Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / MediaContextNotificationWindow.cs / 1 / MediaContextNotificationWindow.cs
//------------------------------------------------------------------------------ // //// Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: // A wrapper for a top-level hidden window that is used to process // messages broadcasted to top-level windows only (such as DWM's // WM_DWMCOMPOSITIONCHANGED). If the WPF application doesn't have // a top-level window (as it is the case for XBAP applications), // such messages would have been ignored. // //----------------------------------------------------------------------------- using System; using System.Windows.Threading; using System.Collections; using System.Diagnostics; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using Microsoft.Win32; using Microsoft.Internal; using MS.Internal; using MS.Win32; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods.MilCoreApi; using SafeNativeMethods=MS.Win32.PresentationCore.SafeNativeMethods; namespace System.Windows.Media { ////// The MediaContextNotificationWindow structure provides its owner /// MediaContext with the ability to receive and forward window /// messages broadcasted to top-level windows. /// internal struct MediaContextNotificationWindow { //+--------------------------------------------------------------------- // // Internal Methods // //--------------------------------------------------------------------- #region Internal Methods ////// Sets the owner MediaContext and creates the notification window. /// ////// Critical - Creates an HwndWrapper and adds a hook. /// TreatAsSafe: The _hwndNotification window is critical and this function is safe to call /// [SecurityCritical, SecurityTreatAsSafe] internal void CreateNotificationWindow(MediaContext ownerMediaContext) { // Remember the pointer to the owner MediaContext that we'll forward the broadcasts to. _ownerMediaContext = ownerMediaContext; // Create a top-level, invisible window so we can get the WM_DWMCOMPOSITIONCHANGED // and other DWM notifications that are broadcasted to top-level windows only. HwndWrapper hwndNotification; hwndNotification = new HwndWrapper(0, NativeMethods.WS_POPUP, 0, 0, 0, 0, 0, "MediaContextNotificationWindow", IntPtr.Zero, null); _hwndNotificationHook = new HwndWrapperHook(MessageFilter); _hwndNotification = new SecurityCriticalDataClass(hwndNotification); _hwndNotification.Value.AddHook(_hwndNotificationHook); } /// /// Critical - Calls dispose on the critical hwnd wrapper. /// TreatAsSafe: It is safe to dispose the wrapper /// [SecurityCritical, SecurityTreatAsSafe] internal void DisposeNotificationWindow() { if (_hwndNotification != null) _hwndNotification.Value.Dispose(); _hwndNotificationHook = null; _hwndNotification = null; _ownerMediaContext = null; } #endregion Internal Methods //+---------------------------------------------------------------------- // // Private Methods // //--------------------------------------------------------------------- #region Private Methods ////// If any of the interesting broadcast messages is seen, forward them to the owner MediaContext. /// private IntPtr MessageFilter(IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam, ref bool handled) { Debug.Assert(_ownerMediaContext != null); if (msg == NativeMethods.WM_DWMCOMPOSITIONCHANGED) { _ownerMediaContext.OnDWMCompositionChanged(); } return IntPtr.Zero; } #endregion Private Methods //+---------------------------------------------------------------------- // // Private Fields // //---------------------------------------------------------------------- #region Private Fields // The owner MediaContext private MediaContext _ownerMediaContext; // A top-level hidden window. private SecurityCriticalDataClass_hwndNotification; // The message filter hook for the top-level hidden window. private HwndWrapperHook _hwndNotificationHook; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: // A wrapper for a top-level hidden window that is used to process // messages broadcasted to top-level windows only (such as DWM's // WM_DWMCOMPOSITIONCHANGED). If the WPF application doesn't have // a top-level window (as it is the case for XBAP applications), // such messages would have been ignored. // //----------------------------------------------------------------------------- using System; using System.Windows.Threading; using System.Collections; using System.Diagnostics; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using Microsoft.Win32; using Microsoft.Internal; using MS.Internal; using MS.Win32; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods.MilCoreApi; using SafeNativeMethods=MS.Win32.PresentationCore.SafeNativeMethods; namespace System.Windows.Media { ////// The MediaContextNotificationWindow structure provides its owner /// MediaContext with the ability to receive and forward window /// messages broadcasted to top-level windows. /// internal struct MediaContextNotificationWindow { //+--------------------------------------------------------------------- // // Internal Methods // //--------------------------------------------------------------------- #region Internal Methods ////// Sets the owner MediaContext and creates the notification window. /// ////// Critical - Creates an HwndWrapper and adds a hook. /// TreatAsSafe: The _hwndNotification window is critical and this function is safe to call /// [SecurityCritical, SecurityTreatAsSafe] internal void CreateNotificationWindow(MediaContext ownerMediaContext) { // Remember the pointer to the owner MediaContext that we'll forward the broadcasts to. _ownerMediaContext = ownerMediaContext; // Create a top-level, invisible window so we can get the WM_DWMCOMPOSITIONCHANGED // and other DWM notifications that are broadcasted to top-level windows only. HwndWrapper hwndNotification; hwndNotification = new HwndWrapper(0, NativeMethods.WS_POPUP, 0, 0, 0, 0, 0, "MediaContextNotificationWindow", IntPtr.Zero, null); _hwndNotificationHook = new HwndWrapperHook(MessageFilter); _hwndNotification = new SecurityCriticalDataClass(hwndNotification); _hwndNotification.Value.AddHook(_hwndNotificationHook); } /// /// Critical - Calls dispose on the critical hwnd wrapper. /// TreatAsSafe: It is safe to dispose the wrapper /// [SecurityCritical, SecurityTreatAsSafe] internal void DisposeNotificationWindow() { if (_hwndNotification != null) _hwndNotification.Value.Dispose(); _hwndNotificationHook = null; _hwndNotification = null; _ownerMediaContext = null; } #endregion Internal Methods //+---------------------------------------------------------------------- // // Private Methods // //--------------------------------------------------------------------- #region Private Methods ////// If any of the interesting broadcast messages is seen, forward them to the owner MediaContext. /// private IntPtr MessageFilter(IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam, ref bool handled) { Debug.Assert(_ownerMediaContext != null); if (msg == NativeMethods.WM_DWMCOMPOSITIONCHANGED) { _ownerMediaContext.OnDWMCompositionChanged(); } return IntPtr.Zero; } #endregion Private Methods //+---------------------------------------------------------------------- // // Private Fields // //---------------------------------------------------------------------- #region Private Fields // The owner MediaContext private MediaContext _ownerMediaContext; // A top-level hidden window. private SecurityCriticalDataClass_hwndNotification; // The message filter hook for the top-level hidden window. private HwndWrapperHook _hwndNotificationHook; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
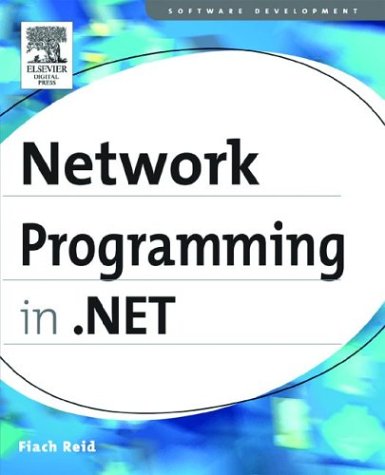
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeDependencyAttribute.cs
- Exceptions.cs
- XmlSchemaProviderAttribute.cs
- DataPointer.cs
- MethodImplAttribute.cs
- PropertySet.cs
- EventProxy.cs
- CodeAccessPermission.cs
- EventItfInfo.cs
- WebBrowserUriTypeConverter.cs
- StringCollectionMarkupSerializer.cs
- SerializableAuthorizationContext.cs
- CodeExpressionStatement.cs
- FastEncoderWindow.cs
- CapabilitiesState.cs
- PlanCompiler.cs
- WebConfigurationHost.cs
- OleDbStruct.cs
- TypeExtensionConverter.cs
- DateTimeConstantAttribute.cs
- VideoDrawing.cs
- GeometryHitTestParameters.cs
- MarkupExtensionParser.cs
- _LazyAsyncResult.cs
- CodeDomSerializerException.cs
- _NetworkingPerfCounters.cs
- DropSource.cs
- HttpContext.cs
- MULTI_QI.cs
- AttachmentCollection.cs
- XmlDigitalSignatureProcessor.cs
- xmlglyphRunInfo.cs
- CompressionTransform.cs
- Variable.cs
- DebugHandleTracker.cs
- XmlReader.cs
- GeneralTransform2DTo3DTo2D.cs
- BufferedWebEventProvider.cs
- RectangleHotSpot.cs
- WebCodeGenerator.cs
- DomainUpDown.cs
- HttpProfileBase.cs
- DesignerSerializerAttribute.cs
- CrossContextChannel.cs
- TabItemAutomationPeer.cs
- ComAdminWrapper.cs
- InkCanvasFeedbackAdorner.cs
- MD5.cs
- MembershipValidatePasswordEventArgs.cs
- PersistenceMetadataNamespace.cs
- DataGridViewCellValidatingEventArgs.cs
- PropertyHelper.cs
- EventLogEntry.cs
- XmlValueConverter.cs
- Privilege.cs
- InvokeCompletedEventArgs.cs
- LinkedResource.cs
- XmlEncodedRawTextWriter.cs
- FixedLineResult.cs
- MdiWindowListStrip.cs
- TransformerConfigurationWizardBase.cs
- TextLine.cs
- DrawingCollection.cs
- StringSource.cs
- NativeActivityMetadata.cs
- MD5CryptoServiceProvider.cs
- ProxyFragment.cs
- WinEventTracker.cs
- NativeMethods.cs
- SqlRecordBuffer.cs
- CompilationUtil.cs
- WriteStateInfoBase.cs
- Control.cs
- PartialList.cs
- TypeUtil.cs
- Bezier.cs
- DBConnectionString.cs
- OutputCacheSettingsSection.cs
- ResumeStoryboard.cs
- ContentIterators.cs
- BoundingRectTracker.cs
- WebPartConnectionsDisconnectVerb.cs
- WebPartManager.cs
- SqlAliaser.cs
- AdPostCacheSubstitution.cs
- ConfigurationSectionHelper.cs
- __Error.cs
- And.cs
- TypeSemantics.cs
- CapiSafeHandles.cs
- MsmqInputSessionChannelListener.cs
- ProbeMatchesMessageCD1.cs
- OdbcParameter.cs
- SqlReferenceCollection.cs
- ArcSegment.cs
- Rectangle.cs
- CancelRequestedQuery.cs
- SaveFileDialog.cs
- XmlSchemaSimpleTypeList.cs
- FlowLayout.cs