotcfProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MotcfProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-CF"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Accompli 6188"; browserCaps.AddBrowser("MotCf"); this.MotcfProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MotcfProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motf6ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motf6ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motf6Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-F6"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["maximumRenderedPageSize"] = "5000"; dictionary["maximumSoftkeyLabelLength"] = "6"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Accompli 008/6288"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlSelectsAsMenuCards"] = "false"; dictionary["screenBitDepth"] = "4"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "240"; browserCaps.AddBrowser("MotF6"); this.Motf6ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motf6ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MotbcProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MotbcProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MotbcProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-BC"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["inputType"] = "keyboard"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Accompli 009"; browserCaps.AddBrowser("MotBc"); this.MotbcProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MotbcProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MotdcProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MotdcProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MotdcProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-DC"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "V3682, V50"; browserCaps.AddBrowser("MotDc"); this.MotdcProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MotdcProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MotpancProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MotpancProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MotpancProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-PAN-C"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Timeport 270c"; browserCaps.AddBrowser("MotPanC"); this.MotpancProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MotpancProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motc4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motc4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motc4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-C4"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderMixedSelects"] = "false"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "V2288, V2282"; dictionary["supportsCacheControlMetaTag"] = "false"; browserCaps.AddBrowser("MotC4"); this.Motc4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motc4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MccaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MccaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MccaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MCCA"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Timeport 8767/ST7868"; browserCaps.AddBrowser("Mcca"); this.MccaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.MccaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mot2000ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mot2000ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mot2000Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-2000"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "V60c"; browserCaps.AddBrowser("Mot2000"); this.Mot2000ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mot2000ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motp2kcProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motp2kcProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motp2kcProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-P2K-C"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "V120c"; browserCaps.AddBrowser("MotP2kC"); this.Motp2kcProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motp2kcProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MotafProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MotafProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MotafProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-AF"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Timeport 260/P7382i/P7389i"; dictionary["screenCharactersHeight"] = "4"; browserCaps.AddBrowser("MotAf"); this.MotafProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=MotAf if (this.Motaf418Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.MotafProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motaf418ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motaf418ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motaf418Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["DeviceVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "4\\.1\\.8"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["maximumRenderedPageSize"] = "1900"; dictionary["maximumSoftkeyLabelLength"] = "5"; dictionary["mobileDeviceModel"] = "Timeport 260"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "64"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsCacheControlMetaTag"] = "false"; dictionary["tables"] = "true"; browserCaps.AddBrowser("MotAf418"); this.Motaf418ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motaf418ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motc2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motc2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motc2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-C2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["inputType"] = "keyboard"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "V100, V.Box"; browserCaps.AddBrowser("MotC2"); this.Motc2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motc2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void XeniumProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void XeniumProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool XeniumProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Philips-Xenium9@9"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Philips"; dictionary["mobileDeviceModel"] = "Xenium 9"; browserCaps.AddBrowser("Xenium"); this.XeniumProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.XeniumProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sagem959ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sagem959ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sagem959Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Sagem-959"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sagem"; dictionary["mobileDeviceModel"] = "MW-959"; browserCaps.AddBrowser("Sagem959"); this.Sagem959ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sagem959ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sgha300ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sgha300ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sgha300Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SAMSUNG-SGH-A300"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2000"; dictionary["maximumSoftkeyLabelLength"] = "19"; dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SGH-A300"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "13"; dictionary["screenPixelsHeight"] = "128"; dictionary["screenPixelsWidth"] = "128"; browserCaps.AddBrowser("SghA300"); this.Sgha300ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sgha300ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sghn100ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sghn100ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sghn100Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Samsung-SGH-N100/"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SGH-N100"; browserCaps.AddBrowser("SghN100"); this.Sghn100ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sghn100ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C304saProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C304saProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C304saProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Sanyo-C304SA/"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "C304SA"; browserCaps.AddBrowser("C304sa"); this.C304saProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C304saProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sy11ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sy11ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sy11Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SY11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "C304SA"; browserCaps.AddBrowser("Sy11"); this.Sy11ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sy11ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void St12ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void St12ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool St12Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "ST12"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "C411ST"; browserCaps.AddBrowser("St12"); this.St12ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.St12ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sy14ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sy14ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sy14Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SY14"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "C412SA"; browserCaps.AddBrowser("Sy14"); this.Sy14ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sy14ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sies40ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sies40ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sies40Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SIE-S40"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["maximumRenderedPageSize"] = "2048"; dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "S40, S42"; browserCaps.AddBrowser("SieS40"); this.Sies40ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sies40ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Siesl45ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Siesl45ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Siesl45Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SIE-SL45"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "SL-45"; browserCaps.AddBrowser("SieSl45"); this.Siesl45ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Siesl45ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sies35ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sies35ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sies35Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SIE-S35"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderMixedSelects"] = "false"; dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "S35"; browserCaps.AddBrowser("SieS35"); this.Sies35ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sies35ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sieme45ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sieme45ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sieme45Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SIE-ME45"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2800"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "ME45"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "65"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; browserCaps.AddBrowser("SieMe45"); this.Sieme45ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sieme45ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sies45ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sies45ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sies45Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SIE-S45"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2765"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "S45"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "64"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; browserCaps.AddBrowser("SieS45"); this.Sies45ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sies45ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Gm832ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Gm832ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Gm832Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "GM832"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Telit"; dictionary["mobileDeviceModel"] = "GM832"; browserCaps.AddBrowser("Gm832"); this.Gm832ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Gm832ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Gm910iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Gm910iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Gm910iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Telit-GM910i"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Telit"; dictionary["mobileDeviceModel"] = "GM910i"; browserCaps.AddBrowser("Gm910i"); this.Gm910iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Gm910iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mot32ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mot32ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mot32Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-32"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "i85s, i50sx"; browserCaps.AddBrowser("Mot32"); this.Mot32ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mot32ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mot28ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mot28ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mot28Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-28"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "i700+, i1000+"; browserCaps.AddBrowser("Mot28"); this.Mot28ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mot28ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void D2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void D2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool D2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "D2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["mobileDeviceModel"] = "D2"; browserCaps.AddBrowser("D2"); this.D2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.D2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PpatProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PpatProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PpatProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "P-PAT"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["mobileDeviceModel"] = "P-PAT"; browserCaps.AddBrowser("PPat"); this.PpatProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PpatProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void AlazProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void AlazProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool AlazProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "ALAZ"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Alcatel"; dictionary["mobileDeviceModel"] = "OneTouch"; browserCaps.AddBrowser("Alaz"); this.AlazProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.AlazProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Cdm9100ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Cdm9100ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Cdm9100Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "AUDIOVOX-CDM9100"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Audiovox"; dictionary["mobileDeviceModel"] = "CDM-9100"; browserCaps.AddBrowser("Cdm9100"); this.Cdm9100ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Cdm9100ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Cdm135ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Cdm135ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Cdm135Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HD-MMD1010"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Audiovox"; dictionary["mobileDeviceModel"] = "CDM-135"; browserCaps.AddBrowser("Cdm135"); this.Cdm135ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Cdm135ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Cdm9000ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Cdm9000ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Cdm9000Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TSCA"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Audiovox"; dictionary["mobileDeviceModel"] = "CDM-9000"; browserCaps.AddBrowser("Cdm9000"); this.Cdm9000ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Cdm9000ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C303caProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C303caProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C303caProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "CA11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Casio"; dictionary["mobileDeviceModel"] = "C303CA"; browserCaps.AddBrowser("C303ca"); this.C303caProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C303caProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C311caProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C311caProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C311caProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "CA12"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Casio"; dictionary["mobileDeviceModel"] = "C311CA"; browserCaps.AddBrowser("C311ca"); this.C311caProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C311caProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C202deProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C202deProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C202deProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "DN01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Casio"; dictionary["mobileDeviceModel"] = "C202DE"; browserCaps.AddBrowser("C202de"); this.C202deProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C202deProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C409caProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C409caProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C409caProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "CA13"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Casio"; dictionary["mobileDeviceModel"] = "C409CA"; browserCaps.AddBrowser("C409ca"); this.C409caProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C409caProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C402deProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C402deProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C402deProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "DN11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Denso"; dictionary["mobileDeviceModel"] = "C402DE"; browserCaps.AddBrowser("C402de"); this.C402deProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C402deProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ds15ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ds15ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ds15Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "DS15"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Denso"; dictionary["mobileDeviceModel"] = "Touchpoint DS15"; browserCaps.AddBrowser("Ds15"); this.Ds15ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ds15ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tp2200ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tp2200ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tp2200Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "DS1[34]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Denso"; dictionary["mobileDeviceModel"] = "TouchPoint TP2200"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "15"; browserCaps.AddBrowser("Tp2200"); this.Tp2200ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tp2200ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tp120ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tp120ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tp120Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "DS12"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Denso"; dictionary["mobileDeviceModel"] = "TouchPoint TP120"; browserCaps.AddBrowser("Tp120"); this.Tp120ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tp120ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ds10ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ds10ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ds10Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "DS10"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Denso"; dictionary["mobileDeviceModel"] = "Eagle 10"; browserCaps.AddBrowser("Ds10"); this.Ds10ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ds10ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void R280ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void R280ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool R280Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "ERK0"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Ericsson"; dictionary["mobileDeviceModel"] = "R280"; browserCaps.AddBrowser("R280"); this.R280ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.R280ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C201hProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C201hProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C201hProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HI01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hitachi"; dictionary["mobileDeviceModel"] = "C201H"; browserCaps.AddBrowser("C201h"); this.C201hProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C201hProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void S71ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void S71ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool S71Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HW01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hanwha"; dictionary["mobileDeviceModel"] = "S71"; browserCaps.AddBrowser("S71"); this.S71ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.S71ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C302hProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C302hProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C302hProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HI11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hitachi"; dictionary["mobileDeviceModel"] = "C302H"; browserCaps.AddBrowser("C302h"); this.C302hProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C302hProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C309hProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C309hProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C309hProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HI12"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hitachi"; dictionary["mobileDeviceModel"] = "C309H"; browserCaps.AddBrowser("C309h"); this.C309hProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C309hProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C407hProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C407hProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C407hProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HI13"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["mobileDeviceManufacturer"] = "Hitachi"; dictionary["mobileDeviceModel"] = "C407H"; browserCaps.AddBrowser("C407h"); this.C407hProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C407hProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void C451hProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void C451hProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool C451hProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HI14"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hitachi"; dictionary["mobileDeviceModel"] = "C451H"; browserCaps.AddBrowser("C451h"); this.C451hProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.C451hProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void R201ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void R201ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool R201Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HD03"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hyundai"; dictionary["mobileDeviceModel"] = "HGC-R201"; browserCaps.AddBrowser("R201"); this.R201ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.R201ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void P21ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void P21ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool P21Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HD02"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hyundai"; dictionary["mobileDeviceModel"] = "P-21"; browserCaps.AddBrowser("P21"); this.P21ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.P21ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Kyocera702gProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Kyocera702gProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Kyocera702gProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCI1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "702G"; browserCaps.AddBrowser("Kyocera702g"); this.Kyocera702gProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Kyocera702gProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Kyocera703gProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Kyocera703gProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Kyocera703gProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCI2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "703G"; browserCaps.AddBrowser("Kyocera703g"); this.Kyocera703gProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Kyocera703gProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Kyocerac307kProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Kyocerac307kProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Kyocerac307kProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KC11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "C307K"; browserCaps.AddBrowser("KyoceraC307k"); this.Kyocerac307kProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Kyocerac307kProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tk01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tk01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tk01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCT1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "TK01"; browserCaps.AddBrowser("Tk01"); this.Tk01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tk01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tk02ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tk02ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tk02Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCT2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "TK02"; browserCaps.AddBrowser("Tk02"); this.Tk02ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tk02ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tk03ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tk03ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tk03Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCT4"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "TK03"; browserCaps.AddBrowser("Tk03"); this.Tk03ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tk03ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tk04ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tk04ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tk04Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCT5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "TK04"; browserCaps.AddBrowser("Tk04"); this.Tk04ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tk04ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tk05ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tk05ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tk05Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCT6"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "TK05"; browserCaps.AddBrowser("Tk05"); this.Tk05ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tk05ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void D303kProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void D303kProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool D303kProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCC1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "D303K"; browserCaps.AddBrowser("D303k"); this.D303kProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.D303kProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void D304kProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void D304kProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool D304kProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KCC2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "D304K"; browserCaps.AddBrowser("D304k"); this.D304kProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.D304kProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Qcp2035ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Qcp2035ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Qcp2035Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "QC06"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "QCP2035/2037"; browserCaps.AddBrowser("Qcp2035"); this.Qcp2035ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Qcp2035ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Qcp3035ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Qcp3035ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Qcp3035Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "QC07"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "QCP3035"; browserCaps.AddBrowser("Qcp3035"); this.Qcp3035ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Qcp3035ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void D512ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void D512ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool D512Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG22"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "D-512"; browserCaps.AddBrowser("D512"); this.D512ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.D512ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Dm110ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Dm110ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Dm110Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG05"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "DM-110"; browserCaps.AddBrowser("Dm110"); this.Dm110ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Dm110ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tm510ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tm510ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tm510Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG21"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderPostBackCards"] = "false"; dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "TM-510"; browserCaps.AddBrowser("Tm510"); this.Tm510ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tm510ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lg13ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lg13ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lg13Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG13"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "DM-510"; browserCaps.AddBrowser("Lg13"); this.Lg13ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lg13ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void P100ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void P100ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool P100Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "P-100"; browserCaps.AddBrowser("P100"); this.P100ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.P100ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lgc875fProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lgc875fProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lgc875fProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG07"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "LGC-875F"; browserCaps.AddBrowser("Lgc875f"); this.Lgc875fProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lgc875fProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lgp680fProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lgp680fProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lgp680fProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG03"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "LGP-6800F"; browserCaps.AddBrowser("Lgp680f"); this.Lgp680fProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lgp680fProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lgp7800fProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lgp7800fProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lgp7800fProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG04"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "LGP-7800F"; browserCaps.AddBrowser("Lgp7800f"); this.Lgp7800fProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lgp7800fProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lgc840fProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lgc840fProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lgc840fProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG09"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "LGC-840F"; browserCaps.AddBrowser("Lgc840f"); this.Lgc840fProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lgc840fProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lgi2100ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lgi2100ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lgi2100Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG02"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "LGI-2100"; browserCaps.AddBrowser("Lgi2100"); this.Lgi2100ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lgi2100ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lgp7300fProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lgp7300fProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lgp7300fProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "LGP-7300F"; browserCaps.AddBrowser("Lgp7300f"); this.Lgp7300fProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lgp7300fProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sd500ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sd500ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sd500Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG10"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "SD-500"; browserCaps.AddBrowser("Sd500"); this.Sd500ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sd500ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tp1100ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tp1100ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tp1100Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG06"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "Touchpoint TP1100"; browserCaps.AddBrowser("Tp1100"); this.Tp1100ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tp1100ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tp3000ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tp3000ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tp3000Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG08"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderAfterInputOrSelectElement"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "Touchpoint TP3000"; browserCaps.AddBrowser("Tp3000"); this.Tp3000ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tp3000ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void T250ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void T250ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool T250Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "T250"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["mobileDeviceModel"] = "T250"; browserCaps.AddBrowser("T250"); this.T250ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.T250ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mo01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mo01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mo01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MO01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "i500+, i700+, i1000+"; browserCaps.AddBrowser("Mo01"); this.Mo01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mo01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mo02ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mo02ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mo02Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MO02"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "i2000+"; browserCaps.AddBrowser("Mo02"); this.Mo02ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mo02ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mc01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mc01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mc01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MC01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "StarTac ST786x, Talkabout T816x, Timeport P816x"; browserCaps.AddBrowser("Mc01"); this.Mc01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mc01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void McccProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void McccProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool McccProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MCCC"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Talkabout V2267"; browserCaps.AddBrowser("Mccc"); this.McccProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.McccProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mcc9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mcc9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mcc9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MCC9"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Talkabout V8162"; browserCaps.AddBrowser("Mcc9"); this.Mcc9ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mcc9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nk00ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nk00ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nk00Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "NK00"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2252"; dictionary["maximumSoftkeyLabelLength"] = "6"; dictionary["mobileDeviceManufacturer"] = "Nokia"; dictionary["mobileDeviceModel"] = "nokia 3285"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["screenCharactersWidth"] = "15"; dictionary["supportsBold"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Nk00"); this.Nk00ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nk00ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mai12ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mai12ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mai12Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MAI[12]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["mobileDeviceModel"] = "704G"; browserCaps.AddBrowser("Mai12"); this.Mai12ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mai12ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ma112ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ma112ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ma112Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MA1[12]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["mobileDeviceModel"] = "C308P"; browserCaps.AddBrowser("Ma112"); this.Ma112ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ma112ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ma13ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ma13ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ma13Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MA13"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["mobileDeviceModel"] = "C408P"; browserCaps.AddBrowser("Ma13"); this.Ma13ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ma13ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mac1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mac1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mac1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MAC1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["mobileDeviceModel"] = "D305P"; browserCaps.AddBrowser("Mac1"); this.Mac1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mac1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mat1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mat1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mat1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MAT1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["mobileDeviceModel"] = "TP01"; browserCaps.AddBrowser("Mat1"); this.Mat1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mat1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sc01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sc01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sc01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SC01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SCH-3500"; browserCaps.AddBrowser("Sc01"); this.Sc01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sc01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sc03ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sc03ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sc03Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SC03"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SCH-6100"; browserCaps.AddBrowser("Sc03"); this.Sc03ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sc03ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sc02ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sc02ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sc02Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SC02"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SCH-8500"; browserCaps.AddBrowser("Sc02"); this.Sc02ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sc02ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sc04ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sc04ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sc04Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SC04"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SCH-850"; browserCaps.AddBrowser("Sc04"); this.Sc04ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sc04ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sg08ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sg08ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sg08Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SG08"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SGH-800"; browserCaps.AddBrowser("Sg08"); this.Sg08ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sg08ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sc13ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sc13ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sc13Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SC13"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "Uproar M100"; browserCaps.AddBrowser("Sc13"); this.Sc13ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sc13ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sc11ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sc11ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sc11Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SC11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SCH-N105"; browserCaps.AddBrowser("Sc11"); this.Sc11ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sc11ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sec01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sec01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sec01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SEC01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SCH-U03"; browserCaps.AddBrowser("Sec01"); this.Sec01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sec01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sc10ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sc10ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sc10Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SC10"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "SCH-U02"; browserCaps.AddBrowser("Sc10"); this.Sc10ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sc10ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sy12ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sy12ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sy12Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SY12"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "C401SA"; browserCaps.AddBrowser("Sy12"); this.Sy12ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sy12ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void St11ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void St11ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool St11Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "ST11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "C403ST"; browserCaps.AddBrowser("St11"); this.St11ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.St11ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sy13ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sy13ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sy13Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SY13"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "C405SA"; browserCaps.AddBrowser("Sy13"); this.Sy13ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sy13ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Syc1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Syc1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Syc1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SYC1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "D301SA"; browserCaps.AddBrowser("Syc1"); this.Syc1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Syc1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sy01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sy01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sy01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SY01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "SCP-4000"; browserCaps.AddBrowser("Sy01"); this.Sy01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sy01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Syt1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Syt1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Syt1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SYT1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "TS01"; browserCaps.AddBrowser("Syt1"); this.Syt1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Syt1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sty2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sty2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sty2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SYT2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "TS02"; browserCaps.AddBrowser("Sty2"); this.Sty2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sty2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sy02ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sy02ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sy02Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SY02"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "SCP-4500"; browserCaps.AddBrowser("Sy02"); this.Sy02ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sy02ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sy03ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sy03ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sy03Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SY03"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "SCP-5000"; browserCaps.AddBrowser("Sy03"); this.Sy03ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sy03ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Si01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Si01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Si01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SI01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "S25"; browserCaps.AddBrowser("Si01"); this.Si01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Si01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sni1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sni1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sni1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SNI1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "705G"; browserCaps.AddBrowser("Sni1"); this.Sni1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sni1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sn11ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sn11ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sn11Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SN11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "C305SN"; browserCaps.AddBrowser("Sn11"); this.Sn11ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sn11ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sn12ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sn12ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sn12Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SN12"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "C404S"; browserCaps.AddBrowser("Sn12"); this.Sn12ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sn12ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sn134ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sn134ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sn134Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SN1[34]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "C406S"; browserCaps.AddBrowser("Sn134"); this.Sn134ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sn134ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sn156ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sn156ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sn156Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SN1[56]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "C413S"; browserCaps.AddBrowser("Sn156"); this.Sn156ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sn156ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Snc1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Snc1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Snc1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SNC1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "D306S"; browserCaps.AddBrowser("Snc1"); this.Snc1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Snc1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tsc1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tsc1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tsc1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TSC1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "D302T"; browserCaps.AddBrowser("Tsc1"); this.Tsc1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tsc1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tsi1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tsi1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tsi1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TSI1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "701G"; browserCaps.AddBrowser("Tsi1"); this.Tsi1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tsi1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ts11ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ts11ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ts11Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TS11"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "C301T"; browserCaps.AddBrowser("Ts11"); this.Ts11ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ts11ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ts12ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ts12ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ts12Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TS12"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "C310T"; browserCaps.AddBrowser("Ts12"); this.Ts12ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ts12ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ts13ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ts13ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ts13Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TS13"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "C410T"; browserCaps.AddBrowser("Ts13"); this.Ts13ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ts13ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tst1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tst1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tst1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TST1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "TT01"; browserCaps.AddBrowser("Tst1"); this.Tst1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tst1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tst2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tst2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tst2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TST2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "TT02"; browserCaps.AddBrowser("Tst2"); this.Tst2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tst2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tst3ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tst3ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tst3Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "TST3"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "TT03"; browserCaps.AddBrowser("Tst3"); this.Tst3ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tst3ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ig01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ig01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ig01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "IG01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "NeoPoint"; dictionary["mobileDeviceModel"] = "NP1000"; browserCaps.AddBrowser("Ig01"); this.Ig01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ig01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ig02ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ig02ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ig02Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "IG02"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "NeoPoint"; dictionary["mobileDeviceModel"] = "NP1660"; browserCaps.AddBrowser("Ig02"); this.Ig02ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ig02ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Ig03ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Ig03ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Ig03Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "IG03"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "NeoPoint"; dictionary["mobileDeviceModel"] = "NP2000"; browserCaps.AddBrowser("Ig03"); this.Ig03ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Ig03ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Qc31ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Qc31ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Qc31Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "QC31"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Qualcomm"; dictionary["mobileDeviceModel"] = "QCP-860, QCP-1960"; browserCaps.AddBrowser("Qc31"); this.Qc31ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Qc31ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Qc12ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Qc12ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Qc12Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "QC12"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Qualcomm"; dictionary["mobileDeviceModel"] = "QCP-1900, QCP-2700"; browserCaps.AddBrowser("Qc12"); this.Qc12ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Qc12ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Qc32ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Qc32ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Qc32Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "QC32"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Qualcomm"; dictionary["mobileDeviceModel"] = "QCP-2760"; browserCaps.AddBrowser("Qc32"); this.Qc32ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Qc32ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sp01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sp01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sp01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SP01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Mitsubishi"; dictionary["mobileDeviceModel"] = "MA120"; browserCaps.AddBrowser("Sp01"); this.Sp01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sp01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void ShProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void ShProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool ShProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SH"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "Duette"; browserCaps.AddBrowser("Sh"); this.ShProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.ShProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Upg1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Upg1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Upg1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "UPG1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "false"; dictionary["mobileDeviceManufacturer"] = "OpenWave"; dictionary["mobileDeviceModel"] = "Generic Simulator"; browserCaps.AddBrowser("Upg1"); this.Upg1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Upg1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opwv1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opwv1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opwv1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "OPWV1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "false"; dictionary["inputType"] = "keyboard"; dictionary["maximumRenderedPageSize"] = "3584"; dictionary["maximumSoftkeyLabelLength"] = "9"; dictionary["mobileDeviceManufacturer"] = "Openwave"; dictionary["mobileDeviceModel"] = "Openwave 5.0 emulator"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "19"; dictionary["screenPixelsHeight"] = "188"; dictionary["screenPixelsWidth"] = "144"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; browserCaps.AddBrowser("Opwv1"); this.Opwv1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Opwv1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void AlavProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void AlavProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool AlavProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "ALAV"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Alcatel"; dictionary["mobileDeviceModel"] = "OneTouch"; browserCaps.AddBrowser("Alav"); this.AlavProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.AlavProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Im1kProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Im1kProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Im1kProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "IM1K"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "iDEN"; browserCaps.AddBrowser("Im1k"); this.Im1kProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Im1kProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Nt95ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Nt95ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Nt95Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "NT95"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "cdmaOne"; browserCaps.AddBrowser("Nt95"); this.Nt95ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Nt95ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mot2001ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mot2001ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mot2001Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-2001"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "1946"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Timeport 270c"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["requiresUrlEncodedPostfieldValues"] = "true"; dictionary["screenCharactersWidth"] = "19"; browserCaps.AddBrowser("Mot2001"); this.Mot2001ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mot2001ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motv200ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motv200ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motv200Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-v200"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hasBackButton"] = "false"; dictionary["inputType"] = "keyboard"; dictionary["maximumRenderedPageSize"] = "2000"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Motorola v200"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["requiresUrlEncodedPostfieldValues"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Motv200"); this.Motv200ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motv200ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mot72ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mot72ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mot72Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-72"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hasBackButton"] = "false"; dictionary["maximumRenderedPageSize"] = "2900"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Motorola i80s"; dictionary["numberOfSoftkeys"] = "4"; dictionary["rendersBreaksAfterWmlAnchor"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["requiresUrlEncodedPostfieldValues"] = "true"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "13"; browserCaps.AddBrowser("Mot72"); this.Mot72ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mot72ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mot76ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mot76ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mot76Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-76"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2969"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Motorola i90c"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["screenCharactersWidth"] = "14"; browserCaps.AddBrowser("Mot76"); this.Mot76ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mot76ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Scp6000ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Scp6000ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Scp6000Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Sanyo-SCP6000"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderInputAndSelectElementsTogether"] = "false"; dictionary["hasBackButton"] = "false"; dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "Sanyo SCP-6000"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["screenBitDepth"] = "1"; dictionary["screenPixelsHeight"] = "120"; dictionary["screenPixelsWidth"] = "128"; dictionary["supportsBold"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Scp6000"); this.Scp6000ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Scp6000ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motd5ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motd5ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motd5Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-D5"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2000"; dictionary["maximumSoftkeyLabelLength"] = "6"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Motorola Talkabout 191/192/193"; dictionary["numberOfSoftkeys"] = "3"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "13"; dictionary["screenPixelsHeight"] = "51"; dictionary["screenPixelsWidth"] = "91"; browserCaps.AddBrowser("MotD5"); this.Motd5ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motd5ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motf0ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motf0ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motf0Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-F0"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2000"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "Motorola v50"; dictionary["numberOfSoftkeys"] = "3"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["requiresUrlEncodedPostfieldValues"] = "true"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "40"; dictionary["screenPixelsWidth"] = "96"; browserCaps.AddBrowser("MotF0"); this.Motf0ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motf0ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sgha400ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sgha400ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sgha400Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SAMSUNG-SGH-A400"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2000"; dictionary["maximumSoftkeyLabelLength"] = "6"; dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "Samsung SGH-A400"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["requiresNoSoftkeyLabels"] = "true"; dictionary["screenCharactersHeight"] = "3"; dictionary["screenCharactersWidth"] = "13"; dictionary["screenPixelsHeight"] = "96"; dictionary["screenPixelsWidth"] = "128"; browserCaps.AddBrowser("SghA400"); this.Sgha400ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sgha400ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sec03ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sec03ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sec03Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SEC03"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "false"; dictionary["maximumRenderedPageSize"] = "3000"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "Samsung SPH-i300"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "38"; dictionary["screenPixelsHeight"] = "240"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsBold"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Sec03"); this.Sec03ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sec03ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Siec3iProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Siec3iProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Siec3iProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SIE-C3I"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderMixedSelects"] = "false"; dictionary["maximumRenderedPageSize"] = "1900"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "C35/M35"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "false"; dictionary["requiresSpecialViewStateEncoding"] = "false"; dictionary["requiresUrlEncodedPostfieldValues"] = "false"; dictionary["screenCharactersHeight"] = "3"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "54"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsBold"] = "true"; browserCaps.AddBrowser("SieC3i"); this.Siec3iProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Siec3iProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sn17ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sn17ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sn17Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SN17"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["maximumRenderedPageSize"] = "12000"; dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "C1002S"; dictionary["numberOfSoftkeys"] = "3"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "120"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Sn17"); this.Sn17ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sn17ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Scp4700ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Scp4700ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Scp4700Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Sanyo-SCP4700"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "3072"; dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "Sanyo SCP 4700"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "15"; dictionary["screenPixelsHeight"] = "32"; dictionary["screenPixelsWidth"] = "91"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Scp4700"); this.Scp4700ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Scp4700ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sec02ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sec02ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sec02Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SEC02"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2867"; dictionary["mobileDeviceManufacturer"] = "Samsung"; dictionary["mobileDeviceModel"] = "Samsung SPH-N200"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["rendersBreaksAfterWmlAnchor"] = "true"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "15"; dictionary["screenPixelsHeight"] = "96"; dictionary["screenPixelsWidth"] = "128"; dictionary["supportsItalic"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Sec02"); this.Sec02ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sec02ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sy15ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sy15ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sy15Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SY15"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["maximumRenderedPageSize"] = "7500"; dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "Sanyo C1001SA"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "8"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Sy15"); this.Sy15ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sy15ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Db520ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Db520ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Db520Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LGE-DB520"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "3072"; dictionary["mobileDeviceManufacturer"] = "Sprint"; dictionary["mobileDeviceModel"] = "TP5200"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/wnd.wap.wml"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("Db520"); this.Db520ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Db520ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void L430v03j02ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void L430v03j02ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool L430v03j02Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LGE-L430V03J02"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2900"; dictionary["maximumSoftkeyLabelLength"] = "10"; dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "LG-LP9000"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["requiresNoSoftkeyLabels"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "19"; dictionary["screenPixelsHeight"] = "133"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsBold"] = "true"; browserCaps.AddBrowser("L430V03J02"); this.L430v03j02ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.L430v03j02ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void OpwvsdkProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void OpwvsdkProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool OpwvsdkProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^OPWV-SDK"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "false"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceManufacturer"] = "Openwave"; dictionary["mobileDeviceModel"] = "Mobile Browser"; dictionary["preferredImageMime"] = "image/gif"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "9"; dictionary["screenCharactersWidth"] = "19"; dictionary["screenPixelsHeight"] = "188"; dictionary["screenPixelsWidth"] = "144"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("OPWVSDK"); this.OpwvsdkProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=OPWVSDK if (this.Opwvsdk6Process(headers, browserCaps)) { } else { if (this.Opwvsdk6plusProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.OpwvsdkProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opwvsdk6ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opwvsdk6ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opwvsdk6Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^OPWV-SDK/61"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "UP.Browser"; dictionary["canSendMail"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["mobileDeviceModel"] = "WAP Simulator 6.1"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "15"; dictionary["screenPixelsHeight"] = "108"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; browserCaps.AddBrowser("OPWVSDK6"); this.Opwvsdk6ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Opwvsdk6ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opwvsdk6plusProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opwvsdk6plusProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opwvsdk6plusProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^OPWV-SDK/(?\'modelVersion\'[7-9]|6[^01])"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Openwave"; dictionary["canSendMail"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["maximumRenderedPageSize"] = "66000"; dictionary["mobileDeviceModel"] = regexWorker["Mobile Browser ${version}"]; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresPragmaNoCacheHeader"] = "true"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsNoWrapStyle"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; browserCaps.AddBrowser("OPWVSDK6Plus"); this.Opwvsdk6plusProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Opwvsdk6plusProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Kddica21ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Kddica21ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Kddica21Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^KDDI-CA21$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "9000"; dictionary["mobileDeviceManufacturer"] = "Casio"; dictionary["mobileDeviceModel"] = "A3012CA"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "147"; dictionary["screenPixelsWidth"] = "125"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsCss"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("KDDICA21"); this.Kddica21ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Kddica21ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Kddits21ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Kddits21ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Kddits21Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KDDI-TS21"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "9000"; dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "C5001T"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "135"; dictionary["screenPixelsWidth"] = "144"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsCss"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("KDDITS21"); this.Kddits21ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Kddits21ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Kddisa21ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Kddisa21ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Kddisa21Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KDDI-SA21"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "9000"; dictionary["mobileDeviceManufacturer"] = "Sanyo"; dictionary["mobileDeviceModel"] = "A3011SA"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "176"; dictionary["screenPixelsWidth"] = "132"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsItalic"] = "false"; dictionary["tables"] = "true"; browserCaps.AddBrowser("KDDISA21"); this.Kddisa21ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Kddisa21ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Km100ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Km100ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Km100Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KM100"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cookies"] = "false"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "2900"; dictionary["maximumSoftkeyLabelLength"] = "12"; dictionary["mobileDeviceManufacturer"] = "OKWap"; dictionary["mobileDeviceModel"] = "i108"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["rendersWmlSelectsAsMenuCards"] = "false"; dictionary["requiresNoSoftkeyLabels"] = "true"; dictionary["requiresPhoneNumbersAsPlainText"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("KM100"); this.Km100ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Km100ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Lgelx5350ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Lgelx5350ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Lgelx5350Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LGE-LX5350"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceManufacturer"] = "LG"; dictionary["mobileDeviceModel"] = "LX5350"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "5"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "108"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("LGELX5350"); this.Lgelx5350ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Lgelx5350ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Hitachip300ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Hitachip300ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Hitachip300Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Hitachi-P300"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceManufacturer"] = "Hitachi"; dictionary["mobileDeviceModel"] = "SH-P300"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "17"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsFontSize"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("HitachiP300"); this.Hitachip300ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Hitachip300ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sies46ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sies46ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sies46Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SIE-S46"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["hasBackButton"] = "false"; dictionary["maximumRenderedPageSize"] = "2700"; dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "S46"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "19"; dictionary["screenPixelsHeight"] = "72"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsBold"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("SIES46"); this.Sies46ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sies46ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motorolav60gProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motorolav60gProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motorolav60gProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-PHX4_"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "2000"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "V60G"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["requiresUrlEncodedPostfieldValues"] = "true"; dictionary["screenCharactersHeight"] = "3"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "36"; dictionary["screenPixelsWidth"] = "90"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("MotorolaV60G"); this.Motorolav60gProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motorolav60gProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motorolav708ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motorolav708ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motorolav708Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-V708_"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "false"; dictionary["maximumRenderedPageSize"] = "1900"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "V70"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "2"; dictionary["screenCharactersWidth"] = "15"; dictionary["screenPixelsHeight"] = "34"; dictionary["screenPixelsWidth"] = "90"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("MotorolaV708"); this.Motorolav708ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motorolav708ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motorolav708aProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motorolav708aProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motorolav708aProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MOT-V708A"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canRenderInputAndSelectElementsTogether"] = "false"; dictionary["hasBackButton"] = "false"; dictionary["maximumRenderedPageSize"] = "2000"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "V70"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["screenCharactersHeight"] = "3"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "94"; dictionary["screenPixelsWidth"] = "96"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("MotorolaV708A"); this.Motorolav708aProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motorolav708aProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Motorolae360ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Motorolae360ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Motorolae360Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Motorola-T33"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canSendMail"] = "true"; dictionary["hasBackButton"] = "false"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "3500"; dictionary["maximumSoftkeyLabelLength"] = "8"; dictionary["mobileDeviceManufacturer"] = "Motorola"; dictionary["mobileDeviceModel"] = "E360"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlDoAcceptsInline"] = "true"; dictionary["screenBitDepth"] = "12"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "27"; dictionary["screenPixelsHeight"] = "96"; dictionary["screenPixelsWidth"] = "128"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("MotorolaE360"); this.Motorolae360ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Motorolae360ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sonyericssona1101sProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sonyericssona1101sProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sonyericssona1101sProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KDDI-SN22"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "9000"; dictionary["mobileDeviceManufacturer"] = "SonyEricsson"; dictionary["mobileDeviceModel"] = "A1101S"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "9"; dictionary["screenPixelsHeight"] = "123"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("SonyericssonA1101S"); this.Sonyericssona1101sProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sonyericssona1101sProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Philipsfisio820ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Philipsfisio820ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Philipsfisio820Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "PHILIPS-FISIO 820"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "3000"; dictionary["maximumSoftkeyLabelLength"] = "6"; dictionary["mobileDeviceManufacturer"] = "Philips"; dictionary["mobileDeviceModel"] = "Fisio 820"; dictionary["numberOfSoftkeys"] = "3"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["requiresNoSoftkeyLabels"] = "true"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "20"; dictionary["screenPixelsHeight"] = "112"; dictionary["screenPixelsWidth"] = "112"; dictionary["supportsBold"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; browserCaps.AddBrowser("PhilipsFisio820"); this.Philipsfisio820ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Philipsfisio820ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Casioa5302ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Casioa5302ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Casioa5302Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KDDI-CA22"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["canSendMail"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "9000"; dictionary["mobileDeviceManufacturer"] = "Casio"; dictionary["mobileDeviceModel"] = "A5302CA"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenPixelsHeight"] = "147"; dictionary["screenPixelsWidth"] = "132"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("CasioA5302"); this.Casioa5302ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Casioa5302ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Tcll668ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Tcll668ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Tcll668Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Compal-Seville"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Openwave"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "3800"; dictionary["maximumSoftkeyLabelLength"] = "7"; dictionary["mobileDeviceManufacturer"] = "TCL"; dictionary["mobileDeviceModel"] = "L668"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "false"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "14"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "128"; dictionary["supportsBold"] = "true"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsItalic"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["type"] = regexWorker["Openwave ${majorVersion}.x Browser"]; browserCaps.AddBrowser("TCLL668"); this.Tcll668ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Tcll668ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Kddits24ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Kddits24ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Kddits24Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "KDDI-TS24"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Openwave"; dictionary["canSendMail"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "9000"; dictionary["mobileDeviceManufacturer"] = "Toshiba"; dictionary["mobileDeviceModel"] = "A5304T"; dictionary["PreferredImageMime"] = "image/jpeg"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenPixelsHeight"] = "176"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsNoWrapStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsTitleElement"] = "false"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("KDDITS24"); this.Kddits24ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Kddits24ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sies55ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sies55ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sies55Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SIE-S55"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Openwave"; dictionary["canSendMail"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumHrefLength"] = "980"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceManufacturer"] = "Siemens"; dictionary["mobileDeviceModel"] = "S55"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "4"; dictionary["screenCharactersWidth"] = "19"; dictionary["screenPixelsHeight"] = "80"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "false"; dictionary["supportsNoWrapStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("SIES55"); this.Sies55ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sies55ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sharpgx10ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sharpgx10ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sharpgx10Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^SHARP\\-TQ\\-GX10"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Openwave"; dictionary["canSendMail"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceManufacturer"] = "Sharp"; dictionary["mobileDeviceModel"] = "GX10"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "9"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "120"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsNoWrapStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsTitleElement"] = "false"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("SHARPGx10"); this.Sharpgx10ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sharpgx10ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void BenqathenaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void BenqathenaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool BenqathenaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^BENQ\\-Athena"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Openwave"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceManufacturer"] = "BenQ"; dictionary["mobileDeviceModel"] = "S830c"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "8"; dictionary["screenCharactersWidth"] = "18"; dictionary["screenPixelsHeight"] = "0"; dictionary["screenPixelsWidth"] = "0"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsNoWrapStyle"] = "true"; dictionary["supportsRedirectWithCookie"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("BenQAthena"); this.BenqathenaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.BenqathenaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void OperaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void OperaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool OperaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Opera[ /](?\'version\'(?\'major\'\\d+)(?\'minor\'\\.\\d+)(?\'letters\'\\w*))"); if ((result == false)) { return false; } // Capture: header values regexWorker.ProcessRegex(((string)(browserCaps[string.Empty])), " (?\'screenWidth\'\\d*)x(?\'screenHeight\'\\d*)"); // Capabilities: set capabilities dictionary["browser"] = "Opera"; dictionary["cookies"] = "true"; dictionary["css1"] = "true"; dictionary["css2"] = "true"; dictionary["defaultScreenCharactersHeight"] = "40"; dictionary["defaultScreenCharactersWidth"] = "80"; dictionary["defaultScreenPixelsHeight"] = "480"; dictionary["defaultScreenPixelsWidth"] = "640"; dictionary["ecmascriptversion"] = "1.1"; dictionary["frames"] = "true"; dictionary["javascript"] = "true"; dictionary["letters"] = regexWorker["${letters}"]; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "false"; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["screenBitDepth"] = "8"; dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsDivNoWrap"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["tables"] = "true"; dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["type"] = regexWorker["Opera${major}"]; dictionary["version"] = regexWorker["${version}"]; browserCaps.Adapters["System.Web.UI.WebControls.CheckBox, System.Web, Version=2.0.0.0, Culture=neutral," + " PublicKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.HideDisabledControlAdapter"; browserCaps.Adapters["System.Web.UI.WebControls.RadioButton, System.Web, Version=2.0.0.0, Culture=neutr" + "al, PublicKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.HideDisabledControlAdapter"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("Opera"); this.OperaProcessGateways(headers, browserCaps); // gateway, parent=Opera this.OperamobileProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Opera if (this.Opera1to3betaProcess(headers, browserCaps)) { } else { if (this.Opera4Process(headers, browserCaps)) { } else { if (this.Opera5to9Process(headers, browserCaps)) { } else { if (this.OperapsionProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } this.OperaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void OperamobileProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void OperamobileProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool OperamobileProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Linux \\S+embedix"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isMobileDevice"] = "true"; this.OperamobileProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.OperamobileProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opera1to3betaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opera1to3betaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opera1to3betaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["letters"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^b"); if ((result == false)) { return false; } headerValue = ((string)(dictionary["majorversion"])); result = regexWorker.ProcessRegex(headerValue, "[1-3]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; browserCaps.AddBrowser("Opera1to3beta"); this.Opera1to3betaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Opera1to3betaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opera4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opera4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opera4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "4"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.3"; dictionary["supportsFileUpload"] = "true"; dictionary["xml"] = "true"; browserCaps.AddBrowser("Opera4"); this.Opera4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Opera4 if (this.Opera4betaProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Opera4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opera4betaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opera4betaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opera4betaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["letters"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^b"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; browserCaps.AddBrowser("Opera4beta"); this.Opera4betaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Opera4betaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opera5to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opera5to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opera5to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "[5-9]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.3"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "7000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["screenBitDepth"] = "24"; dictionary["supportsFileUpload"] = "true"; dictionary["supportsFontName"] = "false"; dictionary["supportsItalic"] = "false"; dictionary["w3cdomversion"] = "1.0"; dictionary["xml"] = "true"; browserCaps.AddBrowser("Opera5to9"); this.Opera5to9ProcessGateways(headers, browserCaps); // gateway, parent=Opera5to9 this.Opera5to9betaProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Opera5to9 if (this.Opera6to9Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Opera5to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opera6to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opera6to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opera6to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "[6-9]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["backgroundsounds"] = "true"; dictionary["css1"] = "true"; dictionary["css2"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["javaapplets"] = "true"; dictionary["maximumRenderedPageSize"] = "2000000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["screenBitDepth"] = "32"; dictionary["supportsFontName"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["xml"] = "false"; browserCaps.Adapters["System.Web.UI.WebControls.CheckBox, System.Web, Version=2.0.0.0, Culture=neutral," + " PublicKeyToken=b03f5f7f11d50a3a"] = ""; browserCaps.Adapters["System.Web.UI.WebControls.RadioButton, System.Web, Version=2.0.0.0, Culture=neutr" + "al, PublicKeyToken=b03f5f7f11d50a3a"] = ""; browserCaps.Adapters["System.Web.UI.WebControls.TextBox, System.Web, Version=2.0.0.0, Culture=neutral, " + "PublicKeyToken=b03f5f7f11d50a3a"] = ""; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = ""; browserCaps.HtmlTextWriter = ""; browserCaps.AddBrowser("Opera6to9"); this.Opera6to9ProcessGateways(headers, browserCaps); // gateway, parent=Opera6to9 this.OperamobilebrowserProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Opera6to9 if (this.Opera7to9Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Opera6to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opera7to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opera7to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opera7to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "[7-9]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ecmascriptversion"] = "1.4"; dictionary["supportsMaintainScrollPositionOnPostback"] = "true"; browserCaps.AddBrowser("Opera7to9"); this.Opera7to9ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Opera7to9 if (this.Opera8to9Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Opera7to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opera8to9ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opera8to9ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opera8to9Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["majorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "[8-9]"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["supportsCallback"] = "true"; browserCaps.AddBrowser("Opera8to9"); this.Opera8to9ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Opera8to9ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void OperamobilebrowserProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void OperamobilebrowserProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool OperamobilebrowserProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "; (?\'screenWidth\'\\d+)x(?\'screenHeight\'\\d+)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["backgroundSounds"] = "false"; dictionary["canSendMail"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["JavaApplets"] = "false"; dictionary["maximumRenderedPageSize"] = "200000"; dictionary["msDomVersion"] = "0.0"; dictionary["preferredImageMime"] = "image/gif"; dictionary["requiredMetaTagNameValue"] = "HandheldFriendly"; dictionary["requiresPragmaNoCacheHeader"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsItalic"] = "true"; dictionary["tagwriter"] = "System.Web.UI.Html32TextWriter"; this.OperamobilebrowserProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.OperamobilebrowserProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Opera5to9betaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Opera5to9betaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Opera5to9betaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["letters"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^b"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; this.Opera5to9betaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Opera5to9betaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void OperapsionProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void OperapsionProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool OperapsionProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Mozilla/.* \\(compatible; Opera .*; EPOC; (?\'screenWidth\'\\d*)x(?\'screenHeight\'\\d*)" + "\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["canRenderEmptySelects"] = "false"; dictionary["canSendMail"] = "false"; dictionary["css1"] = "false"; dictionary["css2"] = "false"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["maximumRenderedPageSize"] = "2560"; dictionary["mobileDeviceManufacturer"] = "Psion"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "50"; dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; dictionary["supportsBodyColor"] = "false"; dictionary["supportsCss"] = "false"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsSelectMultiple"] = "false"; dictionary["tagwriter"] = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("OperaPsion"); this.OperapsionProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.OperapsionProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MypalmProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MypalmProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MypalmProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Mozilla/2\\.0 \\(compatible; Elaine/(?\'gatewayMajorVersion\'\\w*)(?\'gatewayMinorVersi" + "on\'\\.\\w*)\\)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "MyPalm"; dictionary["cookies"] = "false"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "12"; dictionary["defaultScreenPixelsHeight"] = "72"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["ecmascriptversion"] = "1.1"; dictionary["frames"] = "true"; dictionary["gatewayMajorVersion"] = regexWorker["${gatewayMajorVersion}"]; dictionary["gatewayMinorVersion"] = regexWorker["${gatewayMinorVersion}"]; dictionary["gatewayVersion"] = regexWorker["${gatewayMajorVersion}${gatewayMinorVersion}"]; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["javaapplets"] = "false"; dictionary["javascript"] = "false"; dictionary["mobileDeviceManufacturer"] = "PalmOS-licensee"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiredMetaTagNameValue"] = "PalmComputingPlatform"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["screenBitDepth"] = "2"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "36"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "false"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsEmptyStringInCookieValue"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsImageSubmit"] = "false"; dictionary["tables"] = "false"; dictionary["type"] = "MyPalm"; dictionary["vbscript"] = "false"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("Mypalm"); this.MypalmProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Mypalm if (this.Mypalm1Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.MypalmProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mypalm1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mypalm1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mypalm1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["gatewayMajorVersion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^1"); if ((result == false)) { return false; } headerValue = ((string)(dictionary["gatewayMinorVersion"])); result = regexWorker.ProcessRegex(headerValue, "\\.0$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "EarthLink"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "false"; dictionary["maximumRenderedPageSize"] = "7000"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["rendersBreaksAfterHtmlLists"] = "false"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["requiresUniqueHtmlCheckboxNames"] = "true"; dictionary["requiresUniqueHtmlInputNames"] = "true"; dictionary["screenBitDepth"] = "4"; dictionary["screenCharactersHeight"] = "13"; dictionary["screenCharactersWidth"] = "30"; dictionary["supportsFontSize"] = "false"; dictionary["tables"] = "true"; dictionary["type"] = "EarthLink"; browserCaps.AddBrowser("MyPalm1"); this.Mypalm1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mypalm1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PalmwebproProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PalmwebproProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PalmwebproProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "PalmOS.*WebPro"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Palm WebPro"; dictionary["canSendMail"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["javascript"] = "false"; dictionary["maximumRenderedPageSize"] = "7000"; dictionary["mobileDeviceManufacturer"] = "Palm"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiredMetaTagNameValue"] = "PalmComputingPlatform"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOutputOptimization"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "30"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "320"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsCss"] = "false"; dictionary["supportsDivAlign"] = "false"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFileUpload"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["tables"] = "false"; dictionary["type"] = "Palm WebPro"; browserCaps.AddBrowser("PalmWebPro"); this.PalmwebproProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=PalmWebPro if (this.Palmwebpro3Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.PalmwebproProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Palmwebpro3ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Palmwebpro3ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Palmwebpro3Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "WebPro3\\."); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["CanSendMail"] = "true"; dictionary["InputType"] = "keyboard"; dictionary["JavaScript"] = "true"; dictionary["MaximumRenderedPageSize"] = "520000"; dictionary["maximumHrefLength"] = "2000"; dictionary["preferredRequestEncoding"] = "ISO-8859-1"; dictionary["preferredResponseEncoding"] = "ISO-8859-1"; dictionary["RequiresControlStateInSession"] = "false"; dictionary["RequiresOutputOptimization"] = "false"; dictionary["RequiresPragmaNoCacheHeader"] = "false"; dictionary["RequiresUniqueFilePathSuffix"] = "false"; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["Tables"] = "true"; browserCaps.AddBrowser("PalmWebPro3"); this.Palmwebpro3ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Palmwebpro3ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void EudorawebProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void EudorawebProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool EudorawebProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "EudoraWeb (?\'browserMajorVersion\'\\d+)(?\'browserMinorVersion\'\\.\\d+)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "EudoraWeb"; dictionary["canInitiateVoiceCall"] = "false"; dictionary["cookies"] = "true"; dictionary["isColor"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isMobileDevice"] = "true"; dictionary["javaapplets"] = "false"; dictionary["javascript"] = "false"; dictionary["maximumRenderedPageSize"] = "30000"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceManufacturer"] = "PalmOS-licensee"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["screenBitDepth"] = "1"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "36"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "false"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsImageSubmit"] = "false"; dictionary["supportsItalic"] = "true"; dictionary["tables"] = "false"; dictionary["type"] = "EudoraWeb"; dictionary["vbscript"] = "false"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("Eudoraweb"); this.EudorawebProcessGateways(headers, browserCaps); // gateway, parent=Eudoraweb this.EudorawebmsieProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Eudoraweb if (this.PdqbrowserProcess(headers, browserCaps)) { } else { if (this.Eudoraweb21plusProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.EudorawebProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void EudorawebmsieProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void EudorawebmsieProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool EudorawebmsieProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MSIE (?\'version\'(?\'msMajorVersion\'\\d+)(?\'msMinorVersion\'\\.\\d+)(?\'letters\'\\w*))(?\'" + "extra\'[^)]*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["activexcontrols"] = "true"; dictionary["backgroundsounds"] = "true"; dictionary["ecmaScriptVersion"] = "1.2"; dictionary["frames"] = "true"; dictionary["msdomversion"] = regexWorker["${msMajorVersion}${msMinorVersion}"]; dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["w3cdomversion"] = "1.0"; this.EudorawebmsieProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.EudorawebmsieProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PdqbrowserProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PdqbrowserProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PdqbrowserProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "pdQbrowser"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "true"; dictionary["mobileDeviceManufacturer"] = "Kyocera"; dictionary["mobileDeviceModel"] = "QCP 6035"; dictionary["supportsFontSize"] = "false"; browserCaps.AddBrowser("PdQbrowser"); this.PdqbrowserProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PdqbrowserProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Eudoraweb21plusProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Eudoraweb21plusProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Eudoraweb21plusProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "([3-9]\\.\\d+)|(2\\.[1-9]\\d*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["requiresUniqueHtmlCheckboxNames"] = "true"; dictionary["screenCharactersHeight"] = "11"; dictionary["screenCharactersWidth"] = "30"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsFontColor"] = "false"; dictionary["tables"] = "true"; browserCaps.AddBrowser("Eudoraweb21Plus"); this.Eudoraweb21plusProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Eudoraweb21plusProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PalmscapeProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PalmscapeProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PalmscapeProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Palmscape/.*\\(v. (?\'version\'[^;]+);"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Palmscape"; dictionary["cookies"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isMobileDevice"] = "true"; dictionary["mobileDeviceManufacturer"] = "PalmOS-licensee"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "36"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsCharacterEntityEncodign"] = "false"; dictionary["type"] = "Palmscape"; dictionary["version"] = regexWorker["${version}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("Palmscape"); this.PalmscapeProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Palmscape if (this.PalmscapeversionProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.PalmscapeProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PalmscapeversionProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PalmscapeversionProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PalmscapeversionProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "(?\'browserMajorVersion\'\\d+)(?\'browserMinorVersion\'\\.\\d+)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; browserCaps.AddBrowser("PalmscapeVersion"); this.PalmscapeversionProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PalmscapeversionProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void AuspalmProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void AuspalmProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool AuspalmProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "AUS PALM WAPPER"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "AU-System Demo WAP Browser"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isMobileDevice"] = "true"; dictionary["mobileDeviceManufacturer"] = "PalmOS-licensee"; dictionary["optimumPageWeight"] = "900"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml11"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "36"; dictionary["screenPixelsHeight"] = "160"; dictionary["screenPixelsWidth"] = "160"; dictionary["supportsCharacterEntityEncoding"] = "true"; dictionary["type"] = "AU-System"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("AusPalm"); this.AuspalmProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.AuspalmProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SharppdaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SharppdaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SharppdaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "sharp pda browser/(?\'browserMajorVersion\'\\d+)(?\'browserMinorVersion\'\\.\\d+)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Sharp PDA Browser"; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["mobileDeviceManufacturer"] = "Sharp"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["supportsCharacterEntityEncoding"] = "false"; dictionary["type"] = "Sharp PDA Browser"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("SharpPda"); this.SharppdaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=SharpPda if (this.Zaurusmie1Process(headers, browserCaps)) { } else { if (this.Zaurusmie21Process(headers, browserCaps)) { } else { if (this.Zaurusmie25Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.SharppdaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Zaurusmie1ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Zaurusmie1ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Zaurusmie1Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MI-E1"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["defaultCharacterHeight"] = "18"; dictionary["defaultCharacterWidth"] = "7"; dictionary["frames"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["javascript"] = "false"; dictionary["mobileDeviceModel"] = "Zaurus MI-E1"; dictionary["requiresDBCSCharacter"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenPixelsHeight"] = "240"; dictionary["screenPixelsWidth"] = "320"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("ZaurusMiE1"); this.Zaurusmie1ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Zaurusmie1ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Zaurusmie21ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Zaurusmie21ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Zaurusmie21Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MI-E21"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["canRenderEmptySelects"] = "false"; dictionary["cookies"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "60000"; dictionary["mobileDeviceModel"] = "Zaurus MI-E21"; dictionary["requiresAttributeColonSubstitution"] = "true"; dictionary["requiresDBCSCharacter"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "18"; dictionary["screenCharactersWidth"] = "40"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "240"; dictionary["supportsFontSize"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("ZaurusMiE21"); this.Zaurusmie21ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Zaurusmie21ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Zaurusmie25ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Zaurusmie25ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Zaurusmie25Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MI-E25"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["cookies"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "60000"; dictionary["mobileDeviceModel"] = "Zaurus MI-E25DC"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["requiresDBCSCharacter"] = "true"; dictionary["requiresOutputOptimization"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "11"; dictionary["screenCharactersWidth"] = "50"; dictionary["screenPixelsHeight"] = "240"; dictionary["screenPixelsWidth"] = "320"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["tables"] = "true"; browserCaps.AddBrowser("ZaurusMiE25"); this.Zaurusmie25ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Zaurusmie25ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PanasonicProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PanasonicProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PanasonicProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Panasonic-(?\'deviceModel\'.*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Panasonic"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["maximumSoftkeyLabelLength"] = "16"; dictionary["mobileDeviceManufacturer"] = "Panasonic"; dictionary["mobileDeviceModel"] = regexWorker["${deviceModel}"]; dictionary["numberOfSoftkeys"] = "2"; dictionary["preferredImageMime"] = "image/vnd.wap.wbmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersWmlDoAcceptsInline"] = "false"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "16"; dictionary["screenPixelsHeight"] = "130"; dictionary["screenPixelsWidth"] = "100"; dictionary["supportsCacheControlMetaTag"] = "false"; dictionary["tables"] = "false"; dictionary["type"] = "Panasonic"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("Panasonic"); this.PanasonicProcessGateways(headers, browserCaps); // gateway, parent=Panasonic this.PanasonicexchangesupporteddeviceProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Panasonic if (this.Panasonicgad95Process(headers, browserCaps)) { } else { if (this.Panasonicgad87Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.PanasonicProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Panasonicgad95ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Panasonicgad95ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Panasonicgad95Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "GAD95"); if ((result == false)) { return false; } // Capabilities: set capabilities browserCaps.AddBrowser("PanasonicGAD95"); this.Panasonicgad95ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Panasonicgad95ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Panasonicgad87ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Panasonicgad87ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Panasonicgad87Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "GAD87"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Panasonic"; dictionary["canSendMail"] = "true"; dictionary["cookies"] = "true"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "12000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-basic"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenPixelsHeight"] = "176"; dictionary["screenPixelsWidth"] = "132"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "false"; dictionary["type"] = "Panasonic"; browserCaps.AddBrowser("PanasonicGAD87"); this.Panasonicgad87ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=PanasonicGAD87 if (this.Panasonicgad87a39Process(headers, browserCaps)) { } else { if (this.Panasonicgad87a38Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.Panasonicgad87ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PanasonicexchangesupporteddeviceProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PanasonicexchangesupporteddeviceProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PanasonicexchangesupporteddeviceProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "\\/(A3[89]|A[4-9]\\d|A\\d{3,})"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["ExchangeOmaSupported"] = "true"; this.PanasonicexchangesupporteddeviceProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PanasonicexchangesupporteddeviceProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Panasonicgad87a39ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Panasonicgad87a39ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Panasonicgad87a39Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "GAD87\\/A39"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceModel"] = "GD87"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "7"; dictionary["screenCharactersWidth"] = "14"; dictionary["tables"] = "false"; browserCaps.AddBrowser("PanasonicGAD87A39"); this.Panasonicgad87a39ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Panasonicgad87a39ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Panasonicgad87a38ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Panasonicgad87a38ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Panasonicgad87a38Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["mobileDeviceModel"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "GAD87\\/A38"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["maximumRenderedPageSize"] = "12155"; dictionary["mobileDeviceModel"] = "GU87"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "20"; browserCaps.AddBrowser("PanasonicGAD87A38"); this.Panasonicgad87a38ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Panasonicgad87a38ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void WinceProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void WinceProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool WinceProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Mozilla/\\S* \\(compatible; MSIE (?\'majorVersion\'\\d*)(?\'minorVersion\'\\.\\d*);\\D* Wi" + "ndows CE(;(?\'deviceID\' \\D\\w*))?(; (?\'screenWidth\'\\d+)x(?\'screenHeight\'\\d+))?"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["activexcontrols"] = "true"; dictionary["backgroundsounds"] = "true"; dictionary["browser"] = "WinCE"; dictionary["cookies"] = "true"; dictionary["css1"] = "true"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "12"; dictionary["defaultScreenPixelsHeight"] = "72"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["deviceID"] = regexWorker["${deviceID}"]; dictionary["ecmascriptversion"] = "1.0"; dictionary["frames"] = "true"; dictionary["inputType"] = "telephoneKeypad"; dictionary["isColor"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["javascript"] = "true"; dictionary["jscriptversion"] = "1.0"; dictionary["majorVersion"] = regexWorker["${majorVersion}"]; dictionary["minorVersion"] = regexWorker["${minorVersion}"]; dictionary["platform"] = "WinCE"; dictionary["screenBitDepth"] = "1"; dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; dictionary["supportsMultilineTextBoxDisplay"] = "true"; dictionary["tables"] = "true"; dictionary["version"] = regexWorker["${majorVersion}${minorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("WinCE"); this.WinceProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=WinCE if (this.PieProcess(headers, browserCaps)) { } else { if (this.Pie4Process(headers, browserCaps)) { } else { if (this.Pie5plusProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.WinceProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PieProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PieProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PieProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^3\\.02$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Pocket IE"; dictionary["defaultCharacterHeight"] = "18"; dictionary["defaultCharacterWidth"] = "7"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["javascript"] = "true"; dictionary["majorVersion"] = "4"; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["minorVersion"] = ".0"; dictionary["mobileDeviceModel"] = "Pocket PC"; dictionary["optimumPageWeight"] = "4000"; dictionary["requiresContentTypeMetaTag"] = "true"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["supportsBodyColor"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "false"; dictionary["supportsDivAlign"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFontColor"] = "true"; dictionary["supportsFontName"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["type"] = "Pocket IE"; dictionary["version"] = "4.0"; browserCaps.AddBrowser("PIE"); this.PieProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=PIE if (this.PieppcProcess(headers, browserCaps)) { } else { if (this.PienodeviceidProcess(headers, browserCaps)) { } else { if (this.PiesmartphoneProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } this.PieProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PieppcProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PieppcProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PieppcProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, " PPC;"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["minorVersion"] = ".1"; dictionary["version"] = "4.1"; browserCaps.AddBrowser("PIEPPC"); this.PieppcProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PieppcProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Pie4ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Pie4ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Pie4Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MSIE 4(\\.\\d*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["activexcontrols"] = "true"; dictionary["browser"] = "Pocket IE"; dictionary["cdf"] = "true"; dictionary["defaultScreenCharactersHeight"] = "6"; dictionary["defaultScreenCharactersWidth"] = "12"; dictionary["defaultScreenPixelsHeight"] = "72"; dictionary["defaultScreenPixelsWidth"] = "96"; dictionary["ecmascriptversion"] = "1.2"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["javaapplets"] = "true"; dictionary["maximumRenderedPageSize"] = "7000"; dictionary["msdomversion"] = "4.0"; dictionary["platform"] = "WinCE"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "9"; dictionary["screenCharactersWidth"] = "50"; dictionary["screenPixelsHeight"] = "240"; dictionary["screenPixelsWidth"] = "640"; dictionary["supportsCss"] = "true"; dictionary["supportsDivNoWrap"] = "true"; dictionary["supportsFileUpload"] = "false"; dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["type"] = "Pocket IE"; dictionary["vbscript"] = "true"; browserCaps.AddBrowser("PIE4"); this.Pie4ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=PIE4 if (this.Pie4ppcProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Pie4ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Pie5plusProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Pie5plusProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Pie5plusProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MSIE 5(\\.\\d*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "IE"; dictionary["ecmascriptversion"] = "1.2"; dictionary["javaapplets"] = "true"; dictionary["msdomversion"] = "5.5"; dictionary["tagwriter"] = "System.Web.UI.HtmlTextWriter"; dictionary["type"] = "Pocket IE"; dictionary["vbscript"] = "true"; dictionary["w3cdomversion"] = "1.0"; dictionary["xml"] = "true"; browserCaps.AddBrowser("PIE5Plus"); this.Pie5plusProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=PIE5Plus if (this.Sigmarion3Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Pie5plusProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PienodeviceidProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PienodeviceidProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PienodeviceidProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["supportsQueryStringInFormAction"] = "false"; browserCaps.AddBrowser("PIEnoDeviceID"); this.PienodeviceidProcessGateways(headers, browserCaps); // gateway, parent=PIEnoDeviceID this.PiescreenbitdepthProcess(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PienodeviceidProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PiescreenbitdepthProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PiescreenbitdepthProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PiescreenbitdepthProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["UA-COLOR"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "color32"); if ((result == false)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["screenBitDepth"] = "32"; this.PiescreenbitdepthProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PiescreenbitdepthProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void NetfrontProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void NetfrontProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool NetfrontProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "\\((?\'deviceID\'.*)\\) NetFront\\/(?\'browserMajorVersion\'\\d*)(?\'browserMinorVersion\'\\" + ".\\d*).*"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Compact NetFront"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canSendMail"] = "false"; dictionary["inputType"] = "telephoneKeypad"; dictionary["isMobileDevice"] = "true"; dictionary["javascript"] = "false"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresFullyQualifiedRedirectUrl"] = "true"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFileUpload"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsImageSubmit"] = "false"; dictionary["supportsItalic"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["type"] = regexWorker["Compact NetFront ${browserMajorVersion}"]; browserCaps.AddBrowser("NetFront"); this.NetfrontProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=NetFront if (this.Slb500Process(headers, browserCaps)) { } else { if (this.VrnaProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.NetfrontProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Slb500ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Slb500ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Slb500Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SL-B500"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "NetFront"; dictionary["canInitiateVoiceCall"] = "false"; dictionary["canSendMail"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "60000"; dictionary["mobileDeviceManufacturer"] = "Sharp"; dictionary["mobileDeviceModel"] = "SL-B500"; dictionary["preferredImageMime"] = "image/gif"; dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "html32"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["requiresNoBreakInFormatting"] = "true"; dictionary["requiresOutputOptimization"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "21"; dictionary["screenCharactersWidth"] = "47"; dictionary["screenPixelsHeight"] = "240"; dictionary["screenPixelsWidth"] = "320"; dictionary["supportsAccessKeyAttribute"] = "false"; dictionary["supportsBold"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsMultilineTextboxDisplay"] = "true"; browserCaps.AddBrowser("SLB500"); this.Slb500ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Slb500ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void VrnaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void VrnaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool VrnaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "sony/model vrna"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Sony"; dictionary["mobileDeviceModel"] = "CLIE PEG-TG50"; dictionary["canInitiateVoiceCall"] = "false"; dictionary["canSendMail"] = "true"; dictionary["ExchangeOmaSupported"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["javascript"] = "true"; dictionary["maximumRenderedPageSize"] = "65000"; dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "html32"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOutputOptimization"] = "true"; dictionary["requiresPragmaNoCacheHeader"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenCharactersWidth"] = "31"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "320"; dictionary["supportsAccessKeyAttribute"] = "false"; dictionary["supportsImageSubmit"] = "true"; dictionary["supportsMultilineTextboxDisplay"] = "true"; browserCaps.AddBrowser("VRNA"); this.VrnaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.VrnaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Pie4ppcProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Pie4ppcProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Pie4ppcProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "PPC(; (?\'screenWidth\'\\d+)x(?\'screenHeight\'\\d+))?"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "MSIE"; dictionary["ecmascriptversion"] = "1.0"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["maximumRenderedPageSize"] = "800000"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingType"] = "html32"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "false"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "16"; dictionary["screenCharactersWidth"] = "32"; dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsNoWrapStyle"] = "false"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "false"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["type"] = regexWorker["MSIE ${majorVersion}"]; browserCaps.AddBrowser("PIE4PPC"); this.Pie4ppcProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Pie4ppcProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void PiesmartphoneProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void PiesmartphoneProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool PiesmartphoneProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Smartphone"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["canInitiateVoiceCall"] = "true"; dictionary["frames"] = "false"; dictionary["inputType"] = "telephoneKeypad"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "300000"; dictionary["minorVersion"] = ".1"; dictionary["mobileDeviceModel"] = "Smartphone"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresContentTypeMetaTag"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["screenCharactersHeight"] = "13"; dictionary["screenCharactersWidth"] = "28"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsFontName"] = "false"; dictionary["version"] = "4.1"; browserCaps.AddBrowser("PIESmartphone"); this.PiesmartphoneProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.PiesmartphoneProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sigmarion3ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sigmarion3ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sigmarion3Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "sigmarion3"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["cachesAllResponsesWithExpires"] = "true"; dictionary["inputType"] = "keyboard"; dictionary["isColor"] = "true"; dictionary["maximumRenderedPageSize"] = "64000"; dictionary["mobileDeviceManufacturer"] = "NTT DoCoMo"; dictionary["MobileDeviceModel"] = "Sigmarion III"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRequestEncoding"] = "shift_jis"; dictionary["preferredResponseEncoding"] = "shift_jis"; dictionary["requiresOutputOptimization"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["screenCharactersHeight"] = "19"; dictionary["screenCharactersWidth"] = "94"; dictionary["screenPixelsHeight"] = "480"; dictionary["screenPixelsWidth"] = "800"; browserCaps.AddBrowser("sigmarion3"); this.Sigmarion3ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sigmarion3ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mspie06ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mspie06ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mspie06Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Microsoft Pocket Internet Explorer/0\\.6"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["backgroundsounds"] = "true"; dictionary["browser"] = "PIE"; dictionary["cookies"] = "false"; dictionary["isMobileDevice"] = "true"; dictionary["majorversion"] = "1"; dictionary["minorversion"] = "0"; dictionary["platform"] = "WinCE"; dictionary["tables"] = "true"; dictionary["type"] = "PIE"; dictionary["version"] = "1.0"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("MSPIE06"); this.Mspie06ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mspie06ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void MspieProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void MspieProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool MspieProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^Mozilla[^(]*\\(compatible; MSPIE (?\'version\'(?\'major\'\\d+)(?\'minor\'\\.\\d+)(?\'letter" + "s\'\\w*))(?\'extra\'.*)"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["backgroundsounds"] = "true"; dictionary["browser"] = "PIE"; dictionary["cookies"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["PIE${major}"]; dictionary["version"] = regexWorker["${version}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("MSPIE"); this.MspieProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=MSPIE if (this.Mspie2Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.MspieProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Mspie2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Mspie2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Mspie2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "2\\."); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["frames"] = "true"; browserCaps.AddBrowser("MSPIE2"); this.Mspie2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Mspie2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, @"^(?'carrier'\S{3})(?'serviceType'\d)(?'deviceType'\d)(?'deviceManufacturer'\S{2})(?'deviceID'\S{2})(?'browserType'\d{2})(?'majorVersion'\d)(?'minorVersion'\d)(?'screenWidth'\d{3})(?'screenHeight'\d{3})(?'screenColumn'\d{2})(?'screenRow'\d{2})(?'colorDepth'\d{2})(?'MINNumber'\d{8})"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browserType"] = regexWorker["${browserType}"]; dictionary["colorDepth"] = regexWorker["${colorDepth}"]; dictionary["cookies"] = "false"; dictionary["deviceManufacturer"] = regexWorker["${deviceManufacturer}"]; dictionary["deviceID"] = regexWorker["${deviceID}"]; dictionary["deviceType"] = regexWorker["${deviceType}"]; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${majorVersion}"]; dictionary["minorVersion"] = regexWorker["${minorVersion}"]; dictionary["screenColumn"] = regexWorker["${screenColumn}"]; dictionary["screenHeight"] = regexWorker["${screenHeight}"]; dictionary["screenWidth"] = regexWorker["${screenWidth}"]; dictionary["screenRow"] = regexWorker["${screenRow}"]; dictionary["version"] = regexWorker["${majorVersion}.${minorVersion}"]; browserCaps.AddBrowser("SKTDevices"); this.SktdevicesProcessGateways(headers, browserCaps); // gateway, parent=SKTDevices this.SktdevicescolordepthProcess(headers, browserCaps); // gateway, parent=SKTDevices this.SktdevicesscreenrowProcess(headers, browserCaps); // gateway, parent=SKTDevices this.SktdevicesscreencolumnProcess(headers, browserCaps); // gateway, parent=SKTDevices this.SktdevicesscreenheightProcess(headers, browserCaps); // gateway, parent=SKTDevices this.SktdevicesscreenwidthProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=SKTDevices if (this.SktdeviceshyundaiProcess(headers, browserCaps)) { } else { if (this.SktdeviceshanhwaProcess(headers, browserCaps)) { } else { if (this.SktdevicesjtelProcess(headers, browserCaps)) { } else { if (this.SktdeviceslgProcess(headers, browserCaps)) { } else { if (this.SktdevicesmotorolaProcess(headers, browserCaps)) { } else { if (this.SktdevicesnokiaProcess(headers, browserCaps)) { } else { if (this.SktdevicesskttProcess(headers, browserCaps)) { } else { if (this.SktdevicessamsungProcess(headers, browserCaps)) { } else { if (this.SktdevicesericssonProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } } } } } } } } this.SktdevicesProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicescolordepthProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicescolordepthProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicescolordepthProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["colorDepth"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^(\\d[1-9])|([1-9]0)$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenBitDepth"] = regexWorker["${colorDepth}"]; this.SktdevicescolordepthProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=SKTDevicesColorDepth if (this.SktdevicesiscolorProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.SktdevicescolordepthProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesiscolorProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesiscolorProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesiscolorProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["colorDepth"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^(0[5-9])|([1-9]\\d)$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["isColor"] = "true"; this.SktdevicesiscolorProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdevicesiscolorProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesscreenrowProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesscreenrowProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesscreenrowProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["screenRow"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^(\\d[1-9])|([1-9]0)$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenCharactersHeight"] = regexWorker["${screenRow}"]; this.SktdevicesscreenrowProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdevicesscreenrowProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesscreencolumnProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesscreencolumnProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesscreencolumnProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["screenColumn"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^(\\d[1-9])|([1-9]0)$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenCharactersWidth"] = regexWorker["${screenColumn}"]; this.SktdevicesscreencolumnProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdevicesscreencolumnProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesscreenheightProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesscreenheightProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesscreenheightProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["screenHeight"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^(\\d\\d[1-9])|(((\\d[1-9])|([1-9]0))0)$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; this.SktdevicesscreenheightProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdevicesscreenheightProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesscreenwidthProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesscreenwidthProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesscreenwidthProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["screenWidth"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^(\\d\\d[1-9])|(((\\d[1-9])|([1-9]0))0)$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; this.SktdevicesscreenwidthProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdevicesscreenwidthProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdeviceshyundaiProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdeviceshyundaiProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdeviceshyundaiProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceManufacturer"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HD"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hyundai"; browserCaps.AddBrowser("SKTDevicesHyundai"); this.SktdeviceshyundaiProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=SKTDevicesHyundai if (this.Pse200Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.SktdeviceshyundaiProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Pse200ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Pse200ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Pse200Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "10"); if ((result == false)) { return false; } headerValue = ((string)(dictionary["browserType"])); result = regexWorker.ProcessRegex(headerValue, "15"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Infraware"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceManufacturer"] = "Pantech&Curitel"; dictionary["mobileDeviceModel"] = "PS-E200"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresOnEnterForwardForCheckboxLists"] = "true"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "10"; dictionary["screenPixelsHeight"] = "80"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsNoWrapStyle"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["${browser} ${majorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.XhtmlTextWriter"; browserCaps.AddBrowser("PSE200"); this.Pse200ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Pse200ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdeviceshanhwaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdeviceshanhwaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdeviceshanhwaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceManufacturer"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "HH"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Hanhwa"; browserCaps.AddBrowser("SKTDevicesHanhwa"); this.SktdeviceshanhwaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdeviceshanhwaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesjtelProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesjtelProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesjtelProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceManufacturer"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "JT"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "JTEL"; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.HtmlTextWriter = "System.Web.UI.ChtmlTextWriter"; browserCaps.AddBrowser("SKTDevicesJTEL"); this.SktdevicesjtelProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=SKTDevicesJTEL if (this.Jtel01Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.SktdevicesjtelProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Jtel01ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Jtel01ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Jtel01Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["inputType"] = "virtualKeyboard"; dictionary["mobileDeviceModel"] = "Cellvic XG"; dictionary["screenCharactersHeight"] = "6"; dictionary["screenCharactersWidth"] = "12"; browserCaps.AddBrowser("JTEL01"); this.Jtel01ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=JTEL01 if (this.JtelnateProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.Jtel01ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void JtelnateProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void JtelnateProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool JtelnateProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["browserType"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "03"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "NATE"; dictionary["cookies"] = "true"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "true"; dictionary["requiresLeadingPageBreak"] = "true"; dictionary["supportsBodyColor"] = "false"; dictionary["supportsBold"] = "true"; dictionary["supportsFontColor"] = "false"; dictionary["tables"] = "true"; dictionary["type"] = "NATE 1"; browserCaps.AddBrowser("JTELNate"); this.JtelnateProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.JtelnateProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdeviceslgProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdeviceslgProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdeviceslgProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceManufacturer"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "LG"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "LG"; browserCaps.AddBrowser("SKTDevicesLG"); this.SktdeviceslgProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdeviceslgProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesmotorolaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesmotorolaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesmotorolaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceManufacturer"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "MT"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Motorola"; browserCaps.AddBrowser("SKTDevicesMotorola"); this.SktdevicesmotorolaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=SKTDevicesMotorola if (this.Sktdevicesv730Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.SktdevicesmotorolaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sktdevicesv730ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sktdevicesv730ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sktdevicesv730Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceID"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "07"); if ((result == false)) { return false; } headerValue = ((string)(dictionary["browserType"])); result = regexWorker.ProcessRegex(headerValue, "00"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "AU-System"; dictionary["canInitiateVoiceCall"] = "true"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "false"; dictionary["maximumRenderedPageSize"] = "2900"; dictionary["mobileDeviceModel"] = "V730"; dictionary["numberOfSoftkeys"] = "2"; dictionary["preferredImageMime"] = "image/bmp"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml11"; dictionary["rendersBreakBeforeWmlSelectAndInput"] = "true"; dictionary["rendersBreaksAfterWmlInput"] = "true"; dictionary["rendersWmlSelectsAsMenuCards"] = "true"; dictionary["requiresNoSoftkeyLabels"] = "true"; dictionary["requiresUniqueFilePathSuffix"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["type"] = regexWorker["${browser} ${majorVersion}"]; browserCaps.AddBrowser("SKTDevicesV730"); this.Sktdevicesv730ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sktdevicesv730ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesnokiaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesnokiaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesnokiaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceManufacturer"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "NO"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Nokia"; browserCaps.AddBrowser("SKTDevicesNokia"); this.SktdevicesnokiaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdevicesnokiaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesskttProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesskttProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesskttProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceManufacturer"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SK"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "SKTT"; browserCaps.AddBrowser("SKTDevicesSKTT"); this.SktdevicesskttProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdevicesskttProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicessamsungProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicessamsungProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicessamsungProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["deviceManufacturer"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "SS"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["mobileDeviceManufacturer"] = "Samsung"; browserCaps.AddBrowser("SKTDevicesSamSung"); this.SktdevicessamsungProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=SKTDevicesSamSung if (this.Sche150Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.SktdevicessamsungProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Sche150ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Sche150ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Sche150Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["browserType"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "15"); if ((result == false)) { return false; } headerValue = ((string)(dictionary["deviceID"])); result = regexWorker.ProcessRegex(headerValue, "50"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["breaksOnInlineElements"] = "false"; dictionary["browser"] = "Infraware"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "true"; dictionary["maximumRenderedPageSize"] = "10000"; dictionary["mobileDeviceModel"] = "SCH-E150"; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "application/xhtml+xml"; dictionary["preferredRenderingType"] = "xhtml-mp"; dictionary["requiresAbsolutePostbackUrl"] = "false"; dictionary["requiresCommentInStyleElement"] = "false"; dictionary["requiresHiddenFieldValues"] = "false"; dictionary["requiresHtmlAdaptiveErrorReporting"] = "true"; dictionary["requiresXhtmlCssSuppression"] = "false"; dictionary["screenBitDepth"] = "24"; dictionary["screenPixelsHeight"] = "80"; dictionary["screenPixelsWidth"] = "101"; dictionary["supportsAccessKeyAttribute"] = "true"; dictionary["supportsBodyClassAttribute"] = "true"; dictionary["supportsBold"] = "true"; dictionary["supportsCss"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsNoWrapStyle"] = "true"; dictionary["supportsSelectFollowingTable"] = "true"; dictionary["supportsStyleElement"] = "true"; dictionary["supportsTitleElement"] = "true"; dictionary["supportsUrlAttributeEncoding"] = "true"; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["${browser} ${majorVersion}"]; browserCaps.AddBrowser("SCHE150"); this.Sche150ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Sche150ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void SktdevicesericssonProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void SktdevicesericssonProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool SktdevicesericssonProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["browserType"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "01"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Ericsson"; browserCaps.AddBrowser("SKTDevicesEricsson"); this.SktdevicesericssonProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.SktdevicesericssonProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void WebtvProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void WebtvProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool WebtvProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "WebTV/(?\'version\'(?\'major\'\\d+)(?\'minor\'\\.\\d+)(?\'letters\'\\w*))"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["backgroundsounds"] = "true"; dictionary["browser"] = "WebTV"; dictionary["cookies"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["letters"] = regexWorker["${letters}"]; dictionary["majorversion"] = regexWorker["${major}"]; dictionary["minorversion"] = regexWorker["${minor}"]; dictionary["tables"] = "true"; dictionary["type"] = regexWorker["WebTV${major}"]; dictionary["version"] = regexWorker["${version}"]; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("WebTV"); this.WebtvProcessGateways(headers, browserCaps); // gateway, parent=WebTV this.WebtvbetaProcess(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=WebTV if (this.Webtv2Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.WebtvProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Webtv2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Webtv2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Webtv2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["minorversion"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "2"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["css1"] = "true"; dictionary["ecmascriptversion"] = "1.0"; dictionary["isMobileDevice"] = "false"; dictionary["javascript"] = "true"; dictionary["supportsBold"] = "false"; dictionary["supportsCss"] = "false"; dictionary["supportsDivNoWrap"] = "false"; dictionary["supportsFontName"] = "false"; dictionary["supportsFontSize"] = "false"; dictionary["supportsImageSubmit"] = "false"; dictionary["supportsItalic"] = "false"; browserCaps.AddBrowser("WebTV2"); this.Webtv2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Webtv2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void WebtvbetaProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void WebtvbetaProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool WebtvbetaProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["letters"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^b"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["beta"] = "true"; this.WebtvbetaProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.WebtvbetaProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void WinwapProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void WinwapProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool WinwapProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^WinWAP-(?\'platform\'\\w*)/(?\'browserMajorVersion\'\\w*)(?\'browserMinorVersion\'\\.\\w*)" + ""); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "WinWAP"; dictionary["canRenderAfterInputOrSelectElement"] = "false"; dictionary["canSendMail"] = "false"; dictionary["cookies"] = "false"; dictionary["hasBackButton"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "3500"; dictionary["maximumSoftkeyLabelLength"] = "21"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["platform"] = regexWorker["${platform}"]; dictionary["preferredImageMime"] = "image/jpeg"; dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml12"; dictionary["rendersBreaksAfterWmlInput"] = "false"; dictionary["rendersWmlSelectsAsMenuCards"] = "false"; dictionary["requiresSpecialViewStateEncoding"] = "true"; dictionary["requiresUniqueFilepathSuffix"] = "true"; dictionary["screenBitDepth"] = "24"; dictionary["screenCharactersHeight"] = "16"; dictionary["screenCharactersWidth"] = "43"; dictionary["screenPixelsHeight"] = "320"; dictionary["screenPixelsWidth"] = "240"; dictionary["supportsBold"] = "true"; dictionary["supportsFontSize"] = "true"; dictionary["supportsItalic"] = "true"; dictionary["supportsRedirectWithCookie"] = "false"; dictionary["type"] = regexWorker["WinWAP ${browserMajorVersion}${browserMinorVersion}"]; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.Adapters["System.Web.UI.WebControls.Menu, System.Web, Version=2.0.0.0, Culture=neutral, Pub" + "licKeyToken=b03f5f7f11d50a3a"] = "System.Web.UI.WebControls.Adapters.MenuAdapter"; browserCaps.AddBrowser("WinWap"); this.WinwapProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.WinwapProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void XiinoProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void XiinoProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool XiinoProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(browserCaps[string.Empty])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "Xiino/(?\'browserMajorVersion\'\\d+)(?\'browserMinorVersion\'\\.\\d+).* (?\'screenWidth\'\\" + "d+)x(?\'screenHeight\'\\d+);"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["browser"] = "Xiino"; dictionary["canRenderEmptySelects"] = "true"; dictionary["canSendMail"] = "false"; dictionary["hidesRightAlignedMultiselectScrollbars"] = "false"; dictionary["inputType"] = "virtualKeyboard"; dictionary["isColor"] = "true"; dictionary["isMobileDevice"] = "true"; dictionary["majorVersion"] = regexWorker["${browserMajorVersion}"]; dictionary["maximumRenderedPageSize"] = "65000"; dictionary["minorVersion"] = regexWorker["${browserMinorVersion}"]; dictionary["requiresAdaptiveErrorReporting"] = "true"; dictionary["requiresAttributeColonSubstitution"] = "false"; dictionary["screenBitDepth"] = "8"; dictionary["screenCharactersHeight"] = "12"; dictionary["screenCharactersWidth"] = "30"; dictionary["screenPixelsHeight"] = regexWorker["${screenHeight}"]; dictionary["screenPixelsWidth"] = regexWorker["${screenWidth}"]; dictionary["supportsBold"] = "true"; dictionary["supportsCharacterEntityEncoding"] = "false"; dictionary["supportsFontSize"] = "true"; dictionary["type"] = "Xiino"; dictionary["version"] = regexWorker["${browserMajorVersion}${browserMinorVersion}"]; browserCaps.HtmlTextWriter = "System.Web.UI.Html32TextWriter"; browserCaps.AddBrowser("Xiino"); this.XiinoProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = true; // browser, parent=Xiino if (this.Xiinov2Process(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } this.XiinoProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void Xiinov2ProcessGateways(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } protected virtual void Xiinov2ProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool Xiinov2Process(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check capability matches string headerValue; headerValue = ((string)(dictionary["version"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "^2\\.0$"); if ((result == false)) { return false; } // Capabilities: set capabilities dictionary["preferredImageMime"] = "image/jpeg"; dictionary["requiresOutputOptimization"] = "true"; dictionary["screenBitDepth"] = "16"; dictionary["supportsItalic"] = "true"; browserCaps.AddBrowser("XiinoV2"); this.Xiinov2ProcessGateways(headers, browserCaps); bool ignoreApplicationBrowsers = false; this.Xiinov2ProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void DefaultDefaultProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool DefaultDefaultProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Capabilities: set capabilities dictionary["ecmascriptversion"] = "0.0"; dictionary["javascript"] = "false"; dictionary["jscriptversion"] = "0.0"; bool ignoreApplicationBrowsers = true; // browser, parent=Default if (this.DefaultWmlProcess(headers, browserCaps)) { } else { if (this.DefaultXhtmlmpProcess(headers, browserCaps)) { } else { ignoreApplicationBrowsers = false; } } this.DefaultDefaultProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void DefaultWmlProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool DefaultWmlProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["Accept"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "text/vnd\\.wap\\.wml|text/hdml"); if ((result == false)) { return false; } headerValue = ((string)(headers["Accept"])); result = regexWorker.ProcessRegex(headerValue, "application/xhtml\\+xml; profile|application/vnd\\.wap\\.xhtml\\+xml"); if ((result == true)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["preferredRenderingMime"] = "text/vnd.wap.wml"; dictionary["preferredRenderingType"] = "wml11"; bool ignoreApplicationBrowsers = false; this.DefaultWmlProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected virtual void DefaultXhtmlmpProcessBrowsers(bool ignoreApplicationBrowsers, System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { } private bool DefaultXhtmlmpProcess(System.Collections.Specialized.NameValueCollection headers, System.Web.HttpBrowserCapabilities browserCaps) { System.Collections.IDictionary dictionary; dictionary = browserCaps.Capabilities; // Identification: check header matches string headerValue; headerValue = ((string)(headers["Accept"])); bool result; RegexWorker regexWorker; regexWorker = new RegexWorker(browserCaps); result = regexWorker.ProcessRegex(headerValue, "application/xhtml\\+xml; profile|application/vnd\\.wap\\.xhtml\\+xml"); if ((result == false)) { return false; } headerValue = ((string)(headers["Accept"])); result = regexWorker.ProcessRegex(headerValue, "text/hdml"); if ((result == true)) { return false; } headerValue = ((string)(headers["Accept"])); result = regexWorker.ProcessRegex(headerValue, "text/vnd\\.wap\\.wml"); if ((result == true)) { return false; } browserCaps.DisableOptimizedCacheKey(); // Capabilities: set capabilities dictionary["preferredRenderingMime"] = "text/html"; dictionary["preferredRenderingType"] = "xhtml-mp"; browserCaps.HtmlTextWriter = "System.Web.UI.XhtmlTextWriter"; bool ignoreApplicationBrowsers = false; this.DefaultXhtmlmpProcessBrowsers(ignoreApplicationBrowsers, headers, browserCaps); return true; } protected override void PopulateMatchedHeaders(System.Collections.IDictionary dictionary) { base.PopulateMatchedHeaders(dictionary); dictionary["X-UP-DEVCAP-NUMSOFTKEYS"] = null; dictionary["UA-COLOR"] = null; dictionary["UA-PIXELS"] = null; dictionary["HTTP_X_WAP_PROFILE"] = null; dictionary["X-UP-DEVCAP-SCREENDEPTH"] = null; dictionary["X-UP-DEVCAP-MSIZE"] = null; dictionary["X-UP-DEVCAP-MAX-PDU"] = null; dictionary["X-UP-DEVCAP-CHARSET"] = null; dictionary["X-GA-TABLES"] = null; dictionary["X-AVANTGO-VERSION"] = null; dictionary["X-UP-DEVCAP-SOFTKEYSIZE"] = null; dictionary["UA-VOICE"] = null; dictionary["X-UP-DEVCAP-ISCOLOR"] = null; dictionary["X-JPHONE-DISPLAY"] = null; dictionary["X-UP-DEVCAP-SCREENPIXELS"] = null; dictionary["X-JPHONE-COLOR"] = null; dictionary["X-UP-DEVCAP-SCREENCHARS"] = null; dictionary["Accept"] = null; dictionary[""] = null; dictionary["VIA"] = null; dictionary["X-GA-MAX-TRANSFER"] = null; } protected override void PopulateBrowserElements(System.Collections.IDictionary dictionary) { base.PopulateBrowserElements(dictionary); dictionary["Default"] = new System.Web.UI.Triplet(null, string.Empty, 0); dictionary["Mozilla"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["IE"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["IE5to9"] = new System.Web.UI.Triplet("Ie", string.Empty, 3); dictionary["IE6to9"] = new System.Web.UI.Triplet("Ie5to9", string.Empty, 4); dictionary["Treo600"] = new System.Web.UI.Triplet("Ie6to9", string.Empty, 5); dictionary["IE5"] = new System.Web.UI.Triplet("Ie5to9", string.Empty, 4); dictionary["IE50"] = new System.Web.UI.Triplet("Ie5", string.Empty, 5); dictionary["IE55"] = new System.Web.UI.Triplet("Ie5", string.Empty, 5); dictionary["IE5to9Mac"] = new System.Web.UI.Triplet("Ie5to9", string.Empty, 4); dictionary["IE4"] = new System.Web.UI.Triplet("Ie", string.Empty, 3); dictionary["IE3"] = new System.Web.UI.Triplet("Ie", string.Empty, 3); dictionary["IE3win16"] = new System.Web.UI.Triplet("Ie3", string.Empty, 4); dictionary["IE3win16a"] = new System.Web.UI.Triplet("Ie3win16", string.Empty, 5); dictionary["IE3Mac"] = new System.Web.UI.Triplet("Ie3", string.Empty, 4); dictionary["IE2"] = new System.Web.UI.Triplet("Ie", string.Empty, 3); dictionary["WebTV"] = new System.Web.UI.Triplet("Ie2", string.Empty, 4); dictionary["WebTV2"] = new System.Web.UI.Triplet("Webtv", string.Empty, 5); dictionary["IE1minor5"] = new System.Web.UI.Triplet("Ie", string.Empty, 3); dictionary["PowerBrowser"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["Gecko"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["MozillaRV"] = new System.Web.UI.Triplet("Gecko", string.Empty, 3); dictionary["MozillaFirebird"] = new System.Web.UI.Triplet("Mozillarv", string.Empty, 4); dictionary["MozillaFirefox"] = new System.Web.UI.Triplet("Mozillarv", string.Empty, 4); dictionary["Safari"] = new System.Web.UI.Triplet("Gecko", string.Empty, 3); dictionary["Safari60"] = new System.Web.UI.Triplet("Safari", string.Empty, 4); dictionary["Safari85"] = new System.Web.UI.Triplet("Safari", string.Empty, 4); dictionary["Safari1Plus"] = new System.Web.UI.Triplet("Safari", string.Empty, 4); dictionary["Netscape5"] = new System.Web.UI.Triplet("Gecko", string.Empty, 3); dictionary["Netscape6to9"] = new System.Web.UI.Triplet("Netscape5", string.Empty, 4); dictionary["Netscape6to9Beta"] = new System.Web.UI.Triplet("Netscape6to9", string.Empty, 5); dictionary["NetscapeBeta"] = new System.Web.UI.Triplet("Netscape5", string.Empty, 4); dictionary["AvantGo"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["TMobileSidekick"] = new System.Web.UI.Triplet("Avantgo", string.Empty, 3); dictionary["GoAmerica"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["GoAmericaWinCE"] = new System.Web.UI.Triplet("Goamerica", string.Empty, 3); dictionary["GoAmericaPalm"] = new System.Web.UI.Triplet("Goamerica", string.Empty, 3); dictionary["GoAmericaRIM"] = new System.Web.UI.Triplet("Goamerica", string.Empty, 3); dictionary["GoAmericaRIM950"] = new System.Web.UI.Triplet("Goamericarim", string.Empty, 4); dictionary["GoAmericaRIM850"] = new System.Web.UI.Triplet("Goamericarim", string.Empty, 4); dictionary["GoAmericaRIM957"] = new System.Web.UI.Triplet("Goamericarim", string.Empty, 4); dictionary["GoAmericaRIM957major6minor2"] = new System.Web.UI.Triplet("Goamericarim957", string.Empty, 5); dictionary["GoAmericaRIM857"] = new System.Web.UI.Triplet("Goamericarim", string.Empty, 4); dictionary["GoAmericaRIM857major6"] = new System.Web.UI.Triplet("Goamericarim857", string.Empty, 5); dictionary["GoAmericaRIM857major6minor2to9"] = new System.Web.UI.Triplet("Goamericarim857major6", string.Empty, 6); dictionary["GoAmerica7to9"] = new System.Web.UI.Triplet("Goamericarim857", string.Empty, 5); dictionary["Netscape3"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["Netscape4"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["Casiopeia"] = new System.Web.UI.Triplet("Netscape4", string.Empty, 3); dictionary["PalmWebPro"] = new System.Web.UI.Triplet("Netscape4", string.Empty, 3); dictionary["PalmWebPro3"] = new System.Web.UI.Triplet("Palmwebpro", string.Empty, 4); dictionary["NetFront"] = new System.Web.UI.Triplet("Netscape4", string.Empty, 3); dictionary["SLB500"] = new System.Web.UI.Triplet("Netfront", string.Empty, 4); dictionary["VRNA"] = new System.Web.UI.Triplet("Netfront", string.Empty, 4); dictionary["Mypalm"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["MyPalm1"] = new System.Web.UI.Triplet("Mypalm", string.Empty, 3); dictionary["Eudoraweb"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["PdQbrowser"] = new System.Web.UI.Triplet("Eudoraweb", string.Empty, 3); dictionary["Eudoraweb21Plus"] = new System.Web.UI.Triplet("Eudoraweb", string.Empty, 3); dictionary["WinCE"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["PIE"] = new System.Web.UI.Triplet("Wince", string.Empty, 3); dictionary["PIEPPC"] = new System.Web.UI.Triplet("Pie", string.Empty, 4); dictionary["PIEnoDeviceID"] = new System.Web.UI.Triplet("Pie", string.Empty, 4); dictionary["PIESmartphone"] = new System.Web.UI.Triplet("Pie", string.Empty, 4); dictionary["PIE4"] = new System.Web.UI.Triplet("Wince", string.Empty, 3); dictionary["PIE4PPC"] = new System.Web.UI.Triplet("Pie4", string.Empty, 4); dictionary["PIE5Plus"] = new System.Web.UI.Triplet("Wince", string.Empty, 3); dictionary["sigmarion3"] = new System.Web.UI.Triplet("Pie5plus", string.Empty, 4); dictionary["MSPIE"] = new System.Web.UI.Triplet("Mozilla", string.Empty, 2); dictionary["MSPIE2"] = new System.Web.UI.Triplet("Mspie", string.Empty, 3); dictionary["Docomo"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["DocomoSH251i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoSH251iS"] = new System.Web.UI.Triplet("Docomosh251i", string.Empty, 3); dictionary["DocomoN251i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN251iS"] = new System.Web.UI.Triplet("Docomon251i", string.Empty, 3); dictionary["DocomoP211i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF212i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoD501i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF501i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN501i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP501i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoD502i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF502i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN502i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP502i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoNm502i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoSo502i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF502it"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN502it"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoSo502iwm"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF504i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN504i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP504i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN821i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP821i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoD209i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoEr209i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF209i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoKo209i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN209i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP209i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP209is"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoR209i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoR691i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF503i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF503is"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoD503i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoD503is"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoD210i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF210i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN210i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN2001"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoD211i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN211i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP210i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoKo210i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP2101v"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP2102v"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF211i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoF671i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN503is"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN503i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoSo503i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP503is"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP503i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoSo210i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoSo503is"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoSh821i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN2002"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoSo505i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoP505i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoN505i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoD505i"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["DocomoISIM60"] = new System.Web.UI.Triplet("Docomo", string.Empty, 2); dictionary["EricssonR380"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["Ericsson"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["EricssonR320"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonT20"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonT65"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonT68"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["Ericsson301A"] = new System.Web.UI.Triplet("Ericssont68", string.Empty, 3); dictionary["EricssonT68R1A"] = new System.Web.UI.Triplet("Ericssont68", string.Empty, 3); dictionary["EricssonT68R101"] = new System.Web.UI.Triplet("Ericssont68", string.Empty, 3); dictionary["EricssonT68R201A"] = new System.Web.UI.Triplet("Ericssont68", string.Empty, 3); dictionary["EricssonT300"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonP800"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonP800R101"] = new System.Web.UI.Triplet("Ericssonp800", string.Empty, 3); dictionary["EricssonT61"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonT31"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonR520"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonA2628"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EricssonT39"] = new System.Web.UI.Triplet("Ericsson", string.Empty, 2); dictionary["EzWAP"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["GenericDownlevel"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["Jataayu"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["JataayuPPC"] = new System.Web.UI.Triplet("Jataayu", string.Empty, 2); dictionary["Jphone"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["JphoneMitsubishi"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphoneDenso"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphoneKenwood"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphoneNec"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphoneNecN51"] = new System.Web.UI.Triplet("Jphonenec", string.Empty, 3); dictionary["JphonePanasonic"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphonePioneer"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphoneSanyo"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphoneSA51"] = new System.Web.UI.Triplet("Jphonesanyo", string.Empty, 3); dictionary["JphoneSharp"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphoneSharpSh53"] = new System.Web.UI.Triplet("Jphonesharp", string.Empty, 3); dictionary["JphoneSharpSh07"] = new System.Web.UI.Triplet("Jphonesharp", string.Empty, 3); dictionary["JphoneSharpSh08"] = new System.Web.UI.Triplet("Jphonesharp", string.Empty, 3); dictionary["JphoneSharpSh51"] = new System.Web.UI.Triplet("Jphonesharp", string.Empty, 3); dictionary["JphoneSharpSh52"] = new System.Web.UI.Triplet("Jphonesharp", string.Empty, 3); dictionary["JphoneToshiba"] = new System.Web.UI.Triplet("Jphone", string.Empty, 2); dictionary["JphoneToshibaT06a"] = new System.Web.UI.Triplet("Jphonetoshiba", string.Empty, 3); dictionary["JphoneToshibaT08"] = new System.Web.UI.Triplet("Jphonetoshiba", string.Empty, 3); dictionary["JphoneToshibaT51"] = new System.Web.UI.Triplet("Jphonetoshiba", string.Empty, 3); dictionary["Legend"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["LGG5200"] = new System.Web.UI.Triplet("Legend", string.Empty, 2); dictionary["MME"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["MMEF20"] = new System.Web.UI.Triplet("Mme", string.Empty, 2); dictionary["MMECellphone"] = new System.Web.UI.Triplet("Mmef20", string.Empty, 3); dictionary["MMEBenefonQ"] = new System.Web.UI.Triplet("Mmecellphone", string.Empty, 4); dictionary["MMESonyCMDZ5"] = new System.Web.UI.Triplet("Mmecellphone", string.Empty, 4); dictionary["MMESonyCMDZ5Pj020e"] = new System.Web.UI.Triplet("Mmesonycmdz5", string.Empty, 5); dictionary["MMESonyCMDJ5"] = new System.Web.UI.Triplet("Mmecellphone", string.Empty, 4); dictionary["MMESonyCMDJ7"] = new System.Web.UI.Triplet("Mmecellphone", string.Empty, 4); dictionary["MMEGenericSmall"] = new System.Web.UI.Triplet("Mmecellphone", string.Empty, 4); dictionary["MMEGenericLarge"] = new System.Web.UI.Triplet("Mmecellphone", string.Empty, 4); dictionary["MMEGenericFlip"] = new System.Web.UI.Triplet("Mmecellphone", string.Empty, 4); dictionary["MMEGeneric3D"] = new System.Web.UI.Triplet("Mmecellphone", string.Empty, 4); dictionary["MMEMobileExplorer"] = new System.Web.UI.Triplet("Mme", string.Empty, 2); dictionary["Nokia"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["NokiaBlueprint"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["NokiaWapSimulator"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["NokiaMobileBrowser"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia7110"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia6220"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia6250"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia6310"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia6510"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia8310"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia9110i"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia9110"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia3330"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia9210"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia9210HTML"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia3590"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia3590V1"] = new System.Web.UI.Triplet("Nokia3590", string.Empty, 3); dictionary["Nokia3595"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia3560"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia3650"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia3650P12Plus"] = new System.Web.UI.Triplet("Nokia3650", string.Empty, 3); dictionary["Nokia5100"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia6200"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia6590"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia6800"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["Nokia7650"] = new System.Web.UI.Triplet("Nokia", string.Empty, 2); dictionary["NokiaMobileBrowserRainbow"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["NokiaEpoc32wtl"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["NokiaEpoc32wtl20"] = new System.Web.UI.Triplet("Nokiaepoc32wtl", string.Empty, 2); dictionary["Up"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["AuMic"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["AuMicV2"] = new System.Web.UI.Triplet("Aumic", string.Empty, 3); dictionary["a500"] = new System.Web.UI.Triplet("Aumic", string.Empty, 3); dictionary["n400"] = new System.Web.UI.Triplet("Aumic", string.Empty, 3); dictionary["AlcatelBe4"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["AlcatelBe5"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["AlcatelBe5v2"] = new System.Web.UI.Triplet("Alcatelbe5", string.Empty, 3); dictionary["AlcatelBe3"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["AlcatelBf3"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["AlcatelBf4"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotCb"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotF5"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotD8"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotCf"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotF6"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotBc"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotDc"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotPanC"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotC4"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mcca"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mot2000"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotP2kC"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotAf"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotAf418"] = new System.Web.UI.Triplet("Motaf", string.Empty, 3); dictionary["MotC2"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Xenium"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sagem959"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SghA300"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SghN100"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C304sa"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sy11"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["St12"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sy14"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SieS40"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SieSl45"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SieS35"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SieMe45"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SieS45"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Gm832"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Gm910i"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mot32"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mot28"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["D2"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["PPat"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Alaz"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Cdm9100"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Cdm135"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Cdm9000"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C303ca"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C311ca"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C202de"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C409ca"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C402de"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ds15"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tp2200"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tp120"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ds10"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["R280"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C201h"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["S71"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C302h"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C309h"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C407h"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["C451h"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["R201"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["P21"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Kyocera702g"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Kyocera703g"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["KyoceraC307k"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tk01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tk02"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tk03"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tk04"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tk05"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["D303k"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["D304k"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Qcp2035"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Qcp3035"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["D512"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Dm110"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tm510"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Lg13"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["P100"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Lgc875f"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Lgp680f"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Lgp7800f"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Lgc840f"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Lgi2100"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Lgp7300f"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sd500"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tp1100"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tp3000"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["T250"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mo01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mo02"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mc01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mccc"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mcc9"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Nk00"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mai12"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ma112"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ma13"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mac1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mat1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sc01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sc03"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sc02"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sc04"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sg08"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sc13"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sc11"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sec01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sc10"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sy12"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["St11"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sy13"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Syc1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sy01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Syt1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sty2"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sy02"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sy03"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Si01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sni1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sn11"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sn12"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sn134"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sn156"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Snc1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tsc1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tsi1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ts11"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ts12"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ts13"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tst1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tst2"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Tst3"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ig01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ig02"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Ig03"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Qc31"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Qc12"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Qc32"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sp01"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sh"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Upg1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Opwv1"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Alav"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Im1k"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Nt95"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mot2001"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Motv200"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mot72"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Mot76"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Scp6000"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotD5"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotF0"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SghA400"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sec03"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SieC3i"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sn17"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Scp4700"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sec02"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Sy15"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Db520"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["L430V03J02"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["OPWVSDK"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["OPWVSDK6"] = new System.Web.UI.Triplet("Opwvsdk", string.Empty, 3); dictionary["OPWVSDK6Plus"] = new System.Web.UI.Triplet("Opwvsdk", string.Empty, 3); dictionary["KDDICA21"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["KDDITS21"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["KDDISA21"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["KM100"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["LGELX5350"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["HitachiP300"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SIES46"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotorolaV60G"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotorolaV708"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotorolaV708A"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["MotorolaE360"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SonyericssonA1101S"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["PhilipsFisio820"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["CasioA5302"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["TCLL668"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["KDDITS24"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SIES55"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["SHARPGx10"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["BenQAthena"] = new System.Web.UI.Triplet("Up", string.Empty, 2); dictionary["Opera"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["Opera1to3beta"] = new System.Web.UI.Triplet("Opera", string.Empty, 2); dictionary["Opera4"] = new System.Web.UI.Triplet("Opera", string.Empty, 2); dictionary["Opera4beta"] = new System.Web.UI.Triplet("Opera4", string.Empty, 3); dictionary["Opera5to9"] = new System.Web.UI.Triplet("Opera", string.Empty, 2); dictionary["Opera6to9"] = new System.Web.UI.Triplet("Opera5to9", string.Empty, 3); dictionary["Opera7to9"] = new System.Web.UI.Triplet("Opera6to9", string.Empty, 4); dictionary["Opera8to9"] = new System.Web.UI.Triplet("Opera7to9", string.Empty, 5); dictionary["OperaPsion"] = new System.Web.UI.Triplet("Opera", string.Empty, 2); dictionary["Palmscape"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["PalmscapeVersion"] = new System.Web.UI.Triplet("Palmscape", string.Empty, 2); dictionary["AusPalm"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["SharpPda"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["ZaurusMiE1"] = new System.Web.UI.Triplet("Sharppda", string.Empty, 2); dictionary["ZaurusMiE21"] = new System.Web.UI.Triplet("Sharppda", string.Empty, 2); dictionary["ZaurusMiE25"] = new System.Web.UI.Triplet("Sharppda", string.Empty, 2); dictionary["Panasonic"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["PanasonicGAD95"] = new System.Web.UI.Triplet("Panasonic", string.Empty, 2); dictionary["PanasonicGAD87"] = new System.Web.UI.Triplet("Panasonic", string.Empty, 2); dictionary["PanasonicGAD87A39"] = new System.Web.UI.Triplet("Panasonicgad87", string.Empty, 3); dictionary["PanasonicGAD87A38"] = new System.Web.UI.Triplet("Panasonicgad87", string.Empty, 3); dictionary["MSPIE06"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["SKTDevices"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["SKTDevicesHyundai"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["PSE200"] = new System.Web.UI.Triplet("Sktdeviceshyundai", string.Empty, 3); dictionary["SKTDevicesHanhwa"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["SKTDevicesJTEL"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["JTEL01"] = new System.Web.UI.Triplet("Sktdevicesjtel", string.Empty, 3); dictionary["JTELNate"] = new System.Web.UI.Triplet("Jtel01", string.Empty, 4); dictionary["SKTDevicesLG"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["SKTDevicesMotorola"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["SKTDevicesV730"] = new System.Web.UI.Triplet("Sktdevicesmotorola", string.Empty, 3); dictionary["SKTDevicesNokia"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["SKTDevicesSKTT"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["SKTDevicesSamSung"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["SCHE150"] = new System.Web.UI.Triplet("Sktdevicessamsung", string.Empty, 3); dictionary["SKTDevicesEricsson"] = new System.Web.UI.Triplet("Sktdevices", string.Empty, 2); dictionary["WinWap"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["Xiino"] = new System.Web.UI.Triplet("Default", string.Empty, 1); dictionary["XiinoV2"] = new System.Web.UI.Triplet("Xiino", string.Empty, 2); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
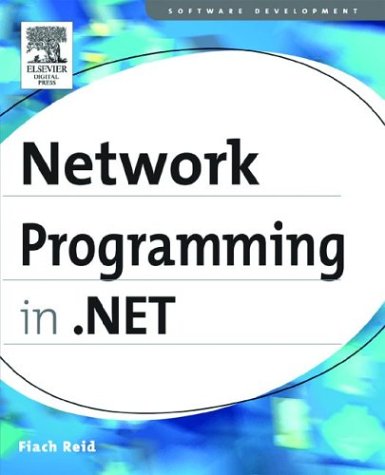
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CalendarBlackoutDatesCollection.cs
- ConfigurationSectionCollection.cs
- RuleElement.cs
- BufferedStream.cs
- Contracts.cs
- FreezableCollection.cs
- TransactionValidationBehavior.cs
- PolicyLevel.cs
- ReflectTypeDescriptionProvider.cs
- GeometryDrawing.cs
- RtfFormatStack.cs
- ContentWrapperAttribute.cs
- PageContentAsyncResult.cs
- CollectionConverter.cs
- BaseCodeDomTreeGenerator.cs
- WinInet.cs
- TreeNodeStyleCollection.cs
- AttachedPropertyBrowsableAttribute.cs
- ImageBrush.cs
- UriGenerator.cs
- BitmapDecoder.cs
- TextTabProperties.cs
- ZipIOLocalFileDataDescriptor.cs
- RelatedEnd.cs
- QilStrConcatenator.cs
- WebPartEditorApplyVerb.cs
- WebPartDisplayModeCancelEventArgs.cs
- XmlnsCompatibleWithAttribute.cs
- HScrollProperties.cs
- _ProxyRegBlob.cs
- COM2TypeInfoProcessor.cs
- SocketPermission.cs
- UnlockInstanceCommand.cs
- OdbcConnection.cs
- FrameworkRichTextComposition.cs
- HtmlListAdapter.cs
- NodeLabelEditEvent.cs
- DataIdProcessor.cs
- PaginationProgressEventArgs.cs
- ArgumentOutOfRangeException.cs
- TailPinnedEventArgs.cs
- WebConfigurationHost.cs
- WebSysDisplayNameAttribute.cs
- Classification.cs
- WasEndpointConfigContainer.cs
- ButtonBaseDesigner.cs
- OuterGlowBitmapEffect.cs
- GenericParameterDataContract.cs
- PingReply.cs
- HttpListenerElement.cs
- CodeMemberProperty.cs
- PropertyEmitter.cs
- MonitorWrapper.cs
- MarkupProperty.cs
- DataGridViewColumnTypeEditor.cs
- MultipleViewProviderWrapper.cs
- __Filters.cs
- StateManagedCollection.cs
- ProfilePropertySettings.cs
- BufferedStream.cs
- CodeDomLocalizationProvider.cs
- AudioSignalProblemOccurredEventArgs.cs
- SchemaLookupTable.cs
- GlobalizationSection.cs
- ProviderException.cs
- CroppedBitmap.cs
- XmlNodeReader.cs
- NativeMethods.cs
- Route.cs
- RelationshipEndCollection.cs
- ConnectionStringSettings.cs
- ActivityBuilder.cs
- ModifiableIteratorCollection.cs
- ButtonRenderer.cs
- SystemIdentity.cs
- CopyNamespacesAction.cs
- StandardMenuStripVerb.cs
- SessionParameter.cs
- DataGridLinkButton.cs
- __FastResourceComparer.cs
- InteropAutomationProvider.cs
- ReflectionHelper.cs
- designeractionlistschangedeventargs.cs
- PropertyPath.cs
- TrackingMemoryStreamFactory.cs
- OdbcCommand.cs
- EventDrivenDesigner.cs
- EmbeddedMailObject.cs
- FontInfo.cs
- ImageCodecInfo.cs
- Rule.cs
- MethodAccessException.cs
- TreeNodeMouseHoverEvent.cs
- DesignSurfaceEvent.cs
- RelationshipEnd.cs
- DefaultAssemblyResolver.cs
- Script.cs
- PasswordDeriveBytes.cs
- CodeArrayIndexerExpression.cs
- URL.cs